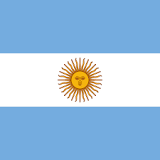
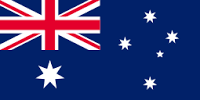
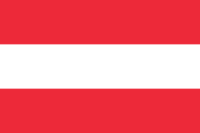
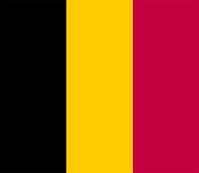
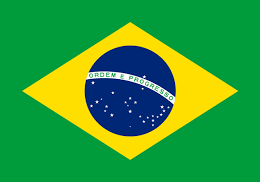
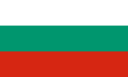
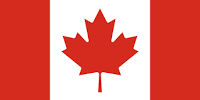
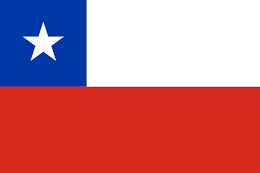
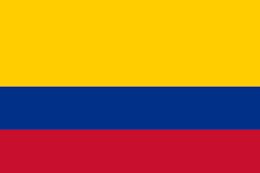
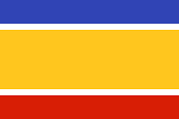
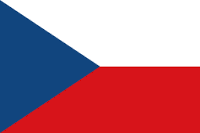
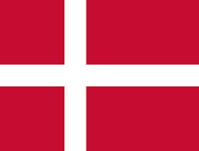
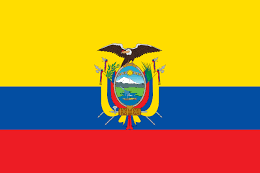
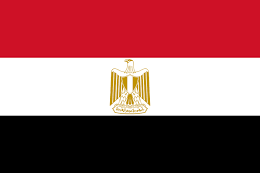
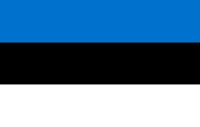
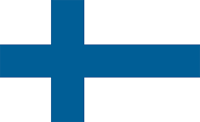
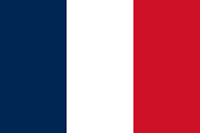
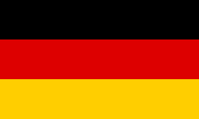
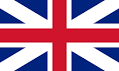
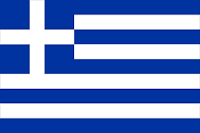
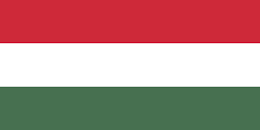
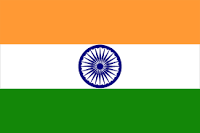
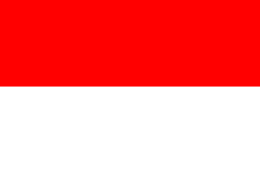
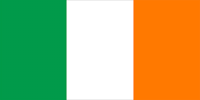
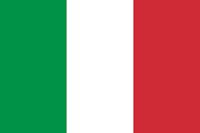
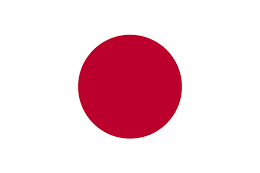
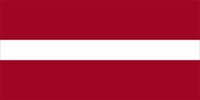
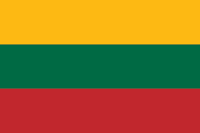
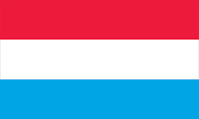
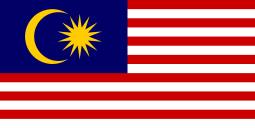
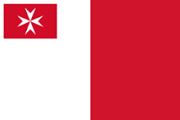
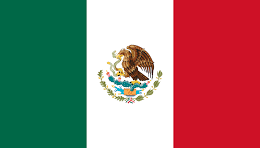
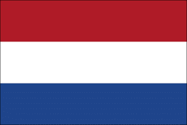
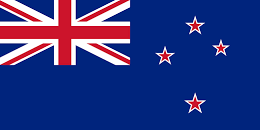
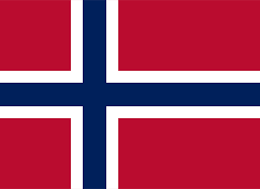
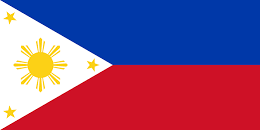
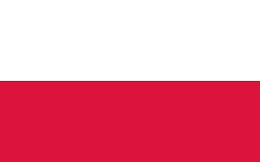
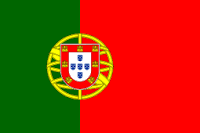
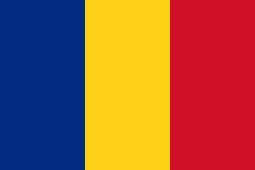
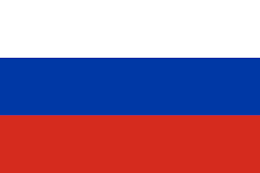

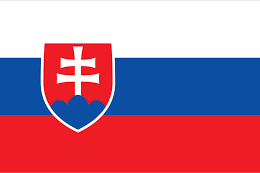
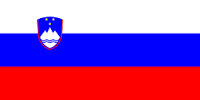
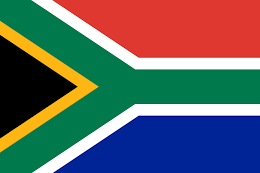
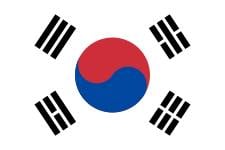
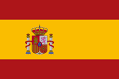
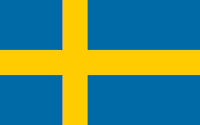
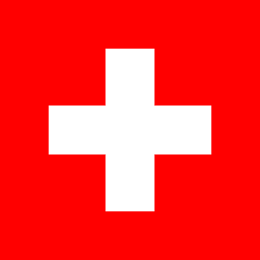
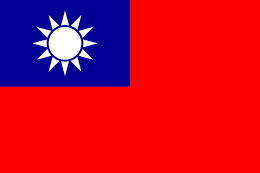
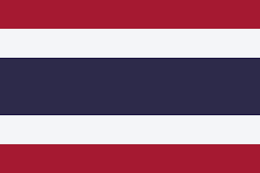
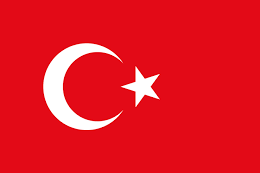
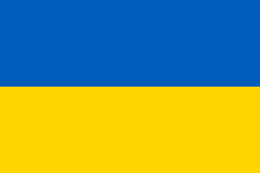
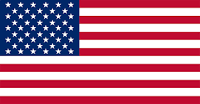
A list is, as the name hints, a list of objects of any kind:
L = ['a' 20.0, 5] M = [3,['a', -3.0, 5]]
The individual objects are enumerated by assigning each element an index. The first element in the list gets index 0. This zero-based indexing is frequently used in mathematical notation. Consider the usual indexing of coefficients of a polynomial.
The index allows us to access the following objects:
L[1] # returns 20.0 L[0] # returns 'a' M[1] # returns ['a',-3.0,5] M[1][2] # returns 5
The bracket notation here corresponds to the use of subscripts in mathematical formulas. L
is a simple list, while M
itself contains a list so that one needs two indexes to access an element of the inner list.
A list containing subsequent integers can easily be generated by the command range
:
L=list(range(4)) # generates a list with four elements: [0, 1, 2 ,3]
A more general use is to provide this command with start, stop, and step parameters:
L=list(range(17,29,4)) # generates [17, 21, 25...
The NumPy package offers arrays, which are container structures for manipulating vectors, matrices, or even higher order tensors in mathematics. In this section, we point out the similarities between arrays and lists. But arrays deserve a broader presentation, which will be given in Chapter 4, Linear Algebra – Arrays, and Chapter 5, Advanced Array Concepts.
Arrays are constructed from lists by the function array
:
v = array([1.,2.,3.]) A = array([[1.,2.,3.],[4.,5.,6.]])
To access an element of a vector, we need one index, while an element of a matrix is addressed by two indexes:
v[2] # returns 3.0 A[1,2] # returns 6.0
At first glance, arrays are similar to lists, but be aware that they are different in a fundamental way, which can be explained by the following points:
M = array([[1.,2.],[3.,4.]]) v = array([1., 2., 3.]) ...
A tuple is an immutable list. Immutable means that it cannot be modified. A tuple is just a comma-separated sequence of objects (a list without brackets). To increase readability, one often encloses a tuple in a pair of parentheses:
my_tuple = 1, 2, 3 # our first tuple my_tuple = (1, 2, 3) # the same my_tuple = 1, 2, 3, # again the same len(my_tuple) # 3, same as for lists my_tuple[0] = 'a' # error! tuples are immutable
The comma indicates that the object is a tuple:
singleton = 1, # note the comma len(singleton) # 1
Tuples are useful when a group of values goes together; for example, they are used to return multiple values from functions (refer to section Returns Values in Chapter 7, Functions. One may assign several variables at once by unpacking a list or tuple:
a, b = 0, 1 # a gets 0 and b gets 1 a, b = [0, 1] # exactly the same effect (a, b) = 0, 1 # same [a,b] = [0,1] # same thing
Lists, tuples, and arrays are ordered sets of objects. The individual objects are inserted, accessed, and processed according to their place in the list. On the other hand, dictionaries are unordered sets of pairs. One accesses dictionary data by keys.
For example, we may create a dictionary containing the data of a rigid body in mechanics, as follows:
truck_wheel = {'name':'wheel','mass':5.7, 'Ix':20.0,'Iy':1.,'Iz':17., 'center of mass':[0.,0.,0.]}
A key/data pair is indicated by a colon, :
. These pairs are comma separated and listed inside a pair of curly brackets, {}
.
Individual elements are accessed by their keys:
truck_wheel['name'] # returns 'wheel' truck_wheel['mass'] # returns 5.7
New objects are added to the dictionary by creating a new key:
truck_wheel['Ixy'] = 0.0
Dictionaries are also used to provide parameters to a function (refer to section Parameters and arguments in Chapter 7, Functions...
Sets are containers that share properties and operations with sets in mathematics. A mathematical set is a collection of distinct objects. Here are some mathematical set expressions:
And their Python counterparts:
A = {1,2,3,4} B = {5} C = A.union(B) # returns set([1,2,3,4,5]) D = A.intersection(C) # returns set([1,2,3,4]) E = C.difference(A) # returns set([5]) 5 in C # returns True
Sets contain an element only once, corresponding to the aforementioned definition:
A = {1,2,3,3,3} B = {1,2,3} A == B # returns True
And a set is unordered; that is, the order of the elements in the set is not defined:
A = {1,2,3} B = {1,3,2} A == B # returns True
Sets in Python can contain all kinds of hashable objects, that is, numeric objects, strings, and Booleans.
There are union
and intersection
methods:
A={1,2,3,4} A.union({5}) A.intersection({2,4,6}) # returns set([2, 4])
Also, sets can be compared using the methods issubset
and issuperset
:
...
We summarize in the following Table 3.2 the most important properties of the container types presented so far. Arrays will be treated in Chapter 4, Linear Algebra – Arrays.
Table 3.2 : Container Types
As you can see in the previous table, there is a difference in accessing container elements, and sets and dictionaries are not ordered.
Due to the different properties of the various container types, we frequently convert one type to another:
The direct way to see the type of a variable is to use the type
command:
label = 'local error' type(label) # returns str x = [1, 2] # list type(x) # returns list
However, if you want to test for a variable to be of a certain type, you should use isinstance
(instead of comparing the types with type
):
isinstance(x, list) # True
The reason for using isinstance
becomes apparent after having read Chapter 8, Classes, and in particular the concept of subclassing and inheritance in section Subclassing and Inheritance in Chapter 8, Classes. In short, often different types share some common properties with some basic type. The classical example is the type bool
, which is derived by subclassing from the more general type int
. In this situation, we see how the command isinstance
can be used in a more general way:
test = True isinstance(test, bool) # True isinstance(test, int) # True type(test) == int # False type(test) == bool # True
So, in order to make sure...
In this chapter, you learned to work with container types, mainly lists. It is important to know how to fill these containers and how to access their content. We saw that there is access by position or by keyword.
We will meet the important concept of slicing again in the next chapter on arrays. These are containers specially designed for mathematical operations.
Ex. 1 → Execute the following statements:
L = [1, 2] L3 = 3*L
L3
?L3[0] L3[-1] L3[10]
L4 = [k**2 for k in L3]
L3
and L4
to a new list L5
.
Ex. 2 → Use the range
command and a list comprehension to generate a list with 100 equidistantly spaced values between 0 and 1.
Ex. 3 → Assume that the following signal is stored in a list:
L = [0,1,2,1,0,-1,-2,-1,0]
What is the outcome of:
L[0] L[-1] L[:-1] L + L[1:-1] + L L[2:2] = [-3] L[3:4] = [] L[2:5] = [-5]
Do this exercise by inspection only, that is, without using your Python Shell.
Ex. 4 → Consider the Python statements:
L = [n-m/2 for n in range(m)] ans = 1 + L[0] + L[-1]
and assume that the variable m
has been previously assigned an integer value. What is the value of ans
? Answer this question without executing the statements in Python...