We got a fairly high-level overview of Tkinter in Chapter 1, Meet Tkinter. Now that we know some things about Tkinter's core widgets, geometry management, and the binding of commands and events to callbacks, let's use our skills in this project to create a text editor.
You're reading from Tkinter GUI Application Development Blueprints
We will, in the process of creating a text editor, take a closer look at some widgets and learn how to tweak them to meet our specific needs.
The following are the key objectives for this project:
Delving into some commonly used widgets, such as the Menu, Menubutton, Text, Entry, Checkbutton, and Button widgets
Exploring the
filedialog
andmessagebox
modules of TkinterLearning the vital concepts of indexing and tagging, as applied to Tkinter
Identifying the different types of Toplevel windows
The goal here is to build a text editor with some cool nifty features. Let's call it the Footprint Editor
.
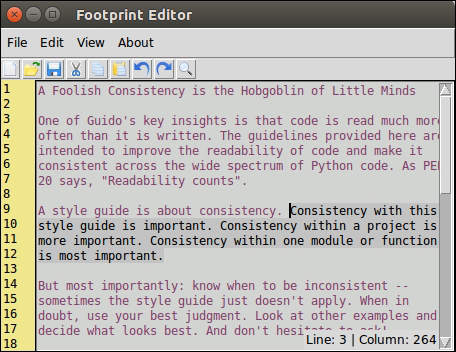
We intend to include the following features in the text editor:
Creating new documents, opening and editing the existing documents, and saving documents
Implementing common editing options such as cut, copy, paste, undo, and redo
Searching within a file for a given search term
Implementing line numbering and the ability to show/hide line numbers
Implementing theme selection to let a user choose custom color themes for the editor
Implementing the about and help windows
Our first goal is to implement the broad visual elements of the text editor. As programmers, we have all used text editors to edit code. We are mostly aware of the common GUI elements of a text editor. So, without much of an introduction, let's get started.
The first phase implements the Menu, Menubutton, Label, Button, Text and Scrollbar widgets. Although we'll cover all of these in detail, you might find it helpful to look at the widget-specific options in the documentation of Tkinter maintained by its author Frederick Lundh at http://effbot.org/tkinterbook/. You can also use the interactive shell, as discussed in Chapter 1, Meet Tkinter.
You might also want to bookmark the official documentation page of Tcl/Tk at http://www.tcl.tk/man/tcl8.6/TkCmd/contents.htm. This site includes the original Tcl/Tk reference. While it does not relate to Python, it provides a detailed overview of each widget and is a useful reference. Remember that Tkinter is just a wrapper...
Tkinter's Text widget comes with some handy built-in functionality to handle common text-related functions. Let's leverage these functionalities to implement some common features in the text editor.
Let's start by implementing the cut, copy, and paste features. We now have the editor GUI ready. If you open the program and play with the Text widget, you will see that you can perform basic functions such as cut, copy, and paste in the text area by using Ctrl + X, Ctrl + C, and Ctrl + V respectively. All of these functions exist without us having to add a single line of code toward these functionalities.
The text widget clearly comes with these built-in events. Now, we simply want to connect these events to their respective menu items.
The documentation of Tcl/Tk Universal widget methods tells us that we can trigger events without an external stimulus by using the following command:
widget.event_generate(sequence, **kw)
To trigger the cut event, all we need is the...
Though we managed to leverage some built-in functionality to gain a quick advantage, we need more control over the text area so that we can bend it to our will. This will require the ability to target each character or location of the text with precision.
We will need to know the exact position of each character, the cursor, or the selected area in order to do anything with the contents of the editor.
The Text widget offers us the ability to manipulate its content using index
, tags
, and mark
, which let us target a position or place within the text area for manipulation.
Indexing helps you target a particular place within a piece of text. For example, if you want to mark a particular word in bold, red, or in a different font size, you can do so if you know the index of the starting point and the index of the end point that needs to be targeted.
The index must be specified in one of the following formats:
The index format |
Description |
---|---|
|
Functions |
Description |
---|---|
| |
|
This returns the filename string, not the opened file object |
| |
|
This returns a list of open file objects or an empty list if |
|
This asks for a filename to save as and returns the opened file object |
|