About the Book
As the most popular JavaScript library for building modern, interactive user interfaces, React is an in-demand framework that'll bring real value to your career or next project. But like any technology, learning React can be tricky, and finding the right teacher can make things a whole lot easier.
Maximilian Schwarzmüller is a bestselling instructor who has helped over two million students worldwide learn how to code, and his latest React video course (React—The Complete Guide) has over six hundred thousand students on Udemy.
Max has written this quick-start reference to help you get to grips with the world of React programming. Simple explanations, relevant examples, and a clear, concise approach make this fast-paced guide the ideal resource for busy developers.
This book distills the core concepts of React and draws together its key features with neat summaries, thus perfectly complementing other in-depth teaching resources. So, whether you've just finished Max's React video course and are looking for a handy reference tool, or you've been using a variety of other learning material and now need a single study guide to bring everything together, this is the ideal companion to support you through your next React projects. Plus, it's fully up to date for React 18, so you can be sure you're ready to go with the latest version.
About the Author
Maximilian Schwarzmüller is a professional web developer and bestselling online course instructor. Having learned to build websites and web user interfaces the hard way with just HTML, CSS, and (old-school) JavaScript, he embraced modern frontend frameworks and libraries like Angular and React right from the start.
Having the perspective of a self-taught freelancer, Maximilian started teaching web development professionally in 2015. On Udemy, he is now one of the most popular and biggest online instructors, teaching more than 2mn students worldwide. Students can become developers by exploring more than 40 courses, most of those courses being bestsellers in their respective categories. In 2017, together with a friend, Maximilian also founded Academind to deliver even more and better courses to even more students. For example, Academind's "React – The Complete Guide" course is the bestselling React course on the Udemy platform, reaching more than 500,000 students.
Besides helping students from all over the world as an online instructor, Maximilian never stopped working as a web developer. He still loves exploring and mastering new technologies, building exciting digital products, and sharing his knowledge with fellow developers. He's driven by his passion for good code and engaging websites and apps. Beyond web development, Maximilian also works as a mobile app developer and cloud expert. He holds multiple AWS certifications, including the "AWS Certified Solutions Architect – Professional" certification.
Apart from his courses on Udemy, Maximilian also publishes free tutorial videos on Academind's YouTube channel (https://youtube.com/c/academind) and articles on academind.com. You can also follow him on Twitter (@maxedapps).
Audience
This book is designed for developers who already have some familiarity with React basics. It can be used as a standalone resource to consolidate understanding or as a companion guide to a more in-depth course. To get the most value from this book, it is advised that readers have some understanding of the fundamentals of JavaScript, HTML, and CSS.
Prospective Table of Contents
Chapter 1, React – What and Why, will re-introduce the reader to React.js. Assuming that React.js is not brand-new to the reader, this chapter will clarify which problems React solves, which alternatives exist, how React generally works, and how React projects may be created.
Chapter 2, Understanding React Components and JSX, will explain the general structure of a React app (a tree of components) and how components are created and used in React apps.
Chapter 3, Components and Props, will ensure that readers are able to build reusable components by using a key concept called "props".
Chapter 4, Working with Events and State, will cover how to work with state in React components, which different options exist (single state vs multiple state slices) and how state changes can be performed and used for UI updates.
Chapter 5, Rendering Lists and Conditional Content, will explain how React apps can render lists of content (e.g. list of user posts) and conditional content (e.g. alert if incorrect values were entered into an input field).
Chapter 6, Styling React Apps, will clarify how React components can be styled and how styles can be applied dynamically or conditionally, touching on popular styling solutions like vanilla CSS, styled components, and CSS modules for scoped styles.
Chapter 7, Portals and Refs, will explain how direct DOM access and manipulation is facilitated via the "refs" feature which is built-into React. In addition, readers will learn how Portals may be used to optimize the rendered DOM element structure.
Chapter 8, Handling Side Effects, will discuss the useEffect
hook, explaining how it works, how it can be configured for different use-cases and scenarios, and how side effects can be handled optimally with this React hook.
Chapter 9, Behind the Scenes of React and Optimization Opportunities, will take a look behind the scenes of React and dive into core topics like the virtual DOM, state update batching and key optimization techniques that help developers avoid unnecessary re-render cycles (and thus improve performance).
Chapter 10, Working with Complex State, will explain how the advanced React hook useReducer
works, when and why you might want to use it and how it can be used in React components to manage more complex component state with it. In addition, React's Context API will be explored and discussed in-depth, allowing developers to manage app-wide state with ease.
Chapter 11, Building Custom React Hooks, will explain how developers can build their own, custom React hooks and what the advantage of doing so is.
Chapter 12, Multipage Apps with React Router, will explain what React Router is and how this extra library can be used to build multipage experiences in a React single-page-application.
Chapter 13, Managing Data with React Router, will dive deeper into React Router and explore how this package can also help with fetching and managing data.
Chapter 14, Next Steps and Further Resources, will further cover the core and "extended" React ecosystem and which resources may be helpful for next steps.
Conventions
Code words in text, database table names, folder names, filenames, file extensions, pathnames, dummy URLs, user input, and Twitter handles are shown as follows: "Store the paragraph element reference in a constant named paragraphElement
."
Words that you see on the screen, for example, in menus or dialog boxes, also appear in the text like this: "In the header with the navigation bar you will find the following components: the navigation items (Login
and Profile
) and the Logout
button."
A block of code is set as follows:
const buttonElement = document.querySelector('button'); const paragraphElement = document.querySelector('p'); function updateTextHandler() { paragraphElement.textContent = 'Text was changed!'; } buttonElement.addEventListener('click', updateTextHandler);
New terms and important words are shown like this: "It is currently used by over 5% of the top 1,000 websites and compared to other popular frontend JavaScript frameworks like Angular, React is leading by a huge margin, when looking at key metrics like weekly package downloads via npm (Node Package Manager), which is a tool commonly used for downloading and managing JavaScript packages."
Setting Up Your Environment
Before you can successfully install React.js on your system, you will need to ensure you have the following software installed:
Node.js and npm (included with your installation by default)
These are available for download at https://nodejs.org/en/.
The home page of this site should automatically provide you with the most recent installation options for your platform and system. For more options, select Other Downloads
(the first of three links visible beneath each of your default options). This will open a new page through which you can explore all installation choices for all main platforms, as shown in the screenshot below:
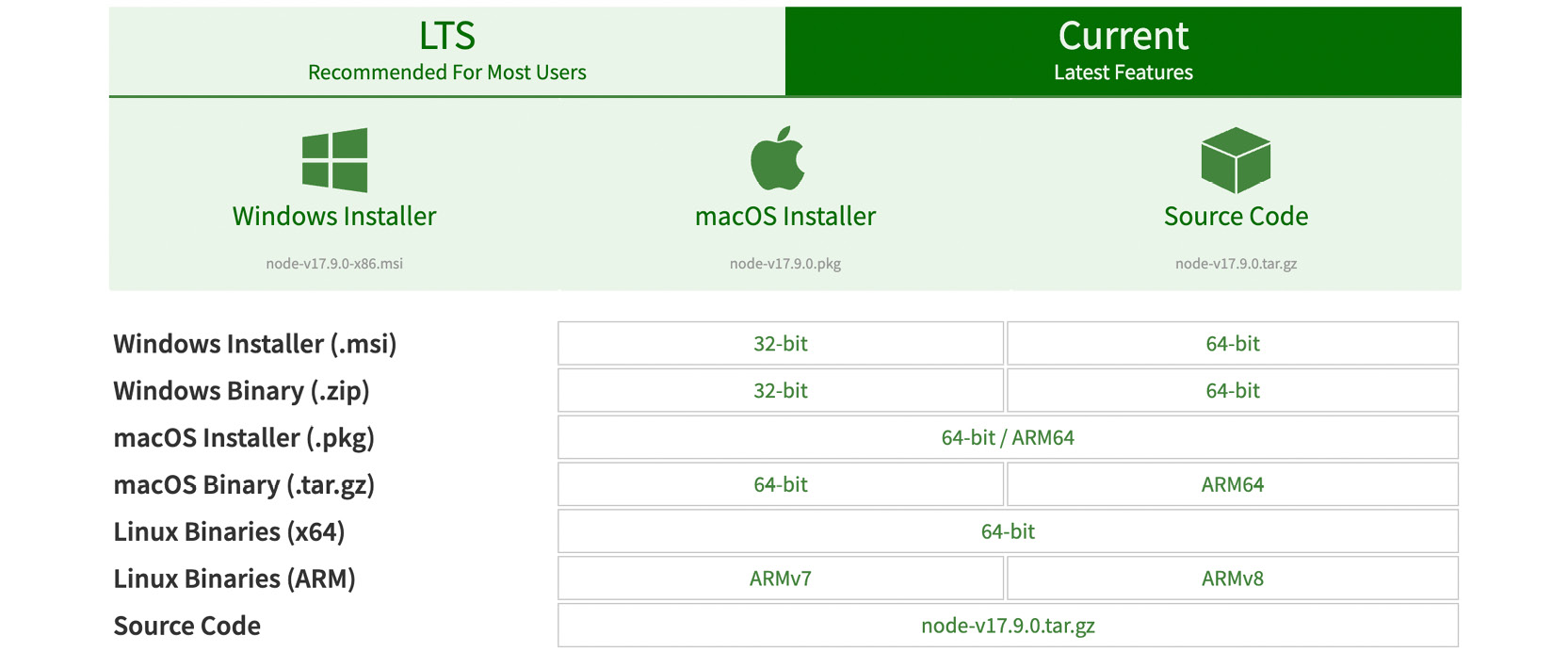
Figure 0.1: Download Node.js source code or pre-built installer
At the bottom of this page, you will find a bullet list of available resources should your system require specialised instructions, including guidance on Node.js installation via source code and node package manager.
Once you have downloaded Node.js through this website, find the .pkg
file in your downloads folder. Double-click this file to open the Install Node.js
pop-up window, then simply follow given instructions to complete your installation.
Installing React.js
React.js projects can be created in various ways, including custom-built project setups that incorporate webpack, babel and other tools. The easiest and recommended way is the usage of the create-react-app
command though. This book uses this method. The creation of a react app will be covered in Chapter 1, React.js – What and Why, but you may refer to this section for step-by-step instructions on this task.
Note
For further guidance regarding the installation and setup of your React.js environment, resources are available at the following: https://reactjs.org/docs/getting-started.html
Perform the following steps to install React.js on your system:
- Open your terminal (Powershell/command prompt for Windows; bash for Linux).
- Use the make directory command to create a new project folder with a name of your choosing (e.g.
mkdir react-projects
) and navigate to that directory using the change directory command (e.g.cd react-projects
). - Enter the following command prompt to create a new project directory within this folder:
npx create-react-app my-app
- Grant permission when prompted to install all required files and folders needed for basic React setup. This may take several minutes.
- Once completed, navigate to your new directory using the cd command:
cd my-app
- Open a terminal window in this new project directory and run the following command to start a Node.js development server and lauch a new browser to preview your app locally:
npm start
- This should open a new browser window automatically, but if it does not, open your browser manually type
http://localhost:3000
in the address/location bar to navigate tolocalhost:3000
, as shown in the screenshot below:
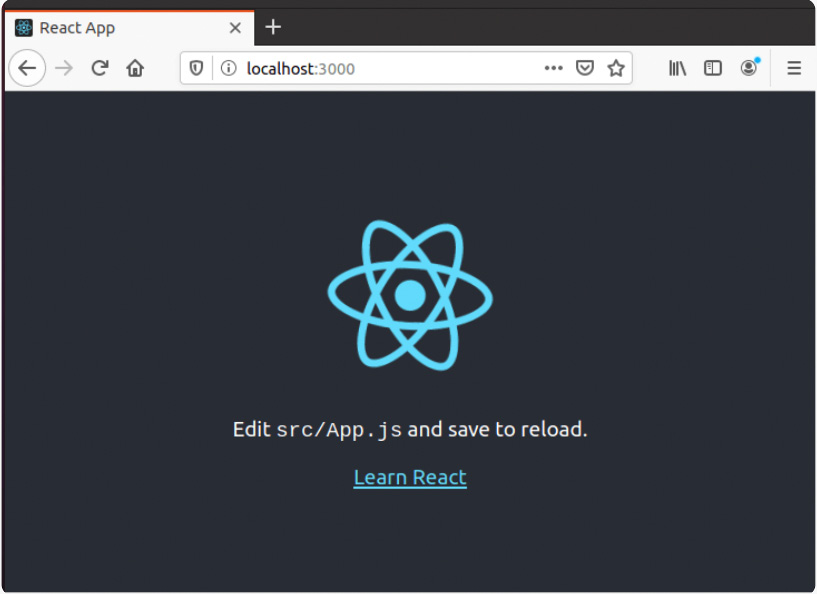
Figure 0.2: Access React App in Your Browser
- When you are ready to stop development for the time being, use
Ctrl
+C
in the same terminal as in step 6 to quit running your server. To relaunch it, simply run thenpm start
command in that terminal once again. Keep the process started bynpm start
up and running while developing, as it will automatically update the website loaded onlocalhost:3000
with any changes you make.
Downloading the Code Bundle
Download the code files from GitHub at https://packt.link/IeoCT. Refer to these code files for the complete code bundle.
Get in Touch
Feedback from our readers is always welcome.
General feedback: If you have any questions about this book, please mention the book title in the subject of your message and email us at customercare@packtpub.com.
Errata: Although we have taken every care to ensure the accuracy of our content, mistakes do happen. If you have found a mistake in this book, we would be grateful if you could report this to us. Please visit www.packtpub.com/support/errata and complete the form.
Piracy: If you come across any illegal copies of our works in any form on the Internet, we would be grateful if you could provide us with the location address or website name. Please contact us at copyright@packt.com with a link to the material.
If you are interested in becoming an author: If there is a topic that you have expertise in and you are interested in either writing or contributing to a book, please visit authors.packtpub.com.
Please Leave a Review
Let us know what you think by leaving a detailed, impartial review on O'Reilly or Amazon. We appreciate all feedback. It helps us continue to make great products and help aspiring developers build their skills. Please spare a few minutes to give your thoughts. It makes a big difference to us.