This chapter introduces a collection of components and strategies to make animated and dynamic applications. Most of them are particularly useful for game development. This chapter is full of examples of how to combine different Kivy elements and teaches strategies to control multiple events happening at the same time. The examples are all integrated in a completely new project, a version of the classic Space Invaders game (Copyright ©1978 Taito Corporation, http://en.wikipedia.org/wiki/Space_Invaders). The following is a list of the main components that we will work on in this chapter:
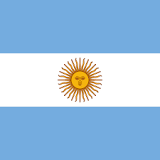
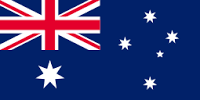
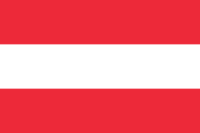
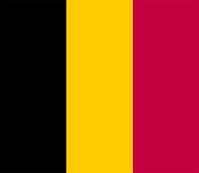
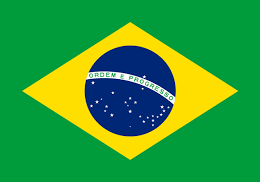
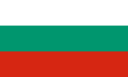
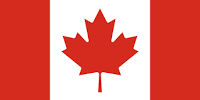
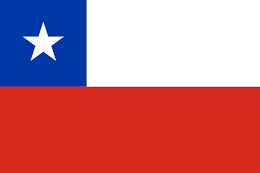
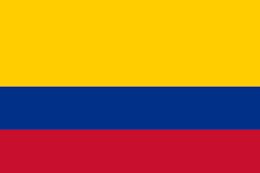
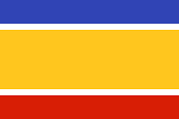
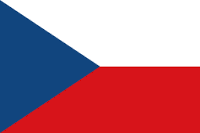
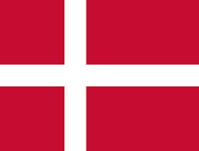
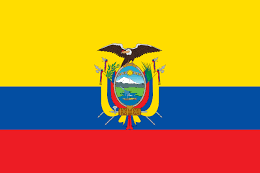
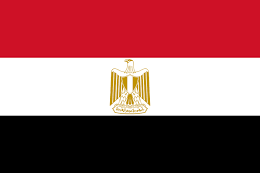
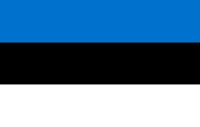
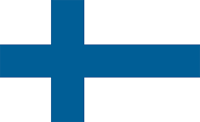
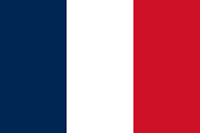
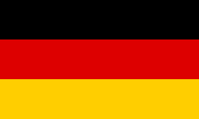
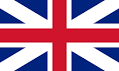
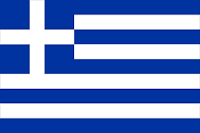
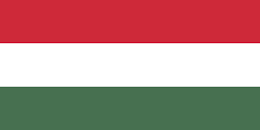
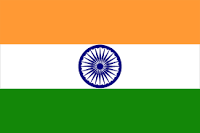
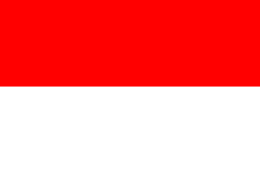
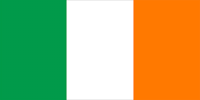
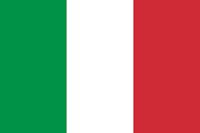
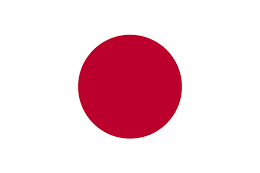
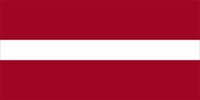
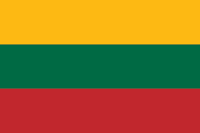
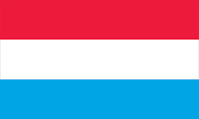
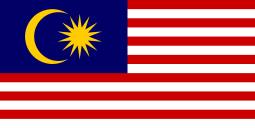
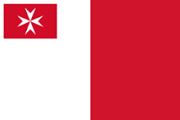
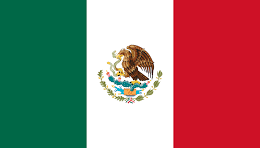
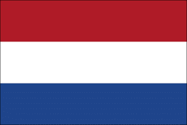
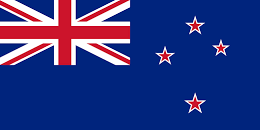
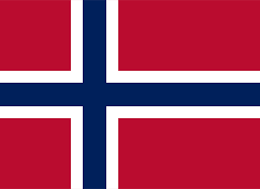
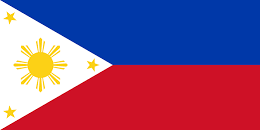
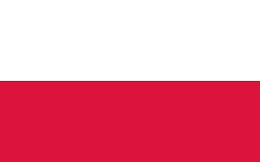
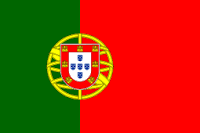
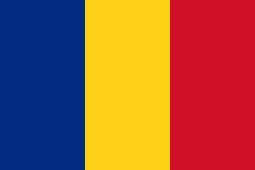
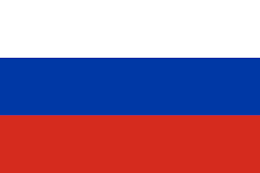

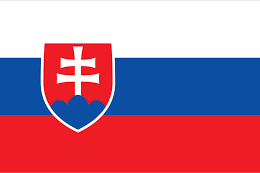
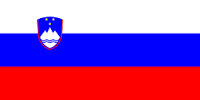
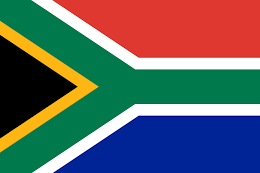
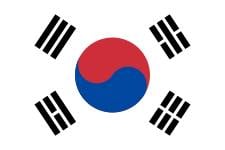
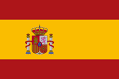
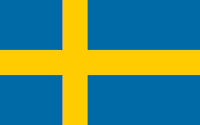
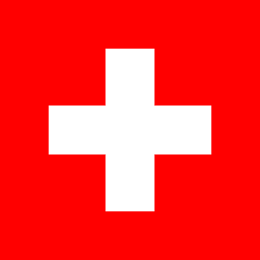
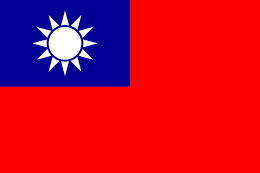
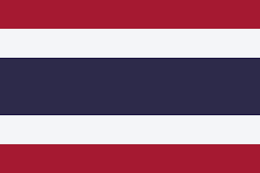
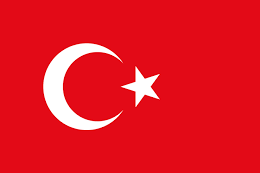
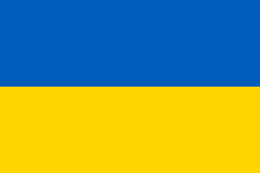
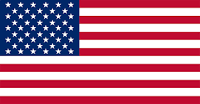