In crowd-simulation games, it would be unnatural to see agents behaving entirely like particles in a physics-based system. The goal of this recipe is to create an agent capable of mimicking our peer-evasion movement.
We need to create a tag called Agent and assign it to those game objects that we would like to avoid, and we also need to have the Agent script component attached to them.
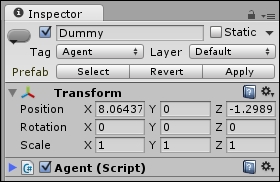
An example of how should look the Inspector of a dummy agent to avoid
This recipe will require the creation and handling of just one file:
Create the
AvoidAgent
behavior, which is composed of a collision avoidance radius and the list of agents to avoid:using UnityEngine; using System.Collections; using System.Collections.Generic; public class AvoidAgent : AgentBehaviour { public float collisionRadius = 0.4f; GameObject[] targets; }
Implement the
Start
function in order to set the list of agents according to the tag we created earlier:void Start () { targets = GameObject.FindGameObjectsWithTag("Agent"); }
Define the
GetSteering
function:public override Steering GetSteering() { // body }
Add the following variables to compute distances and velocities from agents that are nearby:
Steering steering = new Steering(); float shortestTime = Mathf.Infinity; GameObject firstTarget = null; float firstMinSeparation = 0.0f; float firstDistance = 0.0f; Vector3 firstRelativePos = Vector3.zero; Vector3 firstRelativeVel = Vector3.zero;
Find the closest agent that is prone to collision with the current one:
foreach (GameObject t in targets) { Vector3 relativePos; Agent targetAgent = t.GetComponent<Agent>(); relativePos = t.transform.position - transform.position; Vector3 relativeVel = targetAgent.velocity - agent.velocity; float relativeSpeed = relativeVel.magnitude; float timeToCollision = Vector3.Dot(relativePos, relativeVel); timeToCollision /= relativeSpeed * relativeSpeed * -1; float distance = relativePos.magnitude; float minSeparation = distance - relativeSpeed * timeToCollision; if (minSeparation > 2 * collisionRadius) continue; if (timeToCollision > 0.0f && timeToCollision < shortestTime) { shortestTime = timeToCollision; firstTarget = t; firstMinSeparation = minSeparation; firstRelativePos = relativePos; firstRelativeVel = relativeVel; } }
If there is one, then get away:
if (firstTarget == null) return steering; if (firstMinSeparation <= 0.0f || firstDistance < 2 * collisionRadius) firstRelativePos = firstTarget.transform.position; else firstRelativePos += firstRelativeVel * shortestTime; firstRelativePos.Normalize(); steering.linear = -firstRelativePos * agent.maxAccel; return steering;
Given a list of agents, we take into consideration which one is closest, and if it is close enough, we make it so the agent tries to escape from the expected route of that first one according to its current velocity so that they don't collide.