The request/response cycle
Let’s review the request/response cycle of Django with the application we built. The following schema shows a simplified example of how Django processes HTTP requests and generates HTTP responses:
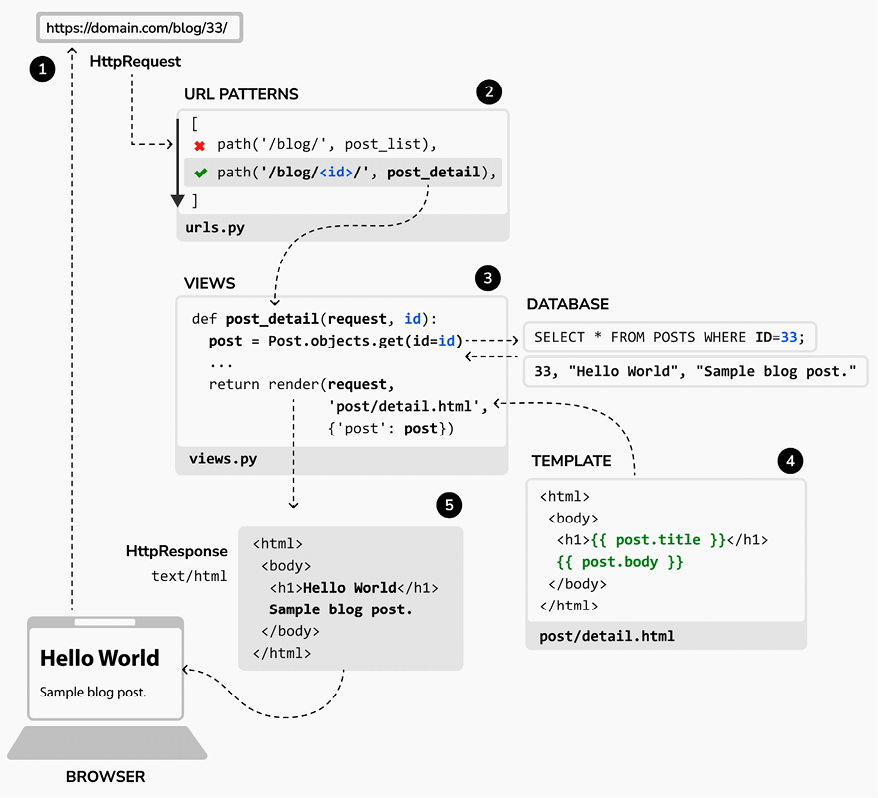
Let’s review the Django request/response process:
- A web browser requests a page by its URL, for example,
https://domain.com/blog/33/
. The web server receives the HTTP request and passes it over to Django. - Django runs through each URL pattern defined in the URL patterns configuration. The framework checks each pattern against the given URL path, in order of appearance, and stops at the first one that matches the requested URL. In this case, the pattern
/blog/<id>/
matches the path/blog/33/
. - Django imports the view of the matching URL pattern and executes it, passing an instance of the
HttpRequest
class and the keyword or positional arguments. The view uses the models to retrieve information from...