In this recipe, we will add a button widget, and we will use this button to change an attribute of another widget that is a part of our GUI. This introduces us to callback functions and event handling in a Python GUI environment.
Creating buttons and changing their text attributes
Getting ready
This recipe extends the previous one, Adding a label to the GUI form. You can download the entire code from https://github.com/PacktPublishing/Python-GUI-Programming-Cookbook-Third-Edition/.
How to do it...
In this recipe, we will update the label we added in the previous recipe as well as the text attribute of the button. The steps to add a button that performs an action when clicked are as follows:
- Start with the GUI_add_label.py module and save it as GUI_create_button_change_property.py.
- Define a function and name it click_me():
def click_me()
- Use ttk to create a button and give it a text attribute:
action.configure(text="** I have been Clicked! **")
a_label.configure (foreground='red')
a_label.configure(text='A Red Label')
- Bind the function to the button:
action = ttk.Button(win, text="Click Me!", command=click_me)
- Use the grid layout to position the button:
action.grid(column=1, row=0)
The preceding instructions produce the following code (GUI_create_button_change_property.py):
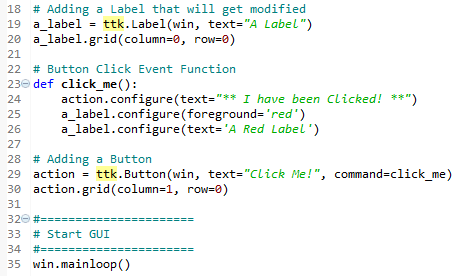
- Run the code and observe the output.
The following screenshot shows how our GUI looks before clicking the button:
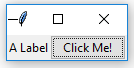
After clicking the button, the color of the label changed and so did the text of the button, which can be seen in the following screenshot:
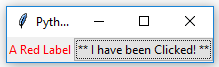
Let's go behind the scenes to understand the code better.
How it works...
In line 19, we assign the label to a variable, a_label, and in line 20, we use this variable to position the label within the form. We need this variable in order to change its attributes in the click_me() function. By default, this is a module-level variable, so as long as we declare the variable above the function that calls it, we can access it inside the function.
Line 23 is the event handler that is invoked once the button gets clicked.
In line 29, we create the button and bind the command to the click_me() function.
Lines 20 and 30 both use the grid layout manager, which will be discussed in Chapter 2, Layout Management, in the Using the grid layout manager recipe. This aligns both the label and the button. We also change the text of the label to include the word red to make it more obvious that the color has been changed. We will continue to add more and more widgets to our GUI, and we will make use of many built-in attributes in the other recipes of this book.
We've successfully learned how to create buttons and change their text attributes. Now, let's move on to the next recipe.