Data structures are a particular way of storing data in software engineering. They play a vital role in storing data in a computer so that it can be accessed and modified efficiently, and they provide different storing mechanisms for storing different types of data. There are many types of data structure, and each one is designed to store a definite type of data. In this chapter, we will cover data structures in detail and learn which data structures should be used for particular scenarios in order to improve the performance of the system as regards data storage and retrieval. We will also learn how we can write optimized code in C# and what primary factors can affect performance, which is sometimes overlooked by developers when coding programs. We will learn some best practices that can be used to optimize code that is performance...
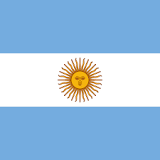
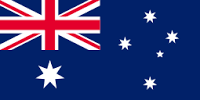
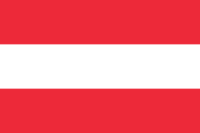
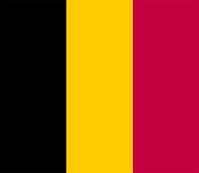
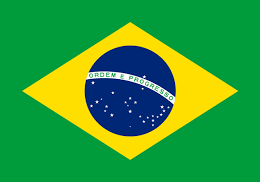
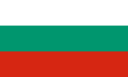
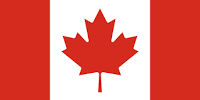
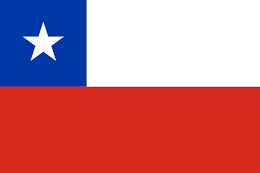
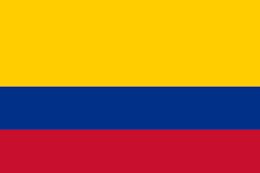
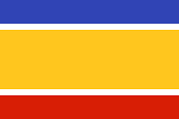
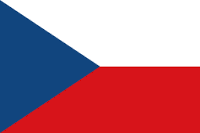
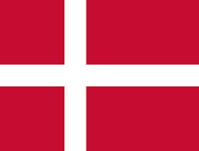
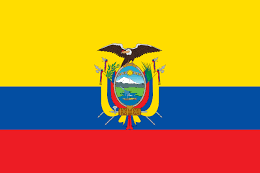
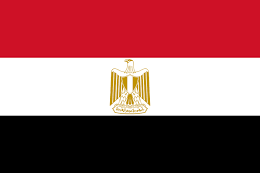
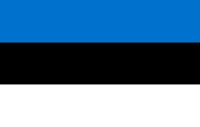
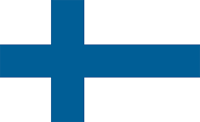
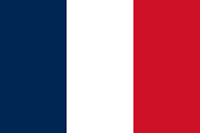
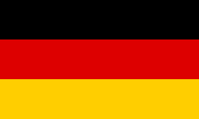
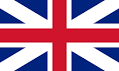
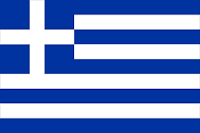
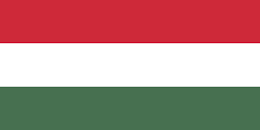
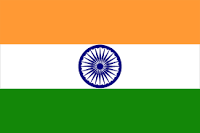
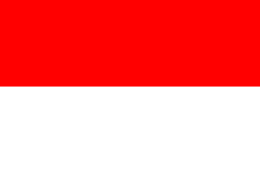
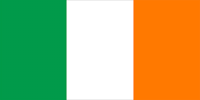
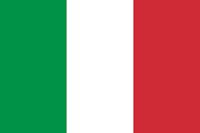
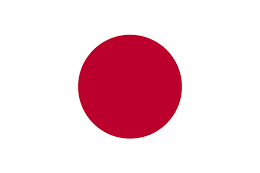
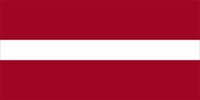
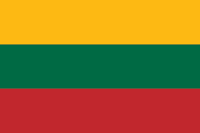
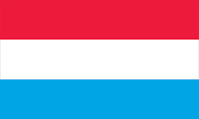
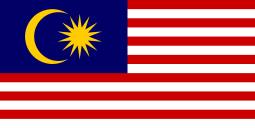
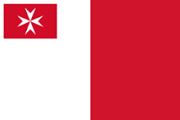
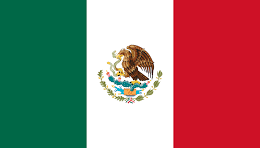
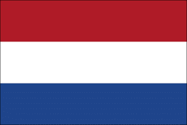
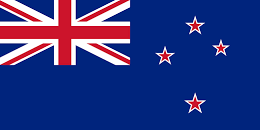
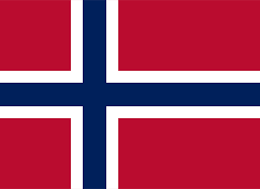
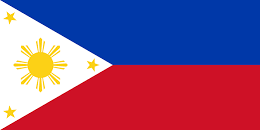
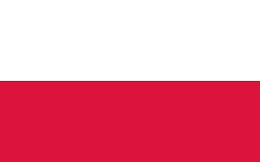
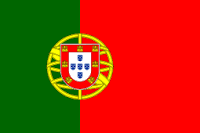
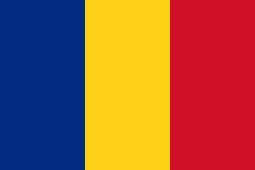
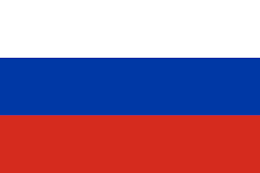

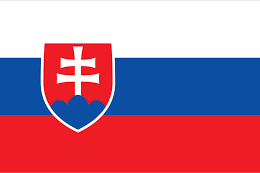
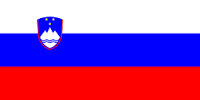
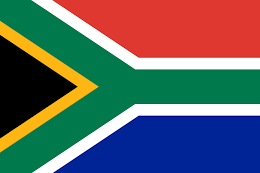
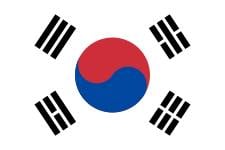
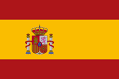
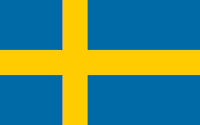
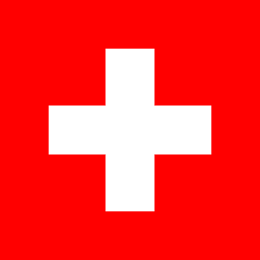
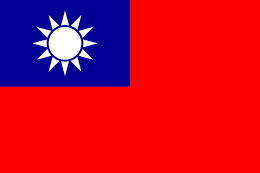
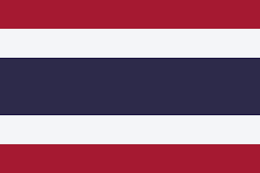
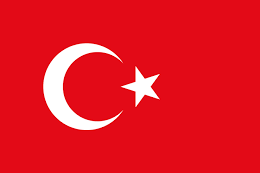
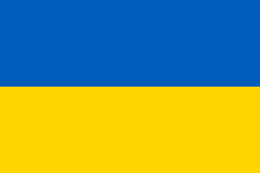
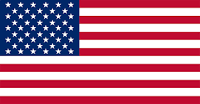