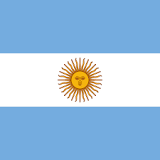
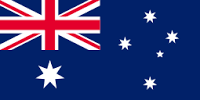
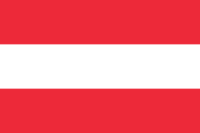
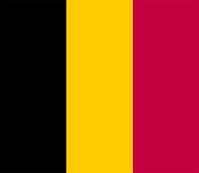
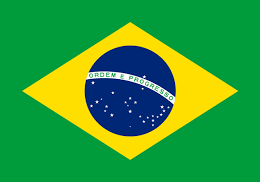
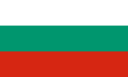
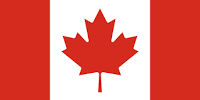
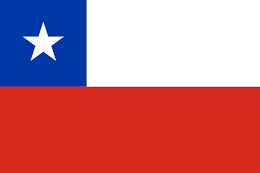
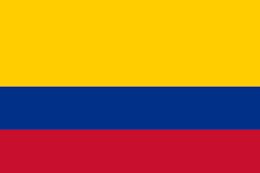
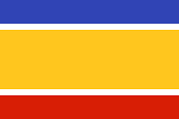
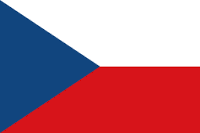
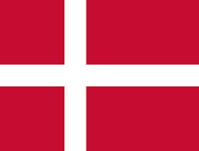
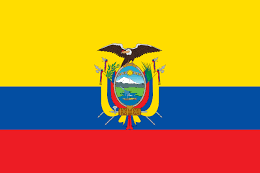
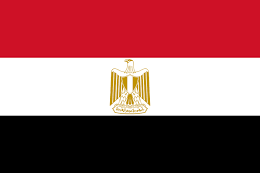
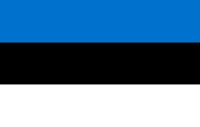
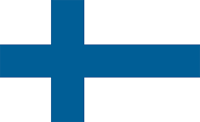
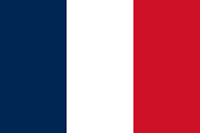
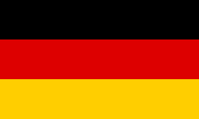
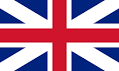
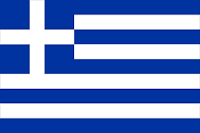
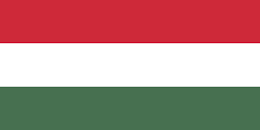
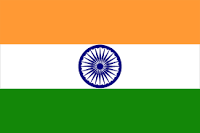
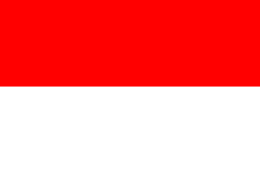
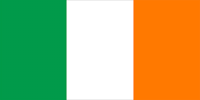
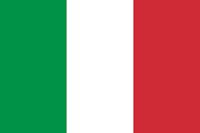
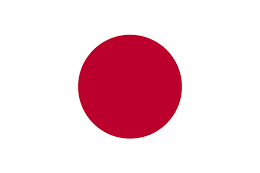
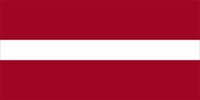
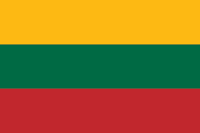
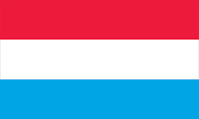
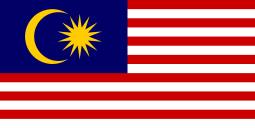
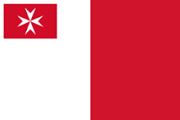
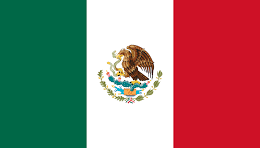
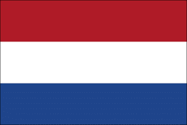
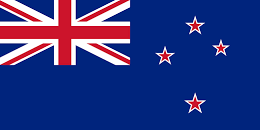
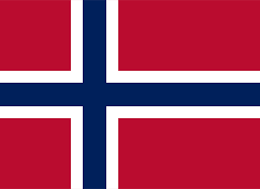
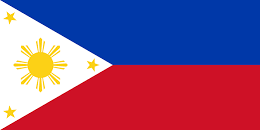
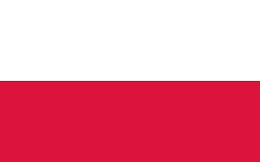
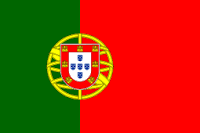
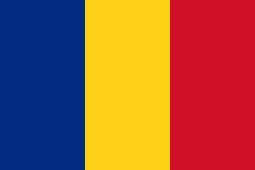
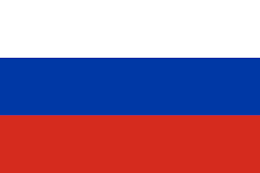

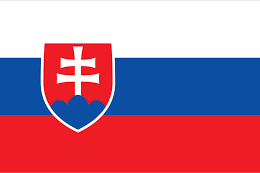
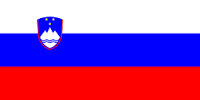
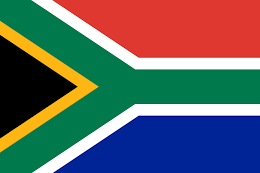
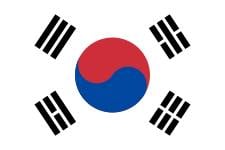
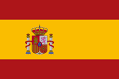
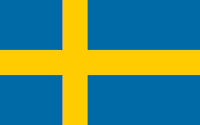
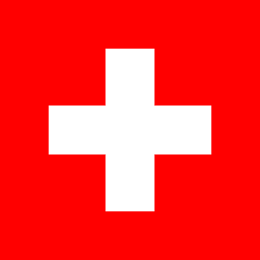
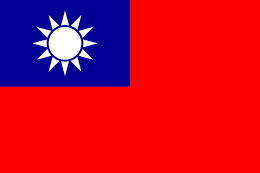
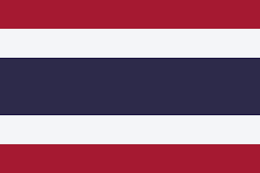
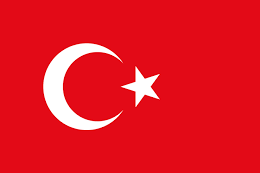
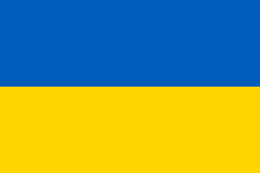
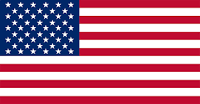
Solution:
Products
table with the same column names that were provided in the Excel spreadsheet:use packt_online_shop; CREATE TABLE Products ( ProductID INT NOT NULL, ProductCategoryID INT NOT NULL, SupplierID INT NOT NULL, ProductName CHAR(50) NOT NULL, NetRetailPrice DECIMAL(10, 2) NULL, AvailableQuantity INT NOT NULL, WholesalePrice DECIMAL(10, 2) NOT NULL, UnitKGWeight DECIMAL(10, 5) NULL, Notes VARCHAR(750) NULL, PRIMARY KEY (ProductID) );
Products
table:INSERT INTO Products ( ProductID, ProductCategoryID, SupplierID, ProductName, NetRetailPrice, AvailableQuantity, WholesalePrice, UnitKGWeight, Notes ) VALUES...
Solution:
FoodProducts
table with default values:CREATE TABLE FoodProducts ( ProductID INT NOT NULL AUTO_INCREMENT, ProductCategoryID INT NOT NULL DEFAULT 1, SupplierID INT NOT NULL DEFAULT 1, ProductName CHAR(50) NOT NULL, NetRetailPrice DECIMAL(10, 2) NULL DEFAULT 5.99, AvailableQuantity INT NOT NULL, WholesalePrice DECIMAL(10, 2) NOT NULL DEFAULT 3.99, UnitKGWeight DECIMAL(10, 5) NULL, Notes VARCHAR(750) NULL, PRIMARY KEY (ProductID) );
insert into FoodProducts ( ProductName, AvailableQuantity, UnitKGWeight, Notes ) values ('Pancake batter', 50, 0.25, 'Contains eggs'), ('Breakfast cereal', 10, 0.25, 'Add milk'), ('Siracha sauce', 10, 0.25, 'Spicey');
select * from foodProducts;
Your output should be as follows:
Figure 2.14: Populated Products...
Solution:
OrderItems
table:Use packt_online_shop; CREATE TABLE OrderItems ( OrderItemID INT NOT NULL AUTO_INCREMENT, OrderID INT NOT NULL, ProductID INT NOT NULL, Quantity INT NOT NULL, UnitPrice DECIMAL(10, 2) NOT NULL, Discount DECIMAL(10, 2) NULL, Notes VARCHAR(750) NULL, PRIMARY KEY (OrderItemID) );
Orders
(child) table:Create Table Orders( OrderID INT NOT NULL AUTO_INCREMENT, CustomerID INT NOT NULL, OrderNumber CHAR(50) NOT NULL, OrderDate DATETIME NOT NULL, ShipmentDate DATETIME NULL, OrderStatus CHAR(10) NULL...
Solution:
New Query
window, switch to the PACKT_ONLINE_SHOP
database:USE PACKT_ONLINE_SHOP
SELECT FirstName as 'First Name', LastName as 'Last Name', Phone as 'Phone Number' FROM Customers
Figure 4.21: Columns from the Customers table
The query will list all the rows of the FirstName
, LastName
, Phone
columns renamed as First Name
, Last Name
, and Phone Number
.
Solution:
SELECT Products.ProductName as 'Product Name', Products.NetRetailPrice as 'Product Retail Price', Products.AvailableQuantity as &apos...
Solution:
SELECT
statement. This is used so as to display the results using the requested column names:SELECT ProductName as 'Product Name', NetRetailPrice as 'Product Retail Price', AvailableQuantity as 'Available Quantity' From Products WHERE NetRetailPrice <= 24.99 AND AvailableQuantity >=38
Figure 5.19: Columns from the Customers table
Solution:
Orders
, OrderItems
, and Products
.In cases like these, we'll have to perform multiple joins, with the first join being between the Orders
and OrderItems
table to get price details, and the second join being between the OrderItems
and Products
table to get the product information.
SELECT Orders.OrderNumber, OrderItems.UnitPrice, OrderItems.Quantity, Products.ProductName FROM Orders JOIN OrderItems ON Orders.OrderID = OrderItems.OrderID JOIN Products ON OrderItems.ProductID = Products.ProductID
In this query, we joined the Orders
and OrderItems
tables using the OrderID
and joined the Products
and OrderItems
tables using...
Solution:
USE packt_online_shop; SELECT PC.ProductCategoryName FROM ProductCategories PC WHERE ProductCategoryID IN (SELECT ProductCategoryID FROM Products WHERE ProductName = 'habanero peppers');
Figure 7.10: Category of the habanero peppers food item
Solution:
USE packt_online_shop; SELECT OrderNumber, ShipmentDate, CASE WHEN ShipmentDate < ' 2010-12-10' THEN 'Past Shipment Date' WHEN ShipmentDate >= ' 2010-12-10' AND ShipmentDate < ' 2019-12-18' THEN 'Recent Shipment Date' ELSE 'Future Shipment Date' END AS 'Shipment Date Category' FROM Orders;
Solution:
CREATE DEFINER=`root`@`localhost` PROCEDURE `spFilterOrdersByItemQuantity`(IN orderItemQuantityVal int) BEGIN SELECT OI.OrderID, SUM(OI.Quantity) AS 'Total Order Item Quantity' FROM OrderItems OI GROUP BY OI.OrderID HAVING SUM(OI.Quantity) <= orderItemQuantityVal ORDER BY OI.OrderID; END
USE packt_online_shop; CALL spFilterOrdersByItemQuantity(25);
Solution:
CREATE DEFINER =`root`@`localhost` FUNCTION `fnProductTotalOrderQty`(ProductID INT) RETURNS INT DETERMINISTIC BEGIN DECLARE retVal INT; SET retVal = ( SELECT CASE WHEN SUM(OI.quantity) IS NULL THEN 0 ELSE SUM(OI.quantity) END AS 'quantity' FROM OrderItems...
Solution:
TEMP_ACCOUNT_2
using the following code: USE packt_online_shop; GRANT UPDATE ON TABLE packt_online_shop.products TO 'TEMP_ACCOUNT_2';
SHOW GRANTS FOR 'TEMP_ACCOUNT_2';
Figure 9.6: All permissions provided to TEMP_ACCOUNT_2
SELECT OI.OrderID, P.ProductName, SUM(OI.Quantity) AS 'COUNT OF EACH SEPARATE PRODUCT IN THE ORDER', SUM(SUM(OI.Quantity)) OVER(PARTITION BY OI.OrderID) AS 'TOTAL COUNT OF PRODUCTS IN THE ORDER', MAX(MAX(OI.Quantity)) OVER(PARTITION BY OI.OrderID) AS 'COUNT OF THE PRODUCT WITH THE HIGHEST ORDER COUNT IN THE ORDER' FROM OrderItems OI INNER JOIN Products P ON OI.ProductID = P.ProductID GROUP BY OI.OrderID, P.ProductName ORDER BY OI.OrderID;
Solution:
SELECT O.OrderID, O.CustomerID, O.OrderNumber, O.OrderDate, O.ShipmentDate, O.OrderStatus, O.Notes FROM Orders O WHERE O.Notes LIKE '%CUST%' AND O.ShipmentDate > '01051995'
We have set two filter conditions. Only when both are satisfied will the product be displayed.
Figure 11.22: Customer notes post May 5, 1995
Notice that there are only three orders that have an order date post May 1, 1995
and have CUST
as part of the Notes
section.
Solution:
OrderItems
rows:USE PACKT_ONLINE_SHOP; SELECT OrderItemID, OrderID, ProductID, Quantity, UnitPrice, Discount, Notes FROM OrderItems WHERE OrderID = 5;
The output...
Now that you've mastered the fundamentals of SQL, continue to build your knowledge and advance your career with one of our other Workshops…
The Applied SQL Data Analytics Workshop
|
|
...