Often, we will want to activate one activity from within another activity. Although this is not a difficult task, it will require a little more setting up to be done than the previous recipes as it requires two activities. We will create two activity classes and declare them both in the manifest. We'll also create a button, as we did in the previous recipe, to switch to the activity.
We'll create a new project in Android Studio, just as we did in the previous recipes, and call this one ActivitySwitcher
. Android Studio will create the first activity, ActivityMain
, and automatically declare it in the manifest.
- Since the Android Studio New Project wizard has already created the first activity, we just need to create the second activity. Open the
ActivitySwitcher
project and navigate toFile
|New
|Activity
|Empty
Activity
, as shown in this screenshot:
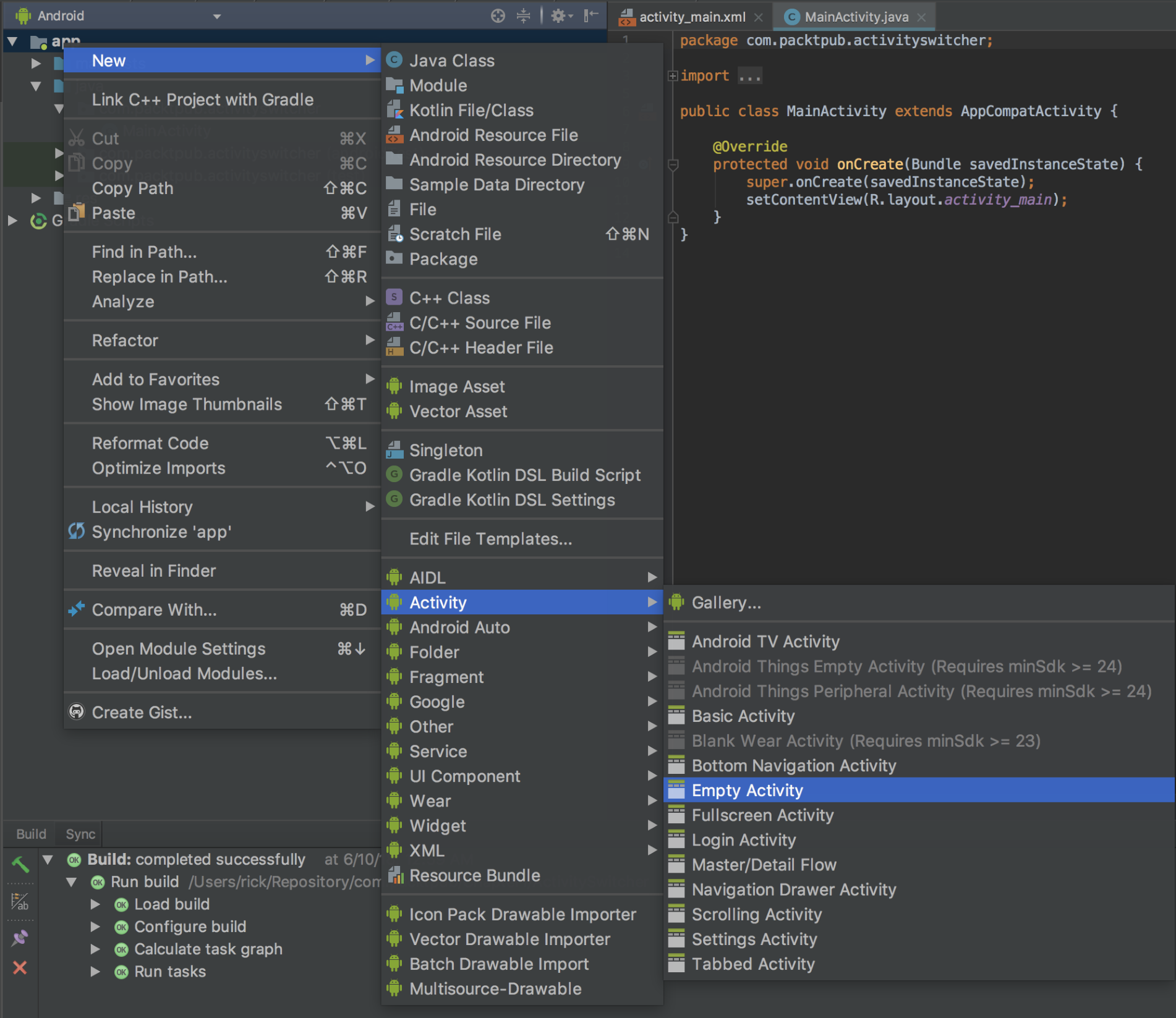
- In the
New Android Activity
dialog, you can leave the defaultActivity Name
as is, or change it toSecondActivity
, as follows:
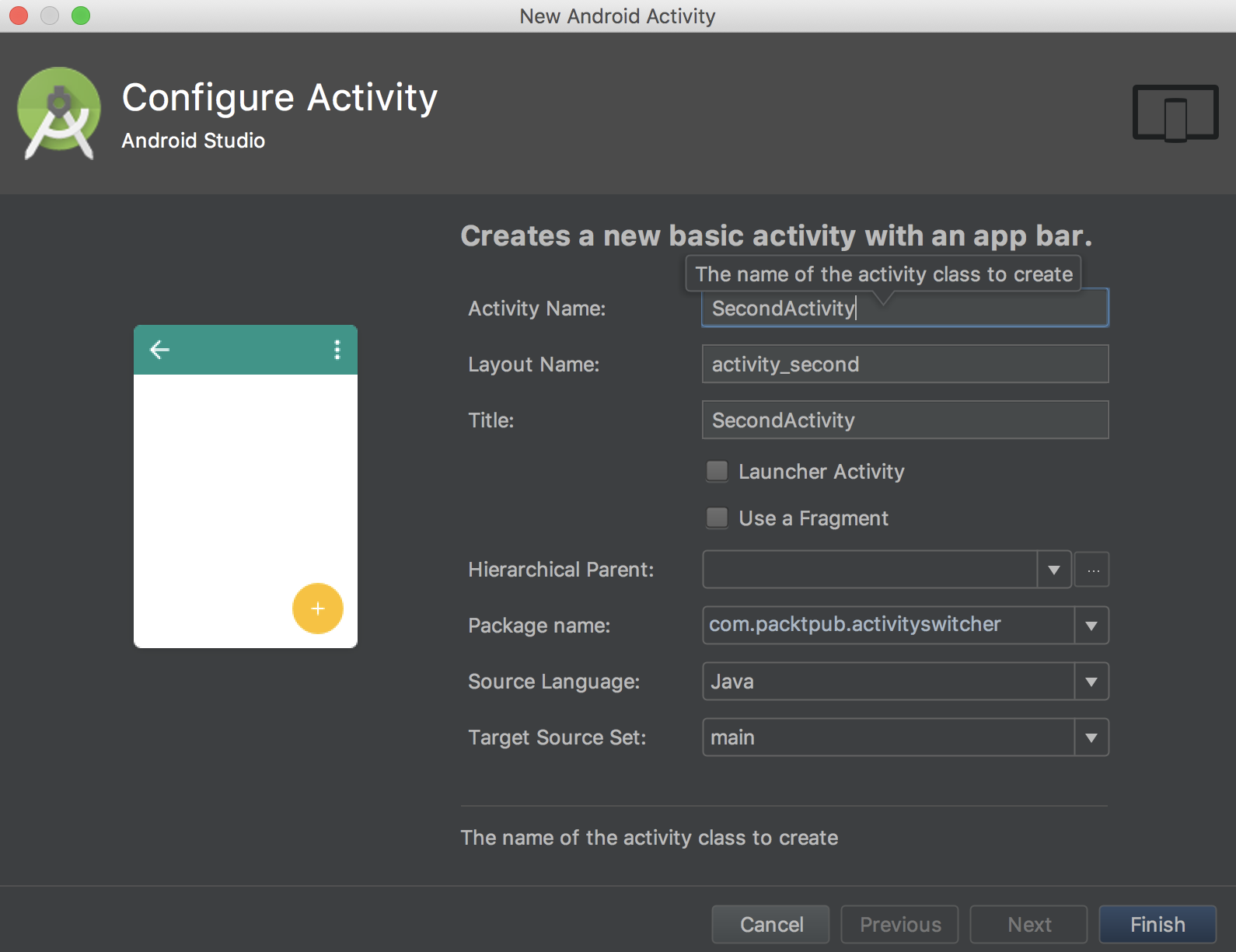
public void onClickSwitchActivity(View view) { Intent intent = new Intent(this, SecondActivity.class); startActivity(intent); }
- Now, open the
activity_main.xml
file located in theres/layout
folder and replace the<TextView />
with the following XML to create the button:
<Button android:id="@+id/button" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_centerVertical="true" android:layout_centerHorizontal="true" android:text="Launch Second Activity" android:onClick="onClickSwitchActivity" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintLeft_toLeftOf="parent" app:layout_constraintRight_toRightOf="parent" app:layout_constraintTop_toTopOf="parent"/>
- You can run the code at this point and see the second activity open. We're going to go further and add a button to
SecondActivity
to close it, which will bring us back to the first activity. Open theSecondActivity.java
file and add this function:
public void onClickClose(View view) { finish(); }
- Finally, add the
Close
button to theSecondActivity
layout. Open theactivity_second.xml
file and add the following<Button>
element to the auto-generatedConstraintLayout
:
<Button android:id="@+id/buttonClose" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Close" android:layout_centerVertical="true" android:layout_centerHorizontal="true" android:onClick="onClickClose" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintLeft_toLeftOf="parent" app:layout_constraintRight_toRightOf="parent" app:layout_constraintTop_toTopOf="parent"/>
- Run the application on your device or emulator and see the buttons in action.
The real work of this exercise is in the onClickSwitchActivity()
method from step 3. This is where we declare the second activity for the Intent using SecondActivity.class
. We went one step further by adding the close button to the second activity to show a common real-world situation: launching a new activity, then returning to the original calling activity. This behavior is accomplished in the onClickClose()
function. All it does is call finish()
, but that tells the OS that we're done with the activity. Finish doesn't actually return us to the calling activity (or any specific activity for that matter); it just closes the current activity and relies on the application's back stack to show the last activity. If we want a specific activity, we can again use the Intent object and specify the activity class name when creating the Intent.
This activity switching does not make a very exciting application. Our activity does nothing but demonstrates how to switch from one activity to another, which of course will form a fundamental aspect of almost any application that we develop.
If we had manually created the activities, we would need to add them to the manifest. Using the New Android Activity wizard will automatically add the necessary elements to the Android Manifest file. To see what Android Studio did for you, open the AndroidManifest.xml
file and look at the <application>
element:
<activity android:name=".MainActivity"> <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> <activity android:name=".SecondActivity"></activity>
One thing to note in the preceding auto-generated code is that the second activity does not have the <intent-filter>
element. The main activity is generally the entry point when starting the application. That's why MAIN
and LAUNCHER
are defined so that the system will know which activity to launch when the application starts.
- To learn more about embedding widgets such as the Button, visit Chapter 2, Views, Widgets, and Styles