Using Flutter Packages
Packages are certainly one of the greatest features that Flutter offers. Both the Flutter team and third-party developers add and maintain packages in the Flutter ecosystem daily. This makes building apps much faster and more reliable, as you can focus on the specific features of your app while leveraging classes and functions that have been created and tested by other developers.
Packages are published at https://pub.dev. This is the hub where you can go to search for packages and verify their platform compatibility (iOS, Android, web, and desktop), popularity, versions, and use cases. Chances are that you’ve already used pub.dev
several times before reading this chapter.
Here is the current home of the pub.dev
repository, with the search box at the center of the screen:
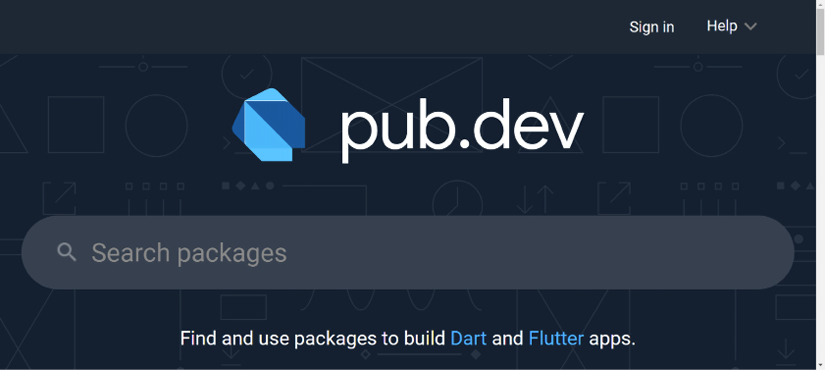
Figure 11.1: pub.dev home page
In this chapter, we will cover the following topics:
- Importing packages and dependencies
- Using dev packages ...