Django overview
Django is a framework consisting of a set of components that solve common web development problems. Django components are loosely coupled, which means they can be managed independently. This helps separate the responsibilities of the different layers of the framework; the database layer knows nothing about how the data is displayed, the template system knows nothing about web requests, and so on.
Django offers maximum code reusability by following the DRY (don’t repeat yourself) principle. Django also fosters rapid development and allows you to use less code by taking advantage of Python’s dynamic capabilities, such as introspection.
You can read more about Django’s design philosophies at https://docs.djangoproject.com/en/5.0/misc/design-philosophies/.
Main framework components
Django follows the MTV (Model-Template-View) pattern. It is a slightly similar pattern to the well-known MVC (Model-View-Controller) pattern, where the template acts as the view and the framework itself acts as the controller.
The responsibilities in the Django MTV pattern are divided as follows:
- Model: This defines the logical data structure and is the data handler between the database and the view.
- Template: This is the presentation layer. Django uses a plain-text template system that keeps everything that the browser renders.
- View: This communicates with the database via the model and transfers the data to the template for viewing.
The framework itself acts as the controller. It sends a request to the appropriate view, according to the Django URL configuration.
When developing any Django project, you will always work with models, views, templates, and URLs. In this chapter, you will learn how they fit together.
The Django architecture
Figure 1.1 shows how Django processes requests and how the request/response cycle is managed with the different main Django components – URLs, views, models, and templates:
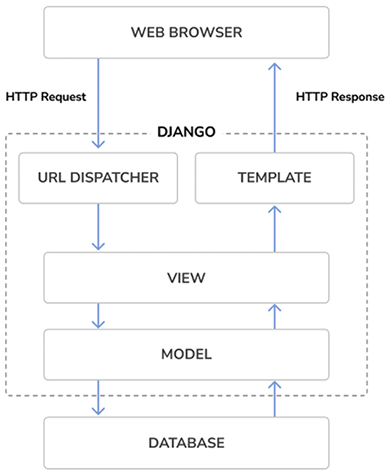
Figure 1.1: The Django architecture
This is how Django handles HTTP requests and generates responses:
- A web browser requests a page by its URL and the web server passes the HTTP request to Django.
- Django runs through its configured URL patterns and stops at the first one that matches the requested URL.
- Django executes the view that corresponds to the matched URL pattern.
- The view potentially uses data models to retrieve information from the database.
- Data models provide data definitions and behaviors. They are used to query the database.
- The view renders a template (usually HTML) to display the data and returns it with an HTTP response.
We will get back to the Django request/response cycle at the end of this chapter in the The request/response cycle section.
Django also includes hooks in the request/response process, which are called middleware. Middleware has been intentionally left out of this diagram for the sake of simplicity. You will use middleware in different examples of this book, and you will learn how to create custom middleware in Chapter 17, Going Live.
We have covered the foundational elements of Django and how it processes requests. Let’s explore the new features introduced in Django 5.
New features in Django 5
Django 5 introduces several key features that you will use in the examples of this book. This version also deprecates certain features and eliminates previously deprecated functionalities. Django 5.0 presents the following new major features:
- Facet filters in the administration site: Facet filters can be added now to the administration site. When enabled, facet counts are displayed for applied filters in the admin object list. This feature is presented in the Added facet counts to filters section of this chapter.
- Simplified templates for form field rendering: Form field rendering has been simplified with the capability to define field groups with associated templates. This aims to make the process of rendering related elements of a Django form field, such as labels, widgets, help text, and errors, more streamlined. An example of using field groups can be found in the Creating templates for the comment form section of Chapter 2, Enhancing Your Blog and Adding Social Features.
- Database-computed default values: Django adds database-computed default values. An example of this feature is presented in the Adding datetime fields section of this chapter.
- Database-generated model fields: This is a new type of field that enables you to create database-generated columns. An expression is used to automatically set the field value each time the model is changed. The field value is set using the
GENERATED
ALWAYS
SQL syntax. - More options for declaring model field choices: Fields that support choices no longer require accessing the
.choices
attribute to access enumeration types. A mapping or callable instead of an iterable can be used directly to expand enumeration types. Choices with enumeration types in this book have been updated to reflect these changes. An instance of this can be found in the Adding a status field section of this chapter.
Django 5 also comes with some improvements in asynchronous support. Asynchronous Server Gateway Interface (ASGI) support was first introduced in Django 3 and improved in Django 4.1 with asynchronous handlers for class-based views and an asynchronous ORM interface. Django 5 adds asynchronous functions to the authentication framework, provides support for asynchronous signal dispatching, and adds asynchronous support to multiple built-in decorators.
Django 5.0 drops support for Python 3.8 and 3.9.
You can read the complete list of changes in the Django 5.0 release notes at https://docs.djangoproject.com/en/5.0/releases/5.0/.
As a time-based release, there are no drastic changes in Django 5, making it straightforward to upgrade Django 4 applications to the 5.0 release.
If you want to quickly upgrade an existing Django project to the 5.0 release, you can use the django-upgrade
tool. This package rewrites the files of your project by applying fixers up to a target version. You can find instructions to use django-upgrade
at https://github.com/adamchainz/django-upgrade.
The django-upgrade
tool is inspired by the pyupgrade
package. You can use pyupgrade
to automatically upgrade syntax for newer versions of Python. You can find more information about pyupgrade
at https://github.com/asottile/pyupgrade.