So far, our object-oriented solution includes six classes with their properties and methods. However, if we take another look at these six classes, we will notice that all of them have the same two methods: calculateArea
and calculatePerimeter
. The code for the methods in each class is different because each shape uses a special formula to calculate either the area or perimeter. However, the declarations, contracts, or protocols for the methods are the same. Both methods have the same name, are always parameterless, and return a floating point value. Thus, all of them return the same type.
When we talked about the six classes, we said we were talking about six different geometrical shapes or simply shapes. Thus, we can generalize the required behavior or protocol for the six shapes. The six shapes must define the calculateArea
and calculatePerimeter
methods with the previously explained declarations. We can create a protocol to make sure that the six classes provide the required behavior.
The protocol is a special class named Shape
, and it generalizes the requirements for the geometrical shapes in our application. In this case, we will work with a special class, but in the future, we will use protocols for the same goal. The Shape
class declares two parameterless methods that return a floating point value: calculateArea
and calculatePerimeter
. Then, we will declare the six classes as subclasses of the Shape
class, which will inherit these definitions, and provide the specific code for each of these methods.
The subclasses of Shape
(Square
, EquilateralTriangle
, Rectangle
, Circle
, Ellipse
, and RegularHexagon
) implement the methods because they provide code while maintaining the same method declarations specified in the Shape
superclass. Abstraction and hierarchy are two major pillars of object-oriented programming.
Object-oriented programming allows us to discover whether an object is an instance of a specific superclass. After we chang the organization of the six classes and they become subclasses of Shape
, any instance of Square
, EquilateralTriangle
, Rectangle
, Circle
, Ellipse
, or RegularHexagon
is also a Shape
class. In fact, it isn't difficult to explain the abstraction because we speak the truth about the object-oriented model when we say that it represents the real world. It makes sense to say that a regular hexagon is indeed a shape, and therefore, an instance of RegularHexagon
is a Shape
class. An instance of RegularHexagon
is both a Shape
(the superclass of RegularHexagon
) and a RegularHexagon
(the class that we used to create the object) class.
The following figure shows an updated version of the UML diagram with the superclass or base class (Shape
), its six subclasses, and their attributes and methods. Note that the diagram uses a line that ends in an arrow that connects each subclass to its superclass. You can read the line that ends in an arrow as the following: the class where the line begins "is a subclass of" the class that has the line ending with an arrow. For example, Square
is a subclass of Shape
, and EquilateralTriangle
is a subclass of Shape
.
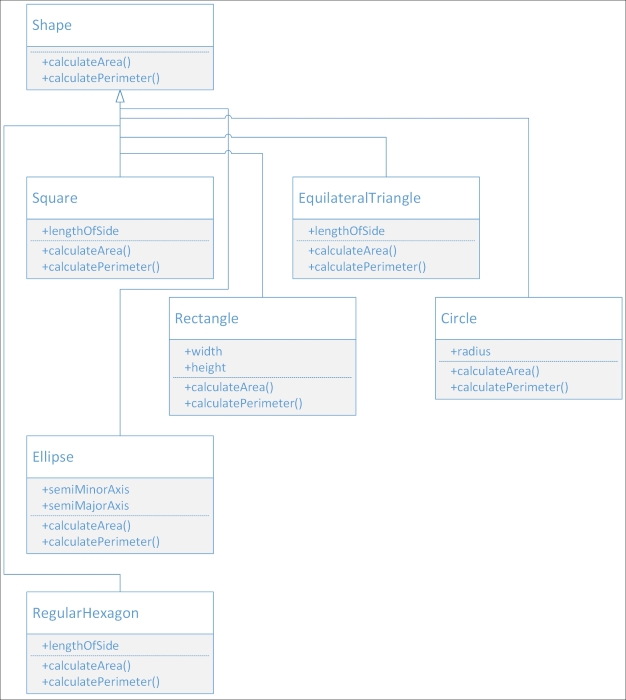
Now, it is time to have a meeting with a domain expert—that is, someone that has an excellent knowledge of geometry. We can use the UML diagram to explain the object-oriented design for the solution. After we explain the different classes that we will use to abstract behavior, the domain expert explains to us that many of the shapes have something in common and that we can generalize behavior even further. The following three shapes are regular polygons:
An equilateral triangle (the
EquilateralTriangle
class)A square (the
Square
class)A regular hexagon (the
RegularHexagon
class)
Regular polygons are polygons that are both equiangular and equilateral. All the sides that compose a regular polygon have the same length and are placed around a common center. This way, all the angles between any two sides are equal. An equilateral triangle is a regular polygon with three sides, the square has four sides, and the regular hexagon has six sides. The following picture shows the three regular polygons and the generalized formulas that we can use to calculate their areas and perimeters. The generalized formula to calculate the area requires us to calculate a cotangent, which is abbreviated as cot:
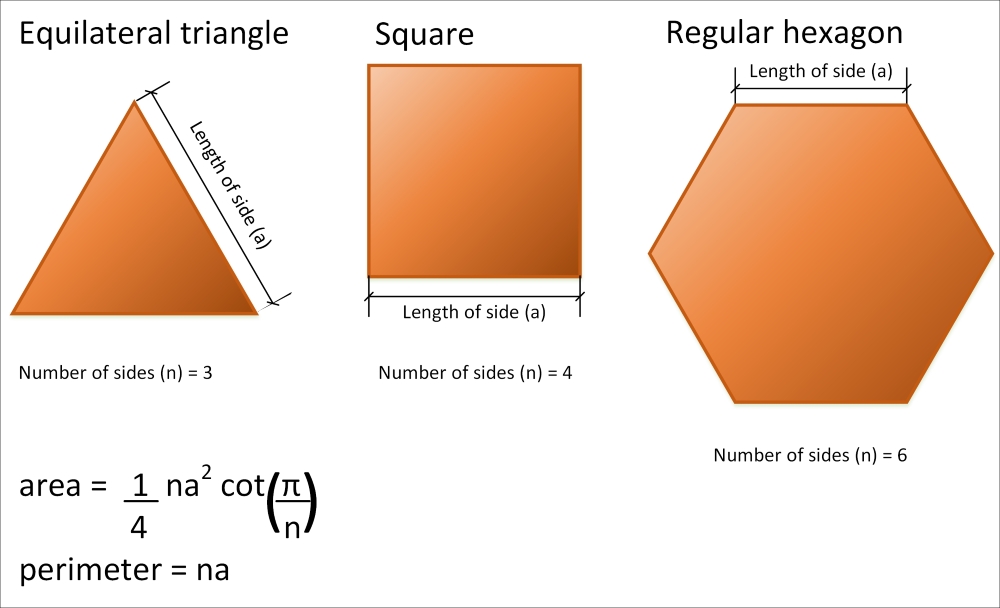
As the three shapes use the same formula with just a different value for the number of sides (n) parameter, we can generalize the required protocol for the three regular polygons. The protocol is a special class named RegularPolygon
that defines a new numberOfSides
property that specifies the number of sides with an integer value. The RegularPolygon
class is a subclass of the previously defined Shape
class. It makes sense because a regular polygon is indeed a shape. The three classes that represent regular polygons become subclasses of RegularPolygon
. However, both the calculateArea
and calculatePerimeter
methods are coded in the RegularPolygon
class using the generalized formulas. The subclasses just specify the right value for the inherited numberOfSides
property, as follows:
EquilateralTriangle
: 3Square
: 4RegularHexagon
: 6
The RegularPolygon
class also defines the lengthOfSide
property that was previously defined in the three classes that represent regular polygons. Now, the three classes become subclasses or RegularPolygon
and inherit the lengthOfSide
property. The following figure shows an updated version of the UML diagram with the new RegularPolygon
class and the changes in the three classes that represent regular polygons. The three classes that represent regular polygons do not declare either the calculateArea
or calculatePerimeter
methods because these classes inherit them from the RegularPolygon
superclass and don't need to make changes to these methods that apply a general formula.
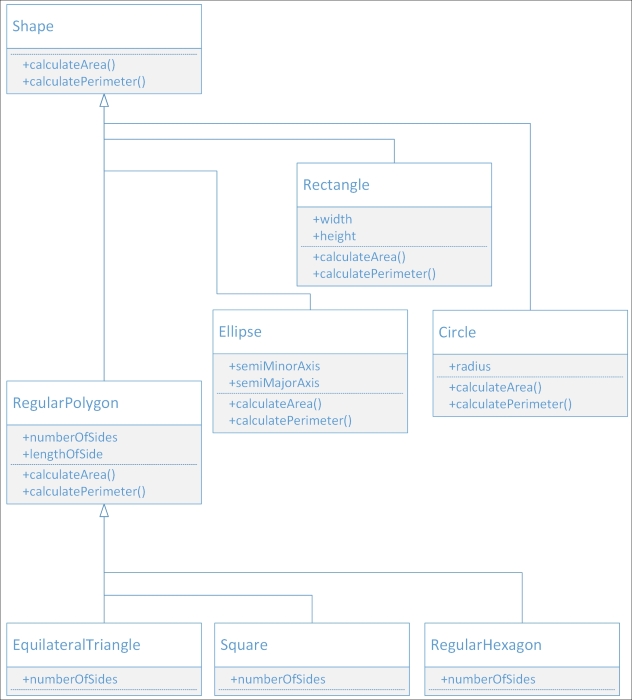
Our domain expert also explains to us a specific issue with ellipses. There are many formulas that provide approximations of the perimeter value for this shape. Thus, it makes sense to add additional methods that calculate the perimeter using other formulas. He suggests us to make it possible to calculate the perimeters with the following formulas:
The second version of the formula developed by Srinivasa Aiyangar Ramanujan
The formula proposed by David W. Cantrell
We will define the following two additional parameterless methods to the Ellipse
class. The new methods will return a floating point value and solve the specific problem of the ellipse shape:
CalculatePerimeterWithRamanujanII
CalculatePerimeterWithCantrell
This way, the Ellipse
class will implement the methods specified in the Shape
superclass and also add two specific methods that aren't included in any of the other subclasses of Shape
. The following figure shows an updated version of the UML diagram with the new methods for the Ellipse
class:
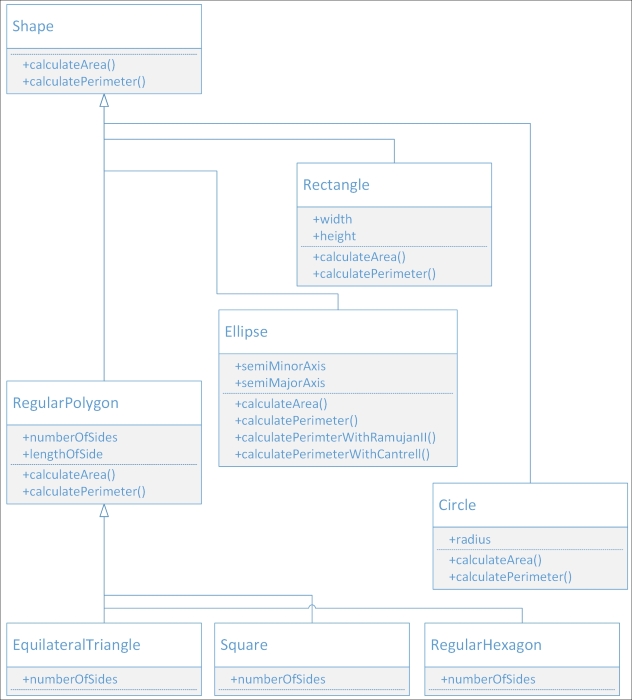