You're reading from An iOS Developer's Guide to SwiftUI
What this book covers
Chapter 1, Exploring the Environment – Xcode, Playgrounds, and SwiftUI, is an introduction to the software tools used when working with SwiftUI, the new exciting, efficient, and simple-to-use Apple framework for user interfaces.
Chapter 2, Adding Basic UI Elements and Designing Layouts, shows you how to properly refactor view code. Then, it describes how to combine basic views with stacks and control their visual layout.
Chapter 3, Adding Interactivity to a SwiftUI View, discusses the conversion from static to dynamic SwiftUI views, with a focus on responsive design, including taps and gestures. This chapter explores mechanisms to enable views to be made interactive. It covers topics such as view creation, interactivity enhancement, property wrappers, limitations of @State
, bidirectional bindings, subviews, and the use of @ObservableObject
and @
StateObject
classes.
Chapter 4, Iterating Views, Scroll Views, FocusState, Lists, and Scroll View Reader, focuses on showing lists in SwiftUI, through scrollable views such as scroll views or lists. It shows how to handle the visibility of the iOS system keyboard. It introduces NavigationView
for view titles and covers iterating views, @ViewBuilder
, scroll views, @FocusState
for keyboard control, lists, and ScrollViewReader
for element positioning within lists or scroll views.
Chapter 5, The Art of Displaying Grids, moves on to creating grid structures in SwiftUI. Topics covered include displaying grids in iOS, the grid view, lazy grids, using GridItem
for layout control, conditional view formatting, and responding design to device orientation changes.
Chapter 6, Tab Bars and Modal View Presentation, focuses on using tab bars and modal view presentations in SwiftUI. It begins with the TabView
, which is the most common way of moving between views in a small-scale iOS app. Topics covered include how to add a tab bar using TabView
and tabItem
, implementing custom tab bars, and an exhaustive investigation into modal views such as sheets, alerts, and popovers.
Chapter 7, All About Navigation, introduces the concept of navigation in SwiftUI. It starts with an overview of iOS navigation and then deals with programmatic and user-initiated navigation. It illustrates the changes with Swift 4 and iOS 16. Topics discussed are navigation across platforms, basic navigation with NavigationView
and NavigationLink
, .navigationDestination
, user-controlled and split view navigation, programmatic navigation with NavigationPath
, and saving/restoring the navigation stack in the JSON format.
Chapter 8, Creating Custom Graphics, shows you how to style apps by creating custom modifiers, diving into the use of core graphics inside the Canvas view, CALayers
integration with SwiftUI. Then, the chapter goes further, illustrating how to use CustomLayout
.
Chapter 9, An Introduction to Animations in SwiftUI, highlights SwiftUI animations, explaining their state-driven, reactive nature, made possible by SwiftUI’s declarative syntax. It explains the built-in modifiers of animation, transition, and scaleEffect
.
Chapter 10, App Architecture and SwiftUI Part I – the Practical Tools, dissects the impact that SwiftUI has had in restructuring the application architecture on Apple’s operating systems. It introduces conceptual tools that allow a developer to segment an app into manageable components. This chapter focuses on ad hoc architecture rather than offering a one-size-fits-all solution. Key topics include diagrams, dependency inversion, clean architecture, decoupling techniques, state management, and iOS 17 changes on state bindings.
Chapter 11, App Architecture and SwiftUI Part II – the Theory, introduces modern application architecture, taking note of the specificity of the iOS context. It explains the concept of software architecture to give a theoretical understanding and criteria for evaluating well-designed architecture. Key topics include the principles of lightweight architecture, conflict resolution, defining good architecture, the importance of software patterns, the role of the architect, consulting experts, the difference between full-scale applications and examples, and the impact of Conway’s law.
Chapter 12, Persistence with Core Data, focuses on defining persistence, explaining Core Data’s structure, its integration with SwiftUI, and its practical use in Xcode. It touches on CloudKit for cloud-based data storage. Key topics include the Core Data’s framework classes, Core Data with SwiftUI, project creation and migrations, the SQLite data file, and CloudKit.
Chapter 13, Modern Structured Concurrency, discusses concurrency in mobile application development nowadays, applied specifically to Swift. The chapter outlines the history of concurrency from traditional mechanisms, such as threads and callbacks, to Apple’s modern structured concurrency approach. Its topics include async
/await
, tasks, task groups, asynchronous sequences and streams, actors, and integrating old-fashioned concurrency with modern structured concurrency.
Chapter 14, An Introduction to SwiftData, describes Apple’s ORM (Object Relational Mapping) framework, SwiftData, which is set to replace Core Data in SwiftUI development. The topics covered include SwiftData versus Core Data, SwiftData’s features, SwiftUI integration, data modeling, and the changes in binding.
Chapter 15, Consuming REST services in SwiftUI, explains HTTP, and REST as concepts and how to integrate REST services into SwiftUI applications for iOS apps that demand communication over the internet. Topics covered include HTTP requests made using URLSession
, converting to and from JSON using Codable, watching UI changes with ObservableObject
and @Published
, avoiding man-in-the-middle attacks, and handling network errors.
Chapter 16, Exploring the Apple Vision Pro, introduces Apple Vision Pro, an advanced mixed-reality headset, and its importance for spatial computing. It describes the device’s immersive three-dimensional interface. It also goes into the development tools for visionOS, starting development with visionOS, and the initial steps in visionOS development.
To get the most out of this book
It is assumed that you will be familiar with basic computer science and programming in the Swift programming language on Apple devices.
Software/hardware covered in the book |
Operating system requirements |
macOS |
A recent macOS Version, at least Sonoma (14.2.1 or later), in order to follow examples based on Xcode 15.2 for the last chapters. |
Xcode |
Most examples will run on Xcode 14.3 or later except when indicated at the beginning of the chapter. The chapters on SwiftData and visionOS require Xcode 15.2 or later. |
Physical devices |
You can follow the examples for iOS and iPadOS by using the simulator; you don’t need physical devices for learning. To run the code in this book on macOS, you will need macOS 14.2. |
If you are using the digital version of this book, we advise you to type the code yourself or access the code from the book’s GitHub repository (a link is available in the next section). Doing so will help you avoid any potential errors related to the copying and pasting of code.
You will need a developer’s account only if you want to use physical devices. Xcode can be downloaded for free from the Mac App Store and won’t require a developer’s account in order to run your own applications on the simulator.
Download the example code files
You can download the example code files for this book from GitHub at https://github.com/PacktPublishing/An-iOS-Developer-s-Guide-to-SwiftUI. If there’s an update to the code, it will be updated in the GitHub repository.
We also have other code bundles from our rich catalog of books and videos available at https://github.com/PacktPublishing/. Check them out!
Conventions used
There are a number of text conventions used throughout this book.
Code in text
: Indicates code words in text, database table names, folder names, filenames, file extensions, pathnames, dummy URLs, user input, and Twitter handles. Here is an example: “In order to create explicit animations, you use the .animation(_: value:)
modifier rather than the simpler .
withAnimation
closure.”
A block of code is set as follows:
// if you are using Xcode 14.x you will need this syntax for the preview functionality: struct ContentView_Previews: PreviewProvider { static var previews: some View { ContentView() }
When we wish to draw your attention to a particular part of a code block, the relevant lines or items are set in bold:
[default] // if you are using Xcode 15 or later, the preview can be simplified as follows: #Preview { ContentView() }
Any command-line input or output is written as follows:
$ cd projectFolder $ open .
Bold: Indicates a new term, an important word, or words that you see on screen. For instance, words in menus or dialog boxes appear in bold. Here is an example: “Select Settings from the Xcode menu.”
Tips or important notes
Appear like this.
Get in touch
Feedback from our readers is always welcome.
General feedback: If you have questions about any aspect of this book, email us at customercare@packtpub.com and mention the book title in the subject of your message.
Errata: Although we have taken every care to ensure the accuracy of our content, mistakes do happen. If you have found a mistake in this book, we would be grateful if you would report this to us. Please visit www.packtpub.com/support/errata and fill in the form.
Piracy: If you come across any illegal copies of our works in any form on the internet, we would be grateful if you would provide us with the location address or website name. Please contact us at copyright@packt.com with a link to the material.
If you are interested in becoming an author: If there is a topic that you have expertise in and you are interested in either writing or contributing to a book, please visit authors.packtpub.com.
Download a free PDF copy of this book
Thanks for purchasing this book!
Do you like to read on the go but are unable to carry your print books everywhere?
Is your e-book purchase not compatible with the device of your choice?
Don’t worry!, Now with every Packt book, you get a DRM-free PDF version of that book at no cost.
Read anywhere, any place, on any device. Search, copy, and paste code from your favorite technical books directly into your application.
The perks don’t stop there, you can get exclusive access to discounts, newsletters, and great free content in your inbox daily
Follow these simple steps to get the benefits:
- Scan the QR code or visit the following link:
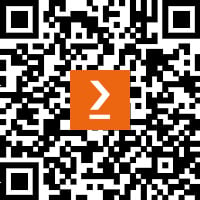
https://packt.link/free-ebook/9781801813624
- Submit your proof of purchase.
- That’s it! We’ll send your free PDF and other benefits to your email directly.