You need variables with a wide variety of widgets. You likely need a string variable to track what the user enters into the entry widget or text widget. You most probably need Boolean variables to track whether the user has checked off the Checkbox widget. You need integer variables to track the value entered in a Spinbox or Slider widget.
In order to respond to changes in widget-specific variables, Tkinter offers its own variable class. The variable that you can use to track widget-specific values must be subclassed from this Tkinter variable class. Tkinter offers some commonly used predefined variables. They are StringVar
, IntVar
, BooleanVar
, and DoubleVar
.
You can use these variables to capture and play with the changes in the values of variables from within your callback functions. You can also define your own variable type, if required.
Creating a Tkinter variable is simple. You simply have to call the constructor:
my_string = StringVar() ticked_yes = BooleanVar() group_choice = IntVar() volume = DoubleVar()
Once the variable is created, you can use it as a widget option, as follows:
Entry(root, textvariable=my_string) Checkbutton(root, text="Remember Me", variable=ticked_yes) Radiobutton(root, text="Option1", variable=group_choice, value="option1") #radiobutton Scale(root, label="Volume Control", variable=volume, from =0, to=10) # slider
Additionally, Tkinter provides access to the values of variables via the set()
and get()
methods, as follows:
my_var.set("FooBar") # setting value of variable my_var.get() # Assessing the value of variable from say a callback
A demonstration of the Tkinter variable class is available in the 1.11.py
code file. The code generates a window, as shown in the following screenshot:
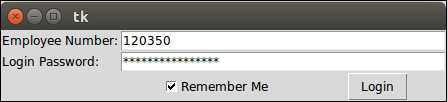
This concludes our brief discussion on events and callbacks. Here's a brief summary of the things that we discussed:
The command binding, which is used to bind simple widgets to certain functions
The use of the
lambda
function in case you need to process argumentsEvent binding using the
widget.bind(event, callback, add=None)
method to bind keyboard and mouse events to your widgets and invoke callbacks when certain events occurThe passing of extra arguments to a callback
The binding of events to an entire application or to a particular class of widget by using
bind_all()
andbind_class()
Using the Tkinter variable class to set and get the values of widget-specific variables
In short, you now know how to make your GUI program responsive to end-user requests!