Interactive text entry with Input Field
While we often just wish to display non-interactive text messages to the user, there are times (such as name entry for high scores) where we want the user to be able to enter text or numbers into our game. Unity provides the UI Input Field component for this purpose. In this recipe, we’ll create an input field that prompts the user to enter their name:

Figure 2.27: Example of interactive text entry
Having interactive text on the screen isn’t of much use unless we can retrieve the text that’s entered to be used in our game logic, and we may need to know each time the user changes the text’s content and act accordingly. In this recipe, we’ll add an event handler C# script that detects each time the user finishes editing the text and updates an extra message onscreen, confirming the newly entered content.
Getting ready
For this recipe, we have prepared the required assets in a folder named IconsCursors in the 02_09
folder.
How to do it...
To create an interactive text input box for the user, follow these steps:
- Create a new Unity 2D project and install TextMeshPro by choosing: Window | TextMeshPro | Import TMP Essential Resources.
- Change the background of Main Camera to solid white. Do this in the Inspector for the Camera component by setting Clear Flags to Solid Color, and then choosing a white color for the Background property. The complete background of the Game panel should now be white.
- Add a UI Input Field - TextMeshPro named
InputField
to the scene (importing TMP Essential Resources). Position this at the top center of the screen. - Add a UI Text - TextMeshPro GameObject to the scene, naming it
Text-prompt
. Position this to the left of Input Field. Change the Text property of this GameObject toName:
. - Create a new UI Text - TextMeshPro GameObject named Text-display. Position this to the right of the Input Text control, and make its text red.
- Delete all of the content of the Text property of GameObject Text-display (so that, initially, the user won’t see any text onscreen for this GameObject).
- Create a new C# script class named
DisplayChangedTextContent
containing the following code:using UnityEngine; using TMPro; public class DisplayChangedTextContent : MonoBehaviour { public TMP_InputField inputField; private TextMeshProUGUI textDisplay; void Awake() { textDisplay = GetComponent<TextMeshProUGUI>(); } public void DISPLAY_NEW_VALUE() { textDisplay.text = "last entry = '" + inputField.text + "'"; } }
- Add an instance of the
DisplayChangedTextContent
C# script class to theText-display
GameObject. - With
Text-display
selected in the Hierarchy window, from the Project window, drag the InputField GameObject into the public Input Field variable of the Display Changed Text Content (Script) component:
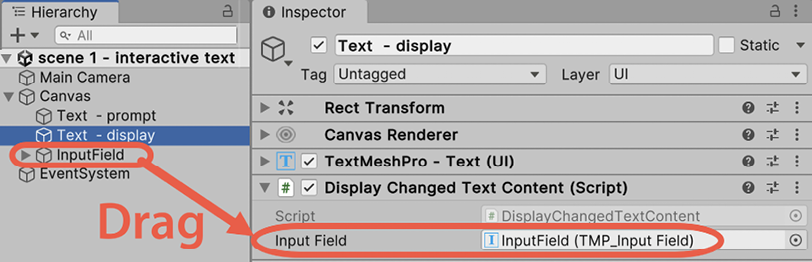
Figure 2.28: Setting the Input Field variable
- Select the
Input Field
GameObject in the Hierarchy. Add an On Value Changed (String) event to the list of event handlers for the Input Field (Script) component. Click on the plus (+) button to add an event handler slot and drag the Text-display GameObject into the Object slot. - Then, from the Function drop-down menu, choose the DisplayChangedTextContent component and then choose the DISPLAY_NEW_VALUE method (we named this in capitals to make it easy to find in the menu!).
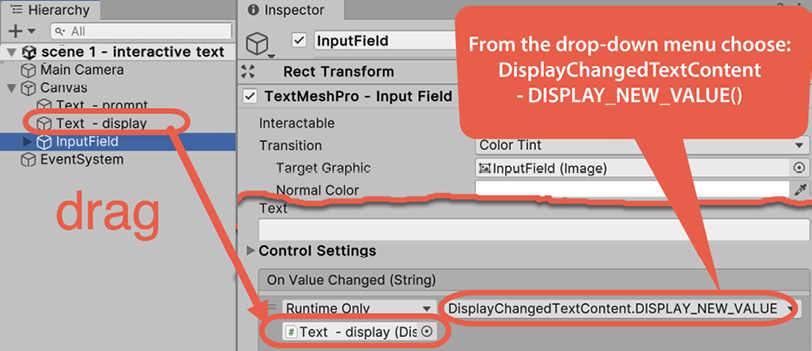
Figure 2.29: Making DISPLAY_NEW_VALUE the method to execute each time the Input Text changes
- Save and run the scene. Each time the user changes the text in the input field, the On Value Changed event will fire, and you’ll see a new content text message displayed in red on the screen.
How it works...
The core of interactive text input in Unity is the responsibility of the Input Field component. This needs a reference to a UI Text GameObject. To make it easier to see where the text can be typed, Text Input (TMP) (similar to buttons) includes a default rounded rectangle image with a white background.
There are usually three Text GameObjects involved in user text input:
- The static prompt text, which, in our recipe, displays the text Name:.
- The faint placeholder text, reminding users where and what they should type.
- The editable text object (with the font and color settings) that is actually displayed to the user, showing the characters as they type.
First, you created an InputField
GameObject, which automatically provides two child Text
GameObjects, named Placeholder
and Text
. These represent the faint placeholder text and the editable text, which you renamed Text-input
. You then added a third Text
GameObject, Text-prompt
, containing Name:.
The built-in scripting that is part of Input Field components does lots of work for us. At runtime, a Text-Input Input Caret (text insertion cursor) GameObject is created, displaying the blinking vertical line to inform the user where their next letter will be typed. When there is no text content, the faint placeholder text will be displayed. As soon as any characters have been typed, the placeholder will be hidden and the characters typed will appear in black text. Then, if all the characters are deleted, the placeholder will appear again.
You then added a fourth Text GameObject called Text-display
and made it red to tell the user what they last entered in the input field. You created the DisplayChangedTextContent
C# script class and added an instance as a component of the Text-display
GameObject. You linked the InputField
GameObject to the Input Field public variable of the scripted component (so that the script can access the text content entered by the user).
Finally, you registered an On Value Changed event handler of the Input Field so that each time the user changes the text in the input field, the DISPLAY_NEW_VALUE()
method of your DisplayChangedTextContent
scripted object is invoked (executed), and the red text content of Text-display
is updated to tell the user what the newly edited text consisted of.
I suggest you use capital letters and underscores when naming methods you plan to register for event handlers, such as our input field On Value Changed event. This makes them much easier to find navigating component methods when setting up the event handler in the Inspector.
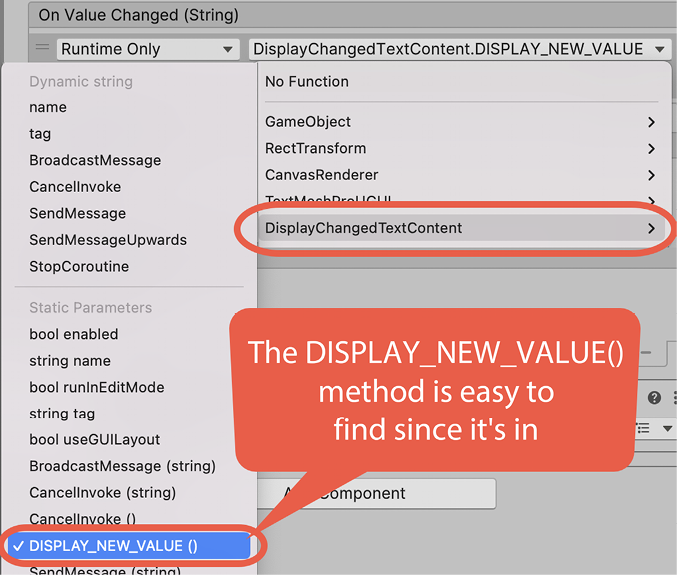
Figure 2.30: Making DISPLAY_NEW_VALUE the method to execute each time the Input Text changes
There’s more...
Rather than the updated text being displayed with every key press, we can wait until Tab or Enter is pressed to submit a new text string by using an On End Edit event handler rather than On Value Changed.
Also, the content type of Input Field (Script) can be set (restricted) to several specific types of text input, including email addresses, integer or decimal numbers only, or password text (where an asterisk is displayed for each character that’s entered). You can learn more about input fields by reading the Unity Manual page: https://docs.unity3d.com/Manual/script-InputField.html.