Creating your first Template-Driven form with validation
Let's start getting familiar with Angular forms in this recipe. In this one, you'll learn about the basic concepts of template-driven forms and will create a basic Angular form using the template-driven forms API.
Getting ready
The app that we are going to work with resides in start/apps/chapter08/ng-tdf
inside the cloned repository:
- Open the code repository in your Code Editor.
- Open the terminal, navigate to the code repository directory and run
npm run serve ng-tdf
to serve the project
This should open the app in a new browser tab. And you should see the following:
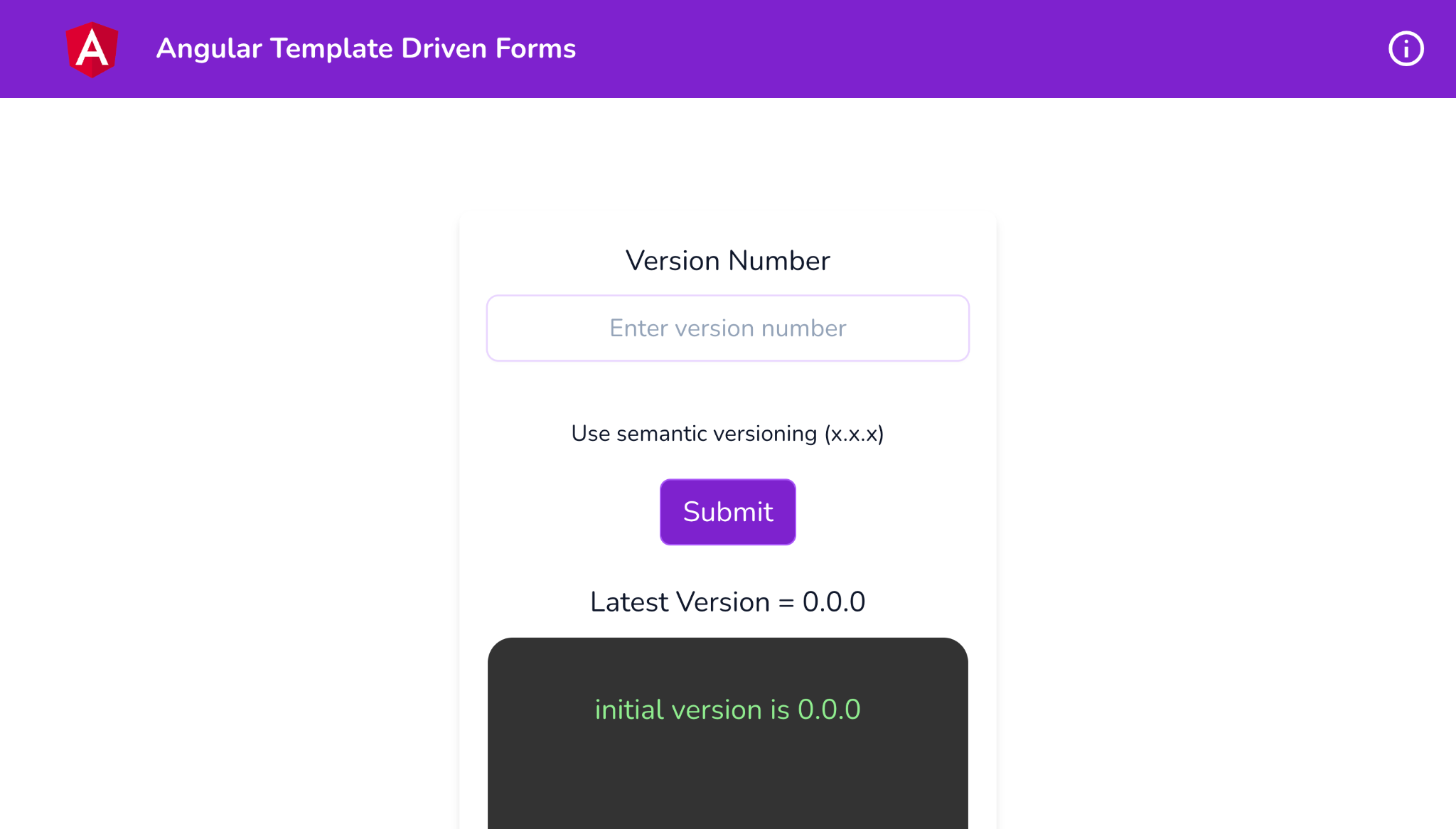
How to do it…
We have an Angular app that already has a bunch of components and some UI setup. need to implement the functionality to add a new version to the logs. We’ll use a template-driven form to allow the user to pick an app and submit a release version. Let's get...