Applying groups of styles using ViewModifier
SwiftUI comes with built-in modifiers such as background()
and fontWeight()
, among others. It also gives you the ability to create your own custom modifiers. You can use custom modifiers to combine multiple existing modifiers into one.
In this section, we will create a custom modifier that adds rounded corners and a background to a Text view.
Getting ready
Create a new SwiftUI project named UsingViewModifiers
.
How to do it…
Let’s create a view modifier and use a single line of code to apply it to a Text
view. The steps are given here:
- Replace the current body of the
ContentView
view with:Text("Perfect")
- At the end of the
ContentView.swift
file, create a struct that conforms to theViewModifier
protocol, accepts a parameter of typeColor
, and applies styles to the view’s body:struct BackgroundStyle: ViewModifier { var bgColor: Color func body(content: Content) -> some View{ content .frame(width:UIScreen.main.bounds.width * 0.3) .foregroundStyle(.black) .padding() .background(bgColor) .cornerRadius(20) } }
- Add a custom style to the text using the
modifier()
modifier:Text("Perfect").modifier(BackgroundStyle(bgColor: .blue))
- To apply styles without using a modifier, create an
extension
to theView
protocol. The extension should be created outside the struct or Xcode will issue an error:extension View { func backgroundStyle(color: Color) -> some View{ self.modifier(BackgroundStyle(bgColor: color)) } }
- Replace the modifier on the
Text
view with thebackgroundStyle()
modifier that you just created, which will add your custom styles:Text("Perfect") .backgroundStyle(color: Color.red)
- The result should look like this:
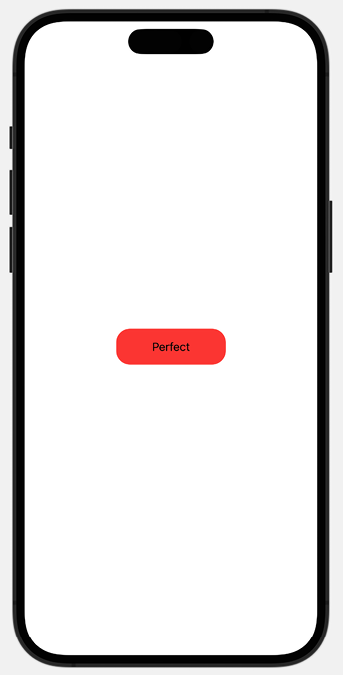
Figure 1.15: Custom view modifier
This concludes the section on view modifiers. View modifiers promote clean coding and reduce repetition.
How it works…
A view modifier
creates a new view by altering the original view to which it is applied. We create a new view modifier by creating a struct that conforms to the ViewModifier
protocol and apply our styles in the implementation of the required body
function. You can make the ViewModifier
customizable by requiring input parameters/properties that would be used when applying styles.
In the example here, the bgColor
property is used in our BackGroundStyle
struct, which alters the background color of the content passed to the body function.
At the end of Step 2, we have a functioning ViewModifier
but decide to make it easier to use by creating a View
extension and adding in a function that calls our struct:
extension View {
func backgroundStyle(color: Color) -> some View {
modifier(BackgroundStyle(bgColor: color))
}
}
We are thus able to use .backgroundStyle(color: Color)
directly on our views instead of .modifier(BackgroundStyle(bgColor:Color))
.
See also
Apple documentation on view modifiers: https://developer.apple.com/documentation/swiftui/viewmodifier.