In this chapter, we're going to build a Windows Phone application and an Azure mobile service for the sales business unit that will allow customers to view orders they've placed and receive notifications when the order status changes and when new products are added to the system. In the supply business unit, we'll create a Windows Store application and Azure mobile service for the warehouse staff to use on a tablet device in order to view which orders are waiting for dispatch while they are working without having to return to a central terminal. We'll be implementing the notification hub (from the Service Bus family of services) into the sales system so that when the order status changes and new products are created, customers will receive toast and tile push notifications to alert them.
Once we've finished, the sales system will have an architecture like this:
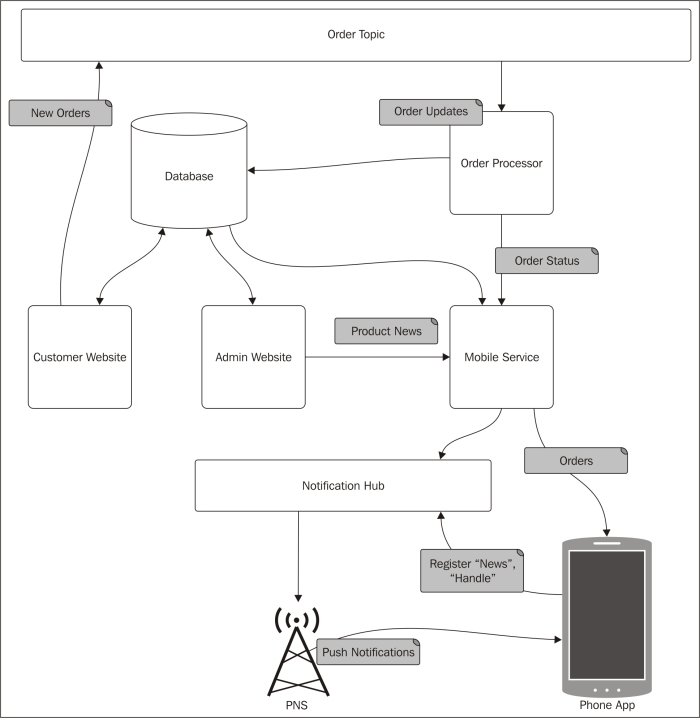
I also add another Order Processor Cloud service to the supply...