Chapter 1: Hello Blazor
Thank you for picking up your copy of Web Development with Blazor. This book intends to get you started as quickly and pain-free as possible, chapter by chapter, without you having to read this book from cover to cover before getting your Blazor on.
This book will start by guiding you through the most common scenarios you'll come across when you start your journey with Blazor, and will also dive into a few more advanced scenarios. The goal of this book is to show you what Blazor is – both Blazor Server and Blazor WebAssembly – how it all works practically, and to help you avoid any traps along the way.
A common belief is that Blazor is WebAssembly, but WebAssembly is just one way of running Blazor. Many books, workshops, and blog posts on Blazor focus heavily on WebAssembly. This book will cover both WebAssembly and server side. There are a few differences between Blazor Server and Blazor WebAssembly, and I will point those out as we go along.
This first chapter will explore where Blazor came from, what technologies made Blazor possible, and the different ways of running Blazor. We will also touch on which type is best for you.
In this chapter, we will cover the following topics:
- Preceding Blazor
- Introducing WebAssembly
- Introducing .NET 5
- Introducing Blazor
Technical requirements
It is recommended that you have some knowledge of .NET before you start as this book is aimed at .NET developers who wants to utilize their skills to make interactive web applications. However, it's more than possible that you will pick up a few .NET tricks along the way if you are new to the world of .NET.
Preceding Blazor
You probably didn't get this book to read about JavaScript, but it helps to remember that we are coming from a pre-Blazor time. I recall that time – the dark times. Many of the concepts used in Blazor are not that far from the concepts used in many JavaScript frameworks, so I will start with a brief overview of the challenges we faced.
As developers, we have many different platforms we can develop for, including desktop, mobile, games, the cloud (or server side), AI, and even IoT. All these platforms have a lot of different languages to choose from but there is, of course, one more platform: the apps that run inside the browser.
I have been a web developer for a long time, and I've seen code move from the server so that it can run within the browser. It has changed the way we develop our apps. Frameworks such as Angular, React, Aurelia, and Vue have changed the web from having to reload the whole page to updating just small parts of the page on the fly. This new on-the-fly update method has enabled pages to load quicker, as the perceived load time has been lowered (not necessarily the whole page load).
But for many developers, this is an entirely new skill set to learn; that is, switching between a server (most likely C#, if you are reading this book) to a frontend that's been developed in JavaScript. Data objects are written in C# in the backend and then serialized into JSON, sent via an API, and then deserialized into another object written in JavaScript in the frontend.
JavaScript used to work differently in different browsers, which jQuery tried to solve by having a common API that was translated into something the web browser could understand. Now, the differences between different web browsers are much smaller, which has rendered jQuery obsolete in many cases.
JavaScript differs a bit from other languages, since it is not object-oriented or typed, for example. In 2010, Anders Hejlsberg (known for being the original language designer of C#, Delphi, and Turbo Pascal) started to work on TypeScript, an object-oriented language that can be compiled/transpiled into JavaScript.
You can use Typescript with Angular, React, Aurelia, and Vue, but in the end, it is JavaScript that will run the actual code. Simply put, to create interactive web applications today using JavaScript/TypeScript, you need to switch between languages, and also choose and keep up with different frameworks.
In this book, we will look at this in another way. Even though we will talk about JavaScript, our main focus will be on developing interactive web applications using mostly C#.
Now, we know a bit of history about JavaScript. JavaScript is no longer the only language that can run within a browser, thanks to WebAssembly, which we will cover in the next section.
Introducing WebAssembly
In this section, we will look at how WebAssembly works. One way of running Blazor is by using WebAssembly, but for now, let's focus on what WebAssembly is.
WebAssembly a binary instruction format that is compiled and therefore smaller. It is designed for native speeds, which means that when it comes to speed, it is closer to C++ than it is to JavaScript. When loading JavaScript, the JS files (or inline) are downloaded, parsed, optimized, and JIT-compiled; most of those steps are not needed when it comes to WebAssembly.
WebAssembly has a very strict security model that protects users from buggy or malicious code. It runs within a sandbox and cannot escape that sandbox without going through the appropriate APIs. If you want to communicate outside of WebAssembly, for example, by changing the Document Object Model (DOM) or downloading a file from the web, you will need to do that with JavaScript interop (more on that later, and don't worry – Blazor will solve this for us).
To get a bit more familiar with WebAssembly, let's look at some code.
In this section, we will create an app that sums two numbers and returns the result, written in C (to be honest, this is about the level of C I'm comfortable with).
We can compile C into WebAssembly in a few easy steps:
- Navigate to https://wasdk.github.io/WasmFiddle/.
- Add the following code:
int main() { return 1+2; }
- Press Build and then Run.
You will see the number 3
being displayed in the output window toward the bottom of the page, as shown in the following screenshot:
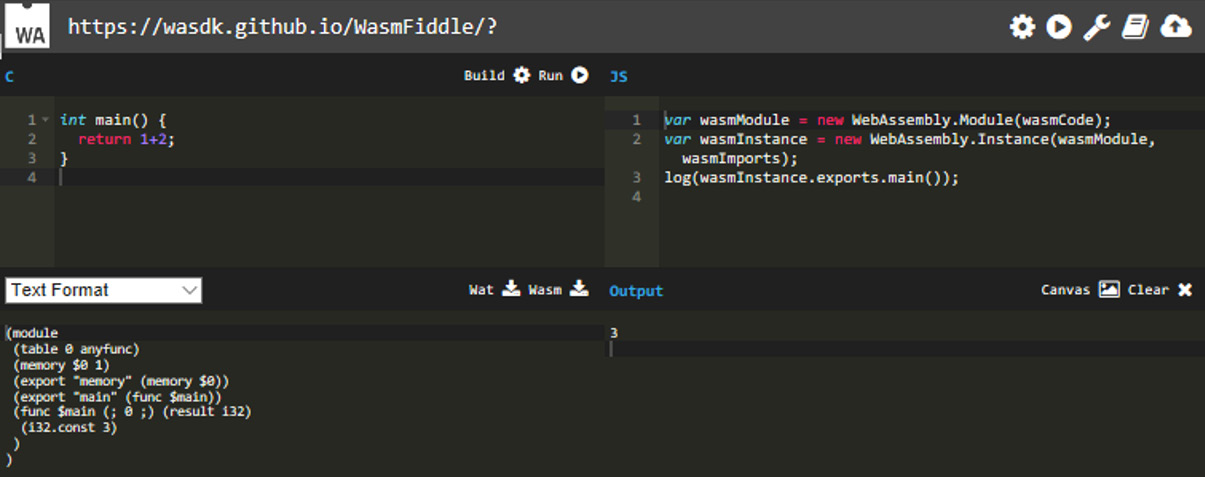
Figure 1.1 – WasmFiddle
WebAssembly is a stack machine language, which means that it uses a stack to perform its operations.
Consider this code:
1+2
Most compilers (including the one we just used) are going to optimize the code and simply return 3
.
But let's assume that all the instructions should be executed. This is the way WebAssembly would do things:
- It will start by pushing
1
onto the stack (instruction: i32.const 1
), followed by pushing2
onto the stack (instruction: i32.const 2
). At this point, the stack contains1
and2
. - Then, we must execute the add-instruction (
i32.add
), which will pop (get
) the two top values (1
and2
) from the stack, add them up, and push the new value onto the stack (3
).
This demo shows that we can build WebAssembly from C code. Now, we have C code that's been compiled into WebAssembly running in our browser.
Other languages
Generally, it is only low-level languages that can be compiled into WebAssembly (such as C or Rust). However, there are a plethora of languages that can run on top of WebAssembly. Here is a great collection of some of these languages: https://github.com/appcypher/awesome-wasm-langs.
WebAssembly is super performant (near-native speeds) – so performant that game engines have already adapted this technology for that very reason. Unity, as well as Unreal Engine, can be compiled into WebAssembly.
Here are a couple of examples of games running on top of WebAssembly:
- Angry Bots (Unity): https://beta.unity3d.com/jonas/AngryBots/
- Doom: https://wasm.continuation-labs.com/d3demo/
This is an amazing list of different WebAssembly projects: https://github.com/mbasso/awesome-wasm.
This section touched the surface of how WebAssembly works and in most cases, you won't need to know much more than that. We will dive into how Blazor uses this technology later in this chapter.
To write Blazor apps, we must leverage the power of .NET 5, which we'll look at next.
Introducing .NET 5
To build Blazor apps, we must use .NET 5. The .NET team has been working hard on tightening everything up for us developers for years. They have been making everything simpler, smaller, cross-platform, and open source – not to mention easier to utilize your existing knowledge of .NET development.
.NET core was a step of the journey toward a more unified .NET. It allowed Microsoft to reenvision the whole .NET platform and build it in a completely new way.
There are three different types of .NET runtimes:
- .NET Framework (full .NET)
- .NET Core
- Mono/Xamarin
Different runtimes had different capabilities and performances. This also meant that creating a .NET core app (for example) had different tooling and frameworks that needed to be installed.
.NET 5 is the start of our journey toward one single .NET. With this unified toolchain, the experience to create, run, and so on will be the same across all the different project types. .NET 5 is still modular in a similar way that we are used to, so we do not have to worry that merging all the different .NET versions is going to result in a bloated .NET.
Thanks to the .NET platform, you will be able to reach all the platforms we talked about at the beginning of this chapter (web, desktop, mobile, games, the cloud (or server side), AI, and even IoT) using only C# and with the same tooling.
Now that you know about some of the surrounding technologies, in the next section, it's time to introduce the main character of this book: Blazor.
Introducing Blazor
Blazor is an open source web UI SPA framework. That's a lot of buzzwords in the same sentence, but simply put, it means that you can create interactive SPA web applications using HTML, CSS, and C# with full support for bindings, events, forms and validation, dependency injection, debugging, and much more. We will take a look at these this book.
In 2017, Steve Sanderson (well-known for creating the Knockout JavaScript framework, and who works for the ASP.NET team at Microsoft) was about to do a session called Web Apps can't really do *that*, can they? at the developer conference NDC Oslo.
But Steve wanted to show a cool demo, so he thought to himself, would it be possible to run C# in WebAssembly? He found an old inactive project on GitHub called Dot Net Anywhere, which was written in C and used tools (similar to what we just did) to compile the C code into WebAssembly.
He got a simple console app running inside the browser. For most people, this would have been an amazing demo, but Steve wanted to take it one step further. He thought, is it possible to create a simple web framework on top of this?, and went on to see if he could get the tooling working as well.
When it was time for his session, he had a working sample where he could create a new project, create a todo-list with great tooling support, and then run the project inside the browser.
Damian Edwards (the .NET team) and David Fowler (the .NET team) were at the NDC conferences as well. Steve showed them what he was about to demo, and they described the event as their heads exploded and their jaws dropped.
And that's how the prototype of Blazor came into existence.
The name Blazor comes from a combination of Browser and Razor (which is the technology used to combine code and HTML). Adding an L made the name sound better, but other than that, it has no real meaning or acronym.
There are a couple of different flavors of Blazor Server, including Blazor WebAssembly, WebWindow, and Mobile Bindings. There are some pros and cons of the different versions, all of which I will cover in the upcoming sections and chapters.
Blazor Server
Blazor Server uses SignalR to communicate between the client and the server, as shown in the following diagram:
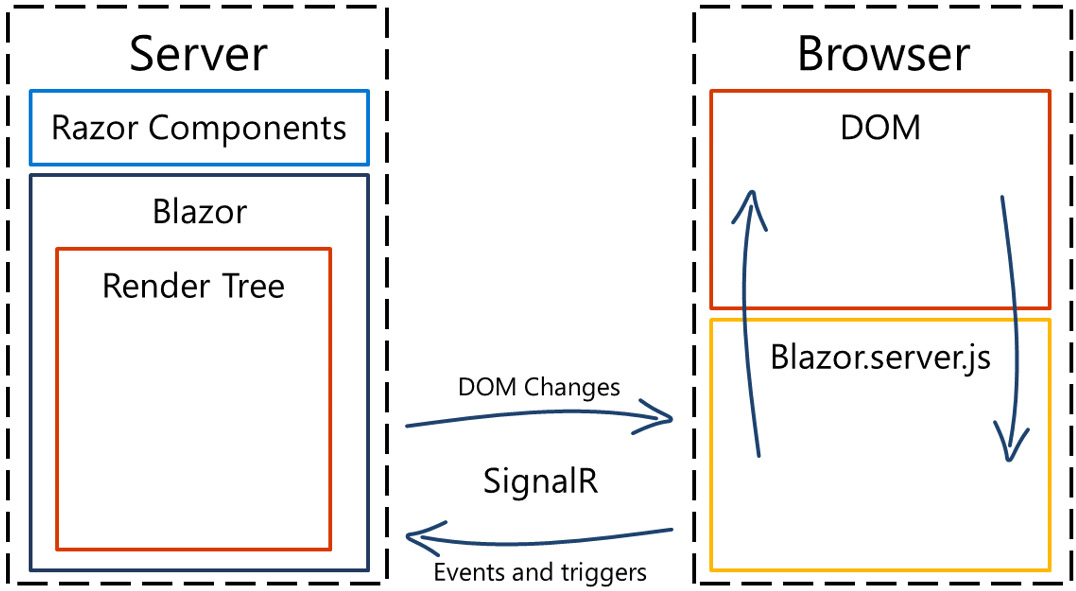
Figure 1.2 – Overview of Blazor Server
SignalR is an open source, real-time communication library that will create a connection between the client and the server. SignalR can use many different means of transporting data and automatically select the best transport protocol for you, based on your server and client capabilities. SignalR will always try to use WebSockets, which is a transport protocol built into HTML5. If WebSockets is not enabled for any reason, it will gracefully fall back to another protocol.
Blazor is built with reusable UI elements called components (more on components in Chapter 3, Introducing Entity Framework Core). Each component contains C# code, markup, and can even include another component. You can use Razor syntax to mix markup and C# code or even do everything in C# if you wish to. The components can be updated by user interaction (pressing a button) or by triggers (such as a timer).
The components get rendered into a render tree, a binary representation of the DOM that contains object states and any properties or values. The render tree will keep track of any changes compared to the previous render tree, and then send only the things that changed over SignalR using a binary format to update the DOM.
On the client side, JavaScript will receive the changes and update the page accordingly. If we compare this to traditional ASP.NET, we only render the component itself, not the entire page, and we only send over the actual changes to the DOM, not the entire page.
There are, of course, some disadvantages to Blazor Server:
- You need to always be connected to the server since the rendering is done on the server. If you have a bad internet connection, the site might not work. The big difference compared to a non-Blazor Server site is that a non-Blazor Server site can deliver a page and then disconnect until it requests another page. With Blazor, that connection (SignalR) must always be connected (minor disconnections are ok).
- There is no offline/PWA mode since it needs to be connected.
- Every click or page update must do a round trip to the server, which might result in higher latency. It is important to remember that Blazor Server will only send the data that was changed. I have not experienced any slow response times.
- Since we have to have a connection to the server, the load on that server increases and makes scaling difficult. To solve this problem, you can use the Azure SignalR hub, which will handle the constant connections and let your server concentrate on delivering content.
- To be able to run it, you have to host it on an ASP.NET Core-enabled server.
However, there are advantages to Blazor Server as well:
- It contains just enough code to establish that the connection is downloaded to the client so that the site has a small footprint.
- Since we are running on the server, the app can take full advantage of the server's capabilities.
- The site will work on older web browsers that don't support WebAssembly.
- The code runs on the server and stays on the server; there is no way to decompile the code.
- Since the code is executed on your server (or in the cloud), you can make direct calls to services and databases within your organization.
At my workplace, we already had a large site in place, so we decided to use Blazor Server for our projects. We had a customer portal and an internal CRM tool. Our approach was to take one component at a time and convert it into a Blazor component.
We quickly realized that, in most cases, it was faster to remake the component in Blazor rather than continuing to use ASP.NET MVC and add functionality on top of that. The User Experience (UX) for the end user became even better as we converted.
The pages loaded faster, we could reload parts of the page as we needed instead of the whole page, and so on.
We did find that Blazor introduced a new problem, though: the pages became too fast. Our users didn't understand if data had been saved because nothing happened; things did happen, but too fast for the users to notice. Suddenly, we had to think more about UX and how to inform the user that something had changed. This is, of course, a very positive side effect from Blazor in my opinion.
Blazor Server is not the only way to run Blazor – you can also run it on the client (in the web browser) using WebAssembly.
Blazor WebAssembly
There is another option: instead of running Blazor on a server, you can run it inside your web browser using WebAssembly.
As we mentioned previously, there is currently no way to compile C# into WebAssembly. Instead, Microsoft has taken the mono runtime (which is written in C) and compiled that into WebAssembly.
The WebAssembly version of Blazor works very similar to the server version, as shown in the following diagram. We have moved everything off the server and it is now running within our web browser:
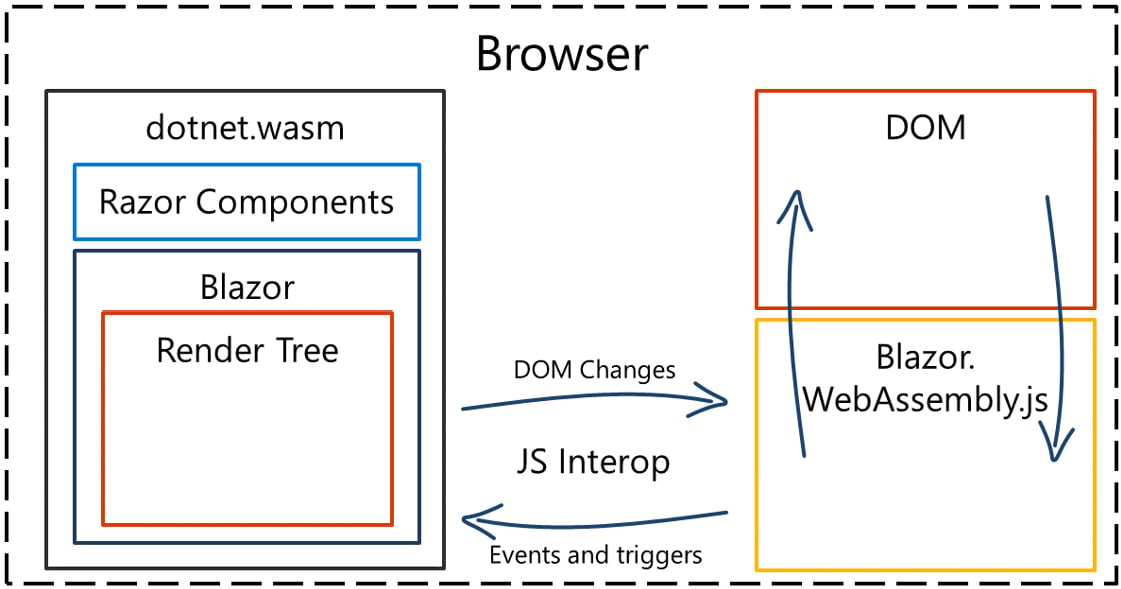
Figure 1.3 – Overview of Blazor Web Assembly
A render tree is still created and instead of running the Razor pages on the server, they are now running inside our web browser. Instead of SignalR, since WebAssembly doesn't have direct DOM access, Blazor updates the DOM with direct JavaScript interop.
The mono runtime that's compiled into WebAssembly is called dotnet.wasm. The page contains a small piece of JavaScript that will make sure to load dotnet.wasm
. Then, it will download blazor.boot.json
, which is a JSON file containing all the files the application needs to be able to run, as well as the entry point of the application.
If we look at the default sample site that is created when we start a new Blazor project in Visual Studio, the Blazor.boot.json
file contains 63 dependencies that need to be downloaded. All the dependencies get downloaded and the app boots up.
As we mentioned previously, dotnet.wasm
is the mono runtime that's compiled into WebAssembly. It runs .NET DLLs – the ones you have written, as well as the ones from .NET Framework (which is needed to run your app) – inside your browser.
When I first heard of this, I got a bit of a bad taste in my mouth. It's running the whole .NET runtime inside my browser?! But then, after a while, I realized how amazing that is. You can use any .NET Standard DLLs and run them in your web browser.
In the next chapter, we will look at exactly what happens and in what order code gets executed when a WebAssembly app boots up.
The big concern is the download size of the site. The simple file new sample app is about 1.3 MB in size, which is quite large if you are putting a lot of effort into download size. What you should remember, though, is that this is more like a Single-Page Application (SPA) – it is the whole site that has been downloaded to the client. I compared the size to some well-known sites on the internet; I then only included the JS files for these sites but also included all the DLLs and JavaScript files for Blazor.
The following is a diagram of my findings:
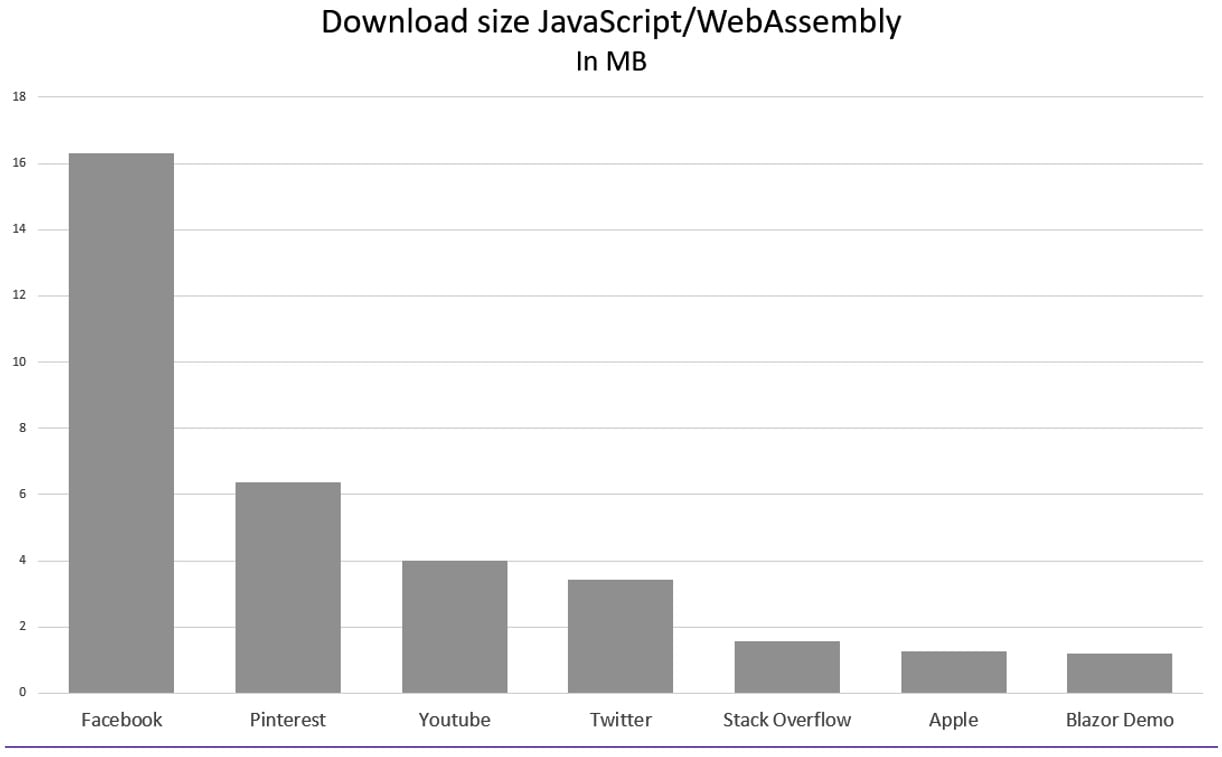
Figure 1.4 – JavaScript download size for popular sites
Even though the other sites are larger than the sample Blazor site, you should remember that the Blazor DLLs are compiled and should take up less space than a JavaScript file. WebAssembly is also faster than JavaScript is.
There are some disadvantages to Blazor WebAssembly:
- Even if we compare it to other large sites, the footprint of a Blazor WebAssembly is large and there are a large number of files to download.
- To access any on-site resources, you will need to create a Web API to access them. You cannot access the database directly.
- The code is running in the browser, which means that it can be decompiled. This is something all app developers are used to, but for web developers, it is perhaps not as common.
There are, of course, some advantages of Blazor WebAssembly as well:
- Since the code is running in the browser, it is easy to create a Progressive Web App (PWA).
- Since we're not running anything on the server, we can use any kind of backend server or even a file share (no need for a .NET-compatible server in the backend).
- No round trips mean that you will be able to update the screen faster (that is why there are game engines that use WebAssembly).
I wanted to put that last advantage to the test! When I was 7 years old, I got my first computer, a Sinclair ZX Spectrum. I remember that I sat down and wrote the following:
10 PRINT "Jimmy" 20 GOTO 10
That was my code; I made the computer write my name on the screen over and over!
That was the moment I decided that I wanted to become a developer, so that I could make computers do stuff.
After becoming a developer, I wanted to revisit my childhood and decided I wanted to and to build a ZX Spectrum emulator. In many ways, the emulator has become my test project, instead of a simple Hello World, when I encounter new technology. I've had it running on a Gadgeteer, Xbox One, and even on a HoloLens (to name a few).
But is it possible to run my emulator in Blazor?
It took me only a couple of hours to get the emulator working with Blazor WebAssembly by leveraging my already built .NET Standard DLL; I only had to write the code that was specific to this implementation, such as the keyboard and graphics. This is one of the reasons Blazor (both Server and WebAssembly) is so powerful: it can run libraries that have already been made. Not only can you leverage your knowledge of C#, but you can also take advantage of the large ecosystem and .NET community.
You can find the emulator here: https://zxspectrum.azurewebsites.net/. This is one of my favorite projects to work on, as I keep finding ways to optimize and improve the emulator.
Building this type of web application used to only be possible with JavaScript. Now, we know can use Blazor WebAssembly and Blazor Server, but which one of these new options is the best?
Blazor WebAssembly versus Blazor Server
Which one should we choose? The answer is, as always, it depends. You have seen the advantages and disadvantages of both.
If you have a current site that you want to port over to Blazor, I would go for server side; once you have ported it, you can make a new decision as to whether you want to go for WebAssembly as well.
If your site runs on a mobile browser or another unreliable internet connection, you might want to consider going for an offline-capable (PWA) scenario with Blazor WebAssembly since Blazor Server needs a constant connection.
The startup time for WebAssembly is a bit slow, but there are ways of combining the two hosting models so that you can have the best of two worlds. We will cover this in Chapter 9, Sharing Code and Resources.
There is no silver bullet when it comes to this question, but read up on the advantages and disadvantages and see how those affect your project and use cases.
We can run Blazor server side and on the client, but what about desktop and mobile apps? There are solutions for that as well, by using WebWindow and Mobile Blazor Bindings.
WebWindow
There is an experimental technology called WebWindow, which is an open source project from Steve Sanderson. It enables us to create Windows applications using Blazor.
WebWindow is outside the scope of this book, but I still want to mention it because it shows how powerful the technology really is and that there is no end to the possibilities with Blazor.
You can find out and read more about this project here: https://github.com/SteveSandersonMS/WebWindow.
Blazor Mobile Bindings
Another example of a project that is outside the scope of this book but is still worth mentioning is Blazor Mobile Bindings. It's a project that makes it possible to create mobile applications for iOS and Android by leveraging Blazor.
Blazor Mobile Bindings uses Razor syntax just like Blazor does; however, the components are completely different.
Although Microsoft is behind both Blazor and Blazor Mobile Bindings, we can't actually share the code between the different web versions (WebAssembly, Server, or WebWindow).
You can find out and read more about this project here: https://docs.microsoft.com/en-us/mobile-blazor-bindings/.
As you can see, there are a lot of things you can do with Blazor, and this is just the beginning.
Summary
In this chapter, you were provided with an overview of the different technologies you can use with Blazor, such as server side, client side (WebAssembly), desktop, and mobile. This overview should have helped you make an informed decision about what technology to choose for your next project.
We then talked about how Blazor was created and its underlying technologies, such as SignalR and WebAssembly. You also learned about the render tree and how the DOM gets updated to give you an understanding of how Blazor works under the hood.
In the upcoming chapters, I will walk you through various scenarios to equip you with the knowledge to handle everything from upgrading an old/existing site, creating a new server-side site, to creating a new WebAssembly site.
In the next chapter, we'll get our hands dirty by configuring our development environment and creating and examining our first Blazor App.
Further reading
As a .NET developer, you might be interested in the Uno Platform (https://platform.uno/), which makes it possible to create a UI in XAML and deploy it to many different platforms, including WebAssembly.
If you want to see how the ZX Spectrum emulator is built, you can download the source code here: https://github.com/EngstromJimmy/ZXSpectrum.