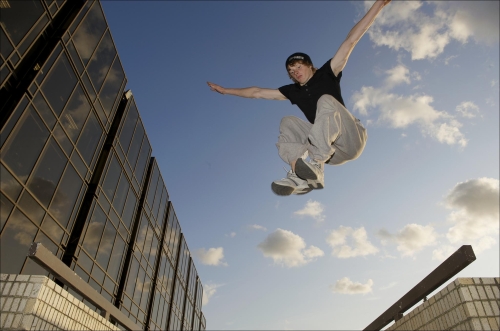
Parkour jumper: THOR/Parkour Foundations/Flickr, Creative Commons (source https://www.flickr.com/photos/geishaboy500/2911897958/in/album-72157607724308547/)
In the previous chapters, the first-person character was mostly limited to the X-Z ground plane. This chapter will focus more on the y-axis, as we explore adding physics to the virtual experience. You will see how properties and materials based on physics can be added to objects, as well as how one can transfer physical forces between objects using the C# scripting.
In this chapter, you will learn the following topics:
The Unity physics engine, the Unity Rigidbody component, and physic materials
Transferring physical forces from one object to another with scripting
Implementing velocity and gravity on first-person characters
Interacting with the environment, including headshots and jump gestures
Building models in Blender
Note that the projects in this chapter are separate and are not directly required...