Sequential vs Parallel animations in Angular
In this recipe, you'll learn how to run angular animations in a sequence vs in parallel. This is handy for when we want to have one animation finished before we start the next one, or to run the animations simultaneously.
Getting ready
The app that we are going to work with resides in start/apps/chapter04/ng-seq-parallel-animations
inside the cloned repository:
- Open the code repository in your Code Editor.
- Open the terminal, navigate to the code repository directory and run
npm run serve ng-seq-parallel-animations
to serve the project
This should open the app in a new browser tab and you should see the following:
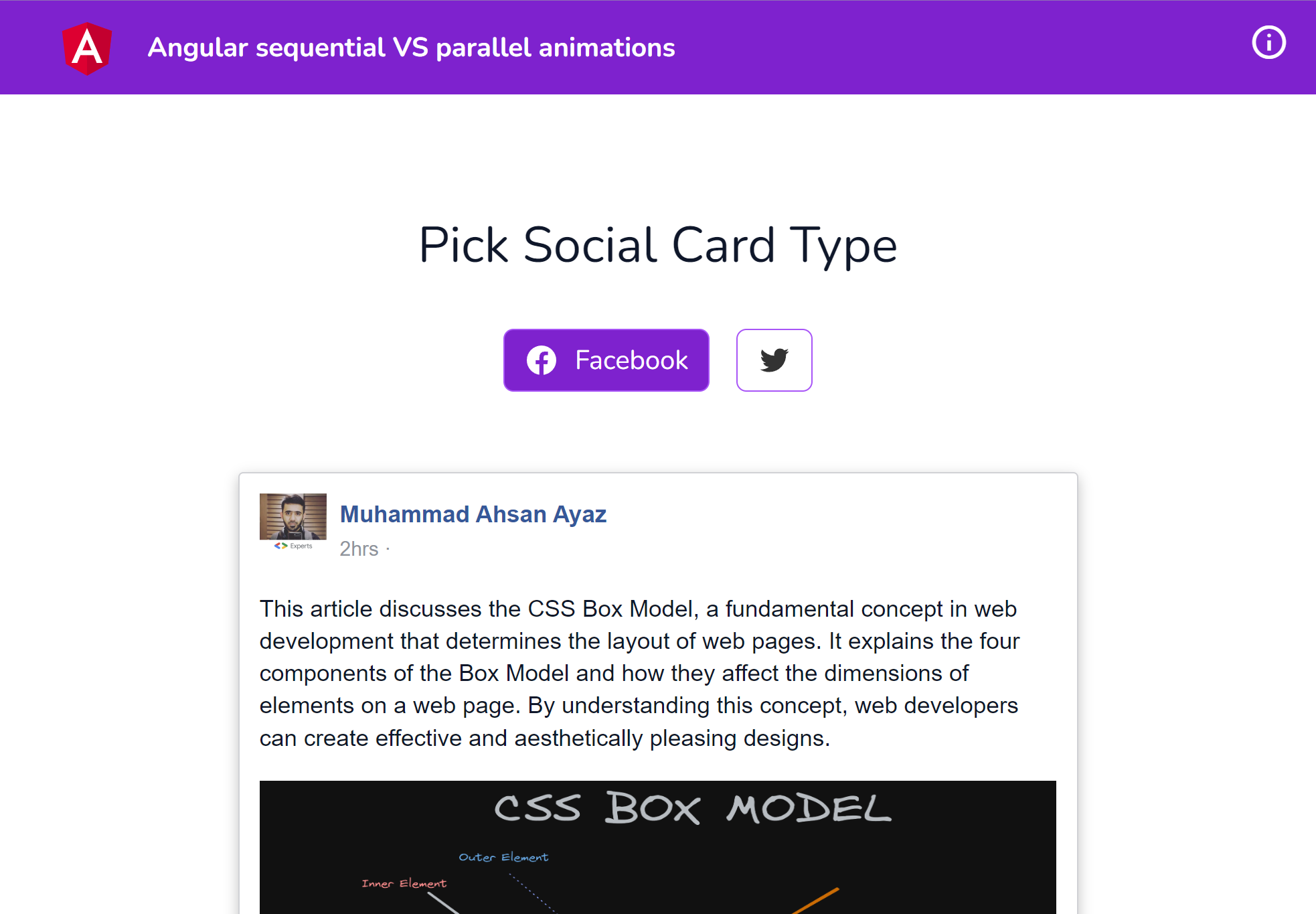
Now that we have the app running locally, let's see the steps of the recipe in the next section.
How to do it…
We have an app that displays two cards we used in the previous recipes. To see how to use animation callbacks, we...