How to apply multiple structural directives on the same element
In certain situations, you might want to use more than one structural directive on the same host or for the same element —for example, a combination of *ngIf
and *ngFor
together. Which is not something Angular supports out of the box. In this recipe, we will show a message conditionally using *ngIf
for the case when we have no items in the bucket. Since we’re supposed to show conditionally, and apply the for loop on the element, this is a perfect example to use for the recipe.
Getting ready
The app that we are going to work with resides in start/apps/chapter02/ng-multi-struc-directives
inside the cloned repository:
- Open the code repository in your Code Editor.
- Open the terminal, navigate to the code repository directory and run
npm run serve ng-multi-struc-directives
to serve the project
This should open the app in a new browser tab and you should see the followin
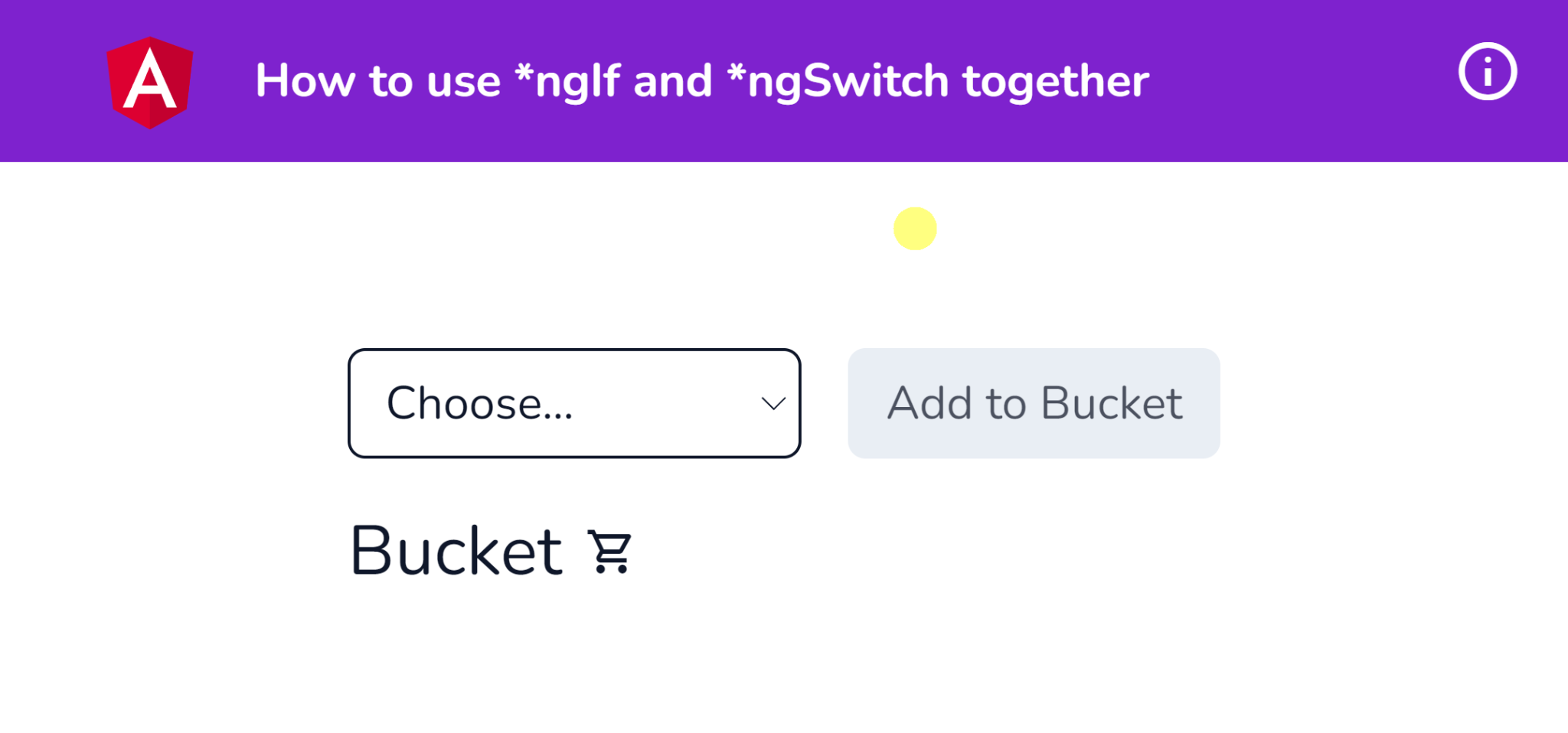