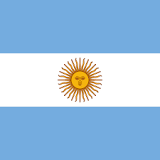
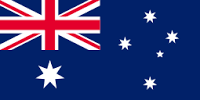
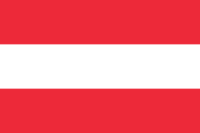
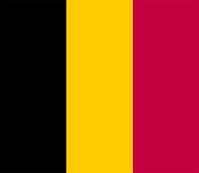
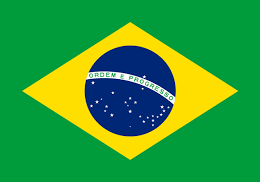
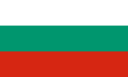
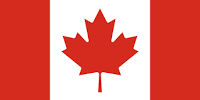
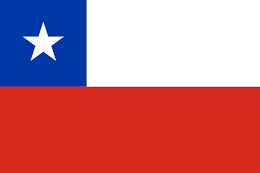
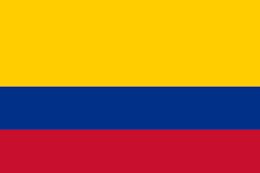
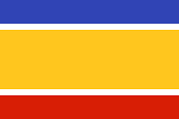
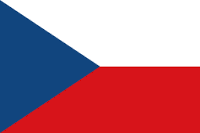
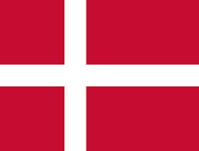
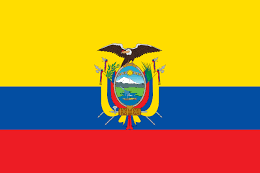
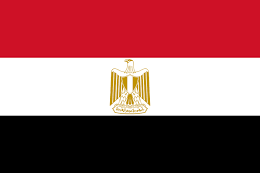
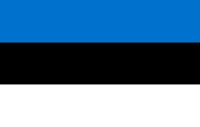
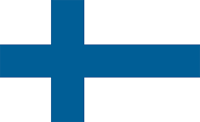
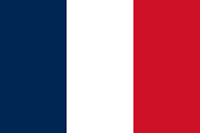
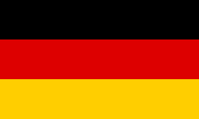
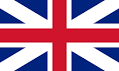
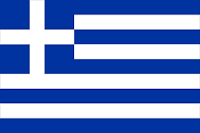
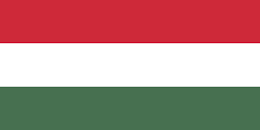
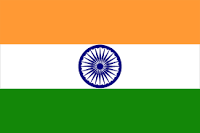
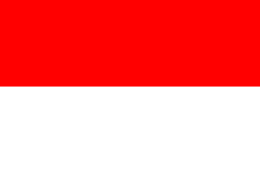
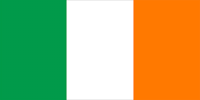
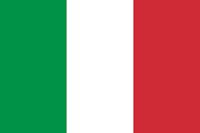
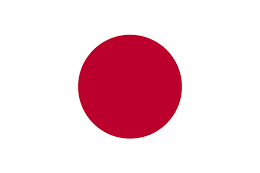
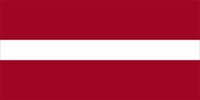
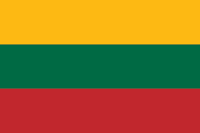
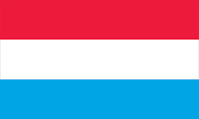
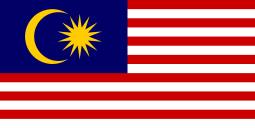
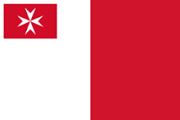
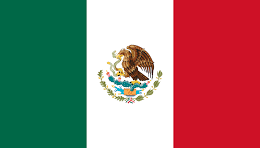
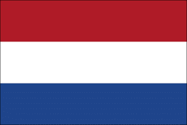
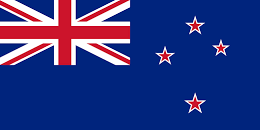
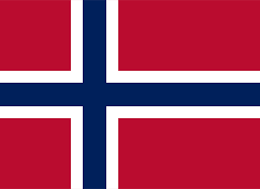
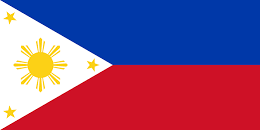
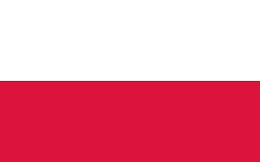
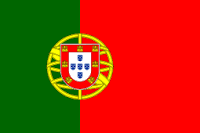
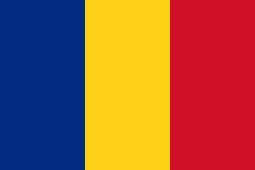
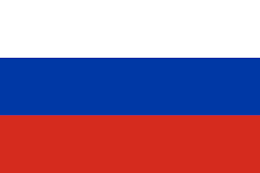

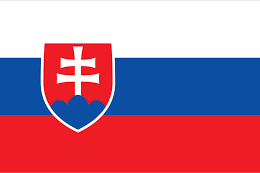
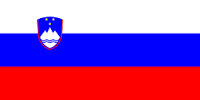
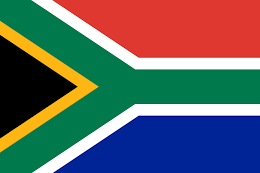
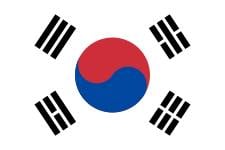
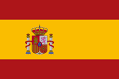
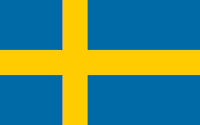
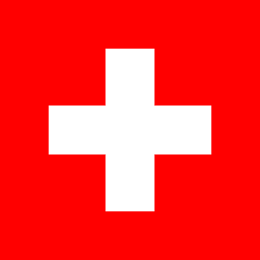
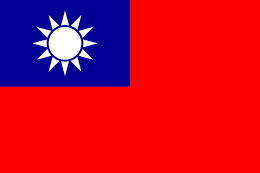
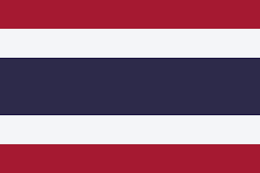
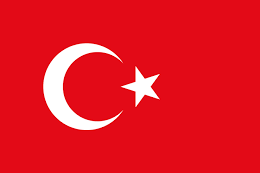
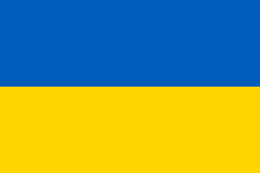
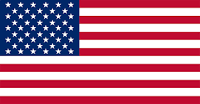
One of the simplest forms of hardware output that we can add to a program is an LED attached to a GPIO pin. We've already blinked LEDs, but not in a way that conveyed any sort of meaningful information; so first things first, we'll need something to communicate to the user. Here's a simple program that uses Internet Message Access Protocol (IMAP) to login to your email account and retrieve the number of unread messages in your inbox. Save it as a new file called email_counter.py
, either in Cloud9 or your editor of choice over SSH, filling in the IMAP details for your account, as shown in the following code:
import imaplib IMAP_host = "imap.gmail.com" IMAP_email = "username@gmail.com" IMAP_pass = "password" email = imaplib.IMAP4_SSL(IMAP_host) def connect(): email.login(IMAP_email, IMAP_pass) def disconnect(): email.close() email.logout() def get_num_emails(): email.select("INBOX") ret, data = email.search(None, "UNSEEN") return len(data[0].split(...
We've looked at various forms of displays that you can attach to your BeagleBone, but those are only useful when you're looking at it. If your BeagleBone is not accessible, for instance, it's monitoring something at your home while you're out, you might still need a way for it to send you information. One simple way to achieve this is to use Simple Mail Transfer Protocol (SMTP) to send e-mails from your BeagleBone. Save this code to a new file called email_sender.py
by filling in your account's SMTP details:
import smtplib from email.mime.text import MIMEText SMTP_host = 'smtp.gmail.com' SMTP_email = 'username@gmail.com' SMTP_pass = 'password' def send_email(to, subject, body): msg = MIMEText(body) msg['Subject'] = subject msg['From'] = SMTP_email msg['To'] = to server = smtplib.SMTP_SSL(SMTP_host) server.login(SMTP_email, SMTP_pass) server.sendmail(SMTP_email, to, msg.as_string())
We've looked at a few different types of LED displays, but because of the large size and spacing of their pixels they tend to be poor at conveying much information. When you want to display text, one of the simplest ways to do so is with a character LCD (liquid crystal display). These are rectangular displays typically consisting of 1, 2, or 4 lines of 8, 16, or 20 characters each, and are commonly used in radios and other low-cost electronics. Each character is typically made up of a matrix of 5 x 8 pixels, which is enough resolution to display any of the ASCII characters, along with some additional symbols.
For this example, I'm using a 2 x 16 character LCD. It will work with different sizes, but with a width of less than 16 characters, some of the text will be cut off. Character LCDs usually require a 5 V supply, but some companies such as SparkFun sell 3.3 V versions. Either version will work with the BeagleBone, just make sure to hook it up to the correct power supply....
In this chapter, you learned a number of ways to provide output to the user from your BeagleBone programs. You learned how to interface with and control different types of LED and LCD displays, as well as how to have your BeagleBone send messages to you remotely. You built an unread e-mail notification system, a thermometer, a motion detection system, and two different system resource monitors for your BeagleBone Black.
In the next chapter, you will learn how the I2C and SPI protocols work, and how to use them with Python.