In this chapter, we will save our GUI data into tkinter variables. We will also start using object-oriented programming (OOP), writing our own classes in Python. This will lead us to creating reusable OOP components. By the end of this chapter, you will know how to save data from the GUI into local tkinter variables. You will also learn how to display tooltips over widgets, which give the user additional information. Knowing how to do this makes our GUI more functional and easier to use.
Here is an overview of the Python modules for this chapter:
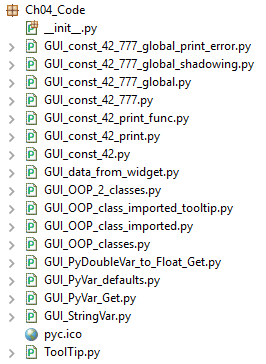
In this chapter, we will use data and OOP classes using Python 3.7 and above. We will cover the following recipes:
- How to use StringVar()
- How to get data from a widget
- Using module-level global variables
- How coding in classes can improve the GUI
- Writing callback functions
- Creating reusable GUI components