The next collection type we will look at is a set. Sets differ from arrays in two important ways. The elements in a set are stored unordered, and each unique element is only held once. In this recipe, we will learn how to create and manipulate sets.
How to do it...
First, let's explore some ways we can create sets and perform set algebra on them:
- Create an array that contains the first nine Fibonacci numbers, and also a set containing the same:
let fibonacciArray: Array<Int> = [1, 1, 2, 3, 5, 8, 13, 21, 34]
let fibonacciSet: Set<Int> = [1, 1, 2, 3, 5, 8, 13, 21, 34]
print(fibonacciArray.count) // 9
print(fibonacciSet.count) // 8
- Print out the number of elements in each collection using the count property. Despite being created with the same elements, the count value is different:
print(fibonacciArray.count) // 9
print(fibonacciSet.count) // 8
- Insert an element into a set of animals, remove an element, and check whether a set contains a given element:
var animals: Set<String> = ["cat", "dog", "mouse", "elephant"]
animals.insert("rabbit")
print(animals.contains("dog")) // true
animals.remove("dog")
print(animals.contains("dog")) // false
- Create some sets containing common mathematical number groups. We will use these to explore some methods for set algebra:
let evenNumbers = Set<Int>(arrayLiteral: 2, 4, 6, 8, 10)
let oddNumbers: Set<Int> = [1, 3, 5, 7, 9]
let squareNumbers: Set<Int> = [1, 4, 9]
let triangularNumbers: Set<Int> = [1, 3, 6, 10]
- Obtain the union of two sets and print the result:
let evenOrTriangularNumbers = evenNumbers.union(triangularNumbers)
// 2, 4, 6, 8, 10, 1, 3, unordered
print(evenOrTriangularNumbers.count) // 7
- Obtain the intersection of two sets and print the result:
let oddAndSquareNumbers = oddNumbers.intersection(squareNumbers)
// 1, 9, unordered
print(oddAndSquareNumbers.count) // 2
- Obtain the symmetric difference of two sets and print the result:
let squareOrTriangularNotBoth =
squareNumbers.symmetricDifference(triangularNumbers)
// 4, 9, 3, 6, 10, unordered
print(squareOrTriangularNotBoth.count) // 5
- Obtain the result of subtracting one set from another and print the result:
let squareNotOdd = squareNumbers.subtracting(oddNumbers) // 4
print(squareNotOdd.count) // 1
Next, we will examine the set membership comparison methods that are available:
- Create some sets with overlapping membership:
let animalKingdom: Set<String> = ["dog", "cat", "pidgeon",
"chimpanzee", "snake", "kangaroo",
"giraffe", "elephant", "tiger",
"lion", "panther"]
let vertebrates: Set<String> = ["dog", "cat", "pidgeon",
"chimpanzee", "snake", "kangaroo",
"giraffe", "elephant", "tiger",
"lion", "panther"]
let reptile: Set<String> = ["snake"]
let mammals: Set<String> = ["dog", "cat", "chimpanzee",
"kangaroo", "giraffe", "elephant",
"tiger", "lion", "panther"]
let catFamily: Set<String> = ["cat", "tiger", "lion", "panther"]
let domesticAnimals: Set<String> = ["cat", "dog"]
- Use the isSubset method to determine whether one set is a subset of another. Then, print the result:
print(mammals.isSubset(of: animalKingdom)) // true
- Use the isSuperset method to determine whether one set is a superset of another. Then, print the result:
print(mammals.isSuperset(of: catFamily)) // true
- Use the isStrictSubset method to determine whether one set is a strict subset of another. Then, print the result:
print(vertebrates.isStrictSubset(of: animalKingdom)) // false
print(mammals.isStrictSubset(of: animalKingdom)) // true
- Use the isStrictSuperset method to determine whether one set is a strict superset of another. Then, print the result:
print(animalKingdom.isStrictSuperset(of: vertebrates)) // false
print(animalKingdom.isStrictSuperset(of: domesticAnimals)) // true
- Use the isDisjoint method to determine whether one set is disjointed with another. Then, print the result:
print(catFamily.isDisjoint(with: reptile)) // true
How it works...
Sets are created in almost the same way as arrays, and like arrays, we have to specify the element type that we will be stored in them:
let fibonacciArray: Array<Int> = [1, 1, 2, 3, 5, 8, 13, 21, 34]
let fibonacciSet: Set<Int> = [1, 1, 2, 3, 5, 8, 13, 21, 34]
Arrays and sets store their elements differently. If you provide multiple elements of the same value to an array, it will store them multiple times. A set works differently; it will only store one version of each unique element. Therefore, in the preceding Fibonacci number sequence, the array stores two elements for the first two values, 1, 1, but the set will store this as just one 1 element. This leads to the collections having different counts, despite being created with the same values:
print(fibonacciArray.count) // 9
print(fibonacciSet.count) // 8
This ability to store elements uniquely is made possible due to a requirement that a set has regarding the type of elements it can hold. A set's elements must conform to the Hashable protocol. This protocol requires a hashValue property to be provided as an Int, and the set uses this hashValue to do its uniqueness comparison. Both the Int and String types conform to Hashable, but any custom types that will be stored in a set will also need to conform to Hashable.
A set's insert, remove, and contains methods work as you would expect, with the compiler enforcing that the correct types are provided. This compiler type checking is done thanks to the generics constraints that all the collection types have. We will cover generics in more detail in Chapter 4, Generics, Operators, and Nested Types.
Union
The union method returns a set containing all the unique elements from the set that the method is called on, as well as the set that was provided as a parameter:
let evenOrTriangularNumbers = evenNumbers.union(triangularNumbers)
// 2,4,6,8,10,1,3,unordered
The following diagram depicts the Union of Set A and Set B:
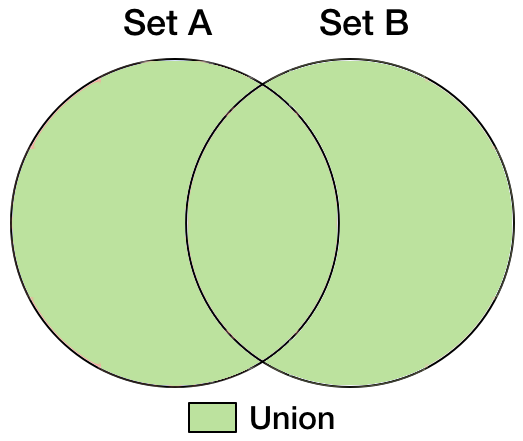
Intersection
The intersection method returns a set of unique elements that were contained in both the set that the method was called on and the set that was provided as a parameter:
let oddAndSquareNumbers = oddNumbers.intersection(squareNumbers)
// 1, 9, unordered
The following diagram depicts the Intersection of Set A and Set B:
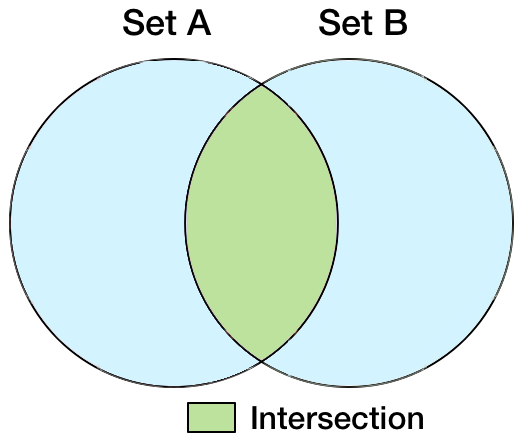
Symmetric difference
The symmetricDifference method returns a set of unique elements that are in either the set the method is called on, or the set that's provided as a parameter, but not elements that are in both:
let squareOrTriangularNotBoth =
squareNumbers.symmetricDifference(triangularNumbers)
// 4, 9, 3, 6, 10, unordered
The following diagram depicts the Symmetric Difference of Set A and Set B:
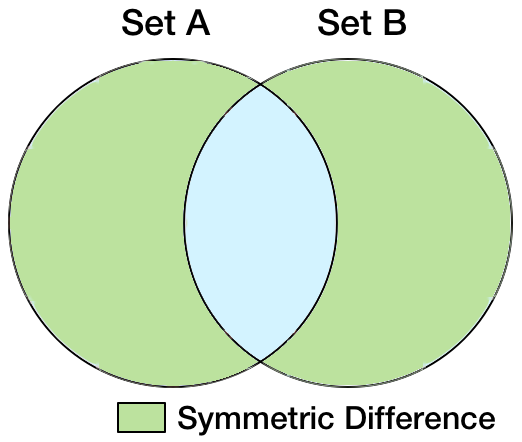
Subtracting
The subtracting method returns a unique set of elements that can be found in the set the method was called on, but not in the set that was passed as a parameter. Unlike the other set manipulation methods we've mentioned, this will not necessarily return the same value if you swap the set that the method is called on with the set provided as a parameter:
let squareNotOdd = squareNumbers.subtracting(oddNumbers) // 4
The following diagram depicts the set that's created by Subtracting Set B from Set A:
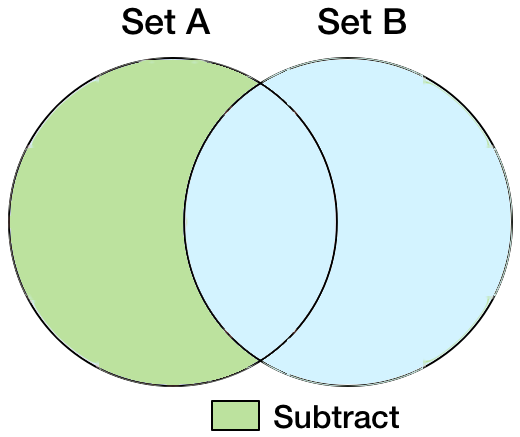
Membership comparison
In addition to set manipulation methods, there are a number of methods we can use to determine information about set membership.
The isSubset method will return true if all the elements in the set that the method is called on are contained within the set that's passed as a parameter:
print(mammals.isSubset(of: animalKingdom)) // true
The following diagram depicts Set B as the subset of Set A:
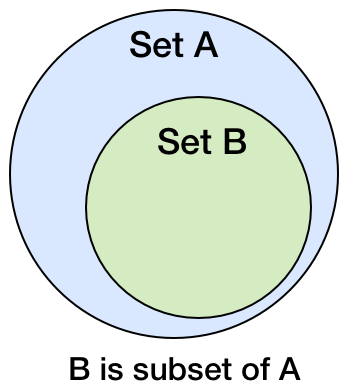
This will also return true if the two sets are equal (they contain the same elements). If you only want a true value if the set that the method is called on is a subset and not equal, then you can use isStrictSubset:
print(vertebrates.isStrictSubset(of: animalKingdom)) // false
print(mammals.isStrictSubset(of: animalKingdom)) // true
The isSuperset method will return true if all the elements in the set that have been passed as a parameter are within the set that the method is called on:
print(mammals.isSuperset(of: catFamily)) // true
The following diagram depicts Set A as the superset of Set B:
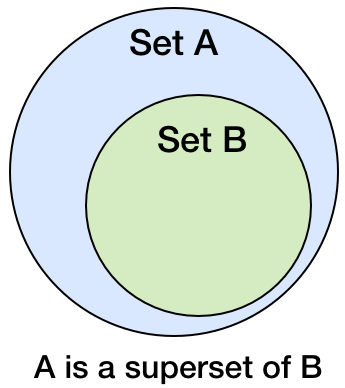
This will also return true if the two sets are equal (they contain the same elements). If you only want a true value if the set that the method is called on is a superset and not equal, then you can use isStrictSuperset:
print(animalKingdom.isStrictSuperset(of: vertebrates)) // false
print(animalKingdom.isStrictSuperset(of: domesticAnimals)) // true
The isDisjoint method will return true if there are no common elements between the set that the method is called on and the set that was passed as a parameter:
print(catFamily.isDisjoint(with: reptile)) // true
The following diagram shows that Set A and Set B are disjoint:
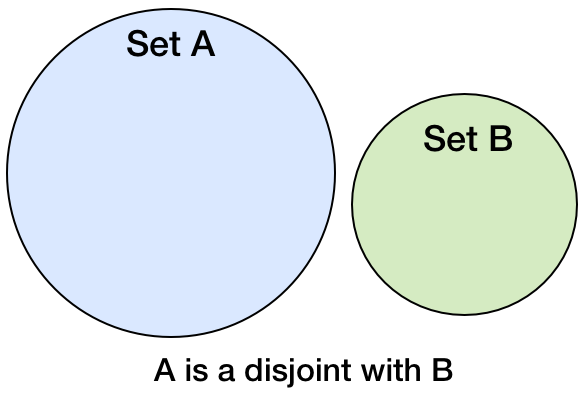
As with arrays, a set can be declared immutable by assigning it to a let constant instead of a var variable:
let planets: Set<String> = ["Mercury", "Venus", "Earth",
"Mars", "Jupiter", "Saturn",
"Uranus", "Neptune", "Pluto"]
planets.remove("Pluto") // Doesn't compile
This is because a set, like the other collection types, is a value type. Removing an element would mutate the set, which creates a new copy, but a let constant can't have a new value assigned to it, so the compiler prevents any mutating operations.
See also
Further information about arrays can be found in Apple's documentation on the Swift language at https://docs.swift.org/swift-book/LanguageGuide/CollectionTypes.html.
Sets use generics to define the element types they contain. Generics will be discussed in detail in Chapter 4, Generics, Operators, and Nested Types.