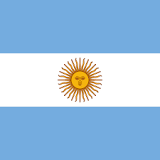
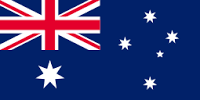
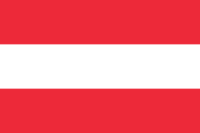
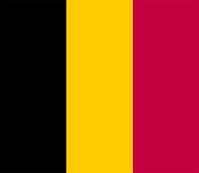
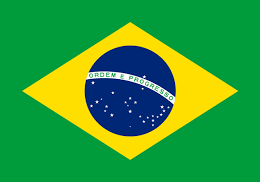
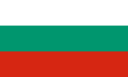
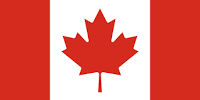
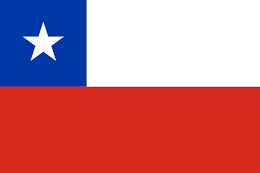
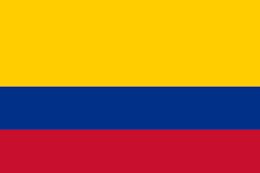
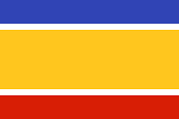
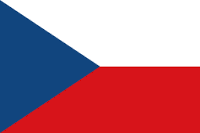
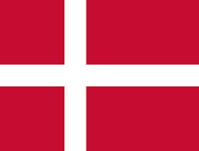
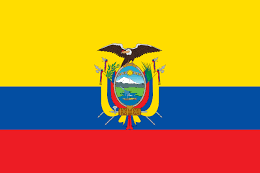
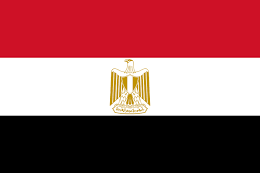
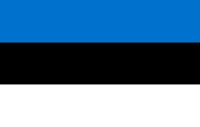
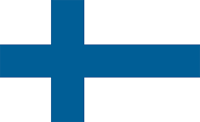
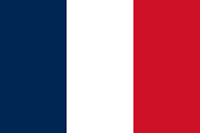
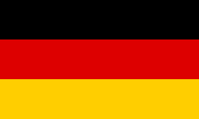
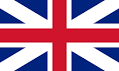
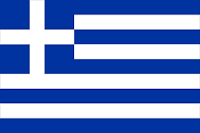
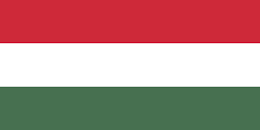
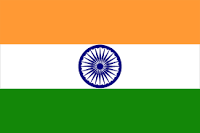
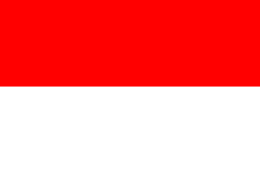
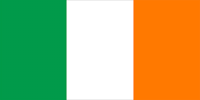
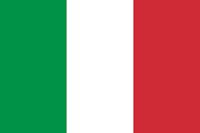
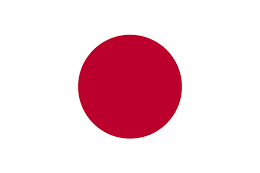
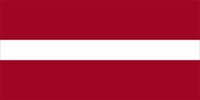
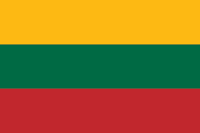
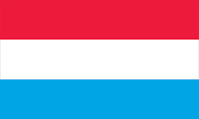
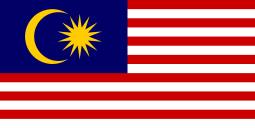
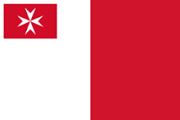
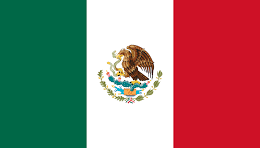
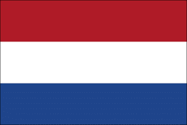
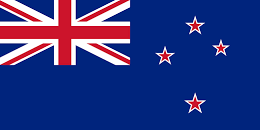
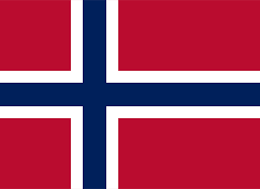
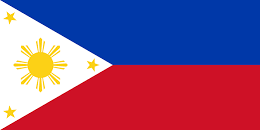
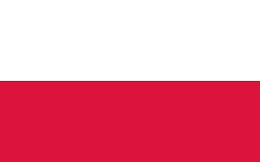
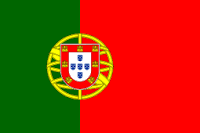
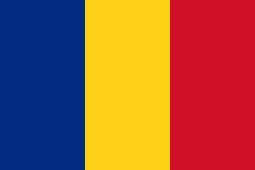
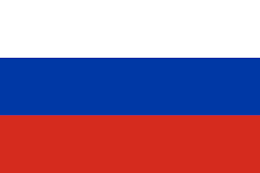

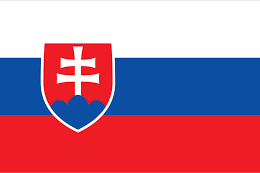
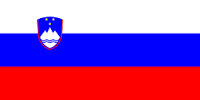
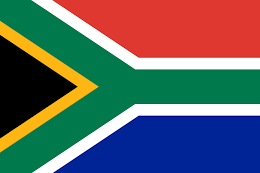
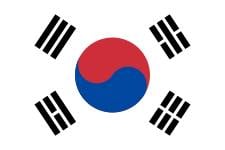
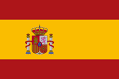
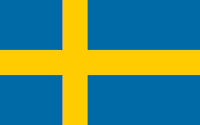
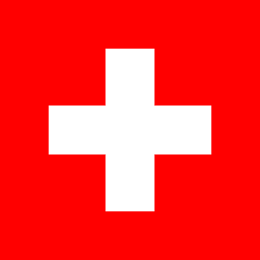
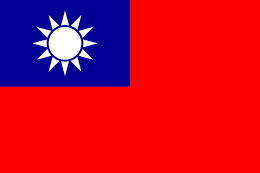
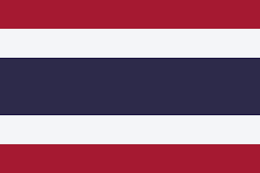
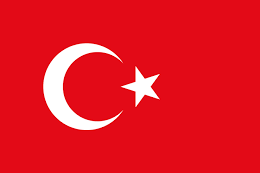
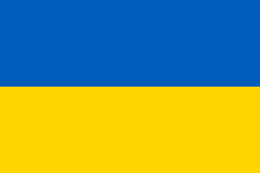
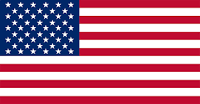
Documents in Couchbase are simply key/value pairs where the value is stored as a valid JSON document. The key/value API we learnt in Chapter 2, Using Couchbase CRUD Operations, is the same API we'll use to create JSON documents in the server. Generally, you'll use the client SDKs in combination with your platform's preferred JSON serializer, as shown in this C# snippet:
var user = new User { Name = "John" }; var json = JsonConvert.SerializeObject(user); bucket.Upsert("jsmith", json);
In this example, the popular .NET JSON serializer is used to transform an instance of a .NET class into a valid JSON string. That string is then stored on Couchbase Server using the key/value set
operation.
Similarly, to retrieve a JSON document from the server, you'll also use the key/value Get
operation:
var json = bucket.Get<string>("jsmith"); var user = JsonConvert.DeserializeObject<User>(json);
In the case of retrieving a document, you'll typically retrieve the JSON string and...
We've already seen how Couchbase handles primary indexes for documents. The key in the key/value pair is the unique primary key for a document. Using the key/value API, documents may be retrieved only by this key. While this might seem limiting, there are key/value schema design patterns that help to provide flexibility. We'll explore them in Chapter 5, Introducing N1QL.
Fortunately, Couchbase as a document store provides a much more powerful approach for finding your documents. To illustrate the problem and the solution, we'll walk through a brief example. Imagine having a simple JSON document such as this:
{ "Username": "jsmith", "Email": "jsmith@somedomain.com" }
The key/value limitation is easy to see. Imagine we want to find a user by their username. The key/value solution might be to use the username as the key. While that would certainly work, what happens when we also want to query a user by their e-mail address? We can't have both e-mail and username as a key...
Before we can start our exploration of the Couchbase Server views, we first need to understand what MapReduce is and how we'll use it to create secondary indexes for our documents.
At its simplest, MapReduce is a programming pattern used to process large amounts of data that is typically distributed across several nodes in parallel. In the NoSQL world, MapReduce implementations may be found on many platforms from MongoDB to Hadoop, and of course Couchbase.
Even if you're new to the NoSQL landscape, it's quite possible that you've already worked with a form of MapReduce. The inspiration for MapReduce in distributed NoSQL systems was drawn from the functional programming concepts of map and reduce. While purely functional programming languages haven't quite reached mainstream status, languages such as Python, C#, and JavaScript all support map and reduce operations.
At this point, you should have a general sense of what MapReduce is, where it came from, and how it will affect the creation of a Couchbase Server view. So without further ado, let's see how to write our first Couchbase view.
In Chapter 1, Getting Comfortable with Couchbase, we saw that when we install Couchbase Server, we have the option of including a sample bucket. In fact, there were two to choose from. The bucket we'll use is beer-sample. If you didn't install it, don't worry. You can add it by opening the Couchbase Console and navigating to the Settings tab. Here, you'll find the option to install the bucket, as shown next:
In the next sections, we'll return to the console to create our view, examine documents, and query our views. For now, however, we'll simply examine the code. First, you need to understand the document structures with which you're working. The following JSON object is a beer document (abbreviated for brevity):
{ "name": "Sundog", "type": "beer", ...
In Chapter 1, Getting Comfortable with Couchbase, we skipped the Views tab in the Couchbase Web Console with the promise of returning to it in later chapters. It's just about time to fulfill that promise, but first we'll take a look at another tab we skipped—the Data Buckets tab.
Open Couchbase Console. As a reminder, it's found at http://localhost:8091
. If you're using Couchbase on a server other than your laptop, substitute that server's name for localhost
. After logging in, navigate to the Data Buckets tab, as shown here:
The Data Buckets tab provides you with a high-level overview of your buckets. You'll see each bucket listed with information ranging from server resource utilization to item (document) count. Feel free to explore some of the other features of this tab. This is where you are able to create and edit buckets. What we're most interested in is checking out the documents in our bucket. Click on the Documents button in the beer-sample bucket row, as shown next...
An important distinction to make at this point is that views are not queries, but means of querying for documents. You'll run queries against the view index in order to find the original documents. It is a common misconception that the views you write are actual queries.
Couchbase views have an API that supports a variety of search options, from an exact key search to a key range search. Continuing to use the Couchbase Console, we'll explore the various parameters we are able to use as we query our views. Begin by clicking on the down arrow next to the Filter Results text above the results panel.
Filtering views
To continue with the reduce example, check the box next to group and click on Close. Click on Show Results again, and you'll now see that the results are grouped by the name of the category, as shown here:
There's an additional parameter called group_level under the group option. This parameter takes an integer argument and is meant to be used with composite (array...
For another perspective on your view, click on the link right above the grid of results. This link will lead to a JSON view of our index:
{ "total_rows": 5891, "rows": [ { "id": "sullivan_s_black_forest_brew_haus_grill", "key": ".38 Special Bitter", "value": null }, { "id": "512_brewing_company-512_alt", "key": "(512) ALT", "value": null }, { "id": "512_brewing_company-512_bruin", "key": "(512) Bruin", "value": null } ] }
The client libraries will use this JSON when querying a view. This view also introduces an important element of the Couchbase Server view API—the fact that it's HTTP-based. Unlike the key/value API, which is a binary protocol, views are queried over a RESTful API, with parameter values supplied as query string arguments. Being able to see the JSON that your client library sees...
We covered a lot of ground in this chapter. In the beginning, you saw Couchbase only as a key/value store. Since then, you learned that Couchbase is a very capable document store as well, treating JSON documents as first-class citizens.
You learned the purpose of secondary indexes in a key/value store. We dug deep into MapReduce, both in terms of its history in functional languages and as a tool for NoSQL and big data systems.
As far as Couchbase MapReduce is concerned, we only scratched the surface. While you learned how to develop, test, and query views, the queries covered so far were simple. Couchbase view queries are capable of a lot more, which you will see as we move forward.
In the next chapter, we'll cover MapReduce in detail. We will have to start exploring more complex views, with a special focus on queries you're probably used to in SQL. From complex keys to simulating joins, you'll soon see that Couchbase views can be used for a lot more than simple queries.