In the previous chapter on dynamic typing, we explored topics such as type-coercion and detection; we also covered several operators. In this chapter, we'll continue this exploration by delving into every single operator that the JavaScript language makes available. Having a rich understanding of JavaScript's operators will make us feel utterly empowered in a language that can, at times, appear confusing. There is, unfortunately, no shortcut to understanding JavaScript, but as you begin to explore its operators, you will see patterns emerge. For example, many of the multiplicative operators work in a similar manner, as do the logical operators. Once you are comfortable with the main operators, you will begin to see that there is a grace underlying the complexity.
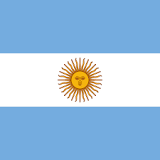
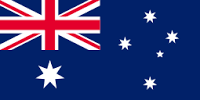
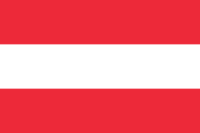
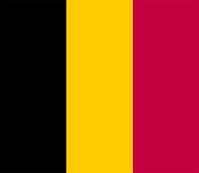
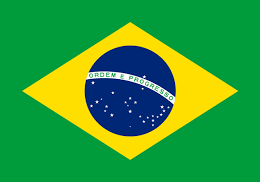
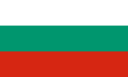
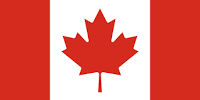
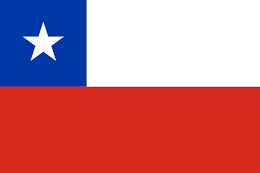
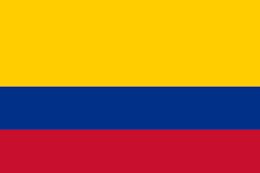
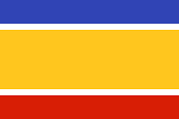
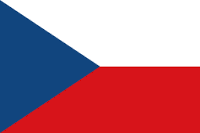
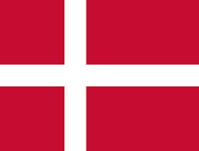
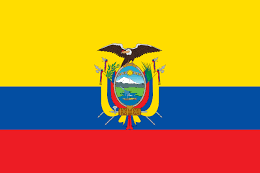
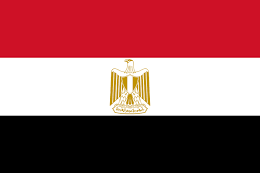
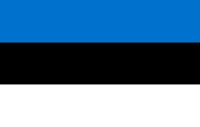
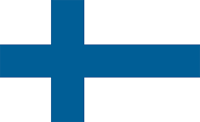
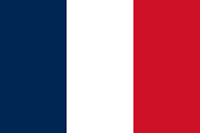
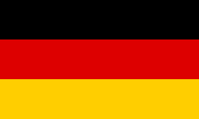
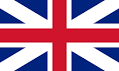
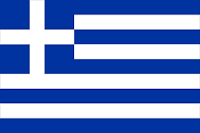
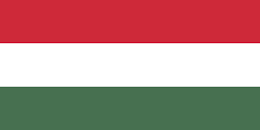
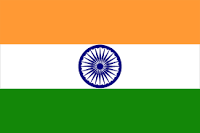
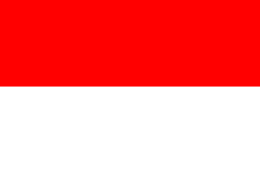
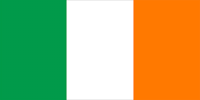
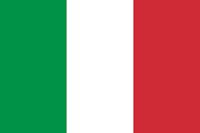
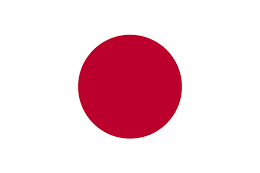
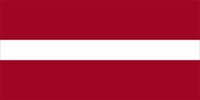
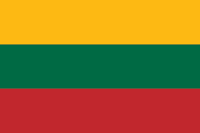
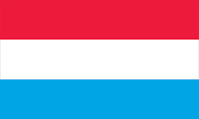
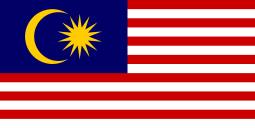
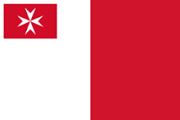
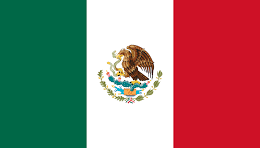
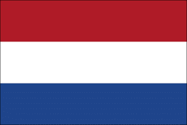
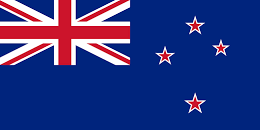
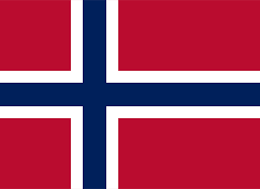
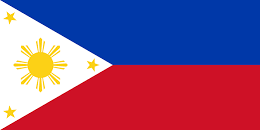
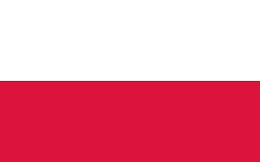
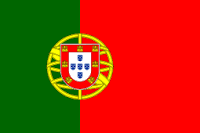
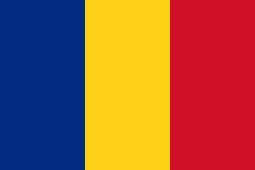
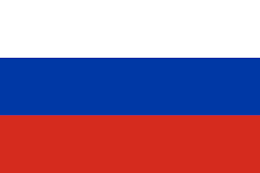

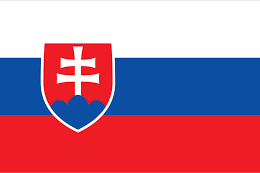
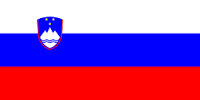
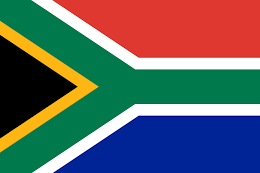
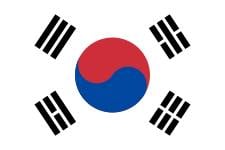
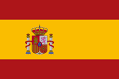
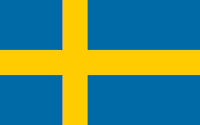
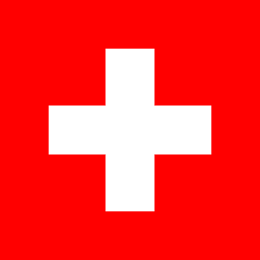
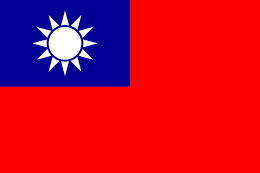
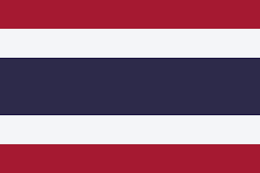
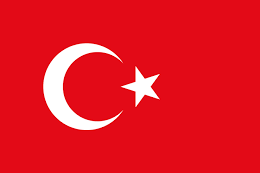
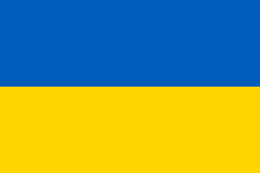
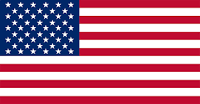