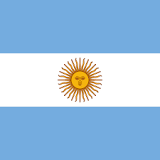
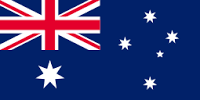
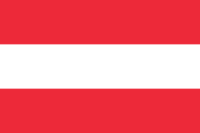
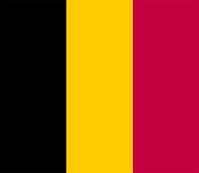
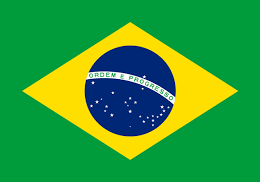
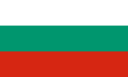
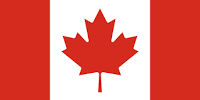
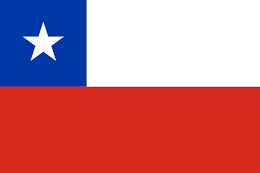
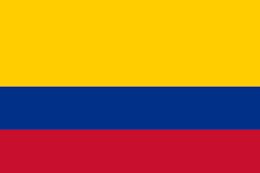
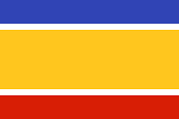
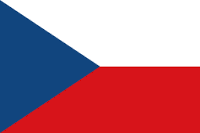
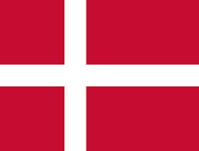
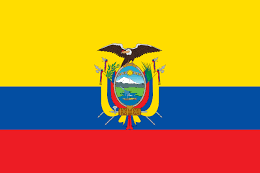
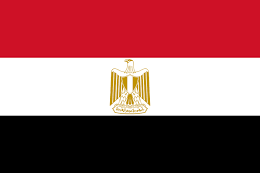
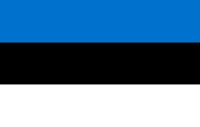
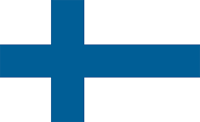
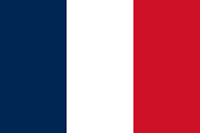
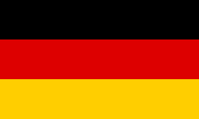
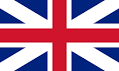
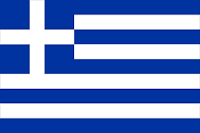
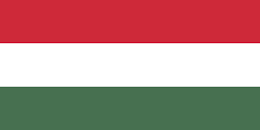
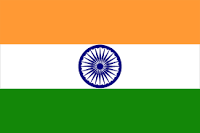
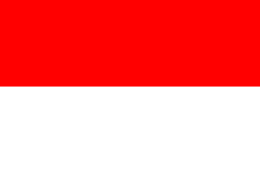
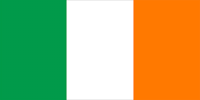
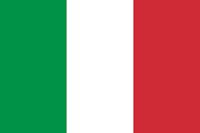
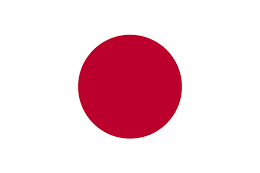
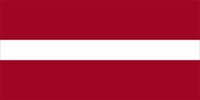
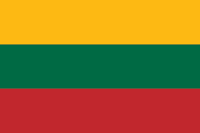
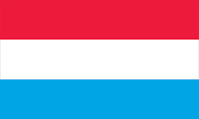
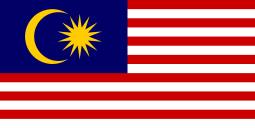
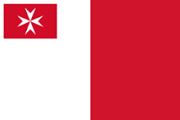
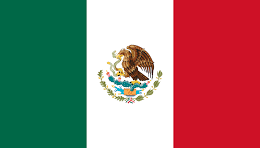
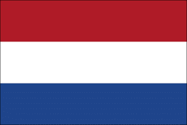
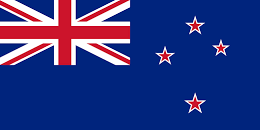
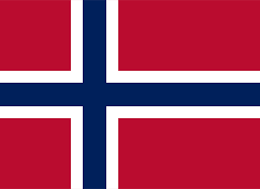
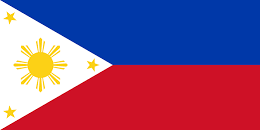
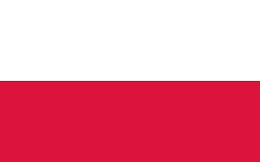
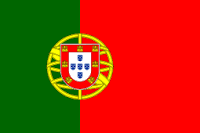
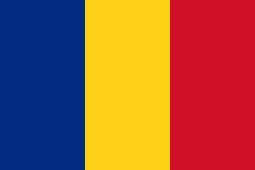
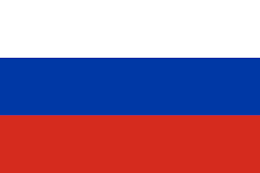

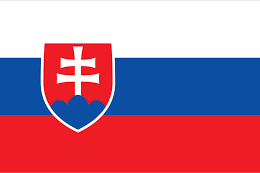
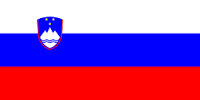
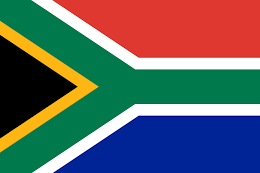
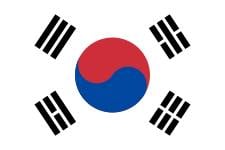
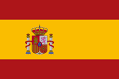
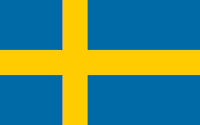
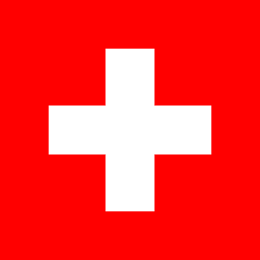
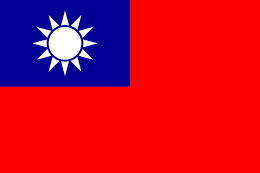
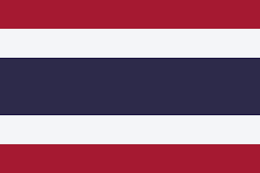
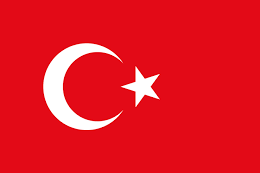
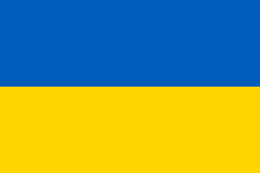
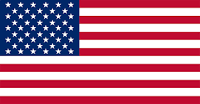
In this chapter, we discuss how to use Python to perform some basic encryption by scrambling letters. This will introduce some basic string manipulation, user input, progressing on to creating reusable modules, and graphical user interfaces.
To follow, we will create some useful Python scripts that can be added to run as the Raspberry Pi boots or an easy-to-run command that will provide quick shortcuts to common or frequently-used commands. Taking this further, we will make use of threading to run multiple tasks and introduce classes to define multiple objects.
As it is customary to start any programming exercise with a Hello World example, we will kick off with that now.
Create the hellopi.py
file using nano
, as follows:
nano -c hellopi.py
Within our hellopi.py
file, add the following code:
#!/usr/bin/python3 #hellopi.py print ("Hello Raspberry Pi")
When done, save and exit (Ctrl + X, Y, and Enter). To run the file, use the following command:
python3 hellopi.py
Congratulations,...
A good starting point for Python is to gain an understanding of basic text handling and strings. A string is a block of characters stored together as a value. As you will learn, they can be viewed as a simple list of characters.
We will create a script to obtain the user's input, use string manipulation to switch around the letters, and print out a coded version of the message. We will then extend this example by demonstrating how encoded messages can be passed between parties without revealing the encoding methods, while also showing how to reuse sections of the code within other Python modules.
You can use most text editors to write Python code. They can be used directly on the Raspberry Pi or remotely through VNC or SSH.
The following are a few text editors that are available with the Raspberry Pi:
In addition to easy string handling, Python allows you to read, edit, and create files easily. So, by building upon the previous scripts, we can make use of our encryptText()
function to encode complete files.
Reading and writing to files can be quite dependent on factors that are outside of the direct control of the script, such as whether the file that we are trying to open exists or the filesystem has space to store a new file. Therefore, we will also take a look at how to handle exceptions and protect operations that may result in errors.
The following script will allow you to specify a file through the command line, which will be read and encoded to produce an output file. Create a small text file named infile.txt
and save it so that we can test the script. It should include a short message similar to the following:
This is a short message to test our file encryption program.
We will now apply the methods introduced in the previous scripts and reapply them to create a menu that we can customize to present a range of quick-to-run commands and programs.
Create the menu.py
script using the following code:
#!/usr/bin/python3 #menu.py from subprocess import call filename="menu.ini" DESC=0 KEY=1 CMD=2 print ("Start Menu:") try: with open(filename) as f: menufile = f.readlines() except IOError: print ("Unable to open %s" % (filename)) for item in menufile: line = item.split(',') print ("(%s):%s" % (line[KEY],line[DESC])) #Get user input running = True while(running): user_input = input() #Check input, and execute command for item in menufile: line = item.split(',') if (user_input == line[KEY]): print ("Command: " + line[CMD]) #call the script #e.g. call(["ls", "-l"]) commands = line[CMD].rstrip().split() print (commands) running = False #Only run command is one if...
While the previous menu is very useful for defining the most common commands and functions we may use when running the Raspberry Pi, we will often change what we are doing or develop scripts to automate complex tasks.
To avoid the need to continuously update and edit the menu.ini
file, we can create a menu that can list the installed scripts and dynamically build a menu from it, as shown in the following screenshot:
A menu of all the Python scripts in the current directory
Create the menuadv.py
script using the following code:
#!/usr/bin/python3 #menuadv.py import os from subprocess import call SCRIPT_DIR="." #Use current directory SCRIPT_NAME=os.path.basename(__file__) print ("Start Menu:") scripts=[] item_num=1 for files in os.listdir(SCRIPT_DIR): if files.endswith(".py"): if files != SCRIPT_NAME: print ("%s:%s"%(item_num,files)) scripts.append(files) item_num+=1 running = True while (running): print ("Enter script number...