In this chapter, we will continue to explore JavaScript's syntax and constructs. We'll be delving into the fundamentals of expressions, statements, blocks, scopes, and closures. These are the less visible parts of the language. Most programmers assume that they already have a good grasp of how things such as expressions and scopes work, but, as we've seen, our intuitions of how things should work may not always align with how they truly do work. The constructs we'll be learning about in this chapter are the crucial larger building blocks of our programs, so it is of vital importance to understand them fully before we explore more abstract concepts such as control flow and design patterns.
You're reading from Clean Code in JavaScript
Expressions, statements, and blocks
There are broadly three types of syntactic container that exist within JavaScript: expressions, statements, and blocks. They are all containers in that they all hold other pieces of syntax and all have distinct behaviors that are worth distinguishing.
It's best to visualize the individual syntactic parts of a program as a hierarchy:
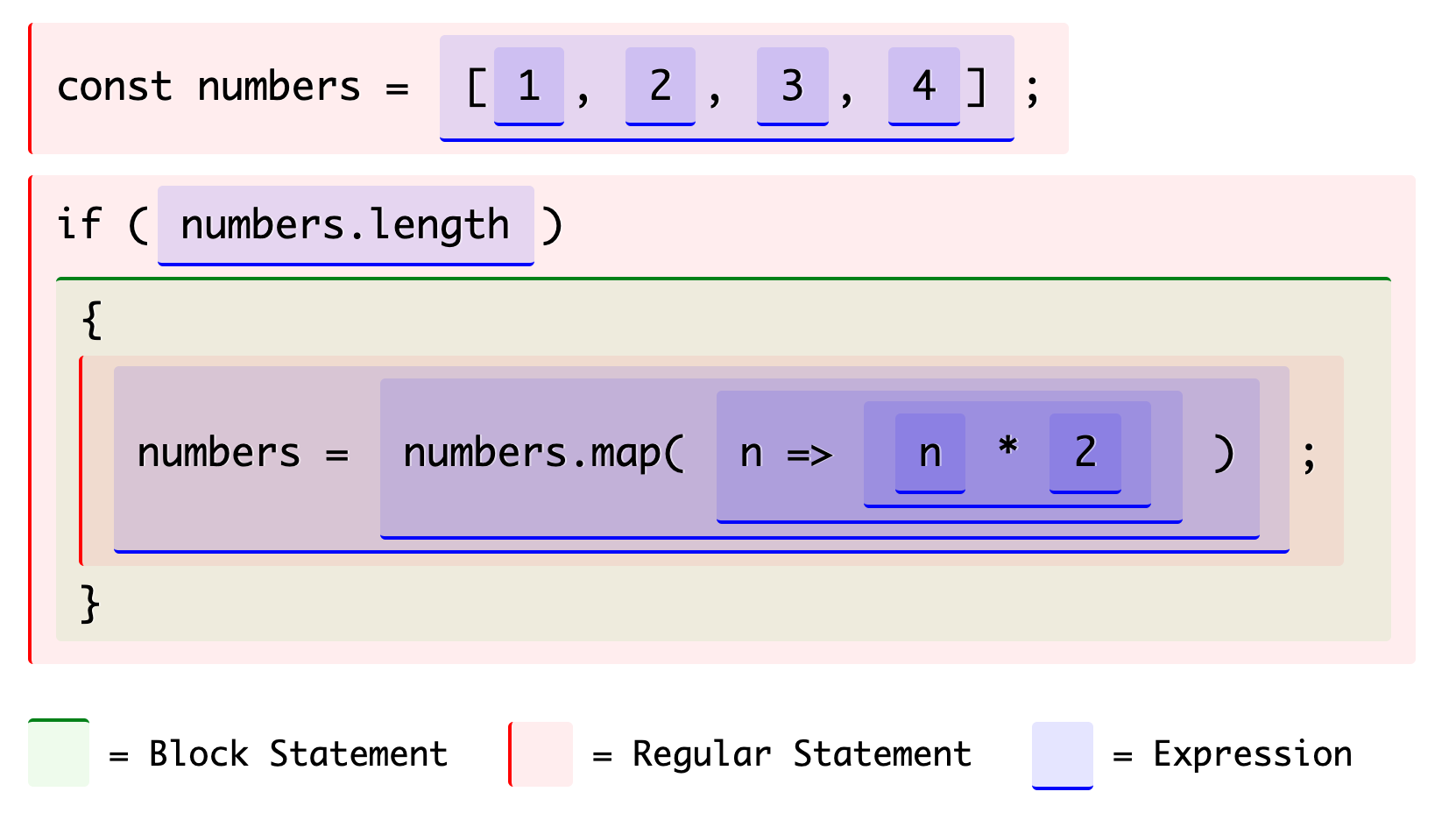
Here, we can see that individual expressions (with a lower border) are wrapped in statements, either of the regular or block...
Scopes and declarations
The scope of a given variable can be thought of as the areas within the program where that variable can be accessed.
When we declare a variable at the beginning of a module (outside all functions), we think that it's only natural that this variable should then be accessible to all functions within the module:
var hello = 'hi';
function a() {
hello; // a() can "see" the hello variable
}
function b() {
hello; // b() can "see" the hello variable
}
And if we define a variable within a function, then we expect all inner functions to have access to it:
var value = 'I exist';
function doSomething() {
value; // => "I exist"
}
The fact that we can access value in the doSomething function here is thanks to its scope. The scope of a given variable will depend on how it is declared. When you declare a variable...
Summary
In this chapter, we continued to explore the JavaScript language, zooming out from previous chapters to consider larger pieces of syntax, such as expressions, statements, and blocks. These are programmatic scaffolding components in which we can place the types and operations we've previously learned about. We also covered the complicated mechanisms of scopes, hoisting, and closures. Understanding how these concepts all work together is vital to understanding other people's JavaScript programs and constructing your own.
In the next chapter, we explore how to control flow within JavaScript. This'll allow us to weave together expressions and statements into larger bodies of logic in a clean way. We will then explore the art of abstraction design by learning about design patterns. Though the process of learning these topics individually may appear arduous, by...