After delving into the theory of CSS, you can finally start using Less. As mentioned earlier, the syntax of Less is very similar to the syntax of CSS. More precisely, Less extends the CSS syntax. This means that any valid CSS code is, in fact, a valid Less code too. With Less, you can produce the CSS code that can be used to style your website. The process used to make CSS from Less is called compiling, where you can compile the Less code via server side or client side. The examples given in this book will make use of client-side compiling. Client side, in this context, means loading a Less file in the browser and using JavaScript on the client machine to compile and apply the resulting CSS. Client-side compiling is used in this book because it is the easiest way to get started, while still being good enough for developing your Less skills.
Tip
It is important to note that the results from client-side compiling serve only demonstration purposes. For production, and especially when considering the performance of an application, it is recommended that you use server-side precompiling. Less bundles a compiler based on Node.js
, and many other GUIs are available to precompile your code. These GUIs will be discussed toward the end of this chapter.
You can finally start using Less. The first thing you have to do is download Less from http://www.lesscss.org/. In this book, Version 2 of less.js
will be used. After downloading it, an HTML5 document will be created. It will include less.js
and your very first Less file. Instead of a local version of the less.js
compiler, you include the latest version from a content delivery network (CDN). Flexboxes have been mentioned because they have the potential to play an important role in the future of web design. For now, they are beyond the scope of this book. This book will focus on creating responsive and flexible layouts with Less using CSS media queries and grids.
Note
The client-side examples in this book load from the less.js
compiler CDN by using the following code in the section HEAD
of the HTML files:
<script src="//cdnjs.cloudflare.com/ajax/libs/less.js/2.x.x/less.min.js"></script>
Replace 2.x.x in the preceding code with the latest version available.
Note that you can download the examples from the support files for this chapter in the downloadable files for the book at www.packtpub.com.
Tip
Downloading the example code
You can download the example code files for all Packt Publishing books you have purchased from your account at http://www.packtpub.com/. If you purchased this book elsewhere, you can visit http://www.packtpub.com/support/ and register to have the files e-mailed directly to you.
To start with, have a look at this plain yet well-structured HTML5 file:
<!doctype html> <html lang="en"> <head> <meta charset="utf-8"> <title>Example code</title> <meta name="description" content="Example code"> <meta name="author" content="Bass Jobsen"> <link rel="stylesheet/less" type="text/css" href="less/styles.less" /> <script src="//cdnjs.cloudflare.com/ajax/libs/less.js/2.2.0/less.min.js"></script> </head> <body> <h1>Less makes me Happy!</h1> </body> </html>
As you can see, a Less file has been added to this document by using the following code:
<link rel="stylesheet/less" type="text/css" href="less/styles.less" />
When rel="stylesheet/less"
is used, the code will be the same as for a style sheet. After the Less file, you can call less.js
by using the following code:
<script src="//cdnjs.cloudflare.com/ajax/libs/less.js/2.2.0/less.min.js"></script>
Or alternatively, load a local file with a code that looks like the following:
<script src="js/less.js" type="text/javascript"></script>
In fact, that's all that you need to get started!
To keep things clear, html5shiv
(which you can access at http://code.google.com/p/html5shiv/) and Modernizr
(which you can access at http://modernizr.com/) have been ignored for now. These scripts add support and detect the new CSS3 and HTML5 features for older browsers such as IE7 and IE8. It is expected that you will be using a modern browser such as Mozilla Firefox, Google Chrome, or any version of Internet Explorer beyond IE8. These will offer full support of HTML5, CSS3, and media queries, which you will need when reading this book and doing the exercises.
Tip
You already know that you should only use less.js
for development and testing in most of the cases. There can still be use cases, which do justice to the client-side use of less.js
in production. To support less.js
for older browsers, you could try es5-shim
(https://github.com/es-shims/es5-shim/).
Now open http://localhost/index.html
in your browser. You will see the Less makes me happy! header text in its default font and color.
Tip
It is not necessary to have a local web server running. Navigating to the index.html
file on your hard drive with your browser should be enough. Or double click on the index.html
file to open it in your default browser. Unfortunately, this won't work for all browsers, so use Mozilla Firefox in order to be sure. The examples in this book use http://localhost/map/
, but this can be replaced with something similar to file:///map/
or c:\map\
, depending on your situation. Note that you cannot run less.js
from CDN when using the file:// protocol in Google Chrome. You can not use the less.js
compiler from CDN. You should replace it with a local version.
After this, you should open less/styles.less
in your favorite text editor. The syntax of Less and CSS doesn't differ here, so you can enter the following code into this file:
h1{color:red;}
Following this, reload your browser. You will see the header text in red.
From the preceding code, h1
is the selector that selects the HTML H1
attribute in your HTML. The color
property has been set to red
between the accolades. The properties will then be applied onto your selectors, just like CSS does.
The less.js
file has a watch
function, which checks your files for changes and reloads your browser views when they are found. It is pretty simple to use. Execute the following steps:
Add
#!watch
after the URL you want to open, and then reload your browser.Open
http://localhost/index.html#!watch
in your browser and start editing your Less files. Your browser will reflect your changes without having to reload.Now, open
less/styles.less
in your text editor. In this file, writeh1{color:red;}
, and then save the file.You should now navigate to your browser, which will show Less makes me Happy! in red.
Rearrange your screen in order to see both the text editor and browser together in the same window.
Furthermore, if you change
red
toblue
inless/styles.less
, you will see that the browser tracks these changes and shows Less makes me Happy! in blue, once the file is saved.
Pretty cool, isn't it?
As we are only human, we are prone to making mistakes or typos. It is important to be able to see what you did wrong and debug your code. If your Less file contains errors, it won't compile at all. So one small typo breaks the complete style of the document.
Debugging is also easy with less.js
. To use debugging or allow less.js
to display errors, you can add the following line of code to your index.html
file:
<link rel="stylesheet/less" type="text/css" href="less/styles.less" /> <script type="text/javascript">var less = { env: 'development' };</script> <script src="//cdnjs.cloudflare.com/ajax/libs/less.js/2.2.0/less.min.js"></script><script src="less.js" type="text/javascript"></script>
As you can see, the line with var less = { env: 'development' };
is new here. This line contains less
as a JavaScript variable used by less.js
. In fact, this is a global Less object used to parse some settings to less.js
. The only setting that will be used in this book is env: 'development'
. For more settings, you can check http://lesscss.org/#client-side-usage-browser-options.
Tip
The env: 'development'
line also prevents Less from caching. Less doesn't cache files in the browser cache. Instead, files are cached in the browser's local storage. If env
is set to production
, this caching could yield unexpected results as the changed and saved files are not compiled.
Since Version 2 of Less, options can also be specified on the script
tag, as shown in the following code:
<script src="less.js" data-env="development"></script>
Alternatively, you can use the link
tag that refers to your source file, as follows:
<link data-env="development"' rel="stylesheet/less" type="text/css" href="less/styles.less">
To try this new setting, edit less/styles.less
again and remove an accolade curly bracket to create an invalid syntax of the h1{color:red
form, and then save the file.
In your browser, you will see a page like the following screenshot:
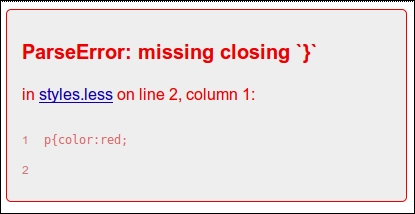
An example of a Less parse error
Besides syntax errors, there will also be name errors displayed. In the case of a name error, an undeclared function or variable would have been used.
It is possible to set other settings for debugging, either in the global Less object or by appending the setting to the URL. For example, you can specify the dumpLineNumbers
setting by adding the following lines of code to your HTML file:
<script type="text/javascript">less = { env: 'development',dumpLineNumbers: "mediaQuery" };</script>
Alternatively, you can add !dumpLineNumbers:mediaQuery
to the URL. You can, for instance, open http://localhost/index.html#!dumpLineNumbers:mediaQuery
in your browser to enable the setting. This setting enables other tools to find the line number of the error in the Less source file. Setting this option to mediaQuery
makes error reporting available for the Firebug or Chrome development tools.
Similarly, setting this to comments
achieves the same for tools such as FireLESS. For instance, using FireLESS allows Firebug to display the original Less filename and the line number of CSS styles generated by Less. Older and experimental versions of Chrome did support this mediaQuery
format. Currently, most browsers offer support for the CSS source maps, as described in the next section, in favor of other source mapping formats. The current version of Less does not support CSS sourcemap for in-browser usage. When developing in browser with the less.js
compiler, you can use the FireLESS add-on for Firefox.
The https://addons.mozilla.org/en-us/firefox/addon/firebug/ path shows that the Firebug add-on integrates with Firefox to put additional development tools, and the FireLESS add-on allows Firebug to display the original Less file name and line number of the Less-generated CSS styles. More information of using FireLESS with the latest version of Less can be found at http://bassjobsen.weblogs.fm/fireless-less-v2/.
Tools like Firebug, or the default Chrome browser development tools, or the default browser used to inspect the elements and functions of your webpage (which you can access by right-clicking on your browser screen) can also be used to see and evaluate the compiled CSS code. The CSS code is displayed as the inline CSS code wrapped inside a <style type="text/css" id="less:book-less-styles">
tag. In the example given in the following screenshot, you will see an ID with the value less:book-less-styles
. The values of this ID have been automatically generated by Less, based on the path and name of the book/less/styles.less
Less file:

The Less-generated CSS styles
Plugins have been used since Version 2 of Less. They can be used with both the client-side and the server-side compiler. The current list of plugins can be found at http://lesscss.org/usage/#plugins-list-of-less-plugins. Writing a plugin yourself is not that difficult, but beyond the scope of this book. You can be sure that the list of useful plugins will grow over time. Less plugins described in this book only work in a node.js application (including Grunt and Gulp) and sometimes also for in browser usage.
The Less plugins can be grouped into the following four or five types:
The first type, the so-called visitors, enable you to change the compile process. The inline URLs plugin converting
url()
to a call todata-uri()
is an example of a visitor plugin.The second type are file manager plugins, which help you to set the files that should be compiled. Examples of file manager plugins are the
npm import
(https://github.com/less/less-plugin-npm-import) andbower resolve
(https://github.com/Mercateo/less-plugin-bower-resolve) plugins.The post-process plugins form the third type. The
autoprefix
(https://github.com/less/less-plugin-autoprefix) andclean-css
(https://github.com/less/less-plugin-clean-css) plugins are examples. They are able to change and modify the already compiled CSS code.The fourth type are the pre-process plugins. Pre-process plugins can automatically add the Less code before your custom code. You can use this type of plugin to load a complete library before your custom code. The
Bootstrap
plugin loads Bootstrap's Less code. You can find theBootstrap
plugin at https://github.com/bassjobsen/less-plugin-bootstrap.Finally, plugins can also be used to extend Less with your own custom functions. Plugins that extend Less with custom functions can be grouped in the fifth type, although these plugins, just like the visitor plugins, change the compile process. An example of a plugin, which extends Less with some custom functions, can be found at https://github.com/less/less-plugin-advanced-color-functions.
To use a plugin with Less in browser, you should first include the plugin source, and then set the plugin option of the less.js
compiler. Your code will look like that shown in the following code snippet:
<script src="plugin.js"></script> <script> var less = { env: "development", plugins: [Plugin] }; </script> <script src="//cdnjs.cloudflare.com/ajax/libs/less.js/2.2.0/less.min.js"></script>
In this book, you will find many code examples. Unless explicitly mentioned, the format of these examples always shows the Less code first, followed by the compiled CSS code. For instance, you can write the following lines of code in Less:
mixin() { color: green; } p { .mixin(); }
This code will be compiled into the following CSS syntax:
p { color: green; }