Displaying a countdown timer graphically with a UI Slider
There are many cases where we wish to inform the player of how much time is left in a game or how much longer an element will take to download – for example, a loading progress bar, the time or health remaining compared to the starting maximum, or how much the player has filled up their water bottle from the fountain of youth.
In this recipe, we’ll illustrate how to remove the interactive “handle” of a UI Slider, and then change the size and color of its components to provide us with an easy-to-use, general-purpose progress/proportion bar:
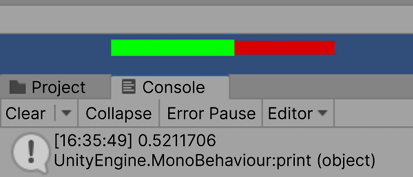
Figure 2.20: Example of a countdown timer with a UI Slider
In this recipe, we’ll use our modified UI Slider to graphically present to the user how much time remains on a countdown timer.
Getting ready
For this recipe, we have prepared the script and images that you need in the 02_04
folder, respectively named _Scripts
and Images
.
How to do it...
To create a digital countdown timer with a graphical display, follow these steps:
- Create a new Unity 2D project and install TextMeshPro by choosing: Window | TextMeshPro | Import TMP Essential Resources.
- Import the
CountdownTimer
script and thered_square
andgreen_square
images into this project. - Add a
UI Text(TMP)
GameObject to the scene with a Font size of30
and placeholder text such as aUI Slider
value
(this text will be replaced with the slider value when the scene starts). Check that Overflow is set to Overflow. - In the Hierarchy window, add a
Slider
GameObject to the scene by going to GameObject | UI | Slider. - In the Inspector window, modify the settings for the position of the
Slider
GameObject’s Rect Transform to the top-center part of the screen. - Ensure that the
Slider
GameObject is selected in the Hierarchy window. - Deactivate the
Handle
Slide
Area
child GameObject (by unchecking it). - You’ll see the “drag circle” disappear in the Game window (the user will not be dragging the slider since we want this slider to be display-only):
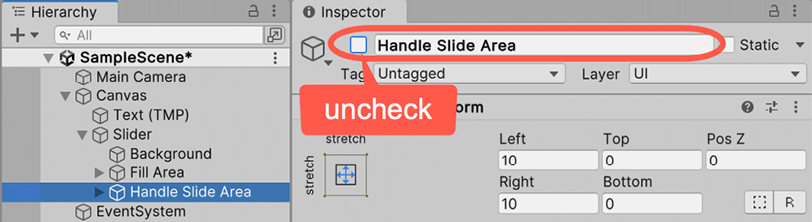
Figure 2.21: Ensuring Handle Slide Area is deactivated
- Select the Background child and do the following:
- Drag the
red_square
image into the Source Image property of the Image component in the Inspector window.
- Drag the
- Select the
Fill
child of theFill Area
child and do the following:- Drag the
green_square
image into the Source Image property of the Image component in the Inspector window.
- Drag the
- Select the
Fill
Area
child and do the following:- In the Rect Transform component, use the Anchors preset position of left-middle.
- Set Width to 155 and Height to 12:
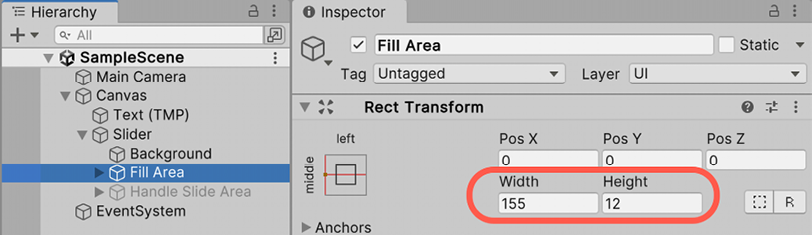
Figure 2.22: Selections in the Rect Transform component
- Create a C# script class called
SliderTimerDisplay
that contains the following code and add an instance as a scripted component to theSlider
GameObject:using UnityEngine; using UnityEngine.UI; using TMPro; [RequireComponent(typeof(CountdownTimer))] public class SliderTimerDisplay : MonoBehaviour { private CountdownTimer countdownTimer; private Slider sliderUI; void Awake() { countdownTimer = GetComponent<CountdownTimer>(); sliderUI = GetComponent<Slider>(); } void Start() { SetupSlider(); countdownTimer.ResetTimer( 30 ); } void Update () { sliderUI.value = countdownTimer.GetProportionTimeRemaining(); print (countdownTimer.GetProportionTimeRemaining()); } private void SetupSlider () { sliderUI.minValue = 0; sliderUI.maxValue = 1; sliderUI.wholeNumbers = false; } }
Run your game. You will see the slider move with each second, revealing more and more of the red background to indicate the time remaining.
How it works...
In this recipe, you hid the Handle Slide Area child so that the UI Slider is for display only, which means it cannot be interacted with by the user. The Background color of the UI Slider was set to red so that, as the counter goes down, more and more red is revealed, warning the user that the time is running out.
The Fill property of the UI Slider was set to green so that the proportion remaining is displayed in green – the more green that’s displayed, the greater the value of the slider/timer.
An instance of the provided CountdownTimer
script class was automatically added as a component to the UI Slider via [RequireComponent(...)]
.
The Awake()
method caches references to the CountdownTimer and Slider components in the countdownTimer
and sliderUI variables
.
The Start()
method calls the SetupSlider()
method and then resets the countdown timer so that it starts counting down from 30 seconds.
The SetupSlider()
method sets up this slider for float (decimal) values between 0.0
and 1.0
.
In each frame, the Update()
method sets the slider value to the float that’s returned by calling the GetProportionRemaining()
method from the running timer. At runtime, Unity adjusts the proportion of red/green that’s displayed in the UI Slider so that it matches the slider’s value.