In this chapter, we will make use of the Rainbow HAT to learn how to interact with hardware. This HAT (Hardware on Top) is a selection of components that you can plug into a developer kit to start working with them without the need for any wiring. We will start with an overview of the architecture of Android Things—explaining peripheral lifecycle and user space drivers—and then work with each component of the Rainbow HAT (as shown in the following screenshot) including some best practices along the way.
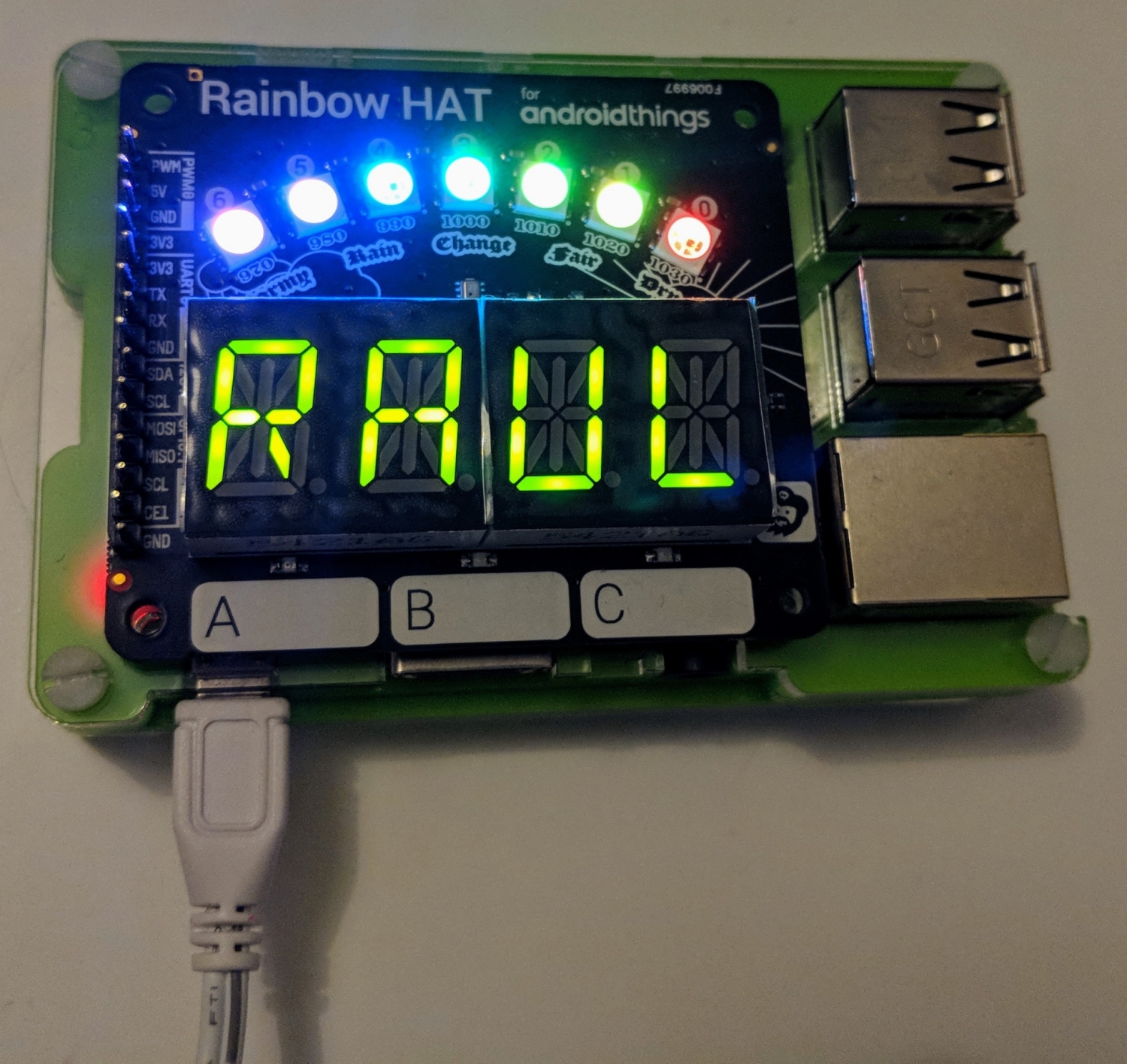
For each part we will use a complete self-contained example and we will analyze how the code works. Along this chapter, we will be using the high-level abstraction drivers for the components that come from the contrib-drivers repository from Google.
We will also take advantage of the meta driver for the...