Reactive programming
Angular supports multiple styles of programming. The plurality of coding styles within Angular is one of the reasons it is approachable to programmers with varying backgrounds. Whether you come from an object-oriented programming background or are a staunch believer in functional programming, you can build viable apps using Angular. In Chapter 2, Forms, Observables, Signals, and Subjects, you’ll begin leveraging reactive programming concepts in building the LocalCast Weather app.
As a programmer, you are most likely used to imperative programming. Imperative programming is when you, as the programmer, write sequential code describing everything that must be done in the order that you’ve defined them and the state of your application, depending on just the right variables to be set to function correctly. You write loops, conditionals, and call functions; you fire off events and expect them to be handled. Imperative and sequential logic is how you’re used to coding.
Reactive programming is a subset of functional programming. In functional programming, you can’t rely on variables you’ve set previously. Every function you write must stand on its own, receive its own set of inputs, and return a result without being influenced by the state of an outer function or class. Functional programming supports Test Driven Development (TDD) very well because every function is a unit that can be tested in isolation. As such, every function you write becomes composable. So you can mix, match, and combine any function you write with any other and construct a series of calls that yield the result you expect.
Reactive programming adds a twist to functional programming. You no longer deal with pure logic but an asynchronous data stream that you transform and mold into any shape you need with a composable set of functions. So when you subscribe to an event in a reactive stream, you’re shifting your coding paradigm from reactive programming to imperative programming.
Later in the book, when implementing the LocalCast Weather app, you’ll leverage subscribe
in action in the CurrentWeatherComponent
and CitySearchComponent
.
Consider the following example, aptly put by Mike Pearson in his presentation Thinking Reactively: Most Difficult, of providing instructions to get hot water from the faucet to help understand the differences between imperative and reactive programming:
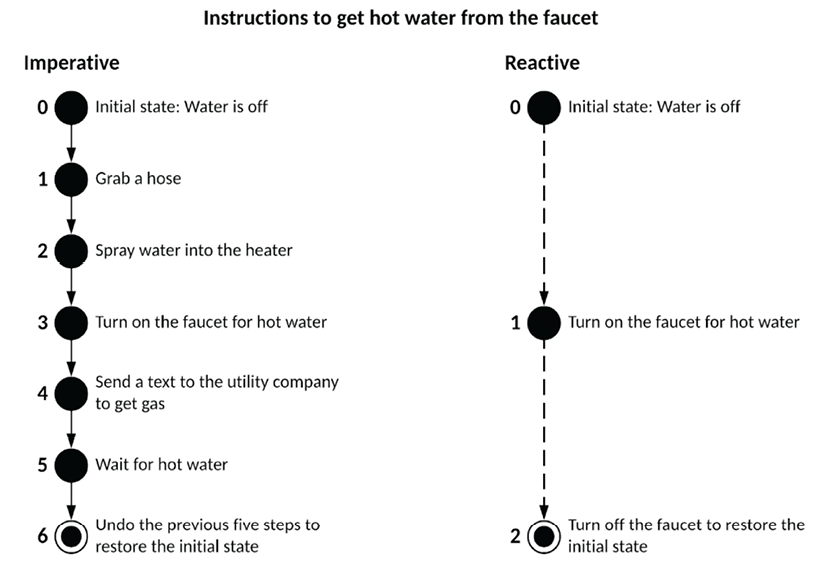
Figure 1.11: Imperative vs Reactive methodology
As you can see, with imperative programming, you must define every step of the code execution. There are six steps in total. Every step depends on the previous step, which means you must consider the state of the environment to ensure a successful operation. In such an environment, it is easy to forget a step and very difficult to test the correctness of every individual step. In functional reactive programming, you work with asynchronous data streams resulting in a stateless workflow that is easy to compose with other actions. There are two steps in total, but step 2 doesn’t require any new logic. It simply disconnects the code in step 1.
RxJS is the library that allows you to implement your code in the reactive paradigm.
Angular 16 introduced signals, in a developer preview, as a new paradigm to enable fine-grained reactivity within Angular. In Chapter 2, Forms, Observables, Signals, and Subjects, you will implement signals in your Angular application. Refer to the Future of Angular section later in the chapter for more information.
RxJS
RxJS stands for Reactive Extensions, a modular library that enables reactive programming. It is an asynchronous programming paradigm that allows data stream manipulation through transformation, filtering, and control functions. You can think of reactive programming as an evolution of event-based programming.
Reactive data streams
In event-driven programming, you would define an event handler and attach it to an event source. In more concrete terms, if you had a Save button, which exposes an onClick
event, you would implement a confirmSave
function that, when triggered, would show a popup to ask the user ‘Are you sure?’. Look at the following diagram for a visualization of this process:
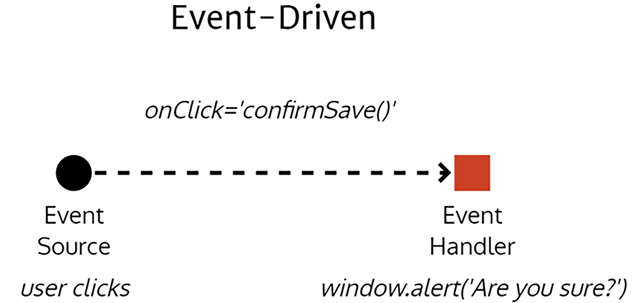
Figure 1.12: Event-driven implementation
In short, you would have an event firing once per user action. If the user clicks on the Save button many times, this pattern will gladly render as many popups as there are clicks, which doesn’t make much sense.
The publish-subscribe (pub/sub) pattern is a different type of event-driven programming. In this case, we can write multiple handlers to all simultaneously act on a given event’s result. Let’s say that your app just received some updated data. The publisher goes through its list of subscribers and passes the updated data to each.
Refer to the following diagram on how the updated data event triggers multiple functions:
- An
updateCache
function updates your local cache with new data - A
fetchDetails
function retrieves further details about the data from the server - A
showToastMessage
function informs the user that the app just received new data
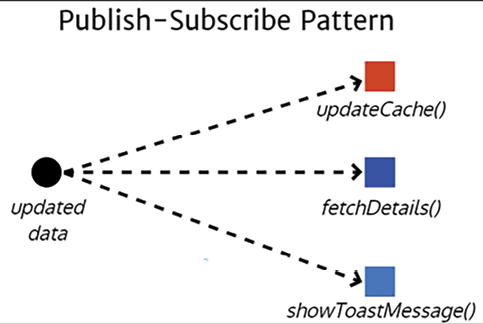
Figure 1.13: Pub/sub pattern implementation
All these events can happen asynchronously; however, the fetchDetails
and showToastMessage
functions will receive more data than they need, and it can get convoluted to try to compose these events in different ways to modify application behavior.
In reactive programming, everything is treated as a stream. A stream will contain events that happen over time, which can contain some or no data. The following diagram visualizes a scenario where your app is listening for mouse clicks from the user. Uncontrolled streams of user clicks are meaningless. You exert some control over this stream by applying the throttle
function, so you only get updates every 250 milliseconds (ms). If you subscribe to this new event stream, every 250 ms, you will receive a list of click events. You may try to extract some data from each click event, but in this case, you’re only interested in the number of click events that happened. Using the map
function, we can shape the raw event data into the sum of all clicks.
Further down the stream, we may only be interested in listening for events with two or more clicks, so we can use the filter
function to act only on what is essentially a double-click event. Every time our filter event fires, it means that the user intended to double-click, and you can act on that information by popping up an alert.
The true power of streams comes from the fact that you can choose to act on the event at any time as it passes through various control, transformation, and filter functions. You can choose to display click data on an HTML list using @for
and Angular’s async
pipe so that the user can monitor the types of click data being captured every 250 ms.
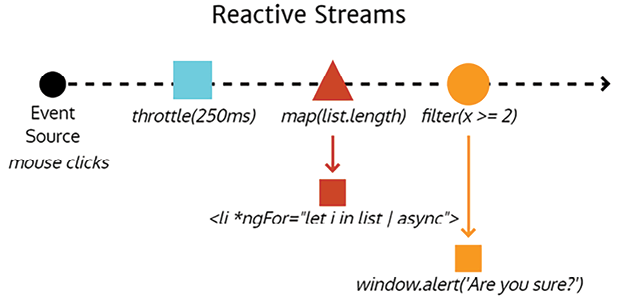
Figure 1.14: A reactive data stream implementation
Now let’s consider some more advanced Angular architectural patterns.