In this chapter, we're going to learn more about Python and its available modules by creating a program that will allow us to create notes on a map of our local area.
A program such as this needs a proper Graphical User Interface (GUI), which is just a complicated way of saying that it is a visual program with things to see and buttons to click. Here's what the program looks like when it's finished:
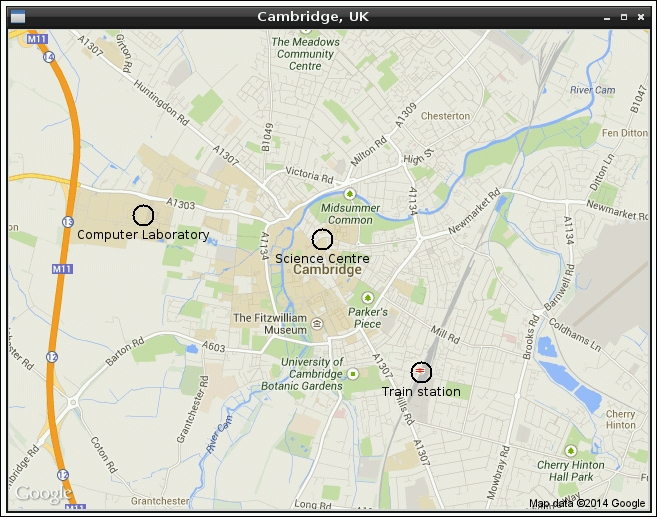
As you can see, the program looks quite professional with its title bar and buttons. You will be able to click on locations on the map and give helpful labels. By the end of this chapter, you will know enough about building GUIs to be able to add all sorts of additional features.