Project overview
In this project, we will build a component UI library for our Angular projects. Initially, we will use the Angular CLI to scaffold a new Angular workspace for our library. We will then use the Angular CDK and the Bulma CSS framework to create the following components:
- A list of cards that we can rearrange using drag-and-drop features
- A button that will allow us to copy arbitrary content to the clipboard
We will learn to deploy the library into a package registry such as npm. Finally, we will convert one of our components into an Angular element for sharing it with non-Angular applications using the ngx-build-plus library. The following diagram describes an architectural overview of the project:
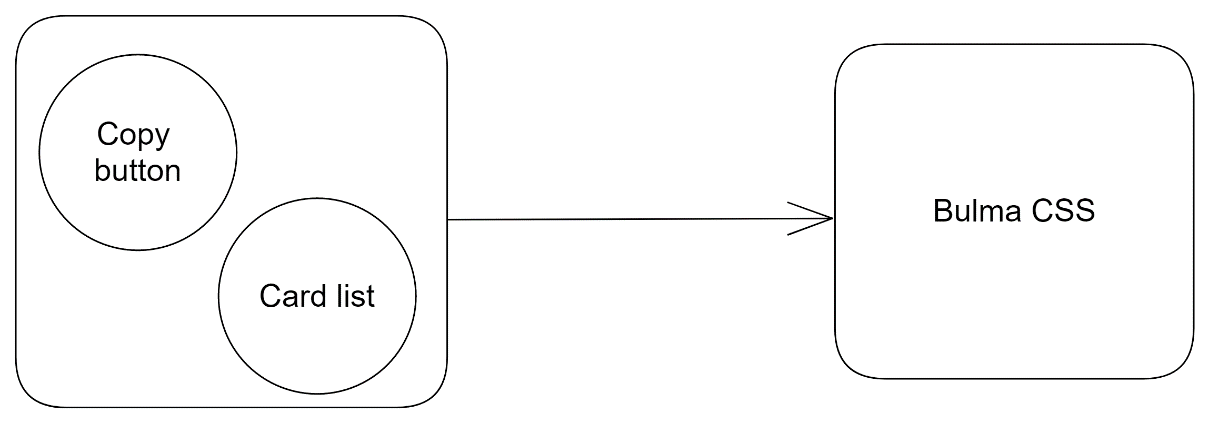
Build time: 1½ hours