In this chapter, we will put together many pieces of the object-oriented puzzle. We will take advantage of extensions to add features to classes, protocols, and types that we can't modify through source code editing. We will interact with a simple object-oriented data repository through Picker View and consider how object-oriented code is everywhere in an iOS app.
You're reading from Object???Oriented Programming with Swift 2
In Chapter 1, Objects from the Real World to Playground, you learned how to recognize objects from real-life situations. We understood that working with objects makes it easier to write code that is easier to understand and reuse. You learned how to recognize real-world elements and translate them into the different components of the object-oriented paradigm supported in Swift: classes, protocols, properties, methods, and instances.
We discussed that classes represent blueprints or templates to generate the objects, which are also known as instances. We designed a few classes with properties and methods that represent blueprints for real-life objects. Then, we improved the initial design by taking advantage of the power of abstraction and specialized different classes.
In Chapter 2, Structures, Classes, and Instances, you learned about an object's life cycle. We worked with many examples to understand how object initializers and...
Sometimes, we would like to add methods to an existing class. We already know how to do this; we just need to go to its Swift source file and add a new method within the class body. However, sometimes, we cannot access the source code for the class, or it isn't convenient to make changes to it. A typical example of this situation is a class, struct, or any other type that is part of the standard language elements. For example, we might want to add a method that we can call in any Int
value to initialize either a 2D or 3D point with all its elements set to the Int
value.
The following lines declare a simple Point2D
class that represents a mutable 2D point with the x
and y
elements. The class conforms to the CustomStringConvertible
protocol; therefore, it declares a description computed property that returns a string representation for the 2D point:
public class Point2D: CustomStringConvertible { public var x: Int public var y: Int public var valuesAsDescription...
Swift allows us to add both computed instance properties and computed type properties to an existing type. These are the only types of properties that we can add to an existing type, so we cannot add simpler stored properties using extensions.
When you need to perform calculations with values that have an associated unit of measurement, it is very common to make mistakes by mixing different units of measurement. It is also common to perform incorrect conversions between the different units that generate wrong results. Swift doesn't allow us to associate a specific numerical value with a unit of measurement. However, we can add computed properties to provide some information about the units of measurement for a specific domain.
Tip
We worked with units when we analyzed the object-oriented approach of the HealthKit framework in Chapter 1, Objects from the Real World to Playground. However, in this case, we just want to simplify a sum operation...
So far, we always worked with one specific type of initializer for all the classes: designated initializers. These are the primary initializers for a class in Swift, and they make sure that all the properties are initialized. In fact, every class must have at least one designated initializer. However, it is important to note that a class can satisfy this requirement by inheriting a designated initializer from its superclass.
There is another type of initializer known as convenience initializer that acts as a secondary initializer and always ends up calling a designated initializer. Convenience initializers are optional, so any class can declare one or more convenience initializers to provide initializers that cover specific use cases or more convenient shortcuts to create instances of a class.
Now, imagine that we cannot access the code for the previously declared Point3D
class. We are working on an app, and we discover too many use cases...
Let's consider that we still cannot access the code for the previously declared Point3D
class. We are working on an app, and we discover that it would be nice to access the x
, y
, and z
values of a Point3D
instance with [0]
, [1]
, and [2]
. We can easily add a subscript by extending the Point3D
class.
The following lines use the extension
keyword to a subscript to the existing Point3D
class:
extension Point3D { public subscript(index: Int) -> Int? { switch index { case 0: return x case 1: return y case 2: return z default: return nil } } }
The following lines use the recently added subscript to access the elements of a Point3D
instance:
var point3D7 = Point3D(x: 10, y: 15, z: 4) if let point3D7X = point3D7[0] { print("X or [0]: \(point3D7X)") } if let point3D7Y = point3D7[1] { print("Y or [1]: \(point3D7Y)") } if let point3D7Z = point3D7[2] { print("Z or [2]: \(point3D7Z)") }
The following...
So far, we created and extended classes in the Playground. In fact, we could execute the same sample code in the Swift REPL. The Swift REPL is a read-eval-print loop, also known as an interactive language shell, where we can enter expressions or pieces of code, make Swift evaluate them, and prints the results.
Now, we will create a simple iOS app based on the Single View Application template with Xcode. We will recognize the usage of object-oriented code included in the template—that is, before we add components and code to the app. Then, we will take advantage of the GameRepository
class we created in the previous chapter and use it to populate a UI element.
Navigate to File | New | Project… in Xcode. Then, navigate to iOS | Application on the left-hand side of the Choose a template for your new project dialog box. Select Single View Application on the right-hand side and click on Next, as shown in the following screenshot:
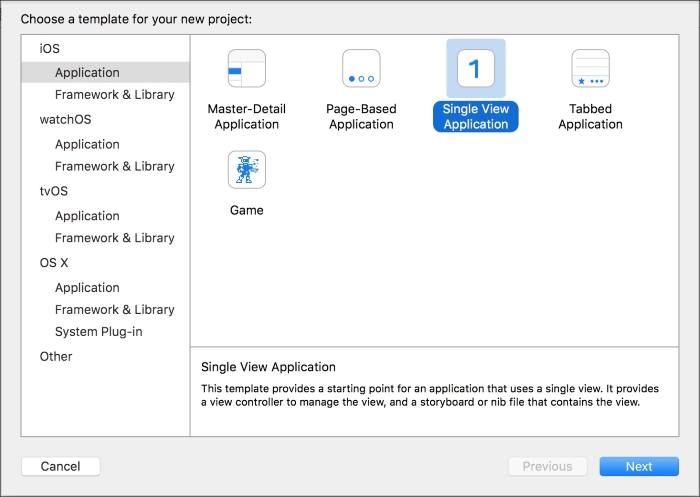
Now, we will add one protocol and many classes we created in the previous chapter to generate the GameRepository
class. We want to display a list of game names in the Picker View. We will add the following Swift source files in the project within the Chapter8
group:
EntityProtocol.swift
Entity.swift
Repository.swift
Game.swift
GameRepository.swift
Click on the Chapter8 group in Project Navigator (the icon represents a folder). Do not confuse it with the Chapter8 project that is the parent for the Chapter8 group. Navigate to File | New | File… and select Swift File as the template for your new file. Then, click on Next and enter EntityProtocol in the Save As textbox. Make sure that Chapter8 with the folder icon is selected in the Group drop-down menu, as shown in the next screenshot, and then click on Create. Swift will add the new EntityProtocol.swift
source file to the Chapter8 group within the Chapter8 project:
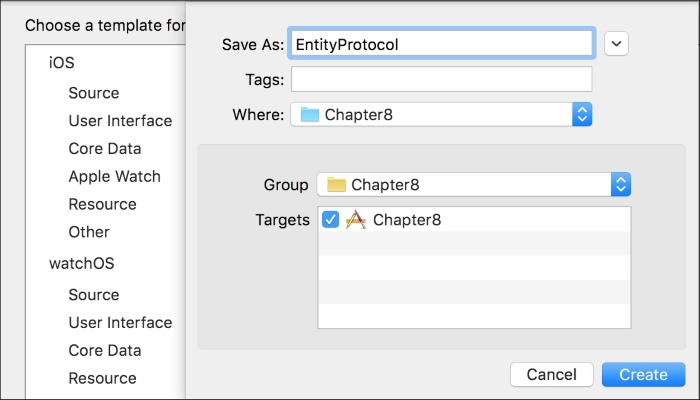
Add the following...
Now, we have to add code to the ViewController
class in the ViewController.swift
source file to make the class conform to two additional protocols: UIPickerViewDataSource
and UIPickerViewDelegate
. The conformance to the UIPickerViewDataSource
protocol allows us to use the class as a data source for the UIPickerView
class that represents the Picker View component. The conformance to the UIPickerViewDelegate
protocol allows us to handle the events raised by the UIPickerView
class.
The following lines show the new code for the ViewController
class:
class ViewController: UIViewController, UIPickerViewDelegate, UIPickerViewDataSource { @IBOutlet weak var picker: UIPickerView! private var gamesList: [Game] = [Game]() override func viewDidLoad() { super.viewDidLoad() // Do any additional setup after loading the view, typically from a nib. picker.delegate = self picker.dataSource...
Use the recently created iOS app as the baseline and extend it to provide the following features:
Add a text box to allow the user to enter the text that the game names must match in order to be displayed as an option in View Picker
After the user selects a game in View Picker, display a new view that shows the highest score and the played count for the chosen game
We can add the following type of initializers to a class with extensions:
Convenience initializers.
Designated initializers.
Primary initializers.
We can add the following type of properties to a class with extensions:
Read/write stored type properties.
Primary properties.
Computed instance properties and computed type properties.
Convenience initializers are:
Optional.
Required.
Required only in superclasses.
A convenience initializer acts as:
A required initializer that doesn't need to call any other initializer.
A secondary initializer that doesn't need to call any other initializer.
A secondary initializer that always ends up calling a designated initializer.
If we declare the type for a property as
UIPickerView!
, Swift will treat the property as:An implicitly wrapped optional.
An implicitly unwrapped optional.
An exact equivalent of
UIPickerView?
.
In this chapter, you learned how to add methods, computed properties, convenience initializers, and scripts using extensions and without editing the original source code for the original classes or types. Then, we analyzed the initial object-oriented code in the Single View Application template for an iOS app.
We added a simple UI element to the template and then we added classes that we tested in the Swift Playground in the previous chapter. We interacted with a simple object-oriented data repository through Picker View and discussed how object-oriented code is everywhere in an iOS app.
Now that you have learned to write object-oriented code in Swift, you are ready to use everything you learned in real-life applications that will not only rock, but also maximize code reuse and simplify maintenance.