In this chapter, we develop a classic Tetris game. We look further into the Window
class, including text writing and drawing figures that are more complex. We look also into timing, random numbers, and graphical updates such as falling figures and flash effects. An illustration of it is shown next:
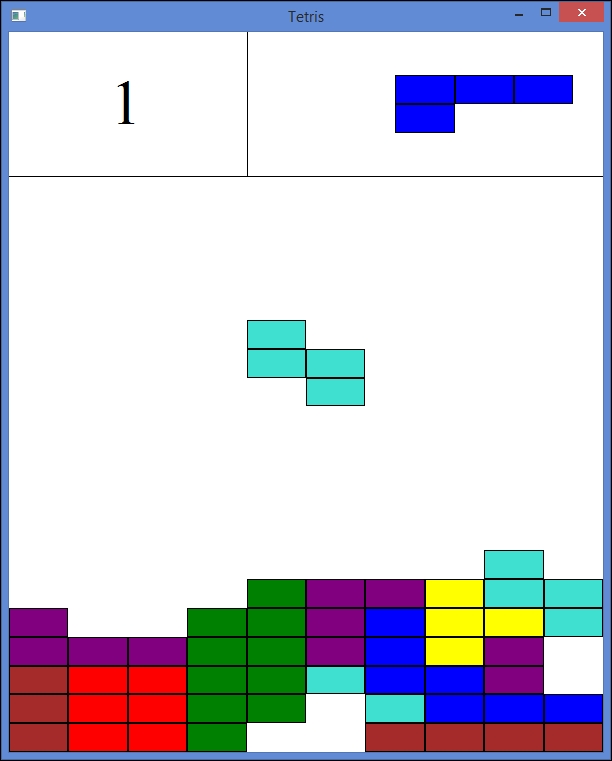