Jungle Jump – Running and Jumping in a 2D Platformer
In this chapter, you’ll build a platformer game in the tradition of classics such as Super Mario Bros. Platform games are a very popular genre, and understanding how they work can help you make a variety of different game styles. If you’ve never attempted making one before, the player movement in platformers can be surprisingly complex to implement, and you’ll see how Godot’s CharacterBody2D
node has features to help you in that process.
In this project, you will learn about the following:
- Using the
CharacterBody2D
node - Using the
Camera2D
node - Combining animations and user input to produce complex character behavior
- Designing levels using
TileMap
- Creating an infinitely scrolling background using
ParallaxLayer
- Transitioning between scenes
- Organizing your project and planning for expansion
Here is a screenshot of the completed game:
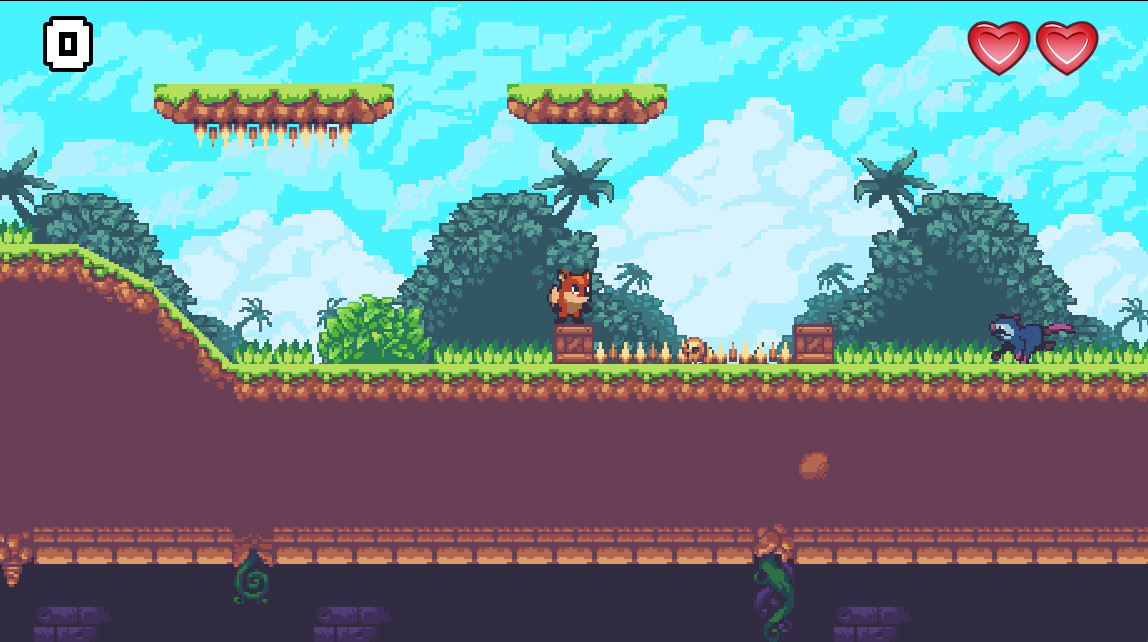