In this chapter, we will explore the different best practices that can help us to build our GUI efficiently and keep it both maintainable and extendable.
These best practices will also help you to debug your GUI to get it just the way you want it to be.
Here is the overview of Python modules for this chapter:
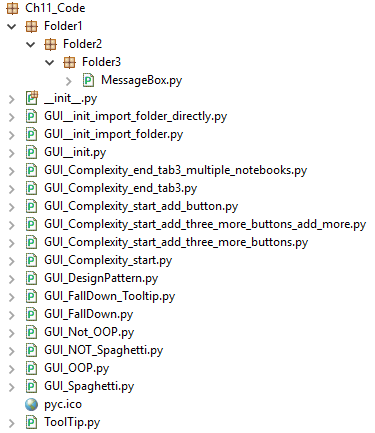
Knowing how to code using best practices will greatly enhance your Python programming skills.
The recipes that will be discussed in this chapter are the following:
-
- Avoiding spaghetti code
- Using __init__ to connect modules
- Mixing fall-down and OOP coding
- Using a code naming convention
- When not to use OOP
- How to use design patterns successfully
- Avoiding complexity
- GUI design using multiple notebooks