In this chapter, we will cover:
- Setting up the OpenGL v3.3 core profile on Visual Studio 2010 using the GLEW and freeglut libraries
- Designing a GLSL shader class
- Rendering a simple colored triangle using shaders
- Doing a ripple mesh deformer using the vertex shader
- Dynamically subdividing a plane using the geometry shader
- Dynamically subdividing a plane using the geometry shader with instanced rendering
- Drawing a 2D image in a window using the fragment shader and SOIL image loading library
The OpenGL API has seen various changes since its creation in 1992. With every new version, new features were added and additional functionality was exposed on supporting hardware through extensions. Until OpenGL v2.0 (which was introduced in 2004), the functionality in the graphics pipeline was fixed, that is, there were fixed set of operations hardwired in the graphics hardware and it was impossible to modify the graphics pipeline. With OpenGL v2.0, the shader objects were introduced for the first time. That enabled programmers to modify the graphics pipeline through special programs called shaders, which were written in a special language called OpenGL shading language (GLSL).
After OpenGL v2.0, the next major version was v3.0. This version introduced two profiles for working with OpenGL; the core profile and the compatibility profile. The core profile basically contains all of the non-deprecated functionality whereas the compatibility profile retains deprecated functionality for backwards compatibility. As of 2012, the latest version of OpenGL available is OpenGL v4.3. Beyond OpenGL v3.0, the changes introduced in the application code are not as drastic as compared to those required for moving from OpenGL v2.0 to OpenGL v3.0 and above.
We will start with a very basic example in which we will set up the modern OpenGL v3.3 core profile. This example will simply create a blank window and clear the window with red color.
OpenGL or any other graphics API for that matter requires a window to display graphics in. This is carried out through platform specific codes. Previously, the GLUT library was invented to provide windowing functionality in a platform independent manner. However, this library was not maintained with each new OpenGL release. Fortunately, another independent project, freeglut, followed in the GLUT footsteps by providing similar (and in some cases better) windowing support in a platform independent way. In addition, it also helps with the creation of the OpenGL core/compatibility profile contexts. The latest version of freeglut may be downloaded from http://freeglut.sourceforge.net. The version used in the source code accompanying this book is v2.8.0. After downloading the freeglut library, you will have to compile it to generate the libs/dlls.
The extension mechanism provided by OpenGL still exists. To aid with getting the appropriate function pointers, the GLEW library is used. The latest version can be downloaded from http://glew.sourceforge.net. The version of GLEW used in the source code accompanying this book is v1.9.0. If the source release is downloaded, you will have to build GLEW first to generate the libs and dlls on your platform. You may also download the pre-built binaries.
Prior to OpenGL v3.0, the OpenGL API provided support for matrices by providing specific matrix stacks such as the modelview, projection, and texture matrix stacks. In addition, transformation functions such as translate, rotate, and scale, as well as projection functions were also provided. Moreover, immediate mode rendering was supported, allowing application programmers to directly push the vertex information to the hardware.
In OpenGL v3.0 and above, all of these functionalities are removed from the core profile, whereas for backward compatibility they are retained in the compatibility profile. If we use the core profile (which is the recommended approach), it is our responsibility to implement all of these functionalities including all matrix handling and transformations. Fortunately, a library called glm
exists that provides math related classes such as vectors and matrices. It also provides additional convenience functions and classes. For all of the demos in this book, we will use the glm
library. Since this is a headers only library, there are no linker libraries for glm
. The latest version of glm
can be downloaded from http://glm.g-truc.net. The version used for the source code in this book is v0.9.4.0.
There are several image formats available. It is not a trivial task to write an image loader for such a large number of image formats. Fortunately, there are several image loading libraries that make image loading a trivial task. In addition, they provide support for both loading as well as saving of images into various formats. One such library is the
SOIL
image loading library. The latest version of SOIL
can be downloaded from http://www.lonesock.net/soil.html.
Once we have downloaded the SOIL
library, we extract the file to a location on the hard disk. Next, we set up the include and library paths in the Visual Studio environment. The include path on my development machine is D:\Libraries\soil\Simple OpenGL Image Library\src
whereas, the library path is set to D:\Libraries\soil\Simple OpenGL Image Library\lib\VC10_Debug
. Of course, the path for your system will be different than mine but these are the folders that the directories should point to.
These steps will help us to set up our development environment. For all of the recipes in this book, Visual Studio 2010 Professional version is used. Readers may also use the free express edition or any other version of Visual Studio (for example, Ultimate/Enterprise). Since there are a myriad of development environments, to make it easier for users on other platforms, we have provided premake script files as well.
The code for this recipe is in the Chapter1/GettingStarted
directory.
Tip
You can download the example code files for all Packt books you have purchased from your account at http://www.packtpub.com. If you purchased this book elsewhere, you can visit http://www.packtpub.com/support and register to have the files e-mailed directly to you.
Let us setup the development environment using the following steps:
- After downloading the required libraries, we set up the Visual Studio 2010 environment settings.
- We first create a new Win32 Console Application project as shown in the preceding screenshot. We set up an empty Win32 project as shown in the following screenshot:
- Next, we set up the include and library paths for the project by going into the Project menu and selecting project Properties. This opens a new dialog box. In the left pane, click on the Configuration Properties option and then on VC++ Directories.
- In the right pane, in the Include Directories field, add the GLEW and freeglut subfolder paths.
- Similarly, in the Library Directories, add the path to the lib subfolder of GLEW and freeglut libraries as shown in the following screenshot:
- Next, we add a new
.cpp
file to the project and name itmain.cpp
. This is the main source file of our project. You may also browse throughChapter1/ GettingStarted/GettingStarted/main.cpp
which does all this setup already. - Let us skim through the
Chapter1/ GettingStarted/GettingStarted/main.cpp
file piece by piece.#include <GL/glew.h> #include <GL/freeglut.h> #include <iostream>
These lines are the include files that we will add to all of our projects. The first is the GLEW header, the second is the freeglut header, and the final include is the standard input/output header.
- In Visual Studio, we can add the required linker libraries in two ways. The first way is through the Visual Studio environment (by going to the Properties menu item in the Project menu). This opens the project's property pages. In the configuration properties tree, we collapse the Linker subtree and click on the Input item. The first field in the right pane is
Additional Dependencies
. We can add the linker library in this field as shown in the following screenshot: - The second way is to add the
glew32.lib
file to the linker settings programmatically. This can be achieved by adding the followingpragma
:#pragma comment(lib, "glew32.lib")
- The next line is the using directive to enable access to the functions in the std namespace. This is not mandatory but we include this here so that we do not have to prefix
std::
to any standard library function from the iostream header file.using namespace std;
- The next lines define the width and height constants which will be the screen resolution for the window. After these declarations, there are five function definitions . The
OnInit()
function is used for initializing any OpenGL state or object,OnShutdown()
is used to delete an OpenGL object,OnResize()
is used to handle the resize event,OnRender()
helps to handle the paint event, andmain()
is the entry point of the application. We start with the definition of themain()
function.const int WIDTH = 1280; const int HEIGHT = 960; int main(int argc, char** argv) { glutInit(&argc, argv); glutInitDisplayMode(GLUT_DEPTH | GLUT_DOUBLE | GLUT_RGBA); glutInitContextVersion (3, 3); glutInitContextFlags (GLUT_CORE_PROFILE | GLUT_DEBUG); glutInitContextProfile(GLUT_FORWARD_COMPATIBLE); glutInitWindowSize(WIDTH, HEIGHT);
- The first line
glutInit
initializes the GLUT environment. We pass the command line arguments to this function from our entry point. Next, we set up the display mode for our application. In this case, we request the GLUT framework to provide support for a depth buffer, double buffering (that is a front and a back buffer for smooth, flicker-free rendering), and the format of the frame buffer to be RGBA (that is with red, green, blue, and alpha channels). Next, we set the required OpenGL context version we desire by using theglutInitContextVersion
. The first parameter is the major version of OpenGL and the second parameter is the minor version of OpenGL. For example, if we want to create an OpenGL v4.3 context, we will callglutInitContextVersion (4, 3)
. Next, the context flags are specified:glutInitContextFlags (GLUT_CORE_PROFILE | GLUT_DEBUG); glutInitContextProfile(GLUT_FORWARD_COMPATIBLE);
- For any version of OpenGL including OpenGL v3.3 and above, there are two profiles available: the core profile (which is a pure shader based profile without support for OpenGL fixed functionality) and the compatibility profile (which supports the OpenGL fixed functionality). All of the matrix stack functionality
glMatrixMode(*)
,glTranslate*
,glRotate*
,glScale*
, and so on, and immediate mode calls such asglVertex*
,glTexCoord*
, andglNormal*
of legacy OpenGL, are retained in the compatibility profile. However, they are removed from the core profile. In our case, we will request a forward compatible core profile which means that we will not have any fixed function OpenGL functionality available. - Next, we set the screen size and create the window:
glutInitWindowSize(WIDTH, HEIGHT); glutCreateWindow("Getting started with OpenGL 3.3");
- Next, we initialize the GLEW library. It is important to initialize the GLEW library after the OpenGL context has been created. If the function returns
GLEW_OK
the function succeeds, otherwise the GLEW initialization fails.glewExperimental = GL_TRUE; GLenum err = glewInit(); if (GLEW_OK != err){ cerr<<"Error: "<<glewGetErrorString(err)<<endl; } else { if (GLEW_VERSION_3_3) { cout<<"Driver supports OpenGL 3.3\nDetails:"<<endl; } } cout<<"\tUsing glew "<<glewGetString(GLEW_VERSION)<<endl; cout<<"\tVendor: "<<glGetString (GL_VENDOR)<<endl; cout<<"\tRenderer: "<<glGetString (GL_RENDERER)<<endl; cout<<"\tVersion: "<<glGetString (GL_VERSION)<<endl; cout<<"\tGLSL: "<<glGetString(GL_SHADING_LANGUAGE_VERSION)<<endl;
The
glewExperimental
global switch allows the GLEW library to report an extension if it is supported by the hardware but is unsupported by the experimental or pre-release drivers. After the function is initialized, the GLEW diagnostic information such as the GLEW version, the graphics vendor, the OpenGL renderer, and the shader language version are printed to the standard output. - Finally, we call our initialization function
OnInit()
and then attach our uninitialization functionOnShutdown()
as theglutCloseFunc
method—the close callback function which will be called when the window is about to close. Next, we attach our display and reshape function to their corresponding callbacks. The main function is terminated with a call to theglutMainLoop()
function which starts the application's main loop.OnInit(); glutCloseFunc(OnShutdown); glutDisplayFunc(OnRender); glutReshapeFunc(OnResize); glutMainLoop(); return 0; }
The remaining functions are defined as follows:
For this simple example, we set the clear color to red (R:1, G:0, B:0, A:0). The first three are the red, green, and blue channels and the last is the alpha channel which is used in alpha blending. The only other function defined in this simple example is the OnRender()
function, which is our display callback function that is called on the paint event. This function first clears the color and depth buffers to the clear color and clear depth values respectively.
Tip
Similar to the color buffer, there is another buffer called the depth buffer. Its clear value can be set using the glClearDepth
function. It is used for hardware based hidden surface removal. It simply stores the depth of the nearest fragment encountered so far. The incoming fragment's depth value overwrites the depth buffer value based on the depth clear function specified for the depth test using the glDepthFunc
function. By default the depth value gets overwritten if the current fragment's depth is lower than the existing depth in the depth buffer.
The glutSwapBuffers
function is then called to set the current back buffer as the current front buffer that is shown on screen. This call is required in a double buffered OpenGL application. Running the code gives us the output shown in the following screenshot.
setup the development environment using the following steps:
- After downloading the required libraries, we set up the Visual Studio 2010 environment settings.
- We first create a new Win32 Console Application project as shown in the preceding screenshot. We set up an empty Win32 project as shown in the following screenshot:
- Next, we set up the include and library paths for the project by going into the Project menu and selecting project Properties. This opens a new dialog box. In the left pane, click on the Configuration Properties option and then on VC++ Directories.
- In the right pane, in the Include Directories field, add the GLEW and freeglut subfolder paths.
- Similarly, in the Library Directories, add the path to the lib subfolder of GLEW and freeglut libraries as shown in the following screenshot:
- Next, we add a new
.cpp
file to the project and name itmain.cpp
. This is the main source file of our project. You may also browse throughChapter1/ GettingStarted/GettingStarted/main.cpp
which does all this setup already. - Let us skim through the
Chapter1/ GettingStarted/GettingStarted/main.cpp
file piece by piece.#include <GL/glew.h> #include <GL/freeglut.h> #include <iostream>
These lines are the include files that we will add to all of our projects. The first is the GLEW header, the second is the freeglut header, and the final include is the standard input/output header.
- In Visual Studio, we can add the required linker libraries in two ways. The first way is through the Visual Studio environment (by going to the Properties menu item in the Project menu). This opens the project's property pages. In the configuration properties tree, we collapse the Linker subtree and click on the Input item. The first field in the right pane is
Additional Dependencies
. We can add the linker library in this field as shown in the following screenshot: - The second way is to add the
glew32.lib
file to the linker settings programmatically. This can be achieved by adding the followingpragma
:#pragma comment(lib, "glew32.lib")
- The next line is the using directive to enable access to the functions in the std namespace. This is not mandatory but we include this here so that we do not have to prefix
std::
to any standard library function from the iostream header file.using namespace std;
- The next lines define the width and height constants which will be the screen resolution for the window. After these declarations, there are five function definitions . The
OnInit()
function is used for initializing any OpenGL state or object,OnShutdown()
is used to delete an OpenGL object,OnResize()
is used to handle the resize event,OnRender()
helps to handle the paint event, andmain()
is the entry point of the application. We start with the definition of themain()
function.const int WIDTH = 1280; const int HEIGHT = 960; int main(int argc, char** argv) { glutInit(&argc, argv); glutInitDisplayMode(GLUT_DEPTH | GLUT_DOUBLE | GLUT_RGBA); glutInitContextVersion (3, 3); glutInitContextFlags (GLUT_CORE_PROFILE | GLUT_DEBUG); glutInitContextProfile(GLUT_FORWARD_COMPATIBLE); glutInitWindowSize(WIDTH, HEIGHT);
- The first line
glutInit
initializes the GLUT environment. We pass the command line arguments to this function from our entry point. Next, we set up the display mode for our application. In this case, we request the GLUT framework to provide support for a depth buffer, double buffering (that is a front and a back buffer for smooth, flicker-free rendering), and the format of the frame buffer to be RGBA (that is with red, green, blue, and alpha channels). Next, we set the required OpenGL context version we desire by using theglutInitContextVersion
. The first parameter is the major version of OpenGL and the second parameter is the minor version of OpenGL. For example, if we want to create an OpenGL v4.3 context, we will callglutInitContextVersion (4, 3)
. Next, the context flags are specified:glutInitContextFlags (GLUT_CORE_PROFILE | GLUT_DEBUG); glutInitContextProfile(GLUT_FORWARD_COMPATIBLE);
- For any version of OpenGL including OpenGL v3.3 and above, there are two profiles available: the core profile (which is a pure shader based profile without support for OpenGL fixed functionality) and the compatibility profile (which supports the OpenGL fixed functionality). All of the matrix stack functionality
glMatrixMode(*)
,glTranslate*
,glRotate*
,glScale*
, and so on, and immediate mode calls such asglVertex*
,glTexCoord*
, andglNormal*
of legacy OpenGL, are retained in the compatibility profile. However, they are removed from the core profile. In our case, we will request a forward compatible core profile which means that we will not have any fixed function OpenGL functionality available. - Next, we set the screen size and create the window:
glutInitWindowSize(WIDTH, HEIGHT); glutCreateWindow("Getting started with OpenGL 3.3");
- Next, we initialize the GLEW library. It is important to initialize the GLEW library after the OpenGL context has been created. If the function returns
GLEW_OK
the function succeeds, otherwise the GLEW initialization fails.glewExperimental = GL_TRUE; GLenum err = glewInit(); if (GLEW_OK != err){ cerr<<"Error: "<<glewGetErrorString(err)<<endl; } else { if (GLEW_VERSION_3_3) { cout<<"Driver supports OpenGL 3.3\nDetails:"<<endl; } } cout<<"\tUsing glew "<<glewGetString(GLEW_VERSION)<<endl; cout<<"\tVendor: "<<glGetString (GL_VENDOR)<<endl; cout<<"\tRenderer: "<<glGetString (GL_RENDERER)<<endl; cout<<"\tVersion: "<<glGetString (GL_VERSION)<<endl; cout<<"\tGLSL: "<<glGetString(GL_SHADING_LANGUAGE_VERSION)<<endl;
The
glewExperimental
global switch allows the GLEW library to report an extension if it is supported by the hardware but is unsupported by the experimental or pre-release drivers. After the function is initialized, the GLEW diagnostic information such as the GLEW version, the graphics vendor, the OpenGL renderer, and the shader language version are printed to the standard output. - Finally, we call our initialization function
OnInit()
and then attach our uninitialization functionOnShutdown()
as theglutCloseFunc
method—the close callback function which will be called when the window is about to close. Next, we attach our display and reshape function to their corresponding callbacks. The main function is terminated with a call to theglutMainLoop()
function which starts the application's main loop.OnInit(); glutCloseFunc(OnShutdown); glutDisplayFunc(OnRender); glutReshapeFunc(OnResize); glutMainLoop(); return 0; }
The remaining functions are defined as follows:
For this simple example, we set the clear color to red (R:1, G:0, B:0, A:0). The first three are the red, green, and blue channels and the last is the alpha channel which is used in alpha blending. The only other function defined in this simple example is the OnRender()
function, which is our display callback function that is called on the paint event. This function first clears the color and depth buffers to the clear color and clear depth values respectively.
Tip
Similar to the color buffer, there is another buffer called the depth buffer. Its clear value can be set using the glClearDepth
function. It is used for hardware based hidden surface removal. It simply stores the depth of the nearest fragment encountered so far. The incoming fragment's depth value overwrites the depth buffer value based on the depth clear function specified for the depth test using the glDepthFunc
function. By default the depth value gets overwritten if the current fragment's depth is lower than the existing depth in the depth buffer.
The glutSwapBuffers
function is then called to set the current back buffer as the current front buffer that is shown on screen. This call is required in a double buffered OpenGL application. Running the code gives us the output shown in the following screenshot.
OnRender()
function,
Tip
Similar to the color buffer, there is another buffer called the depth buffer. Its clear value can be set using the glClearDepth
function. It is used for hardware based hidden surface removal. It simply stores the depth of the nearest fragment encountered so far. The incoming fragment's depth value overwrites the depth buffer value based on the depth clear function specified for the depth test using the glDepthFunc
function. By default the depth value gets overwritten if the current fragment's depth is lower than the existing depth in the depth buffer.
The glutSwapBuffers
function is then called to set the current back buffer as the current front buffer that is shown on screen. This call is required in a double buffered OpenGL application. Running the code gives us the output shown in the following screenshot.
We will now have a look at how to set up shaders. Shaders are special programs that are run on the GPU. There are different shaders for controlling different stages of the programmable graphics pipeline. In the modern GPU, these include the vertex shader (which is responsible for calculating the clip-space position of a vertex), the tessellation control shader (which is responsible for determining the amount of tessellation of a given patch), the tessellation evaluation shader (which computes the interpolated positions and other attributes on the tessellation result), the geometry shader (which processes primitives and can add additional primitives and vertices if needed), and the fragment shader (which converts a rasterized fragment into a colored pixel and a depth). The modern GPU pipeline highlighting the different shader stages is shown in the following figure.
The GLSLShader
class is defined in the GLSLShader.[h/cpp]
files. We first declare the constructor and destructor which initialize the member variables. The next three functions, LoadFromString
, LoadFromFile
, and CreateAndLinkProgram
handle the shader compilation, linking, and program creation. The next two functions, Use
and UnUse
functions bind and unbind the program. Two std::map
datastructures are used. They store the attribute's/uniform's name as the key and its location as the value. This is done to remove the redundant call to get the attribute's/uniform's location each frame or when the location is required to access the attribute/uniform. The next two functions, AddAttribute
and AddUniform
add the locations of the attribute and uniforms into their respective std::map
(_attributeList
and _uniformLocationList
).
In a typical shader application, the usage of the GLSLShader
object is as follows:
- Create the
GLSLShader
object either on stack (for example,GLSLShader
shader;) or on the heap (for example,GLSLShader* shader=new GLSLShader();
) - Call
LoadFromFile
on theGLSLShader
object reference - Call
CreateAndLinkProgram
on theGLSLShader
object reference - Call
Use
on theGLSLShader
object reference to bind the shader object - Call
AddAttribute
/AddUniform
to store locations of all of the shader's attributes and uniforms respectively - Call
UnUse
on theGLSLShader
object reference to unbind the shader object
Execution of the above four functions creates a shader object. After the shader object is created, a shader program object is created using the following set of functions in the following sequence:
In the GLSLShader
class, the first four steps are handled in the LoadFromString
function and the later four steps are handled by the CreateAndLinkProgram
member function. After the shader program object has been created, we can set the program for execution on the GPU. This process is called shader binding. This is carried out by the glUseProgram
function which is called through the Use
/UnUse
functions in the GLSLShader
class.
For accessing any attribute/uniform location, we provide an indexer in the GLSLShader
class. In cases where there is an error in the compilation or linking stage, the shader log is printed to the console. Say for example, our GLSLshader
object is called shader
and our shader
contains a uniform called MVP
. We can first add it to the map of GLSLShader
by calling shader.AddUniform("MVP")
. This function adds the uniform's location to the map. Then when we want to access the uniform, we directly call shader("MVP")
and it returns the location of our uniform.
GLSLShader
class is
In a typical shader application, the usage of the GLSLShader
object is as follows:
- Create the
GLSLShader
object either on stack (for example,GLSLShader
shader;) or on the heap (for example,GLSLShader* shader=new GLSLShader();
) - Call
LoadFromFile
on theGLSLShader
object reference - Call
CreateAndLinkProgram
on theGLSLShader
object reference - Call
Use
on theGLSLShader
object reference to bind the shader object - Call
AddAttribute
/AddUniform
to store locations of all of the shader's attributes and uniforms respectively - Call
UnUse
on theGLSLShader
object reference to unbind the shader object
Execution of the above four functions creates a shader object. After the shader object is created, a shader program object is created using the following set of functions in the following sequence:
In the GLSLShader
class, the first four steps are handled in the LoadFromString
function and the later four steps are handled by the CreateAndLinkProgram
member function. After the shader program object has been created, we can set the program for execution on the GPU. This process is called shader binding. This is carried out by the glUseProgram
function which is called through the Use
/UnUse
functions in the GLSLShader
class.
For accessing any attribute/uniform location, we provide an indexer in the GLSLShader
class. In cases where there is an error in the compilation or linking stage, the shader log is printed to the console. Say for example, our GLSLshader
object is called shader
and our shader
contains a uniform called MVP
. We can first add it to the map of GLSLShader
by calling shader.AddUniform("MVP")
. This function adds the uniform's location to the map. Then when we want to access the uniform, we directly call shader("MVP")
and it returns the location of our uniform.
usage of the GLSLShader
object is as follows:
- Create the
GLSLShader
object either on stack (for example,GLSLShader
shader;) or on the heap (for example,GLSLShader* shader=new GLSLShader();
) - Call
LoadFromFile
on theGLSLShader
object reference - Call
CreateAndLinkProgram
on theGLSLShader
object reference - Call
Use
on theGLSLShader
object reference to bind the shader object - Call
AddAttribute
/AddUniform
to store locations of all of the shader's attributes and uniforms respectively - Call
UnUse
on theGLSLShader
object reference to unbind the shader object
Execution of the above four functions creates a shader object. After the shader object is created, a shader program object is created using the following set of functions in the following sequence:
In the GLSLShader
class, the first four steps are handled in the LoadFromString
function and the later four steps are handled by the CreateAndLinkProgram
member function. After the shader program object has been created, we can set the program for execution on the GPU. This process is called shader binding. This is carried out by the glUseProgram
function which is called through the Use
/UnUse
functions in the GLSLShader
class.
For accessing any attribute/uniform location, we provide an indexer in the GLSLShader
class. In cases where there is an error in the compilation or linking stage, the shader log is printed to the console. Say for example, our GLSLshader
object is called shader
and our shader
contains a uniform called MVP
. We can first add it to the map of GLSLShader
by calling shader.AddUniform("MVP")
. This function adds the uniform's location to the map. Then when we want to access the uniform, we directly call shader("MVP")
and it returns the location of our uniform.
In the GLSLShader
class, the first four steps are handled in the LoadFromString
function and the later four steps are handled by the CreateAndLinkProgram
member function. After the shader program object has been created, we can set the program for execution on the GPU. This process is called shader binding. This is carried out by the glUseProgram
function which is called through the Use
/UnUse
functions in the GLSLShader
class.
For accessing any attribute/uniform location, we provide an indexer in the GLSLShader
class. In cases where there is an error in the compilation or linking stage, the shader log is printed to the console. Say for example, our GLSLshader
object is called shader
and our shader
contains a uniform called MVP
. We can first add it to the map of GLSLShader
by calling shader.AddUniform("MVP")
. This function adds the uniform's location to the map. Then when we want to access the uniform, we directly call shader("MVP")
and it returns the location of our uniform.
GLSLShader
class, the first four steps are handled in the LoadFromString
function and the later four steps are handled by the CreateAndLinkProgram
member function. After the shader program object has been created, we can set the program for execution on the GPU. This process is called shader binding
For accessing any attribute/uniform location, we provide an indexer in the GLSLShader
class. In cases where there is an error in the compilation or linking stage, the shader log is printed to the console. Say for example, our GLSLshader
object is called shader
and our shader
contains a uniform called MVP
. We can first add it to the map of GLSLShader
by calling shader.AddUniform("MVP")
. This function adds the uniform's location to the map. Then when we want to access the uniform, we directly call shader("MVP")
and it returns the location of our uniform.
We will now put the GLSLShader
class to use by implementing an application to render a simple colored triangle on screen.
Let us start this recipe using the following steps:
- Define a vertex shader (
shaders/shader.vert
) to transform the object space vertex position to clip space.#version 330 core layout(location = 0) in vec3 vVertex; layout(location = 1) in vec3 vColor; smooth out vec4 vSmoothColor; uniform mat4 MVP; void main() { vSmoothColor = vec4(vColor,1); gl_Position = MVP*vec4(vVertex,1); }
- Define a fragment shader (
shaders/shader.frag
) to output a smoothly interpolated color from the vertex shader to the frame buffer.#version 330 core smooth in vec4 vSmoothColor; layout(location=0) out vec4 vFragColor; void main() { vFragColor = vSmoothColor; }
- Load the two shaders using the
GLSLShader
class in theOnInit()
function.shader.LoadFromFile(GL_VERTEX_SHADER, "shaders/shader.vert"); shader.LoadFromFile(GL_FRAGMENT_SHADER,"shaders/shader.frag"); shader.CreateAndLinkProgram(); shader.Use(); shader.AddAttribute("vVertex"); shader.AddAttribute("vColor"); shader.AddUniform("MVP"); shader.UnUse();
- Create the geometry and topology. We will store the attributes together in an interleaved vertex format, that is, we will store the vertex attributes in a struct containing two attributes, position and color.
vertices[0].color=glm::vec3(1,0,0); vertices[1].color=glm::vec3(0,1,0); vertices[2].color=glm::vec3(0,0,1); vertices[0].position=glm::vec3(-1,-1,0); vertices[1].position=glm::vec3(0,1,0); vertices[2].position=glm::vec3(1,-1,0); indices[0] = 0; indices[1] = 1; indices[2] = 2;
- Store the geometry and topology in the buffer object(s). The stride parameter controls the number of bytes to jump to reach the next element of the same attribute. For the interleaved format, it is typically the size of our vertex struct in bytes, that is,
sizeof(Vertex)
.glGenVertexArrays(1, &vaoID); glGenBuffers(1, &vboVerticesID); glGenBuffers(1, &vboIndicesID); glBindVertexArray(vaoID); glBindBuffer (GL_ARRAY_BUFFER, vboVerticesID); glBufferData (GL_ARRAY_BUFFER, sizeof(vertices), &vertices[0], GL_STATIC_DRAW); glEnableVertexAttribArray(shader["vVertex"]); glVertexAttribPointer(shader["vVertex"], 3, GL_FLOAT, GL_FALSE,stride,0); glEnableVertexAttribArray(shader["vColor"]); glVertexAttribPointer(shader["vColor"], 3, GL_FLOAT, GL_FALSE,stride, (const GLvoid*)offsetof(Vertex, color)); glBindBuffer(GL_ELEMENT_ARRAY_BUFFER, vboIndicesID); glBufferData(GL_ELEMENT_ARRAY_BUFFER, sizeof(indices), &indices[0], GL_STATIC_DRAW);
- Set up the resize handler to set up the viewport and projection matrix.
void OnResize(int w, int h) { glViewport (0, 0, (GLsizei) w, (GLsizei) h); P = glm::ortho(-1,1,-1,1); }
- Set up the rendering code to bind the
GLSLShader
shader, pass the uniforms, and then draw the geometry.void OnRender() { glClear(GL_COLOR_BUFFER_BIT|GL_DEPTH_BUFFER_BIT); shader.Use(); glUniformMatrix4fv(shader("MVP"), 1, GL_FALSE, glm::value_ptr(P*MV)); glDrawElements(GL_TRIANGLES, 3, GL_UNSIGNED_SHORT, 0); shader.UnUse(); glutSwapBuffers(); }
- Delete the shader and other OpenGL objects.
void OnShutdown() { shader.DeleteShaderProgram(); glDeleteBuffers(1, &vboVerticesID); glDeleteBuffers(1, &vboIndicesID); glDeleteVertexArrays(1, &vaoID); }
For this simple example, we will only use a vertex shader (shaders/shader.vert
) and a fragment shader (shaders/shader.frag
). The first line in the shader signifies the GLSL version of the shader. Starting from OpenGL v3.0, the version specifiers correspond to the OpenGL version used. So for OpenGL v3.3, the GLSL version is 330. In addition, since we are interested in the core profile, we add another keyword following the version number to signify that we have a core profile shader.
The vertex shader simply outputs the input per-vertex color to the output (vSmoothColor
). Such attributes that are interpolated across shader stages are called varying attributes. It also calculates the clip space position by multiplying the per-vertex position (vVertex
) with the combined modelview projection (MVP) matrix.
The fragment shader writes the input color (vSmoothColor
) to the frame buffer output (vFragColor
).
In the simple triangle demo application code, we store the GLSLShader
object reference in the global scope so that we can access it in any function we desire. We modify the OnInit()
function by adding the following lines:
The first two lines create the GLSL shader of the given type by reading the contents of the file with the given filename. In all of the recipes in this book, the vertex shader files are stored with a .vert
extension, the geometry shader files with a .geom
extension, and the fragment shader files with a .frag
extension. Next, the GLSLShader::CreateAndLinkProgram
function is called to create the shader program from the shader object. Next, the program is bound and then the locations of attributes and uniforms are stored.
In OpenGL v3.3 and above, we typically store the geometry information in buffer objects, which is a linear array of memory managed by the GPU. In order to facilitate the handling of buffer object(s) during rendering, we use a vertex array object (VAO). This object stores references to buffer objects that are bound after the VAO is bound. The advantage we get from using a VAO is that after the VAO is bound, we do not have to bind the buffer object(s).
In this demo, we declare three variables in global scope; vaoID
for VAO handling, and vboVerticesID
and vboIndicesID
for buffer object handling. The VAO object is created by calling the glGenVertexArrays
function. The buffer objects are generated using the glGenBuffers
function. The first parameter for both of these functions is the total number of objects required, and the second parameter is the reference to where the object handle is stored. These functions are called in the OnInit()
function.
In the next few calls, we enable the vertex attributes. This function needs the location of the attribute, which we obtain by the GLSLShader::operator[]
, passing it the name of the attribute whose location we require. We then call glVertexAttributePointer
to tell the GPU how many elements there are and what is their type, whether the attribute is normalized, the stride (which means the total number of bytes to skip to reach the next element; for our case since the attributes are stored in a Vertex
struct, the next element's stride is the size of our Vertex
struct), and finally, the pointer to the attribute in the given array. The last parameter requires explanation in case we have interleaved attributes (as we have). The offsetof
operator returns the offset in bytes, to the attribute in the given struct. Hence, the GPU knows how many bytes it needs to skip in order to access the next attribute of the given type. For the vVertex
attribute, the last parameter is 0
since the next element is accessed immediately after the stride. For the second attribute vColor
, it needs to hop 12 bytes before the next vColor
attribute is obtained from the given vertices array.
To complement the object generation in the OnInit()
function, we must provide the object deletion code. This is handled in the OnShutdown()
function. We first delete the shader program by calling the GLSLShader::DeleteShaderProgram
function. Next, we delete the two buffer objects (vboVerticesID
and vboIndicesID
) and finally we delete the vertex array object (vaoID
).
The rendering code of the simple triangle demo is as follows:
The rendering code first clears the color and depth buffer and binds the shader program by calling the GLSLShader::Use()
function. It then passes the combined modelview and projection matrix to the GPU by invoking the glUniformMatrix4fv
function. The first parameter is the location of the uniform which we obtain from the GLSLShader::operator()
function, by passing it the name of the uniform whose location we need. The second parameter is the total number of matrices we wish to pass. The third parameter is a Boolean signifying if the matrix needs to be transposed, and the final parameter is the float pointer to the matrix object. Here we use the glm::value_ptr
function to get the float pointer from the matrix object. Note that the OpenGL matrices are concatenated right to left since it follows a right handed coordinate system in a column major layout. Hence we keep the projection matrix on the left and the modelview matrix on the right. For this simple example, the modelview matrix (MV
) is set as the identity matrix.
After this function, the glDrawElements
call is made. Since we have left our VAO object (vaoID
) bound, we pass 0
to the final parameter of this function. This tells the GPU to use the references of the GL_ELEMENT_ARRAY_BUFFER
and GL_ARRAY_BUFFER
binding points of the bound VAO. Thus we do not need to explicitly bind the vboVerticesID
and vboIndicesID
buffer objects again. After this call, we unbind the shader program by calling the GLSLShader::UnUse()
function. Finally, we call the glutSwapBuffer
function to show the back buffer on screen. After compiling and running, we get the output as shown in the following figure:
Chapter1/SimpleTriangle
directory.
Let us start this recipe using the following steps:
- Define a vertex shader (
shaders/shader.vert
) to transform the object space vertex position to clip space.#version 330 core layout(location = 0) in vec3 vVertex; layout(location = 1) in vec3 vColor; smooth out vec4 vSmoothColor; uniform mat4 MVP; void main() { vSmoothColor = vec4(vColor,1); gl_Position = MVP*vec4(vVertex,1); }
- Define a fragment shader (
shaders/shader.frag
) to output a smoothly interpolated color from the vertex shader to the frame buffer.#version 330 core smooth in vec4 vSmoothColor; layout(location=0) out vec4 vFragColor; void main() { vFragColor = vSmoothColor; }
- Load the two shaders using the
GLSLShader
class in theOnInit()
function.shader.LoadFromFile(GL_VERTEX_SHADER, "shaders/shader.vert"); shader.LoadFromFile(GL_FRAGMENT_SHADER,"shaders/shader.frag"); shader.CreateAndLinkProgram(); shader.Use(); shader.AddAttribute("vVertex"); shader.AddAttribute("vColor"); shader.AddUniform("MVP"); shader.UnUse();
- Create the geometry and topology. We will store the attributes together in an interleaved vertex format, that is, we will store the vertex attributes in a struct containing two attributes, position and color.
vertices[0].color=glm::vec3(1,0,0); vertices[1].color=glm::vec3(0,1,0); vertices[2].color=glm::vec3(0,0,1); vertices[0].position=glm::vec3(-1,-1,0); vertices[1].position=glm::vec3(0,1,0); vertices[2].position=glm::vec3(1,-1,0); indices[0] = 0; indices[1] = 1; indices[2] = 2;
- Store the geometry and topology in the buffer object(s). The stride parameter controls the number of bytes to jump to reach the next element of the same attribute. For the interleaved format, it is typically the size of our vertex struct in bytes, that is,
sizeof(Vertex)
.glGenVertexArrays(1, &vaoID); glGenBuffers(1, &vboVerticesID); glGenBuffers(1, &vboIndicesID); glBindVertexArray(vaoID); glBindBuffer (GL_ARRAY_BUFFER, vboVerticesID); glBufferData (GL_ARRAY_BUFFER, sizeof(vertices), &vertices[0], GL_STATIC_DRAW); glEnableVertexAttribArray(shader["vVertex"]); glVertexAttribPointer(shader["vVertex"], 3, GL_FLOAT, GL_FALSE,stride,0); glEnableVertexAttribArray(shader["vColor"]); glVertexAttribPointer(shader["vColor"], 3, GL_FLOAT, GL_FALSE,stride, (const GLvoid*)offsetof(Vertex, color)); glBindBuffer(GL_ELEMENT_ARRAY_BUFFER, vboIndicesID); glBufferData(GL_ELEMENT_ARRAY_BUFFER, sizeof(indices), &indices[0], GL_STATIC_DRAW);
- Set up the resize handler to set up the viewport and projection matrix.
void OnResize(int w, int h) { glViewport (0, 0, (GLsizei) w, (GLsizei) h); P = glm::ortho(-1,1,-1,1); }
- Set up the rendering code to bind the
GLSLShader
shader, pass the uniforms, and then draw the geometry.void OnRender() { glClear(GL_COLOR_BUFFER_BIT|GL_DEPTH_BUFFER_BIT); shader.Use(); glUniformMatrix4fv(shader("MVP"), 1, GL_FALSE, glm::value_ptr(P*MV)); glDrawElements(GL_TRIANGLES, 3, GL_UNSIGNED_SHORT, 0); shader.UnUse(); glutSwapBuffers(); }
- Delete the shader and other OpenGL objects.
void OnShutdown() { shader.DeleteShaderProgram(); glDeleteBuffers(1, &vboVerticesID); glDeleteBuffers(1, &vboIndicesID); glDeleteVertexArrays(1, &vaoID); }
For this simple example, we will only use a vertex shader (shaders/shader.vert
) and a fragment shader (shaders/shader.frag
). The first line in the shader signifies the GLSL version of the shader. Starting from OpenGL v3.0, the version specifiers correspond to the OpenGL version used. So for OpenGL v3.3, the GLSL version is 330. In addition, since we are interested in the core profile, we add another keyword following the version number to signify that we have a core profile shader.
The vertex shader simply outputs the input per-vertex color to the output (vSmoothColor
). Such attributes that are interpolated across shader stages are called varying attributes. It also calculates the clip space position by multiplying the per-vertex position (vVertex
) with the combined modelview projection (MVP) matrix.
The fragment shader writes the input color (vSmoothColor
) to the frame buffer output (vFragColor
).
In the simple triangle demo application code, we store the GLSLShader
object reference in the global scope so that we can access it in any function we desire. We modify the OnInit()
function by adding the following lines:
The first two lines create the GLSL shader of the given type by reading the contents of the file with the given filename. In all of the recipes in this book, the vertex shader files are stored with a .vert
extension, the geometry shader files with a .geom
extension, and the fragment shader files with a .frag
extension. Next, the GLSLShader::CreateAndLinkProgram
function is called to create the shader program from the shader object. Next, the program is bound and then the locations of attributes and uniforms are stored.
In OpenGL v3.3 and above, we typically store the geometry information in buffer objects, which is a linear array of memory managed by the GPU. In order to facilitate the handling of buffer object(s) during rendering, we use a vertex array object (VAO). This object stores references to buffer objects that are bound after the VAO is bound. The advantage we get from using a VAO is that after the VAO is bound, we do not have to bind the buffer object(s).
In this demo, we declare three variables in global scope; vaoID
for VAO handling, and vboVerticesID
and vboIndicesID
for buffer object handling. The VAO object is created by calling the glGenVertexArrays
function. The buffer objects are generated using the glGenBuffers
function. The first parameter for both of these functions is the total number of objects required, and the second parameter is the reference to where the object handle is stored. These functions are called in the OnInit()
function.
In the next few calls, we enable the vertex attributes. This function needs the location of the attribute, which we obtain by the GLSLShader::operator[]
, passing it the name of the attribute whose location we require. We then call glVertexAttributePointer
to tell the GPU how many elements there are and what is their type, whether the attribute is normalized, the stride (which means the total number of bytes to skip to reach the next element; for our case since the attributes are stored in a Vertex
struct, the next element's stride is the size of our Vertex
struct), and finally, the pointer to the attribute in the given array. The last parameter requires explanation in case we have interleaved attributes (as we have). The offsetof
operator returns the offset in bytes, to the attribute in the given struct. Hence, the GPU knows how many bytes it needs to skip in order to access the next attribute of the given type. For the vVertex
attribute, the last parameter is 0
since the next element is accessed immediately after the stride. For the second attribute vColor
, it needs to hop 12 bytes before the next vColor
attribute is obtained from the given vertices array.
To complement the object generation in the OnInit()
function, we must provide the object deletion code. This is handled in the OnShutdown()
function. We first delete the shader program by calling the GLSLShader::DeleteShaderProgram
function. Next, we delete the two buffer objects (vboVerticesID
and vboIndicesID
) and finally we delete the vertex array object (vaoID
).
The rendering code of the simple triangle demo is as follows:
The rendering code first clears the color and depth buffer and binds the shader program by calling the GLSLShader::Use()
function. It then passes the combined modelview and projection matrix to the GPU by invoking the glUniformMatrix4fv
function. The first parameter is the location of the uniform which we obtain from the GLSLShader::operator()
function, by passing it the name of the uniform whose location we need. The second parameter is the total number of matrices we wish to pass. The third parameter is a Boolean signifying if the matrix needs to be transposed, and the final parameter is the float pointer to the matrix object. Here we use the glm::value_ptr
function to get the float pointer from the matrix object. Note that the OpenGL matrices are concatenated right to left since it follows a right handed coordinate system in a column major layout. Hence we keep the projection matrix on the left and the modelview matrix on the right. For this simple example, the modelview matrix (MV
) is set as the identity matrix.
After this function, the glDrawElements
call is made. Since we have left our VAO object (vaoID
) bound, we pass 0
to the final parameter of this function. This tells the GPU to use the references of the GL_ELEMENT_ARRAY_BUFFER
and GL_ARRAY_BUFFER
binding points of the bound VAO. Thus we do not need to explicitly bind the vboVerticesID
and vboIndicesID
buffer objects again. After this call, we unbind the shader program by calling the GLSLShader::UnUse()
function. Finally, we call the glutSwapBuffer
function to show the back buffer on screen. After compiling and running, we get the output as shown in the following figure:
this recipe using the following steps:
- Define a vertex shader (
shaders/shader.vert
) to transform the object space vertex position to clip space.#version 330 core layout(location = 0) in vec3 vVertex; layout(location = 1) in vec3 vColor; smooth out vec4 vSmoothColor; uniform mat4 MVP; void main() { vSmoothColor = vec4(vColor,1); gl_Position = MVP*vec4(vVertex,1); }
- Define a fragment shader (
shaders/shader.frag
) to output a smoothly interpolated color from the vertex shader to the frame buffer.#version 330 core smooth in vec4 vSmoothColor; layout(location=0) out vec4 vFragColor; void main() { vFragColor = vSmoothColor; }
- Load the two shaders using the
GLSLShader
class in theOnInit()
function.shader.LoadFromFile(GL_VERTEX_SHADER, "shaders/shader.vert"); shader.LoadFromFile(GL_FRAGMENT_SHADER,"shaders/shader.frag"); shader.CreateAndLinkProgram(); shader.Use(); shader.AddAttribute("vVertex"); shader.AddAttribute("vColor"); shader.AddUniform("MVP"); shader.UnUse();
- Create the geometry and topology. We will store the attributes together in an interleaved vertex format, that is, we will store the vertex attributes in a struct containing two attributes, position and color.
vertices[0].color=glm::vec3(1,0,0); vertices[1].color=glm::vec3(0,1,0); vertices[2].color=glm::vec3(0,0,1); vertices[0].position=glm::vec3(-1,-1,0); vertices[1].position=glm::vec3(0,1,0); vertices[2].position=glm::vec3(1,-1,0); indices[0] = 0; indices[1] = 1; indices[2] = 2;
- Store the geometry and topology in the buffer object(s). The stride parameter controls the number of bytes to jump to reach the next element of the same attribute. For the interleaved format, it is typically the size of our vertex struct in bytes, that is,
sizeof(Vertex)
.glGenVertexArrays(1, &vaoID); glGenBuffers(1, &vboVerticesID); glGenBuffers(1, &vboIndicesID); glBindVertexArray(vaoID); glBindBuffer (GL_ARRAY_BUFFER, vboVerticesID); glBufferData (GL_ARRAY_BUFFER, sizeof(vertices), &vertices[0], GL_STATIC_DRAW); glEnableVertexAttribArray(shader["vVertex"]); glVertexAttribPointer(shader["vVertex"], 3, GL_FLOAT, GL_FALSE,stride,0); glEnableVertexAttribArray(shader["vColor"]); glVertexAttribPointer(shader["vColor"], 3, GL_FLOAT, GL_FALSE,stride, (const GLvoid*)offsetof(Vertex, color)); glBindBuffer(GL_ELEMENT_ARRAY_BUFFER, vboIndicesID); glBufferData(GL_ELEMENT_ARRAY_BUFFER, sizeof(indices), &indices[0], GL_STATIC_DRAW);
- Set up the resize handler to set up the viewport and projection matrix.
void OnResize(int w, int h) { glViewport (0, 0, (GLsizei) w, (GLsizei) h); P = glm::ortho(-1,1,-1,1); }
- Set up the rendering code to bind the
GLSLShader
shader, pass the uniforms, and then draw the geometry.void OnRender() { glClear(GL_COLOR_BUFFER_BIT|GL_DEPTH_BUFFER_BIT); shader.Use(); glUniformMatrix4fv(shader("MVP"), 1, GL_FALSE, glm::value_ptr(P*MV)); glDrawElements(GL_TRIANGLES, 3, GL_UNSIGNED_SHORT, 0); shader.UnUse(); glutSwapBuffers(); }
- Delete the shader and other OpenGL objects.
void OnShutdown() { shader.DeleteShaderProgram(); glDeleteBuffers(1, &vboVerticesID); glDeleteBuffers(1, &vboIndicesID); glDeleteVertexArrays(1, &vaoID); }
For this simple example, we will only use a vertex shader (shaders/shader.vert
) and a fragment shader (shaders/shader.frag
). The first line in the shader signifies the GLSL version of the shader. Starting from OpenGL v3.0, the version specifiers correspond to the OpenGL version used. So for OpenGL v3.3, the GLSL version is 330. In addition, since we are interested in the core profile, we add another keyword following the version number to signify that we have a core profile shader.
The vertex shader simply outputs the input per-vertex color to the output (vSmoothColor
). Such attributes that are interpolated across shader stages are called varying attributes. It also calculates the clip space position by multiplying the per-vertex position (vVertex
) with the combined modelview projection (MVP) matrix.
The fragment shader writes the input color (vSmoothColor
) to the frame buffer output (vFragColor
).
In the simple triangle demo application code, we store the GLSLShader
object reference in the global scope so that we can access it in any function we desire. We modify the OnInit()
function by adding the following lines:
The first two lines create the GLSL shader of the given type by reading the contents of the file with the given filename. In all of the recipes in this book, the vertex shader files are stored with a .vert
extension, the geometry shader files with a .geom
extension, and the fragment shader files with a .frag
extension. Next, the GLSLShader::CreateAndLinkProgram
function is called to create the shader program from the shader object. Next, the program is bound and then the locations of attributes and uniforms are stored.
In OpenGL v3.3 and above, we typically store the geometry information in buffer objects, which is a linear array of memory managed by the GPU. In order to facilitate the handling of buffer object(s) during rendering, we use a vertex array object (VAO). This object stores references to buffer objects that are bound after the VAO is bound. The advantage we get from using a VAO is that after the VAO is bound, we do not have to bind the buffer object(s).
In this demo, we declare three variables in global scope; vaoID
for VAO handling, and vboVerticesID
and vboIndicesID
for buffer object handling. The VAO object is created by calling the glGenVertexArrays
function. The buffer objects are generated using the glGenBuffers
function. The first parameter for both of these functions is the total number of objects required, and the second parameter is the reference to where the object handle is stored. These functions are called in the OnInit()
function.
In the next few calls, we enable the vertex attributes. This function needs the location of the attribute, which we obtain by the GLSLShader::operator[]
, passing it the name of the attribute whose location we require. We then call glVertexAttributePointer
to tell the GPU how many elements there are and what is their type, whether the attribute is normalized, the stride (which means the total number of bytes to skip to reach the next element; for our case since the attributes are stored in a Vertex
struct, the next element's stride is the size of our Vertex
struct), and finally, the pointer to the attribute in the given array. The last parameter requires explanation in case we have interleaved attributes (as we have). The offsetof
operator returns the offset in bytes, to the attribute in the given struct. Hence, the GPU knows how many bytes it needs to skip in order to access the next attribute of the given type. For the vVertex
attribute, the last parameter is 0
since the next element is accessed immediately after the stride. For the second attribute vColor
, it needs to hop 12 bytes before the next vColor
attribute is obtained from the given vertices array.
To complement the object generation in the OnInit()
function, we must provide the object deletion code. This is handled in the OnShutdown()
function. We first delete the shader program by calling the GLSLShader::DeleteShaderProgram
function. Next, we delete the two buffer objects (vboVerticesID
and vboIndicesID
) and finally we delete the vertex array object (vaoID
).
The rendering code of the simple triangle demo is as follows:
The rendering code first clears the color and depth buffer and binds the shader program by calling the GLSLShader::Use()
function. It then passes the combined modelview and projection matrix to the GPU by invoking the glUniformMatrix4fv
function. The first parameter is the location of the uniform which we obtain from the GLSLShader::operator()
function, by passing it the name of the uniform whose location we need. The second parameter is the total number of matrices we wish to pass. The third parameter is a Boolean signifying if the matrix needs to be transposed, and the final parameter is the float pointer to the matrix object. Here we use the glm::value_ptr
function to get the float pointer from the matrix object. Note that the OpenGL matrices are concatenated right to left since it follows a right handed coordinate system in a column major layout. Hence we keep the projection matrix on the left and the modelview matrix on the right. For this simple example, the modelview matrix (MV
) is set as the identity matrix.
After this function, the glDrawElements
call is made. Since we have left our VAO object (vaoID
) bound, we pass 0
to the final parameter of this function. This tells the GPU to use the references of the GL_ELEMENT_ARRAY_BUFFER
and GL_ARRAY_BUFFER
binding points of the bound VAO. Thus we do not need to explicitly bind the vboVerticesID
and vboIndicesID
buffer objects again. After this call, we unbind the shader program by calling the GLSLShader::UnUse()
function. Finally, we call the glutSwapBuffer
function to show the back buffer on screen. After compiling and running, we get the output as shown in the following figure:
simple example, we will only use a vertex shader (shaders/shader.vert
) and a fragment shader (shaders/shader.frag
). The first line in the shader signifies the GLSL version of the shader. Starting from OpenGL v3.0, the version specifiers correspond to the OpenGL version used. So for OpenGL v3.3, the GLSL version is 330. In addition, since we are interested in the core profile, we add another keyword following the version number to signify that we have a core profile shader.
The vertex shader simply outputs the input per-vertex color to the output (vSmoothColor
). Such attributes that are interpolated across shader stages are called varying attributes. It also calculates the clip space position by multiplying the per-vertex position (vVertex
) with the combined modelview projection (MVP) matrix.
The fragment shader writes the input color (vSmoothColor
) to the frame buffer output (vFragColor
).
In the simple triangle demo application code, we store the GLSLShader
object reference in the global scope so that we can access it in any function we desire. We modify the OnInit()
function by adding the following lines:
The first two lines create the GLSL shader of the given type by reading the contents of the file with the given filename. In all of the recipes in this book, the vertex shader files are stored with a .vert
extension, the geometry shader files with a .geom
extension, and the fragment shader files with a .frag
extension. Next, the GLSLShader::CreateAndLinkProgram
function is called to create the shader program from the shader object. Next, the program is bound and then the locations of attributes and uniforms are stored.
In OpenGL v3.3 and above, we typically store the geometry information in buffer objects, which is a linear array of memory managed by the GPU. In order to facilitate the handling of buffer object(s) during rendering, we use a vertex array object (VAO). This object stores references to buffer objects that are bound after the VAO is bound. The advantage we get from using a VAO is that after the VAO is bound, we do not have to bind the buffer object(s).
In this demo, we declare three variables in global scope; vaoID
for VAO handling, and vboVerticesID
and vboIndicesID
for buffer object handling. The VAO object is created by calling the glGenVertexArrays
function. The buffer objects are generated using the glGenBuffers
function. The first parameter for both of these functions is the total number of objects required, and the second parameter is the reference to where the object handle is stored. These functions are called in the OnInit()
function.
In the next few calls, we enable the vertex attributes. This function needs the location of the attribute, which we obtain by the GLSLShader::operator[]
, passing it the name of the attribute whose location we require. We then call glVertexAttributePointer
to tell the GPU how many elements there are and what is their type, whether the attribute is normalized, the stride (which means the total number of bytes to skip to reach the next element; for our case since the attributes are stored in a Vertex
struct, the next element's stride is the size of our Vertex
struct), and finally, the pointer to the attribute in the given array. The last parameter requires explanation in case we have interleaved attributes (as we have). The offsetof
operator returns the offset in bytes, to the attribute in the given struct. Hence, the GPU knows how many bytes it needs to skip in order to access the next attribute of the given type. For the vVertex
attribute, the last parameter is 0
since the next element is accessed immediately after the stride. For the second attribute vColor
, it needs to hop 12 bytes before the next vColor
attribute is obtained from the given vertices array.
To complement the object generation in the OnInit()
function, we must provide the object deletion code. This is handled in the OnShutdown()
function. We first delete the shader program by calling the GLSLShader::DeleteShaderProgram
function. Next, we delete the two buffer objects (vboVerticesID
and vboIndicesID
) and finally we delete the vertex array object (vaoID
).
The rendering code of the simple triangle demo is as follows:
The rendering code first clears the color and depth buffer and binds the shader program by calling the GLSLShader::Use()
function. It then passes the combined modelview and projection matrix to the GPU by invoking the glUniformMatrix4fv
function. The first parameter is the location of the uniform which we obtain from the GLSLShader::operator()
function, by passing it the name of the uniform whose location we need. The second parameter is the total number of matrices we wish to pass. The third parameter is a Boolean signifying if the matrix needs to be transposed, and the final parameter is the float pointer to the matrix object. Here we use the glm::value_ptr
function to get the float pointer from the matrix object. Note that the OpenGL matrices are concatenated right to left since it follows a right handed coordinate system in a column major layout. Hence we keep the projection matrix on the left and the modelview matrix on the right. For this simple example, the modelview matrix (MV
) is set as the identity matrix.
After this function, the glDrawElements
call is made. Since we have left our VAO object (vaoID
) bound, we pass 0
to the final parameter of this function. This tells the GPU to use the references of the GL_ELEMENT_ARRAY_BUFFER
and GL_ARRAY_BUFFER
binding points of the bound VAO. Thus we do not need to explicitly bind the vboVerticesID
and vboIndicesID
buffer objects again. After this call, we unbind the shader program by calling the GLSLShader::UnUse()
function. Finally, we call the glutSwapBuffer
function to show the back buffer on screen. After compiling and running, we get the output as shown in the following figure:
demo application code, we store the GLSLShader
object reference in the global scope so that we can access it in any function we desire. We modify the OnInit()
function by adding the following lines:
The first two lines create the GLSL shader of the given type by reading the contents of the file with the given filename. In all of the recipes in this book, the vertex shader files are stored with a .vert
extension, the geometry shader files with a .geom
extension, and the fragment shader files with a .frag
extension. Next, the GLSLShader::CreateAndLinkProgram
function is called to create the shader program from the shader object. Next, the program is bound and then the locations of attributes and uniforms are stored.
In OpenGL v3.3 and above, we typically store the geometry information in buffer objects, which is a linear array of memory managed by the GPU. In order to facilitate the handling of buffer object(s) during rendering, we use a vertex array object (VAO). This object stores references to buffer objects that are bound after the VAO is bound. The advantage we get from using a VAO is that after the VAO is bound, we do not have to bind the buffer object(s).
In this demo, we declare three variables in global scope; vaoID
for VAO handling, and vboVerticesID
and vboIndicesID
for buffer object handling. The VAO object is created by calling the glGenVertexArrays
function. The buffer objects are generated using the glGenBuffers
function. The first parameter for both of these functions is the total number of objects required, and the second parameter is the reference to where the object handle is stored. These functions are called in the OnInit()
function.
In the next few calls, we enable the vertex attributes. This function needs the location of the attribute, which we obtain by the GLSLShader::operator[]
, passing it the name of the attribute whose location we require. We then call glVertexAttributePointer
to tell the GPU how many elements there are and what is their type, whether the attribute is normalized, the stride (which means the total number of bytes to skip to reach the next element; for our case since the attributes are stored in a Vertex
struct, the next element's stride is the size of our Vertex
struct), and finally, the pointer to the attribute in the given array. The last parameter requires explanation in case we have interleaved attributes (as we have). The offsetof
operator returns the offset in bytes, to the attribute in the given struct. Hence, the GPU knows how many bytes it needs to skip in order to access the next attribute of the given type. For the vVertex
attribute, the last parameter is 0
since the next element is accessed immediately after the stride. For the second attribute vColor
, it needs to hop 12 bytes before the next vColor
attribute is obtained from the given vertices array.
To complement the object generation in the OnInit()
function, we must provide the object deletion code. This is handled in the OnShutdown()
function. We first delete the shader program by calling the GLSLShader::DeleteShaderProgram
function. Next, we delete the two buffer objects (vboVerticesID
and vboIndicesID
) and finally we delete the vertex array object (vaoID
).
The rendering code of the simple triangle demo is as follows:
The rendering code first clears the color and depth buffer and binds the shader program by calling the GLSLShader::Use()
function. It then passes the combined modelview and projection matrix to the GPU by invoking the glUniformMatrix4fv
function. The first parameter is the location of the uniform which we obtain from the GLSLShader::operator()
function, by passing it the name of the uniform whose location we need. The second parameter is the total number of matrices we wish to pass. The third parameter is a Boolean signifying if the matrix needs to be transposed, and the final parameter is the float pointer to the matrix object. Here we use the glm::value_ptr
function to get the float pointer from the matrix object. Note that the OpenGL matrices are concatenated right to left since it follows a right handed coordinate system in a column major layout. Hence we keep the projection matrix on the left and the modelview matrix on the right. For this simple example, the modelview matrix (MV
) is set as the identity matrix.
After this function, the glDrawElements
call is made. Since we have left our VAO object (vaoID
) bound, we pass 0
to the final parameter of this function. This tells the GPU to use the references of the GL_ELEMENT_ARRAY_BUFFER
and GL_ARRAY_BUFFER
binding points of the bound VAO. Thus we do not need to explicitly bind the vboVerticesID
and vboIndicesID
buffer objects again. After this call, we unbind the shader program by calling the GLSLShader::UnUse()
function. Finally, we call the glutSwapBuffer
function to show the back buffer on screen. After compiling and running, we get the output as shown in the following figure:
In this recipe, we will deform a planar mesh using the vertex shader. We know that the vertex shader is responsible for outputting the clip space position of the given object space vertex. In between this conversion, we can apply the modeling transformation to transform the given object space vertex to world space position.
We can implement a ripple shader using the following steps:
- Define the vertex shader that deforms the object space vertex position.
#version 330 core layout(location=0) in vec3 vVertex; uniform mat4 MVP; uniform float time; const float amplitude = 0.125; const float frequency = 4; const float PI = 3.14159; void main() { float distance = length(vVertex); float y = amplitude*sin(-PI*distance*frequency+time); gl_Position = MVP*vec4(vVertex.x, y, vVertex.z,1); }
- Define a fragment shader that simply outputs a constant color.
#version 330 core layout(location=0) out vec4 vFragColor; void main() { vFragColor = vec4(1,1,1,1); }
- Load the two shaders using the
GLSLShader
class in theOnInit()
function.shader.LoadFromFile(GL_VERTEX_SHADER, "shaders/shader.vert"); shader.LoadFromFile(GL_FRAGMENT_SHADER, "shaders/shader.frag"); shader.CreateAndLinkProgram(); shader.Use(); shader.AddAttribute("vVertex"); shader.AddUniform("MVP"); shader.AddUniform("time"); shader.UnUse();
- Create the geometry and topology.
int count = 0; int i=0, j=0; for( j=0;j<=NUM_Z;j++) { for( i=0;i<=NUM_X;i++) { vertices[count++] = glm::vec3( ((float(i)/(NUM_X-1)) *2-1)* HALF_SIZE_X, 0, ((float(j)/(NUM_Z-1))*2-1)*HALF_SIZE_Z); } } GLushort* id=&indices[0]; for (i = 0; i < NUM_Z; i++) { for (j = 0; j < NUM_X; j++) { int i0 = i * (NUM_X+1) + j; int i1 = i0 + 1; int i2 = i0 + (NUM_X+1); int i3 = i2 + 1; if ((j+i)%2) { *id++ = i0; *id++ = i2; *id++ = i1; *id++ = i1; *id++ = i2; *id++ = i3; } else { *id++ = i0; *id++ = i2; *id++ = i3; *id++ = i0; *id++ = i3; *id++ = i1; } } }
- Store the geometry and topology in the buffer object(s).
glGenVertexArrays(1, &vaoID); glGenBuffers(1, &vboVerticesID); glGenBuffers(1, &vboIndicesID); glBindVertexArray(vaoID); glBindBuffer (GL_ARRAY_BUFFER, vboVerticesID); glBufferData (GL_ARRAY_BUFFER, sizeof(vertices), &vertices[0], GL_STATIC_DRAW); glEnableVertexAttribArray(shader["vVertex"]); glVertexAttribPointer(shader["vVertex"], 3, GL_FLOAT, GL_FALSE,0,0); glBindBuffer(GL_ELEMENT_ARRAY_BUFFER, vboIndicesID); glBufferData(GL_ELEMENT_ARRAY_BUFFER, sizeof(indices), &indices[0], GL_STATIC_DRAW);
- Set up the perspective projection matrix in the resize handler.
P = glm::perspective(45.0f, (GLfloat)w/h, 1.f, 1000.f);
- Set up the rendering code to bind the
GLSLShader
shader, pass the uniforms and then draw the geometry.void OnRender() { time = glutGet(GLUT_ELAPSED_TIME)/1000.0f * SPEED; glm::mat4 T=glm::translate(glm::mat4(1.0f), glm::vec3(0.0f, 0.0f, dist)); glm::mat4 Rx= glm::rotate(T, rX, glm::vec3(1.0f, 0.0f, 0.0f)); glm::mat4 MV= glm::rotate(Rx, rY, glm::vec3(0.0f, 1.0f, 0.0f)); glm::mat4 MVP= P*MV; shader.Use(); glUniformMatrix4fv(shader("MVP"), 1, GL_FALSE, glm::value_ptr(MVP)); glUniform1f(shader("time"), time); glDrawElements(GL_TRIANGLES,TOTAL_INDICES,GL_UNSIGNED_SHORT,0); shader.UnUse(); glutSwapBuffers(); }
- Delete the shader and other OpenGL objects.
void OnShutdown() { shader.DeleteShaderProgram(); glDeleteBuffers(1, &vboVerticesID); glDeleteBuffers(1, &vboIndicesID); glDeleteVertexArrays(1, &vaoID); }
In this recipe, the only attribute passed in is the per-vertex position (vVertex
). There are two uniforms: the combined modelview projection matrix (MVP
) and the current time (time
). We will use the time
uniform to allow progression of the deformer so we can observe the ripple movement. After these declarations are three constants, namely amplitude
(which controls how much the ripple moves up and down from the zero base line), frequency
(which controls the total number of waves), and PI
(a constant used in the wave formula). Note that we could have replaced the constants with uniforms and had them modified from the application code.
In our formula, we first find the distance (d
) of the vertex from the origin by using the Euclidean distance formula. This is given to us by the length
built-in GLSL function. Next, we input the distance into the sin
function multiplying the distance by the frequency (f
) and (π
). In our vertex shader, we replace the phase (φ
) with time.
Similar to the previous recipe, we declare the GLSLShader
object in the global scope to allow maximum visibility. Next, we initialize the GLSLShader
object in the OnInit()
function.
The only difference in this recipe is the addition of an additional uniform (time
).
This sort of topology can be generated using the following code:
In order to alternate the triangle directions and maintain their winding order, we take two different combinations, one for an even iteration and second for an odd iteration. This can be achieved using the following code:
After filling the vertices and indices arrays, we push this data to the GPU memory. We first create a vertex array object (vaoID
) and two buffer objects, the GL_ARRAY_BUFFER
binding for vertices and the GL_ELEMENT_ARRAY_BUFFER
binding for the indices array. These calls are exactly the same as in the previous recipe. The only difference is that now we only have a single per-vertex attribute, that is, the vertex position (vVertex
). The OnShutdown()
function is also unchanged as in the previous recipe.
Note that the matrix multiplication in glm
follows from right to left. So the order in which we generate the transformations will be applied in the reverse order. In our case the combined modelview matrix will be calculated as MV = (T*(Rx*Ry))
. The translation amount, dist
, and the rotation values, rX
and rY
, are calculated in the mouse input functions based on the user's input.
The OnMouseMove
function is defined as follows:
The OnMouseMove
function has only two parameters, the x
and y
screen location where the mouse currently is. The mouse move event is raised whenever the mouse enters and moves in the application window. Based on the state set in the OnMouseDown
function, we calculate the zoom amount (dist
) if the middle mouse button is pressed. Otherwise, we calculate the two rotation amounts (rX
and rY
). Next, we update the oldX
and oldY
positions for the next event. Finally we request the freeglut framework to repaint our application window by calling glutPostRedisplay()
function. This call sends the repaint event which re-renders our scene.
Chapter1\RippleDeformer
directory.
We can implement a ripple shader using the following steps:
- Define the vertex shader that deforms the object space vertex position.
#version 330 core layout(location=0) in vec3 vVertex; uniform mat4 MVP; uniform float time; const float amplitude = 0.125; const float frequency = 4; const float PI = 3.14159; void main() { float distance = length(vVertex); float y = amplitude*sin(-PI*distance*frequency+time); gl_Position = MVP*vec4(vVertex.x, y, vVertex.z,1); }
- Define a fragment shader that simply outputs a constant color.
#version 330 core layout(location=0) out vec4 vFragColor; void main() { vFragColor = vec4(1,1,1,1); }
- Load the two shaders using the
GLSLShader
class in theOnInit()
function.shader.LoadFromFile(GL_VERTEX_SHADER, "shaders/shader.vert"); shader.LoadFromFile(GL_FRAGMENT_SHADER, "shaders/shader.frag"); shader.CreateAndLinkProgram(); shader.Use(); shader.AddAttribute("vVertex"); shader.AddUniform("MVP"); shader.AddUniform("time"); shader.UnUse();
- Create the geometry and topology.
int count = 0; int i=0, j=0; for( j=0;j<=NUM_Z;j++) { for( i=0;i<=NUM_X;i++) { vertices[count++] = glm::vec3( ((float(i)/(NUM_X-1)) *2-1)* HALF_SIZE_X, 0, ((float(j)/(NUM_Z-1))*2-1)*HALF_SIZE_Z); } } GLushort* id=&indices[0]; for (i = 0; i < NUM_Z; i++) { for (j = 0; j < NUM_X; j++) { int i0 = i * (NUM_X+1) + j; int i1 = i0 + 1; int i2 = i0 + (NUM_X+1); int i3 = i2 + 1; if ((j+i)%2) { *id++ = i0; *id++ = i2; *id++ = i1; *id++ = i1; *id++ = i2; *id++ = i3; } else { *id++ = i0; *id++ = i2; *id++ = i3; *id++ = i0; *id++ = i3; *id++ = i1; } } }
- Store the geometry and topology in the buffer object(s).
glGenVertexArrays(1, &vaoID); glGenBuffers(1, &vboVerticesID); glGenBuffers(1, &vboIndicesID); glBindVertexArray(vaoID); glBindBuffer (GL_ARRAY_BUFFER, vboVerticesID); glBufferData (GL_ARRAY_BUFFER, sizeof(vertices), &vertices[0], GL_STATIC_DRAW); glEnableVertexAttribArray(shader["vVertex"]); glVertexAttribPointer(shader["vVertex"], 3, GL_FLOAT, GL_FALSE,0,0); glBindBuffer(GL_ELEMENT_ARRAY_BUFFER, vboIndicesID); glBufferData(GL_ELEMENT_ARRAY_BUFFER, sizeof(indices), &indices[0], GL_STATIC_DRAW);
- Set up the perspective projection matrix in the resize handler.
P = glm::perspective(45.0f, (GLfloat)w/h, 1.f, 1000.f);
- Set up the rendering code to bind the
GLSLShader
shader, pass the uniforms and then draw the geometry.void OnRender() { time = glutGet(GLUT_ELAPSED_TIME)/1000.0f * SPEED; glm::mat4 T=glm::translate(glm::mat4(1.0f), glm::vec3(0.0f, 0.0f, dist)); glm::mat4 Rx= glm::rotate(T, rX, glm::vec3(1.0f, 0.0f, 0.0f)); glm::mat4 MV= glm::rotate(Rx, rY, glm::vec3(0.0f, 1.0f, 0.0f)); glm::mat4 MVP= P*MV; shader.Use(); glUniformMatrix4fv(shader("MVP"), 1, GL_FALSE, glm::value_ptr(MVP)); glUniform1f(shader("time"), time); glDrawElements(GL_TRIANGLES,TOTAL_INDICES,GL_UNSIGNED_SHORT,0); shader.UnUse(); glutSwapBuffers(); }
- Delete the shader and other OpenGL objects.
void OnShutdown() { shader.DeleteShaderProgram(); glDeleteBuffers(1, &vboVerticesID); glDeleteBuffers(1, &vboIndicesID); glDeleteVertexArrays(1, &vaoID); }
In this recipe, the only attribute passed in is the per-vertex position (vVertex
). There are two uniforms: the combined modelview projection matrix (MVP
) and the current time (time
). We will use the time
uniform to allow progression of the deformer so we can observe the ripple movement. After these declarations are three constants, namely amplitude
(which controls how much the ripple moves up and down from the zero base line), frequency
(which controls the total number of waves), and PI
(a constant used in the wave formula). Note that we could have replaced the constants with uniforms and had them modified from the application code.
In our formula, we first find the distance (d
) of the vertex from the origin by using the Euclidean distance formula. This is given to us by the length
built-in GLSL function. Next, we input the distance into the sin
function multiplying the distance by the frequency (f
) and (π
). In our vertex shader, we replace the phase (φ
) with time.
Similar to the previous recipe, we declare the GLSLShader
object in the global scope to allow maximum visibility. Next, we initialize the GLSLShader
object in the OnInit()
function.
The only difference in this recipe is the addition of an additional uniform (time
).
This sort of topology can be generated using the following code:
In order to alternate the triangle directions and maintain their winding order, we take two different combinations, one for an even iteration and second for an odd iteration. This can be achieved using the following code:
After filling the vertices and indices arrays, we push this data to the GPU memory. We first create a vertex array object (vaoID
) and two buffer objects, the GL_ARRAY_BUFFER
binding for vertices and the GL_ELEMENT_ARRAY_BUFFER
binding for the indices array. These calls are exactly the same as in the previous recipe. The only difference is that now we only have a single per-vertex attribute, that is, the vertex position (vVertex
). The OnShutdown()
function is also unchanged as in the previous recipe.
Note that the matrix multiplication in glm
follows from right to left. So the order in which we generate the transformations will be applied in the reverse order. In our case the combined modelview matrix will be calculated as MV = (T*(Rx*Ry))
. The translation amount, dist
, and the rotation values, rX
and rY
, are calculated in the mouse input functions based on the user's input.
The OnMouseMove
function is defined as follows:
The OnMouseMove
function has only two parameters, the x
and y
screen location where the mouse currently is. The mouse move event is raised whenever the mouse enters and moves in the application window. Based on the state set in the OnMouseDown
function, we calculate the zoom amount (dist
) if the middle mouse button is pressed. Otherwise, we calculate the two rotation amounts (rX
and rY
). Next, we update the oldX
and oldY
positions for the next event. Finally we request the freeglut framework to repaint our application window by calling glutPostRedisplay()
function. This call sends the repaint event which re-renders our scene.
#version 330 core layout(location=0) in vec3 vVertex; uniform mat4 MVP; uniform float time; const float amplitude = 0.125; const float frequency = 4; const float PI = 3.14159; void main() { float distance = length(vVertex); float y = amplitude*sin(-PI*distance*frequency+time); gl_Position = MVP*vec4(vVertex.x, y, vVertex.z,1); }
- fragment shader that simply outputs a constant color.
#version 330 core layout(location=0) out vec4 vFragColor; void main() { vFragColor = vec4(1,1,1,1); }
- Load the two shaders using the
GLSLShader
class in theOnInit()
function.shader.LoadFromFile(GL_VERTEX_SHADER, "shaders/shader.vert"); shader.LoadFromFile(GL_FRAGMENT_SHADER, "shaders/shader.frag"); shader.CreateAndLinkProgram(); shader.Use(); shader.AddAttribute("vVertex"); shader.AddUniform("MVP"); shader.AddUniform("time"); shader.UnUse();
- Create the geometry and topology.
int count = 0; int i=0, j=0; for( j=0;j<=NUM_Z;j++) { for( i=0;i<=NUM_X;i++) { vertices[count++] = glm::vec3( ((float(i)/(NUM_X-1)) *2-1)* HALF_SIZE_X, 0, ((float(j)/(NUM_Z-1))*2-1)*HALF_SIZE_Z); } } GLushort* id=&indices[0]; for (i = 0; i < NUM_Z; i++) { for (j = 0; j < NUM_X; j++) { int i0 = i * (NUM_X+1) + j; int i1 = i0 + 1; int i2 = i0 + (NUM_X+1); int i3 = i2 + 1; if ((j+i)%2) { *id++ = i0; *id++ = i2; *id++ = i1; *id++ = i1; *id++ = i2; *id++ = i3; } else { *id++ = i0; *id++ = i2; *id++ = i3; *id++ = i0; *id++ = i3; *id++ = i1; } } }
- Store the geometry and topology in the buffer object(s).
glGenVertexArrays(1, &vaoID); glGenBuffers(1, &vboVerticesID); glGenBuffers(1, &vboIndicesID); glBindVertexArray(vaoID); glBindBuffer (GL_ARRAY_BUFFER, vboVerticesID); glBufferData (GL_ARRAY_BUFFER, sizeof(vertices), &vertices[0], GL_STATIC_DRAW); glEnableVertexAttribArray(shader["vVertex"]); glVertexAttribPointer(shader["vVertex"], 3, GL_FLOAT, GL_FALSE,0,0); glBindBuffer(GL_ELEMENT_ARRAY_BUFFER, vboIndicesID); glBufferData(GL_ELEMENT_ARRAY_BUFFER, sizeof(indices), &indices[0], GL_STATIC_DRAW);
- Set up the perspective projection matrix in the resize handler.
P = glm::perspective(45.0f, (GLfloat)w/h, 1.f, 1000.f);
- Set up the rendering code to bind the
GLSLShader
shader, pass the uniforms and then draw the geometry.void OnRender() { time = glutGet(GLUT_ELAPSED_TIME)/1000.0f * SPEED; glm::mat4 T=glm::translate(glm::mat4(1.0f), glm::vec3(0.0f, 0.0f, dist)); glm::mat4 Rx= glm::rotate(T, rX, glm::vec3(1.0f, 0.0f, 0.0f)); glm::mat4 MV= glm::rotate(Rx, rY, glm::vec3(0.0f, 1.0f, 0.0f)); glm::mat4 MVP= P*MV; shader.Use(); glUniformMatrix4fv(shader("MVP"), 1, GL_FALSE, glm::value_ptr(MVP)); glUniform1f(shader("time"), time); glDrawElements(GL_TRIANGLES,TOTAL_INDICES,GL_UNSIGNED_SHORT,0); shader.UnUse(); glutSwapBuffers(); }
- Delete the shader and other OpenGL objects.
void OnShutdown() { shader.DeleteShaderProgram(); glDeleteBuffers(1, &vboVerticesID); glDeleteBuffers(1, &vboIndicesID); glDeleteVertexArrays(1, &vaoID); }
In this recipe, the only attribute passed in is the per-vertex position (vVertex
). There are two uniforms: the combined modelview projection matrix (MVP
) and the current time (time
). We will use the time
uniform to allow progression of the deformer so we can observe the ripple movement. After these declarations are three constants, namely amplitude
(which controls how much the ripple moves up and down from the zero base line), frequency
(which controls the total number of waves), and PI
(a constant used in the wave formula). Note that we could have replaced the constants with uniforms and had them modified from the application code.
In our formula, we first find the distance (d
) of the vertex from the origin by using the Euclidean distance formula. This is given to us by the length
built-in GLSL function. Next, we input the distance into the sin
function multiplying the distance by the frequency (f
) and (π
). In our vertex shader, we replace the phase (φ
) with time.
Similar to the previous recipe, we declare the GLSLShader
object in the global scope to allow maximum visibility. Next, we initialize the GLSLShader
object in the OnInit()
function.
The only difference in this recipe is the addition of an additional uniform (time
).
This sort of topology can be generated using the following code:
In order to alternate the triangle directions and maintain their winding order, we take two different combinations, one for an even iteration and second for an odd iteration. This can be achieved using the following code:
After filling the vertices and indices arrays, we push this data to the GPU memory. We first create a vertex array object (vaoID
) and two buffer objects, the GL_ARRAY_BUFFER
binding for vertices and the GL_ELEMENT_ARRAY_BUFFER
binding for the indices array. These calls are exactly the same as in the previous recipe. The only difference is that now we only have a single per-vertex attribute, that is, the vertex position (vVertex
). The OnShutdown()
function is also unchanged as in the previous recipe.
Note that the matrix multiplication in glm
follows from right to left. So the order in which we generate the transformations will be applied in the reverse order. In our case the combined modelview matrix will be calculated as MV = (T*(Rx*Ry))
. The translation amount, dist
, and the rotation values, rX
and rY
, are calculated in the mouse input functions based on the user's input.
The OnMouseMove
function is defined as follows:
The OnMouseMove
function has only two parameters, the x
and y
screen location where the mouse currently is. The mouse move event is raised whenever the mouse enters and moves in the application window. Based on the state set in the OnMouseDown
function, we calculate the zoom amount (dist
) if the middle mouse button is pressed. Otherwise, we calculate the two rotation amounts (rX
and rY
). Next, we update the oldX
and oldY
positions for the next event. Finally we request the freeglut framework to repaint our application window by calling glutPostRedisplay()
function. This call sends the repaint event which re-renders our scene.
only attribute passed in is the per-vertex position (vVertex
). There are two uniforms: the combined modelview projection matrix (MVP
) and the current time (time
). We will use the time
uniform to allow progression of the deformer so we can observe the ripple movement. After these declarations are three constants, namely amplitude
(which controls how much the ripple moves up and down from the zero base line), frequency
(which controls the total number of waves), and PI
(a constant used in the wave formula). Note that we could have replaced the constants with uniforms and had them modified from the application code.
In our formula, we first find the distance (d
) of the vertex from the origin by using the Euclidean distance formula. This is given to us by the length
built-in GLSL function. Next, we input the distance into the sin
function multiplying the distance by the frequency (f
) and (π
). In our vertex shader, we replace the phase (φ
) with time.
Similar to the previous recipe, we declare the GLSLShader
object in the global scope to allow maximum visibility. Next, we initialize the GLSLShader
object in the OnInit()
function.
The only difference in this recipe is the addition of an additional uniform (time
).
This sort of topology can be generated using the following code:
In order to alternate the triangle directions and maintain their winding order, we take two different combinations, one for an even iteration and second for an odd iteration. This can be achieved using the following code:
After filling the vertices and indices arrays, we push this data to the GPU memory. We first create a vertex array object (vaoID
) and two buffer objects, the GL_ARRAY_BUFFER
binding for vertices and the GL_ELEMENT_ARRAY_BUFFER
binding for the indices array. These calls are exactly the same as in the previous recipe. The only difference is that now we only have a single per-vertex attribute, that is, the vertex position (vVertex
). The OnShutdown()
function is also unchanged as in the previous recipe.
Note that the matrix multiplication in glm
follows from right to left. So the order in which we generate the transformations will be applied in the reverse order. In our case the combined modelview matrix will be calculated as MV = (T*(Rx*Ry))
. The translation amount, dist
, and the rotation values, rX
and rY
, are calculated in the mouse input functions based on the user's input.
The OnMouseMove
function is defined as follows:
The OnMouseMove
function has only two parameters, the x
and y
screen location where the mouse currently is. The mouse move event is raised whenever the mouse enters and moves in the application window. Based on the state set in the OnMouseDown
function, we calculate the zoom amount (dist
) if the middle mouse button is pressed. Otherwise, we calculate the two rotation amounts (rX
and rY
). Next, we update the oldX
and oldY
positions for the next event. Finally we request the freeglut framework to repaint our application window by calling glutPostRedisplay()
function. This call sends the repaint event which re-renders our scene.
previous recipe, we declare the GLSLShader
object in the global scope to allow maximum visibility. Next, we initialize the GLSLShader
object in the OnInit()
function.
The only difference in this recipe is the addition of an additional uniform (time
).
This sort of topology can be generated using the following code:
In order to alternate the triangle directions and maintain their winding order, we take two different combinations, one for an even iteration and second for an odd iteration. This can be achieved using the following code:
After filling the vertices and indices arrays, we push this data to the GPU memory. We first create a vertex array object (vaoID
) and two buffer objects, the GL_ARRAY_BUFFER
binding for vertices and the GL_ELEMENT_ARRAY_BUFFER
binding for the indices array. These calls are exactly the same as in the previous recipe. The only difference is that now we only have a single per-vertex attribute, that is, the vertex position (vVertex
). The OnShutdown()
function is also unchanged as in the previous recipe.
Note that the matrix multiplication in glm
follows from right to left. So the order in which we generate the transformations will be applied in the reverse order. In our case the combined modelview matrix will be calculated as MV = (T*(Rx*Ry))
. The translation amount, dist
, and the rotation values, rX
and rY
, are calculated in the mouse input functions based on the user's input.
The OnMouseMove
function is defined as follows:
The OnMouseMove
function has only two parameters, the x
and y
screen location where the mouse currently is. The mouse move event is raised whenever the mouse enters and moves in the application window. Based on the state set in the OnMouseDown
function, we calculate the zoom amount (dist
) if the middle mouse button is pressed. Otherwise, we calculate the two rotation amounts (rX
and rY
). Next, we update the oldX
and oldY
positions for the next event. Finally we request the freeglut framework to repaint our application window by calling glutPostRedisplay()
function. This call sends the repaint event which re-renders our scene.
After the vertex shader, the next programmable stage in the OpenGL v3.3 graphics pipeline is the geometry shader. This shader contains inputs from the vertex shader stage. We can either feed these unmodified to the next shader stage or we can add/omit/modify vertices and primitives as desired. One thing that the vertex shaders lack is the availability of the other vertices of the primitive. Geometry shaders have information of all on the vertices of a single primitive.
In this recipe, we will dynamically subdivide a planar mesh using the geometry shader.
This recipe assumes that the reader knows how to render a simple triangle using vertex and fragment shaders using the OpenGL v3.3 core profile. We render four planar meshes in this recipe which are placed next to each other to create a bigger planar mesh. Each of these meshes is subdivided using the same geometry shader. The code for this recipe is located in the Chapter1\SubdivisionGeometryShader
directory.
We can implement the geometry shader using the following steps:
- Define a vertex shader (
shaders/shader.vert
) which outputs object space vertex positions directly.#version 330 core layout(location=0) in vec3 vVertex; void main() { gl_Position = vec4(vVertex, 1); }
- Define a geometry shader (
shaders/shader.geom
) which performs the subdivision of the quad. The shader is explained in the next section.#version 330 core layout (triangles) in; layout (triangle_strip, max_vertices=256) out; uniform int sub_divisions; uniform mat4 MVP; void main() { vec4 v0 = gl_in[0].gl_Position; vec4 v1 = gl_in[1].gl_Position; vec4 v2 = gl_in[2].gl_Position; float dx = abs(v0.x-v2.x)/sub_divisions; float dz = abs(v0.z-v1.z)/sub_divisions; float x=v0.x; float z=v0.z; for(int j=0;j<sub_divisions*sub_divisions;j++) { gl_Position = MVP * vec4(x,0,z,1); EmitVertex(); gl_Position = MVP * vec4(x,0,z+dz,1); EmitVertex(); gl_Position = MVP * vec4(x+dx,0,z,1); EmitVertex(); gl_Position = MVP * vec4(x+dx,0,z+dz,1); EmitVertex(); EndPrimitive(); x+=dx; if((j+1) %sub_divisions == 0) { x=v0.x; z+=dz; } } }
- Define a fragment shader (
shaders/shader.frag
) that simply outputs a constant color.#version 330 core layout(location=0) out vec4 vFragColor; void main() { vFragColor = vec4(1,1,1,1); }
- Load the shaders using the GLSLShader class in the
OnInit()
function.shader.LoadFromFile(GL_VERTEX_SHADER, "shaders/shader.vert"); shader.LoadFromFile(GL_GEOMETRY_SHADER,"shaders/shader.geom"); shader.LoadFromFile(GL_FRAGMENT_SHADER,"shaders/shader.frag"); shader.CreateAndLinkProgram(); shader.Use(); shader.AddAttribute("vVertex"); shader.AddUniform("MVP"); shader.AddUniform("sub_divisions"); glUniform1i(shader("sub_divisions"), sub_divisions); shader.UnUse();
- Create the geometry and topology.
vertices[0] = glm::vec3(-5,0,-5); vertices[1] = glm::vec3(-5,0,5); vertices[2] = glm::vec3(5,0,5); vertices[3] = glm::vec3(5,0,-5); GLushort* id=&indices[0]; *id++ = 0; *id++ = 1; *id++ = 2; *id++ = 0; *id++ = 2; *id++ = 3;
- Store the geometry and topology in the buffer object(s). Also enable the line display mode.
glGenVertexArrays(1, &vaoID); glGenBuffers(1, &vboVerticesID); glGenBuffers(1, &vboIndicesID); glBindVertexArray(vaoID); glBindBuffer (GL_ARRAY_BUFFER, vboVerticesID); glBufferData (GL_ARRAY_BUFFER, sizeof(vertices), &vertices[0], GL_STATIC_DRAW); glEnableVertexAttribArray(shader["vVertex"]); glVertexAttribPointer(shader["vVertex"], 3, GL_FLOAT, GL_FALSE,0,0); glBindBuffer(GL_ELEMENT_ARRAY_BUFFER, vboIndicesID); glBufferData(GL_ELEMENT_ARRAY_BUFFER, sizeof(indices), &indices[0], GL_STATIC_DRAW); glPolygonMode(GL_FRONT_AND_BACK, GL_LINE);
- Set up the rendering code to bind the
GLSLShader
shader, pass the uniforms and then draw the geometry.void OnRender() { glClear(GL_COLOR_BUFFER_BIT|GL_DEPTH_BUFFER_BIT); glm::mat4 T = glm::translate( glm::mat4(1.0f), glm::vec3(0.0f,0.0f, dist)); glm::mat4 Rx=glm::rotate(T,rX,glm::vec3(1.0f, 0.0f, 0.0f)); glm::mat4 MV=glm::rotate(Rx,rY, glm::vec3(0.0f,1.0f,0.0f)); MV=glm::translate(MV, glm::vec3(-5,0,-5)); shader.Use(); glUniform1i(shader("sub_divisions"), sub_divisions); glUniformMatrix4fv(shader("MVP"), 1, GL_FALSE, glm::value_ptr(P*MV)); glDrawElements(GL_TRIANGLES, 6, GL_UNSIGNED_SHORT, 0); MV=glm::translate(MV, glm::vec3(10,0,0)); glUniformMatrix4fv(shader("MVP"), 1, GL_FALSE, glm::value_ptr(P*MV)); glDrawElements(GL_TRIANGLES, 6, GL_UNSIGNED_SHORT, 0); MV=glm::translate(MV, glm::vec3(0,0,10)); glUniformMatrix4fv(shader("MVP"), 1, GL_FALSE, glm::value_ptr(P*MV)); glDrawElements(GL_TRIANGLES, 6, GL_UNSIGNED_SHORT, 0); MV=glm::translate(MV, glm::vec3(-10,0,0)); glUniformMatrix4fv(shader("MVP"), 1, GL_FALSE, glm::value_ptr(P*MV)); glDrawElements(GL_TRIANGLES, 6, GL_UNSIGNED_SHORT, 0); shader.UnUse(); glutSwapBuffers(); }
- Delete the shader and other OpenGL objects.
void OnShutdown() { shader.DeleteShaderProgram(); glDeleteBuffers(1, &vboVerticesID); glDeleteBuffers(1, &vboIndicesID); glDeleteVertexArrays(1, &vaoID); cout<<"Shutdown successfull"<<endl; }
Let's dissect the geometry shader.
Next, we calculate the size of the smallest quad for the given subdivision based on the size of the given base triangle and the total number of subdivisions required.
We start from the first vertex. We store the x
and z
values of this vertex in local variables. Next, we iterate N*N
times, where N
is the total number of subdivisions required. For example, if we need to subdivide the mesh three times on both axes, the loop will run nine times, which is the total number of quads. After calculating the positions of the four vertices, they are emitted by calling EmitVertex()
. This function emits the current values of output variables to the current output primitive on the primitive stream. Next, the EndPrimitive()
call is issued to signify that we have emitted the four vertices of triangle_strip
.
The fragment shader outputs a constant color (white: vec4(1,1,1,1)
).
The application code is similar to the last recipes. We have an additional shader (shaders/shader.geom
), which is our geometry shader that is loaded from file.
The notable additions are highlighted, which include the new geometry shader and an additional uniform for the total subdivisions desired (sub_divisions
). We initialize this uniform at initialization. The buffer object handling is similar to the simple triangle recipe. The other difference is in the rendering function where there are some additional modeling transformations (translations) after the viewing transformation.
We can change the subdivision levels by pressing the , and . keys. We then check to make sure that the subdivisions are within the allowed limit. Finally, we request the freeglut function, glutPostRedisplay()
, to repaint the window to show the new mesh. Compiling and running the demo code displays four planar meshes. Pressing the , key decreases the subdivision level and the . key increases the subdivision level. The output from the subdivision geometry shader showing multiple subdivision levels is displayed in the following screenshot:
using the OpenGL v3.3 core profile. We render four planar meshes in this recipe which are placed next to each other to create a bigger planar mesh. Each of these meshes is subdivided using the same geometry shader. The code for this recipe is located in the Chapter1\SubdivisionGeometryShader
directory.
We can implement the geometry shader using the following steps:
- Define a vertex shader (
shaders/shader.vert
) which outputs object space vertex positions directly.#version 330 core layout(location=0) in vec3 vVertex; void main() { gl_Position = vec4(vVertex, 1); }
- Define a geometry shader (
shaders/shader.geom
) which performs the subdivision of the quad. The shader is explained in the next section.#version 330 core layout (triangles) in; layout (triangle_strip, max_vertices=256) out; uniform int sub_divisions; uniform mat4 MVP; void main() { vec4 v0 = gl_in[0].gl_Position; vec4 v1 = gl_in[1].gl_Position; vec4 v2 = gl_in[2].gl_Position; float dx = abs(v0.x-v2.x)/sub_divisions; float dz = abs(v0.z-v1.z)/sub_divisions; float x=v0.x; float z=v0.z; for(int j=0;j<sub_divisions*sub_divisions;j++) { gl_Position = MVP * vec4(x,0,z,1); EmitVertex(); gl_Position = MVP * vec4(x,0,z+dz,1); EmitVertex(); gl_Position = MVP * vec4(x+dx,0,z,1); EmitVertex(); gl_Position = MVP * vec4(x+dx,0,z+dz,1); EmitVertex(); EndPrimitive(); x+=dx; if((j+1) %sub_divisions == 0) { x=v0.x; z+=dz; } } }
- Define a fragment shader (
shaders/shader.frag
) that simply outputs a constant color.#version 330 core layout(location=0) out vec4 vFragColor; void main() { vFragColor = vec4(1,1,1,1); }
- Load the shaders using the GLSLShader class in the
OnInit()
function.shader.LoadFromFile(GL_VERTEX_SHADER, "shaders/shader.vert"); shader.LoadFromFile(GL_GEOMETRY_SHADER,"shaders/shader.geom"); shader.LoadFromFile(GL_FRAGMENT_SHADER,"shaders/shader.frag"); shader.CreateAndLinkProgram(); shader.Use(); shader.AddAttribute("vVertex"); shader.AddUniform("MVP"); shader.AddUniform("sub_divisions"); glUniform1i(shader("sub_divisions"), sub_divisions); shader.UnUse();
- Create the geometry and topology.
vertices[0] = glm::vec3(-5,0,-5); vertices[1] = glm::vec3(-5,0,5); vertices[2] = glm::vec3(5,0,5); vertices[3] = glm::vec3(5,0,-5); GLushort* id=&indices[0]; *id++ = 0; *id++ = 1; *id++ = 2; *id++ = 0; *id++ = 2; *id++ = 3;
- Store the geometry and topology in the buffer object(s). Also enable the line display mode.
glGenVertexArrays(1, &vaoID); glGenBuffers(1, &vboVerticesID); glGenBuffers(1, &vboIndicesID); glBindVertexArray(vaoID); glBindBuffer (GL_ARRAY_BUFFER, vboVerticesID); glBufferData (GL_ARRAY_BUFFER, sizeof(vertices), &vertices[0], GL_STATIC_DRAW); glEnableVertexAttribArray(shader["vVertex"]); glVertexAttribPointer(shader["vVertex"], 3, GL_FLOAT, GL_FALSE,0,0); glBindBuffer(GL_ELEMENT_ARRAY_BUFFER, vboIndicesID); glBufferData(GL_ELEMENT_ARRAY_BUFFER, sizeof(indices), &indices[0], GL_STATIC_DRAW); glPolygonMode(GL_FRONT_AND_BACK, GL_LINE);
- Set up the rendering code to bind the
GLSLShader
shader, pass the uniforms and then draw the geometry.void OnRender() { glClear(GL_COLOR_BUFFER_BIT|GL_DEPTH_BUFFER_BIT); glm::mat4 T = glm::translate( glm::mat4(1.0f), glm::vec3(0.0f,0.0f, dist)); glm::mat4 Rx=glm::rotate(T,rX,glm::vec3(1.0f, 0.0f, 0.0f)); glm::mat4 MV=glm::rotate(Rx,rY, glm::vec3(0.0f,1.0f,0.0f)); MV=glm::translate(MV, glm::vec3(-5,0,-5)); shader.Use(); glUniform1i(shader("sub_divisions"), sub_divisions); glUniformMatrix4fv(shader("MVP"), 1, GL_FALSE, glm::value_ptr(P*MV)); glDrawElements(GL_TRIANGLES, 6, GL_UNSIGNED_SHORT, 0); MV=glm::translate(MV, glm::vec3(10,0,0)); glUniformMatrix4fv(shader("MVP"), 1, GL_FALSE, glm::value_ptr(P*MV)); glDrawElements(GL_TRIANGLES, 6, GL_UNSIGNED_SHORT, 0); MV=glm::translate(MV, glm::vec3(0,0,10)); glUniformMatrix4fv(shader("MVP"), 1, GL_FALSE, glm::value_ptr(P*MV)); glDrawElements(GL_TRIANGLES, 6, GL_UNSIGNED_SHORT, 0); MV=glm::translate(MV, glm::vec3(-10,0,0)); glUniformMatrix4fv(shader("MVP"), 1, GL_FALSE, glm::value_ptr(P*MV)); glDrawElements(GL_TRIANGLES, 6, GL_UNSIGNED_SHORT, 0); shader.UnUse(); glutSwapBuffers(); }
- Delete the shader and other OpenGL objects.
void OnShutdown() { shader.DeleteShaderProgram(); glDeleteBuffers(1, &vboVerticesID); glDeleteBuffers(1, &vboIndicesID); glDeleteVertexArrays(1, &vaoID); cout<<"Shutdown successfull"<<endl; }
Let's dissect the geometry shader.
Next, we calculate the size of the smallest quad for the given subdivision based on the size of the given base triangle and the total number of subdivisions required.
We start from the first vertex. We store the x
and z
values of this vertex in local variables. Next, we iterate N*N
times, where N
is the total number of subdivisions required. For example, if we need to subdivide the mesh three times on both axes, the loop will run nine times, which is the total number of quads. After calculating the positions of the four vertices, they are emitted by calling EmitVertex()
. This function emits the current values of output variables to the current output primitive on the primitive stream. Next, the EndPrimitive()
call is issued to signify that we have emitted the four vertices of triangle_strip
.
The fragment shader outputs a constant color (white: vec4(1,1,1,1)
).
The application code is similar to the last recipes. We have an additional shader (shaders/shader.geom
), which is our geometry shader that is loaded from file.
The notable additions are highlighted, which include the new geometry shader and an additional uniform for the total subdivisions desired (sub_divisions
). We initialize this uniform at initialization. The buffer object handling is similar to the simple triangle recipe. The other difference is in the rendering function where there are some additional modeling transformations (translations) after the viewing transformation.
We can change the subdivision levels by pressing the , and . keys. We then check to make sure that the subdivisions are within the allowed limit. Finally, we request the freeglut function, glutPostRedisplay()
, to repaint the window to show the new mesh. Compiling and running the demo code displays four planar meshes. Pressing the , key decreases the subdivision level and the . key increases the subdivision level. The output from the subdivision geometry shader showing multiple subdivision levels is displayed in the following screenshot:
shaders/shader.vert
) which outputs object space vertex positions directly.#version 330 core layout(location=0) in vec3 vVertex; void main() { gl_Position = vec4(vVertex, 1); }
shaders/shader.geom
) which performs the subdivision of the quad. The shader is explained in the next section.#version 330 core layout (triangles) in; layout (triangle_strip, max_vertices=256) out; uniform int sub_divisions; uniform mat4 MVP; void main() { vec4 v0 = gl_in[0].gl_Position; vec4 v1 = gl_in[1].gl_Position; vec4 v2 = gl_in[2].gl_Position; float dx = abs(v0.x-v2.x)/sub_divisions; float dz = abs(v0.z-v1.z)/sub_divisions; float x=v0.x; float z=v0.z; for(int j=0;j<sub_divisions*sub_divisions;j++) { gl_Position = MVP * vec4(x,0,z,1); EmitVertex(); gl_Position = MVP * vec4(x,0,z+dz,1); EmitVertex(); gl_Position = MVP * vec4(x+dx,0,z,1); EmitVertex(); gl_Position = MVP * vec4(x+dx,0,z+dz,1); EmitVertex(); EndPrimitive(); x+=dx; if((j+1) %sub_divisions == 0) { x=v0.x; z+=dz; } } }
shaders/shader.frag
) that simply outputs a constant color.#version 330 core layout(location=0) out vec4 vFragColor; void main() { vFragColor = vec4(1,1,1,1); }
- shaders using the GLSLShader class in the
OnInit()
function.shader.LoadFromFile(GL_VERTEX_SHADER, "shaders/shader.vert"); shader.LoadFromFile(GL_GEOMETRY_SHADER,"shaders/shader.geom"); shader.LoadFromFile(GL_FRAGMENT_SHADER,"shaders/shader.frag"); shader.CreateAndLinkProgram(); shader.Use(); shader.AddAttribute("vVertex"); shader.AddUniform("MVP"); shader.AddUniform("sub_divisions"); glUniform1i(shader("sub_divisions"), sub_divisions); shader.UnUse();
- Create the geometry and topology.
vertices[0] = glm::vec3(-5,0,-5); vertices[1] = glm::vec3(-5,0,5); vertices[2] = glm::vec3(5,0,5); vertices[3] = glm::vec3(5,0,-5); GLushort* id=&indices[0]; *id++ = 0; *id++ = 1; *id++ = 2; *id++ = 0; *id++ = 2; *id++ = 3;
- Store the geometry and topology in the buffer object(s). Also enable the line display mode.
glGenVertexArrays(1, &vaoID); glGenBuffers(1, &vboVerticesID); glGenBuffers(1, &vboIndicesID); glBindVertexArray(vaoID); glBindBuffer (GL_ARRAY_BUFFER, vboVerticesID); glBufferData (GL_ARRAY_BUFFER, sizeof(vertices), &vertices[0], GL_STATIC_DRAW); glEnableVertexAttribArray(shader["vVertex"]); glVertexAttribPointer(shader["vVertex"], 3, GL_FLOAT, GL_FALSE,0,0); glBindBuffer(GL_ELEMENT_ARRAY_BUFFER, vboIndicesID); glBufferData(GL_ELEMENT_ARRAY_BUFFER, sizeof(indices), &indices[0], GL_STATIC_DRAW); glPolygonMode(GL_FRONT_AND_BACK, GL_LINE);
- Set up the rendering code to bind the
GLSLShader
shader, pass the uniforms and then draw the geometry.void OnRender() { glClear(GL_COLOR_BUFFER_BIT|GL_DEPTH_BUFFER_BIT); glm::mat4 T = glm::translate( glm::mat4(1.0f), glm::vec3(0.0f,0.0f, dist)); glm::mat4 Rx=glm::rotate(T,rX,glm::vec3(1.0f, 0.0f, 0.0f)); glm::mat4 MV=glm::rotate(Rx,rY, glm::vec3(0.0f,1.0f,0.0f)); MV=glm::translate(MV, glm::vec3(-5,0,-5)); shader.Use(); glUniform1i(shader("sub_divisions"), sub_divisions); glUniformMatrix4fv(shader("MVP"), 1, GL_FALSE, glm::value_ptr(P*MV)); glDrawElements(GL_TRIANGLES, 6, GL_UNSIGNED_SHORT, 0); MV=glm::translate(MV, glm::vec3(10,0,0)); glUniformMatrix4fv(shader("MVP"), 1, GL_FALSE, glm::value_ptr(P*MV)); glDrawElements(GL_TRIANGLES, 6, GL_UNSIGNED_SHORT, 0); MV=glm::translate(MV, glm::vec3(0,0,10)); glUniformMatrix4fv(shader("MVP"), 1, GL_FALSE, glm::value_ptr(P*MV)); glDrawElements(GL_TRIANGLES, 6, GL_UNSIGNED_SHORT, 0); MV=glm::translate(MV, glm::vec3(-10,0,0)); glUniformMatrix4fv(shader("MVP"), 1, GL_FALSE, glm::value_ptr(P*MV)); glDrawElements(GL_TRIANGLES, 6, GL_UNSIGNED_SHORT, 0); shader.UnUse(); glutSwapBuffers(); }
- Delete the shader and other OpenGL objects.
void OnShutdown() { shader.DeleteShaderProgram(); glDeleteBuffers(1, &vboVerticesID); glDeleteBuffers(1, &vboIndicesID); glDeleteVertexArrays(1, &vaoID); cout<<"Shutdown successfull"<<endl; }
Let's dissect the geometry shader.
Next, we calculate the size of the smallest quad for the given subdivision based on the size of the given base triangle and the total number of subdivisions required.
We start from the first vertex. We store the x
and z
values of this vertex in local variables. Next, we iterate N*N
times, where N
is the total number of subdivisions required. For example, if we need to subdivide the mesh three times on both axes, the loop will run nine times, which is the total number of quads. After calculating the positions of the four vertices, they are emitted by calling EmitVertex()
. This function emits the current values of output variables to the current output primitive on the primitive stream. Next, the EndPrimitive()
call is issued to signify that we have emitted the four vertices of triangle_strip
.
The fragment shader outputs a constant color (white: vec4(1,1,1,1)
).
The application code is similar to the last recipes. We have an additional shader (shaders/shader.geom
), which is our geometry shader that is loaded from file.
The notable additions are highlighted, which include the new geometry shader and an additional uniform for the total subdivisions desired (sub_divisions
). We initialize this uniform at initialization. The buffer object handling is similar to the simple triangle recipe. The other difference is in the rendering function where there are some additional modeling transformations (translations) after the viewing transformation.
We can change the subdivision levels by pressing the , and . keys. We then check to make sure that the subdivisions are within the allowed limit. Finally, we request the freeglut function, glutPostRedisplay()
, to repaint the window to show the new mesh. Compiling and running the demo code displays four planar meshes. Pressing the , key decreases the subdivision level and the . key increases the subdivision level. The output from the subdivision geometry shader showing multiple subdivision levels is displayed in the following screenshot:
Next, we calculate the size of the smallest quad for the given subdivision based on the size of the given base triangle and the total number of subdivisions required.
We start from the first vertex. We store the x
and z
values of this vertex in local variables. Next, we iterate N*N
times, where N
is the total number of subdivisions required. For example, if we need to subdivide the mesh three times on both axes, the loop will run nine times, which is the total number of quads. After calculating the positions of the four vertices, they are emitted by calling EmitVertex()
. This function emits the current values of output variables to the current output primitive on the primitive stream. Next, the EndPrimitive()
call is issued to signify that we have emitted the four vertices of triangle_strip
.
The fragment shader outputs a constant color (white: vec4(1,1,1,1)
).
The application code is similar to the last recipes. We have an additional shader (shaders/shader.geom
), which is our geometry shader that is loaded from file.
The notable additions are highlighted, which include the new geometry shader and an additional uniform for the total subdivisions desired (sub_divisions
). We initialize this uniform at initialization. The buffer object handling is similar to the simple triangle recipe. The other difference is in the rendering function where there are some additional modeling transformations (translations) after the viewing transformation.
We can change the subdivision levels by pressing the , and . keys. We then check to make sure that the subdivisions are within the allowed limit. Finally, we request the freeglut function, glutPostRedisplay()
, to repaint the window to show the new mesh. Compiling and running the demo code displays four planar meshes. Pressing the , key decreases the subdivision level and the . key increases the subdivision level. The output from the subdivision geometry shader showing multiple subdivision levels is displayed in the following screenshot:
The notable additions are highlighted, which include the new geometry shader and an additional uniform for the total subdivisions desired (sub_divisions
). We initialize this uniform at initialization. The buffer object handling is similar to the simple triangle recipe. The other difference is in the rendering function where there are some additional modeling transformations (translations) after the viewing transformation.
We can change the subdivision levels by pressing the , and . keys. We then check to make sure that the subdivisions are within the allowed limit. Finally, we request the freeglut function, glutPostRedisplay()
, to repaint the window to show the new mesh. Compiling and running the demo code displays four planar meshes. Pressing the , key decreases the subdivision level and the . key increases the subdivision level. The output from the subdivision geometry shader showing multiple subdivision levels is displayed in the following screenshot:
In order to avoid pushing the same data multiple times, we can exploit the instanced rendering functions. We will now see how we can omit the multiple glDrawElements
calls in the previous recipe with a single glDrawElementsInstanced
call.
Converting the previous recipe to use instanced rendering requires the following steps:
- Change the vertex shader to handle the instance modeling matrix and output world space positions (
shaders/shader.vert
).#version 330 core layout(location=0) in vec3 vVertex; uniform mat4 M[4]; void main() { gl_Position = M[gl_InstanceID]*vec4(vVertex, 1); }
- Change the geometry shader to replace the
MVP
matrix with thePV
matrix (shaders/shader.geom
).#version 330 core layout (triangles) in; layout (triangle_strip, max_vertices=256) out; uniform int sub_divisions; uniform mat4 PV; void main() { vec4 v0 = gl_in[0].gl_Position; vec4 v1 = gl_in[1].gl_Position; vec4 v2 = gl_in[2].gl_Position; float dx = abs(v0.x-v2.x)/sub_divisions; float dz = abs(v0.z-v1.z)/sub_divisions; float x=v0.x; float z=v0.z; for(int j=0;j<sub_divisions*sub_divisions;j++) { gl_Position = PV * vec4(x,0,z,1); EmitVertex(); gl_Position = PV * vec4(x,0,z+dz,1); EmitVertex(); gl_Position = PV * vec4(x+dx,0,z,1); EmitVertex(); gl_Position = PV * vec4(x+dx,0,z+dz,1); EmitVertex(); EndPrimitive(); x+=dx; if((j+1) %sub_divisions == 0) { x=v0.x; z+=dz; } } }
- Initialize the per-instance model matrices (
M
).void OnInit() { //set the instance modeling matrix M[0] = glm::translate(glm::mat4(1), glm::vec3(-5,0,-5)); M[1] = glm::translate(M[0], glm::vec3(10,0,0)); M[2] = glm::translate(M[1], glm::vec3(0,0,10)); M[3] = glm::translate(M[2], glm::vec3(-10,0,0)); .. shader.Use(); shader.AddAttribute("vVertex"); shader.AddUniform("PV"); shader.AddUniform("M"); shader.AddUniform("sub_divisions"); glUniform1i(shader("sub_divisions"), sub_divisions); glUniformMatrix4fv(shader("M"), 4, GL_FALSE, glm::value_ptr(M[0])); shader.UnUse();
- Render instances using the
glDrawElementInstanced
call.void OnRender() { glClear(GL_COLOR_BUFFER_BIT|GL_DEPTH_BUFFER_BIT); glm::mat4 T =glm::translate(glm::mat4(1.0f), glm::vec3(0.0f, 0.0f, dist)); glm::mat4 Rx=glm::rotate(T,rX,glm::vec3(1.0f, 0.0f, 0.0f)); glm::mat4 V =glm::rotate(Rx,rY,glm::vec3(0.0f, 1.0f,0.0f)); glm::mat4 PV = P*V; shader.Use(); glUniformMatrix4fv(shader("PV"),1,GL_FALSE,glm::value_ptr(PV)); glUniform1i(shader("sub_divisions"), sub_divisions); glDrawElementsInstanced(GL_TRIANGLES, 6, GL_UNSIGNED_SHORT, 0, 4); shader.UnUse(); glutSwapBuffers(); }
First, we need to store the model matrix for each instance separately. Since we have four instances, we store a uniform array of four elements (M[4]
). Second, we multiply the per-vertex position (vVertex
) with the model matrix for the current instance (M[gl_InstanceID]
).
There is always a limit on the maximum number of matrices one can output from the vertex shader and this has some performance implications as well. Some performance improvements can be obtained by replacing the matrix storage with translation and scaling vectors, and an orientation quaternion which can then be converted on the fly into a matrix in the shader.
The official OpenGL wiki can be found at http://www.opengl.org/wiki/Built-in_Variable_%28GLSL%29.
An instance rendering tutorial from OGLDev can be found at http://ogldev.atspace.co.uk/www/tutorial33/tutorial33.html.
Chapter1\SubdivisionGeometryShader_Instanced
directory.
Converting the previous recipe to use instanced rendering requires the following steps:
- Change the vertex shader to handle the instance modeling matrix and output world space positions (
shaders/shader.vert
).#version 330 core layout(location=0) in vec3 vVertex; uniform mat4 M[4]; void main() { gl_Position = M[gl_InstanceID]*vec4(vVertex, 1); }
- Change the geometry shader to replace the
MVP
matrix with thePV
matrix (shaders/shader.geom
).#version 330 core layout (triangles) in; layout (triangle_strip, max_vertices=256) out; uniform int sub_divisions; uniform mat4 PV; void main() { vec4 v0 = gl_in[0].gl_Position; vec4 v1 = gl_in[1].gl_Position; vec4 v2 = gl_in[2].gl_Position; float dx = abs(v0.x-v2.x)/sub_divisions; float dz = abs(v0.z-v1.z)/sub_divisions; float x=v0.x; float z=v0.z; for(int j=0;j<sub_divisions*sub_divisions;j++) { gl_Position = PV * vec4(x,0,z,1); EmitVertex(); gl_Position = PV * vec4(x,0,z+dz,1); EmitVertex(); gl_Position = PV * vec4(x+dx,0,z,1); EmitVertex(); gl_Position = PV * vec4(x+dx,0,z+dz,1); EmitVertex(); EndPrimitive(); x+=dx; if((j+1) %sub_divisions == 0) { x=v0.x; z+=dz; } } }
- Initialize the per-instance model matrices (
M
).void OnInit() { //set the instance modeling matrix M[0] = glm::translate(glm::mat4(1), glm::vec3(-5,0,-5)); M[1] = glm::translate(M[0], glm::vec3(10,0,0)); M[2] = glm::translate(M[1], glm::vec3(0,0,10)); M[3] = glm::translate(M[2], glm::vec3(-10,0,0)); .. shader.Use(); shader.AddAttribute("vVertex"); shader.AddUniform("PV"); shader.AddUniform("M"); shader.AddUniform("sub_divisions"); glUniform1i(shader("sub_divisions"), sub_divisions); glUniformMatrix4fv(shader("M"), 4, GL_FALSE, glm::value_ptr(M[0])); shader.UnUse();
- Render instances using the
glDrawElementInstanced
call.void OnRender() { glClear(GL_COLOR_BUFFER_BIT|GL_DEPTH_BUFFER_BIT); glm::mat4 T =glm::translate(glm::mat4(1.0f), glm::vec3(0.0f, 0.0f, dist)); glm::mat4 Rx=glm::rotate(T,rX,glm::vec3(1.0f, 0.0f, 0.0f)); glm::mat4 V =glm::rotate(Rx,rY,glm::vec3(0.0f, 1.0f,0.0f)); glm::mat4 PV = P*V; shader.Use(); glUniformMatrix4fv(shader("PV"),1,GL_FALSE,glm::value_ptr(PV)); glUniform1i(shader("sub_divisions"), sub_divisions); glDrawElementsInstanced(GL_TRIANGLES, 6, GL_UNSIGNED_SHORT, 0, 4); shader.UnUse(); glutSwapBuffers(); }
First, we need to store the model matrix for each instance separately. Since we have four instances, we store a uniform array of four elements (M[4]
). Second, we multiply the per-vertex position (vVertex
) with the model matrix for the current instance (M[gl_InstanceID]
).
There is always a limit on the maximum number of matrices one can output from the vertex shader and this has some performance implications as well. Some performance improvements can be obtained by replacing the matrix storage with translation and scaling vectors, and an orientation quaternion which can then be converted on the fly into a matrix in the shader.
The official OpenGL wiki can be found at http://www.opengl.org/wiki/Built-in_Variable_%28GLSL%29.
An instance rendering tutorial from OGLDev can be found at http://ogldev.atspace.co.uk/www/tutorial33/tutorial33.html.
shaders/shader.vert
).#version 330 core
layout(location=0) in vec3 vVertex;
uniform mat4 M[4];
void main()
{
gl_Position = M[gl_InstanceID]*vec4(vVertex, 1);
}
MVP
matrix with the PV
matrix (shaders/shader.geom
).#version 330 core layout (triangles) in; layout (triangle_strip, max_vertices=256) out; uniform int sub_divisions; uniform mat4 PV; void main() { vec4 v0 = gl_in[0].gl_Position; vec4 v1 = gl_in[1].gl_Position; vec4 v2 = gl_in[2].gl_Position; float dx = abs(v0.x-v2.x)/sub_divisions; float dz = abs(v0.z-v1.z)/sub_divisions; float x=v0.x; float z=v0.z; for(int j=0;j<sub_divisions*sub_divisions;j++) { gl_Position = PV * vec4(x,0,z,1); EmitVertex(); gl_Position = PV * vec4(x,0,z+dz,1); EmitVertex(); gl_Position = PV * vec4(x+dx,0,z,1); EmitVertex(); gl_Position = PV * vec4(x+dx,0,z+dz,1); EmitVertex(); EndPrimitive(); x+=dx; if((j+1) %sub_divisions == 0) { x=v0.x; z+=dz; } } }
- the per-instance model matrices (
M
).void OnInit() { //set the instance modeling matrix M[0] = glm::translate(glm::mat4(1), glm::vec3(-5,0,-5)); M[1] = glm::translate(M[0], glm::vec3(10,0,0)); M[2] = glm::translate(M[1], glm::vec3(0,0,10)); M[3] = glm::translate(M[2], glm::vec3(-10,0,0)); .. shader.Use(); shader.AddAttribute("vVertex"); shader.AddUniform("PV"); shader.AddUniform("M"); shader.AddUniform("sub_divisions"); glUniform1i(shader("sub_divisions"), sub_divisions); glUniformMatrix4fv(shader("M"), 4, GL_FALSE, glm::value_ptr(M[0])); shader.UnUse();
- Render instances using the
glDrawElementInstanced
call.void OnRender() { glClear(GL_COLOR_BUFFER_BIT|GL_DEPTH_BUFFER_BIT); glm::mat4 T =glm::translate(glm::mat4(1.0f), glm::vec3(0.0f, 0.0f, dist)); glm::mat4 Rx=glm::rotate(T,rX,glm::vec3(1.0f, 0.0f, 0.0f)); glm::mat4 V =glm::rotate(Rx,rY,glm::vec3(0.0f, 1.0f,0.0f)); glm::mat4 PV = P*V; shader.Use(); glUniformMatrix4fv(shader("PV"),1,GL_FALSE,glm::value_ptr(PV)); glUniform1i(shader("sub_divisions"), sub_divisions); glDrawElementsInstanced(GL_TRIANGLES, 6, GL_UNSIGNED_SHORT, 0, 4); shader.UnUse(); glutSwapBuffers(); }
First, we need to store the model matrix for each instance separately. Since we have four instances, we store a uniform array of four elements (M[4]
). Second, we multiply the per-vertex position (vVertex
) with the model matrix for the current instance (M[gl_InstanceID]
).
There is always a limit on the maximum number of matrices one can output from the vertex shader and this has some performance implications as well. Some performance improvements can be obtained by replacing the matrix storage with translation and scaling vectors, and an orientation quaternion which can then be converted on the fly into a matrix in the shader.
The official OpenGL wiki can be found at http://www.opengl.org/wiki/Built-in_Variable_%28GLSL%29.
An instance rendering tutorial from OGLDev can be found at http://ogldev.atspace.co.uk/www/tutorial33/tutorial33.html.
There is always a limit on the maximum number of matrices one can output from the vertex shader and this has some performance implications as well. Some performance improvements can be obtained by replacing the matrix storage with translation and scaling vectors, and an orientation quaternion which can then be converted on the fly into a matrix in the shader.
The official OpenGL wiki can be found at http://www.opengl.org/wiki/Built-in_Variable_%28GLSL%29.
An instance rendering tutorial from OGLDev can be found at http://ogldev.atspace.co.uk/www/tutorial33/tutorial33.html.
http://www.opengl.org/wiki/Built-in_Variable_%28GLSL%29.
An instance rendering tutorial from OGLDev can be found at http://ogldev.atspace.co.uk/www/tutorial33/tutorial33.html.
We will wrap up this chapter with a recipe for creating a simple image viewer in the OpenGL v3.3 core profile using the SOIL
image loading library.
Let us now implement the image loader by following these steps:
- Load the image using the
SOIL
library. Since the loaded image fromSOIL
is inverted vertically, we flip the image on the Y axis.int texture_width = 0, texture_height = 0, channels=0; GLubyte* pData = SOIL_load_image(filename.c_str(), &texture_width, &texture_height, &channels, SOIL_LOAD_AUTO); if(pData == NULL) { cerr<<"Cannot load image: "<<filename.c_str()<<endl; exit(EXIT_FAILURE); } int i,j; for( j = 0; j*2 < texture_height; ++j ) { int index1 = j * texture_width * channels; int index2 = (texture_height - 1 - j) * texture_width * channels; for( i = texture_width * channels; i > 0; --i ) { GLubyte temp = pData[index1]; pData[index1] = pData[index2]; pData[index2] = temp; ++index1; ++index2; } }
- Set up the OpenGL texture object and free the data allocated by the
SOIL
library.glGenTextures(1, &textureID); glActiveTexture(GL_TEXTURE0); glBindTexture(GL_TEXTURE_2D, textureID); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MIN_FILTER,GL_LINEAR); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MAG_FILTER, GL_LINEAR); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_S, GL_CLAMP); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_T, GL_CLAMP); glTexImage2D(GL_TEXTURE_2D, 0, GL_RGB, texture_width, texture_height, 0, GL_RGB, GL_UNSIGNED_BYTE, pData); SOIL_free_image_data(pData);
- Set up the vertex shader to output the clip space position (
shaders/shader.vert
).#version 330 core layout(location=0) in vec2 vVertex; smooth out vec2 vUV; void main() { gl_Position = vec4(vVertex*2.0-1,0,1); vUV = vVertex; }
- Set up the fragment shader that samples our image texture (
shaders/shader.frag
).#version 330 core layout (location=0) out vec4 vFragColor; smooth in vec2 vUV; uniform sampler2D textureMap; void main() { vFragColor = texture(textureMap, vUV); }
- Set up the application code using the
GLSLShader
shader class.shader.LoadFromFile(GL_VERTEX_SHADER, "shaders/shader.vert"); shader.LoadFromFile(GL_FRAGMENT_SHADER,"shaders/shader.frag"); shader.CreateAndLinkProgram(); shader.Use(); shader.AddAttribute("vVertex"); shader.AddUniform("textureMap"); glUniform1i(shader("textureMap"), 0); shader.UnUse();
- Set up the geometry and topology and pass data to the GPU using buffer objects.
vertices[0] = glm::vec2(0.0,0.0); vertices[1] = glm::vec2(1.0,0.0); vertices[2] = glm::vec2(1.0,1.0); vertices[3] = glm::vec2(0.0,1.0); GLushort* id=&indices[0]; *id++ =0; *id++ =1; *id++ =2; *id++ =0; *id++ =2; *id++ =3; glGenVertexArrays(1, &vaoID); glGenBuffers(1, &vboVerticesID); glGenBuffers(1, &vboIndicesID); glBindVertexArray(vaoID); glBindBuffer (GL_ARRAY_BUFFER, vboVerticesID); glBufferData (GL_ARRAY_BUFFER, sizeof(vertices), &vertices[0], GL_STATIC_DRAW); glEnableVertexAttribArray(shader["vVertex"]); glVertexAttribPointer(shader["vVertex"], 2, GL_FLOAT, GL_FALSE,0,0); glBindBuffer(GL_ELEMENT_ARRAY_BUFFER, vboIndicesID); glBufferData(GL_ELEMENT_ARRAY_BUFFER, sizeof(indices), &indices[0], GL_STATIC_DRAW);
- Set the shader and render the geometry.
void OnRender() { glClear(GL_COLOR_BUFFER_BIT|GL_DEPTH_BUFFER_BIT); shader.Use(); glDrawElements(GL_TRIANGLES, 6, GL_UNSIGNED_SHORT, 0); shader.UnUse(); glutSwapBuffers(); }
- Release the allocated resources.
void OnShutdown() { shader.DeleteShaderProgram(); glDeleteBuffers(1, &vboVerticesID); glDeleteBuffers(1, &vboIndicesID); glDeleteVertexArrays(1, &vaoID); glDeleteTextures(1, &textureID); }
The first parameter is the image file name. The next three parameters return the texture width, texture height, and total color channels in the image. These are used when generating the OpenGL texture object. The final parameter is the flag which is used to control further processing on the image. For this simple example, we will use the SOIL_LOAD_AUTO
flag which keeps all of the loading settings set to default. If the function succeeds, it returns unsigned char*
to the image data. If it fails, the return value is NULL (0)
. Since the image data loaded by SOIL
is vertically flipped, we then use two nested loops to flip the image data on the Y axis.
After the image data is loaded, we generate an OpenGL texture object and pass this data to the texture memory.
The glTexImage2D
function is where the actual allocation of the texture object takes place. The first parameter is the texture target (in our case this is GL_TEXTURE_2D
). The second parameter is the mipmap level which we keep to 0
. The third parameter is the internal format. We can determine this by looking at the image properties. The fourth and fifth parameters store the texture width and height respectively. The sixth parameter is 0
for no border and 1
for border. The seventh parameter is the image format. The eighth parameter is the type of the image data pointer, and the final parameter is the pointer to the raw image data. After this function, we can safely release the image data allocated by SOIL
by calling SOIL_free_image_data(pData)
.
The fragment shader has the texture coordinates smoothly interpolated from the vertex shader stage through the rasterizer. The image that we loaded using SOIL
is passed to a texture sampler (uniform sampler2D textureMap
) which is then sampled using the input texture coordinates (vFragColor = texture(textureMap, vUV)
). So in the end, we get the image displayed on the screen.
The OnShutdown()
function is similar to the earlier recipes. In addition, this code adds deletion of the OpenGL texture object. The rendering code first clears the color and depth buffers. Next, it binds the shader program and then invokes the glDrawElement
call to render the triangles. Finally the shader is unbound and then the glutSwapBuffers
function is called to display the current back buffer as the next front buffer. Compiling and running this code displays the image in a window as shown in the following screenshot:
Chapter1/ImageLoader
directory.
Let us now implement the image loader by following these steps:
- Load the image using the
SOIL
library. Since the loaded image fromSOIL
is inverted vertically, we flip the image on the Y axis.int texture_width = 0, texture_height = 0, channels=0; GLubyte* pData = SOIL_load_image(filename.c_str(), &texture_width, &texture_height, &channels, SOIL_LOAD_AUTO); if(pData == NULL) { cerr<<"Cannot load image: "<<filename.c_str()<<endl; exit(EXIT_FAILURE); } int i,j; for( j = 0; j*2 < texture_height; ++j ) { int index1 = j * texture_width * channels; int index2 = (texture_height - 1 - j) * texture_width * channels; for( i = texture_width * channels; i > 0; --i ) { GLubyte temp = pData[index1]; pData[index1] = pData[index2]; pData[index2] = temp; ++index1; ++index2; } }
- Set up the OpenGL texture object and free the data allocated by the
SOIL
library.glGenTextures(1, &textureID); glActiveTexture(GL_TEXTURE0); glBindTexture(GL_TEXTURE_2D, textureID); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MIN_FILTER,GL_LINEAR); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MAG_FILTER, GL_LINEAR); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_S, GL_CLAMP); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_T, GL_CLAMP); glTexImage2D(GL_TEXTURE_2D, 0, GL_RGB, texture_width, texture_height, 0, GL_RGB, GL_UNSIGNED_BYTE, pData); SOIL_free_image_data(pData);
- Set up the vertex shader to output the clip space position (
shaders/shader.vert
).#version 330 core layout(location=0) in vec2 vVertex; smooth out vec2 vUV; void main() { gl_Position = vec4(vVertex*2.0-1,0,1); vUV = vVertex; }
- Set up the fragment shader that samples our image texture (
shaders/shader.frag
).#version 330 core layout (location=0) out vec4 vFragColor; smooth in vec2 vUV; uniform sampler2D textureMap; void main() { vFragColor = texture(textureMap, vUV); }
- Set up the application code using the
GLSLShader
shader class.shader.LoadFromFile(GL_VERTEX_SHADER, "shaders/shader.vert"); shader.LoadFromFile(GL_FRAGMENT_SHADER,"shaders/shader.frag"); shader.CreateAndLinkProgram(); shader.Use(); shader.AddAttribute("vVertex"); shader.AddUniform("textureMap"); glUniform1i(shader("textureMap"), 0); shader.UnUse();
- Set up the geometry and topology and pass data to the GPU using buffer objects.
vertices[0] = glm::vec2(0.0,0.0); vertices[1] = glm::vec2(1.0,0.0); vertices[2] = glm::vec2(1.0,1.0); vertices[3] = glm::vec2(0.0,1.0); GLushort* id=&indices[0]; *id++ =0; *id++ =1; *id++ =2; *id++ =0; *id++ =2; *id++ =3; glGenVertexArrays(1, &vaoID); glGenBuffers(1, &vboVerticesID); glGenBuffers(1, &vboIndicesID); glBindVertexArray(vaoID); glBindBuffer (GL_ARRAY_BUFFER, vboVerticesID); glBufferData (GL_ARRAY_BUFFER, sizeof(vertices), &vertices[0], GL_STATIC_DRAW); glEnableVertexAttribArray(shader["vVertex"]); glVertexAttribPointer(shader["vVertex"], 2, GL_FLOAT, GL_FALSE,0,0); glBindBuffer(GL_ELEMENT_ARRAY_BUFFER, vboIndicesID); glBufferData(GL_ELEMENT_ARRAY_BUFFER, sizeof(indices), &indices[0], GL_STATIC_DRAW);
- Set the shader and render the geometry.
void OnRender() { glClear(GL_COLOR_BUFFER_BIT|GL_DEPTH_BUFFER_BIT); shader.Use(); glDrawElements(GL_TRIANGLES, 6, GL_UNSIGNED_SHORT, 0); shader.UnUse(); glutSwapBuffers(); }
- Release the allocated resources.
void OnShutdown() { shader.DeleteShaderProgram(); glDeleteBuffers(1, &vboVerticesID); glDeleteBuffers(1, &vboIndicesID); glDeleteVertexArrays(1, &vaoID); glDeleteTextures(1, &textureID); }
The first parameter is the image file name. The next three parameters return the texture width, texture height, and total color channels in the image. These are used when generating the OpenGL texture object. The final parameter is the flag which is used to control further processing on the image. For this simple example, we will use the SOIL_LOAD_AUTO
flag which keeps all of the loading settings set to default. If the function succeeds, it returns unsigned char*
to the image data. If it fails, the return value is NULL (0)
. Since the image data loaded by SOIL
is vertically flipped, we then use two nested loops to flip the image data on the Y axis.
After the image data is loaded, we generate an OpenGL texture object and pass this data to the texture memory.
The glTexImage2D
function is where the actual allocation of the texture object takes place. The first parameter is the texture target (in our case this is GL_TEXTURE_2D
). The second parameter is the mipmap level which we keep to 0
. The third parameter is the internal format. We can determine this by looking at the image properties. The fourth and fifth parameters store the texture width and height respectively. The sixth parameter is 0
for no border and 1
for border. The seventh parameter is the image format. The eighth parameter is the type of the image data pointer, and the final parameter is the pointer to the raw image data. After this function, we can safely release the image data allocated by SOIL
by calling SOIL_free_image_data(pData)
.
The fragment shader has the texture coordinates smoothly interpolated from the vertex shader stage through the rasterizer. The image that we loaded using SOIL
is passed to a texture sampler (uniform sampler2D textureMap
) which is then sampled using the input texture coordinates (vFragColor = texture(textureMap, vUV)
). So in the end, we get the image displayed on the screen.
The OnShutdown()
function is similar to the earlier recipes. In addition, this code adds deletion of the OpenGL texture object. The rendering code first clears the color and depth buffers. Next, it binds the shader program and then invokes the glDrawElement
call to render the triangles. Finally the shader is unbound and then the glutSwapBuffers
function is called to display the current back buffer as the next front buffer. Compiling and running this code displays the image in a window as shown in the following screenshot:
- image using the
SOIL
library. Since the loaded image fromSOIL
is inverted vertically, we flip the image on the Y axis.int texture_width = 0, texture_height = 0, channels=0; GLubyte* pData = SOIL_load_image(filename.c_str(), &texture_width, &texture_height, &channels, SOIL_LOAD_AUTO); if(pData == NULL) { cerr<<"Cannot load image: "<<filename.c_str()<<endl; exit(EXIT_FAILURE); } int i,j; for( j = 0; j*2 < texture_height; ++j ) { int index1 = j * texture_width * channels; int index2 = (texture_height - 1 - j) * texture_width * channels; for( i = texture_width * channels; i > 0; --i ) { GLubyte temp = pData[index1]; pData[index1] = pData[index2]; pData[index2] = temp; ++index1; ++index2; } }
- Set up the OpenGL texture object and free the data allocated by the
SOIL
library.glGenTextures(1, &textureID); glActiveTexture(GL_TEXTURE0); glBindTexture(GL_TEXTURE_2D, textureID); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MIN_FILTER,GL_LINEAR); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MAG_FILTER, GL_LINEAR); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_S, GL_CLAMP); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_T, GL_CLAMP); glTexImage2D(GL_TEXTURE_2D, 0, GL_RGB, texture_width, texture_height, 0, GL_RGB, GL_UNSIGNED_BYTE, pData); SOIL_free_image_data(pData);
- Set up the vertex shader to output the clip space position (
shaders/shader.vert
).#version 330 core layout(location=0) in vec2 vVertex; smooth out vec2 vUV; void main() { gl_Position = vec4(vVertex*2.0-1,0,1); vUV = vVertex; }
- Set up the fragment shader that samples our image texture (
shaders/shader.frag
).#version 330 core layout (location=0) out vec4 vFragColor; smooth in vec2 vUV; uniform sampler2D textureMap; void main() { vFragColor = texture(textureMap, vUV); }
- Set up the application code using the
GLSLShader
shader class.shader.LoadFromFile(GL_VERTEX_SHADER, "shaders/shader.vert"); shader.LoadFromFile(GL_FRAGMENT_SHADER,"shaders/shader.frag"); shader.CreateAndLinkProgram(); shader.Use(); shader.AddAttribute("vVertex"); shader.AddUniform("textureMap"); glUniform1i(shader("textureMap"), 0); shader.UnUse();
- Set up the geometry and topology and pass data to the GPU using buffer objects.
vertices[0] = glm::vec2(0.0,0.0); vertices[1] = glm::vec2(1.0,0.0); vertices[2] = glm::vec2(1.0,1.0); vertices[3] = glm::vec2(0.0,1.0); GLushort* id=&indices[0]; *id++ =0; *id++ =1; *id++ =2; *id++ =0; *id++ =2; *id++ =3; glGenVertexArrays(1, &vaoID); glGenBuffers(1, &vboVerticesID); glGenBuffers(1, &vboIndicesID); glBindVertexArray(vaoID); glBindBuffer (GL_ARRAY_BUFFER, vboVerticesID); glBufferData (GL_ARRAY_BUFFER, sizeof(vertices), &vertices[0], GL_STATIC_DRAW); glEnableVertexAttribArray(shader["vVertex"]); glVertexAttribPointer(shader["vVertex"], 2, GL_FLOAT, GL_FALSE,0,0); glBindBuffer(GL_ELEMENT_ARRAY_BUFFER, vboIndicesID); glBufferData(GL_ELEMENT_ARRAY_BUFFER, sizeof(indices), &indices[0], GL_STATIC_DRAW);
- Set the shader and render the geometry.
void OnRender() { glClear(GL_COLOR_BUFFER_BIT|GL_DEPTH_BUFFER_BIT); shader.Use(); glDrawElements(GL_TRIANGLES, 6, GL_UNSIGNED_SHORT, 0); shader.UnUse(); glutSwapBuffers(); }
- Release the allocated resources.
void OnShutdown() { shader.DeleteShaderProgram(); glDeleteBuffers(1, &vboVerticesID); glDeleteBuffers(1, &vboIndicesID); glDeleteVertexArrays(1, &vaoID); glDeleteTextures(1, &textureID); }
The first parameter is the image file name. The next three parameters return the texture width, texture height, and total color channels in the image. These are used when generating the OpenGL texture object. The final parameter is the flag which is used to control further processing on the image. For this simple example, we will use the SOIL_LOAD_AUTO
flag which keeps all of the loading settings set to default. If the function succeeds, it returns unsigned char*
to the image data. If it fails, the return value is NULL (0)
. Since the image data loaded by SOIL
is vertically flipped, we then use two nested loops to flip the image data on the Y axis.
After the image data is loaded, we generate an OpenGL texture object and pass this data to the texture memory.
The glTexImage2D
function is where the actual allocation of the texture object takes place. The first parameter is the texture target (in our case this is GL_TEXTURE_2D
). The second parameter is the mipmap level which we keep to 0
. The third parameter is the internal format. We can determine this by looking at the image properties. The fourth and fifth parameters store the texture width and height respectively. The sixth parameter is 0
for no border and 1
for border. The seventh parameter is the image format. The eighth parameter is the type of the image data pointer, and the final parameter is the pointer to the raw image data. After this function, we can safely release the image data allocated by SOIL
by calling SOIL_free_image_data(pData)
.
The fragment shader has the texture coordinates smoothly interpolated from the vertex shader stage through the rasterizer. The image that we loaded using SOIL
is passed to a texture sampler (uniform sampler2D textureMap
) which is then sampled using the input texture coordinates (vFragColor = texture(textureMap, vUV)
). So in the end, we get the image displayed on the screen.
The OnShutdown()
function is similar to the earlier recipes. In addition, this code adds deletion of the OpenGL texture object. The rendering code first clears the color and depth buffers. Next, it binds the shader program and then invokes the glDrawElement
call to render the triangles. Finally the shader is unbound and then the glutSwapBuffers
function is called to display the current back buffer as the next front buffer. Compiling and running this code displays the image in a window as shown in the following screenshot:
SOIL
library provides a lot of functions but for now we are only interested in the SOIL_load_image
function.
processing on the image. For this simple example, we will use the SOIL_LOAD_AUTO
flag which keeps all of the loading settings set to default. If the function succeeds, it returns unsigned char*
to the image data. If it fails, the return value is NULL (0)
. Since the image data loaded by SOIL
is vertically flipped, we then use two nested loops to flip the image data on the Y axis.
After the image data is loaded, we generate an OpenGL texture object and pass this data to the texture memory.
The glTexImage2D
function is where the actual allocation of the texture object takes place. The first parameter is the texture target (in our case this is GL_TEXTURE_2D
). The second parameter is the mipmap level which we keep to 0
. The third parameter is the internal format. We can determine this by looking at the image properties. The fourth and fifth parameters store the texture width and height respectively. The sixth parameter is 0
for no border and 1
for border. The seventh parameter is the image format. The eighth parameter is the type of the image data pointer, and the final parameter is the pointer to the raw image data. After this function, we can safely release the image data allocated by SOIL
by calling SOIL_free_image_data(pData)
.
The fragment shader has the texture coordinates smoothly interpolated from the vertex shader stage through the rasterizer. The image that we loaded using SOIL
is passed to a texture sampler (uniform sampler2D textureMap
) which is then sampled using the input texture coordinates (vFragColor = texture(textureMap, vUV)
). So in the end, we get the image displayed on the screen.
The OnShutdown()
function is similar to the earlier recipes. In addition, this code adds deletion of the OpenGL texture object. The rendering code first clears the color and depth buffers. Next, it binds the shader program and then invokes the glDrawElement
call to render the triangles. Finally the shader is unbound and then the glutSwapBuffers
function is called to display the current back buffer as the next front buffer. Compiling and running this code displays the image in a window as shown in the following screenshot:
vVertex
) by simple arithmetic. Using the vertex positions, it also generates the texture coordinates (vUV
) for sampling of the texture in the fragment shader.
The OnShutdown()
function is similar to the earlier recipes. In addition, this code adds deletion of the OpenGL texture object. The rendering code first clears the color and depth buffers. Next, it binds the shader program and then invokes the glDrawElement
call to render the triangles. Finally the shader is unbound and then the glutSwapBuffers
function is called to display the current back buffer as the next front buffer. Compiling and running this code displays the image in a window as shown in the following screenshot:
The recipes covered in this chapter include:
- Implementing a vector-based camera model with FPS style input support
- Implementing the free camera
- Implementing target camera
- Implementing the view frustum culling
- Implementing object picking using the depth buffer
- Implementing object picking using color based picking
- Implementing object picking using scene intersection queries
In this chapter, we will look at the recipes for handling 3D viewing tasks and object picking in OpenGL v3.3 and above. All of the real-time simulations, games, and other graphics applications require a virtual camera or a virtual viewer from the point of view of which the 3D scene is rendered. The virtual camera is itself placed in the 3D world and has a specific direction called the camera look direction. Internally, the virtual camera is itself a collection of translations and rotations, which is stored inside the viewing matrix.
We will begin this chapter by designing a simple class to handle the camera. In a typical OpenGL application, the viewing operations are carried out to place a virtual object on screen. We leave the details of the transformations required in between to a typical graduate text on computer graphics like the one given in the See also section of this recipe. This recipe will focus on designing a simple and efficient camera class. We create a simple inheritance from a base class called CAbstractCamera
. We will inherit two classes from this parent class, CFreeCamera
and CTargetCamera
, as shown in the following figure:
We first declare the constructor/destructor pair. Next, the function for setting the projection for the camera is specified. Then some functions for updating the camera matrices based on rotation values are declared. Following these, the accessors and mutators are defined.
- Check for the keyboard key press event.
- If the W or S key is pressed, move the camera in the
look
vector direction:if( GetAsyncKeyState(VK_W) & 0x8000) cam.Walk(dt); if( GetAsyncKeyState(VK_S) & 0x8000) cam.Walk(-dt);
- If the A or D key is pressed, move the camera in the right vector direction:
if( GetAsyncKeyState(VK_A) & 0x8000) cam.Strafe(-dt); if( GetAsyncKeyState(VK_D) & 0x8000) cam.Strafe(dt);
- If the Q or Z key is pressed, move the camera in the up vector direction:
if( GetAsyncKeyState(VK_Q) & 0x8000) cam.Lift(dt); if( GetAsyncKeyState(VK_Z) & 0x8000) cam.Lift(-dt);
For handling mouse events, we attach two callbacks. One for mouse movement and the other for the mouse click event handling:
- Define the mouse down and mouse move event handlers.
- Determine the mouse input choice (the zoom or rotate state) in the mouse down event handler based on the mouse button clicked:
if(button == GLUT_MIDDLE_BUTTON) state = 0; else state = 1;
- If zoom state is chosen, calculate the
fov
value based on the drag amount and then set up the camera projection matrix:if (state == 0) { fov += (y - oldY)/5.0f; cam.SetupProjection(fov, cam.GetAspectRatio()); }
- If the rotate state is chosen, calculate the rotation amount (pitch and yaw). If mouse filtering is enabled, use the filtered mouse input, otherwise use the raw rotation amount:
else { rY += (y - oldY)/5.0f; rX += (oldX-x)/5.0f; if(useFiltering) filterMouseMoves(rX, rY); else { mouseX = rX; mouseY = rY; } cam.Rotate(mouseX,mouseY, 0); }
It is always better to use filtered mouse input, which gives smoother movement. In the recipes, we use a simple average filter of the last 10 inputs weighted based on their temporal distance. So the previous input is given more weight and the 5th latest input is given less weight. The filtered result is used as shown in the following code snippet:
- Smooth mouse filtering FAQ by Paul Nettle (http://www.flipcode.com/archives/Smooth_Mouse_Filtering.shtml)
- Real-time Rendering 3rd Edition by Tomas Akenine-Moller, Eric Haines, and Naty Hoffman, AK Peters/CRC Press, 2008
Chapter2/src
directory. The CAbstractCamera
class is defined in the AbstractCamera.[h/cpp]
files.
declare the constructor/destructor pair. Next, the function for setting the projection for the camera is specified. Then some functions for updating the camera matrices based on rotation values are declared. Following these, the accessors and mutators are defined.
- Check for the keyboard key press event.
- If the W or S key is pressed, move the camera in the
look
vector direction:if( GetAsyncKeyState(VK_W) & 0x8000) cam.Walk(dt); if( GetAsyncKeyState(VK_S) & 0x8000) cam.Walk(-dt);
- If the A or D key is pressed, move the camera in the right vector direction:
if( GetAsyncKeyState(VK_A) & 0x8000) cam.Strafe(-dt); if( GetAsyncKeyState(VK_D) & 0x8000) cam.Strafe(dt);
- If the Q or Z key is pressed, move the camera in the up vector direction:
if( GetAsyncKeyState(VK_Q) & 0x8000) cam.Lift(dt); if( GetAsyncKeyState(VK_Z) & 0x8000) cam.Lift(-dt);
For handling mouse events, we attach two callbacks. One for mouse movement and the other for the mouse click event handling:
- Define the mouse down and mouse move event handlers.
- Determine the mouse input choice (the zoom or rotate state) in the mouse down event handler based on the mouse button clicked:
if(button == GLUT_MIDDLE_BUTTON) state = 0; else state = 1;
- If zoom state is chosen, calculate the
fov
value based on the drag amount and then set up the camera projection matrix:if (state == 0) { fov += (y - oldY)/5.0f; cam.SetupProjection(fov, cam.GetAspectRatio()); }
- If the rotate state is chosen, calculate the rotation amount (pitch and yaw). If mouse filtering is enabled, use the filtered mouse input, otherwise use the raw rotation amount:
else { rY += (y - oldY)/5.0f; rX += (oldX-x)/5.0f; if(useFiltering) filterMouseMoves(rX, rY); else { mouseX = rX; mouseY = rY; } cam.Rotate(mouseX,mouseY, 0); }
It is always better to use filtered mouse input, which gives smoother movement. In the recipes, we use a simple average filter of the last 10 inputs weighted based on their temporal distance. So the previous input is given more weight and the 5th latest input is given less weight. The filtered result is used as shown in the following code snippet:
- Smooth mouse filtering FAQ by Paul Nettle (http://www.flipcode.com/archives/Smooth_Mouse_Filtering.shtml)
- Real-time Rendering 3rd Edition by Tomas Akenine-Moller, Eric Haines, and Naty Hoffman, AK Peters/CRC Press, 2008
CAbstractCamera
class. Instead, we will use either the CFreeCamera
class or the CTargetCamera
class, as detailed in the following recipes. In this recipe, we will see how to handle input using the mouse and keyboard.
look
vector direction:if( GetAsyncKeyState(VK_W) & 0x8000) cam.Walk(dt); if( GetAsyncKeyState(VK_S) & 0x8000) cam.Walk(-dt);
if( GetAsyncKeyState(VK_A) & 0x8000) cam.Strafe(-dt); if( GetAsyncKeyState(VK_D) & 0x8000) cam.Strafe(dt);
if( GetAsyncKeyState(VK_Q) & 0x8000) cam.Lift(dt); if( GetAsyncKeyState(VK_Z) & 0x8000) cam.Lift(-dt);
handling mouse events, we attach two callbacks. One for mouse movement and the other for the mouse click event handling:
- Define the mouse down and mouse move event handlers.
- Determine the mouse input choice (the zoom or rotate state) in the mouse down event handler based on the mouse button clicked:
if(button == GLUT_MIDDLE_BUTTON) state = 0; else state = 1;
- If zoom state is chosen, calculate the
fov
value based on the drag amount and then set up the camera projection matrix:if (state == 0) { fov += (y - oldY)/5.0f; cam.SetupProjection(fov, cam.GetAspectRatio()); }
- If the rotate state is chosen, calculate the rotation amount (pitch and yaw). If mouse filtering is enabled, use the filtered mouse input, otherwise use the raw rotation amount:
else { rY += (y - oldY)/5.0f; rX += (oldX-x)/5.0f; if(useFiltering) filterMouseMoves(rX, rY); else { mouseX = rX; mouseY = rY; } cam.Rotate(mouseX,mouseY, 0); }
It is always better to use filtered mouse input, which gives smoother movement. In the recipes, we use a simple average filter of the last 10 inputs weighted based on their temporal distance. So the previous input is given more weight and the 5th latest input is given less weight. The filtered result is used as shown in the following code snippet:
- Smooth mouse filtering FAQ by Paul Nettle (http://www.flipcode.com/archives/Smooth_Mouse_Filtering.shtml)
- Real-time Rendering 3rd Edition by Tomas Akenine-Moller, Eric Haines, and Naty Hoffman, AK Peters/CRC Press, 2008
always better to use filtered mouse input, which gives smoother movement. In the recipes, we use a simple average filter of the last 10 inputs weighted based on their temporal distance. So the previous input is given more weight and the 5th latest input is given less weight. The filtered result is used as shown in the following code snippet:
- Smooth mouse filtering FAQ by Paul Nettle (http://www.flipcode.com/archives/Smooth_Mouse_Filtering.shtml)
- Real-time Rendering 3rd Edition by Tomas Akenine-Moller, Eric Haines, and Naty Hoffman, AK Peters/CRC Press, 2008
- FAQ by Paul Nettle (http://www.flipcode.com/archives/Smooth_Mouse_Filtering.shtml)
- Real-time Rendering 3rd Edition by Tomas Akenine-Moller, Eric Haines, and Naty Hoffman, AK Peters/CRC Press, 2008
Free camera is the first camera type which we will implement in this recipe. A free camera does not have a fixed target. However it does have a fixed position from which it can look in any direction.
The following figure shows a free viewing camera. When we rotate the camera, it rotates at its position. When we move the camera, it keeps looking in the same direction.
The steps needed to implement the free camera are as follows:
- Define the
CFreeCamera
class and add a vector to store the current translation. - In the
Update
method, calculate the new orientation (rotation) matrix, using the current camera orientations (that is, yaw, pitch, and roll amount):glm::mat4 R = glm::yawPitchRoll(yaw,pitch,roll);
- Translate the camera position by the translation amount:
position+=translation;
If we need to implement a free camera which gradually comes to a halt, we should gradually decay the translation vector by adding the following code after the key events are handled:
glm::vec3 t = cam.GetTranslation(); if(glm::dot(t,t)>EPSILON2) { cam.SetTranslation(t*0.95f); }
If no decay is needed, then we should clear the translation vector to
0
in theCFreeCamera::Update
function after translating the position:translation = glm::vec3(0);
- Transform the
look
vector by the current rotation matrix, and determine theright
andup
vectors to calculate the orthonormal basis:look = glm::vec3(R*glm::vec4(0,0,1,0)); up = glm::vec3(R*glm::vec4(0,1,0,0)); right = glm::cross(look, up);
- Determine the camera target point:
glm::vec3 tgt = position+look;
- Use the
glm::lookat
function to calculate the new view matrix using the camera position, target, and theup
vector:V = glm::lookAt(position, tgt, up);
The Walk
function simply translates the camera in the look direction:
The Strafe
function translates the camera in the right direction:
The Lift
function translates the camera in the up direction:
- DHPOWare OpenGL camera demo – Part 1 (http://www.dhpoware.com/demos/glCamera1.html)
- DHPOWare OpenGL camera demo – Part 2 (http://www.dhpoware.com/demos/glCamera2.html)
- DHPOWare OpenGL camera demo – Part 3 (http://www.dhpoware.com/demos/glCamera3.html)
The steps needed to implement the free camera are as follows:
- Define the
CFreeCamera
class and add a vector to store the current translation. - In the
Update
method, calculate the new orientation (rotation) matrix, using the current camera orientations (that is, yaw, pitch, and roll amount):glm::mat4 R = glm::yawPitchRoll(yaw,pitch,roll);
- Translate the camera position by the translation amount:
position+=translation;
If we need to implement a free camera which gradually comes to a halt, we should gradually decay the translation vector by adding the following code after the key events are handled:
glm::vec3 t = cam.GetTranslation(); if(glm::dot(t,t)>EPSILON2) { cam.SetTranslation(t*0.95f); }
If no decay is needed, then we should clear the translation vector to
0
in theCFreeCamera::Update
function after translating the position:translation = glm::vec3(0);
- Transform the
look
vector by the current rotation matrix, and determine theright
andup
vectors to calculate the orthonormal basis:look = glm::vec3(R*glm::vec4(0,0,1,0)); up = glm::vec3(R*glm::vec4(0,1,0,0)); right = glm::cross(look, up);
- Determine the camera target point:
glm::vec3 tgt = position+look;
- Use the
glm::lookat
function to calculate the new view matrix using the camera position, target, and theup
vector:V = glm::lookAt(position, tgt, up);
The Walk
function simply translates the camera in the look direction:
The Strafe
function translates the camera in the right direction:
The Lift
function translates the camera in the up direction:
- DHPOWare OpenGL camera demo – Part 1 (http://www.dhpoware.com/demos/glCamera1.html)
- DHPOWare OpenGL camera demo – Part 2 (http://www.dhpoware.com/demos/glCamera2.html)
- DHPOWare OpenGL camera demo – Part 3 (http://www.dhpoware.com/demos/glCamera3.html)
CFreeCamera
class and add a vector to store the current translation.
Update
method, calculate the new orientation (rotation) matrix, using the current camera orientations (that is, yaw, pitch, and roll amount):glm::mat4 R = glm::yawPitchRoll(yaw,pitch,roll);
- Translate the camera position by the translation amount:
position+=translation;
If we need to implement a free camera which gradually comes to a halt, we should gradually decay the translation vector by adding the following code after the key events are handled:
glm::vec3 t = cam.GetTranslation(); if(glm::dot(t,t)>EPSILON2) { cam.SetTranslation(t*0.95f); }
If no decay is needed, then we should clear the translation vector to
0
in theCFreeCamera::Update
function after translating the position:translation = glm::vec3(0);
- Transform the
look
vector by the current rotation matrix, and determine theright
andup
vectors to calculate the orthonormal basis:look = glm::vec3(R*glm::vec4(0,0,1,0)); up = glm::vec3(R*glm::vec4(0,1,0,0)); right = glm::cross(look, up);
- Determine the camera target point:
glm::vec3 tgt = position+look;
- Use the
glm::lookat
function to calculate the new view matrix using the camera position, target, and theup
vector:V = glm::lookAt(position, tgt, up);
The Walk
function simply translates the camera in the look direction:
The Strafe
function translates the camera in the right direction:
The Lift
function translates the camera in the up direction:
- DHPOWare OpenGL camera demo – Part 1 (http://www.dhpoware.com/demos/glCamera1.html)
- DHPOWare OpenGL camera demo – Part 2 (http://www.dhpoware.com/demos/glCamera2.html)
- DHPOWare OpenGL camera demo – Part 3 (http://www.dhpoware.com/demos/glCamera3.html)
Walk
function
simply translates the camera in the look direction:
The Strafe
function translates the camera in the right direction:
The Lift
function translates the camera in the up direction:
- DHPOWare OpenGL camera demo – Part 1 (http://www.dhpoware.com/demos/glCamera1.html)
- DHPOWare OpenGL camera demo – Part 2 (http://www.dhpoware.com/demos/glCamera2.html)
- DHPOWare OpenGL camera demo – Part 3 (http://www.dhpoware.com/demos/glCamera3.html)
- http://www.dhpoware.com/demos/glCamera1.html)
- DHPOWare OpenGL camera demo – Part 2 (http://www.dhpoware.com/demos/glCamera2.html)
- DHPOWare OpenGL camera demo – Part 3 (http://www.dhpoware.com/demos/glCamera3.html)
The target camera works the opposite way. Rather than the position, the target remains fixed, while the camera moves or rotates around the target. Some operations like panning, move both the target and the camera position together.
The following figure shows an illustration of a target camera. Note that the small box is the target position for the camera.
The code for this recipe resides in the Chapter2/TargetCamera
directory. The CTargetCamera
class is defined in the Chapter2/src/TargetCamera.[h/cpp]
files. The class declaration is as follows:
We implement the target camera as follows:
- Define the
CTargetCamera
class with a target position (target
), the rotation limits (minRy
andmaxRy
), the distance between the target and the camera position (distance
), and the distance limits (minDistance
andmaxDistance
). - In the
Update
method, calculate the new orientation (rotation) matrix using the current camera orientations (that is, yaw, pitch, and roll amount):glm::mat4 R = glm::yawPitchRoll(yaw,pitch,roll);
- Use the distance to get a vector and then translate this vector by the current rotation matrix:
glm::vec3 T = glm::vec3(0,0,distance); T = glm::vec3(R*glm::vec4(T,0.0f));
- Get the new camera position by adding the translation vector to the target position:
position = target + T;
- Recalculate the orthonormal basis and then the view matrix:
look = glm::normalize(target-position); up = glm::vec3(R*glm::vec4(UP,0.0f)); right = glm::cross(look, up); V = glm::lookAt(position, target, up);
The Move
function moves both the position and target by the same amount in both look
and right
vector directions.
The Pan
function moves in the xy plane only, hence the up
vector is used instead of the look
vector:
The Zoom
function moves the position in the look
direction:
The demonstration for this recipe renders an infinite checkered plane, as in the previous recipe, and is shown in the following figure:
- DHPOWare OpenGL camera demo – Part 1 (http://www.dhpoware.com/demos/glCamera1.html)
- DHPOWare OpenGL camera demo – Part 2 (http://www.dhpoware.com/demos/glCamera2.html)
- DHPOWare OpenGL camera demo – Part 3 (http://www.dhpoware.com/demos/glCamera3.html)
The code for this recipe resides in the Chapter2/TargetCamera
directory. The CTargetCamera
class is defined in the Chapter2/src/TargetCamera.[h/cpp]
files. The class declaration is as follows:
We implement the target camera as follows:
- Define the
CTargetCamera
class with a target position (target
), the rotation limits (minRy
andmaxRy
), the distance between the target and the camera position (distance
), and the distance limits (minDistance
andmaxDistance
). - In the
Update
method, calculate the new orientation (rotation) matrix using the current camera orientations (that is, yaw, pitch, and roll amount):glm::mat4 R = glm::yawPitchRoll(yaw,pitch,roll);
- Use the distance to get a vector and then translate this vector by the current rotation matrix:
glm::vec3 T = glm::vec3(0,0,distance); T = glm::vec3(R*glm::vec4(T,0.0f));
- Get the new camera position by adding the translation vector to the target position:
position = target + T;
- Recalculate the orthonormal basis and then the view matrix:
look = glm::normalize(target-position); up = glm::vec3(R*glm::vec4(UP,0.0f)); right = glm::cross(look, up); V = glm::lookAt(position, target, up);
The Move
function moves both the position and target by the same amount in both look
and right
vector directions.
The Pan
function moves in the xy plane only, hence the up
vector is used instead of the look
vector:
The Zoom
function moves the position in the look
direction:
The demonstration for this recipe renders an infinite checkered plane, as in the previous recipe, and is shown in the following figure:
- DHPOWare OpenGL camera demo – Part 1 (http://www.dhpoware.com/demos/glCamera1.html)
- DHPOWare OpenGL camera demo – Part 2 (http://www.dhpoware.com/demos/glCamera2.html)
- DHPOWare OpenGL camera demo – Part 3 (http://www.dhpoware.com/demos/glCamera3.html)
CTargetCamera
class with a target position (target
), the rotation limits (minRy
and maxRy
), the distance between the target and the camera position (distance
), and the distance limits (minDistance
and maxDistance
).
Update
method, calculate the new orientation (rotation) matrix using the current camera orientations (that is, yaw, pitch, and roll amount):glm::mat4 R = glm::yawPitchRoll(yaw,pitch,roll);
glm::vec3 T = glm::vec3(0,0,distance); T = glm::vec3(R*glm::vec4(T,0.0f));
position = target + T;
The Move
function moves both the position and target by the same amount in both look
and right
vector directions.
The Pan
function moves in the xy plane only, hence the up
vector is used instead of the look
vector:
The Zoom
function moves the position in the look
direction:
The demonstration for this recipe renders an infinite checkered plane, as in the previous recipe, and is shown in the following figure:
- DHPOWare OpenGL camera demo – Part 1 (http://www.dhpoware.com/demos/glCamera1.html)
- DHPOWare OpenGL camera demo – Part 2 (http://www.dhpoware.com/demos/glCamera2.html)
- DHPOWare OpenGL camera demo – Part 3 (http://www.dhpoware.com/demos/glCamera3.html)
Move
function
moves both the position and target by the same amount in both look
and right
vector directions.
The Pan
function moves in the xy plane only, hence the up
vector is used instead of the look
vector:
The Zoom
function moves the position in the look
direction:
The demonstration for this recipe renders an infinite checkered plane, as in the previous recipe, and is shown in the following figure:
- DHPOWare OpenGL camera demo – Part 1 (http://www.dhpoware.com/demos/glCamera1.html)
- DHPOWare OpenGL camera demo – Part 2 (http://www.dhpoware.com/demos/glCamera2.html)
- DHPOWare OpenGL camera demo – Part 3 (http://www.dhpoware.com/demos/glCamera3.html)
- http://www.dhpoware.com/demos/glCamera1.html)
- DHPOWare OpenGL camera demo – Part 2 (http://www.dhpoware.com/demos/glCamera2.html)
- DHPOWare OpenGL camera demo – Part 3 (http://www.dhpoware.com/demos/glCamera3.html)
When working with a lot of polygonal data, there is a need to reduce the amount of geometry pushed to the GPU for processing. There are several techniques for scene management, such as quadtrees, octrees, and bsp trees. These techniques help in sorting the geometry in visibility order, so that the objects are sorted (and some of these even culled from the display). This helps in reducing the work load on the GPU.
Even before such techniques can be used, there is an additional step which most graphics applications do and that is view frustum culling. This process removes the geometry if it is not in the current camera's view frustum. The idea is that if the object is not viewable, it should not be processed. A frustum is a chopped pyramid with its tip at the camera position and the base is at the far clip plane. The near clip plane is where the pyramid is chopped, as shown in the following figure. Any geometry inside the viewing frustum is displayed.
We will implement view frustum culling by taking the following steps:
- Define a vertex shader that displaces the object-space vertex position using a sine wave in the y axis:
#version 330 core layout(location = 0) in vec3 vVertex; uniform float t; const float PI = 3.141562; void main() { gl_Position=vec4(vVertex,1)+vec4(0,sin(vVertex.x*2*PI+t),0,0); }
- Define a geometry shader that performs the view frustum culling calculation on each vertex passed in from the vertex shader:
#version 330 core layout (points) in; layout (points, max_vertices=3) out; uniform mat4 MVP; uniform vec4 FrustumPlanes[6]; bool PointInFrustum(in vec3 p) { for(int i=0; i < 6; i++) { vec4 plane=FrustumPlanes[i]; if ((dot(plane.xyz, p)+plane.w) < 0) return false; } return true; } void main() { //get the basic vertices for(int i=0;i<gl_in.length(); i++) { vec4 vInPos = gl_in[i].gl_Position; vec2 tmp = (vInPos.xz*2-1.0)*5; vec3 V = vec3(tmp.x, vInPos.y, tmp.y); gl_Position = MVP*vec4(V,1); if(PointInFrustum(V)) { EmitVertex(); } } EndPrimitive(); }
- To render particles as rounded points, we do a simple trigonometric calculation by discarding all fragments that fall outside the radius of the circle:
#version 330 core layout(location = 0) out vec4 vFragColor; void main() { vec2 pos = (gl_PointCoord.xy-0.5); if(0.25<dot(pos,pos)) discard; vFragColor = vec4(0,0,1,1); }
- On the CPU side, call the
CAbstractCamera::CalcFrustumPlanes()
function to calculate the viewing frustum planes. Get the calculated frustum planes as aglm::vec4
array by callingCAbstractCamera::GetFrustumPlanes()
, and then pass these to the shader. Thexyz
components store the plane's normal, and thew
coordinate stores the distance of the plane. After these calls we draw the points:pCurrentCam->CalcFrustumPlanes(); glm::vec4 p[6]; pCurrentCam->GetFrustumPlanes(p); pointShader.Use(); glUniform1f(pointShader("t"), current_time); glUniformMatrix4fv(pointShader("MVP"), 1, GL_FALSE, glm::value_ptr(MVP)); glUniform4fv(pointShader("FrustumPlanes"), 6, glm::value_ptr(p[0])); glBindVertexArray(pointVAOID); glDrawArrays(GL_POINTS,0,MAX_POINTS); pointShader.UnUse();
There are two main parts of this recipe: calculation of the viewing frustum planes and checking if a given point is in the viewing frustum. The first calculation is carried out in the CAbstractCamera::CalcFrustumPlanes()
function. Refer to the Chapter2/src/AbstractCamera.cpp
files for details.
This function iterates through all of the six frustum planes. In each iteration, it checks the signed distance of the given point p
with respect to the ith frustum plane. This is a simple dot product of the plane normal with the given point and adding the plane distance. If the signed distance is negative for any of the planes, the point is outside the viewing frustum so we can safely reject the point. If the point has a positive signed distance for all of the six frustum planes, it is inside the viewing frustum. Note that the frustum planes are oriented in such a way that their normals point inside the viewing frustum.
Chapter2/ViewFrustumCulling
directory.
We will implement view frustum culling by taking the following steps:
- Define a vertex shader that displaces the object-space vertex position using a sine wave in the y axis:
#version 330 core layout(location = 0) in vec3 vVertex; uniform float t; const float PI = 3.141562; void main() { gl_Position=vec4(vVertex,1)+vec4(0,sin(vVertex.x*2*PI+t),0,0); }
- Define a geometry shader that performs the view frustum culling calculation on each vertex passed in from the vertex shader:
#version 330 core layout (points) in; layout (points, max_vertices=3) out; uniform mat4 MVP; uniform vec4 FrustumPlanes[6]; bool PointInFrustum(in vec3 p) { for(int i=0; i < 6; i++) { vec4 plane=FrustumPlanes[i]; if ((dot(plane.xyz, p)+plane.w) < 0) return false; } return true; } void main() { //get the basic vertices for(int i=0;i<gl_in.length(); i++) { vec4 vInPos = gl_in[i].gl_Position; vec2 tmp = (vInPos.xz*2-1.0)*5; vec3 V = vec3(tmp.x, vInPos.y, tmp.y); gl_Position = MVP*vec4(V,1); if(PointInFrustum(V)) { EmitVertex(); } } EndPrimitive(); }
- To render particles as rounded points, we do a simple trigonometric calculation by discarding all fragments that fall outside the radius of the circle:
#version 330 core layout(location = 0) out vec4 vFragColor; void main() { vec2 pos = (gl_PointCoord.xy-0.5); if(0.25<dot(pos,pos)) discard; vFragColor = vec4(0,0,1,1); }
- On the CPU side, call the
CAbstractCamera::CalcFrustumPlanes()
function to calculate the viewing frustum planes. Get the calculated frustum planes as aglm::vec4
array by callingCAbstractCamera::GetFrustumPlanes()
, and then pass these to the shader. Thexyz
components store the plane's normal, and thew
coordinate stores the distance of the plane. After these calls we draw the points:pCurrentCam->CalcFrustumPlanes(); glm::vec4 p[6]; pCurrentCam->GetFrustumPlanes(p); pointShader.Use(); glUniform1f(pointShader("t"), current_time); glUniformMatrix4fv(pointShader("MVP"), 1, GL_FALSE, glm::value_ptr(MVP)); glUniform4fv(pointShader("FrustumPlanes"), 6, glm::value_ptr(p[0])); glBindVertexArray(pointVAOID); glDrawArrays(GL_POINTS,0,MAX_POINTS); pointShader.UnUse();
There are two main parts of this recipe: calculation of the viewing frustum planes and checking if a given point is in the viewing frustum. The first calculation is carried out in the CAbstractCamera::CalcFrustumPlanes()
function. Refer to the Chapter2/src/AbstractCamera.cpp
files for details.
This function iterates through all of the six frustum planes. In each iteration, it checks the signed distance of the given point p
with respect to the ith frustum plane. This is a simple dot product of the plane normal with the given point and adding the plane distance. If the signed distance is negative for any of the planes, the point is outside the viewing frustum so we can safely reject the point. If the point has a positive signed distance for all of the six frustum planes, it is inside the viewing frustum. Note that the frustum planes are oriented in such a way that their normals point inside the viewing frustum.
#version 330 core
layout(location = 0) in vec3 vVertex;
uniform float t;
const float PI = 3.141562;
void main()
{
gl_Position=vec4(vVertex,1)+vec4(0,sin(vVertex.x*2*PI+t),0,0);
}
- geometry shader that performs the view frustum culling calculation on each vertex passed in from the vertex shader:
#version 330 core layout (points) in; layout (points, max_vertices=3) out; uniform mat4 MVP; uniform vec4 FrustumPlanes[6]; bool PointInFrustum(in vec3 p) { for(int i=0; i < 6; i++) { vec4 plane=FrustumPlanes[i]; if ((dot(plane.xyz, p)+plane.w) < 0) return false; } return true; } void main() { //get the basic vertices for(int i=0;i<gl_in.length(); i++) { vec4 vInPos = gl_in[i].gl_Position; vec2 tmp = (vInPos.xz*2-1.0)*5; vec3 V = vec3(tmp.x, vInPos.y, tmp.y); gl_Position = MVP*vec4(V,1); if(PointInFrustum(V)) { EmitVertex(); } } EndPrimitive(); }
- To render particles as rounded points, we do a simple trigonometric calculation by discarding all fragments that fall outside the radius of the circle:
#version 330 core layout(location = 0) out vec4 vFragColor; void main() { vec2 pos = (gl_PointCoord.xy-0.5); if(0.25<dot(pos,pos)) discard; vFragColor = vec4(0,0,1,1); }
- On the CPU side, call the
CAbstractCamera::CalcFrustumPlanes()
function to calculate the viewing frustum planes. Get the calculated frustum planes as aglm::vec4
array by callingCAbstractCamera::GetFrustumPlanes()
, and then pass these to the shader. Thexyz
components store the plane's normal, and thew
coordinate stores the distance of the plane. After these calls we draw the points:pCurrentCam->CalcFrustumPlanes(); glm::vec4 p[6]; pCurrentCam->GetFrustumPlanes(p); pointShader.Use(); glUniform1f(pointShader("t"), current_time); glUniformMatrix4fv(pointShader("MVP"), 1, GL_FALSE, glm::value_ptr(MVP)); glUniform4fv(pointShader("FrustumPlanes"), 6, glm::value_ptr(p[0])); glBindVertexArray(pointVAOID); glDrawArrays(GL_POINTS,0,MAX_POINTS); pointShader.UnUse();
There are two main parts of this recipe: calculation of the viewing frustum planes and checking if a given point is in the viewing frustum. The first calculation is carried out in the CAbstractCamera::CalcFrustumPlanes()
function. Refer to the Chapter2/src/AbstractCamera.cpp
files for details.
This function iterates through all of the six frustum planes. In each iteration, it checks the signed distance of the given point p
with respect to the ith frustum plane. This is a simple dot product of the plane normal with the given point and adding the plane distance. If the signed distance is negative for any of the planes, the point is outside the viewing frustum so we can safely reject the point. If the point has a positive signed distance for all of the six frustum planes, it is inside the viewing frustum. Note that the frustum planes are oriented in such a way that their normals point inside the viewing frustum.
This function iterates through all of the six frustum planes. In each iteration, it checks the signed distance of the given point p
with respect to the ith frustum plane. This is a simple dot product of the plane normal with the given point and adding the plane distance. If the signed distance is negative for any of the planes, the point is outside the viewing frustum so we can safely reject the point. If the point has a positive signed distance for all of the six frustum planes, it is inside the viewing frustum. Note that the frustum planes are oriented in such a way that their normals point inside the viewing frustum.
Often when working on projects, we need the ability to pick graphical objects on screen. While in OpenGL versions before OpenGL 3.0, the selection buffer was used for this purpose, this buffer is removed in the modern OpenGL 3.3 core profile. However, this leaves us with some alternate methods. We will implement a simple picking technique using the depth buffer in this recipe.
Picking using depth buffer can be implemented as follows:
- Enable depth testing:
glEnable(GL_DEPTH_TEST);
- In the mouse down event handler, read the depth value from the depth buffer using the
glReadPixels
function at the clicked point:glReadPixels( x, HEIGHT-y, 1, 1, GL_DEPTH_COMPONENT, GL_FLOAT, &winZ);
- Unproject the 3D point,
vec3(x,HEIGHT-y,winZ)
, to obtain the object-space point from the clicked screen-space pointx,y
and the depth valuewinZ
. Make sure to invert they
value by subtractingHEIGHT
from the screen-spacey
value:glm::vec3 objPt = glm::unProject(glm::vec3(x,HEIGHT-y,winZ), MV, P, glm::vec4(0,0,WIDTH, HEIGHT));
- Check the distances of all of the scene objects from the object-space point
objPt
. If the distance is within the bounds of the object and the distance of the object is the nearest to the camera, store the index of the object:size_t i=0; float minDist = 1000; selected_box=-1; for(i=0;i<3;i++) { float dist = glm::distance(box_positions[i], objPt); if( dist<1 && dist<minDist) { selected_box = i; minDist = dist; } }
- Based on the selected index, color the object as selected:
glm::mat4 T = glm::translate(glm::mat4(1), box_positions[0]); cube->color = (selected_box==0)?glm::vec3(0,1,1):glm::vec3(1,0,0); cube->Render(glm::value_ptr(MVP*T)); T = glm::translate(glm::mat4(1), box_positions[1]); cube->color = (selected_box==1)?glm::vec3(0,1,1):glm::vec3(0,1,0); cube->Render(glm::value_ptr(MVP*T)); T = glm::translate(glm::mat4(1), box_positions[2]); cube->color = (selected_box==2)?glm::vec3(0,1,1):glm::vec3(0,0,1); cube->Render(glm::value_ptr(MVP*T));
This recipe renders three cubes in red, green, and blue on the screen. When the user clicks on any of these cubes, the depth buffer is read to find the depth value at the clicked point. The object-space point is then obtained by unprojecting (glm::unProject
) the clicked point (x,HEIGHT-y, winZ
). A loop is then iterated over all objects in the scene to find the nearest object to the object-space point. The index of the nearest intersected object is then stored.
Chapter2/Picking_DepthBuffer
folder. Relevant source files are in the Chapter2/src
folder.
Picking using depth buffer can be implemented as follows:
- Enable depth testing:
glEnable(GL_DEPTH_TEST);
- In the mouse down event handler, read the depth value from the depth buffer using the
glReadPixels
function at the clicked point:glReadPixels( x, HEIGHT-y, 1, 1, GL_DEPTH_COMPONENT, GL_FLOAT, &winZ);
- Unproject the 3D point,
vec3(x,HEIGHT-y,winZ)
, to obtain the object-space point from the clicked screen-space pointx,y
and the depth valuewinZ
. Make sure to invert they
value by subtractingHEIGHT
from the screen-spacey
value:glm::vec3 objPt = glm::unProject(glm::vec3(x,HEIGHT-y,winZ), MV, P, glm::vec4(0,0,WIDTH, HEIGHT));
- Check the distances of all of the scene objects from the object-space point
objPt
. If the distance is within the bounds of the object and the distance of the object is the nearest to the camera, store the index of the object:size_t i=0; float minDist = 1000; selected_box=-1; for(i=0;i<3;i++) { float dist = glm::distance(box_positions[i], objPt); if( dist<1 && dist<minDist) { selected_box = i; minDist = dist; } }
- Based on the selected index, color the object as selected:
glm::mat4 T = glm::translate(glm::mat4(1), box_positions[0]); cube->color = (selected_box==0)?glm::vec3(0,1,1):glm::vec3(1,0,0); cube->Render(glm::value_ptr(MVP*T)); T = glm::translate(glm::mat4(1), box_positions[1]); cube->color = (selected_box==1)?glm::vec3(0,1,1):glm::vec3(0,1,0); cube->Render(glm::value_ptr(MVP*T)); T = glm::translate(glm::mat4(1), box_positions[2]); cube->color = (selected_box==2)?glm::vec3(0,1,1):glm::vec3(0,0,1); cube->Render(glm::value_ptr(MVP*T));
This recipe renders three cubes in red, green, and blue on the screen. When the user clicks on any of these cubes, the depth buffer is read to find the depth value at the clicked point. The object-space point is then obtained by unprojecting (glm::unProject
) the clicked point (x,HEIGHT-y, winZ
). A loop is then iterated over all objects in the scene to find the nearest object to the object-space point. The index of the nearest intersected object is then stored.
glEnable(GL_DEPTH_TEST);
glReadPixels
function at the clicked point:glReadPixels( x, HEIGHT-y, 1, 1, GL_DEPTH_COMPONENT, GL_FLOAT, &winZ);
vec3(x,HEIGHT-y,winZ)
, to obtain the object-space point from the clicked screen-space point x,y
and the depth value winZ
. Make sure to invert the y
value by subtracting HEIGHT
from the screen-space y
value:glm::vec3 objPt = glm::unProject(glm::vec3(x,HEIGHT-y,winZ), MV, P, glm::vec4(0,0,WIDTH, HEIGHT));
objPt
. If the distance is within the bounds of the object and the distance of the object is the nearest to the camera, store the index of the object:size_t i=0; float minDist = 1000; selected_box=-1; for(i=0;i<3;i++) { float dist = glm::distance(box_positions[i], objPt); if( dist<1 && dist<minDist) { selected_box = i; minDist = dist; } }
glm::mat4 T = glm::translate(glm::mat4(1), box_positions[0]); cube->color = (selected_box==0)?glm::vec3(0,1,1):glm::vec3(1,0,0); cube->Render(glm::value_ptr(MVP*T)); T = glm::translate(glm::mat4(1), box_positions[1]); cube->color = (selected_box==1)?glm::vec3(0,1,1):glm::vec3(0,1,0); cube->Render(glm::value_ptr(MVP*T)); T = glm::translate(glm::mat4(1), box_positions[2]); cube->color = (selected_box==2)?glm::vec3(0,1,1):glm::vec3(0,0,1); cube->Render(glm::value_ptr(MVP*T));
This recipe renders three cubes in red, green, and blue on the screen. When the user clicks on any of these cubes, the depth buffer is read to find the depth value at the clicked point. The object-space point is then obtained by unprojecting (glm::unProject
) the clicked point (x,HEIGHT-y, winZ
). A loop is then iterated over all objects in the scene to find the nearest object to the object-space point. The index of the nearest intersected object is then stored.
recipe renders three cubes in red, green, and blue on the screen. When the user clicks on any of these cubes, the depth buffer is read to find the depth value at the clicked point. The object-space point is then obtained by unprojecting (glm::unProject
) the clicked point (x,HEIGHT-y, winZ
). A loop is then iterated over all objects in the scene to find the nearest object to the object-space point. The index of the nearest intersected object is then stored.
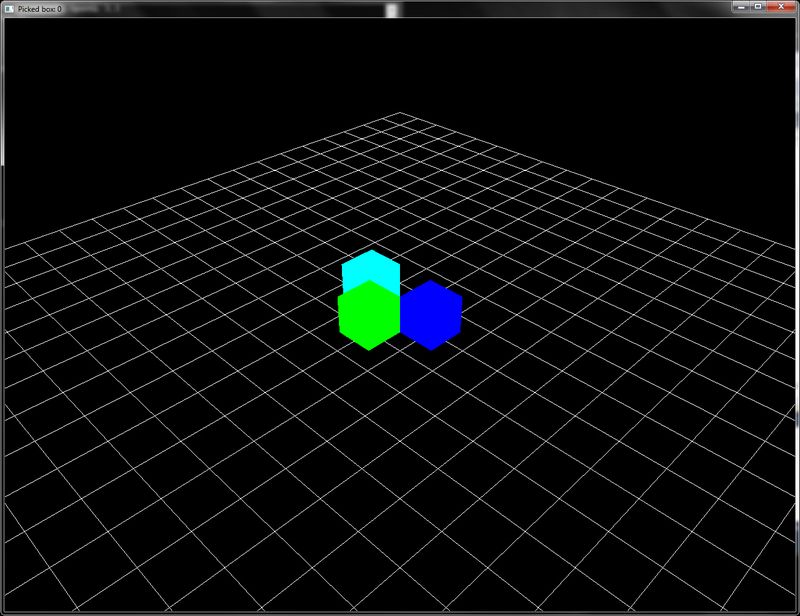
Another method which is used for picking objects in a 3D world is color-based picking. In this recipe, we will use the same scene as in the last recipe.
To enable picking with the color buffer, the following steps are needed:
- Disable dithering. This is done to prevent any color mismatch during the query:
glDisable(GL_DITHER);
- In the mouse down event handler, read the color value at the clicked position from the color buffer using the
glReadPixels
function:GLubyte pixel[4]; glReadPixels(x, HEIGHT-y, 1, 1, GL_RGBA, GL_UNSIGNED_BYTE, pixel);
- Compare the color value at the clicked point to the color values of all objects to find the intersection:
selected_box=-1; if(pixel[0]==255 && pixel[1]==0 && pixel[2]==0) { cout<<"picked box 1"<<endl; selected_box = 0; } if(pixel[0]==0 && pixel[1]==255 && pixel[2]==0) { cout<<"picked box 2"<<endl; selected_box = 1; } if(pixel[0]==0 && pixel[1]==0 && pixel[2]==255) { cout<<"picked box 3"<<endl; selected_box = 2; }
This method is simple to implement. We simply check the color of the pixel where the mouse is clicked. Since dithering might generate a different color value, we disable dithering. The pixel's r, g, and b values are then checked against all of the scene objects and the appropriate object is selected. We could also have used the float data type, GL_FLOAT
, when reading and comparing the pixel value. However, due to floating point imprecision, we might not have an accurate test. Therefore, we use the integral data type GL_UNSIGNED_BYTE
.
Chapter2/Picking_ColorBuffer
folder. Relevant source files are in the Chapter2/src
folder.
To enable picking with the color buffer, the following steps are needed:
- Disable dithering. This is done to prevent any color mismatch during the query:
glDisable(GL_DITHER);
- In the mouse down event handler, read the color value at the clicked position from the color buffer using the
glReadPixels
function:GLubyte pixel[4]; glReadPixels(x, HEIGHT-y, 1, 1, GL_RGBA, GL_UNSIGNED_BYTE, pixel);
- Compare the color value at the clicked point to the color values of all objects to find the intersection:
selected_box=-1; if(pixel[0]==255 && pixel[1]==0 && pixel[2]==0) { cout<<"picked box 1"<<endl; selected_box = 0; } if(pixel[0]==0 && pixel[1]==255 && pixel[2]==0) { cout<<"picked box 2"<<endl; selected_box = 1; } if(pixel[0]==0 && pixel[1]==0 && pixel[2]==255) { cout<<"picked box 3"<<endl; selected_box = 2; }
This method is simple to implement. We simply check the color of the pixel where the mouse is clicked. Since dithering might generate a different color value, we disable dithering. The pixel's r, g, and b values are then checked against all of the scene objects and the appropriate object is selected. We could also have used the float data type, GL_FLOAT
, when reading and comparing the pixel value. However, due to floating point imprecision, we might not have an accurate test. Therefore, we use the integral data type GL_UNSIGNED_BYTE
.
glDisable(GL_DITHER);
glReadPixels
function:GLubyte pixel[4]; glReadPixels(x, HEIGHT-y, 1, 1, GL_RGBA, GL_UNSIGNED_BYTE, pixel);
selected_box=-1; if(pixel[0]==255 && pixel[1]==0 && pixel[2]==0) { cout<<"picked box 1"<<endl; selected_box = 0; } if(pixel[0]==0 && pixel[1]==255 && pixel[2]==0) { cout<<"picked box 2"<<endl; selected_box = 1; } if(pixel[0]==0 && pixel[1]==0 && pixel[2]==255) { cout<<"picked box 3"<<endl; selected_box = 2; }
This method is simple to implement. We simply check the color of the pixel where the mouse is clicked. Since dithering might generate a different color value, we disable dithering. The pixel's r, g, and b values are then checked against all of the scene objects and the appropriate object is selected. We could also have used the float data type, GL_FLOAT
, when reading and comparing the pixel value. However, due to floating point imprecision, we might not have an accurate test. Therefore, we use the integral data type GL_UNSIGNED_BYTE
.
method is simple to implement. We simply check the color of the pixel where the mouse is clicked. Since dithering might generate a different color value, we disable dithering. The pixel's r, g, and b values are then checked against all of the scene objects and the appropriate object is selected. We could also have used the float data type, GL_FLOAT
, when reading and comparing the pixel value. However, due to floating point imprecision, we might not have an accurate test. Therefore, we use the integral data type GL_UNSIGNED_BYTE
.
The final method we will cover for picking involves casting rays in the scene to determine the nearest object to the viewer. We will use the same scene as in the last two recipes, three cubes (red, green, and blue colored) placed near the origin.
For picking with scene intersection queries, take the following steps:
- Get two object-space points by unprojecting the screen-space point (
x, HEIGHT-y
), with different depth value, one at z=0 and the other at z=1:glm::vec3 start = glm::unProject(glm::vec3(x,HEIGHT-y,0), MV, P, glm::vec4(0,0,WIDTH,HEIGHT)); glm::vec3 end = glm::unProject(glm::vec3(x,HEIGHT-y,1), MV, P, glm::vec4(0,0,WIDTH,HEIGHT));
- Get the current camera position as
eyeRay.origin
and geteyeRay.direction
by subtracting and normalizing the difference of the two object-space points,end
andstart
, as follows:eyeRay.origin = cam.GetPosition(); eyeRay.direction = glm::normalize(end-start);
- For all of the objects in the scene, find the intersection of the eye ray with the Axially Aligned Bounding Box (AABB) of the object. Store the nearest intersected object index:
float tMin = numeric_limits<float>::max(); selected_box = -1; for(int i=0;i<3;i++) { glm::vec2 tMinMax = intersectBox(eyeRay, boxes[i]); if(tMinMax.x<tMinMax.y && tMinMax.x<tMin) { selected_box=i; tMin = tMinMax.x; } } if(selected_box==-1) cout<<"No box picked"<<endl; else cout<<"Selected box: "<<selected_box<<endl;
The method discussed in this recipe first casts a ray from the camera origin in the clicked direction, and then checks all of the scene objects' bounding boxes for intersection. There are two sub parts: estimation of the ray direction from the clicked point and the ray AABB intersection. We first focus on the estimation of the ray direction from the clicked point.
After calculating the eye ray, we check it for intersection with all of the scene geometries. If the object-bounding box intersects the eye ray and it is the nearest intersection, we store the index of the object. The intersectBox
function is defined as follows:
The intersectBox
function works by finding the intersection of the ray with a pair of slabs for each of the three axes individually. Next it finds the tNear
and tFar
values. The box can only intersect with the ray if tNear
is less than tFar
for all of the three axes. So the code finds the smallest tFar
value and the largest tMin
value. If the smallest tFar
value is less than the largest tNear
value, the ray misses the box. For further details, refer to the See also section. The output result from the demonstration application for this recipe uses the same scene as in the last two recipes. In this case also, left-clicking the mouse selects the box, which is highlighted in cyan, as shown in the following figure:
Chapter2/Picking_SceneIntersection
folder. Relevant source files are in the Chapter2/src
folder.
For picking with scene intersection queries, take the following steps:
- Get two object-space points by unprojecting the screen-space point (
x, HEIGHT-y
), with different depth value, one at z=0 and the other at z=1:glm::vec3 start = glm::unProject(glm::vec3(x,HEIGHT-y,0), MV, P, glm::vec4(0,0,WIDTH,HEIGHT)); glm::vec3 end = glm::unProject(glm::vec3(x,HEIGHT-y,1), MV, P, glm::vec4(0,0,WIDTH,HEIGHT));
- Get the current camera position as
eyeRay.origin
and geteyeRay.direction
by subtracting and normalizing the difference of the two object-space points,end
andstart
, as follows:eyeRay.origin = cam.GetPosition(); eyeRay.direction = glm::normalize(end-start);
- For all of the objects in the scene, find the intersection of the eye ray with the Axially Aligned Bounding Box (AABB) of the object. Store the nearest intersected object index:
float tMin = numeric_limits<float>::max(); selected_box = -1; for(int i=0;i<3;i++) { glm::vec2 tMinMax = intersectBox(eyeRay, boxes[i]); if(tMinMax.x<tMinMax.y && tMinMax.x<tMin) { selected_box=i; tMin = tMinMax.x; } } if(selected_box==-1) cout<<"No box picked"<<endl; else cout<<"Selected box: "<<selected_box<<endl;
The method discussed in this recipe first casts a ray from the camera origin in the clicked direction, and then checks all of the scene objects' bounding boxes for intersection. There are two sub parts: estimation of the ray direction from the clicked point and the ray AABB intersection. We first focus on the estimation of the ray direction from the clicked point.
After calculating the eye ray, we check it for intersection with all of the scene geometries. If the object-bounding box intersects the eye ray and it is the nearest intersection, we store the index of the object. The intersectBox
function is defined as follows:
The intersectBox
function works by finding the intersection of the ray with a pair of slabs for each of the three axes individually. Next it finds the tNear
and tFar
values. The box can only intersect with the ray if tNear
is less than tFar
for all of the three axes. So the code finds the smallest tFar
value and the largest tMin
value. If the smallest tFar
value is less than the largest tNear
value, the ray misses the box. For further details, refer to the See also section. The output result from the demonstration application for this recipe uses the same scene as in the last two recipes. In this case also, left-clicking the mouse selects the box, which is highlighted in cyan, as shown in the following figure:
x, HEIGHT-y
), with different depth value, one at z=0 and the other at z=1:glm::vec3 start = glm::unProject(glm::vec3(x,HEIGHT-y,0), MV, P, glm::vec4(0,0,WIDTH,HEIGHT)); glm::vec3 end = glm::unProject(glm::vec3(x,HEIGHT-y,1), MV, P, glm::vec4(0,0,WIDTH,HEIGHT));
eyeRay.origin
and get eyeRay.direction
by subtracting and normalizing the difference of the two object-space points, end
and start
, as follows:eyeRay.origin = cam.GetPosition(); eyeRay.direction = glm::normalize(end-start);
The method discussed in this recipe first casts a ray from the camera origin in the clicked direction, and then checks all of the scene objects' bounding boxes for intersection. There are two sub parts: estimation of the ray direction from the clicked point and the ray AABB intersection. We first focus on the estimation of the ray direction from the clicked point.
After calculating the eye ray, we check it for intersection with all of the scene geometries. If the object-bounding box intersects the eye ray and it is the nearest intersection, we store the index of the object. The intersectBox
function is defined as follows:
The intersectBox
function works by finding the intersection of the ray with a pair of slabs for each of the three axes individually. Next it finds the tNear
and tFar
values. The box can only intersect with the ray if tNear
is less than tFar
for all of the three axes. So the code finds the smallest tFar
value and the largest tMin
value. If the smallest tFar
value is less than the largest tNear
value, the ray misses the box. For further details, refer to the See also section. The output result from the demonstration application for this recipe uses the same scene as in the last two recipes. In this case also, left-clicking the mouse selects the box, which is highlighted in cyan, as shown in the following figure:
discussed in this recipe first casts a ray from the camera origin in the clicked direction, and then checks all of the scene objects' bounding boxes for intersection. There are two sub parts: estimation of the ray direction from the clicked point and the ray AABB intersection. We first focus on the estimation of the ray direction from the clicked point.
After calculating the eye ray, we check it for intersection with all of the scene geometries. If the object-bounding box intersects the eye ray and it is the nearest intersection, we store the index of the object. The intersectBox
function is defined as follows:
The intersectBox
function works by finding the intersection of the ray with a pair of slabs for each of the three axes individually. Next it finds the tNear
and tFar
values. The box can only intersect with the ray if tNear
is less than tFar
for all of the three axes. So the code finds the smallest tFar
value and the largest tMin
value. If the smallest tFar
value is less than the largest tNear
value, the ray misses the box. For further details, refer to the See also section. The output result from the demonstration application for this recipe uses the same scene as in the last two recipes. In this case also, left-clicking the mouse selects the box, which is highlighted in cyan, as shown in the following figure:
intersectBox
function
In this chapter, we will cover:
- Implementing the twirl filter using fragment shader
- Rendering a skybox using static cube mapping
- Implementing a mirror with render-to-texture using FBO
- Rendering a reflective object using dynamic cube mapping
- Implementing area filtering (sharpening/blurring/embossing) on an image using convolution
- Implementing the glow effect
Offscreen rendering functionality is a powerful feature of modern graphics API. In modern OpenGL, this is implemented by using the Framebuffer objects (FBOs). Some of the applications of the offscreen rendering include post processing effects such as glows, dynamic cubemaps, mirror effect, deferred rendering techniques, image processing techniques, and so on. Nowadays almost all games use this feature to carry out stunning visual effects with high rendering quality and detail. With the FBOs, the offscreen rendering is greatly simplified, as the programmer uses FBO the way he would use any other OpenGL object. This chapter will focus on using FBO to carry out image processing effects for implementing digital convolution and glow. In addition, we will also elaborate on how to use the FBO for mirror effect and dynamic cube mapping.
We will use a simple image manipulation operator in the fragment shader by implementing the twirl filter on the GPU.
This recipe builds up on the image loading recipe from Chapter 1, Introduction to Modern OpenGL. The code for this recipe is contained in the Chapter3/TwirlFilter
directory.
Let us get started with the recipe as follows:
- Load the image as in the
ImageLoader
recipe from Chapter 1, Introduction to Modern OpenGL. Set the texture wrap mode toGL_CLAMP_TO_BORDER
.int texture_width = 0, texture_height = 0, channels=0; GLubyte* pData = SOIL_load_image(filename.c_str(), &texture_width, &texture_height, &channels, SOIL_LOAD_AUTO); int i,j; for( j = 0; j*2 < texture_height; ++j ) { int index1 = j * texture_width * channels; int index2 = (texture_height - 1 - j) * texture_width * channels; for( i = texture_width * channels; i > 0; --i ) { GLubyte temp = pData[index1]; pData[index1] = pData[index2]; pData[index2] = temp; ++index1; ++index2; } } glGenTextures(1, &textureID); glActiveTexture(GL_TEXTURE0); glBindTexture(GL_TEXTURE_2D, textureID); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MIN_FILTER, GL_LINEAR); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MAG_FILTER, GL_LINEAR); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_S, GL_CLAMP_TO_BORDER); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_T, GL_CLAMP_TO_BORDER); glTexImage2D(GL_TEXTURE_2D, 0, GL_RGB, texture_width, texture_height, 0, GL_RGB, GL_UNSIGNED_BYTE, pData); SOIL_free_image_data(pData);
- Set up a simple pass through vertex shader that outputs the texture coordinates for texture lookup in the fragment shader, as given in the
ImageLoader
recipe of Chapter 1.void main() { gl_Position = vec4(vVertex*2.0-1,0,1); vUV = vVertex; }
- Set up the fragment shader that first shifts the texture coordinates, performs the twirl transformation, and then converts the shifted texture coordinates back for texture lookup.
void main() { vec2 uv = vUV-0.5; float angle = atan(uv.y, uv.x); float radius = length(uv); angle+= radius*twirl_amount; vec2 shifted = radius* vec2(cos(angle), sin(angle)); vFragColor = texture(textureMap, (shifted+0.5)); }
- Render a 2D screen space quad and apply the two shaders as was done in the
ImageLoader
recipe in Chapter 1.void OnRender() { glClear(GL_COLOR_BUFFER_BIT|GL_DEPTH_BUFFER_BIT); shader.Use(); glUniform1f(shader("twirl_amount"), twirl_amount); glDrawElements(GL_TRIANGLES, 6, GL_UNSIGNED_SHORT, 0); shader.UnUse(); glutSwapBuffers(); }
Here, x and y are the two Cartesian coordinates. In the fragment shader, we first offset the texture coordinates so that the origin is at the center of the image. Next, we get the angle θ and radius r.
Since the texture clamping mode was set to GL_CLAMP_TO_BORDER
, the out of image pixels get the black color. In this recipe, we applied the twirl effect to the whole image. As an exercise, we invite the reader to limit the twirl to a specific zone within the image; for example, within a radius of, say, 150 pixels from the center of image. Hint: You can constrain the radius using the given pixel distance.
Chapter 1, Introduction to Modern OpenGL. The code for this recipe is contained in the Chapter3/TwirlFilter
directory.
Let us get started with the recipe as follows:
- Load the image as in the
ImageLoader
recipe from Chapter 1, Introduction to Modern OpenGL. Set the texture wrap mode toGL_CLAMP_TO_BORDER
.int texture_width = 0, texture_height = 0, channels=0; GLubyte* pData = SOIL_load_image(filename.c_str(), &texture_width, &texture_height, &channels, SOIL_LOAD_AUTO); int i,j; for( j = 0; j*2 < texture_height; ++j ) { int index1 = j * texture_width * channels; int index2 = (texture_height - 1 - j) * texture_width * channels; for( i = texture_width * channels; i > 0; --i ) { GLubyte temp = pData[index1]; pData[index1] = pData[index2]; pData[index2] = temp; ++index1; ++index2; } } glGenTextures(1, &textureID); glActiveTexture(GL_TEXTURE0); glBindTexture(GL_TEXTURE_2D, textureID); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MIN_FILTER, GL_LINEAR); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MAG_FILTER, GL_LINEAR); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_S, GL_CLAMP_TO_BORDER); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_T, GL_CLAMP_TO_BORDER); glTexImage2D(GL_TEXTURE_2D, 0, GL_RGB, texture_width, texture_height, 0, GL_RGB, GL_UNSIGNED_BYTE, pData); SOIL_free_image_data(pData);
- Set up a simple pass through vertex shader that outputs the texture coordinates for texture lookup in the fragment shader, as given in the
ImageLoader
recipe of Chapter 1.void main() { gl_Position = vec4(vVertex*2.0-1,0,1); vUV = vVertex; }
- Set up the fragment shader that first shifts the texture coordinates, performs the twirl transformation, and then converts the shifted texture coordinates back for texture lookup.
void main() { vec2 uv = vUV-0.5; float angle = atan(uv.y, uv.x); float radius = length(uv); angle+= radius*twirl_amount; vec2 shifted = radius* vec2(cos(angle), sin(angle)); vFragColor = texture(textureMap, (shifted+0.5)); }
- Render a 2D screen space quad and apply the two shaders as was done in the
ImageLoader
recipe in Chapter 1.void OnRender() { glClear(GL_COLOR_BUFFER_BIT|GL_DEPTH_BUFFER_BIT); shader.Use(); glUniform1f(shader("twirl_amount"), twirl_amount); glDrawElements(GL_TRIANGLES, 6, GL_UNSIGNED_SHORT, 0); shader.UnUse(); glutSwapBuffers(); }
Here, x and y are the two Cartesian coordinates. In the fragment shader, we first offset the texture coordinates so that the origin is at the center of the image. Next, we get the angle θ and radius r.
Since the texture clamping mode was set to GL_CLAMP_TO_BORDER
, the out of image pixels get the black color. In this recipe, we applied the twirl effect to the whole image. As an exercise, we invite the reader to limit the twirl to a specific zone within the image; for example, within a radius of, say, 150 pixels from the center of image. Hint: You can constrain the radius using the given pixel distance.
ImageLoader
recipe from
- Chapter 1, Introduction to Modern OpenGL. Set the texture wrap mode to
GL_CLAMP_TO_BORDER
.int texture_width = 0, texture_height = 0, channels=0; GLubyte* pData = SOIL_load_image(filename.c_str(), &texture_width, &texture_height, &channels, SOIL_LOAD_AUTO); int i,j; for( j = 0; j*2 < texture_height; ++j ) { int index1 = j * texture_width * channels; int index2 = (texture_height - 1 - j) * texture_width * channels; for( i = texture_width * channels; i > 0; --i ) { GLubyte temp = pData[index1]; pData[index1] = pData[index2]; pData[index2] = temp; ++index1; ++index2; } } glGenTextures(1, &textureID); glActiveTexture(GL_TEXTURE0); glBindTexture(GL_TEXTURE_2D, textureID); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MIN_FILTER, GL_LINEAR); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MAG_FILTER, GL_LINEAR); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_S, GL_CLAMP_TO_BORDER); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_T, GL_CLAMP_TO_BORDER); glTexImage2D(GL_TEXTURE_2D, 0, GL_RGB, texture_width, texture_height, 0, GL_RGB, GL_UNSIGNED_BYTE, pData); SOIL_free_image_data(pData);
- Set up a simple pass through vertex shader that outputs the texture coordinates for texture lookup in the fragment shader, as given in the
ImageLoader
recipe of Chapter 1.void main() { gl_Position = vec4(vVertex*2.0-1,0,1); vUV = vVertex; }
- Set up the fragment shader that first shifts the texture coordinates, performs the twirl transformation, and then converts the shifted texture coordinates back for texture lookup.
void main() { vec2 uv = vUV-0.5; float angle = atan(uv.y, uv.x); float radius = length(uv); angle+= radius*twirl_amount; vec2 shifted = radius* vec2(cos(angle), sin(angle)); vFragColor = texture(textureMap, (shifted+0.5)); }
- Render a 2D screen space quad and apply the two shaders as was done in the
ImageLoader
recipe in Chapter 1.void OnRender() { glClear(GL_COLOR_BUFFER_BIT|GL_DEPTH_BUFFER_BIT); shader.Use(); glUniform1f(shader("twirl_amount"), twirl_amount); glDrawElements(GL_TRIANGLES, 6, GL_UNSIGNED_SHORT, 0); shader.UnUse(); glutSwapBuffers(); }
Here, x and y are the two Cartesian coordinates. In the fragment shader, we first offset the texture coordinates so that the origin is at the center of the image. Next, we get the angle θ and radius r.
Since the texture clamping mode was set to GL_CLAMP_TO_BORDER
, the out of image pixels get the black color. In this recipe, we applied the twirl effect to the whole image. As an exercise, we invite the reader to limit the twirl to a specific zone within the image; for example, within a radius of, say, 150 pixels from the center of image. Hint: You can constrain the radius using the given pixel distance.

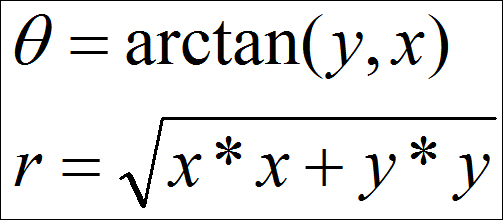
are the two Cartesian coordinates. In the fragment shader, we first offset the texture coordinates so that the origin is at the center of the image. Next, we get the angle θ and radius r.
Since the texture clamping mode was set to GL_CLAMP_TO_BORDER
, the out of image pixels get the black color. In this recipe, we applied the twirl effect to the whole image. As an exercise, we invite the reader to limit the twirl to a specific zone within the image; for example, within a radius of, say, 150 pixels from the center of image. Hint: You can constrain the radius using the given pixel distance.
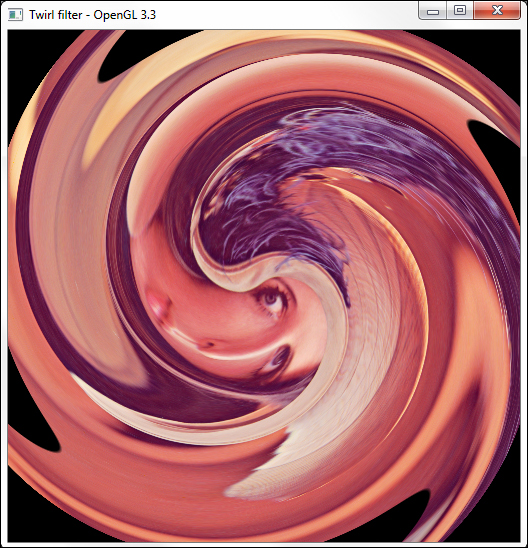
texture clamping mode was set to GL_CLAMP_TO_BORDER
, the out of image pixels get the black color. In this recipe, we applied the twirl effect to the whole image. As an exercise, we invite the reader to limit the twirl to a specific zone within the image; for example, within a radius of, say, 150 pixels from the center of image. Hint: You can constrain the radius using the given pixel distance.
This recipe will show how to render a skybox object using static cube mapping. Cube mapping is a simple technique for generating a surrounding environment. There are several methods, such as sky dome, which uses a spherical geometry; skybox, which uses a cubical geometry; and skyplane, which uses a planar geometry. For this recipe, we will focus on skyboxes using the static cube mapping approach. The cube mapping process needs six images that are placed on each face of a cube. The skybox is a very large cube that moves with the camera but does not rotate with it.
Let us get started with the recipe as follows:
- Set up the vertex array and vertex buffer objects to store a unit cube geometry.
- Load the skybox images using an image loading library, such as
SOIL
.int texture_widths[6]; int texture_heights[6]; int channels[6]; GLubyte* pData[6]; cout<<"Loading skybox images: ..."<<endl; for(int i=0;i<6;i++) { cout<<"\tLoading: "<<texture_names[i]<<" ... "; pData[i] = SOIL_load_image(texture_names[i], &texture_widths[i], &texture_heights[i], &channels[i], SOIL_LOAD_AUTO); cout<<"done."<<endl; }
- Generate a cubemap OpenGL texture object and bind the six loaded images to the
GL_TEXTURE_CUBE_MAP
texture targets. Also make sure that the image data loaded by theSOIL
library is deleted after the texture data has been stored into the OpenGL texture.glGenTextures(1, &skyboxTextureID); glActiveTexture(GL_TEXTURE0); glBindTexture(GL_TEXTURE_CUBE_MAP, skyboxTextureID); glTexParameteri(GL_TEXTURE_CUBE_MAP, GL_TEXTURE_MIN_FILTER, GL_LINEAR); glTexParameteri(GL_TEXTURE_CUBE_MAP, GL_TEXTURE_MAG_FILTER, GL_LINEAR); glTexParameteri(GL_TEXTURE_CUBE_MAP, GL_TEXTURE_WRAP_S, GL_CLAMP_TO_EDGE); glTexParameteri(GL_TEXTURE_CUBE_MAP, GL_TEXTURE_WRAP_T, GL_CLAMP_TO_EDGE); glTexParameteri(GL_TEXTURE_CUBE_MAP, GL_TEXTURE_WRAP_R, GL_CLAMP_TO_EDGE); GLint format = (channels[0]==4)?GL_RGBA:GL_RGB; for(int i=0;i<6;i++) { glTexImage2D(GL_TEXTURE_CUBE_MAP_POSITIVE_X + i, 0, format,texture_widths[i], texture_heights[i], 0, format,GL_UNSIGNED_BYTE, pData[i]); SOIL_free_image_data(pData[i]); }
- Set up a vertex shader (see
Chapter3/Skybox/shaders/skybox.vert
) that outputs the vertex's object space position as the texture coordinate.smooth out vec3 uv; void main() { gl_Position = MVP*vec4(vVertex,1); uv = vVertex; }
- Add a cubemap sampler to the fragment shader. Use the texture coordinates output from the vertex shader to sample the cubemap sampler object in the fragment shader (see
Chapter3/Skybox/shaders/skybox.frag
).layout(location=0) out vec4 vFragColor; uniform samplerCube cubeMap; smooth in vec3 uv; void main() { vFragColor = texture(cubeMap, uv); }
There are two parts of this recipe. The first part, which loads an OpenGL cubemap texture, is self explanatory. We load the six images and bind these to an OpenGL cubemap texture target. There are six cubemap texture targets corresponding to the six sides of a cube. These targets are GL_TEXTURE_CUBE_MAP_POSITIVE_X
, GL_TEXTURE_CUBE_MAP_POSITIVE_Y
, GL_TEXTURE_CUBE_MAP_POSITIVE_Z
, GL_TEXTURE_CUBE_MAP_NEGATIVE_X
, GL_TEXTURE_CUBE_MAP_NEGATIVE_Y
, and GL_TEXTURE_CUBE_MAP_NEGATIVE_Z
. Since their identifiers are linearly generated, we offset the target by the loop variable to move to the next cubemap texture target in the following code:
To sample the correct location in the cubemap texture we need a vector. This vector can be obtained from the object space vertex positions that are passed to the vertex shader. These are passed through the uv
output attribute to the fragment shader.
Chapter3/Skybox
directory.
Let us get started with the recipe as follows:
- Set up the vertex array and vertex buffer objects to store a unit cube geometry.
- Load the skybox images using an image loading library, such as
SOIL
.int texture_widths[6]; int texture_heights[6]; int channels[6]; GLubyte* pData[6]; cout<<"Loading skybox images: ..."<<endl; for(int i=0;i<6;i++) { cout<<"\tLoading: "<<texture_names[i]<<" ... "; pData[i] = SOIL_load_image(texture_names[i], &texture_widths[i], &texture_heights[i], &channels[i], SOIL_LOAD_AUTO); cout<<"done."<<endl; }
- Generate a cubemap OpenGL texture object and bind the six loaded images to the
GL_TEXTURE_CUBE_MAP
texture targets. Also make sure that the image data loaded by theSOIL
library is deleted after the texture data has been stored into the OpenGL texture.glGenTextures(1, &skyboxTextureID); glActiveTexture(GL_TEXTURE0); glBindTexture(GL_TEXTURE_CUBE_MAP, skyboxTextureID); glTexParameteri(GL_TEXTURE_CUBE_MAP, GL_TEXTURE_MIN_FILTER, GL_LINEAR); glTexParameteri(GL_TEXTURE_CUBE_MAP, GL_TEXTURE_MAG_FILTER, GL_LINEAR); glTexParameteri(GL_TEXTURE_CUBE_MAP, GL_TEXTURE_WRAP_S, GL_CLAMP_TO_EDGE); glTexParameteri(GL_TEXTURE_CUBE_MAP, GL_TEXTURE_WRAP_T, GL_CLAMP_TO_EDGE); glTexParameteri(GL_TEXTURE_CUBE_MAP, GL_TEXTURE_WRAP_R, GL_CLAMP_TO_EDGE); GLint format = (channels[0]==4)?GL_RGBA:GL_RGB; for(int i=0;i<6;i++) { glTexImage2D(GL_TEXTURE_CUBE_MAP_POSITIVE_X + i, 0, format,texture_widths[i], texture_heights[i], 0, format,GL_UNSIGNED_BYTE, pData[i]); SOIL_free_image_data(pData[i]); }
- Set up a vertex shader (see
Chapter3/Skybox/shaders/skybox.vert
) that outputs the vertex's object space position as the texture coordinate.smooth out vec3 uv; void main() { gl_Position = MVP*vec4(vVertex,1); uv = vVertex; }
- Add a cubemap sampler to the fragment shader. Use the texture coordinates output from the vertex shader to sample the cubemap sampler object in the fragment shader (see
Chapter3/Skybox/shaders/skybox.frag
).layout(location=0) out vec4 vFragColor; uniform samplerCube cubeMap; smooth in vec3 uv; void main() { vFragColor = texture(cubeMap, uv); }
There are two parts of this recipe. The first part, which loads an OpenGL cubemap texture, is self explanatory. We load the six images and bind these to an OpenGL cubemap texture target. There are six cubemap texture targets corresponding to the six sides of a cube. These targets are GL_TEXTURE_CUBE_MAP_POSITIVE_X
, GL_TEXTURE_CUBE_MAP_POSITIVE_Y
, GL_TEXTURE_CUBE_MAP_POSITIVE_Z
, GL_TEXTURE_CUBE_MAP_NEGATIVE_X
, GL_TEXTURE_CUBE_MAP_NEGATIVE_Y
, and GL_TEXTURE_CUBE_MAP_NEGATIVE_Z
. Since their identifiers are linearly generated, we offset the target by the loop variable to move to the next cubemap texture target in the following code:
To sample the correct location in the cubemap texture we need a vector. This vector can be obtained from the object space vertex positions that are passed to the vertex shader. These are passed through the uv
output attribute to the fragment shader.
- skybox images using an image loading library, such as
SOIL
.int texture_widths[6]; int texture_heights[6]; int channels[6]; GLubyte* pData[6]; cout<<"Loading skybox images: ..."<<endl; for(int i=0;i<6;i++) { cout<<"\tLoading: "<<texture_names[i]<<" ... "; pData[i] = SOIL_load_image(texture_names[i], &texture_widths[i], &texture_heights[i], &channels[i], SOIL_LOAD_AUTO); cout<<"done."<<endl; }
- Generate a cubemap OpenGL texture object and bind the six loaded images to the
GL_TEXTURE_CUBE_MAP
texture targets. Also make sure that the image data loaded by theSOIL
library is deleted after the texture data has been stored into the OpenGL texture.glGenTextures(1, &skyboxTextureID); glActiveTexture(GL_TEXTURE0); glBindTexture(GL_TEXTURE_CUBE_MAP, skyboxTextureID); glTexParameteri(GL_TEXTURE_CUBE_MAP, GL_TEXTURE_MIN_FILTER, GL_LINEAR); glTexParameteri(GL_TEXTURE_CUBE_MAP, GL_TEXTURE_MAG_FILTER, GL_LINEAR); glTexParameteri(GL_TEXTURE_CUBE_MAP, GL_TEXTURE_WRAP_S, GL_CLAMP_TO_EDGE); glTexParameteri(GL_TEXTURE_CUBE_MAP, GL_TEXTURE_WRAP_T, GL_CLAMP_TO_EDGE); glTexParameteri(GL_TEXTURE_CUBE_MAP, GL_TEXTURE_WRAP_R, GL_CLAMP_TO_EDGE); GLint format = (channels[0]==4)?GL_RGBA:GL_RGB; for(int i=0;i<6;i++) { glTexImage2D(GL_TEXTURE_CUBE_MAP_POSITIVE_X + i, 0, format,texture_widths[i], texture_heights[i], 0, format,GL_UNSIGNED_BYTE, pData[i]); SOIL_free_image_data(pData[i]); }
- Set up a vertex shader (see
Chapter3/Skybox/shaders/skybox.vert
) that outputs the vertex's object space position as the texture coordinate.smooth out vec3 uv; void main() { gl_Position = MVP*vec4(vVertex,1); uv = vVertex; }
- Add a cubemap sampler to the fragment shader. Use the texture coordinates output from the vertex shader to sample the cubemap sampler object in the fragment shader (see
Chapter3/Skybox/shaders/skybox.frag
).layout(location=0) out vec4 vFragColor; uniform samplerCube cubeMap; smooth in vec3 uv; void main() { vFragColor = texture(cubeMap, uv); }
There are two parts of this recipe. The first part, which loads an OpenGL cubemap texture, is self explanatory. We load the six images and bind these to an OpenGL cubemap texture target. There are six cubemap texture targets corresponding to the six sides of a cube. These targets are GL_TEXTURE_CUBE_MAP_POSITIVE_X
, GL_TEXTURE_CUBE_MAP_POSITIVE_Y
, GL_TEXTURE_CUBE_MAP_POSITIVE_Z
, GL_TEXTURE_CUBE_MAP_NEGATIVE_X
, GL_TEXTURE_CUBE_MAP_NEGATIVE_Y
, and GL_TEXTURE_CUBE_MAP_NEGATIVE_Z
. Since their identifiers are linearly generated, we offset the target by the loop variable to move to the next cubemap texture target in the following code:
To sample the correct location in the cubemap texture we need a vector. This vector can be obtained from the object space vertex positions that are passed to the vertex shader. These are passed through the uv
output attribute to the fragment shader.
two parts of this recipe. The first part, which loads an OpenGL cubemap texture, is self explanatory. We load the six images and bind these to an OpenGL cubemap texture target. There are six cubemap texture targets corresponding to the six sides of a cube. These targets are GL_TEXTURE_CUBE_MAP_POSITIVE_X
, GL_TEXTURE_CUBE_MAP_POSITIVE_Y
, GL_TEXTURE_CUBE_MAP_POSITIVE_Z
, GL_TEXTURE_CUBE_MAP_NEGATIVE_X
, GL_TEXTURE_CUBE_MAP_NEGATIVE_Y
, and GL_TEXTURE_CUBE_MAP_NEGATIVE_Z
. Since their identifiers are linearly generated, we offset the target by the loop variable to move to the next cubemap texture target in the following code:
To sample the correct location in the cubemap texture we need a vector. This vector can be obtained from the object space vertex positions that are passed to the vertex shader. These are passed through the uv
output attribute to the fragment shader.
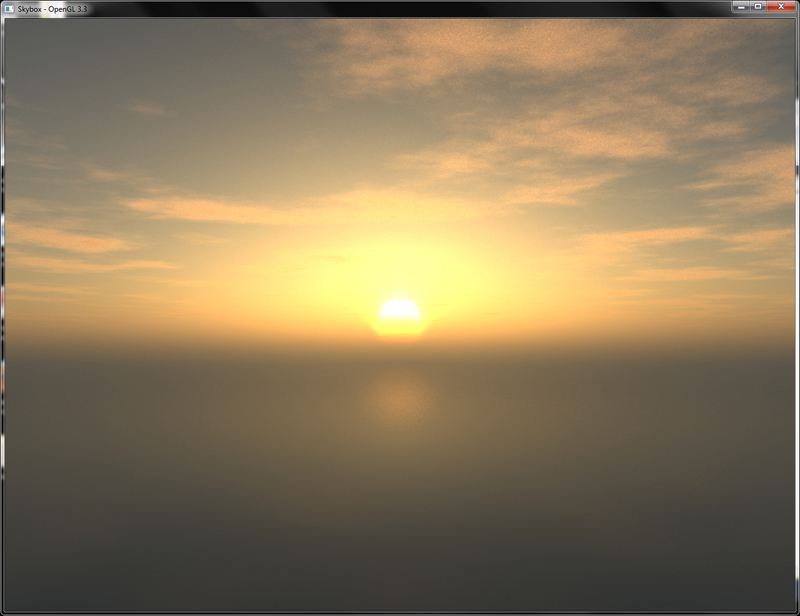
We will now use the FBO to render a mirror object on the screen. In a typical offscreen rendering OpenGL application, we set up the FBO first, by calling the glGenFramebuffers
function and passing it the number of FBOs desired. The second parameter stores the returned identifier. After the FBO object is generated, it has to be bound to the GL_FRAMEBUFFER
, GL_DRAW_FRAMEBUFFER,
or GL_READ_FRAMEBUFFER
target. Following this call, the texture to be bound to the FBOs color attachment is attached by calling the glFramebufferTexture2D
function.
If depth testing is required, a render buffer is also generated and bound by calling glGenRenderbuffers
followed by the glBindRenderbuffer
function. For render buffers, the depth buffer's data type and its dimensions have to be specified. After all these steps, the render buffer is attached to the frame buffer by calling the glFramebufferRenderbuffer
function.
After the setup of the frame buffer and render buffer objects, the frame buffer completeness status has to be checked by calling glCheckFramebufferStatus
by passing it the framebuffer
target. This ensures that the FBO setup is correct. The function returns the status as an identifier. If this returned value is anything other than GL_FRAMEBUFFER_COMPLETE
, the FBO setup is unsuccessful.
Let us get started with the recipe as follows:
- Initialize the
framebuffer
andrenderbuffer
objects' color and depth attachments respectively. The render buffer is required if we need depth testing for the offscreen rendering, and the depth precision is specified using theglRenderbufferStorage
function.glGenFramebuffers(1, &fboID); glBindFramebuffer(GL_DRAW_FRAMEBUFFER, fboID); glGenRenderbuffers(1, &rbID); glBindRenderbuffer(GL_RENDERBUFFER, rbID); glRenderbufferStorage(GL_RENDERBUFFER, GL_DEPTH_COMPONENT32,WIDTH, HEIGHT);
- Generate the offscreen texture on which FBO will render to. The last parameter of
glTexImage2D
isNULL
, which tells OpenGL that we do not have any content yet, please provide a new block of GPU memory which gets filled when the FBO is used as a render target.glGenTextures(1, &renderTextureID); glBindTexture(GL_TEXTURE_2D, renderTextureID); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_S, GL_REPEAT); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_T, L_REPEAT); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MIN_FILTER, GL_NEAREST); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MAG_FILTER, GL_NEAREST); glTexImage2D(GL_TEXTURE_2D, 0, GL_RGBA8, WIDTH, HEIGHT, 0, GL_BGRA, GL_UNSIGNED_BYTE, NULL);
- Attach
Renderbuffer
to the boundFramebuffer
object and check forFramebuffer
completeness.glFramebufferTexture2D(GL_DRAW_FRAMEBUFFER, GL_COLOR_ATTACHMENT0,GL_TEXTURE_2D, renderTextureID, 0); glFramebufferRenderbuffer(GL_DRAW_FRAMEBUFFER, GL_DEPTH_ATTACHMENT,GL_RENDERBUFFER, rbID); GLuint status = glCheckFramebufferStatus(GL_DRAW_FRAMEBUFFER); if(status==GL_FRAMEBUFFER_COMPLETE) { printf("FBO setup succeeded."); } else { printf("Error in FBO setup."); }
- Unbind the
Framebuffer
object as follows:glBindTexture(GL_TEXTURE_2D, 0); glBindFramebuffer(GL_DRAW_FRAMEBUFFER, 0);
- Create a quad geometry to act as a mirror:
mirror = new CQuad(-2);
- Render the scene normally from the point of view of camera. Since the unit color cube is rendered at origin, we translate it on the Y axis to shift it up in Y axis which effectively moves the unit color cube in Y direction so that the unit color cube's image can be viewed completely in the mirror.
glClear(GL_COLOR_BUFFER_BIT|GL_DEPTH_BUFFER_BIT); grid->Render(glm::value_ptr(MVP)); localR[3][1] = 0.5; cube->Render(glm::value_ptr(P*MV*localR));
- Store the current modelview matrix and then change the modelview matrix such that the camera is placed at the mirror object position. Also make sure to laterally invert this modelview matrix by scaling by -1 on the X axis.
glm::mat4 oldMV = MV; glm::vec3 target; glm::vec3 V = glm::vec3(-MV[2][0], -MV[2][1], -MV[2][2]); glm::vec3 R = glm::reflect(V, mirror->normal); MV = glm::lookAt(mirror->position, mirror->position + R, glm::vec3(0,1,0)); MV = glm::scale(MV, glm::vec3(-1,1,1));
- Bind the FBO, set up the FBO color attachment for
Drawbuffer
(GL_COLOR_ATTACHMENT0
) or any other attachment to which texture is attached, and clear the FBO. TheglDrawBuffer
function enables the code to draw to a specific color attachment on the FBO. In our case, there is a single color attachment so we set it as the draw buffer.glBindFramebuffer(GL_DRAW_FRAMEBUFFER, fboID); glDrawBuffer(GL_COLOR_ATTACHMENT0); glClear(GL_COLOR_BUFFER_BIT|GL_DEPTH_BUFFER_BIT);
- Set the modified modelview matrix and render the scene again. Also make sure to only render from the shiny side of the mirror.
if(glm::dot(V,mirror->normal)<0) { grid->Render(glm::value_ptr(P*MV)); cube->Render(glm::value_ptr(P*MV*localR)); }
- Unbind the FBO and restore the default
Drawbuffer
(GL_BACK_LEFT).
glBindFramebuffer(GL_DRAW_FRAMEBUFFER, 0); glDrawBuffer(GL_BACK_LEFT);
- Finally render the mirror quad at the saved modelview matrix.
MV = oldMV; glBindTexture(GL_TEXTURE_2D, renderTextureID); mirror->Render(glm::value_ptr(P*MV));
The mirror algorithm used in the recipe is very simple. We first get the view direction vector (V
) from the viewing matrix. We reflect this vector on the normal of the mirror (N
). Next, the camera position is moved to the place behind the mirror. Finally, the mirror is scaled by -1 on the X axis. This ensures that the image is laterally inverted as in a mirror. Details of the method are covered in the reference in the See also section.
- The Official OpenGL registry-Framebuffer object specifications can be found at http://www.opengl.org/registry/specs/EXT/framebuffer_object.txt.
- OpenGL Superbible, Fifth Edition, Chapter 8, pages 354-358, Richard S. Wright, Addison-Wesley Professional
- FBO tutorial by Song Ho Ahn: http://www.songho.ca/opengl/gl_fbo.html
Chapter3/MirrorUsingFBO
directory.
Let us get started with the recipe as follows:
- Initialize the
framebuffer
andrenderbuffer
objects' color and depth attachments respectively. The render buffer is required if we need depth testing for the offscreen rendering, and the depth precision is specified using theglRenderbufferStorage
function.glGenFramebuffers(1, &fboID); glBindFramebuffer(GL_DRAW_FRAMEBUFFER, fboID); glGenRenderbuffers(1, &rbID); glBindRenderbuffer(GL_RENDERBUFFER, rbID); glRenderbufferStorage(GL_RENDERBUFFER, GL_DEPTH_COMPONENT32,WIDTH, HEIGHT);
- Generate the offscreen texture on which FBO will render to. The last parameter of
glTexImage2D
isNULL
, which tells OpenGL that we do not have any content yet, please provide a new block of GPU memory which gets filled when the FBO is used as a render target.glGenTextures(1, &renderTextureID); glBindTexture(GL_TEXTURE_2D, renderTextureID); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_S, GL_REPEAT); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_T, L_REPEAT); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MIN_FILTER, GL_NEAREST); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MAG_FILTER, GL_NEAREST); glTexImage2D(GL_TEXTURE_2D, 0, GL_RGBA8, WIDTH, HEIGHT, 0, GL_BGRA, GL_UNSIGNED_BYTE, NULL);
- Attach
Renderbuffer
to the boundFramebuffer
object and check forFramebuffer
completeness.glFramebufferTexture2D(GL_DRAW_FRAMEBUFFER, GL_COLOR_ATTACHMENT0,GL_TEXTURE_2D, renderTextureID, 0); glFramebufferRenderbuffer(GL_DRAW_FRAMEBUFFER, GL_DEPTH_ATTACHMENT,GL_RENDERBUFFER, rbID); GLuint status = glCheckFramebufferStatus(GL_DRAW_FRAMEBUFFER); if(status==GL_FRAMEBUFFER_COMPLETE) { printf("FBO setup succeeded."); } else { printf("Error in FBO setup."); }
- Unbind the
Framebuffer
object as follows:glBindTexture(GL_TEXTURE_2D, 0); glBindFramebuffer(GL_DRAW_FRAMEBUFFER, 0);
- Create a quad geometry to act as a mirror:
mirror = new CQuad(-2);
- Render the scene normally from the point of view of camera. Since the unit color cube is rendered at origin, we translate it on the Y axis to shift it up in Y axis which effectively moves the unit color cube in Y direction so that the unit color cube's image can be viewed completely in the mirror.
glClear(GL_COLOR_BUFFER_BIT|GL_DEPTH_BUFFER_BIT); grid->Render(glm::value_ptr(MVP)); localR[3][1] = 0.5; cube->Render(glm::value_ptr(P*MV*localR));
- Store the current modelview matrix and then change the modelview matrix such that the camera is placed at the mirror object position. Also make sure to laterally invert this modelview matrix by scaling by -1 on the X axis.
glm::mat4 oldMV = MV; glm::vec3 target; glm::vec3 V = glm::vec3(-MV[2][0], -MV[2][1], -MV[2][2]); glm::vec3 R = glm::reflect(V, mirror->normal); MV = glm::lookAt(mirror->position, mirror->position + R, glm::vec3(0,1,0)); MV = glm::scale(MV, glm::vec3(-1,1,1));
- Bind the FBO, set up the FBO color attachment for
Drawbuffer
(GL_COLOR_ATTACHMENT0
) or any other attachment to which texture is attached, and clear the FBO. TheglDrawBuffer
function enables the code to draw to a specific color attachment on the FBO. In our case, there is a single color attachment so we set it as the draw buffer.glBindFramebuffer(GL_DRAW_FRAMEBUFFER, fboID); glDrawBuffer(GL_COLOR_ATTACHMENT0); glClear(GL_COLOR_BUFFER_BIT|GL_DEPTH_BUFFER_BIT);
- Set the modified modelview matrix and render the scene again. Also make sure to only render from the shiny side of the mirror.
if(glm::dot(V,mirror->normal)<0) { grid->Render(glm::value_ptr(P*MV)); cube->Render(glm::value_ptr(P*MV*localR)); }
- Unbind the FBO and restore the default
Drawbuffer
(GL_BACK_LEFT).
glBindFramebuffer(GL_DRAW_FRAMEBUFFER, 0); glDrawBuffer(GL_BACK_LEFT);
- Finally render the mirror quad at the saved modelview matrix.
MV = oldMV; glBindTexture(GL_TEXTURE_2D, renderTextureID); mirror->Render(glm::value_ptr(P*MV));
The mirror algorithm used in the recipe is very simple. We first get the view direction vector (V
) from the viewing matrix. We reflect this vector on the normal of the mirror (N
). Next, the camera position is moved to the place behind the mirror. Finally, the mirror is scaled by -1 on the X axis. This ensures that the image is laterally inverted as in a mirror. Details of the method are covered in the reference in the See also section.
- The Official OpenGL registry-Framebuffer object specifications can be found at http://www.opengl.org/registry/specs/EXT/framebuffer_object.txt.
- OpenGL Superbible, Fifth Edition, Chapter 8, pages 354-358, Richard S. Wright, Addison-Wesley Professional
- FBO tutorial by Song Ho Ahn: http://www.songho.ca/opengl/gl_fbo.html
framebuffer
and renderbuffer
objects' color and depth attachments respectively. The render buffer is required if we need depth testing for the offscreen rendering, and the depth precision is specified using the glRenderbufferStorage
function.glGenFramebuffers(1, &fboID); glBindFramebuffer(GL_DRAW_FRAMEBUFFER, fboID); glGenRenderbuffers(1, &rbID); glBindRenderbuffer(GL_RENDERBUFFER, rbID); glRenderbufferStorage(GL_RENDERBUFFER, GL_DEPTH_COMPONENT32,WIDTH, HEIGHT);
glTexImage2D
is NULL
, which tells OpenGL that we do not have any content yet, please provide a new block of GPU memory which gets filled when the FBO is used as a render target.glGenTextures(1, &renderTextureID); glBindTexture(GL_TEXTURE_2D, renderTextureID); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_S, GL_REPEAT); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_T, L_REPEAT); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MIN_FILTER, GL_NEAREST); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MAG_FILTER, GL_NEAREST); glTexImage2D(GL_TEXTURE_2D, 0, GL_RGBA8, WIDTH, HEIGHT, 0, GL_BGRA, GL_UNSIGNED_BYTE, NULL);
Renderbuffer
to the bound Framebuffer
object and check for Framebuffer
completeness.glFramebufferTexture2D(GL_DRAW_FRAMEBUFFER, GL_COLOR_ATTACHMENT0,GL_TEXTURE_2D, renderTextureID, 0); glFramebufferRenderbuffer(GL_DRAW_FRAMEBUFFER, GL_DEPTH_ATTACHMENT,GL_RENDERBUFFER, rbID); GLuint status = glCheckFramebufferStatus(GL_DRAW_FRAMEBUFFER); if(status==GL_FRAMEBUFFER_COMPLETE) { printf("FBO setup succeeded."); } else { printf("Error in FBO setup."); }
- the
Framebuffer
object as follows:glBindTexture(GL_TEXTURE_2D, 0); glBindFramebuffer(GL_DRAW_FRAMEBUFFER, 0);
- Create a quad geometry to act as a mirror:
mirror = new CQuad(-2);
- Render the scene normally from the point of view of camera. Since the unit color cube is rendered at origin, we translate it on the Y axis to shift it up in Y axis which effectively moves the unit color cube in Y direction so that the unit color cube's image can be viewed completely in the mirror.
glClear(GL_COLOR_BUFFER_BIT|GL_DEPTH_BUFFER_BIT); grid->Render(glm::value_ptr(MVP)); localR[3][1] = 0.5; cube->Render(glm::value_ptr(P*MV*localR));
- Store the current modelview matrix and then change the modelview matrix such that the camera is placed at the mirror object position. Also make sure to laterally invert this modelview matrix by scaling by -1 on the X axis.
glm::mat4 oldMV = MV; glm::vec3 target; glm::vec3 V = glm::vec3(-MV[2][0], -MV[2][1], -MV[2][2]); glm::vec3 R = glm::reflect(V, mirror->normal); MV = glm::lookAt(mirror->position, mirror->position + R, glm::vec3(0,1,0)); MV = glm::scale(MV, glm::vec3(-1,1,1));
- Bind the FBO, set up the FBO color attachment for
Drawbuffer
(GL_COLOR_ATTACHMENT0
) or any other attachment to which texture is attached, and clear the FBO. TheglDrawBuffer
function enables the code to draw to a specific color attachment on the FBO. In our case, there is a single color attachment so we set it as the draw buffer.glBindFramebuffer(GL_DRAW_FRAMEBUFFER, fboID); glDrawBuffer(GL_COLOR_ATTACHMENT0); glClear(GL_COLOR_BUFFER_BIT|GL_DEPTH_BUFFER_BIT);
- Set the modified modelview matrix and render the scene again. Also make sure to only render from the shiny side of the mirror.
if(glm::dot(V,mirror->normal)<0) { grid->Render(glm::value_ptr(P*MV)); cube->Render(glm::value_ptr(P*MV*localR)); }
- Unbind the FBO and restore the default
Drawbuffer
(GL_BACK_LEFT).
glBindFramebuffer(GL_DRAW_FRAMEBUFFER, 0); glDrawBuffer(GL_BACK_LEFT);
- Finally render the mirror quad at the saved modelview matrix.
MV = oldMV; glBindTexture(GL_TEXTURE_2D, renderTextureID); mirror->Render(glm::value_ptr(P*MV));
The mirror algorithm used in the recipe is very simple. We first get the view direction vector (V
) from the viewing matrix. We reflect this vector on the normal of the mirror (N
). Next, the camera position is moved to the place behind the mirror. Finally, the mirror is scaled by -1 on the X axis. This ensures that the image is laterally inverted as in a mirror. Details of the method are covered in the reference in the See also section.
- The Official OpenGL registry-Framebuffer object specifications can be found at http://www.opengl.org/registry/specs/EXT/framebuffer_object.txt.
- OpenGL Superbible, Fifth Edition, Chapter 8, pages 354-358, Richard S. Wright, Addison-Wesley Professional
- FBO tutorial by Song Ho Ahn: http://www.songho.ca/opengl/gl_fbo.html
algorithm used in the recipe is very simple. We first get the view direction vector (V
) from the viewing matrix. We reflect this vector on the normal of the mirror (N
). Next, the camera position is moved to the place behind the mirror. Finally, the mirror is scaled by -1 on the X axis. This ensures that the image is laterally inverted as in a mirror. Details of the method are covered in the reference in the See also section.
- The Official OpenGL registry-Framebuffer object specifications can be found at http://www.opengl.org/registry/specs/EXT/framebuffer_object.txt.
- OpenGL Superbible, Fifth Edition, Chapter 8, pages 354-358, Richard S. Wright, Addison-Wesley Professional
- FBO tutorial by Song Ho Ahn: http://www.songho.ca/opengl/gl_fbo.html
Framebuffer
object can be obtained from the Framebuffer
object specifications (see the See also section). The output from the demo application implementing this recipe is as follows:
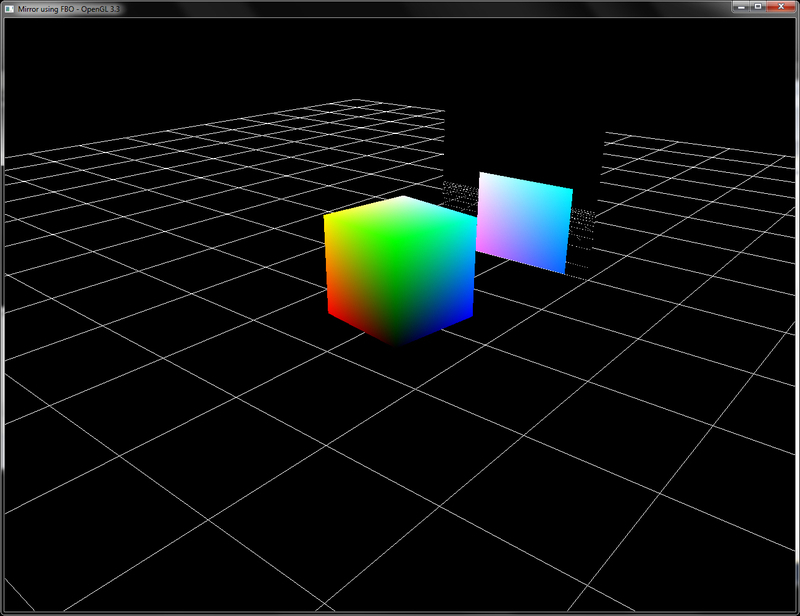
- The Official OpenGL registry-Framebuffer object specifications can be found at http://www.opengl.org/registry/specs/EXT/framebuffer_object.txt.
- OpenGL Superbible, Fifth Edition, Chapter 8, pages 354-358, Richard S. Wright, Addison-Wesley Professional
- FBO tutorial by Song Ho Ahn: http://www.songho.ca/opengl/gl_fbo.html
- http://www.opengl.org/registry/specs/EXT/framebuffer_object.txt.
- OpenGL Superbible, Fifth Edition, Chapter 8, pages 354-358, Richard S. Wright, Addison-Wesley Professional
- FBO tutorial by Song Ho Ahn: http://www.songho.ca/opengl/gl_fbo.html
Now we will see how to use dynamic cube mapping to render a real-time scene to a cubemap render target. This allows us to create reflective surfaces. In modern OpenGL, offscreen rendering (also called render-to-texture) functionality is exposed through FBOs.
Let us get started with the recipe as follows:
- Create a cubemap texture object.
glGenTextures(1, &dynamicCubeMapID); glActiveTexture(GL_TEXTURE1); glBindTexture(GL_TEXTURE_CUBE_MAP, dynamicCubeMapID); glTexParameterf(GL_TEXTURE_CUBE_MAP,GL_TEXTURE_MIN_FILTER, GL_LINEAR); glTexParameterf(GL_TEXTURE_CUBE_MAP, GL_TEXTURE_MAG_FILTER, GL_LINEAR); glTexParameterf(GL_TEXTURE_CUBE_MAP, GL_TEXTURE_WRAP_S, GL_CLAMP_TO_EDGE); glTexParameterf(GL_TEXTURE_CUBE_MAP, GL_TEXTURE_WRAP_T, GL_CLAMP_TO_EDGE); glTexParameterf(GL_TEXTURE_CUBE_MAP, GL_TEXTURE_WRAP_R, GL_CLAMP_TO_EDGE); for (int face = 0; face < 6; face++) { glTexImage2D(GL_TEXTURE_CUBE_MAP_POSITIVE_X + face, 0, GL_RGBA,CUBEMAP_SIZE, CUBEMAP_SIZE, 0, GL_RGBA, GL_FLOAT, NULL); }
- Set up an FBO with the cubemap texture as an attachment.
glGenFramebuffers(1, &fboID); glBindFramebuffer(GL_DRAW_FRAMEBUFFER, fboID); glGenRenderbuffers(1, &rboID); glBindRenderbuffer(GL_RENDERBUFFER, rboID); glRenderbufferStorage(GL_RENDERBUFFER, GL_DEPTH_COMPONENT, CUBEMAP_SIZE, CUBEMAP_SIZE); glFramebufferRenderbuffer(GL_DRAW_FRAMEBUFFER, GL_DEPTH_ATTACHMENT, GL_RENDERBUFFER, fboID); glFramebufferTexture2D(GL_DRAW_FRAMEBUFFER, GL_COLOR_ATTACHMENT0, GL_TEXTURE_CUBE_MAP_POSITIVE_X, dynamicCubeMapID, 0); GLenum status = glCheckFramebufferStatus(GL_DRAW_FRAMEBUFFER); if(status != GL_FRAMEBUFFER_COMPLETE) { cerr<<"Frame buffer object setup error."<<endl; exit(EXIT_FAILURE); } else { cerr<<"FBO setup successfully."<<endl; }
- Set the viewport to the size of the offscreen texture and render the scene six times without the reflective object to the six sides of the cubemap using FBO.
glViewport(0,0,CUBEMAP_SIZE,CUBEMAP_SIZE); glBindFramebuffer(GL_DRAW_FRAMEBUFFER, fboID); glFramebufferTexture2D(GL_DRAW_FRAMEBUFFER, GL_COLOR_ATTACHMENT0,GL_TEXTURE_CUBE_MAP_POSITIVE_X, dynamicCubeMapID, 0); glClear(GL_COLOR_BUFFER_BIT|GL_DEPTH_BUFFER_BIT); glm::mat4 MV1 = glm::lookAt(glm::vec3(0),glm::vec3(1,0,0),glm::vec3(0,-1,0)); DrawScene( MV1*T, Pcubemap); glFramebufferTexture2D(GL_DRAW_FRAMEBUFFER, GL_COLOR_ATTACHMENT0, GL_TEXTURE_CUBE_MAP_NEGATIVE_X, dynamicCubeMapID, 0); glClear(GL_COLOR_BUFFER_BIT|GL_DEPTH_BUFFER_BIT); glm::mat4 MV2 = glm::lookAt(glm::vec3(0),glm::vec3(-1,0,0), glm::vec3(0,-1,0)); DrawScene( MV2*T, Pcubemap); ...//similar for rest of the faces glBindFramebuffer(GL_DRAW_FRAMEBUFFER, 0);
- Restore the viewport and the modelview matrix, and render the scene normally.
glViewport(0,0,WIDTH,HEIGHT); DrawScene(MV, P);
- Set the cubemap shader and then render the reflective object.
glBindVertexArray(sphereVAOID); cubemapShader.Use(); T = glm::translate(glm::mat4(1), p); glUniformMatrix4fv(cubemapShader("MVP"), 1, GL_FALSE, glm::value_ptr(P*(MV*T))); glUniform3fv(cubemapShader("eyePosition"), 1, glm::value_ptr(eyePos)); glDrawElements(GL_TRIANGLES,indices.size(),GL_UNSIGNED_SHORT,0); cubemapShader.UnUse();
Dynamic cube mapping renders the scene six times from the reflective object using six cameras at the reflective object's position. For rendering to the cubemap texture, an FBO is used with a cubemap texture attachment. The cubemap texture's GL_TEXTURE_CUBE_MAP_POSITIVE_X
target is bound to the GL_COLOR_ATTACHMENT0
color attachment of the FBO. The last parameter of glTexImage2D
is NULL
since this call just allocates the memory for offscreen rendering and the real data will be populated when the FBO is set as the render target.
The cubemap vertex shader outputs the object space vertex positions and normals.
The cubemap fragment shader uses the object space vertex positions to determine the view vector. The reflection vector is then obtained by reflecting the view vector at the object space normal.
Chapter3/DynamicCubemap
directory.
Let us get started with the recipe as follows:
- Create a cubemap texture object.
glGenTextures(1, &dynamicCubeMapID); glActiveTexture(GL_TEXTURE1); glBindTexture(GL_TEXTURE_CUBE_MAP, dynamicCubeMapID); glTexParameterf(GL_TEXTURE_CUBE_MAP,GL_TEXTURE_MIN_FILTER, GL_LINEAR); glTexParameterf(GL_TEXTURE_CUBE_MAP, GL_TEXTURE_MAG_FILTER, GL_LINEAR); glTexParameterf(GL_TEXTURE_CUBE_MAP, GL_TEXTURE_WRAP_S, GL_CLAMP_TO_EDGE); glTexParameterf(GL_TEXTURE_CUBE_MAP, GL_TEXTURE_WRAP_T, GL_CLAMP_TO_EDGE); glTexParameterf(GL_TEXTURE_CUBE_MAP, GL_TEXTURE_WRAP_R, GL_CLAMP_TO_EDGE); for (int face = 0; face < 6; face++) { glTexImage2D(GL_TEXTURE_CUBE_MAP_POSITIVE_X + face, 0, GL_RGBA,CUBEMAP_SIZE, CUBEMAP_SIZE, 0, GL_RGBA, GL_FLOAT, NULL); }
- Set up an FBO with the cubemap texture as an attachment.
glGenFramebuffers(1, &fboID); glBindFramebuffer(GL_DRAW_FRAMEBUFFER, fboID); glGenRenderbuffers(1, &rboID); glBindRenderbuffer(GL_RENDERBUFFER, rboID); glRenderbufferStorage(GL_RENDERBUFFER, GL_DEPTH_COMPONENT, CUBEMAP_SIZE, CUBEMAP_SIZE); glFramebufferRenderbuffer(GL_DRAW_FRAMEBUFFER, GL_DEPTH_ATTACHMENT, GL_RENDERBUFFER, fboID); glFramebufferTexture2D(GL_DRAW_FRAMEBUFFER, GL_COLOR_ATTACHMENT0, GL_TEXTURE_CUBE_MAP_POSITIVE_X, dynamicCubeMapID, 0); GLenum status = glCheckFramebufferStatus(GL_DRAW_FRAMEBUFFER); if(status != GL_FRAMEBUFFER_COMPLETE) { cerr<<"Frame buffer object setup error."<<endl; exit(EXIT_FAILURE); } else { cerr<<"FBO setup successfully."<<endl; }
- Set the viewport to the size of the offscreen texture and render the scene six times without the reflective object to the six sides of the cubemap using FBO.
glViewport(0,0,CUBEMAP_SIZE,CUBEMAP_SIZE); glBindFramebuffer(GL_DRAW_FRAMEBUFFER, fboID); glFramebufferTexture2D(GL_DRAW_FRAMEBUFFER, GL_COLOR_ATTACHMENT0,GL_TEXTURE_CUBE_MAP_POSITIVE_X, dynamicCubeMapID, 0); glClear(GL_COLOR_BUFFER_BIT|GL_DEPTH_BUFFER_BIT); glm::mat4 MV1 = glm::lookAt(glm::vec3(0),glm::vec3(1,0,0),glm::vec3(0,-1,0)); DrawScene( MV1*T, Pcubemap); glFramebufferTexture2D(GL_DRAW_FRAMEBUFFER, GL_COLOR_ATTACHMENT0, GL_TEXTURE_CUBE_MAP_NEGATIVE_X, dynamicCubeMapID, 0); glClear(GL_COLOR_BUFFER_BIT|GL_DEPTH_BUFFER_BIT); glm::mat4 MV2 = glm::lookAt(glm::vec3(0),glm::vec3(-1,0,0), glm::vec3(0,-1,0)); DrawScene( MV2*T, Pcubemap); ...//similar for rest of the faces glBindFramebuffer(GL_DRAW_FRAMEBUFFER, 0);
- Restore the viewport and the modelview matrix, and render the scene normally.
glViewport(0,0,WIDTH,HEIGHT); DrawScene(MV, P);
- Set the cubemap shader and then render the reflective object.
glBindVertexArray(sphereVAOID); cubemapShader.Use(); T = glm::translate(glm::mat4(1), p); glUniformMatrix4fv(cubemapShader("MVP"), 1, GL_FALSE, glm::value_ptr(P*(MV*T))); glUniform3fv(cubemapShader("eyePosition"), 1, glm::value_ptr(eyePos)); glDrawElements(GL_TRIANGLES,indices.size(),GL_UNSIGNED_SHORT,0); cubemapShader.UnUse();
Dynamic cube mapping renders the scene six times from the reflective object using six cameras at the reflective object's position. For rendering to the cubemap texture, an FBO is used with a cubemap texture attachment. The cubemap texture's GL_TEXTURE_CUBE_MAP_POSITIVE_X
target is bound to the GL_COLOR_ATTACHMENT0
color attachment of the FBO. The last parameter of glTexImage2D
is NULL
since this call just allocates the memory for offscreen rendering and the real data will be populated when the FBO is set as the render target.
The cubemap vertex shader outputs the object space vertex positions and normals.
The cubemap fragment shader uses the object space vertex positions to determine the view vector. The reflection vector is then obtained by reflecting the view vector at the object space normal.
glGenTextures(1, &dynamicCubeMapID); glActiveTexture(GL_TEXTURE1); glBindTexture(GL_TEXTURE_CUBE_MAP, dynamicCubeMapID); glTexParameterf(GL_TEXTURE_CUBE_MAP,GL_TEXTURE_MIN_FILTER, GL_LINEAR); glTexParameterf(GL_TEXTURE_CUBE_MAP, GL_TEXTURE_MAG_FILTER, GL_LINEAR); glTexParameterf(GL_TEXTURE_CUBE_MAP, GL_TEXTURE_WRAP_S, GL_CLAMP_TO_EDGE); glTexParameterf(GL_TEXTURE_CUBE_MAP, GL_TEXTURE_WRAP_T, GL_CLAMP_TO_EDGE); glTexParameterf(GL_TEXTURE_CUBE_MAP, GL_TEXTURE_WRAP_R, GL_CLAMP_TO_EDGE); for (int face = 0; face < 6; face++) { glTexImage2D(GL_TEXTURE_CUBE_MAP_POSITIVE_X + face, 0, GL_RGBA,CUBEMAP_SIZE, CUBEMAP_SIZE, 0, GL_RGBA, GL_FLOAT, NULL); }
glGenFramebuffers(1, &fboID); glBindFramebuffer(GL_DRAW_FRAMEBUFFER, fboID); glGenRenderbuffers(1, &rboID); glBindRenderbuffer(GL_RENDERBUFFER, rboID); glRenderbufferStorage(GL_RENDERBUFFER, GL_DEPTH_COMPONENT, CUBEMAP_SIZE, CUBEMAP_SIZE); glFramebufferRenderbuffer(GL_DRAW_FRAMEBUFFER, GL_DEPTH_ATTACHMENT, GL_RENDERBUFFER, fboID); glFramebufferTexture2D(GL_DRAW_FRAMEBUFFER, GL_COLOR_ATTACHMENT0, GL_TEXTURE_CUBE_MAP_POSITIVE_X, dynamicCubeMapID, 0); GLenum status = glCheckFramebufferStatus(GL_DRAW_FRAMEBUFFER); if(status != GL_FRAMEBUFFER_COMPLETE) { cerr<<"Frame buffer object setup error."<<endl; exit(EXIT_FAILURE); } else { cerr<<"FBO setup successfully."<<endl; }
- viewport to the size of the offscreen texture and render the scene six times without the reflective object to the six sides of the cubemap using FBO.
glViewport(0,0,CUBEMAP_SIZE,CUBEMAP_SIZE); glBindFramebuffer(GL_DRAW_FRAMEBUFFER, fboID); glFramebufferTexture2D(GL_DRAW_FRAMEBUFFER, GL_COLOR_ATTACHMENT0,GL_TEXTURE_CUBE_MAP_POSITIVE_X, dynamicCubeMapID, 0); glClear(GL_COLOR_BUFFER_BIT|GL_DEPTH_BUFFER_BIT); glm::mat4 MV1 = glm::lookAt(glm::vec3(0),glm::vec3(1,0,0),glm::vec3(0,-1,0)); DrawScene( MV1*T, Pcubemap); glFramebufferTexture2D(GL_DRAW_FRAMEBUFFER, GL_COLOR_ATTACHMENT0, GL_TEXTURE_CUBE_MAP_NEGATIVE_X, dynamicCubeMapID, 0); glClear(GL_COLOR_BUFFER_BIT|GL_DEPTH_BUFFER_BIT); glm::mat4 MV2 = glm::lookAt(glm::vec3(0),glm::vec3(-1,0,0), glm::vec3(0,-1,0)); DrawScene( MV2*T, Pcubemap); ...//similar for rest of the faces glBindFramebuffer(GL_DRAW_FRAMEBUFFER, 0);
- Restore the viewport and the modelview matrix, and render the scene normally.
glViewport(0,0,WIDTH,HEIGHT); DrawScene(MV, P);
- Set the cubemap shader and then render the reflective object.
glBindVertexArray(sphereVAOID); cubemapShader.Use(); T = glm::translate(glm::mat4(1), p); glUniformMatrix4fv(cubemapShader("MVP"), 1, GL_FALSE, glm::value_ptr(P*(MV*T))); glUniform3fv(cubemapShader("eyePosition"), 1, glm::value_ptr(eyePos)); glDrawElements(GL_TRIANGLES,indices.size(),GL_UNSIGNED_SHORT,0); cubemapShader.UnUse();
Dynamic cube mapping renders the scene six times from the reflective object using six cameras at the reflective object's position. For rendering to the cubemap texture, an FBO is used with a cubemap texture attachment. The cubemap texture's GL_TEXTURE_CUBE_MAP_POSITIVE_X
target is bound to the GL_COLOR_ATTACHMENT0
color attachment of the FBO. The last parameter of glTexImage2D
is NULL
since this call just allocates the memory for offscreen rendering and the real data will be populated when the FBO is set as the render target.
The cubemap vertex shader outputs the object space vertex positions and normals.
The cubemap fragment shader uses the object space vertex positions to determine the view vector. The reflection vector is then obtained by reflecting the view vector at the object space normal.
mapping renders the scene six times from the reflective object using six cameras at the reflective object's position. For rendering to the cubemap texture, an FBO is used with a cubemap texture attachment. The cubemap texture's GL_TEXTURE_CUBE_MAP_POSITIVE_X
target is bound to the GL_COLOR_ATTACHMENT0
color attachment of the FBO. The last parameter of glTexImage2D
is NULL
since this call just allocates the memory for offscreen rendering and the real data will be populated when the FBO is set as the render target.
The cubemap vertex shader outputs the object space vertex positions and normals.
The cubemap fragment shader uses the object space vertex positions to determine the view vector. The reflection vector is then obtained by reflecting the view vector at the object space normal.
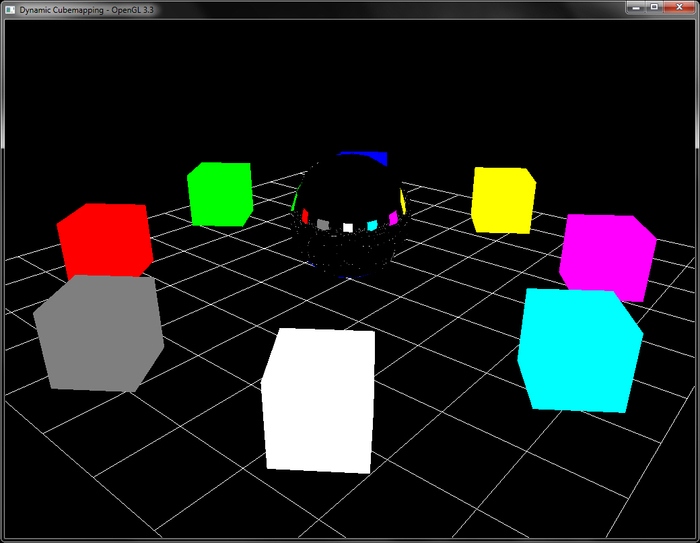
Framebuffer
object layer. This can be achieved by outputting to the appropriate gl_Layer
attribute from the geometry shader and setting the appropriate viewing transformation. This is left as an exercise for the reader.
- http://www.opengl.org/wiki/Geometry_Shader#Layered_rendering
- FBO tutorial by Song Ho Ahn: http://www.songho.ca/opengl/gl_fbo.html
We will now see how to do area filtering, that is, 2D image convolution to implement effects like sharpening, blurring, and embossing. There are several ways to achieve image convolution in the spatial domain. The simplest approach is to use a loop that iterates through a given image window and computes the sum of products of the image intensities with the convolution kernel. The more efficient method, as far as the implementation is concerned, is separable convolution which breaks up the 2D convolution into two 1D convolutions. However, this approach requires an additional pass.
Let us get started with the recipe as follows:
- Create a simple pass-through vertex shader that outputs the clip space position and the texture coordinates which are to be passed into the fragment shader for texture lookup.
#version 330 core in vec2 vVertex; out vec2 vUV; void main() { gl_Position = vec4(vVertex*2.0-1,0,1); vUV = vVertex; }
- In the fragment shader, we declare a constant array called
kernel
which stores our convolutionkernel
. Changing the convolutionkernel
values dictates the output of convolution. The defaultkernel
sets up a sharpening convolution filter. Refer toChapter3/Convolution/shaders/shader_convolution.frag
for details.const float kernel[]=float[9] (-1,-1,-1,-1, 8,-1,-1,-1,-1);
- In the fragment shader, we run a nested loop that loops through the current pixel's neighborhood and multiplies the
kernel
value with the current pixel's value. This is continued in an n x n neighborhood, where n is the width/height of thekernel
.for(int j=-1;j<=1;j++) { for(int i=-1;i<=1;i++) { color += kernel[index--] * texture(textureMap, vUV+(vec2(i,j)*delta)); } }
- After the nested loops, we divide the color value with the total number of values in the
kernel
. For a 3 x 3kernel
, we have nine values. Finally, we add the convolved color value to the current pixel's value.color/=9.0; vFragColor = color + texture(textureMap, vUV);
After the image is loaded and an OpenGL texture has been generated, we render a screen-aligned quad. This allows the fragment shader to run for the whole screen. In the fragment shader, for the current fragment, we iterate through its neighborhood and sum the product of the corresponding entry in the kernel with the look-up value. After the loop is terminated, the sum is divided by the total number of kernel coefficients. Finally, the convolution sum is added to the current pixel's value. There are several different kinds of kernels. We list the ones we will use in this recipe in the following table.
Effect |
Kernel matrix |
---|---|
![]() | |
Blurring / Unweighted Smoothing |
![]() |
![]() | |
![]() | |
![]() | |
![]() | |
![]() |
Chapter3/Convolution
directory. For this recipe, most of the work takes place in the fragment shader.
Let us get started with the recipe as follows:
- Create a simple pass-through vertex shader that outputs the clip space position and the texture coordinates which are to be passed into the fragment shader for texture lookup.
#version 330 core in vec2 vVertex; out vec2 vUV; void main() { gl_Position = vec4(vVertex*2.0-1,0,1); vUV = vVertex; }
- In the fragment shader, we declare a constant array called
kernel
which stores our convolutionkernel
. Changing the convolutionkernel
values dictates the output of convolution. The defaultkernel
sets up a sharpening convolution filter. Refer toChapter3/Convolution/shaders/shader_convolution.frag
for details.const float kernel[]=float[9] (-1,-1,-1,-1, 8,-1,-1,-1,-1);
- In the fragment shader, we run a nested loop that loops through the current pixel's neighborhood and multiplies the
kernel
value with the current pixel's value. This is continued in an n x n neighborhood, where n is the width/height of thekernel
.for(int j=-1;j<=1;j++) { for(int i=-1;i<=1;i++) { color += kernel[index--] * texture(textureMap, vUV+(vec2(i,j)*delta)); } }
- After the nested loops, we divide the color value with the total number of values in the
kernel
. For a 3 x 3kernel
, we have nine values. Finally, we add the convolved color value to the current pixel's value.color/=9.0; vFragColor = color + texture(textureMap, vUV);
After the image is loaded and an OpenGL texture has been generated, we render a screen-aligned quad. This allows the fragment shader to run for the whole screen. In the fragment shader, for the current fragment, we iterate through its neighborhood and sum the product of the corresponding entry in the kernel with the look-up value. After the loop is terminated, the sum is divided by the total number of kernel coefficients. Finally, the convolution sum is added to the current pixel's value. There are several different kinds of kernels. We list the ones we will use in this recipe in the following table.
Effect |
Kernel matrix |
---|---|
![]() | |
Blurring / Unweighted Smoothing |
![]() |
![]() | |
![]() | |
![]() | |
![]() | |
![]() |
#version 330 core in vec2 vVertex; out vec2 vUV; void main() { gl_Position = vec4(vVertex*2.0-1,0,1); vUV = vVertex; }
kernel
which stores our convolution kernel
. Changing the convolution kernel
values dictates the output of convolution. The default kernel
sets up a sharpening convolution filter. Refer to Chapter3/Convolution/shaders/shader_convolution.frag
for details.const float kernel[]=float[9] (-1,-1,-1,-1, 8,-1,-1,-1,-1);
kernel
value with the current pixel's value. This is continued in an n x n neighborhood, where n is the width/height of the kernel
.for(int j=-1;j<=1;j++) { for(int i=-1;i<=1;i++) { color += kernel[index--] * texture(textureMap, vUV+(vec2(i,j)*delta)); } }
After the image is loaded and an OpenGL texture has been generated, we render a screen-aligned quad. This allows the fragment shader to run for the whole screen. In the fragment shader, for the current fragment, we iterate through its neighborhood and sum the product of the corresponding entry in the kernel with the look-up value. After the loop is terminated, the sum is divided by the total number of kernel coefficients. Finally, the convolution sum is added to the current pixel's value. There are several different kinds of kernels. We list the ones we will use in this recipe in the following table.
Effect |
Kernel matrix |
---|---|
![]() | |
Blurring / Unweighted Smoothing |
![]() |
![]() | |
![]() | |
![]() | |
![]() | |
![]() |

is loaded and an OpenGL texture has been generated, we render a screen-aligned quad. This allows the fragment shader to run for the whole screen. In the fragment shader, for the current fragment, we iterate through its neighborhood and sum the product of the corresponding entry in the kernel with the look-up value. After the loop is terminated, the sum is divided by the total number of kernel coefficients. Finally, the convolution sum is added to the current pixel's value. There are several different kinds of kernels. We list the ones we will use in this recipe in the following table.
Effect |
Kernel matrix |
---|---|
![]() | |
Blurring / Unweighted Smoothing |
![]() |
![]() | |
![]() | |
![]() | |
![]() | |
![]() |
Now that we know how to perform offscreen rendering and blurring, we will put this knowledge to use by implementing the glow effect. The code for this recipe is in the Chapter3/Glow
directory. In this recipe, we will render a set of points encircling a cube. Every 50 frames, four alternate points glow.
Let us get started with the recipe as follows:
- Render the scene normally by rendering the points and the cube. The particle shader renders the
GL_POINTS
value (which by default, renders as quads) as circles.grid->Render(glm::value_ptr(MVP)); cube->Render(glm::value_ptr(MVP)); glBindVertexArray(particlesVAO); particleShader.Use(); glUniformMatrix4fv(particleShader("MVP"), 1, GL_FALSE, glm::value_ptr(MVP*Rot)); glDrawArrays(GL_POINTS, 0, 8);
The particle vertex shader is as follows:
#version 330 core layout(location=0) in vec3 vVertex; uniform mat4 MVP; smooth out vec4 color; const vec4 colors[8]=vec4[8](vec4(1,0,0,1), vec4(0,1,0,1), vec4(0,0,1,1),vec4(1,1,0,1), vec4(0,1,1,1), vec4(1,0,1,1), vec4(0.5,0.5,0.5,1), vec4(1,1,1,1)) ; void main() { gl_Position = MVP*vec4(vVertex,1); color = colors[gl_VertexID/4]; }
The particle fragment shader is as follows:
#version 330 core layout(location=0) out vec4 vFragColor; smooth in vec4 color; void main() { vec2 pos = gl_PointCoord-0.5; if(dot(pos,pos)>0.25) discard; else vFragColor = color; }
- Set up a single FBO with two color attachments. The first attachment is for rendering of scene elements requiring glow and the second attachment is for blurring.
glGenFramebuffers(1, &fboID); glBindFramebuffer(GL_DRAW_FRAMEBUFFER, fboID); glGenTextures(2, texID); glActiveTexture(GL_TEXTURE0); for(int i=0;i<2;i++) { glBindTexture(GL_TEXTURE_2D, texID[i]); glTexParameterf(GL_TEXTURE_2D, GL_TEXTURE_MIN_FILTER,GL_LINEAR); glTexParameterf(GL_TXTURE_2D, GL_TEXTURE_MAG_FILTER,GL_LINEAR) glTexParameterf(GL_TEXTURE_2D, GL_TEXTURE_WRAP_S, GL_CLAMP_TO_EDGE); glTexParameterf(GL_TEXTURE_2D, GL_TEXTURE_WRAP_T, GL_CLAMP_TO_EDGE); glTexImage2D(GL_TEXTURE_2D, 0, GL_RGBA, RENDER_TARGET_WIDTH, RENDER_TARGET_HEIGHT, 0, GL_RGBA,GL_UNSIGNED_BYTE, NULL); glFramebufferTexture2D(GL_DRAW_FRAMEBUFFER, GL_COLOR_ATTACHMENT0+i,GL_TEXTURE_2D,texID[i],0); } GLenum status = glCheckFramebufferStatus(GL_DRAW_FRAMEBUFFER); if(status != GL_FRAMEBUFFER_COMPLETE) { cerr<<"Frame buffer object setup error."<<endl; exit(EXIT_FAILURE); } else { cerr<<"FBO set up successfully."<<endl; } glBindFramebuffer(GL_DRAW_FRAMEBUFFER, 0);
- Bind FBO, set the viewport to the size of the attachment texture, set
Drawbuffer
to render to the first color attachment (GL_COLOR_ATTACHMENT0
), and render the part of the scene which needs glow.glBindFramebuffer(GL_DRAW_FRAMEBUFFER, fboID); glViewport(0,0,RENDER_TARGET_WIDTH,RENDER_TARGET_HEIGHT); glDrawBuffer(GL_COLOR_ATTACHMENT0); glClear(GL_COLOR_BUFFER_BIT); glDrawArrays(GL_POINTS, offset, 4); particleShader.UnUse();
- Set
Drawbuffer
to render to the second color attachment (GL_COLOR_ATTACHMENT1
) and bind the FBO texture attached to the first color attachment. Set the blur shader by convolving with a simple unweighted smoothing filter.glDrawBuffer(GL_COLOR_ATTACHMENT1); glBindTexture(GL_TEXTURE_2D, texID[0]);
- Render a screen-aligned quad and apply the blur shader to the rendering result from the first color attachment of the FBO. This output is written to the second color attachment.
blurShader.Use(); glBindVertexArray(quadVAOID); glDrawElements(GL_TRIANGLES,6,GL_UNSIGNED_SHORT,0);
- Disable FBO rendering, reset the default drawbuffer (
GL_BACK_LEFT
) and viewport, bind the texture attached to the FBO's second color attachment, draw a screen-aligned quad, and blend the blur output to the existing scene using additive blending.glBindFramebuffer(GL_DRAW_FRAMEBUFFER, 0); glDrawBuffer(GL_BACK_LEFT); glBindTexture(GL_TEXTURE_2D, texID[1]); glViewport(0,0,WIDTH, HEIGHT); glEnable(GL_BLEND); glBlendFunc(GL_ONE, GL_ONE); glDrawElements(GL_TRIANGLES,6,GL_UNSIGNED_SHORT,0); glBindVertexArray(0); blurShader.UnUse(); glDisable(GL_BLEND);
Note that we could also enable blending in the fragment shader. Assuming that the two images to be blended are bound to their texture units and their shader samplers are texture1
and texture2
, the additive blending shader code will be like this:
The second color attachment is then set as output while the output results from the vertical smoothing pass (which was written to the third color attachment) is set as input. The horizontal smoothing shader is then applied on a column of pixels which smoothes the entire image. The image is then rendered to the second color attachment. Finally, the blend shader combines the result from the first color attachment with the result from the second color attachment. Note that the same effect could be carried out by using two separate FBOs: a rendering FBO and a filtering FBO, which gives us more flexibility as we can down sample the filtering result to take advantage of hardware linear filtering. This technique has been used in the Implementing variance shadow mapping recipe in Chapter 4, Lights and Shadows.
GL_POINTS
value (which by default, renders as quads) as circles.grid->Render(glm::value_ptr(MVP)); cube->Render(glm::value_ptr(MVP)); glBindVertexArray(particlesVAO); particleShader.Use(); glUniformMatrix4fv(particleShader("MVP"), 1, GL_FALSE, glm::value_ptr(MVP*Rot)); glDrawArrays(GL_POINTS, 0, 8);
The particle vertex shader is as follows:
#version 330 core layout(location=0) in vec3 vVertex; uniform mat4 MVP; smooth out vec4 color; const vec4 colors[8]=vec4[8](vec4(1,0,0,1), vec4(0,1,0,1), vec4(0,0,1,1),vec4(1,1,0,1), vec4(0,1,1,1), vec4(1,0,1,1), vec4(0.5,0.5,0.5,1), vec4(1,1,1,1)) ; void main() { gl_Position = MVP*vec4(vVertex,1); color = colors[gl_VertexID/4]; }
The particle fragment shader is as follows:
#version 330 core layout(location=0) out vec4 vFragColor; smooth in vec4 color; void main() { vec2 pos = gl_PointCoord-0.5; if(dot(pos,pos)>0.25) discard; else vFragColor = color; }
- FBO with two color attachments. The first attachment is for rendering of scene elements requiring glow and the second attachment is for blurring.
glGenFramebuffers(1, &fboID); glBindFramebuffer(GL_DRAW_FRAMEBUFFER, fboID); glGenTextures(2, texID); glActiveTexture(GL_TEXTURE0); for(int i=0;i<2;i++) { glBindTexture(GL_TEXTURE_2D, texID[i]); glTexParameterf(GL_TEXTURE_2D, GL_TEXTURE_MIN_FILTER,GL_LINEAR); glTexParameterf(GL_TXTURE_2D, GL_TEXTURE_MAG_FILTER,GL_LINEAR) glTexParameterf(GL_TEXTURE_2D, GL_TEXTURE_WRAP_S, GL_CLAMP_TO_EDGE); glTexParameterf(GL_TEXTURE_2D, GL_TEXTURE_WRAP_T, GL_CLAMP_TO_EDGE); glTexImage2D(GL_TEXTURE_2D, 0, GL_RGBA, RENDER_TARGET_WIDTH, RENDER_TARGET_HEIGHT, 0, GL_RGBA,GL_UNSIGNED_BYTE, NULL); glFramebufferTexture2D(GL_DRAW_FRAMEBUFFER, GL_COLOR_ATTACHMENT0+i,GL_TEXTURE_2D,texID[i],0); } GLenum status = glCheckFramebufferStatus(GL_DRAW_FRAMEBUFFER); if(status != GL_FRAMEBUFFER_COMPLETE) { cerr<<"Frame buffer object setup error."<<endl; exit(EXIT_FAILURE); } else { cerr<<"FBO set up successfully."<<endl; } glBindFramebuffer(GL_DRAW_FRAMEBUFFER, 0);
- Bind FBO, set the viewport to the size of the attachment texture, set
Drawbuffer
to render to the first color attachment (GL_COLOR_ATTACHMENT0
), and render the part of the scene which needs glow.glBindFramebuffer(GL_DRAW_FRAMEBUFFER, fboID); glViewport(0,0,RENDER_TARGET_WIDTH,RENDER_TARGET_HEIGHT); glDrawBuffer(GL_COLOR_ATTACHMENT0); glClear(GL_COLOR_BUFFER_BIT); glDrawArrays(GL_POINTS, offset, 4); particleShader.UnUse();
- Set
Drawbuffer
to render to the second color attachment (GL_COLOR_ATTACHMENT1
) and bind the FBO texture attached to the first color attachment. Set the blur shader by convolving with a simple unweighted smoothing filter.glDrawBuffer(GL_COLOR_ATTACHMENT1); glBindTexture(GL_TEXTURE_2D, texID[0]);
- Render a screen-aligned quad and apply the blur shader to the rendering result from the first color attachment of the FBO. This output is written to the second color attachment.
blurShader.Use(); glBindVertexArray(quadVAOID); glDrawElements(GL_TRIANGLES,6,GL_UNSIGNED_SHORT,0);
- Disable FBO rendering, reset the default drawbuffer (
GL_BACK_LEFT
) and viewport, bind the texture attached to the FBO's second color attachment, draw a screen-aligned quad, and blend the blur output to the existing scene using additive blending.glBindFramebuffer(GL_DRAW_FRAMEBUFFER, 0); glDrawBuffer(GL_BACK_LEFT); glBindTexture(GL_TEXTURE_2D, texID[1]); glViewport(0,0,WIDTH, HEIGHT); glEnable(GL_BLEND); glBlendFunc(GL_ONE, GL_ONE); glDrawElements(GL_TRIANGLES,6,GL_UNSIGNED_SHORT,0); glBindVertexArray(0); blurShader.UnUse(); glDisable(GL_BLEND);
Note that we could also enable blending in the fragment shader. Assuming that the two images to be blended are bound to their texture units and their shader samplers are texture1
and texture2
, the additive blending shader code will be like this:
The second color attachment is then set as output while the output results from the vertical smoothing pass (which was written to the third color attachment) is set as input. The horizontal smoothing shader is then applied on a column of pixels which smoothes the entire image. The image is then rendered to the second color attachment. Finally, the blend shader combines the result from the first color attachment with the result from the second color attachment. Note that the same effect could be carried out by using two separate FBOs: a rendering FBO and a filtering FBO, which gives us more flexibility as we can down sample the filtering result to take advantage of hardware linear filtering. This technique has been used in the Implementing variance shadow mapping recipe in Chapter 4, Lights and Shadows.
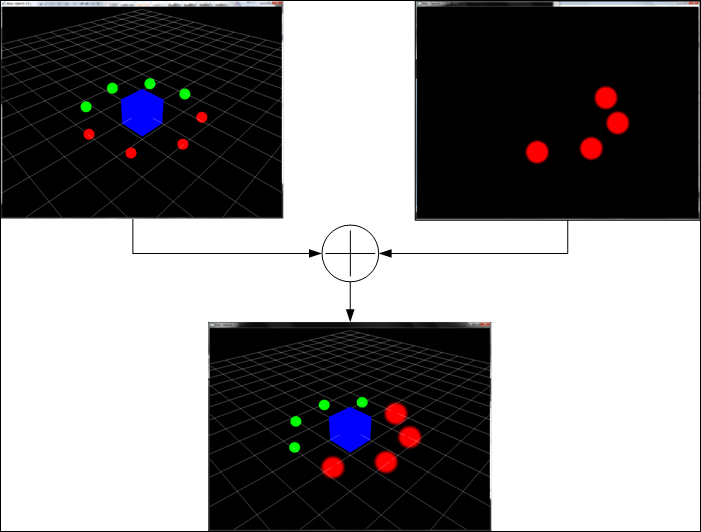
The second color attachment is then set as output while the output results from the vertical smoothing pass (which was written to the third color attachment) is set as input. The horizontal smoothing shader is then applied on a column of pixels which smoothes the entire image. The image is then rendered to the second color attachment. Finally, the blend shader combines the result from the first color attachment with the result from the second color attachment. Note that the same effect could be carried out by using two separate FBOs: a rendering FBO and a filtering FBO, which gives us more flexibility as we can down sample the filtering result to take advantage of hardware linear filtering. This technique has been used in the Implementing variance shadow mapping recipe in Chapter 4, Lights and Shadows.
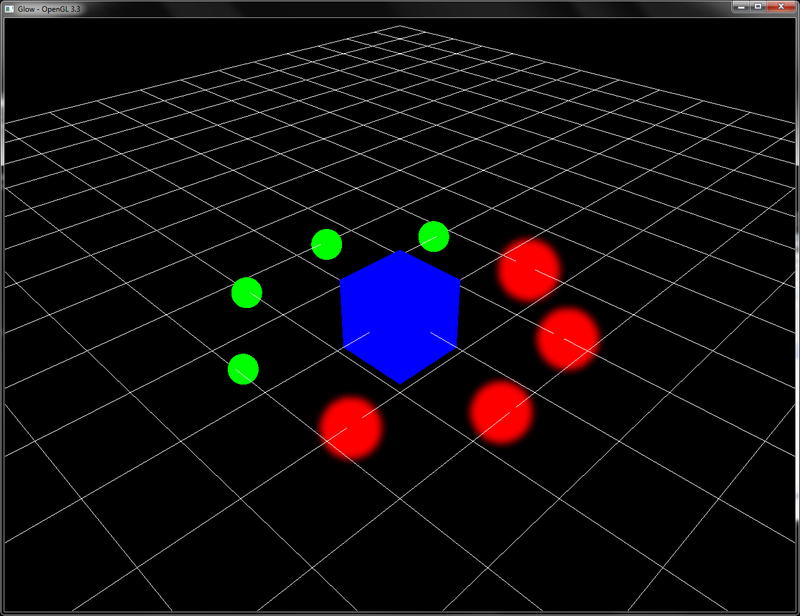
In this chapter, we will cover:
- Implementing per-vertex and per-fragment point lighting
- Implementing per-fragment directional light
- Implementing per-fragment point light with attenuation
- Implementing per-fragment spot light
- Implementing shadow mapping with FBO
- Implementing shadow mapping with percentage closer filtering (PCF)
- Implementing variance shadow mapping
To give more realism to 3D graphic scenes, we add lighting. In OpenGL's fixed function pipeline, per-vertex lighting is provided (which is deprecated in OpenGL v3.3 and above). Using shaders, we can not only replicate the per-vertex lighting of fixed function pipeline but also go a step further by implementing per-fragment lighting. The per-vertex lighting is also known as Gouraud shading and the per-fragment shading is known as Phong shading. So, without further ado, let's get started.
In this recipe, we will render many cubes and a sphere. All of these objects are generated and stored in the buffer objects. For details, refer to the CreateSphere
and CreateCube
functions in Chapter4/PerVertexLighting/main.cpp
. These functions generate both vertex positions as well as per-vertex normals, which are needed for the lighting calculations. All of the lighting calculations take place in the vertex shader of the per-vertex lighting recipe (Chapter4/PerVertexLighting/
), whereas, for the per-fragment lighting recipe (Chapter4/PerFragmentLighting/
) they take place in the fragment shader.
Let us start our recipe by following these simple steps:
- Set up the vertex shader that performs the lighting calculation in the view/eye space. This generates the color after the lighting calculation.
#version 330 core layout(location=0) in vec3 vVertex; layout(location=1) in vec3 vNormal; uniform mat4 MVP; uniform mat4 MV; uniform mat3 N; uniform vec3 light_position; //light position in object space uniform vec3 diffuse_color; uniform vec3 specular_color; uniform float shininess; smooth out vec4 color; const vec3 vEyeSpaceCameraPosition = vec3(0,0,0); void main() { vec4 vEyeSpaceLightPosition = MV*vec4(light_position,1); vec4 vEyeSpacePosition = MV*vec4(vVertex,1); vec3 vEyeSpaceNormal = normalize(N*vNormal); vec3 L = normalize(vEyeSpaceLightPosition.xyz –vEyeSpacePosition.xyz); vec3 V = normalize(vEyeSpaceCameraPosition.xyz- vEyeSpacePosition.xyz); vec3 H = normalize(L+V); float diffuse = max(0, dot(vEyeSpaceNormal, L)); float specular = max(0, pow(dot(vEyeSpaceNormal, H), shininess)); color = diffuse*vec4(diffuse_color,1) + specular*vec4(specular_color, 1); gl_Position = MVP*vec4(vVertex,1); }
- Set up a fragment shader which, inputs the shaded color from the vertex shader interpolated by the rasterizer, and set it as the current output color.
#version 330 core layout(location=0) out vec4 vFragColor; smooth in vec4 color; void main() { vFragColor = color; }
- In the rendering code, set the shader and render the objects by passing their modelview/projection matrices to the shader as shader uniforms.
shader.Use(); glBindVertexArray(cubeVAOID); for(int i=0;i<8;i++) { float theta = (float)(i/8.0f*2*M_PI); glm::mat4 T = glm::translate(glm::mat4(1), glm::vec3(radius*cos(theta), 0.5,radius*sin(theta))); glm::mat4 M = T; glm::mat4 MV = View*M; glm::mat4 MVP = Proj*MV; glUniformMatrix4fv(shader("MVP"), 1, GL_FALSE, glm::value_ptr(MVP)); glUniformMatrix4fv(shader("MV"), 1, GL_FALSE, glm::value_ptr(MV)); glUniformMatrix3fv(shader("N"), 1, GL_FALSE, glm::value_ptr(glm::inverseTranspose(glm::mat3(MV)))); glUniform3fv(shader("diffuse_color"),1, &(colors[i].x)); glUniform3fv(shader("light_position"),1,&(lightPosOS.x)); glDrawElements(GL_TRIANGLES, 36, GL_UNSIGNED_SHORT, 0); } glBindVertexArray(sphereVAOID); glm::mat4 T = glm::translate(glm::mat4(1), glm::vec3(0,1,0)); glm::mat4 M = T; glm::mat4 MV = View*M; glm::mat4 MVP = Proj*MV; glUniformMatrix4fv(shader("MVP"), 1, GL_FALSE, glm::value_ptr(MVP)); glUniformMatrix4fv(shader("MV"), 1, GL_FALSE, glm::value_ptr(MV)); glUniformMatrix3fv(shader("N"), 1, GL_FALSE, glm::value_ptr(glm::inverseTranspose(glm::mat3(MV)))); glUniform3f(shader("diffuse_color"), 0.9f, 0.9f, 1.0f); glUniform3fv(shader("light_position"),1, &(lightPosOS.x)); glDrawElements(GL_TRIANGLES, totalSphereTriangles, GL_UNSIGNED_SHORT, 0); shader.UnUse(); glBindVertexArray(0); grid->Render(glm::value_ptr(Proj*View));
We can perform the lighting calculations in any coordinate space we wish, that is, object space, world space, or eye/view space. Similar to the lighting in the fixed function OpenGL pipeline, in this recipe we also do our calculations in the eye space. The first step in the vertex shader is to obtain the vertex position and light position in the eye space. This is done by multiplying the current vertex and light position with the modelview (MV
) matrix.
These are used for specular component calculation in the Blinn Phong lighting model. The specular component is then obtained using pow(dot(N,H), σ)
, where σ
is the shininess value; the larger the shininess, the more focused the specular.
In the fragment shader, the rest of the calculation, including the diffuse and specular component contributions, is carried out.
Next, the diffuse component is calculated using the dot product with the eye space normal.
The specular component is calculated as in the per-vertex case.
Finally, the combined color is obtained by summing the diffuse and specular contributions. The diffuse contribution is obtained by multiplying the diffuse color with the diffuse component and the specular contribution is obtained by multiplying the specular component with the specular color.
many cubes and a sphere. All of these objects are generated and stored in the buffer objects. For details, refer to the CreateSphere
and CreateCube
functions in Chapter4/PerVertexLighting/main.cpp
. These functions generate both vertex positions as well as per-vertex normals, which are needed for the lighting calculations. All of the lighting calculations take place in the vertex shader of the per-vertex lighting recipe (Chapter4/PerVertexLighting/
), whereas, for the per-fragment lighting recipe (Chapter4/PerFragmentLighting/
) they take place in the fragment shader.
Let us start our recipe by following these simple steps:
- Set up the vertex shader that performs the lighting calculation in the view/eye space. This generates the color after the lighting calculation.
#version 330 core layout(location=0) in vec3 vVertex; layout(location=1) in vec3 vNormal; uniform mat4 MVP; uniform mat4 MV; uniform mat3 N; uniform vec3 light_position; //light position in object space uniform vec3 diffuse_color; uniform vec3 specular_color; uniform float shininess; smooth out vec4 color; const vec3 vEyeSpaceCameraPosition = vec3(0,0,0); void main() { vec4 vEyeSpaceLightPosition = MV*vec4(light_position,1); vec4 vEyeSpacePosition = MV*vec4(vVertex,1); vec3 vEyeSpaceNormal = normalize(N*vNormal); vec3 L = normalize(vEyeSpaceLightPosition.xyz –vEyeSpacePosition.xyz); vec3 V = normalize(vEyeSpaceCameraPosition.xyz- vEyeSpacePosition.xyz); vec3 H = normalize(L+V); float diffuse = max(0, dot(vEyeSpaceNormal, L)); float specular = max(0, pow(dot(vEyeSpaceNormal, H), shininess)); color = diffuse*vec4(diffuse_color,1) + specular*vec4(specular_color, 1); gl_Position = MVP*vec4(vVertex,1); }
- Set up a fragment shader which, inputs the shaded color from the vertex shader interpolated by the rasterizer, and set it as the current output color.
#version 330 core layout(location=0) out vec4 vFragColor; smooth in vec4 color; void main() { vFragColor = color; }
- In the rendering code, set the shader and render the objects by passing their modelview/projection matrices to the shader as shader uniforms.
shader.Use(); glBindVertexArray(cubeVAOID); for(int i=0;i<8;i++) { float theta = (float)(i/8.0f*2*M_PI); glm::mat4 T = glm::translate(glm::mat4(1), glm::vec3(radius*cos(theta), 0.5,radius*sin(theta))); glm::mat4 M = T; glm::mat4 MV = View*M; glm::mat4 MVP = Proj*MV; glUniformMatrix4fv(shader("MVP"), 1, GL_FALSE, glm::value_ptr(MVP)); glUniformMatrix4fv(shader("MV"), 1, GL_FALSE, glm::value_ptr(MV)); glUniformMatrix3fv(shader("N"), 1, GL_FALSE, glm::value_ptr(glm::inverseTranspose(glm::mat3(MV)))); glUniform3fv(shader("diffuse_color"),1, &(colors[i].x)); glUniform3fv(shader("light_position"),1,&(lightPosOS.x)); glDrawElements(GL_TRIANGLES, 36, GL_UNSIGNED_SHORT, 0); } glBindVertexArray(sphereVAOID); glm::mat4 T = glm::translate(glm::mat4(1), glm::vec3(0,1,0)); glm::mat4 M = T; glm::mat4 MV = View*M; glm::mat4 MVP = Proj*MV; glUniformMatrix4fv(shader("MVP"), 1, GL_FALSE, glm::value_ptr(MVP)); glUniformMatrix4fv(shader("MV"), 1, GL_FALSE, glm::value_ptr(MV)); glUniformMatrix3fv(shader("N"), 1, GL_FALSE, glm::value_ptr(glm::inverseTranspose(glm::mat3(MV)))); glUniform3f(shader("diffuse_color"), 0.9f, 0.9f, 1.0f); glUniform3fv(shader("light_position"),1, &(lightPosOS.x)); glDrawElements(GL_TRIANGLES, totalSphereTriangles, GL_UNSIGNED_SHORT, 0); shader.UnUse(); glBindVertexArray(0); grid->Render(glm::value_ptr(Proj*View));
We can perform the lighting calculations in any coordinate space we wish, that is, object space, world space, or eye/view space. Similar to the lighting in the fixed function OpenGL pipeline, in this recipe we also do our calculations in the eye space. The first step in the vertex shader is to obtain the vertex position and light position in the eye space. This is done by multiplying the current vertex and light position with the modelview (MV
) matrix.
These are used for specular component calculation in the Blinn Phong lighting model. The specular component is then obtained using pow(dot(N,H), σ)
, where σ
is the shininess value; the larger the shininess, the more focused the specular.
In the fragment shader, the rest of the calculation, including the diffuse and specular component contributions, is carried out.
Next, the diffuse component is calculated using the dot product with the eye space normal.
The specular component is calculated as in the per-vertex case.
Finally, the combined color is obtained by summing the diffuse and specular contributions. The diffuse contribution is obtained by multiplying the diffuse color with the diffuse component and the specular contribution is obtained by multiplying the specular component with the specular color.
#version 330 core layout(location=0) in vec3 vVertex; layout(location=1) in vec3 vNormal; uniform mat4 MVP; uniform mat4 MV; uniform mat3 N; uniform vec3 light_position; //light position in object space uniform vec3 diffuse_color; uniform vec3 specular_color; uniform float shininess; smooth out vec4 color; const vec3 vEyeSpaceCameraPosition = vec3(0,0,0); void main() { vec4 vEyeSpaceLightPosition = MV*vec4(light_position,1); vec4 vEyeSpacePosition = MV*vec4(vVertex,1); vec3 vEyeSpaceNormal = normalize(N*vNormal); vec3 L = normalize(vEyeSpaceLightPosition.xyz –vEyeSpacePosition.xyz); vec3 V = normalize(vEyeSpaceCameraPosition.xyz- vEyeSpacePosition.xyz); vec3 H = normalize(L+V); float diffuse = max(0, dot(vEyeSpaceNormal, L)); float specular = max(0, pow(dot(vEyeSpaceNormal, H), shininess)); color = diffuse*vec4(diffuse_color,1) + specular*vec4(specular_color, 1); gl_Position = MVP*vec4(vVertex,1); }
- fragment shader which, inputs the shaded color from the vertex shader interpolated by the rasterizer, and set it as the current output color.
#version 330 core layout(location=0) out vec4 vFragColor; smooth in vec4 color; void main() { vFragColor = color; }
- In the rendering code, set the shader and render the objects by passing their modelview/projection matrices to the shader as shader uniforms.
shader.Use(); glBindVertexArray(cubeVAOID); for(int i=0;i<8;i++) { float theta = (float)(i/8.0f*2*M_PI); glm::mat4 T = glm::translate(glm::mat4(1), glm::vec3(radius*cos(theta), 0.5,radius*sin(theta))); glm::mat4 M = T; glm::mat4 MV = View*M; glm::mat4 MVP = Proj*MV; glUniformMatrix4fv(shader("MVP"), 1, GL_FALSE, glm::value_ptr(MVP)); glUniformMatrix4fv(shader("MV"), 1, GL_FALSE, glm::value_ptr(MV)); glUniformMatrix3fv(shader("N"), 1, GL_FALSE, glm::value_ptr(glm::inverseTranspose(glm::mat3(MV)))); glUniform3fv(shader("diffuse_color"),1, &(colors[i].x)); glUniform3fv(shader("light_position"),1,&(lightPosOS.x)); glDrawElements(GL_TRIANGLES, 36, GL_UNSIGNED_SHORT, 0); } glBindVertexArray(sphereVAOID); glm::mat4 T = glm::translate(glm::mat4(1), glm::vec3(0,1,0)); glm::mat4 M = T; glm::mat4 MV = View*M; glm::mat4 MVP = Proj*MV; glUniformMatrix4fv(shader("MVP"), 1, GL_FALSE, glm::value_ptr(MVP)); glUniformMatrix4fv(shader("MV"), 1, GL_FALSE, glm::value_ptr(MV)); glUniformMatrix3fv(shader("N"), 1, GL_FALSE, glm::value_ptr(glm::inverseTranspose(glm::mat3(MV)))); glUniform3f(shader("diffuse_color"), 0.9f, 0.9f, 1.0f); glUniform3fv(shader("light_position"),1, &(lightPosOS.x)); glDrawElements(GL_TRIANGLES, totalSphereTriangles, GL_UNSIGNED_SHORT, 0); shader.UnUse(); glBindVertexArray(0); grid->Render(glm::value_ptr(Proj*View));
We can perform the lighting calculations in any coordinate space we wish, that is, object space, world space, or eye/view space. Similar to the lighting in the fixed function OpenGL pipeline, in this recipe we also do our calculations in the eye space. The first step in the vertex shader is to obtain the vertex position and light position in the eye space. This is done by multiplying the current vertex and light position with the modelview (MV
) matrix.
These are used for specular component calculation in the Blinn Phong lighting model. The specular component is then obtained using pow(dot(N,H), σ)
, where σ
is the shininess value; the larger the shininess, the more focused the specular.
In the fragment shader, the rest of the calculation, including the diffuse and specular component contributions, is carried out.
Next, the diffuse component is calculated using the dot product with the eye space normal.
The specular component is calculated as in the per-vertex case.
Finally, the combined color is obtained by summing the diffuse and specular contributions. The diffuse contribution is obtained by multiplying the diffuse color with the diffuse component and the specular contribution is obtained by multiplying the specular component with the specular color.
perform the lighting calculations in any coordinate space we wish, that is, object space, world space, or eye/view space. Similar to the lighting in the fixed function OpenGL pipeline, in this recipe we also do our calculations in the eye space. The first step in the vertex shader is to obtain the vertex position and light position in the eye space. This is done by multiplying the current vertex and light position with the modelview (MV
) matrix.
These are used for specular component calculation in the Blinn Phong lighting model. The specular component is then obtained using pow(dot(N,H), σ)
, where σ
is the shininess value; the larger the shininess, the more focused the specular.
In the fragment shader, the rest of the calculation, including the diffuse and specular component contributions, is carried out.
Next, the diffuse component is calculated using the dot product with the eye space normal.
The specular component is calculated as in the per-vertex case.
Finally, the combined color is obtained by summing the diffuse and specular contributions. The diffuse contribution is obtained by multiplying the diffuse color with the diffuse component and the specular contribution is obtained by multiplying the specular component with the specular color.
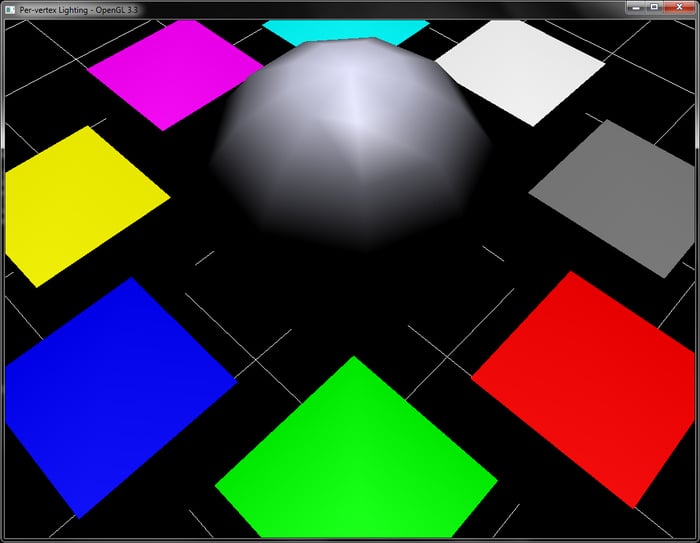
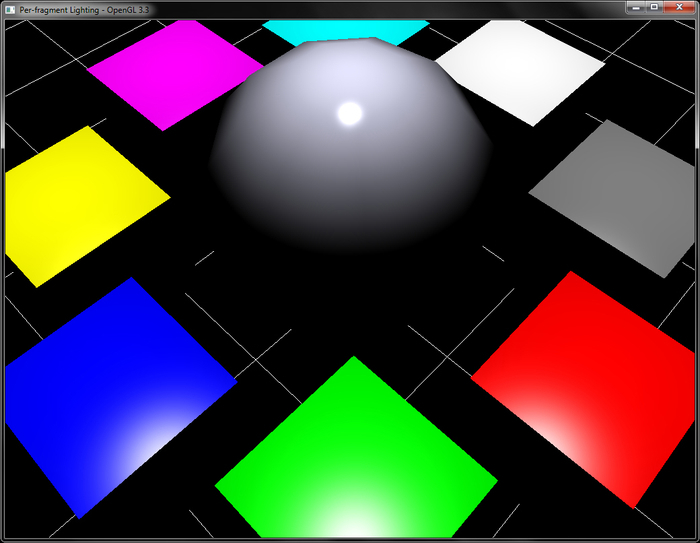
In this recipe, we will now implement directional light. The only difference between a point light and a directional light is that in the case of the directional light source, there is no position, however, there is direction, as shown in the following figure.
We will build on the geometry handling code from the per-fragment lighting recipe, but, instead of the pulsating cubes, we will now render a single cube with a sphere. The code for this recipe is contained in the Chapter4/DirectionalLight
folder. The same code also works for per-vertex directional light.
Let us start the recipe by following these simple steps:
- Calculate the light direction in eye space and pass it as shader uniform. Note that the last component is
0
since now we have a light direction vector.lightDirectionES = glm::vec3(MV*glm::vec4(lightDirectionOS,0));
- In the vertex shader, output the eye space normal.
#version 330 core layout(location=0) in vec3 vVertex; layout(location=1) in vec3 vNormal; uniform mat4 MVP; uniform mat3 N; smooth out vec3 vEyeSpaceNormal; void main() { vEyeSpaceNormal = N*vNormal; gl_Position = MVP*vec4(vVertex,1); }
- In the fragment shader, compute the diffuse component by calculating the dot product between the light direction vector in eye space with the eye space normal, and multiply with the diffuse color to get the fragment color. Note that here, the light vector is independent of the eye space vertex position.
#version 330 core layout(location=0) out vec4 vFragColor; uniform vec3 light_direction; uniform vec3 diffuse_color; smooth in vec3 vEyeSpaceNormal; void main() { vec3 L = (light_direction); float diffuse = max(0, dot(vEyeSpaceNormal, L)); vFragColor = diffuse*vec4(diffuse_color,1); }
The only difference between this recipe and the previous one is that we now pass the light direction instead of the position to the fragment shader. The rest of the calculation remains unchanged. If we want to apply attenuation, we can add the relevant shader snippets from the previous recipe.
Let us start the recipe by following these simple steps:
- Calculate the light direction in eye space and pass it as shader uniform. Note that the last component is
0
since now we have a light direction vector.lightDirectionES = glm::vec3(MV*glm::vec4(lightDirectionOS,0));
- In the vertex shader, output the eye space normal.
#version 330 core layout(location=0) in vec3 vVertex; layout(location=1) in vec3 vNormal; uniform mat4 MVP; uniform mat3 N; smooth out vec3 vEyeSpaceNormal; void main() { vEyeSpaceNormal = N*vNormal; gl_Position = MVP*vec4(vVertex,1); }
- In the fragment shader, compute the diffuse component by calculating the dot product between the light direction vector in eye space with the eye space normal, and multiply with the diffuse color to get the fragment color. Note that here, the light vector is independent of the eye space vertex position.
#version 330 core layout(location=0) out vec4 vFragColor; uniform vec3 light_direction; uniform vec3 diffuse_color; smooth in vec3 vEyeSpaceNormal; void main() { vec3 L = (light_direction); float diffuse = max(0, dot(vEyeSpaceNormal, L)); vFragColor = diffuse*vec4(diffuse_color,1); }
The only difference between this recipe and the previous one is that we now pass the light direction instead of the position to the fragment shader. The rest of the calculation remains unchanged. If we want to apply attenuation, we can add the relevant shader snippets from the previous recipe.
0
since now we have a light direction vector.lightDirectionES = glm::vec3(MV*glm::vec4(lightDirectionOS,0));
#version 330 core layout(location=0) in vec3 vVertex; layout(location=1) in vec3 vNormal; uniform mat4 MVP; uniform mat3 N; smooth out vec3 vEyeSpaceNormal; void main() { vEyeSpaceNormal = N*vNormal; gl_Position = MVP*vec4(vVertex,1); }
#version 330 core layout(location=0) out vec4 vFragColor; uniform vec3 light_direction; uniform vec3 diffuse_color; smooth in vec3 vEyeSpaceNormal; void main() { vec3 L = (light_direction); float diffuse = max(0, dot(vEyeSpaceNormal, L)); vFragColor = diffuse*vec4(diffuse_color,1); }
The only difference between this recipe and the previous one is that we now pass the light direction instead of the position to the fragment shader. The rest of the calculation remains unchanged. If we want to apply attenuation, we can add the relevant shader snippets from the previous recipe.
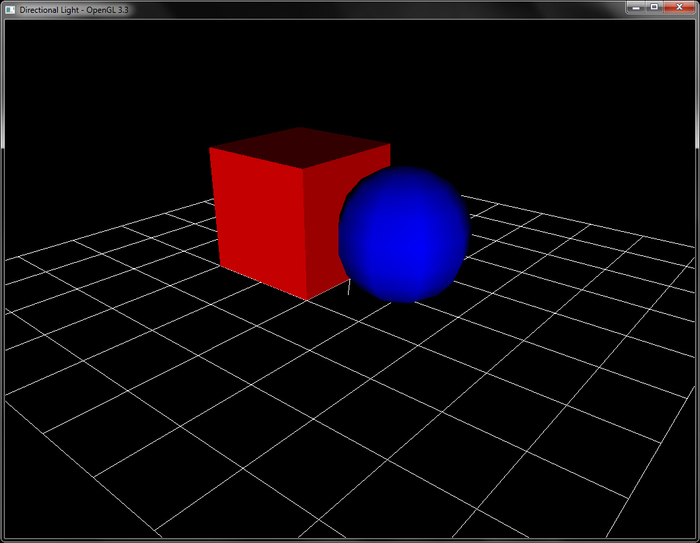
Implementing per-fragment point light is demonstrated by following these steps:
- From the vertex shader, output the eye space vertex position and normal.
#version 330 core layout(location=0) in vec3 vVertex; layout(location=1) in vec3 vNormal; uniform mat4 MVP; uniform mat4 MV; uniform mat3 N; smooth out vec3 vEyeSpaceNormal; smooth out vec3 vEyeSpacePosition; void main() { vEyeSpacePosition = (MV*vec4(vVertex,1)).xyz; vEyeSpaceNormal = N*vNormal; gl_Position = MVP*vec4(vVertex,1); }
- In the fragment shader, calculate the light position in eye space, and then calculate the vector from the eye space vertex position to the eye space light position. Store the light distance before normalizing the light vector.
#version 330 core layout(location=0) out vec4 vFragColor; uniform vec3 light_position; //light position in object space uniform vec3 diffuse_color; uniform mat4 MV; smooth in vec3 vEyeSpaceNormal; smooth in vec3 vEyeSpacePosition; const float k0 = 1.0; //constant attenuation const float k1 = 0.0; //linear attenuation const float k2 = 0.0; //quadratic attenuation void main() { vec3 vEyeSpaceLightPosition = (MV*vec4(light_position,1)).xyz; vec3 L = (vEyeSpaceLightPosition-vEyeSpacePosition); float d = length(L); L = normalize(L); float diffuse = max(0, dot(vEyeSpaceNormal, L)); float attenuationAmount = 1.0/(k0 + (k1*d) + (k2*d*d)); diffuse *= attenuationAmount; vFragColor = diffuse*vec4(diffuse_color,1); }
- Apply attenuation based on the distance from the light source to the diffuse component.
float attenuationAmount = 1.0/(k0 + (k1*d) + (k2*d*d)); diffuse *= attenuationAmount;
- Multiply the diffuse component to the diffuse color and set it as the fragment color.
vFragColor = diffuse*vec4(diffuse_color,1);
The recipe follows the Implementing per-fragment directional light recipe. In addition, it performs the attenuation calculation. The attenuation of light is calculated by using the following formula:
Implementing per-fragment point light is demonstrated by following these steps:
- From the vertex shader, output the eye space vertex position and normal.
#version 330 core layout(location=0) in vec3 vVertex; layout(location=1) in vec3 vNormal; uniform mat4 MVP; uniform mat4 MV; uniform mat3 N; smooth out vec3 vEyeSpaceNormal; smooth out vec3 vEyeSpacePosition; void main() { vEyeSpacePosition = (MV*vec4(vVertex,1)).xyz; vEyeSpaceNormal = N*vNormal; gl_Position = MVP*vec4(vVertex,1); }
- In the fragment shader, calculate the light position in eye space, and then calculate the vector from the eye space vertex position to the eye space light position. Store the light distance before normalizing the light vector.
#version 330 core layout(location=0) out vec4 vFragColor; uniform vec3 light_position; //light position in object space uniform vec3 diffuse_color; uniform mat4 MV; smooth in vec3 vEyeSpaceNormal; smooth in vec3 vEyeSpacePosition; const float k0 = 1.0; //constant attenuation const float k1 = 0.0; //linear attenuation const float k2 = 0.0; //quadratic attenuation void main() { vec3 vEyeSpaceLightPosition = (MV*vec4(light_position,1)).xyz; vec3 L = (vEyeSpaceLightPosition-vEyeSpacePosition); float d = length(L); L = normalize(L); float diffuse = max(0, dot(vEyeSpaceNormal, L)); float attenuationAmount = 1.0/(k0 + (k1*d) + (k2*d*d)); diffuse *= attenuationAmount; vFragColor = diffuse*vec4(diffuse_color,1); }
- Apply attenuation based on the distance from the light source to the diffuse component.
float attenuationAmount = 1.0/(k0 + (k1*d) + (k2*d*d)); diffuse *= attenuationAmount;
- Multiply the diffuse component to the diffuse color and set it as the fragment color.
vFragColor = diffuse*vec4(diffuse_color,1);
The recipe follows the Implementing per-fragment directional light recipe. In addition, it performs the attenuation calculation. The attenuation of light is calculated by using the following formula:
#version 330 core layout(location=0) in vec3 vVertex; layout(location=1) in vec3 vNormal; uniform mat4 MVP; uniform mat4 MV; uniform mat3 N; smooth out vec3 vEyeSpaceNormal; smooth out vec3 vEyeSpacePosition; void main() { vEyeSpacePosition = (MV*vec4(vVertex,1)).xyz; vEyeSpaceNormal = N*vNormal; gl_Position = MVP*vec4(vVertex,1); }
- fragment shader, calculate the light position in eye space, and then calculate the vector from the eye space vertex position to the eye space light position. Store the light distance before normalizing the light vector.
#version 330 core layout(location=0) out vec4 vFragColor; uniform vec3 light_position; //light position in object space uniform vec3 diffuse_color; uniform mat4 MV; smooth in vec3 vEyeSpaceNormal; smooth in vec3 vEyeSpacePosition; const float k0 = 1.0; //constant attenuation const float k1 = 0.0; //linear attenuation const float k2 = 0.0; //quadratic attenuation void main() { vec3 vEyeSpaceLightPosition = (MV*vec4(light_position,1)).xyz; vec3 L = (vEyeSpaceLightPosition-vEyeSpacePosition); float d = length(L); L = normalize(L); float diffuse = max(0, dot(vEyeSpaceNormal, L)); float attenuationAmount = 1.0/(k0 + (k1*d) + (k2*d*d)); diffuse *= attenuationAmount; vFragColor = diffuse*vec4(diffuse_color,1); }
- Apply attenuation based on the distance from the light source to the diffuse component.
float attenuationAmount = 1.0/(k0 + (k1*d) + (k2*d*d)); diffuse *= attenuationAmount;
- Multiply the diffuse component to the diffuse color and set it as the fragment color.
vFragColor = diffuse*vec4(diffuse_color,1);
The recipe follows the Implementing per-fragment directional light recipe. In addition, it performs the attenuation calculation. The attenuation of light is calculated by using the following formula:
follows the Implementing per-fragment directional light recipe. In addition, it performs the attenuation calculation. The attenuation of light is calculated by using the following formula:
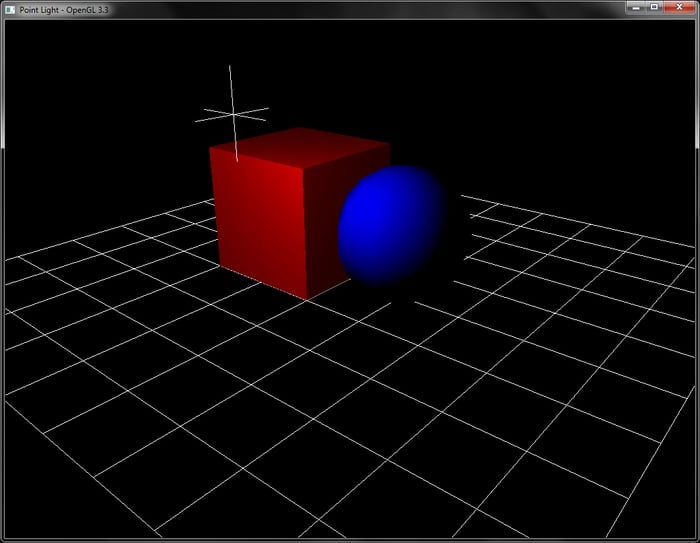
We will now implement per-fragment spot light. Spot light is a special point light that emits light in a directional cone. The size of this cone is determined by the spot cutoff amount, which is given in angles, as shown in the following figure. In addition, the sharpness of the spot is controlled by the parameter spot exponent. A higher value of the exponent gives a sharper falloff and vice versa.
The code for this recipe is contained in the Chapter4/SpotLight
directory. The vertex shader is the same as in the point light recipe. The fragment shader calculates the diffuse component, as in the Implementing per-vertex and per-fragment point lighting recipe.
Let us start this recipe by following these simple steps:
- From the light's object space position and spot light target's position, calculate the spot light direction vector in eye space.
spotDirectionES = glm::normalize(glm::vec3(MV*glm::vec4(spotPositionOS-lightPosOS,0)))
- In the fragment shader, calculate the diffuse component as in point light. In addition, calculate the spot effect by finding the angle between the light direction and the spot direction vector.
vec3 L = (light_position.xyz-vEyeSpacePosition); float d = length(L); L = normalize(L); vec3 D = normalize(spot_direction); vec3 V = -L; float diffuse = 1; float spotEffect = dot(V,D);
- If the angle is greater than the spot cutoff, apply the spot exponent and then use the diffuse shader on the fragment.
if(spotEffect > spot_cutoff) { spotEffect = pow(spotEffect, spot_exponent); diffuse = max(0, dot(vEyeSpaceNormal, L)); float attenuationAmount = spotEffect/(k0 + (k1*d) + (k2*d*d)); diffuse *= attenuationAmount; vFragColor = diffuse*vec4(diffuse_color,1); }
The spot light is a special point light source that illuminates in a certain cone of direction. The amount of cone and the sharpness is controlled using the spot cutoff and spot exponent parameters respectively. Similar to the point light source, we first calculate the diffuse component. Instead of using the vector to light source (L
) we use the opposite vector, which points in the direction of light (V=-L
). Then we find out if the angle between the spot direction and the light direction vector is within the cutoff angle range. If it is, we apply the diffuse shading calculation. In addition, the sharpness of the spot light is controlled using the spot exponent parameter. This reduces the light in a falloff, giving a more pleasing spot light effect.
Let us start this recipe by following these simple steps:
- From the light's object space position and spot light target's position, calculate the spot light direction vector in eye space.
spotDirectionES = glm::normalize(glm::vec3(MV*glm::vec4(spotPositionOS-lightPosOS,0)))
- In the fragment shader, calculate the diffuse component as in point light. In addition, calculate the spot effect by finding the angle between the light direction and the spot direction vector.
vec3 L = (light_position.xyz-vEyeSpacePosition); float d = length(L); L = normalize(L); vec3 D = normalize(spot_direction); vec3 V = -L; float diffuse = 1; float spotEffect = dot(V,D);
- If the angle is greater than the spot cutoff, apply the spot exponent and then use the diffuse shader on the fragment.
if(spotEffect > spot_cutoff) { spotEffect = pow(spotEffect, spot_exponent); diffuse = max(0, dot(vEyeSpaceNormal, L)); float attenuationAmount = spotEffect/(k0 + (k1*d) + (k2*d*d)); diffuse *= attenuationAmount; vFragColor = diffuse*vec4(diffuse_color,1); }
The spot light is a special point light source that illuminates in a certain cone of direction. The amount of cone and the sharpness is controlled using the spot cutoff and spot exponent parameters respectively. Similar to the point light source, we first calculate the diffuse component. Instead of using the vector to light source (L
) we use the opposite vector, which points in the direction of light (V=-L
). Then we find out if the angle between the spot direction and the light direction vector is within the cutoff angle range. If it is, we apply the diffuse shading calculation. In addition, the sharpness of the spot light is controlled using the spot exponent parameter. This reduces the light in a falloff, giving a more pleasing spot light effect.
spotDirectionES = glm::normalize(glm::vec3(MV*glm::vec4(spotPositionOS-lightPosOS,0)))
vec3 L = (light_position.xyz-vEyeSpacePosition); float d = length(L); L = normalize(L); vec3 D = normalize(spot_direction); vec3 V = -L; float diffuse = 1; float spotEffect = dot(V,D);
if(spotEffect > spot_cutoff) { spotEffect = pow(spotEffect, spot_exponent); diffuse = max(0, dot(vEyeSpaceNormal, L)); float attenuationAmount = spotEffect/(k0 + (k1*d) + (k2*d*d)); diffuse *= attenuationAmount; vFragColor = diffuse*vec4(diffuse_color,1); }
The spot light is a special point light source that illuminates in a certain cone of direction. The amount of cone and the sharpness is controlled using the spot cutoff and spot exponent parameters respectively. Similar to the point light source, we first calculate the diffuse component. Instead of using the vector to light source (L
) we use the opposite vector, which points in the direction of light (V=-L
). Then we find out if the angle between the spot direction and the light direction vector is within the cutoff angle range. If it is, we apply the diffuse shading calculation. In addition, the sharpness of the spot light is controlled using the spot exponent parameter. This reduces the light in a falloff, giving a more pleasing spot light effect.
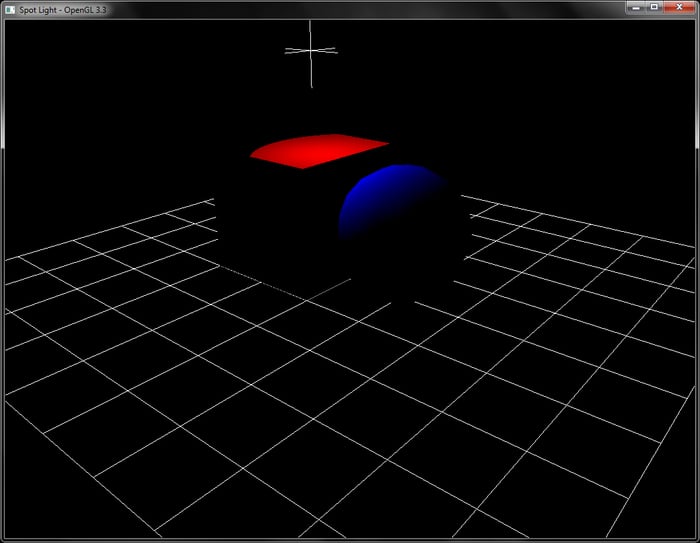
For this recipe, we will use the previous scene but instead of a grid object, we will use a plane object so that the generated shadows can be seen. The code for this recipe is contained in the Chapter4/ShadowMapping
directory.
Let us start with this recipe by following these simple steps:
- Create an OpenGL texture object which will be our shadow map texture. Make sure to set the clamp mode to
GL_CLAMP_TO_BORDER
, set the border color to{1,0,0,0}
, give the texture comparison mode toGL_COMPARE_REF_TO_TEXTURE
, and set the compare function toGL_LEQUAL
. Set the texture internal format toGL_DEPTH_COMPONENT24
.glGenTextures(1, &shadowMapTexID); glActiveTexture(GL_TEXTURE0); glBindTexture(GL_TEXTURE_2D, shadowMapTexID); GLfloat border[4]={1,0,0,0}; glTexParameteri(GL_TEXTURE_2D,GL_TEXTURE_MAG_FILTER,GL_NEAREST); glTexParameteri(GL_TEXTURE_2D,GL_TEXTURE_MIN_FILTER,GL_NEAREST); glTexParameteri(GL_TEXTURE_2D,GL_TEXTURE_WRAP_S,GL_CLAMP_TO_BORDER); glTexParameteri(GL_TEXTURE_2D,GL_TEXTURE_WRAP_T,GL_CLAMP_TO_BORDER); glTexParameteri(GL_TEXTURE_2D,GL_TEXTURE_COMPARE_MODE,GL_COMPARE_REF_TO_TEXTURE); glTexParameteri(GL_TEXTURE_2D,GL_TEXTURE_COMPARE_FUNC,GL_LEQUAL); glTexParameterfv(GL_TEXTURE_2D,GL_TEXTURE_BORDER_COLOR,border); glTexImage2D(GL_TEXTURE_2D,0,GL_DEPTH_COMPONENT24,SHADOWMAP_WIDTH,SHADOWMAP_HEIGHT,0,GL_DEPTH_COMPONENT,GL_UNSIGNED_BYTE,NULL);
- Set up an FBO and use the shadow map texture as a single depth attachment. This will store the scene's depth from the point of view of light.
glGenFramebuffers(1,&fboID); glBindFramebuffer(GL_FRAMEBUFFER,fboID); glFramebufferTexture2D(GL_FRAMEBUFFER,GL_DEPTH_ATTACHMENT,GL_TEXTURE_2D,shadowMapTexID,0); GLenum status = glCheckFramebufferStatus(GL_FRAMEBUFFER); if(status == GL_FRAMEBUFFER_COMPLETE) { cout<<"FBO setup successful."<<endl; } else { cout<<"Problem in FBO setup."<<endl; } glBindFramebuffer(GL_FRAMEBUFFER,0);
- Using the position and the direction of the light, set up the shadow matrix (
S
) by combining the light modelview matrix (MV_L
), projection matrix (P_L
), and bias matrix (B
). For reducing runtime calculation, we store the combined projection and bias matrix (BP
) at initialization.MV_L = glm::lookAt(lightPosOS,glm::vec3(0,0,0), glm::vec3(0,1,0)); P_L = glm::perspective(50.0f,1.0f,1.0f, 25.0f); B = glm::scale(glm::translate(glm::mat4(1), glm::vec3(0.5,0.5,0.5)),glm::vec3(0.5,0.5,0.5)); BP = B*P_L; S = BP*MV_L;
- Bind the FBO and render the scene from the point of view of the light. Make sure to enable front-face culling (
glEnable(GL_CULL_FACE)
andglCullFace(GL_FRONT)
) so that the back-face depth values are rendered. Otherwise our objects will suffer from shadow acne. - Disable FBO, restore default viewport, and render the scene normally from the point of view of the camera.
glBindFramebuffer(GL_FRAMEBUFFER,0); glViewport(0,0,WIDTH, HEIGHT); DrawScene(MV, P, 0 );
- In the vertex shader, multiply the world space vertex positions (
M*vec4(vVertex,1)
) with the shadow matrix (S
) to obtain the shadow coordinates. These will be used for lookup of the depth values from theshadowmap
texture in the fragment shader.#version 330 core layout(location=0) in vec3 vVertex; layout(location=1) in vec3 vNormal; uniform mat4 MVP; //modelview projection matrix uniform mat4 MV; //modelview matrix uniform mat4 M; //model matrix uniform mat3 N; //normal matrix uniform mat4 S; //shadow matrix smooth out vec3 vEyeSpaceNormal; smooth out vec3 vEyeSpacePosition; smooth out vec4 vShadowCoords; void main() { vEyeSpacePosition = (MV*vec4(vVertex,1)).xyz; vEyeSpaceNormal = N*vNormal; vShadowCoords = S*(M*vec4(vVertex,1)); gl_Position = MVP*vec4(vVertex,1); }
- In the fragment shader, use the shadow coordinates to lookup the depth value in the shadow map sampler which is of the
sampler2Dshadow
type. This sampler can be used with thetextureProj
function to return a comparison outcome. We then use the comparison result to darken the diffuse component, simulating shadows.#version 330 core layout(location=0) out vec4 vFragColor; uniform sampler2DShadow shadowMap; uniform vec3 light_position; //light position in eye space uniform vec3 diffuse_color; smooth in vec3 vEyeSpaceNormal; smooth in vec3 vEyeSpacePosition; smooth in vec4 vShadowCoords; const float k0 = 1.0; //constant attenuation const float k1 = 0.0; //linear attenuation const float k2 = 0.0; //quadratic attenuation uniform bool bIsLightPass; //no shadows in light pass void main() { if(bIsLightPass) return; vec3 L = (light_position.xyz-vEyeSpacePosition); float d = length(L); L = normalize(L); float attenuationAmount = 1.0/(k0 + (k1*d) + (k2*d*d)); float diffuse = max(0, dot(vEyeSpaceNormal, L)) * attenuationAmount; if(vShadowCoords.w>1) { float shadow = textureProj(shadowMap, vShadowCoords); diffuse = mix(diffuse, diffuse*shadow, 0.5); } vFragColor = diffuse*vec4(diffuse_color, 1); }
The shadow mapping algorithm works in two passes. In the first pass, the scene is rendered from the point of view of light, and the depth buffer is stored into a texture called shadowmap
. We use a single FBO with a depth attachment for this purpose. Apart from the conventional minification/magnification texture filtering, we set the texture wrapping mode to GL_CLAMP_TO_BORDER
, which ensures that the values are clamped to the specified border color. Had we set this as GL_CLAMP
or GL_CLAMP_TO_EDGE
, the border pixels forming the shadow map would produce visible artefacts.
To render the scene from the point of view of light, the modelview matrix of the light (MV_L
), the projection matrix (P_L
), and the bias matrix (B
) are calculated. After multiplying with the projection matrix, the coordinates are in clip space (that is, they range from [-1,-1,-1]). to [1,1,1]. The bias matrix rescales this range to bring the coordinates from [0,0,0] to [1,1,1] range so that the shadow lookup can be carried out.
The shadow map lookup computation is facilitated by the textureProj
GLSL function. The result from the shadow map lookup returns 1 or 0. This result is multiplied with the shading computation. As it happens in the real world, we never have coal black shadows. Therefore, we combine the shadow outcome with the shading computation by using the mix
GLSL function.
- Real-time Shadows, Elmar Eisemann, Michael Schwarz, Ulf Assarsson, Michael Wimmer, A K Peters/CRC Press
- OpenGL 4.0 Shading Language Cookbook, Chapter 7, Shadows, David Wolff, Packt Publishing
- ShadowMapping with GLSL by Fabien Sanglard: http://www.fabiensanglard.net/shadowmapping/index.php
will use the previous scene but instead of a grid object, we will use a plane object so that the generated shadows can be seen. The code for this recipe is contained in the Chapter4/ShadowMapping
directory.
Let us start with this recipe by following these simple steps:
- Create an OpenGL texture object which will be our shadow map texture. Make sure to set the clamp mode to
GL_CLAMP_TO_BORDER
, set the border color to{1,0,0,0}
, give the texture comparison mode toGL_COMPARE_REF_TO_TEXTURE
, and set the compare function toGL_LEQUAL
. Set the texture internal format toGL_DEPTH_COMPONENT24
.glGenTextures(1, &shadowMapTexID); glActiveTexture(GL_TEXTURE0); glBindTexture(GL_TEXTURE_2D, shadowMapTexID); GLfloat border[4]={1,0,0,0}; glTexParameteri(GL_TEXTURE_2D,GL_TEXTURE_MAG_FILTER,GL_NEAREST); glTexParameteri(GL_TEXTURE_2D,GL_TEXTURE_MIN_FILTER,GL_NEAREST); glTexParameteri(GL_TEXTURE_2D,GL_TEXTURE_WRAP_S,GL_CLAMP_TO_BORDER); glTexParameteri(GL_TEXTURE_2D,GL_TEXTURE_WRAP_T,GL_CLAMP_TO_BORDER); glTexParameteri(GL_TEXTURE_2D,GL_TEXTURE_COMPARE_MODE,GL_COMPARE_REF_TO_TEXTURE); glTexParameteri(GL_TEXTURE_2D,GL_TEXTURE_COMPARE_FUNC,GL_LEQUAL); glTexParameterfv(GL_TEXTURE_2D,GL_TEXTURE_BORDER_COLOR,border); glTexImage2D(GL_TEXTURE_2D,0,GL_DEPTH_COMPONENT24,SHADOWMAP_WIDTH,SHADOWMAP_HEIGHT,0,GL_DEPTH_COMPONENT,GL_UNSIGNED_BYTE,NULL);
- Set up an FBO and use the shadow map texture as a single depth attachment. This will store the scene's depth from the point of view of light.
glGenFramebuffers(1,&fboID); glBindFramebuffer(GL_FRAMEBUFFER,fboID); glFramebufferTexture2D(GL_FRAMEBUFFER,GL_DEPTH_ATTACHMENT,GL_TEXTURE_2D,shadowMapTexID,0); GLenum status = glCheckFramebufferStatus(GL_FRAMEBUFFER); if(status == GL_FRAMEBUFFER_COMPLETE) { cout<<"FBO setup successful."<<endl; } else { cout<<"Problem in FBO setup."<<endl; } glBindFramebuffer(GL_FRAMEBUFFER,0);
- Using the position and the direction of the light, set up the shadow matrix (
S
) by combining the light modelview matrix (MV_L
), projection matrix (P_L
), and bias matrix (B
). For reducing runtime calculation, we store the combined projection and bias matrix (BP
) at initialization.MV_L = glm::lookAt(lightPosOS,glm::vec3(0,0,0), glm::vec3(0,1,0)); P_L = glm::perspective(50.0f,1.0f,1.0f, 25.0f); B = glm::scale(glm::translate(glm::mat4(1), glm::vec3(0.5,0.5,0.5)),glm::vec3(0.5,0.5,0.5)); BP = B*P_L; S = BP*MV_L;
- Bind the FBO and render the scene from the point of view of the light. Make sure to enable front-face culling (
glEnable(GL_CULL_FACE)
andglCullFace(GL_FRONT)
) so that the back-face depth values are rendered. Otherwise our objects will suffer from shadow acne. - Disable FBO, restore default viewport, and render the scene normally from the point of view of the camera.
glBindFramebuffer(GL_FRAMEBUFFER,0); glViewport(0,0,WIDTH, HEIGHT); DrawScene(MV, P, 0 );
- In the vertex shader, multiply the world space vertex positions (
M*vec4(vVertex,1)
) with the shadow matrix (S
) to obtain the shadow coordinates. These will be used for lookup of the depth values from theshadowmap
texture in the fragment shader.#version 330 core layout(location=0) in vec3 vVertex; layout(location=1) in vec3 vNormal; uniform mat4 MVP; //modelview projection matrix uniform mat4 MV; //modelview matrix uniform mat4 M; //model matrix uniform mat3 N; //normal matrix uniform mat4 S; //shadow matrix smooth out vec3 vEyeSpaceNormal; smooth out vec3 vEyeSpacePosition; smooth out vec4 vShadowCoords; void main() { vEyeSpacePosition = (MV*vec4(vVertex,1)).xyz; vEyeSpaceNormal = N*vNormal; vShadowCoords = S*(M*vec4(vVertex,1)); gl_Position = MVP*vec4(vVertex,1); }
- In the fragment shader, use the shadow coordinates to lookup the depth value in the shadow map sampler which is of the
sampler2Dshadow
type. This sampler can be used with thetextureProj
function to return a comparison outcome. We then use the comparison result to darken the diffuse component, simulating shadows.#version 330 core layout(location=0) out vec4 vFragColor; uniform sampler2DShadow shadowMap; uniform vec3 light_position; //light position in eye space uniform vec3 diffuse_color; smooth in vec3 vEyeSpaceNormal; smooth in vec3 vEyeSpacePosition; smooth in vec4 vShadowCoords; const float k0 = 1.0; //constant attenuation const float k1 = 0.0; //linear attenuation const float k2 = 0.0; //quadratic attenuation uniform bool bIsLightPass; //no shadows in light pass void main() { if(bIsLightPass) return; vec3 L = (light_position.xyz-vEyeSpacePosition); float d = length(L); L = normalize(L); float attenuationAmount = 1.0/(k0 + (k1*d) + (k2*d*d)); float diffuse = max(0, dot(vEyeSpaceNormal, L)) * attenuationAmount; if(vShadowCoords.w>1) { float shadow = textureProj(shadowMap, vShadowCoords); diffuse = mix(diffuse, diffuse*shadow, 0.5); } vFragColor = diffuse*vec4(diffuse_color, 1); }
The shadow mapping algorithm works in two passes. In the first pass, the scene is rendered from the point of view of light, and the depth buffer is stored into a texture called shadowmap
. We use a single FBO with a depth attachment for this purpose. Apart from the conventional minification/magnification texture filtering, we set the texture wrapping mode to GL_CLAMP_TO_BORDER
, which ensures that the values are clamped to the specified border color. Had we set this as GL_CLAMP
or GL_CLAMP_TO_EDGE
, the border pixels forming the shadow map would produce visible artefacts.
To render the scene from the point of view of light, the modelview matrix of the light (MV_L
), the projection matrix (P_L
), and the bias matrix (B
) are calculated. After multiplying with the projection matrix, the coordinates are in clip space (that is, they range from [-1,-1,-1]). to [1,1,1]. The bias matrix rescales this range to bring the coordinates from [0,0,0] to [1,1,1] range so that the shadow lookup can be carried out.
The shadow map lookup computation is facilitated by the textureProj
GLSL function. The result from the shadow map lookup returns 1 or 0. This result is multiplied with the shading computation. As it happens in the real world, we never have coal black shadows. Therefore, we combine the shadow outcome with the shading computation by using the mix
GLSL function.
- Real-time Shadows, Elmar Eisemann, Michael Schwarz, Ulf Assarsson, Michael Wimmer, A K Peters/CRC Press
- OpenGL 4.0 Shading Language Cookbook, Chapter 7, Shadows, David Wolff, Packt Publishing
- ShadowMapping with GLSL by Fabien Sanglard: http://www.fabiensanglard.net/shadowmapping/index.php
GL_CLAMP_TO_BORDER
, set the border color to {1,0,0,0}
, give the texture comparison mode to GL_COMPARE_REF_TO_TEXTURE
, and set the compare function to GL_LEQUAL
. Set the texture internal format to GL_DEPTH_COMPONENT24
.glGenTextures(1, &shadowMapTexID); glActiveTexture(GL_TEXTURE0); glBindTexture(GL_TEXTURE_2D, shadowMapTexID); GLfloat border[4]={1,0,0,0}; glTexParameteri(GL_TEXTURE_2D,GL_TEXTURE_MAG_FILTER,GL_NEAREST); glTexParameteri(GL_TEXTURE_2D,GL_TEXTURE_MIN_FILTER,GL_NEAREST); glTexParameteri(GL_TEXTURE_2D,GL_TEXTURE_WRAP_S,GL_CLAMP_TO_BORDER); glTexParameteri(GL_TEXTURE_2D,GL_TEXTURE_WRAP_T,GL_CLAMP_TO_BORDER); glTexParameteri(GL_TEXTURE_2D,GL_TEXTURE_COMPARE_MODE,GL_COMPARE_REF_TO_TEXTURE); glTexParameteri(GL_TEXTURE_2D,GL_TEXTURE_COMPARE_FUNC,GL_LEQUAL); glTexParameterfv(GL_TEXTURE_2D,GL_TEXTURE_BORDER_COLOR,border); glTexImage2D(GL_TEXTURE_2D,0,GL_DEPTH_COMPONENT24,SHADOWMAP_WIDTH,SHADOWMAP_HEIGHT,0,GL_DEPTH_COMPONENT,GL_UNSIGNED_BYTE,NULL);
glGenFramebuffers(1,&fboID);
glBindFramebuffer(GL_FRAMEBUFFER,fboID);
glFramebufferTexture2D(GL_FRAMEBUFFER,GL_DEPTH_ATTACHMENT,GL_TEXTURE_2D,shadowMapTexID,0);
GLenum status = glCheckFramebufferStatus(GL_FRAMEBUFFER);
if(status == GL_FRAMEBUFFER_COMPLETE) {
cout<<"FBO setup successful."<<endl;
} else {
cout<<"Problem in FBO setup."<<endl;
}
glBindFramebuffer(GL_FRAMEBUFFER,0);
- position and the direction of the light, set up the shadow matrix (
S
) by combining the light modelview matrix (MV_L
), projection matrix (P_L
), and bias matrix (B
). For reducing runtime calculation, we store the combined projection and bias matrix (BP
) at initialization.MV_L = glm::lookAt(lightPosOS,glm::vec3(0,0,0), glm::vec3(0,1,0)); P_L = glm::perspective(50.0f,1.0f,1.0f, 25.0f); B = glm::scale(glm::translate(glm::mat4(1), glm::vec3(0.5,0.5,0.5)),glm::vec3(0.5,0.5,0.5)); BP = B*P_L; S = BP*MV_L;
- Bind the FBO and render the scene from the point of view of the light. Make sure to enable front-face culling (
glEnable(GL_CULL_FACE)
andglCullFace(GL_FRONT)
) so that the back-face depth values are rendered. Otherwise our objects will suffer from shadow acne. - Disable FBO, restore default viewport, and render the scene normally from the point of view of the camera.
glBindFramebuffer(GL_FRAMEBUFFER,0); glViewport(0,0,WIDTH, HEIGHT); DrawScene(MV, P, 0 );
- In the vertex shader, multiply the world space vertex positions (
M*vec4(vVertex,1)
) with the shadow matrix (S
) to obtain the shadow coordinates. These will be used for lookup of the depth values from theshadowmap
texture in the fragment shader.#version 330 core layout(location=0) in vec3 vVertex; layout(location=1) in vec3 vNormal; uniform mat4 MVP; //modelview projection matrix uniform mat4 MV; //modelview matrix uniform mat4 M; //model matrix uniform mat3 N; //normal matrix uniform mat4 S; //shadow matrix smooth out vec3 vEyeSpaceNormal; smooth out vec3 vEyeSpacePosition; smooth out vec4 vShadowCoords; void main() { vEyeSpacePosition = (MV*vec4(vVertex,1)).xyz; vEyeSpaceNormal = N*vNormal; vShadowCoords = S*(M*vec4(vVertex,1)); gl_Position = MVP*vec4(vVertex,1); }
- In the fragment shader, use the shadow coordinates to lookup the depth value in the shadow map sampler which is of the
sampler2Dshadow
type. This sampler can be used with thetextureProj
function to return a comparison outcome. We then use the comparison result to darken the diffuse component, simulating shadows.#version 330 core layout(location=0) out vec4 vFragColor; uniform sampler2DShadow shadowMap; uniform vec3 light_position; //light position in eye space uniform vec3 diffuse_color; smooth in vec3 vEyeSpaceNormal; smooth in vec3 vEyeSpacePosition; smooth in vec4 vShadowCoords; const float k0 = 1.0; //constant attenuation const float k1 = 0.0; //linear attenuation const float k2 = 0.0; //quadratic attenuation uniform bool bIsLightPass; //no shadows in light pass void main() { if(bIsLightPass) return; vec3 L = (light_position.xyz-vEyeSpacePosition); float d = length(L); L = normalize(L); float attenuationAmount = 1.0/(k0 + (k1*d) + (k2*d*d)); float diffuse = max(0, dot(vEyeSpaceNormal, L)) * attenuationAmount; if(vShadowCoords.w>1) { float shadow = textureProj(shadowMap, vShadowCoords); diffuse = mix(diffuse, diffuse*shadow, 0.5); } vFragColor = diffuse*vec4(diffuse_color, 1); }
The shadow mapping algorithm works in two passes. In the first pass, the scene is rendered from the point of view of light, and the depth buffer is stored into a texture called shadowmap
. We use a single FBO with a depth attachment for this purpose. Apart from the conventional minification/magnification texture filtering, we set the texture wrapping mode to GL_CLAMP_TO_BORDER
, which ensures that the values are clamped to the specified border color. Had we set this as GL_CLAMP
or GL_CLAMP_TO_EDGE
, the border pixels forming the shadow map would produce visible artefacts.
To render the scene from the point of view of light, the modelview matrix of the light (MV_L
), the projection matrix (P_L
), and the bias matrix (B
) are calculated. After multiplying with the projection matrix, the coordinates are in clip space (that is, they range from [-1,-1,-1]). to [1,1,1]. The bias matrix rescales this range to bring the coordinates from [0,0,0] to [1,1,1] range so that the shadow lookup can be carried out.
The shadow map lookup computation is facilitated by the textureProj
GLSL function. The result from the shadow map lookup returns 1 or 0. This result is multiplied with the shading computation. As it happens in the real world, we never have coal black shadows. Therefore, we combine the shadow outcome with the shading computation by using the mix
GLSL function.
- Real-time Shadows, Elmar Eisemann, Michael Schwarz, Ulf Assarsson, Michael Wimmer, A K Peters/CRC Press
- OpenGL 4.0 Shading Language Cookbook, Chapter 7, Shadows, David Wolff, Packt Publishing
- ShadowMapping with GLSL by Fabien Sanglard: http://www.fabiensanglard.net/shadowmapping/index.php
mapping algorithm works in two passes. In the first pass, the scene is rendered from the point of view of light, and the depth buffer is stored into a texture called shadowmap
. We use a single FBO with a depth attachment for this purpose. Apart from the conventional minification/magnification texture filtering, we set the texture wrapping mode to GL_CLAMP_TO_BORDER
, which ensures that the values are clamped to the specified border color. Had we set this as GL_CLAMP
or GL_CLAMP_TO_EDGE
, the border pixels forming the shadow map would produce visible artefacts.
To render the scene from the point of view of light, the modelview matrix of the light (MV_L
), the projection matrix (P_L
), and the bias matrix (B
) are calculated. After multiplying with the projection matrix, the coordinates are in clip space (that is, they range from [-1,-1,-1]). to [1,1,1]. The bias matrix rescales this range to bring the coordinates from [0,0,0] to [1,1,1] range so that the shadow lookup can be carried out.
The shadow map lookup computation is facilitated by the textureProj
GLSL function. The result from the shadow map lookup returns 1 or 0. This result is multiplied with the shading computation. As it happens in the real world, we never have coal black shadows. Therefore, we combine the shadow outcome with the shading computation by using the mix
GLSL function.
- Real-time Shadows, Elmar Eisemann, Michael Schwarz, Ulf Assarsson, Michael Wimmer, A K Peters/CRC Press
- OpenGL 4.0 Shading Language Cookbook, Chapter 7, Shadows, David Wolff, Packt Publishing
- ShadowMapping with GLSL by Fabien Sanglard: http://www.fabiensanglard.net/shadowmapping/index.php
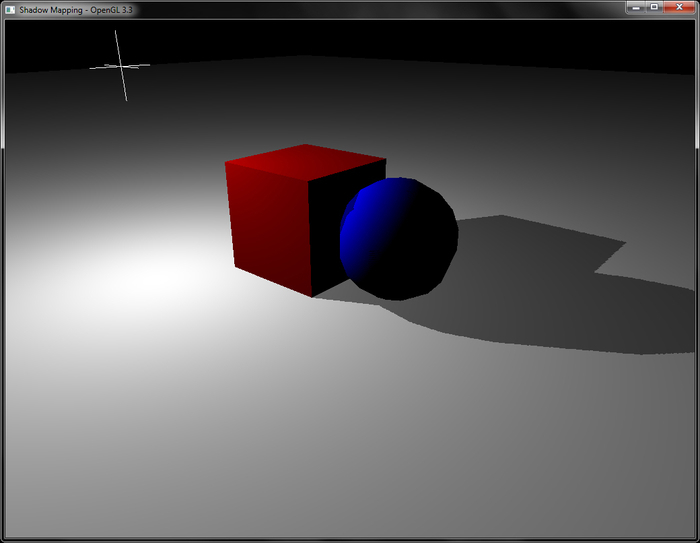
- Real-time Shadows, Elmar Eisemann, Michael Schwarz, Ulf Assarsson, Michael Wimmer, A K Peters/CRC Press
- OpenGL 4.0 Shading Language Cookbook, Chapter 7, Shadows, David Wolff, Packt Publishing
- ShadowMapping with GLSL by Fabien Sanglard: http://www.fabiensanglard.net/shadowmapping/index.php
- Chapter 7, Shadows, David Wolff, Packt Publishing
- ShadowMapping with GLSL by Fabien Sanglard: http://www.fabiensanglard.net/shadowmapping/index.php
The shadow mapping algorithm, though simple to implement, suffers from aliasing artefacts, which are due to the shadowmap
resolution. In addition, the shadows produced using this approach are hard. These can be minimized either by increasing the shadowmap
resolution or taking more samples. The latter approach is called percentage closer filtering (PCF), where more samples are taken for the shadowmap
lookup and the percentage of the samples is used to estimate if a fragment is in shadow. Thus, in PCF, instead of a single lookup, we sample an n×n neighborhood of shadowmap
and then average the values.
The code for this recipe is contained in the Chapter4/ShadowMappingPCF
directory. It builds on top of the previous recipe, Implementing shadow mapping with FBO. We use the same scene but augment it with PCF.
Let us see how to extend the basic shadow mapping with PCF.
- Change the
shadowmap
texture minification/magnification filtering modes toGL_LINEAR
. Here, we exploit the texture filtering capabilities of the GPU to reduce aliasing artefacts during sampling of the shadow map. Even with the linear filtering support, we have to take additional samples to reduce the artefacts.glTexParameteri(GL_TEXTURE_2D,GL_TEXTURE_MAG_FILTER,GL_LINEAR); glTexParameteri(GL_TEXTURE_2D,GL_TEXTURE_MIN_FILTER,GL_LINEAR);
- In the fragment shader, instead of a single texture lookup as in the shadow map recipe, we use a number of samples. GLSL provides a convenient function,
textureProjOffset
, to allow calculation of samples using an offset. For this recipe, we look at a 3×3 neighborhood around the current shadow map point. Hence, we use a large offset of 2. This helps to reduce sampling artefacts.if(vShadowCoords.w>1) { float sum = 0; sum += textureProjOffset(shadowMap,vShadowCoords,ivec2(-2,-2)); sum += textureProjOffset(shadowMap,vShadowCoords,ivec2(-2, 0)); sum += textureProjOffset(shadowMap,vShadowCoords,ivec2(-2, 2)); sum += textureProjOffset(shadowMap,vShadowCoords,ivec2( 0,-2)); sum += textureProjOffset(shadowMap,vShadowCoords,ivec2( 0, 0)); sum += textureProjOffset(shadowMap,vShadowCoords,ivec2( 0, 2)); sum += textureProjOffset(shadowMap,vShadowCoords,ivec2( 2,-2)); sum += textureProjOffset(shadowMap,vShadowCoords,ivec2( 2, 0)); sum += textureProjOffset(shadowMap,vShadowCoords,ivec2( 2, 2)); float shadow = sum/9.0; diffuse = mix(diffuse, diffuse*shadow, 0.5); }
In order to implement PCF, the first change we need is to set the texture filtering mode to linear filtering. This change enabled the GPU to bilinearly interpolate the shadow value. This gives smoother edges since the hardware does PCF filtering underneath. However it is not enough for our purpose. Therefore, we have to take additional samples to improve the result.
Then, the sampling for PCF uses the noise function to shift the shadow offset, as shown in the following shader code:
for this recipe is contained in the Chapter4/ShadowMappingPCF
directory. It builds on top of the previous recipe, Implementing shadow mapping with FBO. We use the same scene but augment it with PCF.
Let us see how to extend the basic shadow mapping with PCF.
- Change the
shadowmap
texture minification/magnification filtering modes toGL_LINEAR
. Here, we exploit the texture filtering capabilities of the GPU to reduce aliasing artefacts during sampling of the shadow map. Even with the linear filtering support, we have to take additional samples to reduce the artefacts.glTexParameteri(GL_TEXTURE_2D,GL_TEXTURE_MAG_FILTER,GL_LINEAR); glTexParameteri(GL_TEXTURE_2D,GL_TEXTURE_MIN_FILTER,GL_LINEAR);
- In the fragment shader, instead of a single texture lookup as in the shadow map recipe, we use a number of samples. GLSL provides a convenient function,
textureProjOffset
, to allow calculation of samples using an offset. For this recipe, we look at a 3×3 neighborhood around the current shadow map point. Hence, we use a large offset of 2. This helps to reduce sampling artefacts.if(vShadowCoords.w>1) { float sum = 0; sum += textureProjOffset(shadowMap,vShadowCoords,ivec2(-2,-2)); sum += textureProjOffset(shadowMap,vShadowCoords,ivec2(-2, 0)); sum += textureProjOffset(shadowMap,vShadowCoords,ivec2(-2, 2)); sum += textureProjOffset(shadowMap,vShadowCoords,ivec2( 0,-2)); sum += textureProjOffset(shadowMap,vShadowCoords,ivec2( 0, 0)); sum += textureProjOffset(shadowMap,vShadowCoords,ivec2( 0, 2)); sum += textureProjOffset(shadowMap,vShadowCoords,ivec2( 2,-2)); sum += textureProjOffset(shadowMap,vShadowCoords,ivec2( 2, 0)); sum += textureProjOffset(shadowMap,vShadowCoords,ivec2( 2, 2)); float shadow = sum/9.0; diffuse = mix(diffuse, diffuse*shadow, 0.5); }
In order to implement PCF, the first change we need is to set the texture filtering mode to linear filtering. This change enabled the GPU to bilinearly interpolate the shadow value. This gives smoother edges since the hardware does PCF filtering underneath. However it is not enough for our purpose. Therefore, we have to take additional samples to improve the result.
Then, the sampling for PCF uses the noise function to shift the shadow offset, as shown in the following shader code:
shadowmap
texture minification/magnification filtering modes to GL_LINEAR
. Here, we exploit the texture filtering capabilities of the GPU to reduce aliasing artefacts during sampling of the shadow map. Even with the linear filtering support, we have to take additional samples to reduce the artefacts.glTexParameteri(GL_TEXTURE_2D,GL_TEXTURE_MAG_FILTER,GL_LINEAR); glTexParameteri(GL_TEXTURE_2D,GL_TEXTURE_MIN_FILTER,GL_LINEAR);
textureProjOffset
, to allow calculation of samples using an offset. For this recipe, we look at a 3×3 neighborhood around the current shadow map point. Hence, we use a large offset of 2. This helps to reduce sampling artefacts.if(vShadowCoords.w>1) { float sum = 0; sum += textureProjOffset(shadowMap,vShadowCoords,ivec2(-2,-2)); sum += textureProjOffset(shadowMap,vShadowCoords,ivec2(-2, 0)); sum += textureProjOffset(shadowMap,vShadowCoords,ivec2(-2, 2)); sum += textureProjOffset(shadowMap,vShadowCoords,ivec2( 0,-2)); sum += textureProjOffset(shadowMap,vShadowCoords,ivec2( 0, 0)); sum += textureProjOffset(shadowMap,vShadowCoords,ivec2( 0, 2)); sum += textureProjOffset(shadowMap,vShadowCoords,ivec2( 2,-2)); sum += textureProjOffset(shadowMap,vShadowCoords,ivec2( 2, 0)); sum += textureProjOffset(shadowMap,vShadowCoords,ivec2( 2, 2)); float shadow = sum/9.0; diffuse = mix(diffuse, diffuse*shadow, 0.5); }
In order to implement PCF, the first change we need is to set the texture filtering mode to linear filtering. This change enabled the GPU to bilinearly interpolate the shadow value. This gives smoother edges since the hardware does PCF filtering underneath. However it is not enough for our purpose. Therefore, we have to take additional samples to improve the result.
Then, the sampling for PCF uses the noise function to shift the shadow offset, as shown in the following shader code:
implement PCF, the first change we need is to set the texture filtering mode to linear filtering. This change enabled the GPU to bilinearly interpolate the shadow value. This gives smoother edges since the hardware does PCF filtering underneath. However it is not enough for our purpose. Therefore, we have to take additional samples to improve the result.
Then, the sampling for PCF uses the noise function to shift the shadow offset, as shown in the following shader code:
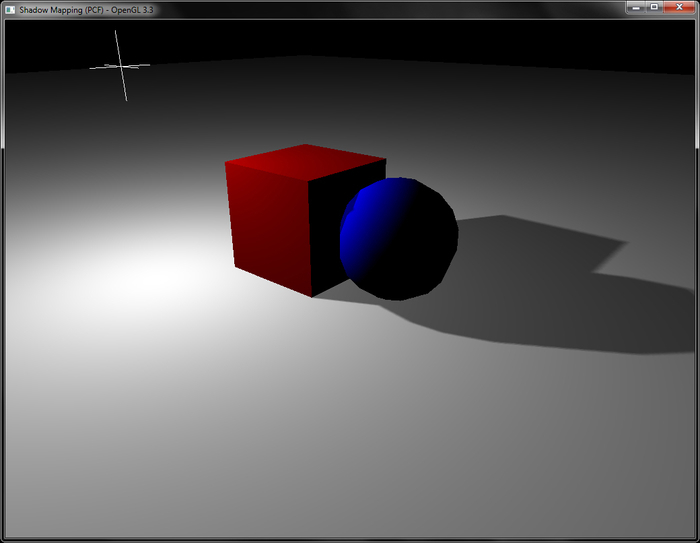
In this recipe, we will cover a technique which gives a much better result, has better performance, and at the same time is easier to calculate. The technique is called variance shadow mapping. In conventional PCF-filtered shadow mapping, we compare the depth value of the current fragment to the mean depth value in the shadow map, and based on the outcome, we shadow the fragment.
For this recipe, we will build on top of the shadow mapping recipe, Implementing shadow mapping with FBO. The code for this recipe is contained in the Chapter4/VarianceShadowMapping
folder.
Let us start our recipe by following these simple steps:
- Set up the
shadowmap
texture as in the shadow map recipe, but this time remove the depth compare mode (glTexParameteri(GL_TEXTURE_2D,GL_TEXTURE_COMPARE_MODE,GL_COMPARE_REF_TO_TEXTURE)
and glTexParameteri(GL_TEXTURE_2D,GL_TEXTURE_COMPARE_FUNC,GL_LEQUAL)
).
Also set the format of the texture to theGL_RGBA32F
format. Also enable the mipmap generation for this texture. The mipmaps provide filtered textures across different scales and produces better alias-free shadows. We request five mipmap levels (by specifying the max level as 4).glGenTextures(1, &shadowMapTexID); glActiveTexture(GL_TEXTURE0); glBindTexture(GL_TEXTURE_2D, shadowMapTexID); glTexParameteri(GL_TEXTURE_2D,GL_TEXTURE_MAG_FILTER,GL_LINEAR; glTexParameteri(GL_TEXTURE_2D,GL_TEXTURE_MIN_FILTER,GL_LINEAR_MIPMAP_LINEAR); glTexParameteri(GL_TEXTURE_2D,GL_TEXTURE_WRAP_S,GL_CLAMP_TO_BORDER); glTexParameteri(GL_TEXTURE_2D,GL_TEXTURE_WRAP_T,GL_CLAMP_TO_BORDER); glTexParameterfv(GL_TEXTURE_2D,GL_TEXTURE_BORDER_COLOR,border; glTexImage2D(GL_TEXTURE_2D,0,GL_RGBA32F,SHADOWMAP_WIDTH,SHADOWMAP_HEIGHT,0,GL_RGBA,GL_FLOAT,NULL); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_BASE_LEVEL, 0); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MAX_LEVEL, 4); glGenerateMipmap(GL_TEXTURE_2D);
- Set up two FBOs: one for shadowmap generation and another for shadowmap filtering. The shadowmap FBO has a
renderbuffer
attached to it for depth testing. The filtering FBO does not have arenderbuffer
attached to it but it has two texture attachments.glGenFramebuffers(1,&fboID); glGenRenderbuffers(1, &rboID); glBindFramebuffer(GL_FRAMEBUFFER,fboID); glBindRenderbuffer(GL_RENDERBUFFER, rboID); glRenderbufferStorage(GL_RENDERBUFFER, GL_DEPTH_COMPONENT32, SHADOWMAP_WIDTH, SHADOWMAP_HEIGHT); glFramebufferTexture2D(GL_FRAMEBUFFER,GL_COLOR_ATTACHMENT0,GL_TEXTURE_2D,shadowMapTexID,0); glFramebufferRenderbuffer(GL_FRAMEBUFFER, GL_DEPTH_ATTACHMENT, GL_RENDERBUFFER, rboID); GLenum status = glCheckFramebufferStatus(GL_FRAMEBUFFER); if(status == GL_FRAMEBUFFER_COMPLETE) { cout<<"FBO setup successful."<<endl; } else { cout<<"Problem in FBO setup."<<endl; } glBindFramebuffer(GL_FRAMEBUFFER,0); glGenFramebuffers(1,&filterFBOID); glBindFramebuffer(GL_FRAMEBUFFER,filterFBOID); glGenTextures(2, blurTexID); for(int i=0;i<2;i++) { glActiveTexture(GL_TEXTURE1+i); glBindTexture(GL_TEXTURE_2D, blurTexID[i]); glTexParameteri(GL_TEXTURE_2D,GL_TEXTURE_MAG_FILTER,GL_LINEAR); glTexParameteri(GL_TEXTURE_2D,GL_TEXTURE_MIN_FILTER,GL_LINEAR); glTexParameteri(GL_TEXTURE_2D,GL_TEXTURE_WRAP_S,GL_CLAMP_TO_BORDER); glTexParameteri(GL_TEXTURE_2D,GL_TEXTURE_WRAP_T,GL_CLAMP_TO_BORDER); glTexParameterfv(GL_TEXTURE_2D,GL_TEXTURE_BORDER_COLOR,border); glTexImage2D(GL_TEXTURE_2D,0,GL_RGBA32F,SHADOWMAP_WIDTH,SHADOWMAP_HEIGHT,0,GL_RGBA,GL_FLOAT,NULL); glFramebufferTexture2D(GL_FRAMEBUFFER,GL_COLOR_ATTACHMENT0+i, GL_TEXTURE_2D,blurTexID[i],0); } status = glCheckFramebufferStatus(GL_FRAMEBUFFER); if(status == GL_FRAMEBUFFER_COMPLETE) { cout<<"Filtering FBO setup successful."<<endl; } else { cout<<"Problem in Filtering FBO setup."<<endl; } glBindFramebuffer(GL_FRAMEBUFFER,0);
- Bind the
shadowmap
FBO, set the viewport to the size of theshadowmap
texture, and render the scene from the point of view of the light, as in the Implementing shadow mapping with FBO recipe. In this pass, instead of storing the depth as in the shadow mapping recipe, we use a custom fragment shader (Chapter4/VarianceShadowmapping/shaders/firststep.frag
) to output the depth and depth*depth values in the red and green channels of the fragment output color.glBindFramebuffer(GL_FRAMEBUFFER,fboID); glViewport(0,0,SHADOWMAP_WIDTH, SHADOWMAP_HEIGHT); glDrawBuffer(GL_COLOR_ATTACHMENT0); glClear(GL_COLOR_BUFFER_BIT|GL_DEPTH_BUFFER_BIT); DrawSceneFirstPass(MV_L, P_L);
The shader code is as follows:
#version 330 core layout(location=0) out vec4 vFragColor; smooth in vec4 clipSpacePos; void main() { vec3 pos = clipSpacePos.xyz/clipSpacePos.w; //-1 to 1 pos.z += 0.001; //add some offset to remove the shadow acne float depth = (pos.z +1)*0.5; // 0 to 1 float moment1 = depth; float moment2 = depth * depth; vFragColor = vec4(moment1,moment2,0,0); }
- Bind the filtering FBO to filter the
shadowmap
texture generated in the first pass using separable Gaussian smoothing filters, which are more efficient and offer better performance. We first attach the vertical smoothing fragment shader (Chapter4/VarianceShadowmapping/shaders/GaussV.frag
) to filter theshadowmap
texture and then the horizontal smoothing fragment shader (Chapter4/VarianceShadowmapping/shaders/GaussH.frag
) to smooth the output from the vertical Gaussian smoothing filter.glBindFramebuffer(GL_FRAMEBUFFER,filterFBOID); glDrawBuffer(GL_COLOR_ATTACHMENT0); glBindVertexArray(quadVAOID); gaussianV_shader.Use(); glDrawElements(GL_TRIANGLES, 6, GL_UNSIGNED_SHORT, 0); glDrawBuffer(GL_COLOR_ATTACHMENT1); gaussianH_shader.Use(); glDrawElements(GL_TRIANGLES, 6, GL_UNSIGNED_SHORT, 0); glBindFramebuffer(GL_FRAMEBUFFER,0);
The horizontal Gaussian blur shader is as follows:
#version 330 core layout(location=0) out vec4 vFragColor; smooth in vec2 vUV; uniform sampler2D textureMap; const float kernel[]=float[21] (0.000272337, 0.00089296, 0.002583865, 0.00659813, 0.014869116, 0.029570767, 0.051898313, 0.080381679, 0.109868729, 0.132526984, 0.14107424, 0.132526984, 0.109868729, 0.080381679, 0.051898313, 0.029570767, 0.014869116, 0.00659813, 0.002583865, 0.00089296, 0.000272337); void main() { vec2 delta = 1.0/textureSize(textureMap,0); vec4 color = vec4(0); int index = 20; for(int i=-10;i<=10;i++) { color += kernel[index--]*texture(textureMap, vUV + (vec2(i*delta.x,0))); } vFragColor = vec4(color.xy,0,0); }
In the vertical Gaussian shader, the loop statement is modified, whereas the rest of the shader is the same.
color += kernel[index--]*texture(textureMap, vUV + (vec2(0,i*delta.y)));
- Unbind the FBO, reset the default viewport, and then render the scene normally, as in the shadow mapping recipe.
glDrawBuffer(GL_BACK_LEFT); glViewport(0,0,WIDTH, HEIGHT); DrawScene(MV, P);
The variance shadowmap technique tries to represent the depth data such that it can be filtered linearly. Instead of storing the depth, it stores the depth
and depth*depth
value in a floating point texture, which is then filtered to reconstruct the first and second moments of the depth distribution. Using the moments, it estimates the variance in the filtering neighborhood. This helps in finding the probability of a fragment at a specific depth to be occluded using Chebyshev's inequality. For more mathematical details, we refer the reader to the See also section of this recipe.
After the first pass, the shadowmap
texture is blurred using a separable Gaussian smoothing filter. First the vertical and then the horizontal filter is applied to the shadowmap
texture by applying the shadowmap
texture to a full-screen quad and alternating the filter FBO's color attachment. Note that the shadowmap
texture is bound to texture unit 0 whereas the textures used for filtering are bound to texture unit 1 (attached to GL_COLOR_ATTTACHMENT0
on the filtering FBO) and texture unit 2 (attached to GL_COLOR_ATTACHMENT1
on the filtering FBO).
In the second pass, the scene is rendered from the point of view of the camera. The blurred shadowmap is used in the second pass as a texture to lookup the sample value (see Chapter4/VarianceShadowmapping/shaders/VarianceShadowMap.{vert, frag}
). The variance shadow mapping vertex shader outputs the shadow texture coordinates, as in the shadow mapping recipe.
The texture coordinates after homogeneous division are used to lookup the shadow map storing the two moments. The two moments are used to estimate the variance. The variance is clamped and then the occlusion probability is estimated. The diffuse component is then modulated based on the obtained occlusion probability.
To recap, here is the complete variance shadow mapping fragment shader:
Variance shadow mapping is an interesting idea. However, it does suffer from light bleeding artefacts. There have been several improvements to the basic technique, such as summed area variance shadow maps, layered variance shadow maps, and more recently, sample distribution shadow maps, that are referred to in the See also section of this recipe. After getting a practical insight into the basic variance shadow mapping idea, we invite the reader to try and implement the different variants of this algorithm, as detailed in the references in the See also section.
- Proceedings of the 2006 symposium on Interactive 3D graphics and games, Variance Shadow Maps, pages 161-165 William Donnelly, Andrew Lauritzen
- GPU Gems 3, Chapter 8, Summed-Area Variance Shadow Maps, Andrew Lauritzen: http://http.developer.nvidia.com/GPUGems3/gpugems3_ch08.html
- Proceedings of the Graphics Interface 2008, Layered variance shadow maps, pages 139-146, Andrew Lauritzen, Michael McCool
- Sample Distribution Shadow Maps, ACM SIGGRAPH Symposium on Interactive 3D Graphics and Games (I3D) 2011, February, Andrew Lauritzen, Marco Salvi, and Aaron Lefohn
Let us start our recipe by following these simple steps:
- Set up the
shadowmap
texture as in the shadow map recipe, but this time remove the depth compare mode (glTexParameteri(GL_TEXTURE_2D,GL_TEXTURE_COMPARE_MODE,GL_COMPARE_REF_TO_TEXTURE)
and glTexParameteri(GL_TEXTURE_2D,GL_TEXTURE_COMPARE_FUNC,GL_LEQUAL)
).
Also set the format of the texture to theGL_RGBA32F
format. Also enable the mipmap generation for this texture. The mipmaps provide filtered textures across different scales and produces better alias-free shadows. We request five mipmap levels (by specifying the max level as 4).glGenTextures(1, &shadowMapTexID); glActiveTexture(GL_TEXTURE0); glBindTexture(GL_TEXTURE_2D, shadowMapTexID); glTexParameteri(GL_TEXTURE_2D,GL_TEXTURE_MAG_FILTER,GL_LINEAR; glTexParameteri(GL_TEXTURE_2D,GL_TEXTURE_MIN_FILTER,GL_LINEAR_MIPMAP_LINEAR); glTexParameteri(GL_TEXTURE_2D,GL_TEXTURE_WRAP_S,GL_CLAMP_TO_BORDER); glTexParameteri(GL_TEXTURE_2D,GL_TEXTURE_WRAP_T,GL_CLAMP_TO_BORDER); glTexParameterfv(GL_TEXTURE_2D,GL_TEXTURE_BORDER_COLOR,border; glTexImage2D(GL_TEXTURE_2D,0,GL_RGBA32F,SHADOWMAP_WIDTH,SHADOWMAP_HEIGHT,0,GL_RGBA,GL_FLOAT,NULL); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_BASE_LEVEL, 0); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MAX_LEVEL, 4); glGenerateMipmap(GL_TEXTURE_2D);
- Set up two FBOs: one for shadowmap generation and another for shadowmap filtering. The shadowmap FBO has a
renderbuffer
attached to it for depth testing. The filtering FBO does not have arenderbuffer
attached to it but it has two texture attachments.glGenFramebuffers(1,&fboID); glGenRenderbuffers(1, &rboID); glBindFramebuffer(GL_FRAMEBUFFER,fboID); glBindRenderbuffer(GL_RENDERBUFFER, rboID); glRenderbufferStorage(GL_RENDERBUFFER, GL_DEPTH_COMPONENT32, SHADOWMAP_WIDTH, SHADOWMAP_HEIGHT); glFramebufferTexture2D(GL_FRAMEBUFFER,GL_COLOR_ATTACHMENT0,GL_TEXTURE_2D,shadowMapTexID,0); glFramebufferRenderbuffer(GL_FRAMEBUFFER, GL_DEPTH_ATTACHMENT, GL_RENDERBUFFER, rboID); GLenum status = glCheckFramebufferStatus(GL_FRAMEBUFFER); if(status == GL_FRAMEBUFFER_COMPLETE) { cout<<"FBO setup successful."<<endl; } else { cout<<"Problem in FBO setup."<<endl; } glBindFramebuffer(GL_FRAMEBUFFER,0); glGenFramebuffers(1,&filterFBOID); glBindFramebuffer(GL_FRAMEBUFFER,filterFBOID); glGenTextures(2, blurTexID); for(int i=0;i<2;i++) { glActiveTexture(GL_TEXTURE1+i); glBindTexture(GL_TEXTURE_2D, blurTexID[i]); glTexParameteri(GL_TEXTURE_2D,GL_TEXTURE_MAG_FILTER,GL_LINEAR); glTexParameteri(GL_TEXTURE_2D,GL_TEXTURE_MIN_FILTER,GL_LINEAR); glTexParameteri(GL_TEXTURE_2D,GL_TEXTURE_WRAP_S,GL_CLAMP_TO_BORDER); glTexParameteri(GL_TEXTURE_2D,GL_TEXTURE_WRAP_T,GL_CLAMP_TO_BORDER); glTexParameterfv(GL_TEXTURE_2D,GL_TEXTURE_BORDER_COLOR,border); glTexImage2D(GL_TEXTURE_2D,0,GL_RGBA32F,SHADOWMAP_WIDTH,SHADOWMAP_HEIGHT,0,GL_RGBA,GL_FLOAT,NULL); glFramebufferTexture2D(GL_FRAMEBUFFER,GL_COLOR_ATTACHMENT0+i, GL_TEXTURE_2D,blurTexID[i],0); } status = glCheckFramebufferStatus(GL_FRAMEBUFFER); if(status == GL_FRAMEBUFFER_COMPLETE) { cout<<"Filtering FBO setup successful."<<endl; } else { cout<<"Problem in Filtering FBO setup."<<endl; } glBindFramebuffer(GL_FRAMEBUFFER,0);
- Bind the
shadowmap
FBO, set the viewport to the size of theshadowmap
texture, and render the scene from the point of view of the light, as in the Implementing shadow mapping with FBO recipe. In this pass, instead of storing the depth as in the shadow mapping recipe, we use a custom fragment shader (Chapter4/VarianceShadowmapping/shaders/firststep.frag
) to output the depth and depth*depth values in the red and green channels of the fragment output color.glBindFramebuffer(GL_FRAMEBUFFER,fboID); glViewport(0,0,SHADOWMAP_WIDTH, SHADOWMAP_HEIGHT); glDrawBuffer(GL_COLOR_ATTACHMENT0); glClear(GL_COLOR_BUFFER_BIT|GL_DEPTH_BUFFER_BIT); DrawSceneFirstPass(MV_L, P_L);
The shader code is as follows:
#version 330 core layout(location=0) out vec4 vFragColor; smooth in vec4 clipSpacePos; void main() { vec3 pos = clipSpacePos.xyz/clipSpacePos.w; //-1 to 1 pos.z += 0.001; //add some offset to remove the shadow acne float depth = (pos.z +1)*0.5; // 0 to 1 float moment1 = depth; float moment2 = depth * depth; vFragColor = vec4(moment1,moment2,0,0); }
- Bind the filtering FBO to filter the
shadowmap
texture generated in the first pass using separable Gaussian smoothing filters, which are more efficient and offer better performance. We first attach the vertical smoothing fragment shader (Chapter4/VarianceShadowmapping/shaders/GaussV.frag
) to filter theshadowmap
texture and then the horizontal smoothing fragment shader (Chapter4/VarianceShadowmapping/shaders/GaussH.frag
) to smooth the output from the vertical Gaussian smoothing filter.glBindFramebuffer(GL_FRAMEBUFFER,filterFBOID); glDrawBuffer(GL_COLOR_ATTACHMENT0); glBindVertexArray(quadVAOID); gaussianV_shader.Use(); glDrawElements(GL_TRIANGLES, 6, GL_UNSIGNED_SHORT, 0); glDrawBuffer(GL_COLOR_ATTACHMENT1); gaussianH_shader.Use(); glDrawElements(GL_TRIANGLES, 6, GL_UNSIGNED_SHORT, 0); glBindFramebuffer(GL_FRAMEBUFFER,0);
The horizontal Gaussian blur shader is as follows:
#version 330 core layout(location=0) out vec4 vFragColor; smooth in vec2 vUV; uniform sampler2D textureMap; const float kernel[]=float[21] (0.000272337, 0.00089296, 0.002583865, 0.00659813, 0.014869116, 0.029570767, 0.051898313, 0.080381679, 0.109868729, 0.132526984, 0.14107424, 0.132526984, 0.109868729, 0.080381679, 0.051898313, 0.029570767, 0.014869116, 0.00659813, 0.002583865, 0.00089296, 0.000272337); void main() { vec2 delta = 1.0/textureSize(textureMap,0); vec4 color = vec4(0); int index = 20; for(int i=-10;i<=10;i++) { color += kernel[index--]*texture(textureMap, vUV + (vec2(i*delta.x,0))); } vFragColor = vec4(color.xy,0,0); }
In the vertical Gaussian shader, the loop statement is modified, whereas the rest of the shader is the same.
color += kernel[index--]*texture(textureMap, vUV + (vec2(0,i*delta.y)));
- Unbind the FBO, reset the default viewport, and then render the scene normally, as in the shadow mapping recipe.
glDrawBuffer(GL_BACK_LEFT); glViewport(0,0,WIDTH, HEIGHT); DrawScene(MV, P);
The variance shadowmap technique tries to represent the depth data such that it can be filtered linearly. Instead of storing the depth, it stores the depth
and depth*depth
value in a floating point texture, which is then filtered to reconstruct the first and second moments of the depth distribution. Using the moments, it estimates the variance in the filtering neighborhood. This helps in finding the probability of a fragment at a specific depth to be occluded using Chebyshev's inequality. For more mathematical details, we refer the reader to the See also section of this recipe.
After the first pass, the shadowmap
texture is blurred using a separable Gaussian smoothing filter. First the vertical and then the horizontal filter is applied to the shadowmap
texture by applying the shadowmap
texture to a full-screen quad and alternating the filter FBO's color attachment. Note that the shadowmap
texture is bound to texture unit 0 whereas the textures used for filtering are bound to texture unit 1 (attached to GL_COLOR_ATTTACHMENT0
on the filtering FBO) and texture unit 2 (attached to GL_COLOR_ATTACHMENT1
on the filtering FBO).
In the second pass, the scene is rendered from the point of view of the camera. The blurred shadowmap is used in the second pass as a texture to lookup the sample value (see Chapter4/VarianceShadowmapping/shaders/VarianceShadowMap.{vert, frag}
). The variance shadow mapping vertex shader outputs the shadow texture coordinates, as in the shadow mapping recipe.
The texture coordinates after homogeneous division are used to lookup the shadow map storing the two moments. The two moments are used to estimate the variance. The variance is clamped and then the occlusion probability is estimated. The diffuse component is then modulated based on the obtained occlusion probability.
To recap, here is the complete variance shadow mapping fragment shader:
Variance shadow mapping is an interesting idea. However, it does suffer from light bleeding artefacts. There have been several improvements to the basic technique, such as summed area variance shadow maps, layered variance shadow maps, and more recently, sample distribution shadow maps, that are referred to in the See also section of this recipe. After getting a practical insight into the basic variance shadow mapping idea, we invite the reader to try and implement the different variants of this algorithm, as detailed in the references in the See also section.
- Proceedings of the 2006 symposium on Interactive 3D graphics and games, Variance Shadow Maps, pages 161-165 William Donnelly, Andrew Lauritzen
- GPU Gems 3, Chapter 8, Summed-Area Variance Shadow Maps, Andrew Lauritzen: http://http.developer.nvidia.com/GPUGems3/gpugems3_ch08.html
- Proceedings of the Graphics Interface 2008, Layered variance shadow maps, pages 139-146, Andrew Lauritzen, Michael McCool
- Sample Distribution Shadow Maps, ACM SIGGRAPH Symposium on Interactive 3D Graphics and Games (I3D) 2011, February, Andrew Lauritzen, Marco Salvi, and Aaron Lefohn
shadowmap
texture as in the shadow map recipe, but this time remove the depth compare mode (glTexParameteri(GL_TEXTURE_2D,GL_TEXTURE_COMPARE_MODE,GL_COMPARE_REF_TO_TEXTURE)
and glTexParameteri(GL_TEXTURE_2D,GL_TEXTURE_COMPARE_FUNC,GL_LEQUAL)
).
Also set the format of the texture to the GL_RGBA32F
format. Also enable the mipmap generation for this texture. The mipmaps provide filtered textures across different scales and produces better alias-free shadows. We request five mipmap levels (by specifying the max level as 4).glGenTextures(1, &shadowMapTexID); glActiveTexture(GL_TEXTURE0); glBindTexture(GL_TEXTURE_2D, shadowMapTexID); glTexParameteri(GL_TEXTURE_2D,GL_TEXTURE_MAG_FILTER,GL_LINEAR; glTexParameteri(GL_TEXTURE_2D,GL_TEXTURE_MIN_FILTER,GL_LINEAR_MIPMAP_LINEAR); glTexParameteri(GL_TEXTURE_2D,GL_TEXTURE_WRAP_S,GL_CLAMP_TO_BORDER); glTexParameteri(GL_TEXTURE_2D,GL_TEXTURE_WRAP_T,GL_CLAMP_TO_BORDER); glTexParameterfv(GL_TEXTURE_2D,GL_TEXTURE_BORDER_COLOR,border; glTexImage2D(GL_TEXTURE_2D,0,GL_RGBA32F,SHADOWMAP_WIDTH,SHADOWMAP_HEIGHT,0,GL_RGBA,GL_FLOAT,NULL); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_BASE_LEVEL, 0); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MAX_LEVEL, 4); glGenerateMipmap(GL_TEXTURE_2D);
- FBOs: one for shadowmap generation and another for shadowmap filtering. The shadowmap FBO has a
renderbuffer
attached to it for depth testing. The filtering FBO does not have arenderbuffer
attached to it but it has two texture attachments.glGenFramebuffers(1,&fboID); glGenRenderbuffers(1, &rboID); glBindFramebuffer(GL_FRAMEBUFFER,fboID); glBindRenderbuffer(GL_RENDERBUFFER, rboID); glRenderbufferStorage(GL_RENDERBUFFER, GL_DEPTH_COMPONENT32, SHADOWMAP_WIDTH, SHADOWMAP_HEIGHT); glFramebufferTexture2D(GL_FRAMEBUFFER,GL_COLOR_ATTACHMENT0,GL_TEXTURE_2D,shadowMapTexID,0); glFramebufferRenderbuffer(GL_FRAMEBUFFER, GL_DEPTH_ATTACHMENT, GL_RENDERBUFFER, rboID); GLenum status = glCheckFramebufferStatus(GL_FRAMEBUFFER); if(status == GL_FRAMEBUFFER_COMPLETE) { cout<<"FBO setup successful."<<endl; } else { cout<<"Problem in FBO setup."<<endl; } glBindFramebuffer(GL_FRAMEBUFFER,0); glGenFramebuffers(1,&filterFBOID); glBindFramebuffer(GL_FRAMEBUFFER,filterFBOID); glGenTextures(2, blurTexID); for(int i=0;i<2;i++) { glActiveTexture(GL_TEXTURE1+i); glBindTexture(GL_TEXTURE_2D, blurTexID[i]); glTexParameteri(GL_TEXTURE_2D,GL_TEXTURE_MAG_FILTER,GL_LINEAR); glTexParameteri(GL_TEXTURE_2D,GL_TEXTURE_MIN_FILTER,GL_LINEAR); glTexParameteri(GL_TEXTURE_2D,GL_TEXTURE_WRAP_S,GL_CLAMP_TO_BORDER); glTexParameteri(GL_TEXTURE_2D,GL_TEXTURE_WRAP_T,GL_CLAMP_TO_BORDER); glTexParameterfv(GL_TEXTURE_2D,GL_TEXTURE_BORDER_COLOR,border); glTexImage2D(GL_TEXTURE_2D,0,GL_RGBA32F,SHADOWMAP_WIDTH,SHADOWMAP_HEIGHT,0,GL_RGBA,GL_FLOAT,NULL); glFramebufferTexture2D(GL_FRAMEBUFFER,GL_COLOR_ATTACHMENT0+i, GL_TEXTURE_2D,blurTexID[i],0); } status = glCheckFramebufferStatus(GL_FRAMEBUFFER); if(status == GL_FRAMEBUFFER_COMPLETE) { cout<<"Filtering FBO setup successful."<<endl; } else { cout<<"Problem in Filtering FBO setup."<<endl; } glBindFramebuffer(GL_FRAMEBUFFER,0);
- Bind the
shadowmap
FBO, set the viewport to the size of theshadowmap
texture, and render the scene from the point of view of the light, as in the Implementing shadow mapping with FBO recipe. In this pass, instead of storing the depth as in the shadow mapping recipe, we use a custom fragment shader (Chapter4/VarianceShadowmapping/shaders/firststep.frag
) to output the depth and depth*depth values in the red and green channels of the fragment output color.glBindFramebuffer(GL_FRAMEBUFFER,fboID); glViewport(0,0,SHADOWMAP_WIDTH, SHADOWMAP_HEIGHT); glDrawBuffer(GL_COLOR_ATTACHMENT0); glClear(GL_COLOR_BUFFER_BIT|GL_DEPTH_BUFFER_BIT); DrawSceneFirstPass(MV_L, P_L);
The shader code is as follows:
#version 330 core layout(location=0) out vec4 vFragColor; smooth in vec4 clipSpacePos; void main() { vec3 pos = clipSpacePos.xyz/clipSpacePos.w; //-1 to 1 pos.z += 0.001; //add some offset to remove the shadow acne float depth = (pos.z +1)*0.5; // 0 to 1 float moment1 = depth; float moment2 = depth * depth; vFragColor = vec4(moment1,moment2,0,0); }
- Bind the filtering FBO to filter the
shadowmap
texture generated in the first pass using separable Gaussian smoothing filters, which are more efficient and offer better performance. We first attach the vertical smoothing fragment shader (Chapter4/VarianceShadowmapping/shaders/GaussV.frag
) to filter theshadowmap
texture and then the horizontal smoothing fragment shader (Chapter4/VarianceShadowmapping/shaders/GaussH.frag
) to smooth the output from the vertical Gaussian smoothing filter.glBindFramebuffer(GL_FRAMEBUFFER,filterFBOID); glDrawBuffer(GL_COLOR_ATTACHMENT0); glBindVertexArray(quadVAOID); gaussianV_shader.Use(); glDrawElements(GL_TRIANGLES, 6, GL_UNSIGNED_SHORT, 0); glDrawBuffer(GL_COLOR_ATTACHMENT1); gaussianH_shader.Use(); glDrawElements(GL_TRIANGLES, 6, GL_UNSIGNED_SHORT, 0); glBindFramebuffer(GL_FRAMEBUFFER,0);
The horizontal Gaussian blur shader is as follows:
#version 330 core layout(location=0) out vec4 vFragColor; smooth in vec2 vUV; uniform sampler2D textureMap; const float kernel[]=float[21] (0.000272337, 0.00089296, 0.002583865, 0.00659813, 0.014869116, 0.029570767, 0.051898313, 0.080381679, 0.109868729, 0.132526984, 0.14107424, 0.132526984, 0.109868729, 0.080381679, 0.051898313, 0.029570767, 0.014869116, 0.00659813, 0.002583865, 0.00089296, 0.000272337); void main() { vec2 delta = 1.0/textureSize(textureMap,0); vec4 color = vec4(0); int index = 20; for(int i=-10;i<=10;i++) { color += kernel[index--]*texture(textureMap, vUV + (vec2(i*delta.x,0))); } vFragColor = vec4(color.xy,0,0); }
In the vertical Gaussian shader, the loop statement is modified, whereas the rest of the shader is the same.
color += kernel[index--]*texture(textureMap, vUV + (vec2(0,i*delta.y)));
- Unbind the FBO, reset the default viewport, and then render the scene normally, as in the shadow mapping recipe.
glDrawBuffer(GL_BACK_LEFT); glViewport(0,0,WIDTH, HEIGHT); DrawScene(MV, P);
The variance shadowmap technique tries to represent the depth data such that it can be filtered linearly. Instead of storing the depth, it stores the depth
and depth*depth
value in a floating point texture, which is then filtered to reconstruct the first and second moments of the depth distribution. Using the moments, it estimates the variance in the filtering neighborhood. This helps in finding the probability of a fragment at a specific depth to be occluded using Chebyshev's inequality. For more mathematical details, we refer the reader to the See also section of this recipe.
After the first pass, the shadowmap
texture is blurred using a separable Gaussian smoothing filter. First the vertical and then the horizontal filter is applied to the shadowmap
texture by applying the shadowmap
texture to a full-screen quad and alternating the filter FBO's color attachment. Note that the shadowmap
texture is bound to texture unit 0 whereas the textures used for filtering are bound to texture unit 1 (attached to GL_COLOR_ATTTACHMENT0
on the filtering FBO) and texture unit 2 (attached to GL_COLOR_ATTACHMENT1
on the filtering FBO).
In the second pass, the scene is rendered from the point of view of the camera. The blurred shadowmap is used in the second pass as a texture to lookup the sample value (see Chapter4/VarianceShadowmapping/shaders/VarianceShadowMap.{vert, frag}
). The variance shadow mapping vertex shader outputs the shadow texture coordinates, as in the shadow mapping recipe.
The texture coordinates after homogeneous division are used to lookup the shadow map storing the two moments. The two moments are used to estimate the variance. The variance is clamped and then the occlusion probability is estimated. The diffuse component is then modulated based on the obtained occlusion probability.
To recap, here is the complete variance shadow mapping fragment shader:
Variance shadow mapping is an interesting idea. However, it does suffer from light bleeding artefacts. There have been several improvements to the basic technique, such as summed area variance shadow maps, layered variance shadow maps, and more recently, sample distribution shadow maps, that are referred to in the See also section of this recipe. After getting a practical insight into the basic variance shadow mapping idea, we invite the reader to try and implement the different variants of this algorithm, as detailed in the references in the See also section.
- Proceedings of the 2006 symposium on Interactive 3D graphics and games, Variance Shadow Maps, pages 161-165 William Donnelly, Andrew Lauritzen
- GPU Gems 3, Chapter 8, Summed-Area Variance Shadow Maps, Andrew Lauritzen: http://http.developer.nvidia.com/GPUGems3/gpugems3_ch08.html
- Proceedings of the Graphics Interface 2008, Layered variance shadow maps, pages 139-146, Andrew Lauritzen, Michael McCool
- Sample Distribution Shadow Maps, ACM SIGGRAPH Symposium on Interactive 3D Graphics and Games (I3D) 2011, February, Andrew Lauritzen, Marco Salvi, and Aaron Lefohn
After the first pass, the shadowmap
texture is blurred using a separable Gaussian smoothing filter. First the vertical and then the horizontal filter is applied to the shadowmap
texture by applying the shadowmap
texture to a full-screen quad and alternating the filter FBO's color attachment. Note that the shadowmap
texture is bound to texture unit 0 whereas the textures used for filtering are bound to texture unit 1 (attached to GL_COLOR_ATTTACHMENT0
on the filtering FBO) and texture unit 2 (attached to GL_COLOR_ATTACHMENT1
on the filtering FBO).
In the second pass, the scene is rendered from the point of view of the camera. The blurred shadowmap is used in the second pass as a texture to lookup the sample value (see Chapter4/VarianceShadowmapping/shaders/VarianceShadowMap.{vert, frag}
). The variance shadow mapping vertex shader outputs the shadow texture coordinates, as in the shadow mapping recipe.
The texture coordinates after homogeneous division are used to lookup the shadow map storing the two moments. The two moments are used to estimate the variance. The variance is clamped and then the occlusion probability is estimated. The diffuse component is then modulated based on the obtained occlusion probability.
To recap, here is the complete variance shadow mapping fragment shader:
Variance shadow mapping is an interesting idea. However, it does suffer from light bleeding artefacts. There have been several improvements to the basic technique, such as summed area variance shadow maps, layered variance shadow maps, and more recently, sample distribution shadow maps, that are referred to in the See also section of this recipe. After getting a practical insight into the basic variance shadow mapping idea, we invite the reader to try and implement the different variants of this algorithm, as detailed in the references in the See also section.
- Proceedings of the 2006 symposium on Interactive 3D graphics and games, Variance Shadow Maps, pages 161-165 William Donnelly, Andrew Lauritzen
- GPU Gems 3, Chapter 8, Summed-Area Variance Shadow Maps, Andrew Lauritzen: http://http.developer.nvidia.com/GPUGems3/gpugems3_ch08.html
- Proceedings of the Graphics Interface 2008, Layered variance shadow maps, pages 139-146, Andrew Lauritzen, Michael McCool
- Sample Distribution Shadow Maps, ACM SIGGRAPH Symposium on Interactive 3D Graphics and Games (I3D) 2011, February, Andrew Lauritzen, Marco Salvi, and Aaron Lefohn
- Proceedings of the 2006 symposium on Interactive 3D graphics and games, Variance Shadow Maps, pages 161-165 William Donnelly, Andrew Lauritzen
- GPU Gems 3, Chapter 8, Summed-Area Variance Shadow Maps, Andrew Lauritzen: http://http.developer.nvidia.com/GPUGems3/gpugems3_ch08.html
- Proceedings of the Graphics Interface 2008, Layered variance shadow maps, pages 139-146, Andrew Lauritzen, Michael McCool
- Sample Distribution Shadow Maps, ACM SIGGRAPH Symposium on Interactive 3D Graphics and Games (I3D) 2011, February, Andrew Lauritzen, Marco Salvi, and Aaron Lefohn
- Chapter 8, Summed-Area Variance Shadow Maps, Andrew Lauritzen: http://http.developer.nvidia.com/GPUGems3/gpugems3_ch08.html
- Proceedings of the Graphics Interface 2008, Layered variance shadow maps, pages 139-146, Andrew Lauritzen, Michael McCool
- Sample Distribution Shadow Maps, ACM SIGGRAPH Symposium on Interactive 3D Graphics and Games (I3D) 2011, February, Andrew Lauritzen, Marco Salvi, and Aaron Lefohn
In this chapter, we will focus on:
While simple demos and applications can get along with basic primitives like cubes and spheres, most real-world applications and games use 3D mesh models which are modelled in 3D modeling software such as 3ds Max and Maya. For games, the models are then exported into the proprietary game format and then the models are loaded into the game.
While there are many formats available, some formats such as Autodesk® 3ds and Wavefront® OBJ are common formats. In this chapter, we will look at recipes for loading these model formats. We will look at how to load the geometry information, stored in the external files, into the vertex buffer object memory of the GPU. In addition, we will also load material and texture information which is required to improve the fidelity of the model so that it appears more realistic. We will also work on loading terrains which are often used to model outdoor environments. Finally, we will implement a basic particle system for simulating fuzzy phenomena such as fire and smoke. All of the discussed techniques will be implemented in the OpenGL v3.3 and above core profile.
Several demos and applications require rendering of terrains. This recipe will show how to implement terrain generation in modern OpenGL. The height map is loaded using the SOIL
image loading library which contains displacement information. A 2D grid is then generated depending on the required terrain resolution. Then, the displacement information contained in the height map is used to displace the 2D grid in the vertex shader. Usually, the obtained displacement value is scaled to increase or decrease the displacement scale as desired.
For the terrain, first the 2D grid geometry is generated depending on the terrain resolution. The steps to generate such geometry were previously covered in the Doing a ripple mesh deformer using vertex shader recipe in Chapter 1, Introduction to Modern OpenGL. The code for this recipe is contained in the Chapter5/TerrainLoading
directory.
Let us start our recipe by following these simple steps:
- Load the height map texture using the
SOIL
image loading library and generate an OpenGL texture from it. The texture filtering is set toGL_NEAREST
as we want to obtain the exact values from the height map. If we had changed this toGL_LINEAR
, we would get interpolated values. Since the terrain height map is not tiled, we set the texture wrap mode toGL_CLAMP
.int texture_width = 0, texture_height = 0, channels=0; GLubyte* pData = SOIL_load_image(filename.c_str(),&texture_width, &texture_height, &channels, SOIL_LOAD_L); //vertically flip the image data for( j = 0; j*2 < texture_height; ++j ) { int index1 = j * texture_width ; int index2 = (texture_height - 1 - j) * texture_width ; for( i = texture_width ; i > 0; --i ) { GLubyte temp = pData[index1]; pData[index1] = pData[index2]; pData[index2] = temp; ++index1; ++index2; } } glGenTextures(1, &heightMapTextureID); glActiveTexture(GL_TEXTURE0); glBindTexture(GL_TEXTURE_2D, heightMapTextureID); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MIN_FILTER, GL_NEAREST); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MAG_FILTER, GL_NEAREST); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_S, GL_CLAMP); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_T, GL_CLAMP); glTexImage2D(GL_TEXTURE_2D, 0, GL_RED, texture_width, texture_height, 0, GL_RED, GL_UNSIGNED_BYTE, pData); SOIL_free_image_data(pData);
- Set up the terrain geometry by generating a set of points in the XZ plane. The
TERRAIN_WIDTH
parameter controls the total number of vertices in the X axis whereas theTERRAIN_DEPTH
parameter controls the total number of vertices in the Z axis.for( j=0;j<TERRAIN_DEPTH;j++) { for( i=0;i<TERRAIN_WIDTH;i++) { vertices[count]=glm::vec3((float(i)/(TERRAIN_WIDTH-1)), 0, (float(j)/(TERRAIN_DEPTH-1))); count++; } }
- Set up the vertex shader that displaces the 2D terrain mesh. Refer to
Chapter5/TerrainLoading/shaders/shader.vert
for details. The height value is obtained from the height map. This value is then added to the current vertex position and finally multiplied with the combined modelview projection (MVP) matrix to get the clip space position. TheHALF_TERRAIN_SIZE
uniform contains half of the total number of vertices in both the X and Z axes, that is,HALF_TERRAIN_SIZE = ivec2(TERRAIN_WIDTH/2, TERRAIN_DEPTH/2)
. Similarly the scale uniform is used to scale the height read from the height map. Thehalf_scale
andHALF_TERRAIN_SIZE
uniforms are used to position the mesh at origin.#version 330 core layout (location=0) in vec3 vVertex; uniform mat4 MVP; uniform ivec2 HALF_TERRAIN_SIZE; uniform sampler2D heightMapTexture; uniform float scale; uniform float half_scale; void main() { float height = texture(heightMapTexture, vVertex.xz).r*scale - half_scale; vec2 pos = (vVertex.xz*2.0-1)*HALF_TERRAIN_SIZE; gl_Position = MVP*vec4(pos.x, height, pos.y, 1); }
- Load the shaders and the corresponding uniform and attribute locations. Also, set the values of the uniforms that never change during the lifetime of the application, at initialization.
shader.LoadFromFile(GL_VERTEX_SHADER, "shaders/shader.vert"); shader.LoadFromFile(GL_FRAGMENT_SHADER, "shaders/shader.frag"); shader.CreateAndLinkProgram(); shader.Use(); shader.AddAttribute("vVertex"); shader.AddUniform("heightMapTexture"); shader.AddUniform("scale"); shader.AddUniform("half_scale"); shader.AddUniform("HALF_TERRAIN_SIZE"); shader.AddUniform("MVP"); glUniform1i(shader("heightMapTexture"), 0); glUniform2i(shader("HALF_TERRAIN_SIZE"), TERRAIN_WIDTH>>1, TERRAIN_DEPTH>>1); glUniform1f(shader("scale"), scale); glUniform1f(shader("half_scale"), half_scale); shader.UnUse();
- In the rendering code, set the shader and render the terrain by passing the modelview/projection matrices to the shader as shader uniforms.
shader.Use(); glUniformMatrix4fv(shader("MVP"), 1, GL_FALSE, glm::value_ptr(MVP)); glDrawElements(GL_TRIANGLES,TOTAL_INDICES, GL_UNSIGNED_INT, 0); shader.UnUse();
Terrain rendering is relatively straight forward to implement. The geometry is first generated on the CPU and is then stored in the GPU buffer objects. Next, the height map is loaded from an image which is then transferred to the vertex shader as a texture sampler uniform.
The output from the demo application for this recipe renders a wireframe terrain as shown in the following screenshot:
The height map used to generate this terrain is shown in the following screenshot:
The method we have presented in this recipe uses the vertex displacement to generate a terrain from a height map. There are several tools available that can help with the terrain height map generation. One of them is Terragen (planetside.co.uk). Another useful tool is World Machine (http://world-machine.com/). A general source of information for terrains is available at the virtual terrain project (http://vterrain.org/).
We can also use procedural methods to generate terrains such as fractal terrain generation. Noise methods can also be helpful in the generation of the terrains.
To know more about implementing terrains, you can check the following:
- Focus on 3D Terrain Programming, by Trent Polack, Premier Press, 2002
- Chapter 7, Terrain Level of Detail in Level of Detail for 3D Graphics by David Luebke, Morgan Kaufmann Publishers, 2003.
Chapter 1, Introduction to Modern OpenGL. The code for this recipe is contained in the Chapter5/TerrainLoading
directory.
Let us start our recipe by following these simple steps:
- Load the height map texture using the
SOIL
image loading library and generate an OpenGL texture from it. The texture filtering is set toGL_NEAREST
as we want to obtain the exact values from the height map. If we had changed this toGL_LINEAR
, we would get interpolated values. Since the terrain height map is not tiled, we set the texture wrap mode toGL_CLAMP
.int texture_width = 0, texture_height = 0, channels=0; GLubyte* pData = SOIL_load_image(filename.c_str(),&texture_width, &texture_height, &channels, SOIL_LOAD_L); //vertically flip the image data for( j = 0; j*2 < texture_height; ++j ) { int index1 = j * texture_width ; int index2 = (texture_height - 1 - j) * texture_width ; for( i = texture_width ; i > 0; --i ) { GLubyte temp = pData[index1]; pData[index1] = pData[index2]; pData[index2] = temp; ++index1; ++index2; } } glGenTextures(1, &heightMapTextureID); glActiveTexture(GL_TEXTURE0); glBindTexture(GL_TEXTURE_2D, heightMapTextureID); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MIN_FILTER, GL_NEAREST); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MAG_FILTER, GL_NEAREST); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_S, GL_CLAMP); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_T, GL_CLAMP); glTexImage2D(GL_TEXTURE_2D, 0, GL_RED, texture_width, texture_height, 0, GL_RED, GL_UNSIGNED_BYTE, pData); SOIL_free_image_data(pData);
- Set up the terrain geometry by generating a set of points in the XZ plane. The
TERRAIN_WIDTH
parameter controls the total number of vertices in the X axis whereas theTERRAIN_DEPTH
parameter controls the total number of vertices in the Z axis.for( j=0;j<TERRAIN_DEPTH;j++) { for( i=0;i<TERRAIN_WIDTH;i++) { vertices[count]=glm::vec3((float(i)/(TERRAIN_WIDTH-1)), 0, (float(j)/(TERRAIN_DEPTH-1))); count++; } }
- Set up the vertex shader that displaces the 2D terrain mesh. Refer to
Chapter5/TerrainLoading/shaders/shader.vert
for details. The height value is obtained from the height map. This value is then added to the current vertex position and finally multiplied with the combined modelview projection (MVP) matrix to get the clip space position. TheHALF_TERRAIN_SIZE
uniform contains half of the total number of vertices in both the X and Z axes, that is,HALF_TERRAIN_SIZE = ivec2(TERRAIN_WIDTH/2, TERRAIN_DEPTH/2)
. Similarly the scale uniform is used to scale the height read from the height map. Thehalf_scale
andHALF_TERRAIN_SIZE
uniforms are used to position the mesh at origin.#version 330 core layout (location=0) in vec3 vVertex; uniform mat4 MVP; uniform ivec2 HALF_TERRAIN_SIZE; uniform sampler2D heightMapTexture; uniform float scale; uniform float half_scale; void main() { float height = texture(heightMapTexture, vVertex.xz).r*scale - half_scale; vec2 pos = (vVertex.xz*2.0-1)*HALF_TERRAIN_SIZE; gl_Position = MVP*vec4(pos.x, height, pos.y, 1); }
- Load the shaders and the corresponding uniform and attribute locations. Also, set the values of the uniforms that never change during the lifetime of the application, at initialization.
shader.LoadFromFile(GL_VERTEX_SHADER, "shaders/shader.vert"); shader.LoadFromFile(GL_FRAGMENT_SHADER, "shaders/shader.frag"); shader.CreateAndLinkProgram(); shader.Use(); shader.AddAttribute("vVertex"); shader.AddUniform("heightMapTexture"); shader.AddUniform("scale"); shader.AddUniform("half_scale"); shader.AddUniform("HALF_TERRAIN_SIZE"); shader.AddUniform("MVP"); glUniform1i(shader("heightMapTexture"), 0); glUniform2i(shader("HALF_TERRAIN_SIZE"), TERRAIN_WIDTH>>1, TERRAIN_DEPTH>>1); glUniform1f(shader("scale"), scale); glUniform1f(shader("half_scale"), half_scale); shader.UnUse();
- In the rendering code, set the shader and render the terrain by passing the modelview/projection matrices to the shader as shader uniforms.
shader.Use(); glUniformMatrix4fv(shader("MVP"), 1, GL_FALSE, glm::value_ptr(MVP)); glDrawElements(GL_TRIANGLES,TOTAL_INDICES, GL_UNSIGNED_INT, 0); shader.UnUse();
Terrain rendering is relatively straight forward to implement. The geometry is first generated on the CPU and is then stored in the GPU buffer objects. Next, the height map is loaded from an image which is then transferred to the vertex shader as a texture sampler uniform.
The output from the demo application for this recipe renders a wireframe terrain as shown in the following screenshot:
The height map used to generate this terrain is shown in the following screenshot:
The method we have presented in this recipe uses the vertex displacement to generate a terrain from a height map. There are several tools available that can help with the terrain height map generation. One of them is Terragen (planetside.co.uk). Another useful tool is World Machine (http://world-machine.com/). A general source of information for terrains is available at the virtual terrain project (http://vterrain.org/).
We can also use procedural methods to generate terrains such as fractal terrain generation. Noise methods can also be helpful in the generation of the terrains.
To know more about implementing terrains, you can check the following:
- Focus on 3D Terrain Programming, by Trent Polack, Premier Press, 2002
- Chapter 7, Terrain Level of Detail in Level of Detail for 3D Graphics by David Luebke, Morgan Kaufmann Publishers, 2003.
SOIL
image loading library and generate an OpenGL texture from it. The texture filtering is set to GL_NEAREST
as we want to obtain the exact values from the height map. If we had changed this to GL_LINEAR
, we would get interpolated values. Since the terrain height map is not tiled, we set the texture wrap mode to GL_CLAMP
.int texture_width = 0, texture_height = 0, channels=0; GLubyte* pData = SOIL_load_image(filename.c_str(),&texture_width, &texture_height, &channels, SOIL_LOAD_L); //vertically flip the image data for( j = 0; j*2 < texture_height; ++j ) { int index1 = j * texture_width ; int index2 = (texture_height - 1 - j) * texture_width ; for( i = texture_width ; i > 0; --i ) { GLubyte temp = pData[index1]; pData[index1] = pData[index2]; pData[index2] = temp; ++index1; ++index2; } } glGenTextures(1, &heightMapTextureID); glActiveTexture(GL_TEXTURE0); glBindTexture(GL_TEXTURE_2D, heightMapTextureID); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MIN_FILTER, GL_NEAREST); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MAG_FILTER, GL_NEAREST); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_S, GL_CLAMP); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_T, GL_CLAMP); glTexImage2D(GL_TEXTURE_2D, 0, GL_RED, texture_width, texture_height, 0, GL_RED, GL_UNSIGNED_BYTE, pData); SOIL_free_image_data(pData);
TERRAIN_WIDTH
parameter
- controls the total number of vertices in the X axis whereas the
TERRAIN_DEPTH
parameter controls the total number of vertices in the Z axis.for( j=0;j<TERRAIN_DEPTH;j++) { for( i=0;i<TERRAIN_WIDTH;i++) { vertices[count]=glm::vec3((float(i)/(TERRAIN_WIDTH-1)), 0, (float(j)/(TERRAIN_DEPTH-1))); count++; } }
- Set up the vertex shader that displaces the 2D terrain mesh. Refer to
Chapter5/TerrainLoading/shaders/shader.vert
for details. The height value is obtained from the height map. This value is then added to the current vertex position and finally multiplied with the combined modelview projection (MVP) matrix to get the clip space position. TheHALF_TERRAIN_SIZE
uniform contains half of the total number of vertices in both the X and Z axes, that is,HALF_TERRAIN_SIZE = ivec2(TERRAIN_WIDTH/2, TERRAIN_DEPTH/2)
. Similarly the scale uniform is used to scale the height read from the height map. Thehalf_scale
andHALF_TERRAIN_SIZE
uniforms are used to position the mesh at origin.#version 330 core layout (location=0) in vec3 vVertex; uniform mat4 MVP; uniform ivec2 HALF_TERRAIN_SIZE; uniform sampler2D heightMapTexture; uniform float scale; uniform float half_scale; void main() { float height = texture(heightMapTexture, vVertex.xz).r*scale - half_scale; vec2 pos = (vVertex.xz*2.0-1)*HALF_TERRAIN_SIZE; gl_Position = MVP*vec4(pos.x, height, pos.y, 1); }
- Load the shaders and the corresponding uniform and attribute locations. Also, set the values of the uniforms that never change during the lifetime of the application, at initialization.
shader.LoadFromFile(GL_VERTEX_SHADER, "shaders/shader.vert"); shader.LoadFromFile(GL_FRAGMENT_SHADER, "shaders/shader.frag"); shader.CreateAndLinkProgram(); shader.Use(); shader.AddAttribute("vVertex"); shader.AddUniform("heightMapTexture"); shader.AddUniform("scale"); shader.AddUniform("half_scale"); shader.AddUniform("HALF_TERRAIN_SIZE"); shader.AddUniform("MVP"); glUniform1i(shader("heightMapTexture"), 0); glUniform2i(shader("HALF_TERRAIN_SIZE"), TERRAIN_WIDTH>>1, TERRAIN_DEPTH>>1); glUniform1f(shader("scale"), scale); glUniform1f(shader("half_scale"), half_scale); shader.UnUse();
- In the rendering code, set the shader and render the terrain by passing the modelview/projection matrices to the shader as shader uniforms.
shader.Use(); glUniformMatrix4fv(shader("MVP"), 1, GL_FALSE, glm::value_ptr(MVP)); glDrawElements(GL_TRIANGLES,TOTAL_INDICES, GL_UNSIGNED_INT, 0); shader.UnUse();
Terrain rendering is relatively straight forward to implement. The geometry is first generated on the CPU and is then stored in the GPU buffer objects. Next, the height map is loaded from an image which is then transferred to the vertex shader as a texture sampler uniform.
The output from the demo application for this recipe renders a wireframe terrain as shown in the following screenshot:
The height map used to generate this terrain is shown in the following screenshot:
The method we have presented in this recipe uses the vertex displacement to generate a terrain from a height map. There are several tools available that can help with the terrain height map generation. One of them is Terragen (planetside.co.uk). Another useful tool is World Machine (http://world-machine.com/). A general source of information for terrains is available at the virtual terrain project (http://vterrain.org/).
We can also use procedural methods to generate terrains such as fractal terrain generation. Noise methods can also be helpful in the generation of the terrains.
To know more about implementing terrains, you can check the following:
- Focus on 3D Terrain Programming, by Trent Polack, Premier Press, 2002
- Chapter 7, Terrain Level of Detail in Level of Detail for 3D Graphics by David Luebke, Morgan Kaufmann Publishers, 2003.
The output from the demo application for this recipe renders a wireframe terrain as shown in the following screenshot:
The height map used to generate this terrain is shown in the following screenshot:
The method we have presented in this recipe uses the vertex displacement to generate a terrain from a height map. There are several tools available that can help with the terrain height map generation. One of them is Terragen (planetside.co.uk). Another useful tool is World Machine (http://world-machine.com/). A general source of information for terrains is available at the virtual terrain project (http://vterrain.org/).
We can also use procedural methods to generate terrains such as fractal terrain generation. Noise methods can also be helpful in the generation of the terrains.
To know more about implementing terrains, you can check the following:
- Focus on 3D Terrain Programming, by Trent Polack, Premier Press, 2002
- Chapter 7, Terrain Level of Detail in Level of Detail for 3D Graphics by David Luebke, Morgan Kaufmann Publishers, 2003.
terrain height map generation. One of them is Terragen (planetside.co.uk). Another useful tool is World Machine (http://world-machine.com/). A general source of information for terrains is available at the virtual terrain project (http://vterrain.org/).
We can also use procedural methods to generate terrains such as fractal terrain generation. Noise methods can also be helpful in the generation of the terrains.
To know more about implementing terrains, you can check the following:
- Focus on 3D Terrain Programming, by Trent Polack, Premier Press, 2002
- Chapter 7, Terrain Level of Detail in Level of Detail for 3D Graphics by David Luebke, Morgan Kaufmann Publishers, 2003.
- Chapter 7, Terrain Level of Detail in Level of Detail for 3D Graphics by David Luebke, Morgan Kaufmann Publishers, 2003.
We will now create model loader and renderer for Autodesk® 3ds model format which is a simple yet efficient binary model format for storing digital assets.
The code for this recipe is contained in the Chapter5/3DsViewer
folder. This recipe will be using the Drawing a 2D image in a window using a fragment shader and the SOIL image loading library recipe from Chapter 1, Introduction to Modern OpenGL, for loading the 3ds mesh file's textures using the SOIL
image loading library.
The steps required to implement a 3ds file viewer are as follows:
- Create an instance of the
C3dsLoader
class. Then call theC3dsLoader::Load3DS
function passing it the name of the mesh file and a set of vectors to store the submeshes, vertices, normals, uvs, indices, and materials.if(!loader.Load3DS(mesh_filename.c_str( ), meshes, vertices, normals, uvs, faces, indices, materials)) { cout<<"Cannot load the 3ds mesh"<<endl; exit(EXIT_FAILURE); }
- After the mesh is loaded, use the mesh's material list to load the material textures into the OpenGL texture object.
for(size_t k=0;k<materials.size();k++) { for(size_t m=0;m< materials[k]->textureMaps.size();m++) { GLuint id = 0; glGenTextures(1, &id); glBindTexture(GL_TEXTURE_2D, id); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MIN_FILTER, GL_LINEAR); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MAG_FILTER, GL_LINEAR); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_S, GL_REPEAT); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_T, GL_REPEAT); int texture_width = 0, texture_height = 0, channels=0; const string& filename = materials[k]->textureMaps[m]->filename; std::string full_filename = mesh_path; full_filename.append(filename); GLubyte* pData = SOIL_load_image(full_filename.c_str(), &texture_width, &texture_height, &channels, SOIL_LOAD_AUTO); if(pData == NULL) { cerr<<"Cannot load image: "<<full_filename.c_str()<<endl; exit(EXIT_FAILURE); } //Flip the image on Y axis int i,j; for( j = 0; j*2 < texture_height; ++j ) { int index1 = j * texture_width * channels; int index2 = (texture_height - 1 - j) * texture_width * channels; for( i = texture_width * channels; i > 0; --i ){ GLubyte temp = pData[index1]; pData[index1] = pData[index2]; pData[index2] = temp; ++index1; ++index2; } } GLenum format = GL_RGBA; switch(channels) { case 2: format = GL_RG32UI; break; case 3: format = GL_RGB; break; case 4: format = GL_RGBA; break; } glTexImage2D(GL_TEXTURE_2D, 0, format, texture_width, texture_height, 0, format, GL_UNSIGNED_BYTE, pData); SOIL_free_image_data(pData); textureMaps[filename]=id; } }
- Pass the loaded per-vertex attributes; that is, positions (
vertices
), texture coordinates (uvs
), per-vertex normals (normals
), and triangle indices (indices
) to GPU memory by allocating separate buffer objects for each attribute. Note that for easier handling of buffer objects, we bind a single vertex array object (vaoID
) first.glBindVertexArray(vaoID); glBindBuffer (GL_ARRAY_BUFFER, vboVerticesID); glBufferData (GL_ARRAY_BUFFER, sizeof(glm::vec3)* vertices.size(), &(vertices[0].x), GL_STATIC_DRAW); glEnableVertexAttribArray(shader["vVertex"]); glVertexAttribPointer(shader["vVertex"], 3, GL_FLOAT, GL_FALSE,0,0); glBindBuffer (GL_ARRAY_BUFFER, vboUVsID); glBufferData (GL_ARRAY_BUFFER, sizeof(glm::vec2)*uvs.size(), &(uvs[0].x), GL_STATIC_DRAW); glEnableVertexAttribArray(shader["vUV"]); glVertexAttribPointer(shader["vUV"],2,GL_FLOAT,GL_FALSE,0, 0); glBindBuffer (GL_ARRAY_BUFFER, vboNormalsID); glBufferData (GL_ARRAY_BUFFER, sizeof(glm::vec3)* normals.size(), &(normals[0].x), GL_STATIC_DRAW); glEnableVertexAttribArray(shader["vNormal"]); glVertexAttribPointer(shader["vNormal"], 3, GL_FLOAT, GL_FALSE, 0, 0);
- If we have only a single material in the 3ds file, we store the face indices into
GL_ELEMENT_ARRAY_BUFFER
so that we can render the whole mesh in a single call. However, if we have more than one material, we bind the appropriate submeshes separately. TheglBufferData
call allocates the GPU memory, however, it is not initialized. In order to initialize the buffer object memory, we can use theglMapBuffer
function to obtain a direct pointer to the GPU memory. Using this pointer, we can then write to the GPU memory. An alternative to usingglMapBuffer
isglBufferSubData
which can modify the GPU memory by copying contents from a CPU buffer.if(materials.size()==1) { glBindBuffer(GL_ELEMENT_ARRAY_BUFFER, vboIndicesID); glBufferData(GL_ELEMENT_ARRAY_BUFFER, sizeof(GLushort)* 3*faces.size(), 0, GL_STATIC_DRAW); GLushort* pIndices = static_cast<GLushort*>(glMapBuffer(GL_ELEMENT_ARRAY_BUFFER, GL_WRITE_ONLY)); for(size_t i=0;i<faces.size();i++) { *(pIndices++)=faces[i].a; *(pIndices++)=faces[i].b; *(pIndices++)=faces[i].c; } glUnmapBuffer(GL_ELEMENT_ARRAY_BUFFER); }
- Set up the vertex shader to output the clip space position as well as the per-vertex texture coordinates. The texture coordinates are then interpolated by the rasterizer to the fragment shader using an output attribute
vUVout
.#version 330 core layout(location = 0) in vec3 vVertex; layout(location = 1) in vec3 vNormal; layout(location = 2) in vec2 vUV; smooth out vec2 vUVout; uniform mat4 P; uniform mat4 MV; uniform mat3 N; smooth out vec3 vEyeSpaceNormal; smooth out vec3 vEyeSpacePosition; void main() { vUVout=vUV; vEyeSpacePosition = (MV*vec4(vVertex,1)).xyz; vEyeSpaceNormal = N*vNormal; gl_Position = P*vec4(vEyeSpacePosition,1); }
- Set up the fragment shader, which looks up the texture map sampler with the interpolated texture coordinates from the rasterizer. Depending on whether the submesh has a texture, we linearly interpolate between the texture map color and the diffused color of the material, using the GLSL mix function.
#version 330 core uniform sampler2D textureMap; uniform float hasTexture; uniform vec3 light_position;//light position in object space uniform mat4 MV; smooth in vec3 vEyeSpaceNormal; smooth in vec3 vEyeSpacePosition; smooth in vec2 vUVout; layout(location=0) out vec4 vFragColor; const float k0 = 1.0;//constant attenuation const float k1 = 0.0;//linear attenuation const float k2 = 0.0;//quadratic attenuation void main() { vec4 vEyeSpaceLightPosition = (MV*vec4(light_position,1)); vec3 L = (vEyeSpaceLightPosition.xyz-vEyeSpacePosition); float d = length(L); L = normalize(L); float diffuse = max(0, dot(vEyeSpaceNormal, L)); float attenuationAmount = 1.0/(k0 + (k1*d) + (k2*d*d)); diffuse *= attenuationAmount; vFragColor = diffuse*mix(vec4(1),texture(textureMap, vUVout), hasTexture); }
- The rendering code binds the shader program, sets the shader uniforms, and then renders the mesh, depending on how many materials the 3ds mesh has. If the mesh has only a single material, it is drawn in a single call to
glDrawElement
by using the indices attached to theGL_ELEMENT_ARRAY_BUFFER
binding point.glBindVertexArray(vaoID); { shader.Use(); glUniformMatrix4fv(shader("MV"), 1, GL_FALSE, glm::value_ptr(MV)); glUniformMatrix3fv(shader("N"), 1, GL_FALSE, glm::value_ptr(glm::inverseTranspose(glm::mat3(MV)))); glUniformMatrix4fv(shader("P"), 1, GL_FALSE, glm::value_ptr(P)); glUniform3fv(shader("light_position"),1, &(lightPosOS.x)); if(materials.size()==1) { GLint whichID[1]; glGetIntegerv(GL_TEXTURE_BINDING_2D, whichID); if(textureMaps.size()>0) { if(whichID[0] != textureMaps[materials[0]->textureMaps[0]->filename]) { glBindTexture(GL_TEXTURE_2D, textureMaps[materials[0]->textureMaps[0]->filename]); glUniform1f(shader("hasTexture"),1.0); } } else { glUniform1f(shader("hasTexture"),0.0); glUniform3fv(shader("diffuse_color"),1, materials[0]->diffuse); } glDrawElements(GL_TRIANGLES, meshes[0]->faces.size()*3, GL_UNSIGNED_SHORT, 0); }
- If the mesh contains more than one material, we iterate through the material list, and bind the texture map (if the material has one), otherwise we use the diffuse color stored in the material for the submesh. Finally, we pass the
sub_indices
array stored in the material to theglDrawElements
function to load those indices only.else { for(size_t i=0;i<materials.size();i++) { GLint whichID[1]; glGetIntegerv(GL_TEXTURE_BINDING_2D, whichID); if(materials[i]->textureMaps.size()>0) { if(whichID[0] != textureMaps[materials[i]->textureMaps[0]->filename]) { glBindTexture(GL_TEXTURE_2D, textureMaps[materials[i]->textureMaps[0]->filename]); } glUniform1f(shader("hasTexture"),1.0); } else { glUniform1f(shader("hasTexture"),0.0); } glUniform3fv(shader("diffuse_color"),1, materials[i]->diffuse); glDrawElements(GL_TRIANGLES, materials[i]->sub_indices.size(), GL_UNSIGNED_SHORT, &(materials[i]->sub_indices[0])); } } shader.UnUse();
The main component of this recipe is the C3dsLoader::Load3DS
function. The 3ds file is a binary file which is organized into a collection of chunks. Typically, a reader reads the first two bytes from the file which are stored in the chunk ID. The next four bytes store the chunk length in bytes. We continue reading chunks, and their lengths, and then store data appropriately into our vectors/variables until there are no more chunks and we pass reading the end of file. The 3ds specifications detail all of the chunks and their lengths as well as subchunks, as shown in the following figure:
Note that if there is a subchunk that we are interested in, we need to read the parent chunk as well, to move the file pointer to the appropriate offset in the file, for our required chunk. The loader first finds the total size of the 3ds mesh file in bytes. Then, it runs a while loop that checks to see if the current file pointer is within the file's size. If it is, it continues to read the first two bytes (the chunk's ID) and the next four bytes (the chunk's length).
All names (object name, material name, or texture map name) have to be read byte-by-byte until the null terminator character (\0
) is found. For reading vertices, we first read two bytes that store the total number of vertices (N
). Two bytes means that the maximum number of vertices one mesh can store is 65536. Then, we read the whole chunk of bytes, that is, sizeof(glm::vec3)*N
, directly into our mesh's vertices, shown as follows:
Note that the 3ds format does not store the per-vertex normal explicitly. It only stores smoothing groups which tell us which faces have shared normals. After we have the vertex positions and face information, we can generate the per-vertex normals by averaging the per-face normals. This is carried out by using the following code snippet in the 3ds.cpp
file. We first allocate space for the per-vertex normals. Then we estimate the face's normal by using the cross product of the two edges. Finally, we add the face normal to the appropriate vertex index and then normalize the normal.
Once we have all the per-vertex attributes and faces information, we use this to group the triangles by material. We loop through all of the materials and expand their face IDs to include the three vertex IDs and make the face.
Chapter5/3DsViewer
folder. This recipe will be using the Drawing a 2D image in a window using a fragment shader and the SOIL image loading library recipe from
Chapter 1, Introduction to Modern OpenGL, for loading the 3ds mesh file's textures using the SOIL
image loading library.
The steps required to implement a 3ds file viewer are as follows:
- Create an instance of the
C3dsLoader
class. Then call theC3dsLoader::Load3DS
function passing it the name of the mesh file and a set of vectors to store the submeshes, vertices, normals, uvs, indices, and materials.if(!loader.Load3DS(mesh_filename.c_str( ), meshes, vertices, normals, uvs, faces, indices, materials)) { cout<<"Cannot load the 3ds mesh"<<endl; exit(EXIT_FAILURE); }
- After the mesh is loaded, use the mesh's material list to load the material textures into the OpenGL texture object.
for(size_t k=0;k<materials.size();k++) { for(size_t m=0;m< materials[k]->textureMaps.size();m++) { GLuint id = 0; glGenTextures(1, &id); glBindTexture(GL_TEXTURE_2D, id); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MIN_FILTER, GL_LINEAR); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MAG_FILTER, GL_LINEAR); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_S, GL_REPEAT); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_T, GL_REPEAT); int texture_width = 0, texture_height = 0, channels=0; const string& filename = materials[k]->textureMaps[m]->filename; std::string full_filename = mesh_path; full_filename.append(filename); GLubyte* pData = SOIL_load_image(full_filename.c_str(), &texture_width, &texture_height, &channels, SOIL_LOAD_AUTO); if(pData == NULL) { cerr<<"Cannot load image: "<<full_filename.c_str()<<endl; exit(EXIT_FAILURE); } //Flip the image on Y axis int i,j; for( j = 0; j*2 < texture_height; ++j ) { int index1 = j * texture_width * channels; int index2 = (texture_height - 1 - j) * texture_width * channels; for( i = texture_width * channels; i > 0; --i ){ GLubyte temp = pData[index1]; pData[index1] = pData[index2]; pData[index2] = temp; ++index1; ++index2; } } GLenum format = GL_RGBA; switch(channels) { case 2: format = GL_RG32UI; break; case 3: format = GL_RGB; break; case 4: format = GL_RGBA; break; } glTexImage2D(GL_TEXTURE_2D, 0, format, texture_width, texture_height, 0, format, GL_UNSIGNED_BYTE, pData); SOIL_free_image_data(pData); textureMaps[filename]=id; } }
- Pass the loaded per-vertex attributes; that is, positions (
vertices
), texture coordinates (uvs
), per-vertex normals (normals
), and triangle indices (indices
) to GPU memory by allocating separate buffer objects for each attribute. Note that for easier handling of buffer objects, we bind a single vertex array object (vaoID
) first.glBindVertexArray(vaoID); glBindBuffer (GL_ARRAY_BUFFER, vboVerticesID); glBufferData (GL_ARRAY_BUFFER, sizeof(glm::vec3)* vertices.size(), &(vertices[0].x), GL_STATIC_DRAW); glEnableVertexAttribArray(shader["vVertex"]); glVertexAttribPointer(shader["vVertex"], 3, GL_FLOAT, GL_FALSE,0,0); glBindBuffer (GL_ARRAY_BUFFER, vboUVsID); glBufferData (GL_ARRAY_BUFFER, sizeof(glm::vec2)*uvs.size(), &(uvs[0].x), GL_STATIC_DRAW); glEnableVertexAttribArray(shader["vUV"]); glVertexAttribPointer(shader["vUV"],2,GL_FLOAT,GL_FALSE,0, 0); glBindBuffer (GL_ARRAY_BUFFER, vboNormalsID); glBufferData (GL_ARRAY_BUFFER, sizeof(glm::vec3)* normals.size(), &(normals[0].x), GL_STATIC_DRAW); glEnableVertexAttribArray(shader["vNormal"]); glVertexAttribPointer(shader["vNormal"], 3, GL_FLOAT, GL_FALSE, 0, 0);
- If we have only a single material in the 3ds file, we store the face indices into
GL_ELEMENT_ARRAY_BUFFER
so that we can render the whole mesh in a single call. However, if we have more than one material, we bind the appropriate submeshes separately. TheglBufferData
call allocates the GPU memory, however, it is not initialized. In order to initialize the buffer object memory, we can use theglMapBuffer
function to obtain a direct pointer to the GPU memory. Using this pointer, we can then write to the GPU memory. An alternative to usingglMapBuffer
isglBufferSubData
which can modify the GPU memory by copying contents from a CPU buffer.if(materials.size()==1) { glBindBuffer(GL_ELEMENT_ARRAY_BUFFER, vboIndicesID); glBufferData(GL_ELEMENT_ARRAY_BUFFER, sizeof(GLushort)* 3*faces.size(), 0, GL_STATIC_DRAW); GLushort* pIndices = static_cast<GLushort*>(glMapBuffer(GL_ELEMENT_ARRAY_BUFFER, GL_WRITE_ONLY)); for(size_t i=0;i<faces.size();i++) { *(pIndices++)=faces[i].a; *(pIndices++)=faces[i].b; *(pIndices++)=faces[i].c; } glUnmapBuffer(GL_ELEMENT_ARRAY_BUFFER); }
- Set up the vertex shader to output the clip space position as well as the per-vertex texture coordinates. The texture coordinates are then interpolated by the rasterizer to the fragment shader using an output attribute
vUVout
.#version 330 core layout(location = 0) in vec3 vVertex; layout(location = 1) in vec3 vNormal; layout(location = 2) in vec2 vUV; smooth out vec2 vUVout; uniform mat4 P; uniform mat4 MV; uniform mat3 N; smooth out vec3 vEyeSpaceNormal; smooth out vec3 vEyeSpacePosition; void main() { vUVout=vUV; vEyeSpacePosition = (MV*vec4(vVertex,1)).xyz; vEyeSpaceNormal = N*vNormal; gl_Position = P*vec4(vEyeSpacePosition,1); }
- Set up the fragment shader, which looks up the texture map sampler with the interpolated texture coordinates from the rasterizer. Depending on whether the submesh has a texture, we linearly interpolate between the texture map color and the diffused color of the material, using the GLSL mix function.
#version 330 core uniform sampler2D textureMap; uniform float hasTexture; uniform vec3 light_position;//light position in object space uniform mat4 MV; smooth in vec3 vEyeSpaceNormal; smooth in vec3 vEyeSpacePosition; smooth in vec2 vUVout; layout(location=0) out vec4 vFragColor; const float k0 = 1.0;//constant attenuation const float k1 = 0.0;//linear attenuation const float k2 = 0.0;//quadratic attenuation void main() { vec4 vEyeSpaceLightPosition = (MV*vec4(light_position,1)); vec3 L = (vEyeSpaceLightPosition.xyz-vEyeSpacePosition); float d = length(L); L = normalize(L); float diffuse = max(0, dot(vEyeSpaceNormal, L)); float attenuationAmount = 1.0/(k0 + (k1*d) + (k2*d*d)); diffuse *= attenuationAmount; vFragColor = diffuse*mix(vec4(1),texture(textureMap, vUVout), hasTexture); }
- The rendering code binds the shader program, sets the shader uniforms, and then renders the mesh, depending on how many materials the 3ds mesh has. If the mesh has only a single material, it is drawn in a single call to
glDrawElement
by using the indices attached to theGL_ELEMENT_ARRAY_BUFFER
binding point.glBindVertexArray(vaoID); { shader.Use(); glUniformMatrix4fv(shader("MV"), 1, GL_FALSE, glm::value_ptr(MV)); glUniformMatrix3fv(shader("N"), 1, GL_FALSE, glm::value_ptr(glm::inverseTranspose(glm::mat3(MV)))); glUniformMatrix4fv(shader("P"), 1, GL_FALSE, glm::value_ptr(P)); glUniform3fv(shader("light_position"),1, &(lightPosOS.x)); if(materials.size()==1) { GLint whichID[1]; glGetIntegerv(GL_TEXTURE_BINDING_2D, whichID); if(textureMaps.size()>0) { if(whichID[0] != textureMaps[materials[0]->textureMaps[0]->filename]) { glBindTexture(GL_TEXTURE_2D, textureMaps[materials[0]->textureMaps[0]->filename]); glUniform1f(shader("hasTexture"),1.0); } } else { glUniform1f(shader("hasTexture"),0.0); glUniform3fv(shader("diffuse_color"),1, materials[0]->diffuse); } glDrawElements(GL_TRIANGLES, meshes[0]->faces.size()*3, GL_UNSIGNED_SHORT, 0); }
- If the mesh contains more than one material, we iterate through the material list, and bind the texture map (if the material has one), otherwise we use the diffuse color stored in the material for the submesh. Finally, we pass the
sub_indices
array stored in the material to theglDrawElements
function to load those indices only.else { for(size_t i=0;i<materials.size();i++) { GLint whichID[1]; glGetIntegerv(GL_TEXTURE_BINDING_2D, whichID); if(materials[i]->textureMaps.size()>0) { if(whichID[0] != textureMaps[materials[i]->textureMaps[0]->filename]) { glBindTexture(GL_TEXTURE_2D, textureMaps[materials[i]->textureMaps[0]->filename]); } glUniform1f(shader("hasTexture"),1.0); } else { glUniform1f(shader("hasTexture"),0.0); } glUniform3fv(shader("diffuse_color"),1, materials[i]->diffuse); glDrawElements(GL_TRIANGLES, materials[i]->sub_indices.size(), GL_UNSIGNED_SHORT, &(materials[i]->sub_indices[0])); } } shader.UnUse();
The main component of this recipe is the C3dsLoader::Load3DS
function. The 3ds file is a binary file which is organized into a collection of chunks. Typically, a reader reads the first two bytes from the file which are stored in the chunk ID. The next four bytes store the chunk length in bytes. We continue reading chunks, and their lengths, and then store data appropriately into our vectors/variables until there are no more chunks and we pass reading the end of file. The 3ds specifications detail all of the chunks and their lengths as well as subchunks, as shown in the following figure:
Note that if there is a subchunk that we are interested in, we need to read the parent chunk as well, to move the file pointer to the appropriate offset in the file, for our required chunk. The loader first finds the total size of the 3ds mesh file in bytes. Then, it runs a while loop that checks to see if the current file pointer is within the file's size. If it is, it continues to read the first two bytes (the chunk's ID) and the next four bytes (the chunk's length).
All names (object name, material name, or texture map name) have to be read byte-by-byte until the null terminator character (\0
) is found. For reading vertices, we first read two bytes that store the total number of vertices (N
). Two bytes means that the maximum number of vertices one mesh can store is 65536. Then, we read the whole chunk of bytes, that is, sizeof(glm::vec3)*N
, directly into our mesh's vertices, shown as follows:
Note that the 3ds format does not store the per-vertex normal explicitly. It only stores smoothing groups which tell us which faces have shared normals. After we have the vertex positions and face information, we can generate the per-vertex normals by averaging the per-face normals. This is carried out by using the following code snippet in the 3ds.cpp
file. We first allocate space for the per-vertex normals. Then we estimate the face's normal by using the cross product of the two edges. Finally, we add the face normal to the appropriate vertex index and then normalize the normal.
Once we have all the per-vertex attributes and faces information, we use this to group the triangles by material. We loop through all of the materials and expand their face IDs to include the three vertex IDs and make the face.
required to implement a 3ds file viewer are as follows:
- Create an instance of the
C3dsLoader
class. Then call theC3dsLoader::Load3DS
function passing it the name of the mesh file and a set of vectors to store the submeshes, vertices, normals, uvs, indices, and materials.if(!loader.Load3DS(mesh_filename.c_str( ), meshes, vertices, normals, uvs, faces, indices, materials)) { cout<<"Cannot load the 3ds mesh"<<endl; exit(EXIT_FAILURE); }
- After the mesh is loaded, use the mesh's material list to load the material textures into the OpenGL texture object.
for(size_t k=0;k<materials.size();k++) { for(size_t m=0;m< materials[k]->textureMaps.size();m++) { GLuint id = 0; glGenTextures(1, &id); glBindTexture(GL_TEXTURE_2D, id); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MIN_FILTER, GL_LINEAR); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MAG_FILTER, GL_LINEAR); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_S, GL_REPEAT); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_T, GL_REPEAT); int texture_width = 0, texture_height = 0, channels=0; const string& filename = materials[k]->textureMaps[m]->filename; std::string full_filename = mesh_path; full_filename.append(filename); GLubyte* pData = SOIL_load_image(full_filename.c_str(), &texture_width, &texture_height, &channels, SOIL_LOAD_AUTO); if(pData == NULL) { cerr<<"Cannot load image: "<<full_filename.c_str()<<endl; exit(EXIT_FAILURE); } //Flip the image on Y axis int i,j; for( j = 0; j*2 < texture_height; ++j ) { int index1 = j * texture_width * channels; int index2 = (texture_height - 1 - j) * texture_width * channels; for( i = texture_width * channels; i > 0; --i ){ GLubyte temp = pData[index1]; pData[index1] = pData[index2]; pData[index2] = temp; ++index1; ++index2; } } GLenum format = GL_RGBA; switch(channels) { case 2: format = GL_RG32UI; break; case 3: format = GL_RGB; break; case 4: format = GL_RGBA; break; } glTexImage2D(GL_TEXTURE_2D, 0, format, texture_width, texture_height, 0, format, GL_UNSIGNED_BYTE, pData); SOIL_free_image_data(pData); textureMaps[filename]=id; } }
- Pass the loaded per-vertex attributes; that is, positions (
vertices
), texture coordinates (uvs
), per-vertex normals (normals
), and triangle indices (indices
) to GPU memory by allocating separate buffer objects for each attribute. Note that for easier handling of buffer objects, we bind a single vertex array object (vaoID
) first.glBindVertexArray(vaoID); glBindBuffer (GL_ARRAY_BUFFER, vboVerticesID); glBufferData (GL_ARRAY_BUFFER, sizeof(glm::vec3)* vertices.size(), &(vertices[0].x), GL_STATIC_DRAW); glEnableVertexAttribArray(shader["vVertex"]); glVertexAttribPointer(shader["vVertex"], 3, GL_FLOAT, GL_FALSE,0,0); glBindBuffer (GL_ARRAY_BUFFER, vboUVsID); glBufferData (GL_ARRAY_BUFFER, sizeof(glm::vec2)*uvs.size(), &(uvs[0].x), GL_STATIC_DRAW); glEnableVertexAttribArray(shader["vUV"]); glVertexAttribPointer(shader["vUV"],2,GL_FLOAT,GL_FALSE,0, 0); glBindBuffer (GL_ARRAY_BUFFER, vboNormalsID); glBufferData (GL_ARRAY_BUFFER, sizeof(glm::vec3)* normals.size(), &(normals[0].x), GL_STATIC_DRAW); glEnableVertexAttribArray(shader["vNormal"]); glVertexAttribPointer(shader["vNormal"], 3, GL_FLOAT, GL_FALSE, 0, 0);
- If we have only a single material in the 3ds file, we store the face indices into
GL_ELEMENT_ARRAY_BUFFER
so that we can render the whole mesh in a single call. However, if we have more than one material, we bind the appropriate submeshes separately. TheglBufferData
call allocates the GPU memory, however, it is not initialized. In order to initialize the buffer object memory, we can use theglMapBuffer
function to obtain a direct pointer to the GPU memory. Using this pointer, we can then write to the GPU memory. An alternative to usingglMapBuffer
isglBufferSubData
which can modify the GPU memory by copying contents from a CPU buffer.if(materials.size()==1) { glBindBuffer(GL_ELEMENT_ARRAY_BUFFER, vboIndicesID); glBufferData(GL_ELEMENT_ARRAY_BUFFER, sizeof(GLushort)* 3*faces.size(), 0, GL_STATIC_DRAW); GLushort* pIndices = static_cast<GLushort*>(glMapBuffer(GL_ELEMENT_ARRAY_BUFFER, GL_WRITE_ONLY)); for(size_t i=0;i<faces.size();i++) { *(pIndices++)=faces[i].a; *(pIndices++)=faces[i].b; *(pIndices++)=faces[i].c; } glUnmapBuffer(GL_ELEMENT_ARRAY_BUFFER); }
- Set up the vertex shader to output the clip space position as well as the per-vertex texture coordinates. The texture coordinates are then interpolated by the rasterizer to the fragment shader using an output attribute
vUVout
.#version 330 core layout(location = 0) in vec3 vVertex; layout(location = 1) in vec3 vNormal; layout(location = 2) in vec2 vUV; smooth out vec2 vUVout; uniform mat4 P; uniform mat4 MV; uniform mat3 N; smooth out vec3 vEyeSpaceNormal; smooth out vec3 vEyeSpacePosition; void main() { vUVout=vUV; vEyeSpacePosition = (MV*vec4(vVertex,1)).xyz; vEyeSpaceNormal = N*vNormal; gl_Position = P*vec4(vEyeSpacePosition,1); }
- Set up the fragment shader, which looks up the texture map sampler with the interpolated texture coordinates from the rasterizer. Depending on whether the submesh has a texture, we linearly interpolate between the texture map color and the diffused color of the material, using the GLSL mix function.
#version 330 core uniform sampler2D textureMap; uniform float hasTexture; uniform vec3 light_position;//light position in object space uniform mat4 MV; smooth in vec3 vEyeSpaceNormal; smooth in vec3 vEyeSpacePosition; smooth in vec2 vUVout; layout(location=0) out vec4 vFragColor; const float k0 = 1.0;//constant attenuation const float k1 = 0.0;//linear attenuation const float k2 = 0.0;//quadratic attenuation void main() { vec4 vEyeSpaceLightPosition = (MV*vec4(light_position,1)); vec3 L = (vEyeSpaceLightPosition.xyz-vEyeSpacePosition); float d = length(L); L = normalize(L); float diffuse = max(0, dot(vEyeSpaceNormal, L)); float attenuationAmount = 1.0/(k0 + (k1*d) + (k2*d*d)); diffuse *= attenuationAmount; vFragColor = diffuse*mix(vec4(1),texture(textureMap, vUVout), hasTexture); }
- The rendering code binds the shader program, sets the shader uniforms, and then renders the mesh, depending on how many materials the 3ds mesh has. If the mesh has only a single material, it is drawn in a single call to
glDrawElement
by using the indices attached to theGL_ELEMENT_ARRAY_BUFFER
binding point.glBindVertexArray(vaoID); { shader.Use(); glUniformMatrix4fv(shader("MV"), 1, GL_FALSE, glm::value_ptr(MV)); glUniformMatrix3fv(shader("N"), 1, GL_FALSE, glm::value_ptr(glm::inverseTranspose(glm::mat3(MV)))); glUniformMatrix4fv(shader("P"), 1, GL_FALSE, glm::value_ptr(P)); glUniform3fv(shader("light_position"),1, &(lightPosOS.x)); if(materials.size()==1) { GLint whichID[1]; glGetIntegerv(GL_TEXTURE_BINDING_2D, whichID); if(textureMaps.size()>0) { if(whichID[0] != textureMaps[materials[0]->textureMaps[0]->filename]) { glBindTexture(GL_TEXTURE_2D, textureMaps[materials[0]->textureMaps[0]->filename]); glUniform1f(shader("hasTexture"),1.0); } } else { glUniform1f(shader("hasTexture"),0.0); glUniform3fv(shader("diffuse_color"),1, materials[0]->diffuse); } glDrawElements(GL_TRIANGLES, meshes[0]->faces.size()*3, GL_UNSIGNED_SHORT, 0); }
- If the mesh contains more than one material, we iterate through the material list, and bind the texture map (if the material has one), otherwise we use the diffuse color stored in the material for the submesh. Finally, we pass the
sub_indices
array stored in the material to theglDrawElements
function to load those indices only.else { for(size_t i=0;i<materials.size();i++) { GLint whichID[1]; glGetIntegerv(GL_TEXTURE_BINDING_2D, whichID); if(materials[i]->textureMaps.size()>0) { if(whichID[0] != textureMaps[materials[i]->textureMaps[0]->filename]) { glBindTexture(GL_TEXTURE_2D, textureMaps[materials[i]->textureMaps[0]->filename]); } glUniform1f(shader("hasTexture"),1.0); } else { glUniform1f(shader("hasTexture"),0.0); } glUniform3fv(shader("diffuse_color"),1, materials[i]->diffuse); glDrawElements(GL_TRIANGLES, materials[i]->sub_indices.size(), GL_UNSIGNED_SHORT, &(materials[i]->sub_indices[0])); } } shader.UnUse();
The main component of this recipe is the C3dsLoader::Load3DS
function. The 3ds file is a binary file which is organized into a collection of chunks. Typically, a reader reads the first two bytes from the file which are stored in the chunk ID. The next four bytes store the chunk length in bytes. We continue reading chunks, and their lengths, and then store data appropriately into our vectors/variables until there are no more chunks and we pass reading the end of file. The 3ds specifications detail all of the chunks and their lengths as well as subchunks, as shown in the following figure:
Note that if there is a subchunk that we are interested in, we need to read the parent chunk as well, to move the file pointer to the appropriate offset in the file, for our required chunk. The loader first finds the total size of the 3ds mesh file in bytes. Then, it runs a while loop that checks to see if the current file pointer is within the file's size. If it is, it continues to read the first two bytes (the chunk's ID) and the next four bytes (the chunk's length).
All names (object name, material name, or texture map name) have to be read byte-by-byte until the null terminator character (\0
) is found. For reading vertices, we first read two bytes that store the total number of vertices (N
). Two bytes means that the maximum number of vertices one mesh can store is 65536. Then, we read the whole chunk of bytes, that is, sizeof(glm::vec3)*N
, directly into our mesh's vertices, shown as follows:
Note that the 3ds format does not store the per-vertex normal explicitly. It only stores smoothing groups which tell us which faces have shared normals. After we have the vertex positions and face information, we can generate the per-vertex normals by averaging the per-face normals. This is carried out by using the following code snippet in the 3ds.cpp
file. We first allocate space for the per-vertex normals. Then we estimate the face's normal by using the cross product of the two edges. Finally, we add the face normal to the appropriate vertex index and then normalize the normal.
Once we have all the per-vertex attributes and faces information, we use this to group the triangles by material. We loop through all of the materials and expand their face IDs to include the three vertex IDs and make the face.
C3dsLoader::Load3DS
function. The 3ds file is a binary file which is organized into a collection of chunks. Typically, a reader reads the first two bytes from the file which are stored in the chunk ID. The next four bytes store the chunk length in bytes. We continue reading chunks, and their lengths, and then store data appropriately into our vectors/variables until there are no more chunks and we pass reading the end of file. The 3ds specifications detail all of the chunks and their lengths as well as subchunks, as shown in the following figure:
Note that if there is a subchunk that we are interested in, we need to read the parent chunk as well, to move the file pointer to the appropriate offset in the file, for our required chunk. The loader first finds the total size of the 3ds mesh file in bytes. Then, it runs a while loop that checks to see if the current file pointer is within the file's size. If it is, it continues to read the first two bytes (the chunk's ID) and the next four bytes (the chunk's length).
All names (object name, material name, or texture map name) have to be read byte-by-byte until the null terminator character (\0
) is found. For reading vertices, we first read two bytes that store the total number of vertices (N
). Two bytes means that the maximum number of vertices one mesh can store is 65536. Then, we read the whole chunk of bytes, that is, sizeof(glm::vec3)*N
, directly into our mesh's vertices, shown as follows:
Note that the 3ds format does not store the per-vertex normal explicitly. It only stores smoothing groups which tell us which faces have shared normals. After we have the vertex positions and face information, we can generate the per-vertex normals by averaging the per-face normals. This is carried out by using the following code snippet in the 3ds.cpp
file. We first allocate space for the per-vertex normals. Then we estimate the face's normal by using the cross product of the two edges. Finally, we add the face normal to the appropriate vertex index and then normalize the normal.
Once we have all the per-vertex attributes and faces information, we use this to group the triangles by material. We loop through all of the materials and expand their face IDs to include the three vertex IDs and make the face.
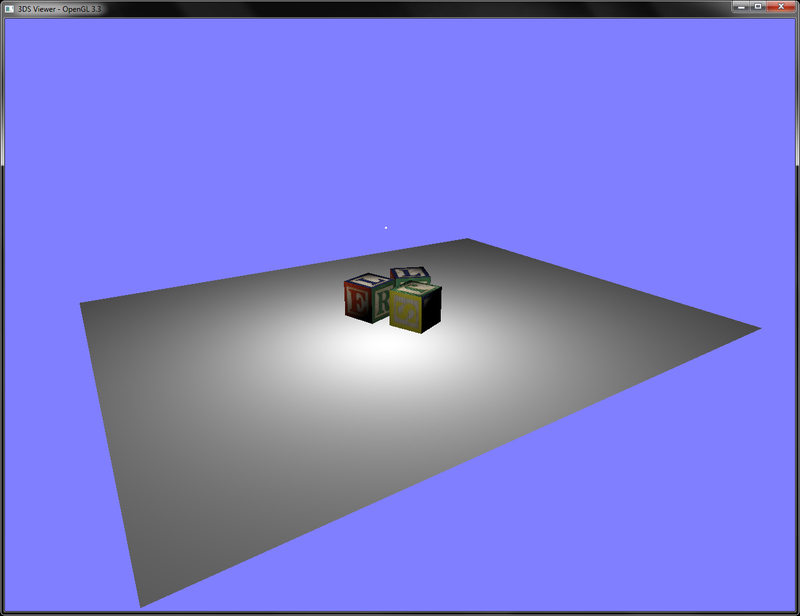
lib3ds
library which provides a more elaborate 3ds file loader with support for smoothing groups, animation tracks, cameras, lights, keyframes, and so on.
See also
In this recipe we will implement the Wavefront ® OBJ model. Instead of using separate buffer objects for storing positions, normals, and texture coordinates as in the previous recipe, we will use a single buffer object with interleaved data. This ensures that we have more chances of a cache hit since related attributes are stored next to each other in the buffer object memory.
Let us start the recipe by following these simple steps:
- Create a global reference of the
ObjLoader
object. Call theObjLoader::Load
function, passing it the name of the OBJ file. Pass vectors to store the meshes, vertices, indices, and materials contained in the OBJ file.ObjLoader obj; if(!obj.Load(mesh_filename.c_str(), meshes, vertices, indices, materials)) { cout<<"Cannot load the 3ds mesh"<<endl; exit(EXIT_FAILURE); }
- Generate OpenGL texture objects for each material using the
SOIL
library if the material has a texture map.for(size_t k=0;k<materials.size();k++) { if(materials[k]->map_Kd != "") { GLuint id = 0; glGenTextures(1, &id); glBindTexture(GL_TEXTURE_2D, id); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MIN_FILTER, GL_LINEAR); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MAG_FILTER, GL_LINEAR); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_S, GL_REPEAT); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_T, GL_REPEAT); int texture_width = 0, texture_height = 0, channels=0; const string& filename = materials[k]->map_Kd; std::string full_filename = mesh_path; full_filename.append(filename); GLubyte* pData = SOIL_load_image(full_filename.c_str(), &texture_width, &texture_height, &channels, SOIL_LOAD_AUTO); if(pData == NULL) { cerr<<"Cannot load image: "<<full_filename.c_str()<<endl; exit(EXIT_FAILURE); } //… image flipping code GLenum format = GL_RGBA; switch(channels) { case 2: format = GL_RG32UI; break; case 3: format = GL_RGB; break; case 4: format = GL_RGBA; break; } glTexImage2D(GL_TEXTURE_2D, 0, format, texture_width, texture_height, 0, format, GL_UNSIGNED_BYTE, pData); SOIL_free_image_data(pData); textures.push_back(id); } }
- Set up shaders and generate buffer objects to store the mesh file data in the GPU memory. The shader setup is similar to the previous recipes.
glGenVertexArrays(1, &vaoID); glGenBuffers(1, &vboVerticesID); glGenBuffers(1, &vboIndicesID); glBindVertexArray(vaoID); glBindBuffer (GL_ARRAY_BUFFER, vboVerticesID); glBufferData (GL_ARRAY_BUFFER, sizeof(Vertex)*vertices.size(), &(vertices[0].pos.x), GL_STATIC_DRAW); glEnableVertexAttribArray(shader["vVertex"]); glVertexAttribPointer(shader["vVertex"], 3, GL_FLOAT, GL_FALSE,sizeof(Vertex),0); glEnableVertexAttribArray(shader["vNormal"]); glVertexAttribPointer(shader["vNormal"], 3, GL_FLOAT, GL_FALSE,sizeof(Vertex),(const GLvoid*)(offsetof( Vertex, normal)) ); glEnableVertexAttribArray(shader["vUV"]); glVertexAttribPointer(shader["vUV"], 2, GL_FLOAT, GL_FALSE, sizeof(Vertex), (const GLvoid*)(offsetof(Vertex, uv)) ); if(materials.size()==1) { glBindBuffer(GL_ELEMENT_ARRAY_BUFFER, vboIndicesID); glBufferData(GL_ELEMENT_ARRAY_BUFFER, sizeof(GLushort)*indices.size(), &(indices[0]), GL_STATIC_DRAW); }
- Bind the vertex array object associated with the mesh, use the shader and pass the shader uniforms, that is, the modelview (
MV
), projection (P
), normal matrices (N
) and light position, and so on.glBindVertexArray(vaoID); { shader.Use(); glUniformMatrix4fv(shader("MV"), 1, GL_FALSE, glm::value_ptr(MV)); glUniformMatrix3fv(shader("N"), 1, GL_FALSE, glm::value_ptr(glm::inverseTranspose(glm::mat3(MV)))); glUniformMatrix4fv(shader("P"), 1, GL_FALSE, glm::value_ptr(P)); glUniform3fv(shader("light_position"),1, &(lightPosOS.x));
- To draw the mesh/submesh, loop through all of the materials in the mesh and then bind the texture to the
GL_TEXTURE_2D
target if the material contains a texture map. Otherwise, use a default color for the mesh. Finally, call theglDrawElements
function to render the mesh/submesh.for(size_t i=0;i<materials.size();i++) { Material* pMat = materials[i]; if(pMat->map_Kd !="") { glUniform1f(shader("useDefault"), 0.0); GLint whichID[1]; glGetIntegerv(GL_TEXTURE_BINDING_2D, whichID); if(whichID[0] != textures[i]) glBindTexture(GL_TEXTURE_2D, textures[i]); } else glUniform1f(shader("useDefault"), 1.0); if(materials.size()==1) glDrawElements(GL_TRIANGLES, indices.size(), GL_UNSIGNED_SHORT, 0); else glDrawElements(GL_TRIANGLES, pMat->count, GL_UNSIGNED_SHORT, (const GLvoid*)(& indices[pMat->offset])); } shader.UnUse();
Following the geometry definition, the topology is defined. In this case, the line is prefixed with f
followed by the indices for the polygon vertices. In case of a triangle, three indices sections are given such that the vertex position indices are given first, followed by texture coordinates indices (if any), and finally the normal indices (if any). Note that the indices start from 1, not 0.
We generate the vertex array object and then the vertex buffer object. Next, we bind the buffer object passing it our vertices. In this case, we specify the stride of each attribute in the data stream separately as follows:
Chapter5/ObjViewer
folder.
Let us start the recipe by following these simple steps:
- Create a global reference of the
ObjLoader
object. Call theObjLoader::Load
function, passing it the name of the OBJ file. Pass vectors to store the meshes, vertices, indices, and materials contained in the OBJ file.ObjLoader obj; if(!obj.Load(mesh_filename.c_str(), meshes, vertices, indices, materials)) { cout<<"Cannot load the 3ds mesh"<<endl; exit(EXIT_FAILURE); }
- Generate OpenGL texture objects for each material using the
SOIL
library if the material has a texture map.for(size_t k=0;k<materials.size();k++) { if(materials[k]->map_Kd != "") { GLuint id = 0; glGenTextures(1, &id); glBindTexture(GL_TEXTURE_2D, id); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MIN_FILTER, GL_LINEAR); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MAG_FILTER, GL_LINEAR); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_S, GL_REPEAT); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_T, GL_REPEAT); int texture_width = 0, texture_height = 0, channels=0; const string& filename = materials[k]->map_Kd; std::string full_filename = mesh_path; full_filename.append(filename); GLubyte* pData = SOIL_load_image(full_filename.c_str(), &texture_width, &texture_height, &channels, SOIL_LOAD_AUTO); if(pData == NULL) { cerr<<"Cannot load image: "<<full_filename.c_str()<<endl; exit(EXIT_FAILURE); } //… image flipping code GLenum format = GL_RGBA; switch(channels) { case 2: format = GL_RG32UI; break; case 3: format = GL_RGB; break; case 4: format = GL_RGBA; break; } glTexImage2D(GL_TEXTURE_2D, 0, format, texture_width, texture_height, 0, format, GL_UNSIGNED_BYTE, pData); SOIL_free_image_data(pData); textures.push_back(id); } }
- Set up shaders and generate buffer objects to store the mesh file data in the GPU memory. The shader setup is similar to the previous recipes.
glGenVertexArrays(1, &vaoID); glGenBuffers(1, &vboVerticesID); glGenBuffers(1, &vboIndicesID); glBindVertexArray(vaoID); glBindBuffer (GL_ARRAY_BUFFER, vboVerticesID); glBufferData (GL_ARRAY_BUFFER, sizeof(Vertex)*vertices.size(), &(vertices[0].pos.x), GL_STATIC_DRAW); glEnableVertexAttribArray(shader["vVertex"]); glVertexAttribPointer(shader["vVertex"], 3, GL_FLOAT, GL_FALSE,sizeof(Vertex),0); glEnableVertexAttribArray(shader["vNormal"]); glVertexAttribPointer(shader["vNormal"], 3, GL_FLOAT, GL_FALSE,sizeof(Vertex),(const GLvoid*)(offsetof( Vertex, normal)) ); glEnableVertexAttribArray(shader["vUV"]); glVertexAttribPointer(shader["vUV"], 2, GL_FLOAT, GL_FALSE, sizeof(Vertex), (const GLvoid*)(offsetof(Vertex, uv)) ); if(materials.size()==1) { glBindBuffer(GL_ELEMENT_ARRAY_BUFFER, vboIndicesID); glBufferData(GL_ELEMENT_ARRAY_BUFFER, sizeof(GLushort)*indices.size(), &(indices[0]), GL_STATIC_DRAW); }
- Bind the vertex array object associated with the mesh, use the shader and pass the shader uniforms, that is, the modelview (
MV
), projection (P
), normal matrices (N
) and light position, and so on.glBindVertexArray(vaoID); { shader.Use(); glUniformMatrix4fv(shader("MV"), 1, GL_FALSE, glm::value_ptr(MV)); glUniformMatrix3fv(shader("N"), 1, GL_FALSE, glm::value_ptr(glm::inverseTranspose(glm::mat3(MV)))); glUniformMatrix4fv(shader("P"), 1, GL_FALSE, glm::value_ptr(P)); glUniform3fv(shader("light_position"),1, &(lightPosOS.x));
- To draw the mesh/submesh, loop through all of the materials in the mesh and then bind the texture to the
GL_TEXTURE_2D
target if the material contains a texture map. Otherwise, use a default color for the mesh. Finally, call theglDrawElements
function to render the mesh/submesh.for(size_t i=0;i<materials.size();i++) { Material* pMat = materials[i]; if(pMat->map_Kd !="") { glUniform1f(shader("useDefault"), 0.0); GLint whichID[1]; glGetIntegerv(GL_TEXTURE_BINDING_2D, whichID); if(whichID[0] != textures[i]) glBindTexture(GL_TEXTURE_2D, textures[i]); } else glUniform1f(shader("useDefault"), 1.0); if(materials.size()==1) glDrawElements(GL_TRIANGLES, indices.size(), GL_UNSIGNED_SHORT, 0); else glDrawElements(GL_TRIANGLES, pMat->count, GL_UNSIGNED_SHORT, (const GLvoid*)(& indices[pMat->offset])); } shader.UnUse();
Following the geometry definition, the topology is defined. In this case, the line is prefixed with f
followed by the indices for the polygon vertices. In case of a triangle, three indices sections are given such that the vertex position indices are given first, followed by texture coordinates indices (if any), and finally the normal indices (if any). Note that the indices start from 1, not 0.
We generate the vertex array object and then the vertex buffer object. Next, we bind the buffer object passing it our vertices. In this case, we specify the stride of each attribute in the data stream separately as follows:
the recipe by following these simple steps:
- Create a global reference of the
ObjLoader
object. Call theObjLoader::Load
function, passing it the name of the OBJ file. Pass vectors to store the meshes, vertices, indices, and materials contained in the OBJ file.ObjLoader obj; if(!obj.Load(mesh_filename.c_str(), meshes, vertices, indices, materials)) { cout<<"Cannot load the 3ds mesh"<<endl; exit(EXIT_FAILURE); }
- Generate OpenGL texture objects for each material using the
SOIL
library if the material has a texture map.for(size_t k=0;k<materials.size();k++) { if(materials[k]->map_Kd != "") { GLuint id = 0; glGenTextures(1, &id); glBindTexture(GL_TEXTURE_2D, id); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MIN_FILTER, GL_LINEAR); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MAG_FILTER, GL_LINEAR); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_S, GL_REPEAT); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_T, GL_REPEAT); int texture_width = 0, texture_height = 0, channels=0; const string& filename = materials[k]->map_Kd; std::string full_filename = mesh_path; full_filename.append(filename); GLubyte* pData = SOIL_load_image(full_filename.c_str(), &texture_width, &texture_height, &channels, SOIL_LOAD_AUTO); if(pData == NULL) { cerr<<"Cannot load image: "<<full_filename.c_str()<<endl; exit(EXIT_FAILURE); } //… image flipping code GLenum format = GL_RGBA; switch(channels) { case 2: format = GL_RG32UI; break; case 3: format = GL_RGB; break; case 4: format = GL_RGBA; break; } glTexImage2D(GL_TEXTURE_2D, 0, format, texture_width, texture_height, 0, format, GL_UNSIGNED_BYTE, pData); SOIL_free_image_data(pData); textures.push_back(id); } }
- Set up shaders and generate buffer objects to store the mesh file data in the GPU memory. The shader setup is similar to the previous recipes.
glGenVertexArrays(1, &vaoID); glGenBuffers(1, &vboVerticesID); glGenBuffers(1, &vboIndicesID); glBindVertexArray(vaoID); glBindBuffer (GL_ARRAY_BUFFER, vboVerticesID); glBufferData (GL_ARRAY_BUFFER, sizeof(Vertex)*vertices.size(), &(vertices[0].pos.x), GL_STATIC_DRAW); glEnableVertexAttribArray(shader["vVertex"]); glVertexAttribPointer(shader["vVertex"], 3, GL_FLOAT, GL_FALSE,sizeof(Vertex),0); glEnableVertexAttribArray(shader["vNormal"]); glVertexAttribPointer(shader["vNormal"], 3, GL_FLOAT, GL_FALSE,sizeof(Vertex),(const GLvoid*)(offsetof( Vertex, normal)) ); glEnableVertexAttribArray(shader["vUV"]); glVertexAttribPointer(shader["vUV"], 2, GL_FLOAT, GL_FALSE, sizeof(Vertex), (const GLvoid*)(offsetof(Vertex, uv)) ); if(materials.size()==1) { glBindBuffer(GL_ELEMENT_ARRAY_BUFFER, vboIndicesID); glBufferData(GL_ELEMENT_ARRAY_BUFFER, sizeof(GLushort)*indices.size(), &(indices[0]), GL_STATIC_DRAW); }
- Bind the vertex array object associated with the mesh, use the shader and pass the shader uniforms, that is, the modelview (
MV
), projection (P
), normal matrices (N
) and light position, and so on.glBindVertexArray(vaoID); { shader.Use(); glUniformMatrix4fv(shader("MV"), 1, GL_FALSE, glm::value_ptr(MV)); glUniformMatrix3fv(shader("N"), 1, GL_FALSE, glm::value_ptr(glm::inverseTranspose(glm::mat3(MV)))); glUniformMatrix4fv(shader("P"), 1, GL_FALSE, glm::value_ptr(P)); glUniform3fv(shader("light_position"),1, &(lightPosOS.x));
- To draw the mesh/submesh, loop through all of the materials in the mesh and then bind the texture to the
GL_TEXTURE_2D
target if the material contains a texture map. Otherwise, use a default color for the mesh. Finally, call theglDrawElements
function to render the mesh/submesh.for(size_t i=0;i<materials.size();i++) { Material* pMat = materials[i]; if(pMat->map_Kd !="") { glUniform1f(shader("useDefault"), 0.0); GLint whichID[1]; glGetIntegerv(GL_TEXTURE_BINDING_2D, whichID); if(whichID[0] != textures[i]) glBindTexture(GL_TEXTURE_2D, textures[i]); } else glUniform1f(shader("useDefault"), 1.0); if(materials.size()==1) glDrawElements(GL_TRIANGLES, indices.size(), GL_UNSIGNED_SHORT, 0); else glDrawElements(GL_TRIANGLES, pMat->count, GL_UNSIGNED_SHORT, (const GLvoid*)(& indices[pMat->offset])); } shader.UnUse();
Following the geometry definition, the topology is defined. In this case, the line is prefixed with f
followed by the indices for the polygon vertices. In case of a triangle, three indices sections are given such that the vertex position indices are given first, followed by texture coordinates indices (if any), and finally the normal indices (if any). Note that the indices start from 1, not 0.
We generate the vertex array object and then the vertex buffer object. Next, we bind the buffer object passing it our vertices. In this case, we specify the stride of each attribute in the data stream separately as follows:
ObjLoader::Load
function defined in the Obj.cpp
file. The Wavefront® OBJ file is a text file which has different text descriptors for different mesh components. Usually, the mesh starts with the geometry definition, that is, vertices that begin with the letter v
followed by three floating point values. If there are normals, their definitions begin with vn
followed by three floating point values. If there are texture coordinates, their definitions begin with vt
, followed by two floating point values. Comments start with the #
character, so whenever a line with this character is encountered, it is ignored.
geometry definition, the topology is defined. In this case, the line is prefixed with f
followed by the indices for the polygon vertices. In case of a triangle, three indices sections are given such that the vertex position indices are given first, followed by texture coordinates indices (if any), and finally the normal indices (if any). Note that the indices start from 1, not 0.
We generate the vertex array object and then the vertex buffer object. Next, we bind the buffer object passing it our vertices. In this case, we specify the stride of each attribute in the data stream separately as follows:
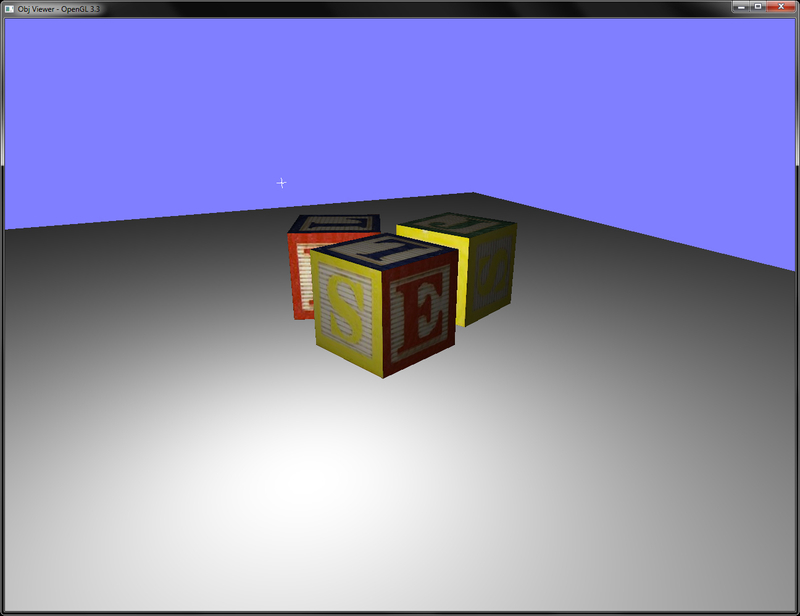
In this recipe, we will learn how to load and render an EZMesh model. There are several skeletal animation formats such as Quake's md2 (.md2
), Autodesk® FBX (.fbx
), and Collada (.dae
). The conventional model formats such as Collada are overly complicated for doing simple skeletal animation. Therefore, in this recipe, we will learn how to load and render an EZMesh (.ezm
) skeletal model.
The code for this recipe is contained in the Chapter5/EZMeshViewer
directory. For this recipe, we will be using two external libraries to aid with the EZMesh (.ezm
) mesh file parsing. The first library is called MeshImport
and it can be downloaded from http://code.google.com/p/meshimport/. Make sure to get the latest svn trunk of the code. After downloading, change directory to the compiler subdirectory which contains the visual studio solution files. Double-click to open the solution and build the project dlls. After the library is built successfully, copy MeshImport_[x86/x64].dll
and MeshImportEZM_[x86/x64].dll
(subject to your machine configuration) into your current project directory. In addition, also copy the MeshImport.[h/cpp]
files which contain some useful library loading routines.
In addition, since EZMesh is an XML format to support loading of textures, we parse the EZMesh XML manually with the help of the pugixml
library. You can download it from http://pugixml.org/downloads/. As pugixml
is tiny, we can directly include the source files with the project.
Let us start this recipe by following these simple steps:
- Create a global reference to an
EzmLoader
object. Call theEzmLoader::Load
function passing it the name of the EZMesh (.ezm
) file. Pass the vectors to store the submeshes, vertices, indices, and materials-to-image map. TheLoad
function also accepts the min and max vectors to store the EZMesh bounding box.if(!ezm.Load(mesh_filename.c_str(), submeshes, vertices, indices, material2ImageMap, min, max)) { cout<<"Cannot load the EZMesh mesh"<<endl; exit(EXIT_FAILURE); }
- Using the material information, generate the OpenGL textures for the EZMesh geometry.
for(size_t k=0;k<materialNames.size();k++) { GLuint id = 0; glGenTextures(1, &id); glBindTexture(GL_TEXTURE_2D, id); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MIN_FILTER, GL_LINEAR); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MAG_FILTER, GL_LINEAR); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_S, GL_REPEAT); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_T, GL_REPEAT); int texture_width = 0, texture_height = 0, channels=0; const string& filename = materialNames[k]; std::string full_filename = mesh_path; full_filename.append(filename); //Image loading using SOIL and vertical image flipping //… GLenum format = GL_RGBA; switch(channels) { case 2: format = GL_RG32UI; break; case 3: format = GL_RGB; break; case 4: format = GL_RGBA; break; } glTexImage2D(GL_TEXTURE_2D, 0, format, texture_width, texture_height, 0, format, GL_UNSIGNED_BYTE, pData); SOIL_free_image_data(pData); materialMap[filename] = id ; }
- Set up the interleaved buffer object as in the previous recipe, Implementing OBJ model loading using interleaved buffers.
glBindVertexArray(vaoID); glBindBuffer (GL_ARRAY_BUFFER, vboVerticesID); glBufferData (GL_ARRAY_BUFFER, sizeof(Vertex)*vertices.size(), &(vertices[0].pos.x), GL_DYNAMIC_DRAW); glEnableVertexAttribArray(shader["vVertex"]); glVertexAttribPointer(shader["vVertex"], 3, GL_FLOAT, GL_FALSE,sizeof(Vertex),0); glEnableVertexAttribArray(shader["vNormal"]); glVertexAttribPointer(shader["vNormal"], 3, GL_FLOAT, GL_FALSE, sizeof(Vertex), (const GLvoid*)(offsetof(Vertex, normal)) ); glEnableVertexAttribArray(shader["vUV"]); glVertexAttribPointer(shader["vUV"], 2, GL_FLOAT, GL_FALSE, sizeof(Vertex), (const GLvoid*)(offsetof(Vertex, uv)) );
- To render the EZMesh, bind the mesh's vertex array object, set up the shader, and pass the shader uniforms.
glBindVertexArray(vaoID); { shader.Use(); glUniformMatrix4fv(shader("MV"), 1, GL_FALSE, glm::value_ptr(MV)); glUniformMatrix3fv(shader("N"), 1, GL_FALSE, glm::value_ptr(glm::inverseTranspose(glm::mat3(MV)))); glUniformMatrix4fv(shader("P"), 1, GL_FALSE, glm::value_ptr(P)); glUniform3fv(shader("light_position"),1, &(lightPosES.x));
- Loop through all submeshes, bind the submesh texture, and then issue the
glDrawEements
call, passing it the submesh indices. If the submesh has no materials, a default solid color material is assigned to the submesh.for(size_t i=0;i<submeshes.size();i++) { if(strlen(submeshes[i].materialName)>0) { GLuint id = materialMap[material2ImageMap[ submeshes[i].materialName]]; GLint whichID[1]; glGetIntegerv(GL_TEXTURE_BINDING_2D, whichID); if(whichID[0] != id) glBindTexture(GL_TEXTURE_2D, id); glUniform1f(shader("useDefault"), 0.0); } else { glUniform1f(shader("useDefault"), 1.0); } glDrawElements(GL_TRIANGLES, submeshes[i].indices.size(), GL_UNSIGNED_INT, &submeshes[i].indices[0]); } }
EZMesh is an XML based skeletal animation format. There are two parts to this recipe: parsing of the EZMesh
file using the MeshImport
/pugixml
libraries and handling of the data using OpenGL buffer objects. The first part is handled by the EzmLoader::Load
function. Along with the filename, this function accepts vectors to store the submeshes, vertices, indices, and material names map contained in the mesh file.
For this recipe, we are interested in the last two subelements: Materials
and Meshes
. We will be using the first two subelements in the skeletal animation recipe in a later chapter of this book. Each Materials
element has a counted number of Material
elements. Each Material
element stores the material's name in the name attribute and the material's details. For example, the texture map file name in the meta_data
attribute. In the EZMLoader::Load
function, we use pugi_xml
to parse the Materials
element and its subelements into a material map. This map stores the material's name and its texture file name. Note that the MeshImport
library does provide functions for reading material information, but they are broken.
Once we have the MeshSystem
object, we can query all of the subelements. These reside in the MeshSystem
object as member variables. So let's say we want to traverse through all of the meshes in the current EZMesh
file and copy the per-vertex attributes to our own vector (vertices
), we would simply do the following:
After the materials, the shaders are loaded as in the previous recipes. The per-vertex data is then transferred to the GPU using vertex array and vertex buffer objects. In this case, we use the interleaved vertex buffer format.
For rendering of the mesh, we first bind the vertex array object of the mesh, attach our shader and pass the shader uniforms. Then we loop over all of the submeshes
and bind the appropriate texture (if the submesh has texture). Otherwise, a default color is used. Finally, the indices of the submesh are used to draw the mesh using the glDrawElements
function.
Chapter5/EZMeshViewer
directory. For this recipe, we will be using two external libraries to aid with the EZMesh (.ezm
) mesh file parsing. The first library is called MeshImport
and it can be downloaded from
http://code.google.com/p/meshimport/. Make sure to get the latest svn trunk of the code. After downloading, change directory to the compiler subdirectory which contains the visual studio solution files. Double-click to open the solution and build the project dlls. After the library is built successfully, copy MeshImport_[x86/x64].dll
and MeshImportEZM_[x86/x64].dll
(subject to your machine configuration) into your current project directory. In addition, also copy the MeshImport.[h/cpp]
files which contain some useful library loading routines.
In addition, since EZMesh is an XML format to support loading of textures, we parse the EZMesh XML manually with the help of the pugixml
library. You can download it from http://pugixml.org/downloads/. As pugixml
is tiny, we can directly include the source files with the project.
Let us start this recipe by following these simple steps:
- Create a global reference to an
EzmLoader
object. Call theEzmLoader::Load
function passing it the name of the EZMesh (.ezm
) file. Pass the vectors to store the submeshes, vertices, indices, and materials-to-image map. TheLoad
function also accepts the min and max vectors to store the EZMesh bounding box.if(!ezm.Load(mesh_filename.c_str(), submeshes, vertices, indices, material2ImageMap, min, max)) { cout<<"Cannot load the EZMesh mesh"<<endl; exit(EXIT_FAILURE); }
- Using the material information, generate the OpenGL textures for the EZMesh geometry.
for(size_t k=0;k<materialNames.size();k++) { GLuint id = 0; glGenTextures(1, &id); glBindTexture(GL_TEXTURE_2D, id); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MIN_FILTER, GL_LINEAR); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MAG_FILTER, GL_LINEAR); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_S, GL_REPEAT); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_T, GL_REPEAT); int texture_width = 0, texture_height = 0, channels=0; const string& filename = materialNames[k]; std::string full_filename = mesh_path; full_filename.append(filename); //Image loading using SOIL and vertical image flipping //… GLenum format = GL_RGBA; switch(channels) { case 2: format = GL_RG32UI; break; case 3: format = GL_RGB; break; case 4: format = GL_RGBA; break; } glTexImage2D(GL_TEXTURE_2D, 0, format, texture_width, texture_height, 0, format, GL_UNSIGNED_BYTE, pData); SOIL_free_image_data(pData); materialMap[filename] = id ; }
- Set up the interleaved buffer object as in the previous recipe, Implementing OBJ model loading using interleaved buffers.
glBindVertexArray(vaoID); glBindBuffer (GL_ARRAY_BUFFER, vboVerticesID); glBufferData (GL_ARRAY_BUFFER, sizeof(Vertex)*vertices.size(), &(vertices[0].pos.x), GL_DYNAMIC_DRAW); glEnableVertexAttribArray(shader["vVertex"]); glVertexAttribPointer(shader["vVertex"], 3, GL_FLOAT, GL_FALSE,sizeof(Vertex),0); glEnableVertexAttribArray(shader["vNormal"]); glVertexAttribPointer(shader["vNormal"], 3, GL_FLOAT, GL_FALSE, sizeof(Vertex), (const GLvoid*)(offsetof(Vertex, normal)) ); glEnableVertexAttribArray(shader["vUV"]); glVertexAttribPointer(shader["vUV"], 2, GL_FLOAT, GL_FALSE, sizeof(Vertex), (const GLvoid*)(offsetof(Vertex, uv)) );
- To render the EZMesh, bind the mesh's vertex array object, set up the shader, and pass the shader uniforms.
glBindVertexArray(vaoID); { shader.Use(); glUniformMatrix4fv(shader("MV"), 1, GL_FALSE, glm::value_ptr(MV)); glUniformMatrix3fv(shader("N"), 1, GL_FALSE, glm::value_ptr(glm::inverseTranspose(glm::mat3(MV)))); glUniformMatrix4fv(shader("P"), 1, GL_FALSE, glm::value_ptr(P)); glUniform3fv(shader("light_position"),1, &(lightPosES.x));
- Loop through all submeshes, bind the submesh texture, and then issue the
glDrawEements
call, passing it the submesh indices. If the submesh has no materials, a default solid color material is assigned to the submesh.for(size_t i=0;i<submeshes.size();i++) { if(strlen(submeshes[i].materialName)>0) { GLuint id = materialMap[material2ImageMap[ submeshes[i].materialName]]; GLint whichID[1]; glGetIntegerv(GL_TEXTURE_BINDING_2D, whichID); if(whichID[0] != id) glBindTexture(GL_TEXTURE_2D, id); glUniform1f(shader("useDefault"), 0.0); } else { glUniform1f(shader("useDefault"), 1.0); } glDrawElements(GL_TRIANGLES, submeshes[i].indices.size(), GL_UNSIGNED_INT, &submeshes[i].indices[0]); } }
EZMesh is an XML based skeletal animation format. There are two parts to this recipe: parsing of the EZMesh
file using the MeshImport
/pugixml
libraries and handling of the data using OpenGL buffer objects. The first part is handled by the EzmLoader::Load
function. Along with the filename, this function accepts vectors to store the submeshes, vertices, indices, and material names map contained in the mesh file.
For this recipe, we are interested in the last two subelements: Materials
and Meshes
. We will be using the first two subelements in the skeletal animation recipe in a later chapter of this book. Each Materials
element has a counted number of Material
elements. Each Material
element stores the material's name in the name attribute and the material's details. For example, the texture map file name in the meta_data
attribute. In the EZMLoader::Load
function, we use pugi_xml
to parse the Materials
element and its subelements into a material map. This map stores the material's name and its texture file name. Note that the MeshImport
library does provide functions for reading material information, but they are broken.
Once we have the MeshSystem
object, we can query all of the subelements. These reside in the MeshSystem
object as member variables. So let's say we want to traverse through all of the meshes in the current EZMesh
file and copy the per-vertex attributes to our own vector (vertices
), we would simply do the following:
After the materials, the shaders are loaded as in the previous recipes. The per-vertex data is then transferred to the GPU using vertex array and vertex buffer objects. In this case, we use the interleaved vertex buffer format.
For rendering of the mesh, we first bind the vertex array object of the mesh, attach our shader and pass the shader uniforms. Then we loop over all of the submeshes
and bind the appropriate texture (if the submesh has texture). Otherwise, a default color is used. Finally, the indices of the submesh are used to draw the mesh using the glDrawElements
function.
recipe by following these simple steps:
- Create a global reference to an
EzmLoader
object. Call theEzmLoader::Load
function passing it the name of the EZMesh (.ezm
) file. Pass the vectors to store the submeshes, vertices, indices, and materials-to-image map. TheLoad
function also accepts the min and max vectors to store the EZMesh bounding box.if(!ezm.Load(mesh_filename.c_str(), submeshes, vertices, indices, material2ImageMap, min, max)) { cout<<"Cannot load the EZMesh mesh"<<endl; exit(EXIT_FAILURE); }
- Using the material information, generate the OpenGL textures for the EZMesh geometry.
for(size_t k=0;k<materialNames.size();k++) { GLuint id = 0; glGenTextures(1, &id); glBindTexture(GL_TEXTURE_2D, id); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MIN_FILTER, GL_LINEAR); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MAG_FILTER, GL_LINEAR); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_S, GL_REPEAT); glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_T, GL_REPEAT); int texture_width = 0, texture_height = 0, channels=0; const string& filename = materialNames[k]; std::string full_filename = mesh_path; full_filename.append(filename); //Image loading using SOIL and vertical image flipping //… GLenum format = GL_RGBA; switch(channels) { case 2: format = GL_RG32UI; break; case 3: format = GL_RGB; break; case 4: format = GL_RGBA; break; } glTexImage2D(GL_TEXTURE_2D, 0, format, texture_width, texture_height, 0, format, GL_UNSIGNED_BYTE, pData); SOIL_free_image_data(pData); materialMap[filename] = id ; }
- Set up the interleaved buffer object as in the previous recipe, Implementing OBJ model loading using interleaved buffers.
glBindVertexArray(vaoID); glBindBuffer (GL_ARRAY_BUFFER, vboVerticesID); glBufferData (GL_ARRAY_BUFFER, sizeof(Vertex)*vertices.size(), &(vertices[0].pos.x), GL_DYNAMIC_DRAW); glEnableVertexAttribArray(shader["vVertex"]); glVertexAttribPointer(shader["vVertex"], 3, GL_FLOAT, GL_FALSE,sizeof(Vertex),0); glEnableVertexAttribArray(shader["vNormal"]); glVertexAttribPointer(shader["vNormal"], 3, GL_FLOAT, GL_FALSE, sizeof(Vertex), (const GLvoid*)(offsetof(Vertex, normal)) ); glEnableVertexAttribArray(shader["vUV"]); glVertexAttribPointer(shader["vUV"], 2, GL_FLOAT, GL_FALSE, sizeof(Vertex), (const GLvoid*)(offsetof(Vertex, uv)) );
- To render the EZMesh, bind the mesh's vertex array object, set up the shader, and pass the shader uniforms.
glBindVertexArray(vaoID); { shader.Use(); glUniformMatrix4fv(shader("MV"), 1, GL_FALSE, glm::value_ptr(MV)); glUniformMatrix3fv(shader("N"), 1, GL_FALSE, glm::value_ptr(glm::inverseTranspose(glm::mat3(MV)))); glUniformMatrix4fv(shader("P"), 1, GL_FALSE, glm::value_ptr(P)); glUniform3fv(shader("light_position"),1, &(lightPosES.x));
- Loop through all submeshes, bind the submesh texture, and then issue the
glDrawEements
call, passing it the submesh indices. If the submesh has no materials, a default solid color material is assigned to the submesh.for(size_t i=0;i<submeshes.size();i++) { if(strlen(submeshes[i].materialName)>0) { GLuint id = materialMap[material2ImageMap[ submeshes[i].materialName]]; GLint whichID[1]; glGetIntegerv(GL_TEXTURE_BINDING_2D, whichID); if(whichID[0] != id) glBindTexture(GL_TEXTURE_2D, id); glUniform1f(shader("useDefault"), 0.0); } else { glUniform1f(shader("useDefault"), 1.0); } glDrawElements(GL_TRIANGLES, submeshes[i].indices.size(), GL_UNSIGNED_INT, &submeshes[i].indices[0]); } }
EZMesh is an XML based skeletal animation format. There are two parts to this recipe: parsing of the EZMesh
file using the MeshImport
/pugixml
libraries and handling of the data using OpenGL buffer objects. The first part is handled by the EzmLoader::Load
function. Along with the filename, this function accepts vectors to store the submeshes, vertices, indices, and material names map contained in the mesh file.
For this recipe, we are interested in the last two subelements: Materials
and Meshes
. We will be using the first two subelements in the skeletal animation recipe in a later chapter of this book. Each Materials
element has a counted number of Material
elements. Each Material
element stores the material's name in the name attribute and the material's details. For example, the texture map file name in the meta_data
attribute. In the EZMLoader::Load
function, we use pugi_xml
to parse the Materials
element and its subelements into a material map. This map stores the material's name and its texture file name. Note that the MeshImport
library does provide functions for reading material information, but they are broken.
Once we have the MeshSystem
object, we can query all of the subelements. These reside in the MeshSystem
object as member variables. So let's say we want to traverse through all of the meshes in the current EZMesh
file and copy the per-vertex attributes to our own vector (vertices
), we would simply do the following:
After the materials, the shaders are loaded as in the previous recipes. The per-vertex data is then transferred to the GPU using vertex array and vertex buffer objects. In this case, we use the interleaved vertex buffer format.
For rendering of the mesh, we first bind the vertex array object of the mesh, attach our shader and pass the shader uniforms. Then we loop over all of the submeshes
and bind the appropriate texture (if the submesh has texture). Otherwise, a default color is used. Finally, the indices of the submesh are used to draw the mesh using the glDrawElements
function.
For this recipe, we are interested in the last two subelements: Materials
and Meshes
. We will be using the first two subelements in the skeletal animation recipe in a later chapter of this book. Each Materials
element has a counted number of Material
elements. Each Material
element stores the material's name in the name attribute and the material's details. For example, the texture map file name in the meta_data
attribute. In the EZMLoader::Load
function, we use pugi_xml
to parse the Materials
element and its subelements into a material map. This map stores the material's name and its texture file name. Note that the MeshImport
library does provide functions for reading material information, but they are broken.
Once we have the MeshSystem
object, we can query all of the subelements. These reside in the MeshSystem
object as member variables. So let's say we want to traverse through all of the meshes in the current EZMesh
file and copy the per-vertex attributes to our own vector (vertices
), we would simply do the following:
After the materials, the shaders are loaded as in the previous recipes. The per-vertex data is then transferred to the GPU using vertex array and vertex buffer objects. In this case, we use the interleaved vertex buffer format.
For rendering of the mesh, we first bind the vertex array object of the mesh, attach our shader and pass the shader uniforms. Then we loop over all of the submeshes
and bind the appropriate texture (if the submesh has texture). Otherwise, a default color is used. Finally, the indices of the submesh are used to draw the mesh using the glDrawElements
function.
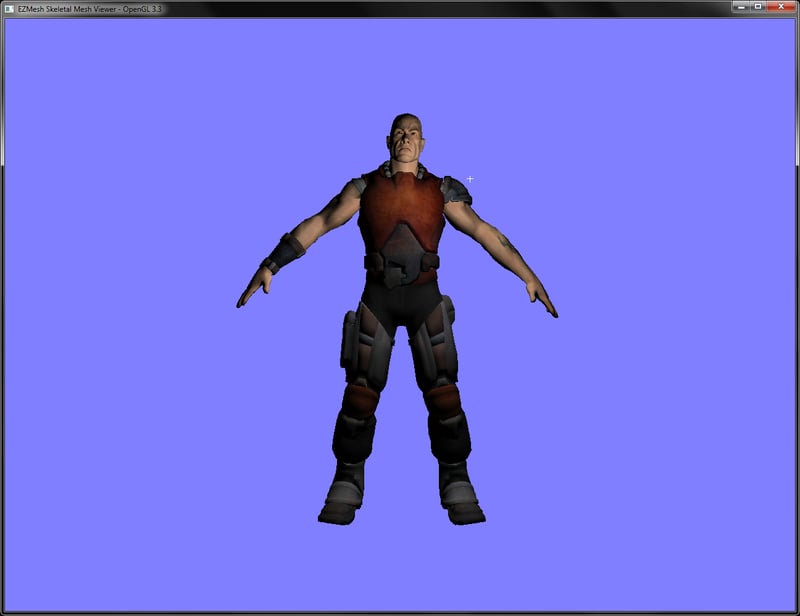
In this recipe, we will implement a simple particle system. Particle systems are a special category of objects that enable us to simulate fuzzy effects in computer graphics; for example, fire or smoke. In this recipe, we will implement a simple particle system that emits particles at the specified rate from an oriented emitter. In this recipe, we will assign particles with a basic fire color map without texture, to give the effect of fire.
The code for this recipe is contained in the Chapter5/SimpleParticles
directory. All of the work for particle simulation is carried out in the vertex shader.
Let us start this recipe by following these simple steps:
- Create a vertex shader without any per-vertex attribute. The vertex shader generates the current particle position and outputs a smooth color to the fragment shader for use as the current fragment color.
#version 330 core smooth out vec4 vSmoothColor; uniform mat4 MVP; uniform float time; const vec3 a = vec3(0,2,0); //acceleration of particles //vec3 g = vec3(0,-9.8,0); // acceleration due to gravity const float rate = 1/500.0; //rate of emission const float life = 2; //life of particle //constants const float PI = 3.14159; const float TWO_PI = 2*PI; //colormap colours const vec3 RED = vec3(1,0,0); const vec3 GREEN = vec3(0,1,0); const vec3 YELLOW = vec3(1,1,0); //pseudorandom number generator float rand(vec2 co){ return fract(sin(dot(co.xy ,vec2(12.9898,78.233))) * 43758.5453); } //pseudorandom direction on a sphere vec3 uniformRadomDir(vec2 v, out vec2 r) { r.x = rand(v.xy); r.y = rand(v.yx); float theta = mix(0.0, PI / 6.0, r.x); float phi = mix(0.0, TWO_PI, r.y); return vec3(sin(theta) * cos(phi), cos(theta), sin(theta) * sin(phi)); } void main() { vec3 pos=vec3(0); float t = gl_VertexID*rate; float alpha = 1; if(time>t) { float dt = mod((time-t), life); vec2 xy = vec2(gl_VertexID,t); vec2 rdm=vec2(0); pos = ((uniformRadomDir(xy, rdm) + 0.5*a*dt)*dt); alpha = 1.0 - (dt/life); } vSmoothColor = vec4(mix(RED,YELLOW,alpha),alpha); gl_Position = MVP*vec4(pos,1); }
- The fragment shader outputs the smooth color as the current fragment output color.
#version 330 core smooth in vec4 vSmoothColor; layout(location=0) out vec4 vFragColor; void main() { vFragColor = vSmoothColor; }
- Set up a single vertex array object and bind it.
glGenVertexArrays(1, &vaoID); glBindVertexArray(vaoID);
- In the rendering code, set up the shader and pass the shader uniforms. For example, pass the current time to the
time
shader uniform and the combined modelview projection matrix (MVP
). Here we add an emitter transform matrix (emitterXForm
) to the combinedMVP
matrix that controls the orientation of our particle emitter.shader.Use(); glUniform1f(shader("time"), time); glUniformMatrix4fv(shader("MVP"), 1, GL_FALSE, glm::value_ptr(P*MV*emitterXForm));
- Finally, we render the total number of particles (
MAX_PARTICLES
) with a call to theglDrawArrays
function and unbind our shader.glDrawArrays(GL_POINTS, 0, MAX_PARTICLES); shader.UnUse();
We have two uniforms in the vertex shader, the combined modelview projection matrix (MVP
) and the current simulation time (time
). The other variables required for particle simulation are stored as shader constants.
The alpha
value is used to linearly interpolate between red and yellow colors by calling the GLSL mix
function to give the fire effect. Finally, the generated position is multiplied with the combined modelview projection (MVP
) matrix to get the clip space position of the particle.
The application loads a particle texture and generates an OpenGL texture object from it.
Next, the texture unit to which the texture is bound is passed to the shader.
Finally, the particles are rendered using the glDrawArrays
call as shown earlier.
If the textured particles shader is used, we get the following output:
The orientation and position of the emitter is controlled using the emitter transformation matrix (emitterXForm
). We can change this matrix to reorient/reposition the particle system in the 3D space.
This gives the following output:
Changing the emitter to a disc shape further filters the points spawned in the rectangle emitter by only accepting those which lie inside the circle of a given radius, as given in the following code snippet:
Using this position calculation gives a disc emitter as shown in the following output:
We can also add additional forces such as air drag, wind, vortex, and so on, by simply adding to the acceleration or velocity component of the particle system. Another option could be to direct the emitter to a specific path such as a b-spline. We could also add deflectors to deflect the generated particles or create particles that spawn other particles as is typically used in a fireworks particle system. Particle systems are an extremely interesting area in computer graphics which help us obtain wonderful effects easily.
To know more about detailed effects you can refer to the following links:
- Real-time particle systems on the GPU in Dynamic Environment SIGGRAPH 2007 Talk: http://developer.amd.com/wordpress/media/2012/10/Drone-Real-Time_Particles_Systems_on_the_GPU_in_Dynamic_Environments%28Siggraph07%29.pdf
- GPU Gems 3 Chapter 23-High speed offscreen particles: http://http.developer.nvidia.com/GPUGems3/gpugems3_ch23.html
- Building a million particle system by Lutz Latta: http://www.gamasutra.com/view/feature/130535/building_a_millionparticle_system.php?print=1
- CG Tutorial chapter 6: http://http.developer.nvidia.com/CgTutorial/cg_tutorial_chapter06.html
Let us start this recipe by following these simple steps:
- Create a vertex shader without any per-vertex attribute. The vertex shader generates the current particle position and outputs a smooth color to the fragment shader for use as the current fragment color.
#version 330 core smooth out vec4 vSmoothColor; uniform mat4 MVP; uniform float time; const vec3 a = vec3(0,2,0); //acceleration of particles //vec3 g = vec3(0,-9.8,0); // acceleration due to gravity const float rate = 1/500.0; //rate of emission const float life = 2; //life of particle //constants const float PI = 3.14159; const float TWO_PI = 2*PI; //colormap colours const vec3 RED = vec3(1,0,0); const vec3 GREEN = vec3(0,1,0); const vec3 YELLOW = vec3(1,1,0); //pseudorandom number generator float rand(vec2 co){ return fract(sin(dot(co.xy ,vec2(12.9898,78.233))) * 43758.5453); } //pseudorandom direction on a sphere vec3 uniformRadomDir(vec2 v, out vec2 r) { r.x = rand(v.xy); r.y = rand(v.yx); float theta = mix(0.0, PI / 6.0, r.x); float phi = mix(0.0, TWO_PI, r.y); return vec3(sin(theta) * cos(phi), cos(theta), sin(theta) * sin(phi)); } void main() { vec3 pos=vec3(0); float t = gl_VertexID*rate; float alpha = 1; if(time>t) { float dt = mod((time-t), life); vec2 xy = vec2(gl_VertexID,t); vec2 rdm=vec2(0); pos = ((uniformRadomDir(xy, rdm) + 0.5*a*dt)*dt); alpha = 1.0 - (dt/life); } vSmoothColor = vec4(mix(RED,YELLOW,alpha),alpha); gl_Position = MVP*vec4(pos,1); }
- The fragment shader outputs the smooth color as the current fragment output color.
#version 330 core smooth in vec4 vSmoothColor; layout(location=0) out vec4 vFragColor; void main() { vFragColor = vSmoothColor; }
- Set up a single vertex array object and bind it.
glGenVertexArrays(1, &vaoID); glBindVertexArray(vaoID);
- In the rendering code, set up the shader and pass the shader uniforms. For example, pass the current time to the
time
shader uniform and the combined modelview projection matrix (MVP
). Here we add an emitter transform matrix (emitterXForm
) to the combinedMVP
matrix that controls the orientation of our particle emitter.shader.Use(); glUniform1f(shader("time"), time); glUniformMatrix4fv(shader("MVP"), 1, GL_FALSE, glm::value_ptr(P*MV*emitterXForm));
- Finally, we render the total number of particles (
MAX_PARTICLES
) with a call to theglDrawArrays
function and unbind our shader.glDrawArrays(GL_POINTS, 0, MAX_PARTICLES); shader.UnUse();
We have two uniforms in the vertex shader, the combined modelview projection matrix (MVP
) and the current simulation time (time
). The other variables required for particle simulation are stored as shader constants.
The alpha
value is used to linearly interpolate between red and yellow colors by calling the GLSL mix
function to give the fire effect. Finally, the generated position is multiplied with the combined modelview projection (MVP
) matrix to get the clip space position of the particle.
The application loads a particle texture and generates an OpenGL texture object from it.
Next, the texture unit to which the texture is bound is passed to the shader.
Finally, the particles are rendered using the glDrawArrays
call as shown earlier.
If the textured particles shader is used, we get the following output:
The orientation and position of the emitter is controlled using the emitter transformation matrix (emitterXForm
). We can change this matrix to reorient/reposition the particle system in the 3D space.
This gives the following output:
Changing the emitter to a disc shape further filters the points spawned in the rectangle emitter by only accepting those which lie inside the circle of a given radius, as given in the following code snippet:
Using this position calculation gives a disc emitter as shown in the following output:
We can also add additional forces such as air drag, wind, vortex, and so on, by simply adding to the acceleration or velocity component of the particle system. Another option could be to direct the emitter to a specific path such as a b-spline. We could also add deflectors to deflect the generated particles or create particles that spawn other particles as is typically used in a fireworks particle system. Particle systems are an extremely interesting area in computer graphics which help us obtain wonderful effects easily.
To know more about detailed effects you can refer to the following links:
- Real-time particle systems on the GPU in Dynamic Environment SIGGRAPH 2007 Talk: http://developer.amd.com/wordpress/media/2012/10/Drone-Real-Time_Particles_Systems_on_the_GPU_in_Dynamic_Environments%28Siggraph07%29.pdf
- GPU Gems 3 Chapter 23-High speed offscreen particles: http://http.developer.nvidia.com/GPUGems3/gpugems3_ch23.html
- Building a million particle system by Lutz Latta: http://www.gamasutra.com/view/feature/130535/building_a_millionparticle_system.php?print=1
- CG Tutorial chapter 6: http://http.developer.nvidia.com/CgTutorial/cg_tutorial_chapter06.html
- for use as the current fragment color.
#version 330 core smooth out vec4 vSmoothColor; uniform mat4 MVP; uniform float time; const vec3 a = vec3(0,2,0); //acceleration of particles //vec3 g = vec3(0,-9.8,0); // acceleration due to gravity const float rate = 1/500.0; //rate of emission const float life = 2; //life of particle //constants const float PI = 3.14159; const float TWO_PI = 2*PI; //colormap colours const vec3 RED = vec3(1,0,0); const vec3 GREEN = vec3(0,1,0); const vec3 YELLOW = vec3(1,1,0); //pseudorandom number generator float rand(vec2 co){ return fract(sin(dot(co.xy ,vec2(12.9898,78.233))) * 43758.5453); } //pseudorandom direction on a sphere vec3 uniformRadomDir(vec2 v, out vec2 r) { r.x = rand(v.xy); r.y = rand(v.yx); float theta = mix(0.0, PI / 6.0, r.x); float phi = mix(0.0, TWO_PI, r.y); return vec3(sin(theta) * cos(phi), cos(theta), sin(theta) * sin(phi)); } void main() { vec3 pos=vec3(0); float t = gl_VertexID*rate; float alpha = 1; if(time>t) { float dt = mod((time-t), life); vec2 xy = vec2(gl_VertexID,t); vec2 rdm=vec2(0); pos = ((uniformRadomDir(xy, rdm) + 0.5*a*dt)*dt); alpha = 1.0 - (dt/life); } vSmoothColor = vec4(mix(RED,YELLOW,alpha),alpha); gl_Position = MVP*vec4(pos,1); }
- The fragment shader outputs the smooth color as the current fragment output color.
#version 330 core smooth in vec4 vSmoothColor; layout(location=0) out vec4 vFragColor; void main() { vFragColor = vSmoothColor; }
- Set up a single vertex array object and bind it.
glGenVertexArrays(1, &vaoID); glBindVertexArray(vaoID);
- In the rendering code, set up the shader and pass the shader uniforms. For example, pass the current time to the
time
shader uniform and the combined modelview projection matrix (MVP
). Here we add an emitter transform matrix (emitterXForm
) to the combinedMVP
matrix that controls the orientation of our particle emitter.shader.Use(); glUniform1f(shader("time"), time); glUniformMatrix4fv(shader("MVP"), 1, GL_FALSE, glm::value_ptr(P*MV*emitterXForm));
- Finally, we render the total number of particles (
MAX_PARTICLES
) with a call to theglDrawArrays
function and unbind our shader.glDrawArrays(GL_POINTS, 0, MAX_PARTICLES); shader.UnUse();
We have two uniforms in the vertex shader, the combined modelview projection matrix (MVP
) and the current simulation time (time
). The other variables required for particle simulation are stored as shader constants.
The alpha
value is used to linearly interpolate between red and yellow colors by calling the GLSL mix
function to give the fire effect. Finally, the generated position is multiplied with the combined modelview projection (MVP
) matrix to get the clip space position of the particle.
The application loads a particle texture and generates an OpenGL texture object from it.
Next, the texture unit to which the texture is bound is passed to the shader.
Finally, the particles are rendered using the glDrawArrays
call as shown earlier.
If the textured particles shader is used, we get the following output:
The orientation and position of the emitter is controlled using the emitter transformation matrix (emitterXForm
). We can change this matrix to reorient/reposition the particle system in the 3D space.
This gives the following output:
Changing the emitter to a disc shape further filters the points spawned in the rectangle emitter by only accepting those which lie inside the circle of a given radius, as given in the following code snippet:
Using this position calculation gives a disc emitter as shown in the following output:
We can also add additional forces such as air drag, wind, vortex, and so on, by simply adding to the acceleration or velocity component of the particle system. Another option could be to direct the emitter to a specific path such as a b-spline. We could also add deflectors to deflect the generated particles or create particles that spawn other particles as is typically used in a fireworks particle system. Particle systems are an extremely interesting area in computer graphics which help us obtain wonderful effects easily.
To know more about detailed effects you can refer to the following links:
- Real-time particle systems on the GPU in Dynamic Environment SIGGRAPH 2007 Talk: http://developer.amd.com/wordpress/media/2012/10/Drone-Real-Time_Particles_Systems_on_the_GPU_in_Dynamic_Environments%28Siggraph07%29.pdf
- GPU Gems 3 Chapter 23-High speed offscreen particles: http://http.developer.nvidia.com/GPUGems3/gpugems3_ch23.html
- Building a million particle system by Lutz Latta: http://www.gamasutra.com/view/feature/130535/building_a_millionparticle_system.php?print=1
- CG Tutorial chapter 6: http://http.developer.nvidia.com/CgTutorial/cg_tutorial_chapter06.html
glDrawArrays
call with the number of particles (MAX_PARTICLES
) we need to render. This calls our vertex shader for each particle in turn.
The alpha
value is used to linearly interpolate between red and yellow colors by calling the GLSL mix
function to give the fire effect. Finally, the generated position is multiplied with the combined modelview projection (MVP
) matrix to get the clip space position of the particle.
The application loads a particle texture and generates an OpenGL texture object from it.
Next, the texture unit to which the texture is bound is passed to the shader.
Finally, the particles are rendered using the glDrawArrays
call as shown earlier.
If the textured particles shader is used, we get the following output:
The orientation and position of the emitter is controlled using the emitter transformation matrix (emitterXForm
). We can change this matrix to reorient/reposition the particle system in the 3D space.
This gives the following output:
Changing the emitter to a disc shape further filters the points spawned in the rectangle emitter by only accepting those which lie inside the circle of a given radius, as given in the following code snippet:
Using this position calculation gives a disc emitter as shown in the following output:
We can also add additional forces such as air drag, wind, vortex, and so on, by simply adding to the acceleration or velocity component of the particle system. Another option could be to direct the emitter to a specific path such as a b-spline. We could also add deflectors to deflect the generated particles or create particles that spawn other particles as is typically used in a fireworks particle system. Particle systems are an extremely interesting area in computer graphics which help us obtain wonderful effects easily.
To know more about detailed effects you can refer to the following links:
- Real-time particle systems on the GPU in Dynamic Environment SIGGRAPH 2007 Talk: http://developer.amd.com/wordpress/media/2012/10/Drone-Real-Time_Particles_Systems_on_the_GPU_in_Dynamic_Environments%28Siggraph07%29.pdf
- GPU Gems 3 Chapter 23-High speed offscreen particles: http://http.developer.nvidia.com/GPUGems3/gpugems3_ch23.html
- Building a million particle system by Lutz Latta: http://www.gamasutra.com/view/feature/130535/building_a_millionparticle_system.php?print=1
- CG Tutorial chapter 6: http://http.developer.nvidia.com/CgTutorial/cg_tutorial_chapter06.html
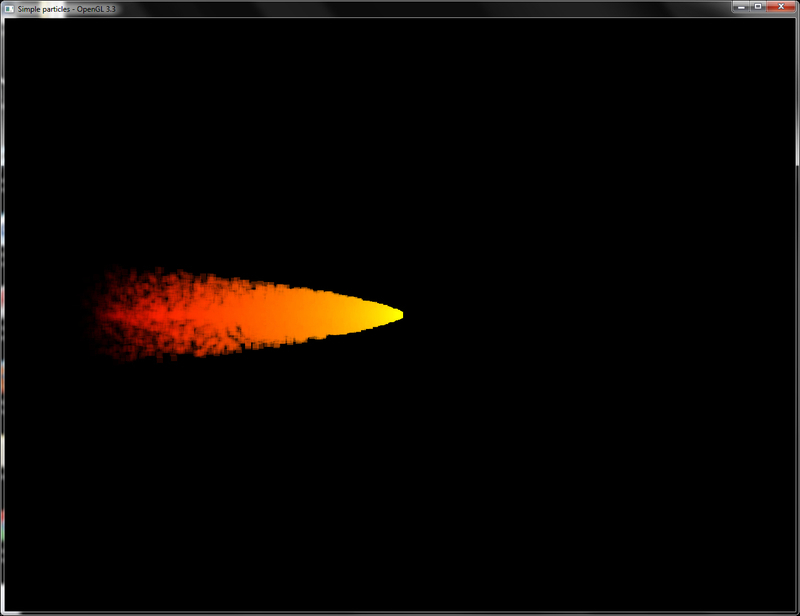
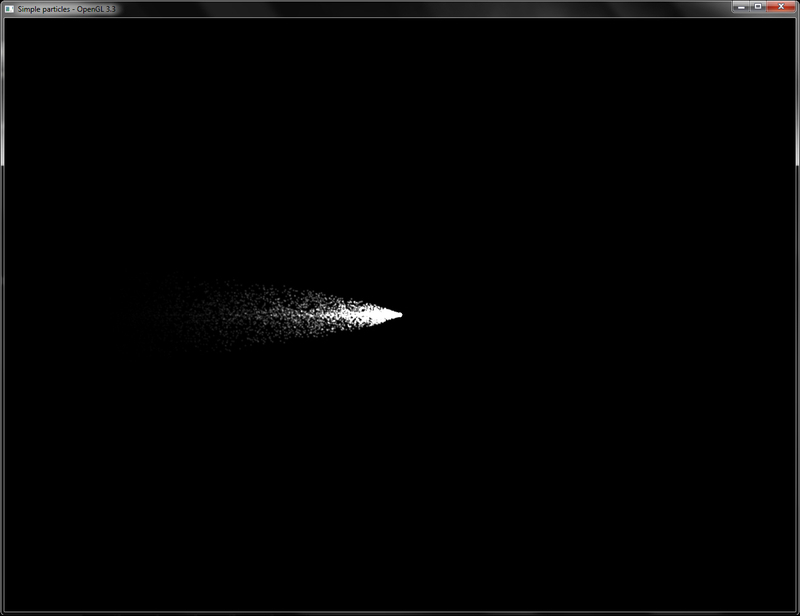
This gives the following output:
Changing the emitter to a disc shape further filters the points spawned in the rectangle emitter by only accepting those which lie inside the circle of a given radius, as given in the following code snippet:
Using this position calculation gives a disc emitter as shown in the following output:
We can also add additional forces such as air drag, wind, vortex, and so on, by simply adding to the acceleration or velocity component of the particle system. Another option could be to direct the emitter to a specific path such as a b-spline. We could also add deflectors to deflect the generated particles or create particles that spawn other particles as is typically used in a fireworks particle system. Particle systems are an extremely interesting area in computer graphics which help us obtain wonderful effects easily.
To know more about detailed effects you can refer to the following links:
- Real-time particle systems on the GPU in Dynamic Environment SIGGRAPH 2007 Talk: http://developer.amd.com/wordpress/media/2012/10/Drone-Real-Time_Particles_Systems_on_the_GPU_in_Dynamic_Environments%28Siggraph07%29.pdf
- GPU Gems 3 Chapter 23-High speed offscreen particles: http://http.developer.nvidia.com/GPUGems3/gpugems3_ch23.html
- Building a million particle system by Lutz Latta: http://www.gamasutra.com/view/feature/130535/building_a_millionparticle_system.php?print=1
- CG Tutorial chapter 6: http://http.developer.nvidia.com/CgTutorial/cg_tutorial_chapter06.html
- http://developer.amd.com/wordpress/media/2012/10/Drone-Real-Time_Particles_Systems_on_the_GPU_in_Dynamic_Environments%28Siggraph07%29.pdf
- GPU Gems 3 Chapter 23-High speed offscreen particles: http://http.developer.nvidia.com/GPUGems3/gpugems3_ch23.html
- Building a million particle system by Lutz Latta: http://www.gamasutra.com/view/feature/130535/building_a_millionparticle_system.php?print=1
- CG Tutorial chapter 6: http://http.developer.nvidia.com/CgTutorial/cg_tutorial_chapter06.html
In this chapter, we will focus on:
- Implementing order-independent transparency using front-to-back peeling
- Implementing order-independent transparency with dual depth peeling
- Implementing screen space ambient occlusion (SSAO)
- Implementing global illumination using spherical harmonics lighting
- Implementing GPU-based ray tracing
- Implementing GPU-based path tracing
Even with the introduction of lighting, our virtual objects don't look and feel real. This is because our lights are a simple approximation of the reflection behavior of the surface. There is a specific category of algorithms that help bridge the gap between the real-world lighting and the virtual-world lighting. These are called global illumination methods. Although these methods had been proven to be expensive to evaluate in real time, new methods have been proposed that fake the global illumination using clever techniques. One such technique is spherical harmonics lighting that uses HDR light probes to light a virtual scene having no light source. The idea is to extract the lighting information from the light probe and give a feeling that the virtual objects are in the same environment.
When we have to render translucent geometry, for example, a glass window in a graphics application, care has to be taken to make sure that the geometry is properly rendered in the depth order such that the opaque objects in the scene are rendered first and the transparent objects are rendered last. This unfortunately incurs additional overhead where the CPU is busy sorting objects. In addition, the blending result will be correct only from a specific viewing direction, as shown in the following figure. Note that the image on the left is the result if we view from the direction of the Z axis. There is no blending at all in the left image. If the same scene is viewed from the opposite side, we can see the correct alpha blending result.
The number of layers to use for peeling is dependent on the depth complexity of the scene. This recipe will show how to implement this technique in modern OpenGL.
Let us start our recipe by following these simple steps:
- Set up two frame buffer objects (FBOs) with two color and depth attachments. For this recipe, we will use rectangle textures (
GL_TEXTURE_RECTANGLE
) since they enable easier handling of images (samplers) in the fragment shader. With rectangle textures we can access texture values using pixel positions directly. In case of normal texture (GL_TEXTUR_2D
), we have to normalize the texture coordinates.glGenFramebuffers(2, fbo); glGenTextures (2, texID); glGenTextures (2, depthTexID); for(int i=0;i<2;i++) { glBindTexture(GL_TEXTURE_RECTANGLE, depthTexID[i]); //set texture parameters like minification etc. glTexImage2D(GL_TEXTURE_RECTANGLE , 0, GL_DEPTH_COMPONENT32F, WIDTH, HEIGHT, 0, GL_DEPTH_COMPONENT, GL_FLOAT, NULL); glBindTexture(GL_TEXTURE_RECTANGLE,texID[i]); //set texture parameters like minification etc. glTexImage2D(GL_TEXTURE_RECTANGLE , 0,GL_RGBA, WIDTH, HEIGHT, 0, GL_RGBA, GL_FLOAT, NULL); glBindFramebuffer(GL_FRAMEBUFFER, fbo[i]); glFramebufferTexture2D(GL_FRAMEBUFFER, GL_DEPTH_ATTACHMENT, GL_TEXTURE_RECTANGLE, depthTexID[i], 0); glFramebufferTexture2D(GL_FRAMEBUFFER, GL_COLOR_ATTACHMENT0, GL_TEXTURE_RECTANGLE,texID[i], 0); } glGenTextures(1, &colorBlenderTexID); glBindTexture(GL_TEXTURE_RECTANGLE, colorBlenderTexID); //set texture parameters like minification etc. glTexImage2D(GL_TEXTURE_RECTANGLE, 0, GL_RGBA, WIDTH, HEIGHT, 0, GL_RGBA, GL_FLOAT, 0);
- Set another FBO for color blending and check the FBO for completeness. The color blending FBO uses the depth texture from the first FBO as a depth attachment, as it uses the depth output from the first step during blending.
glGenFramebuffers(1, &colorBlenderFBOID); glBindFramebuffer(GL_FRAMEBUFFER, colorBlenderFBOID); glFramebufferTexture2D(GL_FRAMEBUFFER, GL_DEPTH_ATTACHMENT, GL_TEXTURE_RECTANGLE, depthTexID[0], 0); glFramebufferTexture2D(GL_FRAMEBUFFER, GL_COLOR_ATTACHMENT0, GL_TEXTURE_RECTANGLE, colorBlenderTexID, 0); GLenum status = glCheckFramebufferStatus(GL_FRAMEBUFFER); if(status == GL_FRAMEBUFFER_COMPLETE ) printf("FBO setup successful !!! \n"); else printf("Problem with FBO setup"); glBindFramebuffer(GL_FRAMEBUFFER, 0);
- In the rendering function, set the color blending FBO as the current render target and then render the scene normally with depth testing enabled.
glBindFramebuffer(GL_FRAMEBUFFER, colorBlenderFBOID); glDrawBuffer(GL_COLOR_ATTACHMENT0); glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT ); glEnable(GL_DEPTH_TEST); DrawScene(MVP, cubeShader);
- Next, bind the other FBO pair alternatively, clear the render target, and enable depth testing, but disable alpha blending. This is to render the nearest surface in the offscreen render target. The number of passes dictate the number of layers the given geometry is peeled into. The more the number of passes, the more continuous the depth peeling result. For the demo in this recipe, the number of passes is set as 6. The number of passes is dependent on the depth complexity of the scene. If the user wants to check the number of samples output from the depth peeling step, then based on the value of the flag (
bUseOQ
) an occlusion query is used to find the number of samples output from the depth peeling step.int numLayers = (NUM_PASSES - 1) * 2; for (int layer = 1; bUseOQ || layer < numLayers; layer++) { int currId = layer % 2; int prevId = 1 - currId; glBindFramebuffer(GL_FRAMEBUFFER, fbo[currId]); glDrawBuffer(GL_COLOR_ATTACHMENT0); glClearColor(0, 0, 0, 0); glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT); glDisable(GL_BLEND); glEnable(GL_DEPTH_TEST); if (bUseOQ) { glBeginQuery(GL_SAMPLES_PASSED_ARB, queryId); }
- Bind the depth texture from the first step so that the nearest fragment can be used with the attached shaders and then render the scene with the front peeling shaders. Refer to
Chapter6/FrontToBackPeeling/shaders/front_peel.{vert,frag}
for details. We then end the hardware query if the query was initiated.glBindTexture(GL_TEXTURE_RECTANGLE, depthTexID[prevId]); DrawScene(MVP, frontPeelShader); if (bUseOQ) { glEndQuery(GL_SAMPLES_PASSED_ARB); }
- Bind the color blender FBO again, disable depth testing, and enable additive blending; however, specify separate blending so that the color and alpha can be blended separately. Finally, bind the rendered output from step 5 and then using a full-screen quad and the blend shader (
Chapter6/FrontToBackPeeling/shaders/blend. {vert,frag}
), blend the whole scene.glBindFramebuffer(GL_FRAMEBUFFER, colorBlenderFBOID); glDrawBuffer(GL_COLOR_ATTACHMENT0); glDisable(GL_DEPTH_TEST); glEnable(GL_BLEND); glBlendEquation(GL_FUNC_ADD); glBlendFuncSeparate(GL_DST_ALPHA, GL_ONE,GL_ZERO, GL_ONE_MINUS_SRC_ALPHA); glBindTexture(GL_TEXTURE_RECTANGLE, texID[currId]); blendShader.Use(); DrawFullScreenQuad(); blendShader.UnUse(); glDisable(GL_BLEND);
- In the final step, restore the default draw buffer (
GL_BACK_LEFT
) and disable alpha blending and depth testing. Use a full-screen quad and a final shader (Chapter6/FrontToBackPeeling/shaders/final.frag
) to blend the output from the color blending FBO.glBindFramebuffer(GL_FRAMEBUFFER, 0); glDrawBuffer(GL_BACK_LEFT); glDisable(GL_DEPTH_TEST); glDisable(GL_BLEND); glBindTexture(GL_TEXTURE_RECTANGLE, colorBlenderTexID); finalShader.Use(); glUniform4fv(finalShader("vBackgroundColor"), 1, &bg.x); DrawFullScreenQuad(); finalShader.UnUse();
The front-to-back depth peeling works in three steps. First, the scene is rendered normally on a depth FBO with depth testing enabled. This ensures that the scene depth values are stored in the depth attachment of the FBO. In the second pass, we bind the depth FBO, bind the depth texture from the first step, and then iteratively clip parts of the geometry by using a fragment shader (see Chapter6/FrontToBackPeeling/shaders/front_peel.frag
) as shown in the following code snippet:
After this step, we bind the color blend FBO, disable depth test, and then enable alpha blending with separate blending of colors and alpha values. The glBlendFunctionSeparate
function is used here as it enables us to handle color and alpha channels for source and destination separately. The first parameter is the source RGB, which is assigned the alpha value of the pixel in the frame buffer. This blends the incoming fragment with the existing color in the frame buffer. The second parameter, that is, the destination RGB, is set as GL_ONE
, which keeps the value in the destination as is. The third parameter is set as GL_ZERO
, which removes the source alpha component as we already applied the alpha from the destination using the first parameter. The final parameter, that is, the destination alpha is set as the conventional over-compositing alpha value (GL_ONE_MINUS_SRC_ALPHA
).
The final shader takes the front peeled result and blends it with the background color using the alpha value from the front peeled result. This way rather than taking the nearest depth fragment all fragments are taken into consideration showing a correctly blended result.
Chapter6/FrontToBackPeeling
directory.
Let us start our recipe by following these simple steps:
- Set up two frame buffer objects (FBOs) with two color and depth attachments. For this recipe, we will use rectangle textures (
GL_TEXTURE_RECTANGLE
) since they enable easier handling of images (samplers) in the fragment shader. With rectangle textures we can access texture values using pixel positions directly. In case of normal texture (GL_TEXTUR_2D
), we have to normalize the texture coordinates.glGenFramebuffers(2, fbo); glGenTextures (2, texID); glGenTextures (2, depthTexID); for(int i=0;i<2;i++) { glBindTexture(GL_TEXTURE_RECTANGLE, depthTexID[i]); //set texture parameters like minification etc. glTexImage2D(GL_TEXTURE_RECTANGLE , 0, GL_DEPTH_COMPONENT32F, WIDTH, HEIGHT, 0, GL_DEPTH_COMPONENT, GL_FLOAT, NULL); glBindTexture(GL_TEXTURE_RECTANGLE,texID[i]); //set texture parameters like minification etc. glTexImage2D(GL_TEXTURE_RECTANGLE , 0,GL_RGBA, WIDTH, HEIGHT, 0, GL_RGBA, GL_FLOAT, NULL); glBindFramebuffer(GL_FRAMEBUFFER, fbo[i]); glFramebufferTexture2D(GL_FRAMEBUFFER, GL_DEPTH_ATTACHMENT, GL_TEXTURE_RECTANGLE, depthTexID[i], 0); glFramebufferTexture2D(GL_FRAMEBUFFER, GL_COLOR_ATTACHMENT0, GL_TEXTURE_RECTANGLE,texID[i], 0); } glGenTextures(1, &colorBlenderTexID); glBindTexture(GL_TEXTURE_RECTANGLE, colorBlenderTexID); //set texture parameters like minification etc. glTexImage2D(GL_TEXTURE_RECTANGLE, 0, GL_RGBA, WIDTH, HEIGHT, 0, GL_RGBA, GL_FLOAT, 0);
- Set another FBO for color blending and check the FBO for completeness. The color blending FBO uses the depth texture from the first FBO as a depth attachment, as it uses the depth output from the first step during blending.
glGenFramebuffers(1, &colorBlenderFBOID); glBindFramebuffer(GL_FRAMEBUFFER, colorBlenderFBOID); glFramebufferTexture2D(GL_FRAMEBUFFER, GL_DEPTH_ATTACHMENT, GL_TEXTURE_RECTANGLE, depthTexID[0], 0); glFramebufferTexture2D(GL_FRAMEBUFFER, GL_COLOR_ATTACHMENT0, GL_TEXTURE_RECTANGLE, colorBlenderTexID, 0); GLenum status = glCheckFramebufferStatus(GL_FRAMEBUFFER); if(status == GL_FRAMEBUFFER_COMPLETE ) printf("FBO setup successful !!! \n"); else printf("Problem with FBO setup"); glBindFramebuffer(GL_FRAMEBUFFER, 0);
- In the rendering function, set the color blending FBO as the current render target and then render the scene normally with depth testing enabled.
glBindFramebuffer(GL_FRAMEBUFFER, colorBlenderFBOID); glDrawBuffer(GL_COLOR_ATTACHMENT0); glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT ); glEnable(GL_DEPTH_TEST); DrawScene(MVP, cubeShader);
- Next, bind the other FBO pair alternatively, clear the render target, and enable depth testing, but disable alpha blending. This is to render the nearest surface in the offscreen render target. The number of passes dictate the number of layers the given geometry is peeled into. The more the number of passes, the more continuous the depth peeling result. For the demo in this recipe, the number of passes is set as 6. The number of passes is dependent on the depth complexity of the scene. If the user wants to check the number of samples output from the depth peeling step, then based on the value of the flag (
bUseOQ
) an occlusion query is used to find the number of samples output from the depth peeling step.int numLayers = (NUM_PASSES - 1) * 2; for (int layer = 1; bUseOQ || layer < numLayers; layer++) { int currId = layer % 2; int prevId = 1 - currId; glBindFramebuffer(GL_FRAMEBUFFER, fbo[currId]); glDrawBuffer(GL_COLOR_ATTACHMENT0); glClearColor(0, 0, 0, 0); glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT); glDisable(GL_BLEND); glEnable(GL_DEPTH_TEST); if (bUseOQ) { glBeginQuery(GL_SAMPLES_PASSED_ARB, queryId); }
- Bind the depth texture from the first step so that the nearest fragment can be used with the attached shaders and then render the scene with the front peeling shaders. Refer to
Chapter6/FrontToBackPeeling/shaders/front_peel.{vert,frag}
for details. We then end the hardware query if the query was initiated.glBindTexture(GL_TEXTURE_RECTANGLE, depthTexID[prevId]); DrawScene(MVP, frontPeelShader); if (bUseOQ) { glEndQuery(GL_SAMPLES_PASSED_ARB); }
- Bind the color blender FBO again, disable depth testing, and enable additive blending; however, specify separate blending so that the color and alpha can be blended separately. Finally, bind the rendered output from step 5 and then using a full-screen quad and the blend shader (
Chapter6/FrontToBackPeeling/shaders/blend. {vert,frag}
), blend the whole scene.glBindFramebuffer(GL_FRAMEBUFFER, colorBlenderFBOID); glDrawBuffer(GL_COLOR_ATTACHMENT0); glDisable(GL_DEPTH_TEST); glEnable(GL_BLEND); glBlendEquation(GL_FUNC_ADD); glBlendFuncSeparate(GL_DST_ALPHA, GL_ONE,GL_ZERO, GL_ONE_MINUS_SRC_ALPHA); glBindTexture(GL_TEXTURE_RECTANGLE, texID[currId]); blendShader.Use(); DrawFullScreenQuad(); blendShader.UnUse(); glDisable(GL_BLEND);
- In the final step, restore the default draw buffer (
GL_BACK_LEFT
) and disable alpha blending and depth testing. Use a full-screen quad and a final shader (Chapter6/FrontToBackPeeling/shaders/final.frag
) to blend the output from the color blending FBO.glBindFramebuffer(GL_FRAMEBUFFER, 0); glDrawBuffer(GL_BACK_LEFT); glDisable(GL_DEPTH_TEST); glDisable(GL_BLEND); glBindTexture(GL_TEXTURE_RECTANGLE, colorBlenderTexID); finalShader.Use(); glUniform4fv(finalShader("vBackgroundColor"), 1, &bg.x); DrawFullScreenQuad(); finalShader.UnUse();
The front-to-back depth peeling works in three steps. First, the scene is rendered normally on a depth FBO with depth testing enabled. This ensures that the scene depth values are stored in the depth attachment of the FBO. In the second pass, we bind the depth FBO, bind the depth texture from the first step, and then iteratively clip parts of the geometry by using a fragment shader (see Chapter6/FrontToBackPeeling/shaders/front_peel.frag
) as shown in the following code snippet:
After this step, we bind the color blend FBO, disable depth test, and then enable alpha blending with separate blending of colors and alpha values. The glBlendFunctionSeparate
function is used here as it enables us to handle color and alpha channels for source and destination separately. The first parameter is the source RGB, which is assigned the alpha value of the pixel in the frame buffer. This blends the incoming fragment with the existing color in the frame buffer. The second parameter, that is, the destination RGB, is set as GL_ONE
, which keeps the value in the destination as is. The third parameter is set as GL_ZERO
, which removes the source alpha component as we already applied the alpha from the destination using the first parameter. The final parameter, that is, the destination alpha is set as the conventional over-compositing alpha value (GL_ONE_MINUS_SRC_ALPHA
).
The final shader takes the front peeled result and blends it with the background color using the alpha value from the front peeled result. This way rather than taking the nearest depth fragment all fragments are taken into consideration showing a correctly blended result.
GL_TEXTURE_RECTANGLE
) since they enable easier handling of images (samplers) in the fragment shader. With rectangle textures we can access texture values using pixel positions directly. In case of normal texture (GL_TEXTUR_2D
), we have to normalize the texture coordinates.glGenFramebuffers(2, fbo); glGenTextures (2, texID); glGenTextures (2, depthTexID); for(int i=0;i<2;i++) { glBindTexture(GL_TEXTURE_RECTANGLE, depthTexID[i]); //set texture parameters like minification etc. glTexImage2D(GL_TEXTURE_RECTANGLE , 0, GL_DEPTH_COMPONENT32F, WIDTH, HEIGHT, 0, GL_DEPTH_COMPONENT, GL_FLOAT, NULL); glBindTexture(GL_TEXTURE_RECTANGLE,texID[i]); //set texture parameters like minification etc. glTexImage2D(GL_TEXTURE_RECTANGLE , 0,GL_RGBA, WIDTH, HEIGHT, 0, GL_RGBA, GL_FLOAT, NULL); glBindFramebuffer(GL_FRAMEBUFFER, fbo[i]); glFramebufferTexture2D(GL_FRAMEBUFFER, GL_DEPTH_ATTACHMENT, GL_TEXTURE_RECTANGLE, depthTexID[i], 0); glFramebufferTexture2D(GL_FRAMEBUFFER, GL_COLOR_ATTACHMENT0, GL_TEXTURE_RECTANGLE,texID[i], 0); } glGenTextures(1, &colorBlenderTexID); glBindTexture(GL_TEXTURE_RECTANGLE, colorBlenderTexID); //set texture parameters like minification etc. glTexImage2D(GL_TEXTURE_RECTANGLE, 0, GL_RGBA, WIDTH, HEIGHT, 0, GL_RGBA, GL_FLOAT, 0);
- FBO for color blending and check the FBO for completeness. The color blending FBO uses the depth texture from the first FBO as a depth attachment, as it uses the depth output from the first step during blending.
glGenFramebuffers(1, &colorBlenderFBOID); glBindFramebuffer(GL_FRAMEBUFFER, colorBlenderFBOID); glFramebufferTexture2D(GL_FRAMEBUFFER, GL_DEPTH_ATTACHMENT, GL_TEXTURE_RECTANGLE, depthTexID[0], 0); glFramebufferTexture2D(GL_FRAMEBUFFER, GL_COLOR_ATTACHMENT0, GL_TEXTURE_RECTANGLE, colorBlenderTexID, 0); GLenum status = glCheckFramebufferStatus(GL_FRAMEBUFFER); if(status == GL_FRAMEBUFFER_COMPLETE ) printf("FBO setup successful !!! \n"); else printf("Problem with FBO setup"); glBindFramebuffer(GL_FRAMEBUFFER, 0);
- In the rendering function, set the color blending FBO as the current render target and then render the scene normally with depth testing enabled.
glBindFramebuffer(GL_FRAMEBUFFER, colorBlenderFBOID); glDrawBuffer(GL_COLOR_ATTACHMENT0); glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT ); glEnable(GL_DEPTH_TEST); DrawScene(MVP, cubeShader);
- Next, bind the other FBO pair alternatively, clear the render target, and enable depth testing, but disable alpha blending. This is to render the nearest surface in the offscreen render target. The number of passes dictate the number of layers the given geometry is peeled into. The more the number of passes, the more continuous the depth peeling result. For the demo in this recipe, the number of passes is set as 6. The number of passes is dependent on the depth complexity of the scene. If the user wants to check the number of samples output from the depth peeling step, then based on the value of the flag (
bUseOQ
) an occlusion query is used to find the number of samples output from the depth peeling step.int numLayers = (NUM_PASSES - 1) * 2; for (int layer = 1; bUseOQ || layer < numLayers; layer++) { int currId = layer % 2; int prevId = 1 - currId; glBindFramebuffer(GL_FRAMEBUFFER, fbo[currId]); glDrawBuffer(GL_COLOR_ATTACHMENT0); glClearColor(0, 0, 0, 0); glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT); glDisable(GL_BLEND); glEnable(GL_DEPTH_TEST); if (bUseOQ) { glBeginQuery(GL_SAMPLES_PASSED_ARB, queryId); }
- Bind the depth texture from the first step so that the nearest fragment can be used with the attached shaders and then render the scene with the front peeling shaders. Refer to
Chapter6/FrontToBackPeeling/shaders/front_peel.{vert,frag}
for details. We then end the hardware query if the query was initiated.glBindTexture(GL_TEXTURE_RECTANGLE, depthTexID[prevId]); DrawScene(MVP, frontPeelShader); if (bUseOQ) { glEndQuery(GL_SAMPLES_PASSED_ARB); }
- Bind the color blender FBO again, disable depth testing, and enable additive blending; however, specify separate blending so that the color and alpha can be blended separately. Finally, bind the rendered output from step 5 and then using a full-screen quad and the blend shader (
Chapter6/FrontToBackPeeling/shaders/blend. {vert,frag}
), blend the whole scene.glBindFramebuffer(GL_FRAMEBUFFER, colorBlenderFBOID); glDrawBuffer(GL_COLOR_ATTACHMENT0); glDisable(GL_DEPTH_TEST); glEnable(GL_BLEND); glBlendEquation(GL_FUNC_ADD); glBlendFuncSeparate(GL_DST_ALPHA, GL_ONE,GL_ZERO, GL_ONE_MINUS_SRC_ALPHA); glBindTexture(GL_TEXTURE_RECTANGLE, texID[currId]); blendShader.Use(); DrawFullScreenQuad(); blendShader.UnUse(); glDisable(GL_BLEND);
- In the final step, restore the default draw buffer (
GL_BACK_LEFT
) and disable alpha blending and depth testing. Use a full-screen quad and a final shader (Chapter6/FrontToBackPeeling/shaders/final.frag
) to blend the output from the color blending FBO.glBindFramebuffer(GL_FRAMEBUFFER, 0); glDrawBuffer(GL_BACK_LEFT); glDisable(GL_DEPTH_TEST); glDisable(GL_BLEND); glBindTexture(GL_TEXTURE_RECTANGLE, colorBlenderTexID); finalShader.Use(); glUniform4fv(finalShader("vBackgroundColor"), 1, &bg.x); DrawFullScreenQuad(); finalShader.UnUse();
The front-to-back depth peeling works in three steps. First, the scene is rendered normally on a depth FBO with depth testing enabled. This ensures that the scene depth values are stored in the depth attachment of the FBO. In the second pass, we bind the depth FBO, bind the depth texture from the first step, and then iteratively clip parts of the geometry by using a fragment shader (see Chapter6/FrontToBackPeeling/shaders/front_peel.frag
) as shown in the following code snippet:
After this step, we bind the color blend FBO, disable depth test, and then enable alpha blending with separate blending of colors and alpha values. The glBlendFunctionSeparate
function is used here as it enables us to handle color and alpha channels for source and destination separately. The first parameter is the source RGB, which is assigned the alpha value of the pixel in the frame buffer. This blends the incoming fragment with the existing color in the frame buffer. The second parameter, that is, the destination RGB, is set as GL_ONE
, which keeps the value in the destination as is. The third parameter is set as GL_ZERO
, which removes the source alpha component as we already applied the alpha from the destination using the first parameter. The final parameter, that is, the destination alpha is set as the conventional over-compositing alpha value (GL_ONE_MINUS_SRC_ALPHA
).
The final shader takes the front peeled result and blends it with the background color using the alpha value from the front peeled result. This way rather than taking the nearest depth fragment all fragments are taken into consideration showing a correctly blended result.
depth peeling works in three steps. First, the scene is rendered normally on a depth FBO with depth testing enabled. This ensures that the scene depth values are stored in the depth attachment of the FBO. In the second pass, we bind the depth FBO, bind the depth texture from the first step, and then iteratively clip parts of the geometry by using a fragment shader (see Chapter6/FrontToBackPeeling/shaders/front_peel.frag
) as shown in the following code snippet:
After this step, we bind the color blend FBO, disable depth test, and then enable alpha blending with separate blending of colors and alpha values. The glBlendFunctionSeparate
function is used here as it enables us to handle color and alpha channels for source and destination separately. The first parameter is the source RGB, which is assigned the alpha value of the pixel in the frame buffer. This blends the incoming fragment with the existing color in the frame buffer. The second parameter, that is, the destination RGB, is set as GL_ONE
, which keeps the value in the destination as is. The third parameter is set as GL_ZERO
, which removes the source alpha component as we already applied the alpha from the destination using the first parameter. The final parameter, that is, the destination alpha is set as the conventional over-compositing alpha value (GL_ONE_MINUS_SRC_ALPHA
).
The final shader takes the front peeled result and blends it with the background color using the alpha value from the front peeled result. This way rather than taking the nearest depth fragment all fragments are taken into consideration showing a correctly blended result.
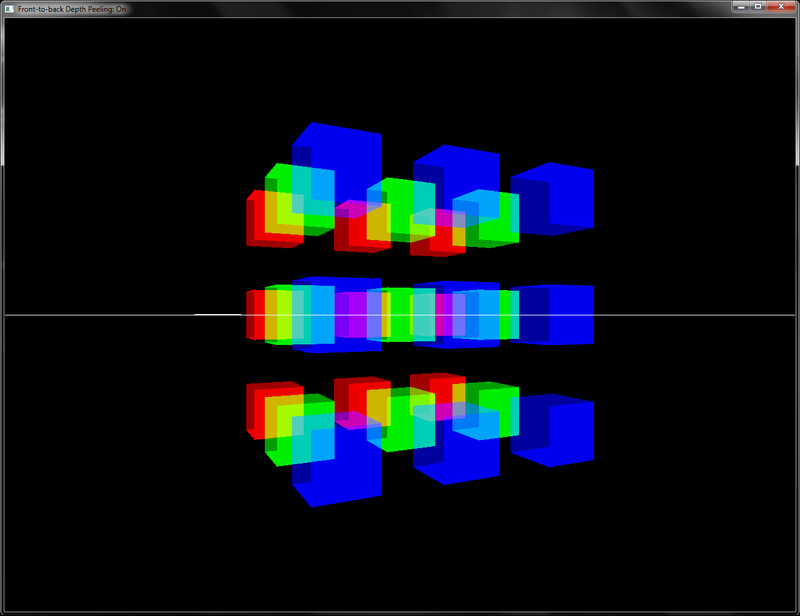
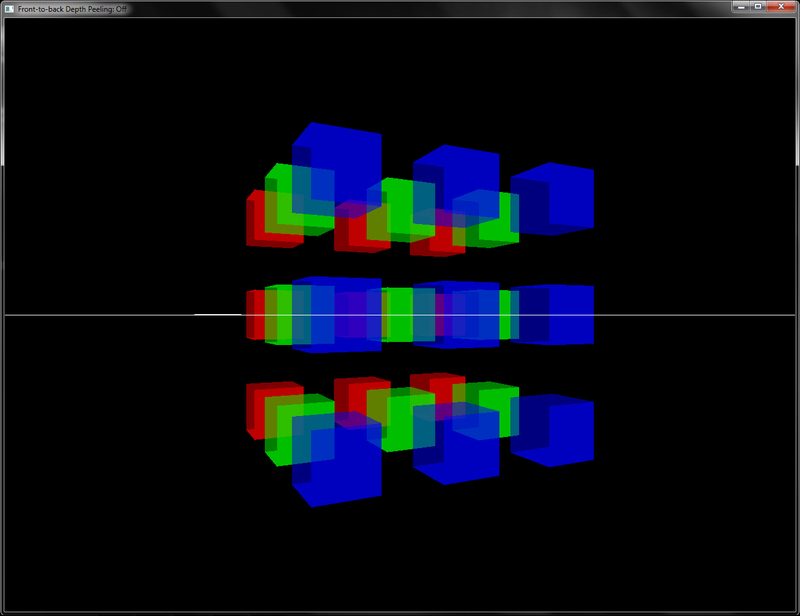
In this recipe, we will implement dual depth peeling. The main idea behind this method is to peel two depth layers at the same time. This results in a much better performance with the same output, as dual depth peeling peels two layers at a time; one from the front and one from the back.
The steps required to implement dual depth peeling are as follows:
- Create an FBO and attach six textures in all: two for storing the front buffer, two for storing the back buffer, and two for storing the depth buffer values.
glGenFramebuffers(1, &dualDepthFBOID); glGenTextures (2, texID); glGenTextures (2, backTexID); glGenTextures (2, depthTexID); for(int i=0;i<2;i++) { glBindTexture(GL_TEXTURE_RECTANGLE, depthTexID[i]); //set texture parameters glTexImage2D(GL_TEXTURE_RECTANGLE , 0, GL_FLOAT_RG32_NV, WIDTH, HEIGHT, 0, GL_RGB, GL_FLOAT, NULL); glBindTexture(GL_TEXTURE_RECTANGLE,texID[i]); //set texture parameters glTexImage2D(GL_TEXTURE_RECTANGLE , 0, GL_RGBA, WIDTH, HEIGHT, 0, GL_RGBA, GL_FLOAT, NULL); glBindTexture(GL_TEXTURE_RECTANGLE,backTexID[i]); //set texture parameters glTexImage2D(GL_TEXTURE_RECTANGLE , 0, GL_RGBA, WIDTH, HEIGHT, 0, GL_RGBA, GL_FLOAT, NULL); }
- Bind the six textures to the appropriate attachment points on the FBO.
glBindFramebuffer(GL_FRAMEBUFFER, dualDepthFBOID); for(int i=0;i<2;i++) { glFramebufferTexture2D(GL_FRAMEBUFFER, attachID[i], GL_TEXTURE_RECTANGLE, depthTexID[i], 0); glFramebufferTexture2D(GL_FRAMEBUFFER, attachID[i]+1, GL_TEXTURE_RECTANGLE, texID[i], 0); glFramebufferTexture2D(GL_FRAMEBUFFER, attachID[i]+2, GL_TEXTURE_RECTANGLE, backTexID[i], 0); }
- Create another FBO for color blending and attach a new texture to it. Also attach this texture to the first FBO and check the FBO completeness.
glGenTextures(1, &colorBlenderTexID); glBindTexture(GL_TEXTURE_RECTANGLE, colorBlenderTexID); //set texture parameters glTexImage2D(GL_TEXTURE_RECTANGLE, 0, GL_RGBA, WIDTH, HEIGHT, 0, GL_RGBA, GL_FLOAT, 0); glGenFramebuffers(1, &colorBlenderFBOID); glBindFramebuffer(GL_FRAMEBUFFER, colorBlenderFBOID); glFramebufferTexture2D(GL_FRAMEBUFFER, GL_COLOR_ATTACHMENT0, GL_TEXTURE_RECTANGLE, colorBlenderTexID, 0); glFramebufferTexture2D(GL_FRAMEBUFFER, GL_COLOR_ATTACHMENT6, GL_TEXTURE_RECTANGLE, colorBlenderTexID, 0); GLenum status = glCheckFramebufferStatus(GL_FRAMEBUFFER); if(status == GL_FRAMEBUFFER_COMPLETE ) printf("FBO setup successful !!! \n"); else printf("Problem with FBO setup"); glBindFramebuffer(GL_FRAMEBUFFER, 0);
- In the render function, first disable depth testing and enable blending and then bind the depth FBO. Initialize and clear
DrawBuffer
to write on the render target attached toGL_COLOR_ATTACHMENT1
andGL_COLOR_ATTACHMENT2
.glDisable(GL_DEPTH_TEST); glEnable(GL_BLEND); glBindFramebuffer(GL_FRAMEBUFFER, dualDepthFBOID); glDrawBuffers(2, &drawBuffers[1]); glClearColor(0, 0, 0, 0); glClear(GL_COLOR_BUFFER_BIT);
- Next, set
GL_COLOR_ATTACHMENT0
as the draw buffer, enable min/max blending (glBlendEquation(GL_MAX)
), and initialize the color attachment using fragment shader (seeChapter6/DualDepthPeeling/shaders/dual_init.frag
). This completes the first step of dual depth peeling, that is, initialization of the buffers.glDrawBuffer(drawBuffers[0]); glClearColor(-MAX_DEPTH, -MAX_DEPTH, 0, 0); glClear(GL_COLOR_BUFFER_BIT); glBlendEquation(GL_MAX); DrawScene(MVP, initShader);
- Next, set
GL_COLOR_ATTACHMENT6
as the draw buffer and clear it with background color. Then, run a loop that alternates two draw buffers and then uses min/max blending. Then draw the scene again.glDrawBuffer(drawBuffers[6]); glClearColor(bg.x, bg.y, bg.z, bg.w); glClear(GL_COLOR_BUFFER_BIT); int numLayers = (NUM_PASSES - 1) * 2; int currId = 0; for (int layer = 1; bUseOQ || layer < numLayers; layer++) { currId = layer % 2; int prevId = 1 - currId; int bufId = currId * 3; glDrawBuffers(2, &drawBuffers[bufId+1]); glClearColor(0, 0, 0, 0); glClear(GL_COLOR_BUFFER_BIT); glDrawBuffer(drawBuffers[bufId+0]); glClearColor(-MAX_DEPTH, -MAX_DEPTH, 0, 0); glClear(GL_COLOR_BUFFER_BIT); glDrawBuffers(3, &drawBuffers[bufId+0]); glBlendEquation(GL_MAX); glActiveTexture(GL_TEXTURE0); glBindTexture(GL_TEXTURE_RECTANGLE, depthTexID[prevId]); glActiveTexture(GL_TEXTURE1); glBindTexture(GL_TEXTURE_RECTANGLE, texID[prevId]); DrawScene(MVP, dualPeelShader, true,true);
- Finally, enable additive blending (
glBlendFunc(GL_FUNC_ADD)
) and then draw a full screen quad with the blend shader. This peels away fragments from the front as well as the back layer of the rendered geometry and blends the result on the current draw buffer.glDrawBuffer(drawBuffers[6]); glBlendEquation(GL_FUNC_ADD); glBlendFunc(GL_SRC_ALPHA, GL_ONE_MINUS_SRC_ALPHA); if (bUseOQ) { glBeginQuery(GL_SAMPLES_PASSED_ARB, queryId); } glActiveTexture(GL_TEXTURE0); glBindTexture(GL_TEXTURE_RECTANGLE, backTexID[currId]); blendShader.Use(); DrawFullScreenQuad(); blendShader.UnUse(); }
- In the final step, we unbind the FBO and enable rendering on the default back buffer (
GL_BACK_LEFT
). Next, we bind the outputs from the depth peeling and blending steps to their appropriate texture location. Finally, we use a final blending shader to combine the two peeled and blended fragments.glBindFramebuffer(GL_FRAMEBUFFER, 0); glDrawBuffer(GL_BACK_LEFT); glBindTexture(GL_TEXTURE_RECTANGLE, colorBlenderTexID); glActiveTexture(GL_TEXTURE0); glBindTexture(GL_TEXTURE_RECTANGLE, depthTexID[currId]); glActiveTexture(GL_TEXTURE1); glBindTexture(GL_TEXTURE_RECTANGLE, texID[currId]); glActiveTexture(GL_TEXTURE2); glBindTexture(GL_TEXTURE_RECTANGLE, colorBlenderTexID); finalShader.Use(); DrawFullScreenQuad(); finalShader.UnUse();
Dual depth peeling works in a similar fashion as the front-to-back peeling. However, the difference is in the way it operates. It peels away depths from both the front and the back layer at the same time using min/max blending. First, we initialize the fragment depth values using the fragment shader (Chapter6/DualDepthPeeling/shaders/dual_init.frag
) and min/max blending.
Finally, the last blend shader (Chapter6/DualDepthPeeling/shaders/final.frag
) takes the blended fragments from the front and back blend textures and blends the results to get the final fragment color.
Chapter6/DualDepthPeeling
folder.
The steps required to implement dual depth peeling are as follows:
- Create an FBO and attach six textures in all: two for storing the front buffer, two for storing the back buffer, and two for storing the depth buffer values.
glGenFramebuffers(1, &dualDepthFBOID); glGenTextures (2, texID); glGenTextures (2, backTexID); glGenTextures (2, depthTexID); for(int i=0;i<2;i++) { glBindTexture(GL_TEXTURE_RECTANGLE, depthTexID[i]); //set texture parameters glTexImage2D(GL_TEXTURE_RECTANGLE , 0, GL_FLOAT_RG32_NV, WIDTH, HEIGHT, 0, GL_RGB, GL_FLOAT, NULL); glBindTexture(GL_TEXTURE_RECTANGLE,texID[i]); //set texture parameters glTexImage2D(GL_TEXTURE_RECTANGLE , 0, GL_RGBA, WIDTH, HEIGHT, 0, GL_RGBA, GL_FLOAT, NULL); glBindTexture(GL_TEXTURE_RECTANGLE,backTexID[i]); //set texture parameters glTexImage2D(GL_TEXTURE_RECTANGLE , 0, GL_RGBA, WIDTH, HEIGHT, 0, GL_RGBA, GL_FLOAT, NULL); }
- Bind the six textures to the appropriate attachment points on the FBO.
glBindFramebuffer(GL_FRAMEBUFFER, dualDepthFBOID); for(int i=0;i<2;i++) { glFramebufferTexture2D(GL_FRAMEBUFFER, attachID[i], GL_TEXTURE_RECTANGLE, depthTexID[i], 0); glFramebufferTexture2D(GL_FRAMEBUFFER, attachID[i]+1, GL_TEXTURE_RECTANGLE, texID[i], 0); glFramebufferTexture2D(GL_FRAMEBUFFER, attachID[i]+2, GL_TEXTURE_RECTANGLE, backTexID[i], 0); }
- Create another FBO for color blending and attach a new texture to it. Also attach this texture to the first FBO and check the FBO completeness.
glGenTextures(1, &colorBlenderTexID); glBindTexture(GL_TEXTURE_RECTANGLE, colorBlenderTexID); //set texture parameters glTexImage2D(GL_TEXTURE_RECTANGLE, 0, GL_RGBA, WIDTH, HEIGHT, 0, GL_RGBA, GL_FLOAT, 0); glGenFramebuffers(1, &colorBlenderFBOID); glBindFramebuffer(GL_FRAMEBUFFER, colorBlenderFBOID); glFramebufferTexture2D(GL_FRAMEBUFFER, GL_COLOR_ATTACHMENT0, GL_TEXTURE_RECTANGLE, colorBlenderTexID, 0); glFramebufferTexture2D(GL_FRAMEBUFFER, GL_COLOR_ATTACHMENT6, GL_TEXTURE_RECTANGLE, colorBlenderTexID, 0); GLenum status = glCheckFramebufferStatus(GL_FRAMEBUFFER); if(status == GL_FRAMEBUFFER_COMPLETE ) printf("FBO setup successful !!! \n"); else printf("Problem with FBO setup"); glBindFramebuffer(GL_FRAMEBUFFER, 0);
- In the render function, first disable depth testing and enable blending and then bind the depth FBO. Initialize and clear
DrawBuffer
to write on the render target attached toGL_COLOR_ATTACHMENT1
andGL_COLOR_ATTACHMENT2
.glDisable(GL_DEPTH_TEST); glEnable(GL_BLEND); glBindFramebuffer(GL_FRAMEBUFFER, dualDepthFBOID); glDrawBuffers(2, &drawBuffers[1]); glClearColor(0, 0, 0, 0); glClear(GL_COLOR_BUFFER_BIT);
- Next, set
GL_COLOR_ATTACHMENT0
as the draw buffer, enable min/max blending (glBlendEquation(GL_MAX)
), and initialize the color attachment using fragment shader (seeChapter6/DualDepthPeeling/shaders/dual_init.frag
). This completes the first step of dual depth peeling, that is, initialization of the buffers.glDrawBuffer(drawBuffers[0]); glClearColor(-MAX_DEPTH, -MAX_DEPTH, 0, 0); glClear(GL_COLOR_BUFFER_BIT); glBlendEquation(GL_MAX); DrawScene(MVP, initShader);
- Next, set
GL_COLOR_ATTACHMENT6
as the draw buffer and clear it with background color. Then, run a loop that alternates two draw buffers and then uses min/max blending. Then draw the scene again.glDrawBuffer(drawBuffers[6]); glClearColor(bg.x, bg.y, bg.z, bg.w); glClear(GL_COLOR_BUFFER_BIT); int numLayers = (NUM_PASSES - 1) * 2; int currId = 0; for (int layer = 1; bUseOQ || layer < numLayers; layer++) { currId = layer % 2; int prevId = 1 - currId; int bufId = currId * 3; glDrawBuffers(2, &drawBuffers[bufId+1]); glClearColor(0, 0, 0, 0); glClear(GL_COLOR_BUFFER_BIT); glDrawBuffer(drawBuffers[bufId+0]); glClearColor(-MAX_DEPTH, -MAX_DEPTH, 0, 0); glClear(GL_COLOR_BUFFER_BIT); glDrawBuffers(3, &drawBuffers[bufId+0]); glBlendEquation(GL_MAX); glActiveTexture(GL_TEXTURE0); glBindTexture(GL_TEXTURE_RECTANGLE, depthTexID[prevId]); glActiveTexture(GL_TEXTURE1); glBindTexture(GL_TEXTURE_RECTANGLE, texID[prevId]); DrawScene(MVP, dualPeelShader, true,true);
- Finally, enable additive blending (
glBlendFunc(GL_FUNC_ADD)
) and then draw a full screen quad with the blend shader. This peels away fragments from the front as well as the back layer of the rendered geometry and blends the result on the current draw buffer.glDrawBuffer(drawBuffers[6]); glBlendEquation(GL_FUNC_ADD); glBlendFunc(GL_SRC_ALPHA, GL_ONE_MINUS_SRC_ALPHA); if (bUseOQ) { glBeginQuery(GL_SAMPLES_PASSED_ARB, queryId); } glActiveTexture(GL_TEXTURE0); glBindTexture(GL_TEXTURE_RECTANGLE, backTexID[currId]); blendShader.Use(); DrawFullScreenQuad(); blendShader.UnUse(); }
- In the final step, we unbind the FBO and enable rendering on the default back buffer (
GL_BACK_LEFT
). Next, we bind the outputs from the depth peeling and blending steps to their appropriate texture location. Finally, we use a final blending shader to combine the two peeled and blended fragments.glBindFramebuffer(GL_FRAMEBUFFER, 0); glDrawBuffer(GL_BACK_LEFT); glBindTexture(GL_TEXTURE_RECTANGLE, colorBlenderTexID); glActiveTexture(GL_TEXTURE0); glBindTexture(GL_TEXTURE_RECTANGLE, depthTexID[currId]); glActiveTexture(GL_TEXTURE1); glBindTexture(GL_TEXTURE_RECTANGLE, texID[currId]); glActiveTexture(GL_TEXTURE2); glBindTexture(GL_TEXTURE_RECTANGLE, colorBlenderTexID); finalShader.Use(); DrawFullScreenQuad(); finalShader.UnUse();
Dual depth peeling works in a similar fashion as the front-to-back peeling. However, the difference is in the way it operates. It peels away depths from both the front and the back layer at the same time using min/max blending. First, we initialize the fragment depth values using the fragment shader (Chapter6/DualDepthPeeling/shaders/dual_init.frag
) and min/max blending.
Finally, the last blend shader (Chapter6/DualDepthPeeling/shaders/final.frag
) takes the blended fragments from the front and back blend textures and blends the results to get the final fragment color.
glGenFramebuffers(1, &dualDepthFBOID); glGenTextures (2, texID); glGenTextures (2, backTexID); glGenTextures (2, depthTexID); for(int i=0;i<2;i++) { glBindTexture(GL_TEXTURE_RECTANGLE, depthTexID[i]); //set texture parameters glTexImage2D(GL_TEXTURE_RECTANGLE , 0, GL_FLOAT_RG32_NV, WIDTH, HEIGHT, 0, GL_RGB, GL_FLOAT, NULL); glBindTexture(GL_TEXTURE_RECTANGLE,texID[i]); //set texture parameters glTexImage2D(GL_TEXTURE_RECTANGLE , 0, GL_RGBA, WIDTH, HEIGHT, 0, GL_RGBA, GL_FLOAT, NULL); glBindTexture(GL_TEXTURE_RECTANGLE,backTexID[i]); //set texture parameters glTexImage2D(GL_TEXTURE_RECTANGLE , 0, GL_RGBA, WIDTH, HEIGHT, 0, GL_RGBA, GL_FLOAT, NULL); }
- six textures to the appropriate attachment points on the FBO.
glBindFramebuffer(GL_FRAMEBUFFER, dualDepthFBOID); for(int i=0;i<2;i++) { glFramebufferTexture2D(GL_FRAMEBUFFER, attachID[i], GL_TEXTURE_RECTANGLE, depthTexID[i], 0); glFramebufferTexture2D(GL_FRAMEBUFFER, attachID[i]+1, GL_TEXTURE_RECTANGLE, texID[i], 0); glFramebufferTexture2D(GL_FRAMEBUFFER, attachID[i]+2, GL_TEXTURE_RECTANGLE, backTexID[i], 0); }
- Create another FBO for color blending and attach a new texture to it. Also attach this texture to the first FBO and check the FBO completeness.
glGenTextures(1, &colorBlenderTexID); glBindTexture(GL_TEXTURE_RECTANGLE, colorBlenderTexID); //set texture parameters glTexImage2D(GL_TEXTURE_RECTANGLE, 0, GL_RGBA, WIDTH, HEIGHT, 0, GL_RGBA, GL_FLOAT, 0); glGenFramebuffers(1, &colorBlenderFBOID); glBindFramebuffer(GL_FRAMEBUFFER, colorBlenderFBOID); glFramebufferTexture2D(GL_FRAMEBUFFER, GL_COLOR_ATTACHMENT0, GL_TEXTURE_RECTANGLE, colorBlenderTexID, 0); glFramebufferTexture2D(GL_FRAMEBUFFER, GL_COLOR_ATTACHMENT6, GL_TEXTURE_RECTANGLE, colorBlenderTexID, 0); GLenum status = glCheckFramebufferStatus(GL_FRAMEBUFFER); if(status == GL_FRAMEBUFFER_COMPLETE ) printf("FBO setup successful !!! \n"); else printf("Problem with FBO setup"); glBindFramebuffer(GL_FRAMEBUFFER, 0);
- In the render function, first disable depth testing and enable blending and then bind the depth FBO. Initialize and clear
DrawBuffer
to write on the render target attached toGL_COLOR_ATTACHMENT1
andGL_COLOR_ATTACHMENT2
.glDisable(GL_DEPTH_TEST); glEnable(GL_BLEND); glBindFramebuffer(GL_FRAMEBUFFER, dualDepthFBOID); glDrawBuffers(2, &drawBuffers[1]); glClearColor(0, 0, 0, 0); glClear(GL_COLOR_BUFFER_BIT);
- Next, set
GL_COLOR_ATTACHMENT0
as the draw buffer, enable min/max blending (glBlendEquation(GL_MAX)
), and initialize the color attachment using fragment shader (seeChapter6/DualDepthPeeling/shaders/dual_init.frag
). This completes the first step of dual depth peeling, that is, initialization of the buffers.glDrawBuffer(drawBuffers[0]); glClearColor(-MAX_DEPTH, -MAX_DEPTH, 0, 0); glClear(GL_COLOR_BUFFER_BIT); glBlendEquation(GL_MAX); DrawScene(MVP, initShader);
- Next, set
GL_COLOR_ATTACHMENT6
as the draw buffer and clear it with background color. Then, run a loop that alternates two draw buffers and then uses min/max blending. Then draw the scene again.glDrawBuffer(drawBuffers[6]); glClearColor(bg.x, bg.y, bg.z, bg.w); glClear(GL_COLOR_BUFFER_BIT); int numLayers = (NUM_PASSES - 1) * 2; int currId = 0; for (int layer = 1; bUseOQ || layer < numLayers; layer++) { currId = layer % 2; int prevId = 1 - currId; int bufId = currId * 3; glDrawBuffers(2, &drawBuffers[bufId+1]); glClearColor(0, 0, 0, 0); glClear(GL_COLOR_BUFFER_BIT); glDrawBuffer(drawBuffers[bufId+0]); glClearColor(-MAX_DEPTH, -MAX_DEPTH, 0, 0); glClear(GL_COLOR_BUFFER_BIT); glDrawBuffers(3, &drawBuffers[bufId+0]); glBlendEquation(GL_MAX); glActiveTexture(GL_TEXTURE0); glBindTexture(GL_TEXTURE_RECTANGLE, depthTexID[prevId]); glActiveTexture(GL_TEXTURE1); glBindTexture(GL_TEXTURE_RECTANGLE, texID[prevId]); DrawScene(MVP, dualPeelShader, true,true);
- Finally, enable additive blending (
glBlendFunc(GL_FUNC_ADD)
) and then draw a full screen quad with the blend shader. This peels away fragments from the front as well as the back layer of the rendered geometry and blends the result on the current draw buffer.glDrawBuffer(drawBuffers[6]); glBlendEquation(GL_FUNC_ADD); glBlendFunc(GL_SRC_ALPHA, GL_ONE_MINUS_SRC_ALPHA); if (bUseOQ) { glBeginQuery(GL_SAMPLES_PASSED_ARB, queryId); } glActiveTexture(GL_TEXTURE0); glBindTexture(GL_TEXTURE_RECTANGLE, backTexID[currId]); blendShader.Use(); DrawFullScreenQuad(); blendShader.UnUse(); }
- In the final step, we unbind the FBO and enable rendering on the default back buffer (
GL_BACK_LEFT
). Next, we bind the outputs from the depth peeling and blending steps to their appropriate texture location. Finally, we use a final blending shader to combine the two peeled and blended fragments.glBindFramebuffer(GL_FRAMEBUFFER, 0); glDrawBuffer(GL_BACK_LEFT); glBindTexture(GL_TEXTURE_RECTANGLE, colorBlenderTexID); glActiveTexture(GL_TEXTURE0); glBindTexture(GL_TEXTURE_RECTANGLE, depthTexID[currId]); glActiveTexture(GL_TEXTURE1); glBindTexture(GL_TEXTURE_RECTANGLE, texID[currId]); glActiveTexture(GL_TEXTURE2); glBindTexture(GL_TEXTURE_RECTANGLE, colorBlenderTexID); finalShader.Use(); DrawFullScreenQuad(); finalShader.UnUse();
Dual depth peeling works in a similar fashion as the front-to-back peeling. However, the difference is in the way it operates. It peels away depths from both the front and the back layer at the same time using min/max blending. First, we initialize the fragment depth values using the fragment shader (Chapter6/DualDepthPeeling/shaders/dual_init.frag
) and min/max blending.
Finally, the last blend shader (Chapter6/DualDepthPeeling/shaders/final.frag
) takes the blended fragments from the front and back blend textures and blends the results to get the final fragment color.
works in a similar fashion as the front-to-back peeling. However, the difference is in the way it operates. It peels away depths from both the front and the back layer at the same time using min/max blending. First, we initialize the fragment depth values using the fragment shader (Chapter6/DualDepthPeeling/shaders/dual_init.frag
) and min/max blending.
Finally, the last blend shader (Chapter6/DualDepthPeeling/shaders/final.frag
) takes the blended fragments from the front and back blend textures and blends the results to get the final fragment color.
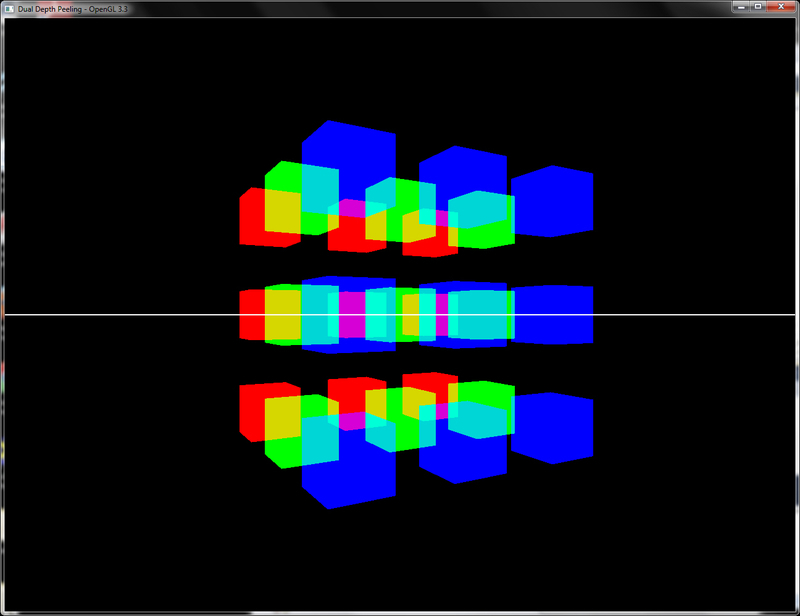
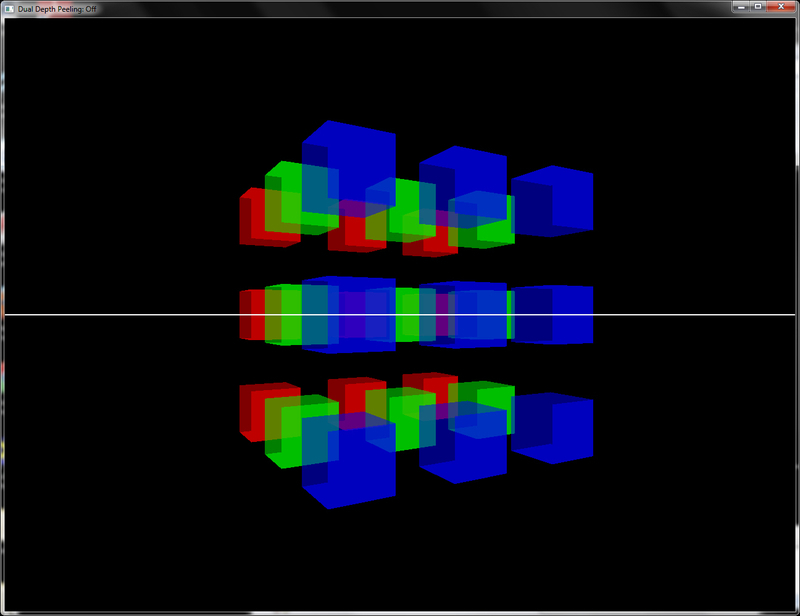
We have implemented simple lighting recipes in previous chapters. These unfortunately approximate some aspects of lighting. However, effects such as global illumination are not handled by the basic lights, as discussed earlier. In this respect, several techniques have been developed over the years which fake the global illumination effects. One such technique is Screen Space Ambient Occlusion (SSAO) which we will implement in this recipe.
The ambient occlusion procedure is defined as follows:
The whole recipe proceeds as follows. We load our 3D model and render it into an offscreen texture using FBO. We use two FBOs: one for storing the eye space normals and depth, and another FBO is for filtering of intermediate results. For both the color attachment and the depth attachment of first FBO, floating point texture formats are used. For the color attachment, GL_RGBA32F
is used, whereas for depth texture, the GL_DEPTH_COMPONENT32F
floating point format is used. Floating point texture formats are used as we require more precision, otherwise truncation errors will show up in the rendering result. The second FBO is used for separable Gaussian smoothing as was carried out in the Implementing variance shadow mapping recipe in Chapter 4, Lights and Shadows. This FBO has two color attachments with the floating point texture format GL_RGBA32F
.
In the rendering function, the scene is first rendered normally. Then, the first shader is used to output the eye space normals. This is stored in the color attachment and the depth values are stored in the depth attachment of the first FBO. After this step, the filtering FBO is bound and the second shader is used, which uses the depth and normal textures from the first FBO to calculate the ambient occlusion result. Since the neighbor points are randomly offset, noise is introduced. The noisy result is then smoothed by applying separable gaussian smoothing. Finally, the filtered result is blended with the existing rendering by using conventional alpha blending.
The code for this recipe is contained in the Chapter6/SSAO
folder. We will be using the Obj model viewer from Chapter 5, Mesh Model Formats and and Particle Systems. We will add SSAO to the Obj model.
Let us start the recipe by following these simple steps:
- Create a global reference of the
ObjLoader
object. Call theObjLoader::Load
function passing it the name of the OBJ file. Pass vectors to store themeshes
,vertices
,indices,
andmaterials
contained in the OBJ file. - Create a framebuffer object (FBO) with two attachments: first to store the scene normals and second to store the depth. We will use a floating point texture format (
GL_RGBA32F
) for both of these. In addition, we create a second FBO for Gaussian smoothing of the SSAO output. We are using multiple texture units here as the second shader expects normal and depth textures to be bound to texture units 1 and 3 respectively.glGenFramebuffers(1, &fboID); glBindFramebuffer(GL_FRAMEBUFFER, fboID); glGenTextures(1, &normalTextureID); glGenTextures(1, &depthTextureID); glActiveTexture(GL_TEXTURE1); glBindTexture(GL_TEXTURE_2D, normalTextureID); //set texture parameters glTexImage2D(GL_TEXTURE_2D, 0, GL_RGBA32F, WIDTH, HEIGHT, 0, GL_BGRA, GL_FLOAT, NULL); glActiveTexture(GL_TEXTURE3); glBindTexture(GL_TEXTURE_2D, depthTextureID); //set texture parameters glTexImage2D(GL_TEXTURE_2D, 0, GL_DEPTH_COMPONENT32F, WIDTH, HEIGHT, 0, GL_DEPTH_COMPONENT, GL_FLOAT, NULL); glFramebufferTexture2D(GL_FRAMEBUFFER, GL_COLOR_ATTACHMENT0, GL_TEXTURE_2D, normalTextureID, 0); glFramebufferTexture2D(GL_FRAMEBUFFER, GL_DEPTH_ATTACHMENT,GL_TEXTURE_2D, depthTextureID, 0); glGenFramebuffers(1,&filterFBOID); glBindFramebuffer(GL_FRAMEBUFFER,filterFBOID); glGenTextures(2, blurTexID); for(int i=0;i<2;i++) { glActiveTexture(GL_TEXTURE4+i); glBindTexture(GL_TEXTURE_2D, blurTexID[i]); //set texture parameters glTexImage2D(GL_TEXTURE_2D,0,GL_RGBA32F,RTT_WIDTH, RTT_HEIGHT,0,GL_RGBA,GL_FLOAT,NULL); glFramebufferTexture2D(GL_FRAMEBUFFER,GL_COLOR_ATTACHMENT0+i,GL_TEXTURE_2D,blurTexID[i],0); }
- In the render function, render the scene meshes normally. After this step, bind the first FBO and then use the first shader program. This program takes the per-vertex positions/normals of the mesh and outputs the view space normals from the fragment shader.
glBindFramebuffer(GL_FRAMEBUFFER, fboID); glViewport(0,0,RTT_WIDTH, RTT_HEIGHT); glDrawBuffer(GL_COLOR_ATTACHMENT0); glClear(GL_COLOR_BUFFER_BIT|GL_DEPTH_BUFFER_BIT); glBindVertexArray(vaoID); { ssaoFirstShader.Use(); glUniformMatrix4fv(ssaoFirstShader("MVP"), 1, GL_FALSE, glm::value_ptr(P*MV)); glUniformMatrix3fv(ssaoFirstShader("N"), 1, GL_FALSE, glm::value_ptr(glm::inverseTranspose(glm::mat3(MV)))); for(size_t i=0;i<materials.size();i++) { Material* pMat = materials[i]; if(materials.size()==1) glDrawElements(GL_TRIANGLES, indices.size(), GL_UNSIGNED_SHORT, 0); else glDrawElements(GL_TRIANGLES, pMat->count, GL_UNSIGNED_SHORT, (const GLvoid*)(&indices[pMat->offset])); } ssaoFirstShader.UnUse(); }
The first vertex shader (
Chapter6/SSAO/shaders/SSAO_FirstStep.vert
) outputs the eye space normal as shown in the following code snippet:#version 330 core layout(location = 0) in vec3 vVertex; layout(location = 1) in vec3 vNormal; uniform mat4 MVP; uniform mat3 N; smooth out vec3 vEyeSpaceNormal; void main() { vEyeSpaceNormal = N*vNormal; gl_Position = MVP*vec4(vVertex,1); }
The fragment shader (
Chapter6/SSAO/shaders/SSAO_FirstStep.frag
) returns the interpolated normal, as the fragment color, shown as follows: - Bind the filtering FBO and use the second shader (
Chapter6/SSAO/shaders/SSAO_SecondStep.frag
). This shader does the actual SSAO calculation. The input to the shader is the normals texture from step 3. This shader is invoked on a full screen quad.glBindFramebuffer(GL_FRAMEBUFFER,filterFBOID); glDrawBuffer(GL_COLOR_ATTACHMENT0); glBindVertexArray(quadVAOID); ssaoSecondShader.Use(); glUniform1f(ssaoSecondShader("radius"), sampling_radius); glDrawElements(GL_TRIANGLES, 6, GL_UNSIGNED_SHORT, 0); ssaoSecondShader.UnUse();
- Filter the output from step 4 by using separable Gaussian convolution using two fragment shaders (
Chapter6/SSAO/shaders/GaussH.frag and Chapter6/SSAO/shaders/GaussV.frag
). The separable Gaussian smoothing is added in to smooth out the ambient occlusion result.glDrawBuffer(GL_COLOR_ATTACHMENT1); glBindVertexArray(quadVAOID); gaussianV_shader.Use(); glDrawElements(GL_TRIANGLES, 6, GL_UNSIGNED_SHORT, 0); glDrawBuffer(GL_COLOR_ATTACHMENT0); gaussianH_shader.Use(); glDrawElements(GL_TRIANGLES, 6, GL_UNSIGNED_SHORT, 0);
- Unbind the filtering FBO, reset the default viewport, and then the default draw buffer. Enable alpha blending and then use the final shader (
Chapter6/SSAO/shaders/final.frag
) to blend the output from steps 3 and 5. This shader simply renders the final output from the filtering stage using a full-screen quad.glBindFramebuffer(GL_FRAMEBUFFER,0); glViewport(0,0,WIDTH, HEIGHT); glDrawBuffer(GL_BACK_LEFT); glEnable(GL_BLEND); glBlendFunc(GL_SRC_ALPHA, GL_ONE_MINUS_SRC_ALPHA); finalShader.Use(); glDrawElements(GL_TRIANGLES, 6, GL_UNSIGNED_SHORT, 0); finalShader.UnUse(); glDisable(GL_BLEND);
There are three steps in the SSAO calculation. The first step is the preparation of inputs, that is, the view space normals and depth. The normals are stored using the first step vertex shader (Chapter6/SSAO/shaders/SSAO_FirstStep.vert
).
After the second shader, we filter the SSAO output using separable Gaussian convolution. The default draw buffer is then restored and then the Gaussian filtered SSAO output is alpha blended with the normal rendering.
recipe is contained in the Chapter6/SSAO
folder. We will be using the Obj model viewer from Chapter 5, Mesh Model Formats and and Particle Systems. We will add SSAO to the Obj model.
Let us start the recipe by following these simple steps:
- Create a global reference of the
ObjLoader
object. Call theObjLoader::Load
function passing it the name of the OBJ file. Pass vectors to store themeshes
,vertices
,indices,
andmaterials
contained in the OBJ file. - Create a framebuffer object (FBO) with two attachments: first to store the scene normals and second to store the depth. We will use a floating point texture format (
GL_RGBA32F
) for both of these. In addition, we create a second FBO for Gaussian smoothing of the SSAO output. We are using multiple texture units here as the second shader expects normal and depth textures to be bound to texture units 1 and 3 respectively.glGenFramebuffers(1, &fboID); glBindFramebuffer(GL_FRAMEBUFFER, fboID); glGenTextures(1, &normalTextureID); glGenTextures(1, &depthTextureID); glActiveTexture(GL_TEXTURE1); glBindTexture(GL_TEXTURE_2D, normalTextureID); //set texture parameters glTexImage2D(GL_TEXTURE_2D, 0, GL_RGBA32F, WIDTH, HEIGHT, 0, GL_BGRA, GL_FLOAT, NULL); glActiveTexture(GL_TEXTURE3); glBindTexture(GL_TEXTURE_2D, depthTextureID); //set texture parameters glTexImage2D(GL_TEXTURE_2D, 0, GL_DEPTH_COMPONENT32F, WIDTH, HEIGHT, 0, GL_DEPTH_COMPONENT, GL_FLOAT, NULL); glFramebufferTexture2D(GL_FRAMEBUFFER, GL_COLOR_ATTACHMENT0, GL_TEXTURE_2D, normalTextureID, 0); glFramebufferTexture2D(GL_FRAMEBUFFER, GL_DEPTH_ATTACHMENT,GL_TEXTURE_2D, depthTextureID, 0); glGenFramebuffers(1,&filterFBOID); glBindFramebuffer(GL_FRAMEBUFFER,filterFBOID); glGenTextures(2, blurTexID); for(int i=0;i<2;i++) { glActiveTexture(GL_TEXTURE4+i); glBindTexture(GL_TEXTURE_2D, blurTexID[i]); //set texture parameters glTexImage2D(GL_TEXTURE_2D,0,GL_RGBA32F,RTT_WIDTH, RTT_HEIGHT,0,GL_RGBA,GL_FLOAT,NULL); glFramebufferTexture2D(GL_FRAMEBUFFER,GL_COLOR_ATTACHMENT0+i,GL_TEXTURE_2D,blurTexID[i],0); }
- In the render function, render the scene meshes normally. After this step, bind the first FBO and then use the first shader program. This program takes the per-vertex positions/normals of the mesh and outputs the view space normals from the fragment shader.
glBindFramebuffer(GL_FRAMEBUFFER, fboID); glViewport(0,0,RTT_WIDTH, RTT_HEIGHT); glDrawBuffer(GL_COLOR_ATTACHMENT0); glClear(GL_COLOR_BUFFER_BIT|GL_DEPTH_BUFFER_BIT); glBindVertexArray(vaoID); { ssaoFirstShader.Use(); glUniformMatrix4fv(ssaoFirstShader("MVP"), 1, GL_FALSE, glm::value_ptr(P*MV)); glUniformMatrix3fv(ssaoFirstShader("N"), 1, GL_FALSE, glm::value_ptr(glm::inverseTranspose(glm::mat3(MV)))); for(size_t i=0;i<materials.size();i++) { Material* pMat = materials[i]; if(materials.size()==1) glDrawElements(GL_TRIANGLES, indices.size(), GL_UNSIGNED_SHORT, 0); else glDrawElements(GL_TRIANGLES, pMat->count, GL_UNSIGNED_SHORT, (const GLvoid*)(&indices[pMat->offset])); } ssaoFirstShader.UnUse(); }
The first vertex shader (
Chapter6/SSAO/shaders/SSAO_FirstStep.vert
) outputs the eye space normal as shown in the following code snippet:#version 330 core layout(location = 0) in vec3 vVertex; layout(location = 1) in vec3 vNormal; uniform mat4 MVP; uniform mat3 N; smooth out vec3 vEyeSpaceNormal; void main() { vEyeSpaceNormal = N*vNormal; gl_Position = MVP*vec4(vVertex,1); }
The fragment shader (
Chapter6/SSAO/shaders/SSAO_FirstStep.frag
) returns the interpolated normal, as the fragment color, shown as follows: - Bind the filtering FBO and use the second shader (
Chapter6/SSAO/shaders/SSAO_SecondStep.frag
). This shader does the actual SSAO calculation. The input to the shader is the normals texture from step 3. This shader is invoked on a full screen quad.glBindFramebuffer(GL_FRAMEBUFFER,filterFBOID); glDrawBuffer(GL_COLOR_ATTACHMENT0); glBindVertexArray(quadVAOID); ssaoSecondShader.Use(); glUniform1f(ssaoSecondShader("radius"), sampling_radius); glDrawElements(GL_TRIANGLES, 6, GL_UNSIGNED_SHORT, 0); ssaoSecondShader.UnUse();
- Filter the output from step 4 by using separable Gaussian convolution using two fragment shaders (
Chapter6/SSAO/shaders/GaussH.frag and Chapter6/SSAO/shaders/GaussV.frag
). The separable Gaussian smoothing is added in to smooth out the ambient occlusion result.glDrawBuffer(GL_COLOR_ATTACHMENT1); glBindVertexArray(quadVAOID); gaussianV_shader.Use(); glDrawElements(GL_TRIANGLES, 6, GL_UNSIGNED_SHORT, 0); glDrawBuffer(GL_COLOR_ATTACHMENT0); gaussianH_shader.Use(); glDrawElements(GL_TRIANGLES, 6, GL_UNSIGNED_SHORT, 0);
- Unbind the filtering FBO, reset the default viewport, and then the default draw buffer. Enable alpha blending and then use the final shader (
Chapter6/SSAO/shaders/final.frag
) to blend the output from steps 3 and 5. This shader simply renders the final output from the filtering stage using a full-screen quad.glBindFramebuffer(GL_FRAMEBUFFER,0); glViewport(0,0,WIDTH, HEIGHT); glDrawBuffer(GL_BACK_LEFT); glEnable(GL_BLEND); glBlendFunc(GL_SRC_ALPHA, GL_ONE_MINUS_SRC_ALPHA); finalShader.Use(); glDrawElements(GL_TRIANGLES, 6, GL_UNSIGNED_SHORT, 0); finalShader.UnUse(); glDisable(GL_BLEND);
There are three steps in the SSAO calculation. The first step is the preparation of inputs, that is, the view space normals and depth. The normals are stored using the first step vertex shader (Chapter6/SSAO/shaders/SSAO_FirstStep.vert
).
After the second shader, we filter the SSAO output using separable Gaussian convolution. The default draw buffer is then restored and then the Gaussian filtered SSAO output is alpha blended with the normal rendering.
ObjLoader
object. Call the ObjLoader::Load
function passing it the name of the OBJ file. Pass vectors to store the meshes
, vertices
, indices,
and materials
contained in the OBJ file.
GL_RGBA32F
) for both of these. In addition, we create a second FBO for Gaussian smoothing of the SSAO output. We are using multiple texture units here as the second shader expects normal and depth textures to be bound to texture units 1 and 3 respectively.glGenFramebuffers(1, &fboID); glBindFramebuffer(GL_FRAMEBUFFER, fboID); glGenTextures(1, &normalTextureID); glGenTextures(1, &depthTextureID); glActiveTexture(GL_TEXTURE1); glBindTexture(GL_TEXTURE_2D, normalTextureID); //set texture parameters glTexImage2D(GL_TEXTURE_2D, 0, GL_RGBA32F, WIDTH, HEIGHT, 0, GL_BGRA, GL_FLOAT, NULL); glActiveTexture(GL_TEXTURE3); glBindTexture(GL_TEXTURE_2D, depthTextureID); //set texture parameters glTexImage2D(GL_TEXTURE_2D, 0, GL_DEPTH_COMPONENT32F, WIDTH, HEIGHT, 0, GL_DEPTH_COMPONENT, GL_FLOAT, NULL); glFramebufferTexture2D(GL_FRAMEBUFFER, GL_COLOR_ATTACHMENT0, GL_TEXTURE_2D, normalTextureID, 0); glFramebufferTexture2D(GL_FRAMEBUFFER, GL_DEPTH_ATTACHMENT,GL_TEXTURE_2D, depthTextureID, 0); glGenFramebuffers(1,&filterFBOID); glBindFramebuffer(GL_FRAMEBUFFER,filterFBOID); glGenTextures(2, blurTexID); for(int i=0;i<2;i++) { glActiveTexture(GL_TEXTURE4+i); glBindTexture(GL_TEXTURE_2D, blurTexID[i]); //set texture parameters glTexImage2D(GL_TEXTURE_2D,0,GL_RGBA32F,RTT_WIDTH, RTT_HEIGHT,0,GL_RGBA,GL_FLOAT,NULL); glFramebufferTexture2D(GL_FRAMEBUFFER,GL_COLOR_ATTACHMENT0+i,GL_TEXTURE_2D,blurTexID[i],0); }
- function, render the scene meshes normally. After this step, bind the first FBO and then use the first shader program. This program takes the per-vertex positions/normals of the mesh and outputs the view space normals from the fragment shader.
glBindFramebuffer(GL_FRAMEBUFFER, fboID); glViewport(0,0,RTT_WIDTH, RTT_HEIGHT); glDrawBuffer(GL_COLOR_ATTACHMENT0); glClear(GL_COLOR_BUFFER_BIT|GL_DEPTH_BUFFER_BIT); glBindVertexArray(vaoID); { ssaoFirstShader.Use(); glUniformMatrix4fv(ssaoFirstShader("MVP"), 1, GL_FALSE, glm::value_ptr(P*MV)); glUniformMatrix3fv(ssaoFirstShader("N"), 1, GL_FALSE, glm::value_ptr(glm::inverseTranspose(glm::mat3(MV)))); for(size_t i=0;i<materials.size();i++) { Material* pMat = materials[i]; if(materials.size()==1) glDrawElements(GL_TRIANGLES, indices.size(), GL_UNSIGNED_SHORT, 0); else glDrawElements(GL_TRIANGLES, pMat->count, GL_UNSIGNED_SHORT, (const GLvoid*)(&indices[pMat->offset])); } ssaoFirstShader.UnUse(); }
The first vertex shader (
Chapter6/SSAO/shaders/SSAO_FirstStep.vert
) outputs the eye space normal as shown in the following code snippet:#version 330 core layout(location = 0) in vec3 vVertex; layout(location = 1) in vec3 vNormal; uniform mat4 MVP; uniform mat3 N; smooth out vec3 vEyeSpaceNormal; void main() { vEyeSpaceNormal = N*vNormal; gl_Position = MVP*vec4(vVertex,1); }
The fragment shader (
Chapter6/SSAO/shaders/SSAO_FirstStep.frag
) returns the interpolated normal, as the fragment color, shown as follows: - Bind the filtering FBO and use the second shader (
Chapter6/SSAO/shaders/SSAO_SecondStep.frag
). This shader does the actual SSAO calculation. The input to the shader is the normals texture from step 3. This shader is invoked on a full screen quad.glBindFramebuffer(GL_FRAMEBUFFER,filterFBOID); glDrawBuffer(GL_COLOR_ATTACHMENT0); glBindVertexArray(quadVAOID); ssaoSecondShader.Use(); glUniform1f(ssaoSecondShader("radius"), sampling_radius); glDrawElements(GL_TRIANGLES, 6, GL_UNSIGNED_SHORT, 0); ssaoSecondShader.UnUse();
- Filter the output from step 4 by using separable Gaussian convolution using two fragment shaders (
Chapter6/SSAO/shaders/GaussH.frag and Chapter6/SSAO/shaders/GaussV.frag
). The separable Gaussian smoothing is added in to smooth out the ambient occlusion result.glDrawBuffer(GL_COLOR_ATTACHMENT1); glBindVertexArray(quadVAOID); gaussianV_shader.Use(); glDrawElements(GL_TRIANGLES, 6, GL_UNSIGNED_SHORT, 0); glDrawBuffer(GL_COLOR_ATTACHMENT0); gaussianH_shader.Use(); glDrawElements(GL_TRIANGLES, 6, GL_UNSIGNED_SHORT, 0);
- Unbind the filtering FBO, reset the default viewport, and then the default draw buffer. Enable alpha blending and then use the final shader (
Chapter6/SSAO/shaders/final.frag
) to blend the output from steps 3 and 5. This shader simply renders the final output from the filtering stage using a full-screen quad.glBindFramebuffer(GL_FRAMEBUFFER,0); glViewport(0,0,WIDTH, HEIGHT); glDrawBuffer(GL_BACK_LEFT); glEnable(GL_BLEND); glBlendFunc(GL_SRC_ALPHA, GL_ONE_MINUS_SRC_ALPHA); finalShader.Use(); glDrawElements(GL_TRIANGLES, 6, GL_UNSIGNED_SHORT, 0); finalShader.UnUse(); glDisable(GL_BLEND);
There are three steps in the SSAO calculation. The first step is the preparation of inputs, that is, the view space normals and depth. The normals are stored using the first step vertex shader (Chapter6/SSAO/shaders/SSAO_FirstStep.vert
).
After the second shader, we filter the SSAO output using separable Gaussian convolution. The default draw buffer is then restored and then the Gaussian filtered SSAO output is alpha blended with the normal rendering.
After the second shader, we filter the SSAO output using separable Gaussian convolution. The default draw buffer is then restored and then the Gaussian filtered SSAO output is alpha blended with the normal rendering.
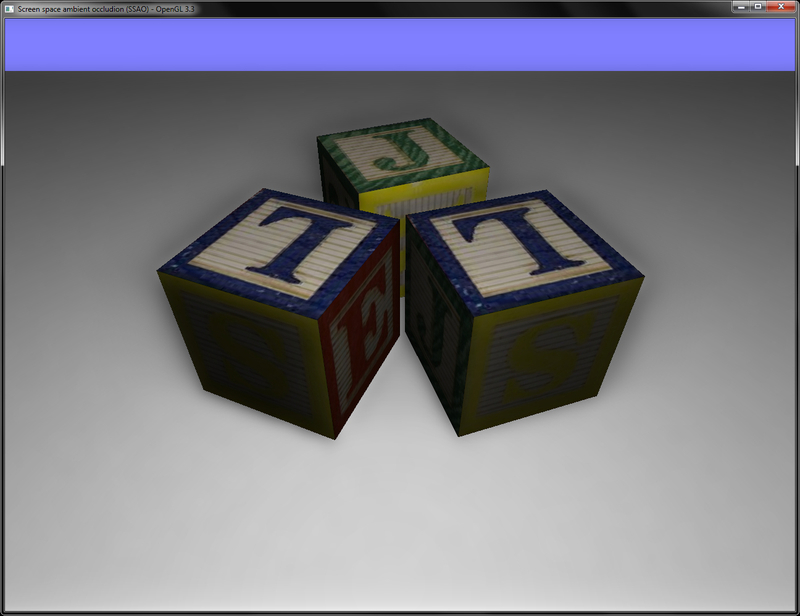
In this recipe, we will learn about implementing simple global illumination using spherical harmonics. Spherical harmonics is a class of methods that enable approximation of functions as a product of a set of coefficients with a set of basis functions. Rather than calculating the lighting contribution by evaluating the bi-directional reflectance distribution function (BRDF), this method uses special HDR/RGBE images that store the lighting information. The only attribute required for this method is the per-vertex normal. These are multiplied with the spherical harmonics coefficients that are extracted from the HDR/RGBE images.
Let us start this recipe by following these simple steps:
- Load an
obj
mesh using theObjLoader
class and fill the OpenGL buffer objects and the OpenGL textures, using the material information loaded from the file, as in the previous recipes. - In the vertex shader that is used for the mesh, perform the lighting calculation using spherical harmonics. The vertex shader is detailed as follows:
#version 330 core layout(location = 0) in vec3 vVertex; layout(location = 1) in vec3 vNormal; layout(location = 2) in vec2 vUV; smooth out vec2 vUVout; smooth out vec4 diffuse; uniform mat4 P; uniform mat4 MV; uniform mat3 N; const float C1 = 0.429043; const float C2 = 0.511664; const float C3 = 0.743125; const float C4 = 0.886227; const float C5 = 0.247708; const float PI = 3.1415926535897932384626433832795; //Old town square probe const vec3 L00 = vec3( 0.871297, 0.875222, 0.864470); const vec3 L1m1 = vec3( 0.175058, 0.245335, 0.312891); const vec3 L10 = vec3( 0.034675, 0.036107, 0.037362); const vec3 L11 = vec3(-0.004629, -0.029448, -0.048028); const vec3 L2m2 = vec3(-0.120535, -0.121160, -0.117507); const vec3 L2m1 = vec3( 0.003242, 0.003624, 0.007511); const vec3 L20 = vec3(-0.028667, -0.024926, -0.020998); const vec3 L21 = vec3(-0.077539, -0.086325, -0.091591); const vec3 L22 = vec3(-0.161784, -0.191783, -0.219152); const vec3 scaleFactor = vec3(0.161784/ (0.871297+0.161784), 0.191783/(0.875222+0.191783), 0.219152/(0.864470+0.219152)); void main() { vUVout=vUV; vec3 tmpN = normalize(N*vNormal); vec3 diff = C1 * L22 * (tmpN.x*tmpN.x - tmpN.y*tmpN.y) + C3 * L20 * tmpN.z*tmpN.z + C4 * L00 - C5 * L20 + 2.0 * C1 * L2m2*tmpN.x*tmpN.y + 2.0 * C1 * L21*tmpN.x*tmpN.z + 2.0 * C1 * L2m1*tmpN.y*tmpN.z + 2.0 * C2 * L11*tmpN.x +2.0 * C2 * L1m1*tmpN.y +2.0 * C2 * L10*tmpN.z; diff *= scaleFactor; diffuse = vec4(diff, 1); gl_Position = P*(MV*vec4(vVertex,1)); }
- The per-vertex color calculated by the vertex shader is interpolated by the rasterizer and then the fragment shader sets the color as the current fragment color.
#version 330 core uniform sampler2D textureMap; uniform float useDefault; smooth in vec4 diffuse; smooth in vec2 vUVout; layout(location=0) out vec4 vFragColor; void main() { vFragColor = mix(texture(textureMap, vUVout)*diffuse, diffuse, useDefault); }
Spherical harmonics is a technique that approximates the lighting, using coefficients and spherical harmonics basis. The coefficients are obtained at initialization from an HDR/RGBE image file that contains information about lighting. This allows us to approximate the same light so the graphical scene feels more immersive.
The demo application implementing this recipe renders the same scene as in the previous recipes, as shown in the following figure. We can rotate the camera view using the left mouse button, whereas, the point light source can be rotated using the right mouse button. Pressing the Space bar toggles the use of spherical harmonics. When spherical harmonics lighting is on, we get the following result:
Without the spherical harmonics lighting, the result is as follows:
The probe image used for this image is shown in the following figure:
- Ravi Ramamoorthi and Pat Hanrahan, An Efficient Representation for Irradiance Environment Maps: http://www1.cs.columbia.edu/~ravir/papers/envmap/index.html
- Randi J. Rost, Mill M. Licea-Kane, Dan Ginsburg, John M. Kessenich, Barthold Lichtenbelt, Hugh Malan, Mike Weiblen, OpenGL Shading Language, Third Edition, Section 12.3, Lighting and Spherical Harmonics, Addison-Wesley Professional
- Kelly Dempski and Emmanuel Viale, Advanced Lighting and Materials with Shaders, Chapter 8, Spherical Harmonic Lighting, Jones & Bartlett Publishers
- The RGBE image format specifications: http://www.graphics.cornell.edu/online/formats/rgbe/
- Paul Debevec HDR light probes: http://www.pauldebevec.com/Probes/
- Spherical harmonics lighting tutorial: http://www.paulsprojects.net/opengl/sh/sh.html
Chapter6/SphericalHarmonics
directory. For this recipe, we will be using the Obj mesh loader discussed in the previous chapter.
Let us start this recipe by following these simple steps:
- Load an
obj
mesh using theObjLoader
class and fill the OpenGL buffer objects and the OpenGL textures, using the material information loaded from the file, as in the previous recipes. - In the vertex shader that is used for the mesh, perform the lighting calculation using spherical harmonics. The vertex shader is detailed as follows:
#version 330 core layout(location = 0) in vec3 vVertex; layout(location = 1) in vec3 vNormal; layout(location = 2) in vec2 vUV; smooth out vec2 vUVout; smooth out vec4 diffuse; uniform mat4 P; uniform mat4 MV; uniform mat3 N; const float C1 = 0.429043; const float C2 = 0.511664; const float C3 = 0.743125; const float C4 = 0.886227; const float C5 = 0.247708; const float PI = 3.1415926535897932384626433832795; //Old town square probe const vec3 L00 = vec3( 0.871297, 0.875222, 0.864470); const vec3 L1m1 = vec3( 0.175058, 0.245335, 0.312891); const vec3 L10 = vec3( 0.034675, 0.036107, 0.037362); const vec3 L11 = vec3(-0.004629, -0.029448, -0.048028); const vec3 L2m2 = vec3(-0.120535, -0.121160, -0.117507); const vec3 L2m1 = vec3( 0.003242, 0.003624, 0.007511); const vec3 L20 = vec3(-0.028667, -0.024926, -0.020998); const vec3 L21 = vec3(-0.077539, -0.086325, -0.091591); const vec3 L22 = vec3(-0.161784, -0.191783, -0.219152); const vec3 scaleFactor = vec3(0.161784/ (0.871297+0.161784), 0.191783/(0.875222+0.191783), 0.219152/(0.864470+0.219152)); void main() { vUVout=vUV; vec3 tmpN = normalize(N*vNormal); vec3 diff = C1 * L22 * (tmpN.x*tmpN.x - tmpN.y*tmpN.y) + C3 * L20 * tmpN.z*tmpN.z + C4 * L00 - C5 * L20 + 2.0 * C1 * L2m2*tmpN.x*tmpN.y + 2.0 * C1 * L21*tmpN.x*tmpN.z + 2.0 * C1 * L2m1*tmpN.y*tmpN.z + 2.0 * C2 * L11*tmpN.x +2.0 * C2 * L1m1*tmpN.y +2.0 * C2 * L10*tmpN.z; diff *= scaleFactor; diffuse = vec4(diff, 1); gl_Position = P*(MV*vec4(vVertex,1)); }
- The per-vertex color calculated by the vertex shader is interpolated by the rasterizer and then the fragment shader sets the color as the current fragment color.
#version 330 core uniform sampler2D textureMap; uniform float useDefault; smooth in vec4 diffuse; smooth in vec2 vUVout; layout(location=0) out vec4 vFragColor; void main() { vFragColor = mix(texture(textureMap, vUVout)*diffuse, diffuse, useDefault); }
Spherical harmonics is a technique that approximates the lighting, using coefficients and spherical harmonics basis. The coefficients are obtained at initialization from an HDR/RGBE image file that contains information about lighting. This allows us to approximate the same light so the graphical scene feels more immersive.
The demo application implementing this recipe renders the same scene as in the previous recipes, as shown in the following figure. We can rotate the camera view using the left mouse button, whereas, the point light source can be rotated using the right mouse button. Pressing the Space bar toggles the use of spherical harmonics. When spherical harmonics lighting is on, we get the following result:
Without the spherical harmonics lighting, the result is as follows:
The probe image used for this image is shown in the following figure:
- Ravi Ramamoorthi and Pat Hanrahan, An Efficient Representation for Irradiance Environment Maps: http://www1.cs.columbia.edu/~ravir/papers/envmap/index.html
- Randi J. Rost, Mill M. Licea-Kane, Dan Ginsburg, John M. Kessenich, Barthold Lichtenbelt, Hugh Malan, Mike Weiblen, OpenGL Shading Language, Third Edition, Section 12.3, Lighting and Spherical Harmonics, Addison-Wesley Professional
- Kelly Dempski and Emmanuel Viale, Advanced Lighting and Materials with Shaders, Chapter 8, Spherical Harmonic Lighting, Jones & Bartlett Publishers
- The RGBE image format specifications: http://www.graphics.cornell.edu/online/formats/rgbe/
- Paul Debevec HDR light probes: http://www.pauldebevec.com/Probes/
- Spherical harmonics lighting tutorial: http://www.paulsprojects.net/opengl/sh/sh.html
obj
mesh using the ObjLoader
class and fill the OpenGL buffer objects and the OpenGL textures, using the material information loaded from the file, as in the previous recipes.
#version 330 core layout(location = 0) in vec3 vVertex; layout(location = 1) in vec3 vNormal; layout(location = 2) in vec2 vUV; smooth out vec2 vUVout; smooth out vec4 diffuse; uniform mat4 P; uniform mat4 MV; uniform mat3 N; const float C1 = 0.429043; const float C2 = 0.511664; const float C3 = 0.743125; const float C4 = 0.886227; const float C5 = 0.247708; const float PI = 3.1415926535897932384626433832795; //Old town square probe const vec3 L00 = vec3( 0.871297, 0.875222, 0.864470); const vec3 L1m1 = vec3( 0.175058, 0.245335, 0.312891); const vec3 L10 = vec3( 0.034675, 0.036107, 0.037362); const vec3 L11 = vec3(-0.004629, -0.029448, -0.048028); const vec3 L2m2 = vec3(-0.120535, -0.121160, -0.117507); const vec3 L2m1 = vec3( 0.003242, 0.003624, 0.007511); const vec3 L20 = vec3(-0.028667, -0.024926, -0.020998); const vec3 L21 = vec3(-0.077539, -0.086325, -0.091591); const vec3 L22 = vec3(-0.161784, -0.191783, -0.219152); const vec3 scaleFactor = vec3(0.161784/ (0.871297+0.161784), 0.191783/(0.875222+0.191783), 0.219152/(0.864470+0.219152)); void main() { vUVout=vUV; vec3 tmpN = normalize(N*vNormal); vec3 diff = C1 * L22 * (tmpN.x*tmpN.x - tmpN.y*tmpN.y) + C3 * L20 * tmpN.z*tmpN.z + C4 * L00 - C5 * L20 + 2.0 * C1 * L2m2*tmpN.x*tmpN.y + 2.0 * C1 * L21*tmpN.x*tmpN.z + 2.0 * C1 * L2m1*tmpN.y*tmpN.z + 2.0 * C2 * L11*tmpN.x +2.0 * C2 * L1m1*tmpN.y +2.0 * C2 * L10*tmpN.z; diff *= scaleFactor; diffuse = vec4(diff, 1); gl_Position = P*(MV*vec4(vVertex,1)); }
#version 330 core uniform sampler2D textureMap; uniform float useDefault; smooth in vec4 diffuse; smooth in vec2 vUVout; layout(location=0) out vec4 vFragColor; void main() { vFragColor = mix(texture(textureMap, vUVout)*diffuse, diffuse, useDefault); }
Spherical harmonics is a technique that approximates the lighting, using coefficients and spherical harmonics basis. The coefficients are obtained at initialization from an HDR/RGBE image file that contains information about lighting. This allows us to approximate the same light so the graphical scene feels more immersive.
The demo application implementing this recipe renders the same scene as in the previous recipes, as shown in the following figure. We can rotate the camera view using the left mouse button, whereas, the point light source can be rotated using the right mouse button. Pressing the Space bar toggles the use of spherical harmonics. When spherical harmonics lighting is on, we get the following result:
Without the spherical harmonics lighting, the result is as follows:
The probe image used for this image is shown in the following figure:
- Ravi Ramamoorthi and Pat Hanrahan, An Efficient Representation for Irradiance Environment Maps: http://www1.cs.columbia.edu/~ravir/papers/envmap/index.html
- Randi J. Rost, Mill M. Licea-Kane, Dan Ginsburg, John M. Kessenich, Barthold Lichtenbelt, Hugh Malan, Mike Weiblen, OpenGL Shading Language, Third Edition, Section 12.3, Lighting and Spherical Harmonics, Addison-Wesley Professional
- Kelly Dempski and Emmanuel Viale, Advanced Lighting and Materials with Shaders, Chapter 8, Spherical Harmonic Lighting, Jones & Bartlett Publishers
- The RGBE image format specifications: http://www.graphics.cornell.edu/online/formats/rgbe/
- Paul Debevec HDR light probes: http://www.pauldebevec.com/Probes/
- Spherical harmonics lighting tutorial: http://www.paulsprojects.net/opengl/sh/sh.html
harmonics is a technique that approximates the lighting, using coefficients and spherical harmonics basis. The coefficients are obtained at initialization from an HDR/RGBE image file that contains information about lighting. This allows us to approximate the same light so the graphical scene feels more immersive.
The demo application implementing this recipe renders the same scene as in the previous recipes, as shown in the following figure. We can rotate the camera view using the left mouse button, whereas, the point light source can be rotated using the right mouse button. Pressing the Space bar toggles the use of spherical harmonics. When spherical harmonics lighting is on, we get the following result:
Without the spherical harmonics lighting, the result is as follows:
The probe image used for this image is shown in the following figure:
- Ravi Ramamoorthi and Pat Hanrahan, An Efficient Representation for Irradiance Environment Maps: http://www1.cs.columbia.edu/~ravir/papers/envmap/index.html
- Randi J. Rost, Mill M. Licea-Kane, Dan Ginsburg, John M. Kessenich, Barthold Lichtenbelt, Hugh Malan, Mike Weiblen, OpenGL Shading Language, Third Edition, Section 12.3, Lighting and Spherical Harmonics, Addison-Wesley Professional
- Kelly Dempski and Emmanuel Viale, Advanced Lighting and Materials with Shaders, Chapter 8, Spherical Harmonic Lighting, Jones & Bartlett Publishers
- The RGBE image format specifications: http://www.graphics.cornell.edu/online/formats/rgbe/
- Paul Debevec HDR light probes: http://www.pauldebevec.com/Probes/
- Spherical harmonics lighting tutorial: http://www.paulsprojects.net/opengl/sh/sh.html
application implementing this recipe renders the same scene as in the previous recipes, as shown in the following figure. We can rotate the camera view using the left mouse button, whereas, the point light source can be rotated using the right mouse button. Pressing the Space bar toggles the use of spherical harmonics. When spherical harmonics lighting is on, we get the following result:
Without the spherical harmonics lighting, the result is as follows:
The probe image used for this image is shown in the following figure:
- Ravi Ramamoorthi and Pat Hanrahan, An Efficient Representation for Irradiance Environment Maps: http://www1.cs.columbia.edu/~ravir/papers/envmap/index.html
- Randi J. Rost, Mill M. Licea-Kane, Dan Ginsburg, John M. Kessenich, Barthold Lichtenbelt, Hugh Malan, Mike Weiblen, OpenGL Shading Language, Third Edition, Section 12.3, Lighting and Spherical Harmonics, Addison-Wesley Professional
- Kelly Dempski and Emmanuel Viale, Advanced Lighting and Materials with Shaders, Chapter 8, Spherical Harmonic Lighting, Jones & Bartlett Publishers
- The RGBE image format specifications: http://www.graphics.cornell.edu/online/formats/rgbe/
- Paul Debevec HDR light probes: http://www.pauldebevec.com/Probes/
- Spherical harmonics lighting tutorial: http://www.paulsprojects.net/opengl/sh/sh.html
- http://www1.cs.columbia.edu/~ravir/papers/envmap/index.html
- Randi J. Rost, Mill M. Licea-Kane, Dan Ginsburg, John M. Kessenich, Barthold Lichtenbelt, Hugh Malan, Mike Weiblen, OpenGL Shading Language, Third Edition, Section 12.3, Lighting and Spherical Harmonics, Addison-Wesley Professional
- Kelly Dempski and Emmanuel Viale, Advanced Lighting and Materials with Shaders, Chapter 8, Spherical Harmonic Lighting, Jones & Bartlett Publishers
- The RGBE image format specifications: http://www.graphics.cornell.edu/online/formats/rgbe/
- Paul Debevec HDR light probes: http://www.pauldebevec.com/Probes/
- Spherical harmonics lighting tutorial: http://www.paulsprojects.net/opengl/sh/sh.html
To this point, all of the recipes rendered 3D geometry using rasterization. In this recipe, we will implement another method for rendering geometry, which is called ray tracing. Simply put, ray tracing uses a probing ray from the camera position into the graphical scene. The intersections of this ray are obtained for each geometry. The good thing with this method is that only the visible objects are rendered.
The ray tracing algorithm can be given in pseudocode as follows:
Let us start with this recipe by following these simple steps:
- Load the Obj mesh model using the Obj loader and store mesh geometry in vectors. Note that for the GPU ray tracer we use the original vertices and indices lists stored in the OBJ file.
vector<unsigned short> indices2; vector<glm::vec3> vertices2; if(!obj.Load(mesh_filename.c_str(), meshes, vertices, indices, materials, aabb, vertices2, indices2)) { cout<<"Cannot load the 3ds mesh"<<endl; exit(EXIT_FAILURE); }
- Load the material texture maps into an OpenGL texture array instead of loading each texture separately, as in previous recipes. We opted for texture arrays because this helps in simplifying the shader code and we would have no way in determining the total samplers we would require, as that is dependent on the material textures we have in the model. In previous recipes, there was a single texture sampler which was modified for each sub-mesh.
for(size_t k=0;k<materials.size();k++) { if(materials[k]->map_Kd != "") { if(k==0) { glGenTextures(1, &textureID); glBindTexture(GL_TEXTURE_2D_ARRAY, textureID); glTexParameteri(GL_TEXTURE_2D_ARRAY, GL_TEXTURE_MIN_FILTER, GL_LINEAR); //set other texture parameters } //set image name GLubyte* pData = SOIL_load_image(full_filename.c_str(), &texture_width, &texture_height, &channels, SOIL_LOAD_AUTO); if(pData == NULL) { cerr<<"Cannot load image: "<<full_filename.c_str()<<endl; exit(EXIT_FAILURE); } //flip the image and set the image format if(k==0) { glTexImage3D(GL_TEXTURE_2D_ARRAY, 0, format, texture_width, texture_height, total, 0, format, GL_UNSIGNED_BYTE, NULL); } glTexSubImage3D(GL_TEXTURE_2D_ARRAY, 0,0,0,k, texture_width, texture_height, 1, format, GL_UNSIGNED_BYTE, pData); SOIL_free_image_data(pData); } }
- Store the vertex positions into a texture for the ray tracing shader. We use a floating point texture with the
GL_RGBA32F
internal format.glGenTextures(1, &texVerticesID); glActiveTexture(GL_TEXTURE1); glBindTexture( GL_TEXTURE_2D, texVerticesID); //set the texture formats GLfloat* pData = new GLfloat[vertices2.size()*4]; int count = 0; for(size_t i=0;i<vertices2.size();i++) { pData[count++] = vertices2[i].x; pData[count++] = vertices2[i].y; pData[count++] = vertices2[i].z; pData[count++] = 0; } glTexImage2D(GL_TEXTURE_2D, 0, GL_RGBA32F, vertices2.size(),1, 0, GL_RGBA, GL_FLOAT, pData); delete [] pData;
- Store the list of indices into an integral texture for the ray tracing shader. Note that for this texture, the internal format is
GL_RGBA16I
and the format isGL_RGBA_INTEGER
.glGenTextures(1, &texTrianglesID); glActiveTexture(GL_TEXTURE2); glBindTexture( GL_TEXTURE_2D, texTrianglesID); //set the texture formats GLushort* pData2 = new GLushort[indices2.size()]; count = 0; for(size_t i=0;i<indices2.size();i+=4) { pData2[count++] = (indices2[i]); pData2[count++] = (indices2[i+1]); pData2[count++] = (indices2[i+2]); pData2[count++] = (indices2[i+3]); } glTexImage2D(GL_TEXTURE_2D, 0, GL_RGBA16I, indices2.size()/4, 1, 0, GL_RGBA_INTEGER, GL_UNSIGNED_SHORT, pData2); delete [] pData2;
- In the
render
function, bind the ray tracing shader and then draw a full-screen quad to invoke the fragment shader for the entire screen.
The main code for ray tracing is the ray tracing fragment shader (Chapter6/GPURaytracing/shaders/raytracer.frag
). We first set up the camera ray origin and direction using the parameters passed to the shader as shader uniforms.
With ray tracing, shadows are very easy to calculate. We simply cast another ray, but this time, just look at if the ray intersects any object on its way to the light source. If it does, we darken the final color, otherwise we leave the color as is. Note that to prevent the shadow acne, we add a slight offset to the ray start position.
- Timothy Purcell, Ian Buck, William R. Mark, and Pat Hanrahan, ACM Transactions on Graphics 21 (3), Ray Tracing on Programmable Graphics Hardware, pages 703-712: http://graphics.stanford.edu/papers/rtongfx/
- Real-time GPU Ray-Tracer at Icare3D: http://www.icare3d.org/codes-and-projects/codes/raytracer_gpu_full_1-0.html
Chapter6/GPURaytracing
directory.
Let us start with this recipe by following these simple steps:
- Load the Obj mesh model using the Obj loader and store mesh geometry in vectors. Note that for the GPU ray tracer we use the original vertices and indices lists stored in the OBJ file.
vector<unsigned short> indices2; vector<glm::vec3> vertices2; if(!obj.Load(mesh_filename.c_str(), meshes, vertices, indices, materials, aabb, vertices2, indices2)) { cout<<"Cannot load the 3ds mesh"<<endl; exit(EXIT_FAILURE); }
- Load the material texture maps into an OpenGL texture array instead of loading each texture separately, as in previous recipes. We opted for texture arrays because this helps in simplifying the shader code and we would have no way in determining the total samplers we would require, as that is dependent on the material textures we have in the model. In previous recipes, there was a single texture sampler which was modified for each sub-mesh.
for(size_t k=0;k<materials.size();k++) { if(materials[k]->map_Kd != "") { if(k==0) { glGenTextures(1, &textureID); glBindTexture(GL_TEXTURE_2D_ARRAY, textureID); glTexParameteri(GL_TEXTURE_2D_ARRAY, GL_TEXTURE_MIN_FILTER, GL_LINEAR); //set other texture parameters } //set image name GLubyte* pData = SOIL_load_image(full_filename.c_str(), &texture_width, &texture_height, &channels, SOIL_LOAD_AUTO); if(pData == NULL) { cerr<<"Cannot load image: "<<full_filename.c_str()<<endl; exit(EXIT_FAILURE); } //flip the image and set the image format if(k==0) { glTexImage3D(GL_TEXTURE_2D_ARRAY, 0, format, texture_width, texture_height, total, 0, format, GL_UNSIGNED_BYTE, NULL); } glTexSubImage3D(GL_TEXTURE_2D_ARRAY, 0,0,0,k, texture_width, texture_height, 1, format, GL_UNSIGNED_BYTE, pData); SOIL_free_image_data(pData); } }
- Store the vertex positions into a texture for the ray tracing shader. We use a floating point texture with the
GL_RGBA32F
internal format.glGenTextures(1, &texVerticesID); glActiveTexture(GL_TEXTURE1); glBindTexture( GL_TEXTURE_2D, texVerticesID); //set the texture formats GLfloat* pData = new GLfloat[vertices2.size()*4]; int count = 0; for(size_t i=0;i<vertices2.size();i++) { pData[count++] = vertices2[i].x; pData[count++] = vertices2[i].y; pData[count++] = vertices2[i].z; pData[count++] = 0; } glTexImage2D(GL_TEXTURE_2D, 0, GL_RGBA32F, vertices2.size(),1, 0, GL_RGBA, GL_FLOAT, pData); delete [] pData;
- Store the list of indices into an integral texture for the ray tracing shader. Note that for this texture, the internal format is
GL_RGBA16I
and the format isGL_RGBA_INTEGER
.glGenTextures(1, &texTrianglesID); glActiveTexture(GL_TEXTURE2); glBindTexture( GL_TEXTURE_2D, texTrianglesID); //set the texture formats GLushort* pData2 = new GLushort[indices2.size()]; count = 0; for(size_t i=0;i<indices2.size();i+=4) { pData2[count++] = (indices2[i]); pData2[count++] = (indices2[i+1]); pData2[count++] = (indices2[i+2]); pData2[count++] = (indices2[i+3]); } glTexImage2D(GL_TEXTURE_2D, 0, GL_RGBA16I, indices2.size()/4, 1, 0, GL_RGBA_INTEGER, GL_UNSIGNED_SHORT, pData2); delete [] pData2;
- In the
render
function, bind the ray tracing shader and then draw a full-screen quad to invoke the fragment shader for the entire screen.
The main code for ray tracing is the ray tracing fragment shader (Chapter6/GPURaytracing/shaders/raytracer.frag
). We first set up the camera ray origin and direction using the parameters passed to the shader as shader uniforms.
With ray tracing, shadows are very easy to calculate. We simply cast another ray, but this time, just look at if the ray intersects any object on its way to the light source. If it does, we darken the final color, otherwise we leave the color as is. Note that to prevent the shadow acne, we add a slight offset to the ray start position.
- Timothy Purcell, Ian Buck, William R. Mark, and Pat Hanrahan, ACM Transactions on Graphics 21 (3), Ray Tracing on Programmable Graphics Hardware, pages 703-712: http://graphics.stanford.edu/papers/rtongfx/
- Real-time GPU Ray-Tracer at Icare3D: http://www.icare3d.org/codes-and-projects/codes/raytracer_gpu_full_1-0.html
recipe by following these simple steps:
- Load the Obj mesh model using the Obj loader and store mesh geometry in vectors. Note that for the GPU ray tracer we use the original vertices and indices lists stored in the OBJ file.
vector<unsigned short> indices2; vector<glm::vec3> vertices2; if(!obj.Load(mesh_filename.c_str(), meshes, vertices, indices, materials, aabb, vertices2, indices2)) { cout<<"Cannot load the 3ds mesh"<<endl; exit(EXIT_FAILURE); }
- Load the material texture maps into an OpenGL texture array instead of loading each texture separately, as in previous recipes. We opted for texture arrays because this helps in simplifying the shader code and we would have no way in determining the total samplers we would require, as that is dependent on the material textures we have in the model. In previous recipes, there was a single texture sampler which was modified for each sub-mesh.
for(size_t k=0;k<materials.size();k++) { if(materials[k]->map_Kd != "") { if(k==0) { glGenTextures(1, &textureID); glBindTexture(GL_TEXTURE_2D_ARRAY, textureID); glTexParameteri(GL_TEXTURE_2D_ARRAY, GL_TEXTURE_MIN_FILTER, GL_LINEAR); //set other texture parameters } //set image name GLubyte* pData = SOIL_load_image(full_filename.c_str(), &texture_width, &texture_height, &channels, SOIL_LOAD_AUTO); if(pData == NULL) { cerr<<"Cannot load image: "<<full_filename.c_str()<<endl; exit(EXIT_FAILURE); } //flip the image and set the image format if(k==0) { glTexImage3D(GL_TEXTURE_2D_ARRAY, 0, format, texture_width, texture_height, total, 0, format, GL_UNSIGNED_BYTE, NULL); } glTexSubImage3D(GL_TEXTURE_2D_ARRAY, 0,0,0,k, texture_width, texture_height, 1, format, GL_UNSIGNED_BYTE, pData); SOIL_free_image_data(pData); } }
- Store the vertex positions into a texture for the ray tracing shader. We use a floating point texture with the
GL_RGBA32F
internal format.glGenTextures(1, &texVerticesID); glActiveTexture(GL_TEXTURE1); glBindTexture( GL_TEXTURE_2D, texVerticesID); //set the texture formats GLfloat* pData = new GLfloat[vertices2.size()*4]; int count = 0; for(size_t i=0;i<vertices2.size();i++) { pData[count++] = vertices2[i].x; pData[count++] = vertices2[i].y; pData[count++] = vertices2[i].z; pData[count++] = 0; } glTexImage2D(GL_TEXTURE_2D, 0, GL_RGBA32F, vertices2.size(),1, 0, GL_RGBA, GL_FLOAT, pData); delete [] pData;
- Store the list of indices into an integral texture for the ray tracing shader. Note that for this texture, the internal format is
GL_RGBA16I
and the format isGL_RGBA_INTEGER
.glGenTextures(1, &texTrianglesID); glActiveTexture(GL_TEXTURE2); glBindTexture( GL_TEXTURE_2D, texTrianglesID); //set the texture formats GLushort* pData2 = new GLushort[indices2.size()]; count = 0; for(size_t i=0;i<indices2.size();i+=4) { pData2[count++] = (indices2[i]); pData2[count++] = (indices2[i+1]); pData2[count++] = (indices2[i+2]); pData2[count++] = (indices2[i+3]); } glTexImage2D(GL_TEXTURE_2D, 0, GL_RGBA16I, indices2.size()/4, 1, 0, GL_RGBA_INTEGER, GL_UNSIGNED_SHORT, pData2); delete [] pData2;
- In the
render
function, bind the ray tracing shader and then draw a full-screen quad to invoke the fragment shader for the entire screen.
The main code for ray tracing is the ray tracing fragment shader (Chapter6/GPURaytracing/shaders/raytracer.frag
). We first set up the camera ray origin and direction using the parameters passed to the shader as shader uniforms.
With ray tracing, shadows are very easy to calculate. We simply cast another ray, but this time, just look at if the ray intersects any object on its way to the light source. If it does, we darken the final color, otherwise we leave the color as is. Note that to prevent the shadow acne, we add a slight offset to the ray start position.
- Timothy Purcell, Ian Buck, William R. Mark, and Pat Hanrahan, ACM Transactions on Graphics 21 (3), Ray Tracing on Programmable Graphics Hardware, pages 703-712: http://graphics.stanford.edu/papers/rtongfx/
- Real-time GPU Ray-Tracer at Icare3D: http://www.icare3d.org/codes-and-projects/codes/raytracer_gpu_full_1-0.html
With ray tracing, shadows are very easy to calculate. We simply cast another ray, but this time, just look at if the ray intersects any object on its way to the light source. If it does, we darken the final color, otherwise we leave the color as is. Note that to prevent the shadow acne, we add a slight offset to the ray start position.
- Timothy Purcell, Ian Buck, William R. Mark, and Pat Hanrahan, ACM Transactions on Graphics 21 (3), Ray Tracing on Programmable Graphics Hardware, pages 703-712: http://graphics.stanford.edu/papers/rtongfx/
- Real-time GPU Ray-Tracer at Icare3D: http://www.icare3d.org/codes-and-projects/codes/raytracer_gpu_full_1-0.html
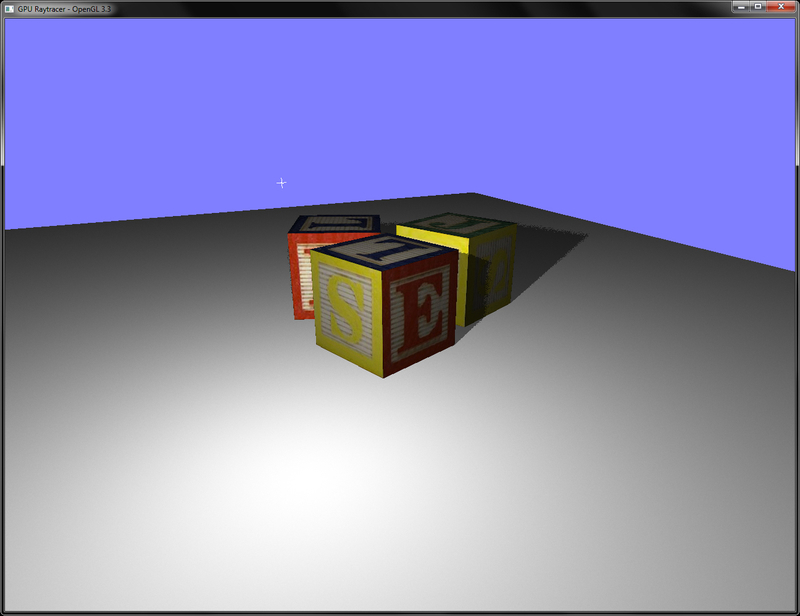
- Timothy Purcell, Ian Buck, William R. Mark, and Pat Hanrahan, ACM Transactions on Graphics 21 (3), Ray Tracing on Programmable Graphics Hardware, pages 703-712: http://graphics.stanford.edu/papers/rtongfx/
- Real-time GPU Ray-Tracer at Icare3D: http://www.icare3d.org/codes-and-projects/codes/raytracer_gpu_full_1-0.html
- http://graphics.stanford.edu/papers/rtongfx/
- Real-time GPU Ray-Tracer at Icare3D: http://www.icare3d.org/codes-and-projects/codes/raytracer_gpu_full_1-0.html
In this recipe, we will implement another method, called path tracing, for rendering geometry. Similar to ray tracing, path tracing casts rays but these rays are shot randomly from the light position(s). Since it is usually difficult to approximate real lighting, we can approximate it using Monte Carlo-based integration schemes. These methods use random sampling and if there are enough samples, the integration result converges to the true solution.
We can give the path tracing pseudocode as follows:
Let us start with this recipe by following these simple steps:
- Load the Obj mesh model using the Obj loader and store the mesh geometry in vectors. Note that for the GPU path tracer we use the original vertices and indices lists stored in the OBJ file, as in the previous recipe.
- Load the material texture maps into an OpenGL texture array instead of loading each texture separately as in the previous recipe.
- Store the vertex positions into a texture for the path tracing shader, similar to how we stored them for ray tracing in the previous recipe. We use a floating point texture with the
GL_RGBA32F
internal format.glGenTextures(1, &texVerticesID); glActiveTexture(GL_TEXTURE1); glBindTexture( GL_TEXTURE_2D, texVerticesID); //set the texture formats GLfloat* pData = new GLfloat[vertices2.size()*4]; int count = 0; for(size_t i=0;i<vertices2.size();i++) { pData[count++] = vertices2[i].x; pData[count++] = vertices2[i].y; pData[count++] = vertices2[i].z; pData[count++] = 0; } glTexImage2D(GL_TEXTURE_2D, 0, GL_RGBA32F, vertices2.size(),1, 0, GL_RGBA, GL_FLOAT, pData); delete [] pData;
- Store the indices list into an integral texture for the path tracing shader, as was done for the ray tracing recipe. Note that for this texture, the internal format is
GL_RGBA16I
and format isGL_RGBA_INTEGER
.glGenTextures(1, &texTrianglesID); glActiveTexture(GL_TEXTURE2); glBindTexture( GL_TEXTURE_2D, texTrianglesID); //set the texture formats GLushort* pData2 = new GLushort[indices2.size()]; count = 0; for(size_t i=0;i<indices2.size();i+=4) { pData2[count++] = (indices2[i]); pData2[count++] = (indices2[i+1]); pData2[count++] = (indices2[i+2]); pData2[count++] = (indices2[i+3]); } glTexImage2D(GL_TEXTURE_2D, 0, GL_RGBA16I, indices2.size()/4, 1, 0, GL_RGBA_INTEGER, GL_UNSIGNED_SHORT, pData2); delete [] pData2;
- In the render function, bind the path tracing shader and then draw a full-screen quad to invoke the fragment shader for the entire screen.
pathtraceShader.Use(); glUniform3fv(pathtraceShader("eyePos"), 1, glm::value_ptr(eyePos)); glUniform1f(pathtraceShader("time"), current); glUniform3fv(pathtraceShader("light_position"),1, &(lightPosOS.x)); glUniformMatrix4fv(pathtraceShader("invMVP"), 1, GL_FALSE, glm::value_ptr(invMVP)); DrawFullScreenQuad(); pathtraceShader.UnUse();
The main code for path tracing is carried out in the path tracing fragment shader (Chapter6/GPUPathtracing/shaders/pathtracer.frag)
. We first set up the camera ray origin and direction using the parameters passed to the shader as shader uniforms.
In the path
trace
function, we run a loop that iterates for a number of passes. In each pass, we check the scene geometry for an intersection with the ray. We use a brute force method of looping through all of the triangles and testing each of them in turn for collision. If we have an intersection, we check to see if this is the nearest intersection. If it is, we store the normal and the texture coordinates at the intersection point.
Note that the path tracing output is noisy and a large number of samples are needed to get a less noisy result.
Ray tracing is poor at approximating global illumination and soft shadows. Path tracing, on the other hand, handles global illumination and soft shadows well, but it suffers from noise. To get a good result, it requires a large number of random sampling points. There are other techniques, such as Metropolis light transport, which uses heuristics to only accept good sample points and reject bad sampling points. As a result, it converges to a less noisier result faster as compared to naïve path tracing.
- Tim Purcell, Ian Buck, William Mark, and Pat Hanrahan, Ray Tracing on Programmable Graphics Hardware, ACM Transactions on Graphics 21(3), pp: 703-712, 2002. Available online: http://graphics.stanford.edu/papers/rtongfx/
- Peter and Karl's GPU Path Tracer: http://gpupathtracer.blogspot.sg/
- Real-time path traced Brigade demo at Siggraph 2012: http://raytracey.blogspot.co.nz/2012/08/real-time-path-traced-brigade-demo-at.html
Chapter6/GPUPathtracing
directory.
Let us start with this recipe by following these simple steps:
- Load the Obj mesh model using the Obj loader and store the mesh geometry in vectors. Note that for the GPU path tracer we use the original vertices and indices lists stored in the OBJ file, as in the previous recipe.
- Load the material texture maps into an OpenGL texture array instead of loading each texture separately as in the previous recipe.
- Store the vertex positions into a texture for the path tracing shader, similar to how we stored them for ray tracing in the previous recipe. We use a floating point texture with the
GL_RGBA32F
internal format.glGenTextures(1, &texVerticesID); glActiveTexture(GL_TEXTURE1); glBindTexture( GL_TEXTURE_2D, texVerticesID); //set the texture formats GLfloat* pData = new GLfloat[vertices2.size()*4]; int count = 0; for(size_t i=0;i<vertices2.size();i++) { pData[count++] = vertices2[i].x; pData[count++] = vertices2[i].y; pData[count++] = vertices2[i].z; pData[count++] = 0; } glTexImage2D(GL_TEXTURE_2D, 0, GL_RGBA32F, vertices2.size(),1, 0, GL_RGBA, GL_FLOAT, pData); delete [] pData;
- Store the indices list into an integral texture for the path tracing shader, as was done for the ray tracing recipe. Note that for this texture, the internal format is
GL_RGBA16I
and format isGL_RGBA_INTEGER
.glGenTextures(1, &texTrianglesID); glActiveTexture(GL_TEXTURE2); glBindTexture( GL_TEXTURE_2D, texTrianglesID); //set the texture formats GLushort* pData2 = new GLushort[indices2.size()]; count = 0; for(size_t i=0;i<indices2.size();i+=4) { pData2[count++] = (indices2[i]); pData2[count++] = (indices2[i+1]); pData2[count++] = (indices2[i+2]); pData2[count++] = (indices2[i+3]); } glTexImage2D(GL_TEXTURE_2D, 0, GL_RGBA16I, indices2.size()/4, 1, 0, GL_RGBA_INTEGER, GL_UNSIGNED_SHORT, pData2); delete [] pData2;
- In the render function, bind the path tracing shader and then draw a full-screen quad to invoke the fragment shader for the entire screen.
pathtraceShader.Use(); glUniform3fv(pathtraceShader("eyePos"), 1, glm::value_ptr(eyePos)); glUniform1f(pathtraceShader("time"), current); glUniform3fv(pathtraceShader("light_position"),1, &(lightPosOS.x)); glUniformMatrix4fv(pathtraceShader("invMVP"), 1, GL_FALSE, glm::value_ptr(invMVP)); DrawFullScreenQuad(); pathtraceShader.UnUse();
The main code for path tracing is carried out in the path tracing fragment shader (Chapter6/GPUPathtracing/shaders/pathtracer.frag)
. We first set up the camera ray origin and direction using the parameters passed to the shader as shader uniforms.
In the path
trace
function, we run a loop that iterates for a number of passes. In each pass, we check the scene geometry for an intersection with the ray. We use a brute force method of looping through all of the triangles and testing each of them in turn for collision. If we have an intersection, we check to see if this is the nearest intersection. If it is, we store the normal and the texture coordinates at the intersection point.
Note that the path tracing output is noisy and a large number of samples are needed to get a less noisy result.
Ray tracing is poor at approximating global illumination and soft shadows. Path tracing, on the other hand, handles global illumination and soft shadows well, but it suffers from noise. To get a good result, it requires a large number of random sampling points. There are other techniques, such as Metropolis light transport, which uses heuristics to only accept good sample points and reject bad sampling points. As a result, it converges to a less noisier result faster as compared to naïve path tracing.
- Tim Purcell, Ian Buck, William Mark, and Pat Hanrahan, Ray Tracing on Programmable Graphics Hardware, ACM Transactions on Graphics 21(3), pp: 703-712, 2002. Available online: http://graphics.stanford.edu/papers/rtongfx/
- Peter and Karl's GPU Path Tracer: http://gpupathtracer.blogspot.sg/
- Real-time path traced Brigade demo at Siggraph 2012: http://raytracey.blogspot.co.nz/2012/08/real-time-path-traced-brigade-demo-at.html
this recipe by following these simple steps:
- Load the Obj mesh model using the Obj loader and store the mesh geometry in vectors. Note that for the GPU path tracer we use the original vertices and indices lists stored in the OBJ file, as in the previous recipe.
- Load the material texture maps into an OpenGL texture array instead of loading each texture separately as in the previous recipe.
- Store the vertex positions into a texture for the path tracing shader, similar to how we stored them for ray tracing in the previous recipe. We use a floating point texture with the
GL_RGBA32F
internal format.glGenTextures(1, &texVerticesID); glActiveTexture(GL_TEXTURE1); glBindTexture( GL_TEXTURE_2D, texVerticesID); //set the texture formats GLfloat* pData = new GLfloat[vertices2.size()*4]; int count = 0; for(size_t i=0;i<vertices2.size();i++) { pData[count++] = vertices2[i].x; pData[count++] = vertices2[i].y; pData[count++] = vertices2[i].z; pData[count++] = 0; } glTexImage2D(GL_TEXTURE_2D, 0, GL_RGBA32F, vertices2.size(),1, 0, GL_RGBA, GL_FLOAT, pData); delete [] pData;
- Store the indices list into an integral texture for the path tracing shader, as was done for the ray tracing recipe. Note that for this texture, the internal format is
GL_RGBA16I
and format isGL_RGBA_INTEGER
.glGenTextures(1, &texTrianglesID); glActiveTexture(GL_TEXTURE2); glBindTexture( GL_TEXTURE_2D, texTrianglesID); //set the texture formats GLushort* pData2 = new GLushort[indices2.size()]; count = 0; for(size_t i=0;i<indices2.size();i+=4) { pData2[count++] = (indices2[i]); pData2[count++] = (indices2[i+1]); pData2[count++] = (indices2[i+2]); pData2[count++] = (indices2[i+3]); } glTexImage2D(GL_TEXTURE_2D, 0, GL_RGBA16I, indices2.size()/4, 1, 0, GL_RGBA_INTEGER, GL_UNSIGNED_SHORT, pData2); delete [] pData2;
- In the render function, bind the path tracing shader and then draw a full-screen quad to invoke the fragment shader for the entire screen.
pathtraceShader.Use(); glUniform3fv(pathtraceShader("eyePos"), 1, glm::value_ptr(eyePos)); glUniform1f(pathtraceShader("time"), current); glUniform3fv(pathtraceShader("light_position"),1, &(lightPosOS.x)); glUniformMatrix4fv(pathtraceShader("invMVP"), 1, GL_FALSE, glm::value_ptr(invMVP)); DrawFullScreenQuad(); pathtraceShader.UnUse();
The main code for path tracing is carried out in the path tracing fragment shader (Chapter6/GPUPathtracing/shaders/pathtracer.frag)
. We first set up the camera ray origin and direction using the parameters passed to the shader as shader uniforms.
In the path
trace
function, we run a loop that iterates for a number of passes. In each pass, we check the scene geometry for an intersection with the ray. We use a brute force method of looping through all of the triangles and testing each of them in turn for collision. If we have an intersection, we check to see if this is the nearest intersection. If it is, we store the normal and the texture coordinates at the intersection point.
Note that the path tracing output is noisy and a large number of samples are needed to get a less noisy result.
Ray tracing is poor at approximating global illumination and soft shadows. Path tracing, on the other hand, handles global illumination and soft shadows well, but it suffers from noise. To get a good result, it requires a large number of random sampling points. There are other techniques, such as Metropolis light transport, which uses heuristics to only accept good sample points and reject bad sampling points. As a result, it converges to a less noisier result faster as compared to naïve path tracing.
- Tim Purcell, Ian Buck, William Mark, and Pat Hanrahan, Ray Tracing on Programmable Graphics Hardware, ACM Transactions on Graphics 21(3), pp: 703-712, 2002. Available online: http://graphics.stanford.edu/papers/rtongfx/
- Peter and Karl's GPU Path Tracer: http://gpupathtracer.blogspot.sg/
- Real-time path traced Brigade demo at Siggraph 2012: http://raytracey.blogspot.co.nz/2012/08/real-time-path-traced-brigade-demo-at.html
In the path
trace
function, we run a loop that iterates for a number of passes. In each pass, we check the scene geometry for an intersection with the ray. We use a brute force method of looping through all of the triangles and testing each of them in turn for collision. If we have an intersection, we check to see if this is the nearest intersection. If it is, we store the normal and the texture coordinates at the intersection point.
Note that the path tracing output is noisy and a large number of samples are needed to get a less noisy result.
Ray tracing is poor at approximating global illumination and soft shadows. Path tracing, on the other hand, handles global illumination and soft shadows well, but it suffers from noise. To get a good result, it requires a large number of random sampling points. There are other techniques, such as Metropolis light transport, which uses heuristics to only accept good sample points and reject bad sampling points. As a result, it converges to a less noisier result faster as compared to naïve path tracing.
- Tim Purcell, Ian Buck, William Mark, and Pat Hanrahan, Ray Tracing on Programmable Graphics Hardware, ACM Transactions on Graphics 21(3), pp: 703-712, 2002. Available online: http://graphics.stanford.edu/papers/rtongfx/
- Peter and Karl's GPU Path Tracer: http://gpupathtracer.blogspot.sg/
- Real-time path traced Brigade demo at Siggraph 2012: http://raytracey.blogspot.co.nz/2012/08/real-time-path-traced-brigade-demo-at.html
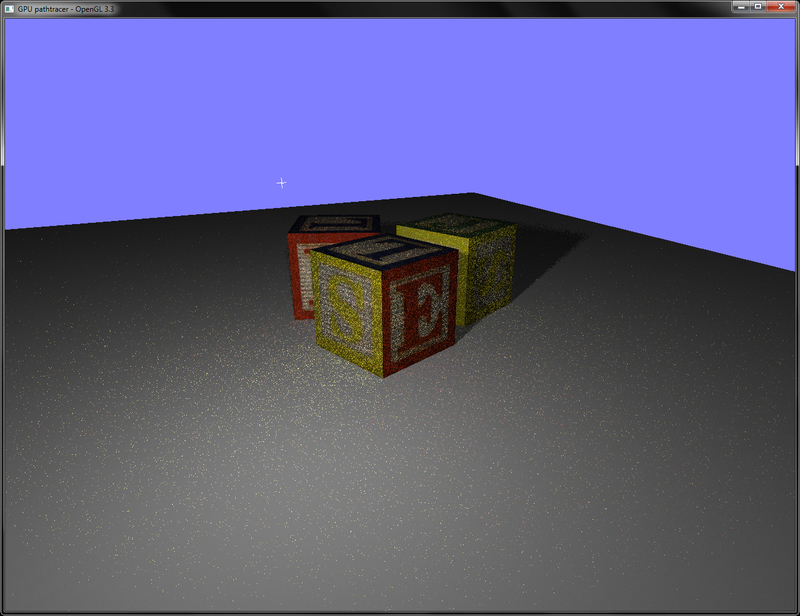
- Tim Purcell, Ian Buck, William Mark, and Pat Hanrahan, Ray Tracing on Programmable Graphics Hardware, ACM Transactions on Graphics 21(3), pp: 703-712, 2002. Available online: http://graphics.stanford.edu/papers/rtongfx/
- Peter and Karl's GPU Path Tracer: http://gpupathtracer.blogspot.sg/
- Real-time path traced Brigade demo at Siggraph 2012: http://raytracey.blogspot.co.nz/2012/08/real-time-path-traced-brigade-demo-at.html
- http://graphics.stanford.edu/papers/rtongfx/
- Peter and Karl's GPU Path Tracer: http://gpupathtracer.blogspot.sg/
- Real-time path traced Brigade demo at Siggraph 2012: http://raytracey.blogspot.co.nz/2012/08/real-time-path-traced-brigade-demo-at.html
In this chapter, we will focus on:
- Implementing volume rendering using 3D texture slicing
- Implementing volume rendering using single-pass GPU ray casting
- Implementing pseudo isosurface rendering in single-pass GPU ray casting
- Implementing volume rendering using splatting
- Implementing the transfer function for volume classification
- Implementing polygonal isosurface extraction using the Marching Tetrahedra algorithm
- Implementing volumetric lighting using half-angle slicing
Volume rendering techniques are used in various domains in biomedical and engineering disciplines. They are often used in biomedical imaging to visualize the CT/MRI datasets. In mechanical engineering, they are used to visualize intermediate results from FEM simulations, flow, and structural analysis. With the advent of GPU, all of the existing models and methods of visualization were ported to GPU to harness their computational power. This chapter will detail several algorithms that are used for volume visualization on the GPU in OpenGL Version 3.3 and above. Specifically, we will look at three widely used methods including 3D texture slicing, single-pass ray casting with alpha compositing as well as isosurface rendering, and splatting.
Volume rendering is a special class of rendering algorithms that allows us to portray fuzzy phenomena, such as smoke. There are numerous algorithms for volume rendering. To start our quest, we will focus on the simplest method called 3D texture slicing. This method approximates the volume-density function by slicing the dataset in front-to-back or back-to-front order and then blends the proxy slices using hardware-supported blending. Since it relies on the rasterization hardware, this method is very fast on the modern GPU.
The pseudo code for view-aligned 3D texture slicing is as follows:
- Get the current view direction vector.
- Calculate the min/max distance of unit cube vertices by doing a dot product of each unit cube vertex with the view direction vector.
- Calculate all possible intersections parameter (λ) of the plane perpendicular to the view direction with all edges of the unit cube going from the nearest to farthest vertex, using min/max distances from step 1.
- Use the intersection parameter λ (from step 3) to move in the viewing direction and find the intersection points. Three to six intersection vertices will be generated.
- Store the intersection points in the specified order to generate triangular primitives, which are the proxy geometries.
- Update the buffer object memory with the new vertices.
Let us start our recipe by following these simple steps:
- Load the volume dataset by reading the external volume datafile and passing the data into an OpenGL texture. Also enable hardware mipmap generation. Typically, the volume datafiles store densities that are obtained from using a cross-sectional imaging modality such as CT or MRI scans. Each CT/MRI scan is a 2D slice. We accumulate these slices in Z direction to obtain a 3D texture, which is simply an array of 2D textures. The densities store different material types, for example, values ranging from 0 to 20 are typically occupied by air. As we have an 8-bit unsigned dataset, we store the dataset into a local array of
GLubyte
type. If we had an unsigned 16-bit dataset, we would have stored it into a local array ofGLushort
type. In case of 3D textures, in addition to theS
andT
parameters, we have an additional parameterR
that controls the slice we are at in the 3D texture.std::ifstream infile(volume_file.c_str(), std::ios_base::binary); if(infile.good()) { GLubyte* pData = new GLubyte[XDIM*YDIM*ZDIM]; infile.read(reinterpret_cast<char*>(pData), XDIM*YDIM*ZDIM*sizeof(GLubyte)); infile.close(); glGenTextures(1, &textureID); glBindTexture(GL_TEXTURE_3D, textureID); glTexParameteri(GL_TEXTURE_3D, GL_TEXTURE_WRAP_S, GL_CLAMP); glTexParameteri(GL_TEXTURE_3D, GL_TEXTURE_WRAP_T, GL_CLAMP); glTexParameteri(GL_TEXTURE_3D, GL_TEXTURE_WRAP_R, GL_CLAMP); glTexParameteri(GL_TEXTURE_3D, GL_TEXTURE_MAG_FILTER, GL_LINEAR); glTexParameteri(GL_TEXTURE_3D, GL_TEXTURE_MIN_FILTER, GL_LINEAR_MIPMAP_LINEAR); glTexParameteri(GL_TEXTURE_3D, GL_TEXTURE_BASE_LEVEL, 0); glTexParameteri(GL_TEXTURE_3D, GL_TEXTURE_MAX_LEVEL, 4); glTexImage3D(GL_TEXTURE_3D,0,GL_RED,XDIM,YDIM,ZDIM,0,GL_RED,GL_UNSIGNED_BYTE,pData); glGenerateMipmap(GL_TEXTURE_3D); return true; } else { return false; }
The filtering parameters for 3D textures are similar to the 2D texture parameters that we have seen before. Mipmaps are collections of down-sampled versions of a texture that are used for level of detail (LOD) functionality. That is, they help to use a down-sampled version of the texture if the viewer is very far from the object on which the texture is applied. This helps improve the performance of the application. We have to specify the max number of levels (
GL_TEXTURE_MAX_LEVEL
), which is the maximum number of mipmaps generated from the given texture. In addition, the base level (GL_TEXTURE_BASE_LEVEL
) denotes the first level for the mipmap that is used when the object is closest. - Setup a vertex array object and a vertex buffer object to store the geometry of the proxy slices. Make sure that the buffer object usage is specified as
GL_DYNAMIC_DRAW
. The initialglBufferData
call allocates GPU memory for the maximum number of slices. ThevTextureSlices
array is defined globally and it stores the vertices produced by texture slicing operation for triangulation. TheglBufferData
is initialized with0
as the data will be filled at runtime dynamically.const int MAX_SLICES = 512; glm::vec3 vTextureSlices[MAX_SLICES*12]; glGenVertexArrays(1, &volumeVAO); glGenBuffers(1, &volumeVBO); glBindVertexArray(volumeVAO); glBindBuffer (GL_ARRAY_BUFFER, volumeVBO); glBufferData (GL_ARRAY_BUFFER, sizeof(vTextureSlices), 0, GL_DYNAMIC_DRAW); glEnableVertexAttribArray(0); glVertexAttribPointer(0, 3, GL_FLOAT, GL_FALSE,0,0); glBindVertexArray(0);
- Implement slicing of volume by finding intersections of a unit cube with proxy slices perpendicular to the viewing direction. This is carried out by the
SliceVolume
function. We use a unit cube since our data has equal size in all three axes that is, 256×256×256. If we have a non-equal sized dataset, we can scale the unit cube appropriately.//determine max and min distances glm::vec3 vecStart[12]; glm::vec3 vecDir[12]; float lambda[12]; float lambda_inc[12]; float denom = 0; float plane_dist = min_dist; float plane_dist_inc = (max_dist-min_dist)/float(num_slices); //determine vecStart and vecDir values glm::vec3 intersection[6]; float dL[12]; for(int i=num_slices-1;i>=0;i--) { for(int e = 0; e < 12; e++) { dL[e] = lambda[e] + i*lambda_inc[e]; } if ((dL[0] >= 0.0) && (dL[0] < 1.0)) { intersection[0] = vecStart[0] + dL[0]*vecDir[0]; } //like wise for all intersection points int indices[]={0,1,2, 0,2,3, 0,3,4, 0,4,5}; for(int i=0;i<12;i++) vTextureSlices[count++]=intersection[indices[i]]; } //update buffer object glBindBuffer(GL_ARRAY_BUFFER, volumeVBO); glBufferSubData(GL_ARRAY_BUFFER, 0, sizeof(vTextureSlices), &(vTextureSlices[0].x));
- In the render function, set the over blending, bind the volume vertex array object, bind the shader, and then call the
glDrawArrays
function.glEnable(GL_BLEND); glBlendFunc(GL_SRC_ALPHA, GL_ONE_MINUS_SRC_ALPHA); glBindVertexArray(volumeVAO); shader.Use(); glUniformMatrix4fv(shader("MVP"), 1, GL_FALSE, glm::value_ptr(MVP)); glDrawArrays(GL_TRIANGLES, 0, sizeof(vTextureSlices)/sizeof(vTextureSlices[0])); shader.UnUse(); glDisable(GL_BLEND);
Volume rendering using 3D texture slicing approximates the volume rendering integral by alpha-blending textured slices. The first step is loading and generating a 3D texture from the volume data. After loading the volume dataset, the slicing of the volume is carried out using proxy slices. These are oriented perpendicular to the viewing direction. Moreover, we have to find the intersection of the proxy polygons with the unit cube boundaries. This is carried out by the SliceVolume
function. Note that slicing is carried out only when the view is rotated.
Next, plane intersection distances are estimated for the 12 edge indices of the unit cube:
In the rendering function, the appropriate shader is bound. The vertex shader calculates the clip space position by multiplying the object space vertex position (vPosition
) with the combined model view projection (MVP
) matrix. It also calculates the 3D texture coordinates (vUV
) for the volume data. Since we render a unit cube, the minimum vertex position will be (-0.5,-0.5,-0.5) and the maximum vertex position will be (0.5,0.5,0.5). Since our 3D texture lookup requires coordinates from (0,0,0) to (1,1,1), we add (0.5,0.5,0.5) to the object space vertex position to obtain the correct 3D texture coordinates.
The fragment shader then uses the 3D texture coordinates to sample the volume data (which is now accessed through a new sampler type sampler3D
for 3D textures) to display the density. At the time of creation of the 3D texture, we specified the internal format as GL_RED
(the third parameter of the glTexImage3D
function). Therefore, we can now access our densities through the red channel of the texture sampler. To get a shader of grey, we set the same value for green, blue, and alpha channels as well.
In previous OpenGL versions, we would store the volume densities in a special internal format GL_INTENSITY
. This is deprecated in the OpenGL3.3 core profile. So now we have to use GL_RED
, GL_GREEN
, GL_BLUE
, or GL_ALPHA
internal formats.
As can be seen, increasing the number of slices improves the volume rendering result. When the total number of slices goes beyond 256 slices, we do not see a significant difference in the rendering result. However, we begin to see a sharp decrease in performance as we increase the total number of slices beyond 350. This is because more geometry is transferred to the GPU and that reduces performance.
- The 3.5.2 Viewport-Aligned Slices section in Chapter 3, GPU-based Volume Rendering, Real-time Volume Graphics, AK Peters/CRC Press, page numbers 73 to 79
Chapter7/3DTextureSlicing
directory.
Let us start our recipe by following these simple steps:
- Load the volume dataset by reading the external volume datafile and passing the data into an OpenGL texture. Also enable hardware mipmap generation. Typically, the volume datafiles store densities that are obtained from using a cross-sectional imaging modality such as CT or MRI scans. Each CT/MRI scan is a 2D slice. We accumulate these slices in Z direction to obtain a 3D texture, which is simply an array of 2D textures. The densities store different material types, for example, values ranging from 0 to 20 are typically occupied by air. As we have an 8-bit unsigned dataset, we store the dataset into a local array of
GLubyte
type. If we had an unsigned 16-bit dataset, we would have stored it into a local array ofGLushort
type. In case of 3D textures, in addition to theS
andT
parameters, we have an additional parameterR
that controls the slice we are at in the 3D texture.std::ifstream infile(volume_file.c_str(), std::ios_base::binary); if(infile.good()) { GLubyte* pData = new GLubyte[XDIM*YDIM*ZDIM]; infile.read(reinterpret_cast<char*>(pData), XDIM*YDIM*ZDIM*sizeof(GLubyte)); infile.close(); glGenTextures(1, &textureID); glBindTexture(GL_TEXTURE_3D, textureID); glTexParameteri(GL_TEXTURE_3D, GL_TEXTURE_WRAP_S, GL_CLAMP); glTexParameteri(GL_TEXTURE_3D, GL_TEXTURE_WRAP_T, GL_CLAMP); glTexParameteri(GL_TEXTURE_3D, GL_TEXTURE_WRAP_R, GL_CLAMP); glTexParameteri(GL_TEXTURE_3D, GL_TEXTURE_MAG_FILTER, GL_LINEAR); glTexParameteri(GL_TEXTURE_3D, GL_TEXTURE_MIN_FILTER, GL_LINEAR_MIPMAP_LINEAR); glTexParameteri(GL_TEXTURE_3D, GL_TEXTURE_BASE_LEVEL, 0); glTexParameteri(GL_TEXTURE_3D, GL_TEXTURE_MAX_LEVEL, 4); glTexImage3D(GL_TEXTURE_3D,0,GL_RED,XDIM,YDIM,ZDIM,0,GL_RED,GL_UNSIGNED_BYTE,pData); glGenerateMipmap(GL_TEXTURE_3D); return true; } else { return false; }
The filtering parameters for 3D textures are similar to the 2D texture parameters that we have seen before. Mipmaps are collections of down-sampled versions of a texture that are used for level of detail (LOD) functionality. That is, they help to use a down-sampled version of the texture if the viewer is very far from the object on which the texture is applied. This helps improve the performance of the application. We have to specify the max number of levels (
GL_TEXTURE_MAX_LEVEL
), which is the maximum number of mipmaps generated from the given texture. In addition, the base level (GL_TEXTURE_BASE_LEVEL
) denotes the first level for the mipmap that is used when the object is closest. - Setup a vertex array object and a vertex buffer object to store the geometry of the proxy slices. Make sure that the buffer object usage is specified as
GL_DYNAMIC_DRAW
. The initialglBufferData
call allocates GPU memory for the maximum number of slices. ThevTextureSlices
array is defined globally and it stores the vertices produced by texture slicing operation for triangulation. TheglBufferData
is initialized with0
as the data will be filled at runtime dynamically.const int MAX_SLICES = 512; glm::vec3 vTextureSlices[MAX_SLICES*12]; glGenVertexArrays(1, &volumeVAO); glGenBuffers(1, &volumeVBO); glBindVertexArray(volumeVAO); glBindBuffer (GL_ARRAY_BUFFER, volumeVBO); glBufferData (GL_ARRAY_BUFFER, sizeof(vTextureSlices), 0, GL_DYNAMIC_DRAW); glEnableVertexAttribArray(0); glVertexAttribPointer(0, 3, GL_FLOAT, GL_FALSE,0,0); glBindVertexArray(0);
- Implement slicing of volume by finding intersections of a unit cube with proxy slices perpendicular to the viewing direction. This is carried out by the
SliceVolume
function. We use a unit cube since our data has equal size in all three axes that is, 256×256×256. If we have a non-equal sized dataset, we can scale the unit cube appropriately.//determine max and min distances glm::vec3 vecStart[12]; glm::vec3 vecDir[12]; float lambda[12]; float lambda_inc[12]; float denom = 0; float plane_dist = min_dist; float plane_dist_inc = (max_dist-min_dist)/float(num_slices); //determine vecStart and vecDir values glm::vec3 intersection[6]; float dL[12]; for(int i=num_slices-1;i>=0;i--) { for(int e = 0; e < 12; e++) { dL[e] = lambda[e] + i*lambda_inc[e]; } if ((dL[0] >= 0.0) && (dL[0] < 1.0)) { intersection[0] = vecStart[0] + dL[0]*vecDir[0]; } //like wise for all intersection points int indices[]={0,1,2, 0,2,3, 0,3,4, 0,4,5}; for(int i=0;i<12;i++) vTextureSlices[count++]=intersection[indices[i]]; } //update buffer object glBindBuffer(GL_ARRAY_BUFFER, volumeVBO); glBufferSubData(GL_ARRAY_BUFFER, 0, sizeof(vTextureSlices), &(vTextureSlices[0].x));
- In the render function, set the over blending, bind the volume vertex array object, bind the shader, and then call the
glDrawArrays
function.glEnable(GL_BLEND); glBlendFunc(GL_SRC_ALPHA, GL_ONE_MINUS_SRC_ALPHA); glBindVertexArray(volumeVAO); shader.Use(); glUniformMatrix4fv(shader("MVP"), 1, GL_FALSE, glm::value_ptr(MVP)); glDrawArrays(GL_TRIANGLES, 0, sizeof(vTextureSlices)/sizeof(vTextureSlices[0])); shader.UnUse(); glDisable(GL_BLEND);
Volume rendering using 3D texture slicing approximates the volume rendering integral by alpha-blending textured slices. The first step is loading and generating a 3D texture from the volume data. After loading the volume dataset, the slicing of the volume is carried out using proxy slices. These are oriented perpendicular to the viewing direction. Moreover, we have to find the intersection of the proxy polygons with the unit cube boundaries. This is carried out by the SliceVolume
function. Note that slicing is carried out only when the view is rotated.
Next, plane intersection distances are estimated for the 12 edge indices of the unit cube:
In the rendering function, the appropriate shader is bound. The vertex shader calculates the clip space position by multiplying the object space vertex position (vPosition
) with the combined model view projection (MVP
) matrix. It also calculates the 3D texture coordinates (vUV
) for the volume data. Since we render a unit cube, the minimum vertex position will be (-0.5,-0.5,-0.5) and the maximum vertex position will be (0.5,0.5,0.5). Since our 3D texture lookup requires coordinates from (0,0,0) to (1,1,1), we add (0.5,0.5,0.5) to the object space vertex position to obtain the correct 3D texture coordinates.
The fragment shader then uses the 3D texture coordinates to sample the volume data (which is now accessed through a new sampler type sampler3D
for 3D textures) to display the density. At the time of creation of the 3D texture, we specified the internal format as GL_RED
(the third parameter of the glTexImage3D
function). Therefore, we can now access our densities through the red channel of the texture sampler. To get a shader of grey, we set the same value for green, blue, and alpha channels as well.
In previous OpenGL versions, we would store the volume densities in a special internal format GL_INTENSITY
. This is deprecated in the OpenGL3.3 core profile. So now we have to use GL_RED
, GL_GREEN
, GL_BLUE
, or GL_ALPHA
internal formats.
As can be seen, increasing the number of slices improves the volume rendering result. When the total number of slices goes beyond 256 slices, we do not see a significant difference in the rendering result. However, we begin to see a sharp decrease in performance as we increase the total number of slices beyond 350. This is because more geometry is transferred to the GPU and that reduces performance.
- The 3.5.2 Viewport-Aligned Slices section in Chapter 3, GPU-based Volume Rendering, Real-time Volume Graphics, AK Peters/CRC Press, page numbers 73 to 79
recipe by following these simple steps:
- Load the volume dataset by reading the external volume datafile and passing the data into an OpenGL texture. Also enable hardware mipmap generation. Typically, the volume datafiles store densities that are obtained from using a cross-sectional imaging modality such as CT or MRI scans. Each CT/MRI scan is a 2D slice. We accumulate these slices in Z direction to obtain a 3D texture, which is simply an array of 2D textures. The densities store different material types, for example, values ranging from 0 to 20 are typically occupied by air. As we have an 8-bit unsigned dataset, we store the dataset into a local array of
GLubyte
type. If we had an unsigned 16-bit dataset, we would have stored it into a local array ofGLushort
type. In case of 3D textures, in addition to theS
andT
parameters, we have an additional parameterR
that controls the slice we are at in the 3D texture.std::ifstream infile(volume_file.c_str(), std::ios_base::binary); if(infile.good()) { GLubyte* pData = new GLubyte[XDIM*YDIM*ZDIM]; infile.read(reinterpret_cast<char*>(pData), XDIM*YDIM*ZDIM*sizeof(GLubyte)); infile.close(); glGenTextures(1, &textureID); glBindTexture(GL_TEXTURE_3D, textureID); glTexParameteri(GL_TEXTURE_3D, GL_TEXTURE_WRAP_S, GL_CLAMP); glTexParameteri(GL_TEXTURE_3D, GL_TEXTURE_WRAP_T, GL_CLAMP); glTexParameteri(GL_TEXTURE_3D, GL_TEXTURE_WRAP_R, GL_CLAMP); glTexParameteri(GL_TEXTURE_3D, GL_TEXTURE_MAG_FILTER, GL_LINEAR); glTexParameteri(GL_TEXTURE_3D, GL_TEXTURE_MIN_FILTER, GL_LINEAR_MIPMAP_LINEAR); glTexParameteri(GL_TEXTURE_3D, GL_TEXTURE_BASE_LEVEL, 0); glTexParameteri(GL_TEXTURE_3D, GL_TEXTURE_MAX_LEVEL, 4); glTexImage3D(GL_TEXTURE_3D,0,GL_RED,XDIM,YDIM,ZDIM,0,GL_RED,GL_UNSIGNED_BYTE,pData); glGenerateMipmap(GL_TEXTURE_3D); return true; } else { return false; }
The filtering parameters for 3D textures are similar to the 2D texture parameters that we have seen before. Mipmaps are collections of down-sampled versions of a texture that are used for level of detail (LOD) functionality. That is, they help to use a down-sampled version of the texture if the viewer is very far from the object on which the texture is applied. This helps improve the performance of the application. We have to specify the max number of levels (
GL_TEXTURE_MAX_LEVEL
), which is the maximum number of mipmaps generated from the given texture. In addition, the base level (GL_TEXTURE_BASE_LEVEL
) denotes the first level for the mipmap that is used when the object is closest. - Setup a vertex array object and a vertex buffer object to store the geometry of the proxy slices. Make sure that the buffer object usage is specified as
GL_DYNAMIC_DRAW
. The initialglBufferData
call allocates GPU memory for the maximum number of slices. ThevTextureSlices
array is defined globally and it stores the vertices produced by texture slicing operation for triangulation. TheglBufferData
is initialized with0
as the data will be filled at runtime dynamically.const int MAX_SLICES = 512; glm::vec3 vTextureSlices[MAX_SLICES*12]; glGenVertexArrays(1, &volumeVAO); glGenBuffers(1, &volumeVBO); glBindVertexArray(volumeVAO); glBindBuffer (GL_ARRAY_BUFFER, volumeVBO); glBufferData (GL_ARRAY_BUFFER, sizeof(vTextureSlices), 0, GL_DYNAMIC_DRAW); glEnableVertexAttribArray(0); glVertexAttribPointer(0, 3, GL_FLOAT, GL_FALSE,0,0); glBindVertexArray(0);
- Implement slicing of volume by finding intersections of a unit cube with proxy slices perpendicular to the viewing direction. This is carried out by the
SliceVolume
function. We use a unit cube since our data has equal size in all three axes that is, 256×256×256. If we have a non-equal sized dataset, we can scale the unit cube appropriately.//determine max and min distances glm::vec3 vecStart[12]; glm::vec3 vecDir[12]; float lambda[12]; float lambda_inc[12]; float denom = 0; float plane_dist = min_dist; float plane_dist_inc = (max_dist-min_dist)/float(num_slices); //determine vecStart and vecDir values glm::vec3 intersection[6]; float dL[12]; for(int i=num_slices-1;i>=0;i--) { for(int e = 0; e < 12; e++) { dL[e] = lambda[e] + i*lambda_inc[e]; } if ((dL[0] >= 0.0) && (dL[0] < 1.0)) { intersection[0] = vecStart[0] + dL[0]*vecDir[0]; } //like wise for all intersection points int indices[]={0,1,2, 0,2,3, 0,3,4, 0,4,5}; for(int i=0;i<12;i++) vTextureSlices[count++]=intersection[indices[i]]; } //update buffer object glBindBuffer(GL_ARRAY_BUFFER, volumeVBO); glBufferSubData(GL_ARRAY_BUFFER, 0, sizeof(vTextureSlices), &(vTextureSlices[0].x));
- In the render function, set the over blending, bind the volume vertex array object, bind the shader, and then call the
glDrawArrays
function.glEnable(GL_BLEND); glBlendFunc(GL_SRC_ALPHA, GL_ONE_MINUS_SRC_ALPHA); glBindVertexArray(volumeVAO); shader.Use(); glUniformMatrix4fv(shader("MVP"), 1, GL_FALSE, glm::value_ptr(MVP)); glDrawArrays(GL_TRIANGLES, 0, sizeof(vTextureSlices)/sizeof(vTextureSlices[0])); shader.UnUse(); glDisable(GL_BLEND);
Volume rendering using 3D texture slicing approximates the volume rendering integral by alpha-blending textured slices. The first step is loading and generating a 3D texture from the volume data. After loading the volume dataset, the slicing of the volume is carried out using proxy slices. These are oriented perpendicular to the viewing direction. Moreover, we have to find the intersection of the proxy polygons with the unit cube boundaries. This is carried out by the SliceVolume
function. Note that slicing is carried out only when the view is rotated.
Next, plane intersection distances are estimated for the 12 edge indices of the unit cube:
In the rendering function, the appropriate shader is bound. The vertex shader calculates the clip space position by multiplying the object space vertex position (vPosition
) with the combined model view projection (MVP
) matrix. It also calculates the 3D texture coordinates (vUV
) for the volume data. Since we render a unit cube, the minimum vertex position will be (-0.5,-0.5,-0.5) and the maximum vertex position will be (0.5,0.5,0.5). Since our 3D texture lookup requires coordinates from (0,0,0) to (1,1,1), we add (0.5,0.5,0.5) to the object space vertex position to obtain the correct 3D texture coordinates.
The fragment shader then uses the 3D texture coordinates to sample the volume data (which is now accessed through a new sampler type sampler3D
for 3D textures) to display the density. At the time of creation of the 3D texture, we specified the internal format as GL_RED
(the third parameter of the glTexImage3D
function). Therefore, we can now access our densities through the red channel of the texture sampler. To get a shader of grey, we set the same value for green, blue, and alpha channels as well.
In previous OpenGL versions, we would store the volume densities in a special internal format GL_INTENSITY
. This is deprecated in the OpenGL3.3 core profile. So now we have to use GL_RED
, GL_GREEN
, GL_BLUE
, or GL_ALPHA
internal formats.
As can be seen, increasing the number of slices improves the volume rendering result. When the total number of slices goes beyond 256 slices, we do not see a significant difference in the rendering result. However, we begin to see a sharp decrease in performance as we increase the total number of slices beyond 350. This is because more geometry is transferred to the GPU and that reduces performance.
- The 3.5.2 Viewport-Aligned Slices section in Chapter 3, GPU-based Volume Rendering, Real-time Volume Graphics, AK Peters/CRC Press, page numbers 73 to 79
using 3D texture slicing approximates the volume rendering integral by alpha-blending textured slices. The first step is loading and generating a 3D texture from the volume data. After loading the volume dataset, the slicing of the volume is carried out using proxy slices. These are oriented perpendicular to the viewing direction. Moreover, we have to find the intersection of the proxy polygons with the unit cube boundaries. This is carried out by the SliceVolume
function. Note that slicing is carried out only when the view is rotated.
Next, plane intersection distances are estimated for the 12 edge indices of the unit cube:
In the rendering function, the appropriate shader is bound. The vertex shader calculates the clip space position by multiplying the object space vertex position (vPosition
) with the combined model view projection (MVP
) matrix. It also calculates the 3D texture coordinates (vUV
) for the volume data. Since we render a unit cube, the minimum vertex position will be (-0.5,-0.5,-0.5) and the maximum vertex position will be (0.5,0.5,0.5). Since our 3D texture lookup requires coordinates from (0,0,0) to (1,1,1), we add (0.5,0.5,0.5) to the object space vertex position to obtain the correct 3D texture coordinates.
The fragment shader then uses the 3D texture coordinates to sample the volume data (which is now accessed through a new sampler type sampler3D
for 3D textures) to display the density. At the time of creation of the 3D texture, we specified the internal format as GL_RED
(the third parameter of the glTexImage3D
function). Therefore, we can now access our densities through the red channel of the texture sampler. To get a shader of grey, we set the same value for green, blue, and alpha channels as well.
In previous OpenGL versions, we would store the volume densities in a special internal format GL_INTENSITY
. This is deprecated in the OpenGL3.3 core profile. So now we have to use GL_RED
, GL_GREEN
, GL_BLUE
, or GL_ALPHA
internal formats.
As can be seen, increasing the number of slices improves the volume rendering result. When the total number of slices goes beyond 256 slices, we do not see a significant difference in the rendering result. However, we begin to see a sharp decrease in performance as we increase the total number of slices beyond 350. This is because more geometry is transferred to the GPU and that reduces performance.
- The 3.5.2 Viewport-Aligned Slices section in Chapter 3, GPU-based Volume Rendering, Real-time Volume Graphics, AK Peters/CRC Press, page numbers 73 to 79
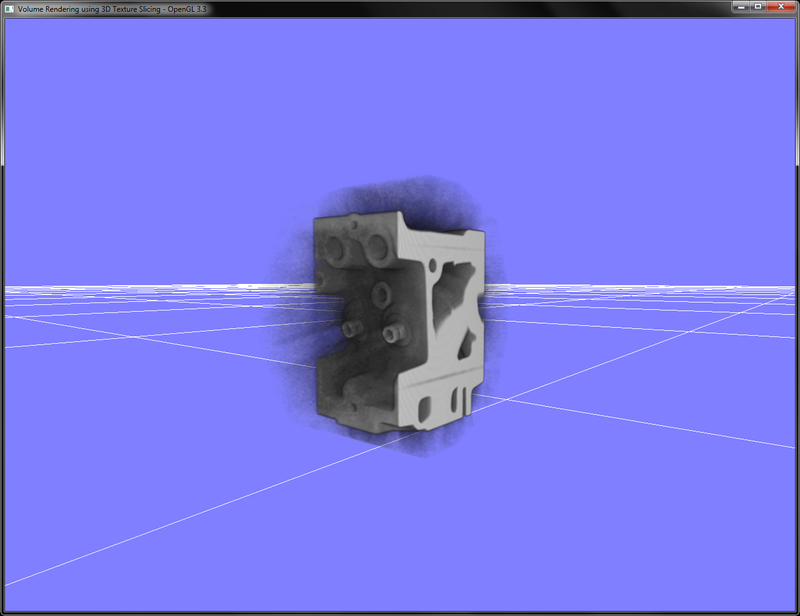
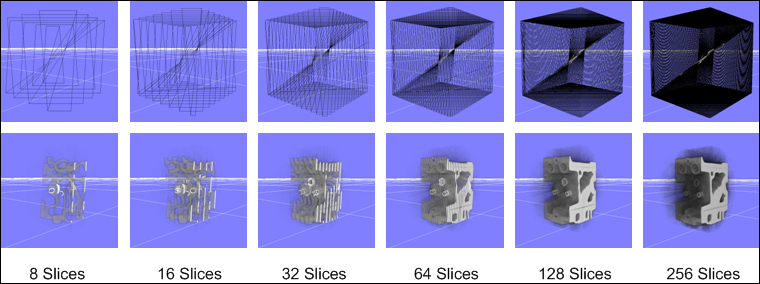
increasing the number of slices improves the volume rendering result. When the total number of slices goes beyond 256 slices, we do not see a significant difference in the rendering result. However, we begin to see a sharp decrease in performance as we increase the total number of slices beyond 350. This is because more geometry is transferred to the GPU and that reduces performance.
- The 3.5.2 Viewport-Aligned Slices section in Chapter 3, GPU-based Volume Rendering, Real-time Volume Graphics, AK Peters/CRC Press, page numbers 73 to 79
- Chapter 3, GPU-based Volume Rendering, Real-time Volume Graphics, AK Peters/CRC Press, page numbers 73 to 79
In this recipe, we will implement volume rendering using single-pass GPU ray casting. There are two basic approaches for doing GPU ray casting: the multi-pass approach and the single-pass approach. Both of these approaches differ in how they estimate the ray marching direction vectors. The single-pass approach uses a single fragment shader. The steps described here can be understood easily from the following diagram:
The steps required to implement single-pass GPU ray casting are as follows:
- Load the volume data from the file into a 3D OpenGL texture as in the previous recipe. Refer to the
LoadVolume
function inChapter7/GPURaycasting/main.cpp
for details. - Set up a vertex array object and a vertex buffer object to render a unit cube as follows:
glGenVertexArrays(1, &cubeVAOID); glGenBuffers(1, &cubeVBOID); glGenBuffers(1, &cubeIndicesID); glm::vec3 vertices[8]={ glm::vec3(-0.5f,-0.5f,-0.5f), glm::vec3( 0.5f,-0.5f,-0.5f),glm::vec3( 0.5f, 0.5f,-0.5f), glm::vec3(-0.5f, 0.5f,-0.5f),glm::vec3(-0.5f,-0.5f, 0.5f), glm::vec3( 0.5f,-0.5f, 0.5f),glm::vec3( 0.5f, 0.5f, 0.5f), glm::vec3(-0.5f, 0.5f, 0.5f)}; GLushort cubeIndices[36]={0,5,4,5,0,1,3,7,6,3,6,2,7,4,6,6,4,5,2,1, 3,3,1,0,3,0,7,7,0,4,6,5,2,2,5,1}; glBindVertexArray(cubeVAOID); glBindBuffer (GL_ARRAY_BUFFER, cubeVBOID); glBufferData (GL_ARRAY_BUFFER, sizeof(vertices), &(vertices[0].x), GL_STATIC_DRAW); glEnableVertexAttribArray(0); glVertexAttribPointer(0, 3, GL_FLOAT, GL_FALSE,0,0); glBindBuffer (GL_ELEMENT_ARRAY_BUFFER, cubeIndicesID); glBufferData (GL_ELEMENT_ARRAY_BUFFER, sizeof(cubeIndices), &cubeIndices[0], GL_STATIC_DRAW); glBindVertexArray(0);
- In the render function, set the ray casting vertex and fragment shaders (
Chapter7/GPURaycasting/shaders/raycaster
.(vert,frag)) and then render the unit cube.glEnable(GL_BLEND); glBindVertexArray(cubeVAOID); shader.Use(); glUniformMatrix4fv(shader("MVP"), 1, GL_FALSE, glm::value_ptr(MVP)); glUniform3fv(shader("camPos"), 1, &(camPos.x)); glDrawElements(GL_TRIANGLES, 36, GL_UNSIGNED_SHORT, 0); shader.UnUse(); glDisable(GL_BLEND);
- From the vertex shader, in addition to the clip space position, output the 3D texture coordinates for lookup in the fragment shader. We simply offset the object space vertex positions.
smooth out vec3 vUV; void main() { gl_Position = MVP*vec4(vVertex.xyz,1); vUV = vVertex + vec3(0.5); }
- In the fragment shader, use the camera position and the 3D texture coordinates to run a loop in the current viewing direction. Terminate the loop if the current sample position is outside the volume or the alpha value of the accumulated color is saturated.
vec3 dataPos = vUV; vec3 geomDir = normalize((vUV-vec3(0.5)) - camPos); vec3 dirStep = geomDir * step_size; bool stop = false; for (int i = 0; i < MAX_SAMPLES; i++) { // advance ray by step dataPos = dataPos + dirStep; // stop condition stop=dot(sign(dataPos-texMin),sign(texMax-dataPos)) < 3.0; if (stop) break;
- Composite the current sample value obtained from the volume using an appropriate operator and finally return the composited color.
float sample = texture(volume, dataPos).r; float prev_alpha = sample - (sample * vFragColor.a); vFragColor.rgb = prev_alpha * vec3(sample) + vFragColor.rgb; vFragColor.a += prev_alpha; //early ray termination if( vFragColor.a>0.99) break; }
In the vertex shader (Chapter7/GPURaycasting/shaders/raycast.vert
), the 3D texture coordinates are estimated using the per-vertex position of the unit cube. Since the unit cube is at origin, we add vec(0.5)
to the position to bring the 3D texture coordinates to the 0 to 1 range.
The demo application for this demo shows the engine dataset rendered using single-pass GPU ray casting. The camera position can be changed using the left-mouse button and the view can be zoomed in/out by using the middle-mouse button.
- Chapter 7, GPU-based Ray Casting, Real-time Volume Graphics, AK Peters/CRC Press, page numbers 163 to 184
- Single pass Raycasting at The Little Grasshopper, http://prideout.net/blog/?p=64
Chapter7/GPURaycasting
folder.
The steps required to implement single-pass GPU ray casting are as follows:
- Load the volume data from the file into a 3D OpenGL texture as in the previous recipe. Refer to the
LoadVolume
function inChapter7/GPURaycasting/main.cpp
for details. - Set up a vertex array object and a vertex buffer object to render a unit cube as follows:
glGenVertexArrays(1, &cubeVAOID); glGenBuffers(1, &cubeVBOID); glGenBuffers(1, &cubeIndicesID); glm::vec3 vertices[8]={ glm::vec3(-0.5f,-0.5f,-0.5f), glm::vec3( 0.5f,-0.5f,-0.5f),glm::vec3( 0.5f, 0.5f,-0.5f), glm::vec3(-0.5f, 0.5f,-0.5f),glm::vec3(-0.5f,-0.5f, 0.5f), glm::vec3( 0.5f,-0.5f, 0.5f),glm::vec3( 0.5f, 0.5f, 0.5f), glm::vec3(-0.5f, 0.5f, 0.5f)}; GLushort cubeIndices[36]={0,5,4,5,0,1,3,7,6,3,6,2,7,4,6,6,4,5,2,1, 3,3,1,0,3,0,7,7,0,4,6,5,2,2,5,1}; glBindVertexArray(cubeVAOID); glBindBuffer (GL_ARRAY_BUFFER, cubeVBOID); glBufferData (GL_ARRAY_BUFFER, sizeof(vertices), &(vertices[0].x), GL_STATIC_DRAW); glEnableVertexAttribArray(0); glVertexAttribPointer(0, 3, GL_FLOAT, GL_FALSE,0,0); glBindBuffer (GL_ELEMENT_ARRAY_BUFFER, cubeIndicesID); glBufferData (GL_ELEMENT_ARRAY_BUFFER, sizeof(cubeIndices), &cubeIndices[0], GL_STATIC_DRAW); glBindVertexArray(0);
- In the render function, set the ray casting vertex and fragment shaders (
Chapter7/GPURaycasting/shaders/raycaster
.(vert,frag)) and then render the unit cube.glEnable(GL_BLEND); glBindVertexArray(cubeVAOID); shader.Use(); glUniformMatrix4fv(shader("MVP"), 1, GL_FALSE, glm::value_ptr(MVP)); glUniform3fv(shader("camPos"), 1, &(camPos.x)); glDrawElements(GL_TRIANGLES, 36, GL_UNSIGNED_SHORT, 0); shader.UnUse(); glDisable(GL_BLEND);
- From the vertex shader, in addition to the clip space position, output the 3D texture coordinates for lookup in the fragment shader. We simply offset the object space vertex positions.
smooth out vec3 vUV; void main() { gl_Position = MVP*vec4(vVertex.xyz,1); vUV = vVertex + vec3(0.5); }
- In the fragment shader, use the camera position and the 3D texture coordinates to run a loop in the current viewing direction. Terminate the loop if the current sample position is outside the volume or the alpha value of the accumulated color is saturated.
vec3 dataPos = vUV; vec3 geomDir = normalize((vUV-vec3(0.5)) - camPos); vec3 dirStep = geomDir * step_size; bool stop = false; for (int i = 0; i < MAX_SAMPLES; i++) { // advance ray by step dataPos = dataPos + dirStep; // stop condition stop=dot(sign(dataPos-texMin),sign(texMax-dataPos)) < 3.0; if (stop) break;
- Composite the current sample value obtained from the volume using an appropriate operator and finally return the composited color.
float sample = texture(volume, dataPos).r; float prev_alpha = sample - (sample * vFragColor.a); vFragColor.rgb = prev_alpha * vec3(sample) + vFragColor.rgb; vFragColor.a += prev_alpha; //early ray termination if( vFragColor.a>0.99) break; }
In the vertex shader (Chapter7/GPURaycasting/shaders/raycast.vert
), the 3D texture coordinates are estimated using the per-vertex position of the unit cube. Since the unit cube is at origin, we add vec(0.5)
to the position to bring the 3D texture coordinates to the 0 to 1 range.
The demo application for this demo shows the engine dataset rendered using single-pass GPU ray casting. The camera position can be changed using the left-mouse button and the view can be zoomed in/out by using the middle-mouse button.
- Chapter 7, GPU-based Ray Casting, Real-time Volume Graphics, AK Peters/CRC Press, page numbers 163 to 184
- Single pass Raycasting at The Little Grasshopper, http://prideout.net/blog/?p=64
implement single-pass GPU ray casting are as follows:
- Load the volume data from the file into a 3D OpenGL texture as in the previous recipe. Refer to the
LoadVolume
function inChapter7/GPURaycasting/main.cpp
for details. - Set up a vertex array object and a vertex buffer object to render a unit cube as follows:
glGenVertexArrays(1, &cubeVAOID); glGenBuffers(1, &cubeVBOID); glGenBuffers(1, &cubeIndicesID); glm::vec3 vertices[8]={ glm::vec3(-0.5f,-0.5f,-0.5f), glm::vec3( 0.5f,-0.5f,-0.5f),glm::vec3( 0.5f, 0.5f,-0.5f), glm::vec3(-0.5f, 0.5f,-0.5f),glm::vec3(-0.5f,-0.5f, 0.5f), glm::vec3( 0.5f,-0.5f, 0.5f),glm::vec3( 0.5f, 0.5f, 0.5f), glm::vec3(-0.5f, 0.5f, 0.5f)}; GLushort cubeIndices[36]={0,5,4,5,0,1,3,7,6,3,6,2,7,4,6,6,4,5,2,1, 3,3,1,0,3,0,7,7,0,4,6,5,2,2,5,1}; glBindVertexArray(cubeVAOID); glBindBuffer (GL_ARRAY_BUFFER, cubeVBOID); glBufferData (GL_ARRAY_BUFFER, sizeof(vertices), &(vertices[0].x), GL_STATIC_DRAW); glEnableVertexAttribArray(0); glVertexAttribPointer(0, 3, GL_FLOAT, GL_FALSE,0,0); glBindBuffer (GL_ELEMENT_ARRAY_BUFFER, cubeIndicesID); glBufferData (GL_ELEMENT_ARRAY_BUFFER, sizeof(cubeIndices), &cubeIndices[0], GL_STATIC_DRAW); glBindVertexArray(0);
- In the render function, set the ray casting vertex and fragment shaders (
Chapter7/GPURaycasting/shaders/raycaster
.(vert,frag)) and then render the unit cube.glEnable(GL_BLEND); glBindVertexArray(cubeVAOID); shader.Use(); glUniformMatrix4fv(shader("MVP"), 1, GL_FALSE, glm::value_ptr(MVP)); glUniform3fv(shader("camPos"), 1, &(camPos.x)); glDrawElements(GL_TRIANGLES, 36, GL_UNSIGNED_SHORT, 0); shader.UnUse(); glDisable(GL_BLEND);
- From the vertex shader, in addition to the clip space position, output the 3D texture coordinates for lookup in the fragment shader. We simply offset the object space vertex positions.
smooth out vec3 vUV; void main() { gl_Position = MVP*vec4(vVertex.xyz,1); vUV = vVertex + vec3(0.5); }
- In the fragment shader, use the camera position and the 3D texture coordinates to run a loop in the current viewing direction. Terminate the loop if the current sample position is outside the volume or the alpha value of the accumulated color is saturated.
vec3 dataPos = vUV; vec3 geomDir = normalize((vUV-vec3(0.5)) - camPos); vec3 dirStep = geomDir * step_size; bool stop = false; for (int i = 0; i < MAX_SAMPLES; i++) { // advance ray by step dataPos = dataPos + dirStep; // stop condition stop=dot(sign(dataPos-texMin),sign(texMax-dataPos)) < 3.0; if (stop) break;
- Composite the current sample value obtained from the volume using an appropriate operator and finally return the composited color.
float sample = texture(volume, dataPos).r; float prev_alpha = sample - (sample * vFragColor.a); vFragColor.rgb = prev_alpha * vec3(sample) + vFragColor.rgb; vFragColor.a += prev_alpha; //early ray termination if( vFragColor.a>0.99) break; }
In the vertex shader (Chapter7/GPURaycasting/shaders/raycast.vert
), the 3D texture coordinates are estimated using the per-vertex position of the unit cube. Since the unit cube is at origin, we add vec(0.5)
to the position to bring the 3D texture coordinates to the 0 to 1 range.
The demo application for this demo shows the engine dataset rendered using single-pass GPU ray casting. The camera position can be changed using the left-mouse button and the view can be zoomed in/out by using the middle-mouse button.
- Chapter 7, GPU-based Ray Casting, Real-time Volume Graphics, AK Peters/CRC Press, page numbers 163 to 184
- Single pass Raycasting at The Little Grasshopper, http://prideout.net/blog/?p=64
shader (Chapter7/GPURaycasting/shaders/raycast.vert
), the 3D texture coordinates are estimated using the per-vertex position of the unit cube. Since the unit cube is at origin, we add vec(0.5)
to the position to bring the 3D texture coordinates to the 0 to 1 range.
The demo application for this demo shows the engine dataset rendered using single-pass GPU ray casting. The camera position can be changed using the left-mouse button and the view can be zoomed in/out by using the middle-mouse button.
- Chapter 7, GPU-based Ray Casting, Real-time Volume Graphics, AK Peters/CRC Press, page numbers 163 to 184
- Single pass Raycasting at The Little Grasshopper, http://prideout.net/blog/?p=64
- Chapter 7, GPU-based Ray Casting, Real-time Volume Graphics, AK Peters/CRC Press, page numbers 163 to 184
- Single pass Raycasting at The Little Grasshopper, http://prideout.net/blog/?p=64
- Chapter 7, GPU-based Ray Casting, Real-time Volume Graphics, AK Peters/CRC Press, page numbers 163 to 184
- Single pass Raycasting at The Little Grasshopper, http://prideout.net/blog/?p=64
We will now implement pseudo-isosurface rendering in single-pass GPU ray casting. While much of the setup is the same as for the single-pass GPU ray casting, the difference will be in the compositing step in the ray casting fragment shader. In this shader, we will try to find the given isosurface. If it is actually found, we estimate the normal at the sampling point to carry out the lighting calculation for the isosurface.
The code for this recipe is in the Chapter7/GPURaycastingIsosurface
folder. We will be starting from the single-pass GPU ray casting recipe using the exact same application side code.
Let us start the recipe by following these simple steps:
- Load the volume data from file into a 3D OpenGL texture as in the previous recipe. Refer to the
LoadVolume
function inChapter7/GPURaycasting/main.cpp
for details. - Set up a vertex array object and a vertex buffer object to render a unit cube as in the previous recipe.
- In the render function, set the ray casting vertex and fragment shaders (
Chapter7/GPURaycasting/shaders/raycasting
(vert,frag)) and then render the unit cube.glEnable(GL_BLEND); glBindVertexArray(cubeVAOID); shader.Use(); glUniformMatrix4fv(shader("MVP"), 1, GL_FALSE, glm::value_ptr(MVP)); glUniform3fv(shader("camPos"), 1, &(camPos.x)); glDrawElements(GL_TRIANGLES, 36, GL_UNSIGNED_SHORT, 0); shader.UnUse(); glDisable(GL_BLEND);
- From the vertex shader, in addition to the clip-space position, output the 3D texture coordinates for lookup in the fragment shader. We simply offset the object space vertex positions as follows:
smooth out vec3 vUV; void main() { gl_Position = MVP*vec4(vVertex.xyz,1); vUV = vVertex + vec3(0.5); }
- In the fragment shader, use the camera position and the 3D texture coordinates to run a loop in the current viewing direction. The loop is terminated if the current sample position is outside the volume or the alpha value of the accumulated color is saturated.
vec3 dataPos = vUV; vec3 geomDir = normalize((vUV-vec3(0.5)) - camPos); vec3 dirStep = geomDir * step_size; bool stop = false; for (int i = 0; i < MAX_SAMPLES; i++) { // advance ray by step dataPos = dataPos + dirStep; // stop condition stop=dot(sign(dataPos-texMin),sign(texMax-dataPos)) < 3.0; if (stop) break;
- For isosurface estimation, we take two sample values to find the zero crossing of the isofunction inside the volume dataset. If there is a zero crossing, we find the exact intersection point using bisection based refinement. Finally, we use the Phong illumination model to shade the isosurface assuming that the light is located at the camera position.
float sample=texture(volume, dataPos).r; float sample2=texture(volume, dataPos+dirStep).r; if( (sample -isoValue) < 0 && (sample2-isoValue) >= 0.0){ vec3 xN = dataPos; vec3 xF = dataPos+dirStep; vec3 tc = Bisection(xN, xF, isoValue); vec3 N = GetGradient(tc); vec3 V = -geomDir; vec3 L = V; vFragColor = PhongLighting(L,N,V,250, vec3(0.5)); break; } }
The Bisection
function is defined as follows:
The Bisection
function takes the two samples between which the given sample value lies. It then runs a loop. In each step, it calculates the midpoint of the two sample points and checks the density value at the midpoint to the given isovalue. If it is less, the left sample point is swapped with the mid position otherwise, the right sample point is swapped. This helps to reduce the search space quickly. The process is repeated and finally, the midpoint between the left sample point and right sample point is returned. The Gradient
function estimates the gradient of the volume density using center finite difference approximation.
While bulk of the code is similar to the single-pass GPU ray casting recipe. There is a major difference in the ray marching loop. In case of isosurface rendering, we do not use compositing. Instead, we find the zero crossing of the volume dataset isofunction by sampling two consecutive samples. This is well illustrated with the following diagram. If there is a zero crossing, we refine the detected isosurface by using bisection-based refinement.
recipe is in the Chapter7/GPURaycastingIsosurface
folder. We will be starting from the single-pass GPU ray casting recipe using the exact same application side code.
Let us start the recipe by following these simple steps:
- Load the volume data from file into a 3D OpenGL texture as in the previous recipe. Refer to the
LoadVolume
function inChapter7/GPURaycasting/main.cpp
for details. - Set up a vertex array object and a vertex buffer object to render a unit cube as in the previous recipe.
- In the render function, set the ray casting vertex and fragment shaders (
Chapter7/GPURaycasting/shaders/raycasting
(vert,frag)) and then render the unit cube.glEnable(GL_BLEND); glBindVertexArray(cubeVAOID); shader.Use(); glUniformMatrix4fv(shader("MVP"), 1, GL_FALSE, glm::value_ptr(MVP)); glUniform3fv(shader("camPos"), 1, &(camPos.x)); glDrawElements(GL_TRIANGLES, 36, GL_UNSIGNED_SHORT, 0); shader.UnUse(); glDisable(GL_BLEND);
- From the vertex shader, in addition to the clip-space position, output the 3D texture coordinates for lookup in the fragment shader. We simply offset the object space vertex positions as follows:
smooth out vec3 vUV; void main() { gl_Position = MVP*vec4(vVertex.xyz,1); vUV = vVertex + vec3(0.5); }
- In the fragment shader, use the camera position and the 3D texture coordinates to run a loop in the current viewing direction. The loop is terminated if the current sample position is outside the volume or the alpha value of the accumulated color is saturated.
vec3 dataPos = vUV; vec3 geomDir = normalize((vUV-vec3(0.5)) - camPos); vec3 dirStep = geomDir * step_size; bool stop = false; for (int i = 0; i < MAX_SAMPLES; i++) { // advance ray by step dataPos = dataPos + dirStep; // stop condition stop=dot(sign(dataPos-texMin),sign(texMax-dataPos)) < 3.0; if (stop) break;
- For isosurface estimation, we take two sample values to find the zero crossing of the isofunction inside the volume dataset. If there is a zero crossing, we find the exact intersection point using bisection based refinement. Finally, we use the Phong illumination model to shade the isosurface assuming that the light is located at the camera position.
float sample=texture(volume, dataPos).r; float sample2=texture(volume, dataPos+dirStep).r; if( (sample -isoValue) < 0 && (sample2-isoValue) >= 0.0){ vec3 xN = dataPos; vec3 xF = dataPos+dirStep; vec3 tc = Bisection(xN, xF, isoValue); vec3 N = GetGradient(tc); vec3 V = -geomDir; vec3 L = V; vFragColor = PhongLighting(L,N,V,250, vec3(0.5)); break; } }
The Bisection
function is defined as follows:
The Bisection
function takes the two samples between which the given sample value lies. It then runs a loop. In each step, it calculates the midpoint of the two sample points and checks the density value at the midpoint to the given isovalue. If it is less, the left sample point is swapped with the mid position otherwise, the right sample point is swapped. This helps to reduce the search space quickly. The process is repeated and finally, the midpoint between the left sample point and right sample point is returned. The Gradient
function estimates the gradient of the volume density using center finite difference approximation.
While bulk of the code is similar to the single-pass GPU ray casting recipe. There is a major difference in the ray marching loop. In case of isosurface rendering, we do not use compositing. Instead, we find the zero crossing of the volume dataset isofunction by sampling two consecutive samples. This is well illustrated with the following diagram. If there is a zero crossing, we refine the detected isosurface by using bisection-based refinement.
LoadVolume
function in Chapter7/GPURaycasting/main.cpp
for details.
Chapter7/GPURaycasting/shaders/raycasting
(vert,frag)) and then render the unit cube.glEnable(GL_BLEND); glBindVertexArray(cubeVAOID); shader.Use(); glUniformMatrix4fv(shader("MVP"), 1, GL_FALSE, glm::value_ptr(MVP)); glUniform3fv(shader("camPos"), 1, &(camPos.x)); glDrawElements(GL_TRIANGLES, 36, GL_UNSIGNED_SHORT, 0); shader.UnUse(); glDisable(GL_BLEND);
smooth out vec3 vUV; void main() { gl_Position = MVP*vec4(vVertex.xyz,1); vUV = vVertex + vec3(0.5); }
- shader, use the camera position and the 3D texture coordinates to run a loop in the current viewing direction. The loop is terminated if the current sample position is outside the volume or the alpha value of the accumulated color is saturated.
vec3 dataPos = vUV; vec3 geomDir = normalize((vUV-vec3(0.5)) - camPos); vec3 dirStep = geomDir * step_size; bool stop = false; for (int i = 0; i < MAX_SAMPLES; i++) { // advance ray by step dataPos = dataPos + dirStep; // stop condition stop=dot(sign(dataPos-texMin),sign(texMax-dataPos)) < 3.0; if (stop) break;
- For isosurface estimation, we take two sample values to find the zero crossing of the isofunction inside the volume dataset. If there is a zero crossing, we find the exact intersection point using bisection based refinement. Finally, we use the Phong illumination model to shade the isosurface assuming that the light is located at the camera position.
float sample=texture(volume, dataPos).r; float sample2=texture(volume, dataPos+dirStep).r; if( (sample -isoValue) < 0 && (sample2-isoValue) >= 0.0){ vec3 xN = dataPos; vec3 xF = dataPos+dirStep; vec3 tc = Bisection(xN, xF, isoValue); vec3 N = GetGradient(tc); vec3 V = -geomDir; vec3 L = V; vFragColor = PhongLighting(L,N,V,250, vec3(0.5)); break; } }
The Bisection
function is defined as follows:
The Bisection
function takes the two samples between which the given sample value lies. It then runs a loop. In each step, it calculates the midpoint of the two sample points and checks the density value at the midpoint to the given isovalue. If it is less, the left sample point is swapped with the mid position otherwise, the right sample point is swapped. This helps to reduce the search space quickly. The process is repeated and finally, the midpoint between the left sample point and right sample point is returned. The Gradient
function estimates the gradient of the volume density using center finite difference approximation.
While bulk of the code is similar to the single-pass GPU ray casting recipe. There is a major difference in the ray marching loop. In case of isosurface rendering, we do not use compositing. Instead, we find the zero crossing of the volume dataset isofunction by sampling two consecutive samples. This is well illustrated with the following diagram. If there is a zero crossing, we refine the detected isosurface by using bisection-based refinement.
samples. This is well illustrated with the following diagram. If there is a zero crossing, we refine the detected isosurface by using bisection-based refinement.
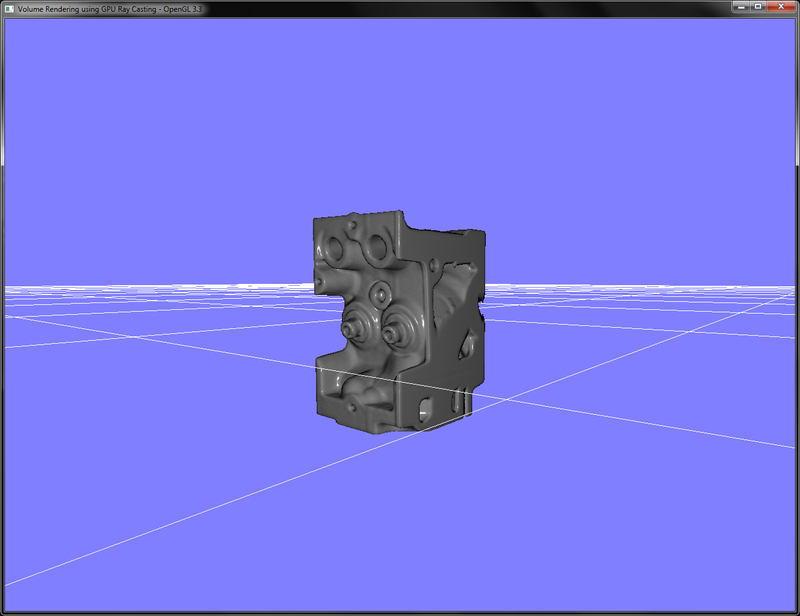
In this recipe, we will implement splatting on the GPU. The splatting algorithm converts the voxel representation into splats by convolving them with a Gaussian kernel. The Gaussian smoothing kernel reduces high frequencies and smoothes out edges giving a smoothed rendered output.
Let us start this recipe by following these simple steps:
- Load the 3D volume data and store it into an array.
std::ifstream infile(filename.c_str(), std::ios_base::binary); if(infile.good()) { pVolume = new GLubyte[XDIM*YDIM*ZDIM]; infile.read(reinterpret_cast<char*>(pVolume), XDIM*YDIM*ZDIM*sizeof(GLubyte)); infile.close(); return true; } else { return false; }
- Depending on the sampling box size, run three loops to iterate through the entire volume voxel by voxel.
vertices.clear(); int dx = XDIM/X_SAMPLING_DIST; int dy = YDIM/Y_SAMPLING_DIST; int dz = ZDIM/Z_SAMPLING_DIST; scale = glm::vec3(dx,dy,dz); for(int z=0;z<ZDIM;z+=dz) { for(int y=0;y<YDIM;y+=dy) { for(int x=0;x<XDIM;x+=dx) { SampleVoxel(x,y,z); } } }
The
SampleVoxel
function is defined in theVolumeSplatter
class as follows: - In each sampling step, estimate the volume density values at the current voxel. If the value is greater than the given isovalue, store the voxel position and normal into a vertex array.
GLubyte data = SampleVolume(x, y, z); if(data>isoValue) { Vertex v; v.pos.x = x; v.pos.y = y; v.pos.z = z; v.normal = GetNormal(x, y, z); v.pos *= invDim; vertices.push_back(v); }
The
SampleVolume
function takes the given sampling point and returns the nearest voxel density. It is defined in theVolumeSplatter
class as follows:GLubyte VolumeSplatter::SampleVolume(const int x, const int y, const int z) { int index = (x+(y*XDIM)) + z*(XDIM*YDIM); if(index<0) index = 0; if(index >= XDIM*YDIM*ZDIM) index = (XDIM*YDIM*ZDIM)-1; return pVolume[index]; }
- After the sampling step, pass the generated vertices to a vertex array object (VAO) containing a vertex buffer object (VBO).
glGenVertexArrays(1, &volumeSplatterVAO); glGenBuffers(1, &volumeSplatterVBO); glBindVertexArray(volumeSplatterVAO); glBindBuffer (GL_ARRAY_BUFFER, volumeSplatterVBO); glBufferData (GL_ARRAY_BUFFER, splatter->GetTotalVertices() *sizeof(Vertex), splatter->GetVertexPointer(), GL_STATIC_DRAW); glEnableVertexAttribArray(0); glVertexAttribPointer(0, 3, GL_FLOAT, GL_FALSE,sizeof(Vertex), 0); glEnableVertexAttribArray(1); glVertexAttribPointer(1, 3, GL_FLOAT, GL_FALSE,sizeof(Vertex), (const GLvoid*) offsetof(Vertex, normal));
- Set up two FBOs for offscreen rendering. The first FBO (
filterFBOID
) is used for Gaussian smoothing.glGenFramebuffers(1,&filterFBOID); glBindFramebuffer(GL_FRAMEBUFFER,filterFBOID); glGenTextures(2, blurTexID); for(int i=0;i<2;i++) { glActiveTexture(GL_TEXTURE1+i); glBindTexture(GL_TEXTURE_2D, blurTexID[i]); //set texture parameters glTexImage2D(GL_TEXTURE_2D,0,GL_RGBA32F,IMAGE_WIDTH, IMAGE_HEIGHT,0,GL_RGBA,GL_FLOAT,NULL); glFramebufferTexture2D(GL_FRAMEBUFFER, GL_COLOR_ATTACHMENT0+i,GL_TEXTURE_2D,blurTexID[i],0); } GLenum status = glCheckFramebufferStatus(GL_FRAMEBUFFER); if(status == GL_FRAMEBUFFER_COMPLETE) { cout<<"Filtering FBO setup successful."<<endl; } else { cout<<"Problem in Filtering FBO setup."<<endl; }
- The second FBO (
fboID
) is used to render the scene so that the smoothing operation can be applied on the rendered output from the first pass. Add a render buffer object to this FBO to enable depth testing.glGenFramebuffers(1,&fboID); glGenRenderbuffers(1, &rboID); glGenTextures(1, &texID); glBindFramebuffer(GL_FRAMEBUFFER,fboID); glBindRenderbuffer(GL_RENDERBUFFER, rboID); glActiveTexture(GL_TEXTURE0); glBindTexture(GL_TEXTURE_2D, texID); //set texture parameters glTexImage2D(GL_TEXTURE_2D,0,GL_RGBA32F,IMAGE_WIDTH, IMAGE_HEIGHT,0,GL_RGBA,GL_FLOAT,NULL); glFramebufferTexture2D(GL_FRAMEBUFFER,GL_COLOR_ATTACHMENT0, GL_TEXTURE_2D, texID, 0); glFramebufferRenderbuffer(GL_FRAMEBUFFER, GL_DEPTH_ATTACHMENT, GL_RENDERBUFFER, rboID); glRenderbufferStorage(GL_RENDERBUFFER, GL_DEPTH_COMPONENT32, IMAGE_WIDTH, IMAGE_HEIGHT); status = glCheckFramebufferStatus(GL_FRAMEBUFFER); if(status == GL_FRAMEBUFFER_COMPLETE) { cout<<"Offscreen rendering FBO setup successful."<<endl; } else { cout<<"Problem in offscreen rendering FBO setup."<<endl; }
- In the render function, first render the point splats to a texture using the first FBO (
fboID
).glBindFramebuffer(GL_FRAMEBUFFER,fboID); glViewport(0,0, IMAGE_WIDTH, IMAGE_HEIGHT); glDrawBuffer(GL_COLOR_ATTACHMENT0); glClear(GL_COLOR_BUFFER_BIT|GL_DEPTH_BUFFER_BIT); glm::mat4 T = glm::translate(glm::mat4(1), glm::vec3(-0.5,-0.5,-0.5)); glBindVertexArray(volumeSplatterVAO); shader.Use(); glUniformMatrix4fv(shader("MV"), 1, GL_FALSE, glm::value_ptr(MV*T)); glUniformMatrix3fv(shader("N"), 1, GL_FALSE, glm::value_ptr(glm::inverseTranspose(glm::mat3(MV*T)))); glUniformMatrix4fv(shader("P"), 1, GL_FALSE, glm::value_ptr(P)); glDrawArrays(GL_POINTS, 0, splatter->GetTotalVertices()); shader.UnUse();
The splatting vertex shader (
Chapter7/Splatting/shaders/splatShader.vert
) is defined as follows. It calculates the eye space normal. The splat size is calculated using the volume dimension and the sampling voxel size. This is then written to thegl_PointSize
variable in the vertex shader.The splatting fragment shader (
Chapter7/Splatting/shaders/splatShader.frag
) is defined as follows: - Next, set the filtering FBO and first apply the vertical and then the horizontal Gaussian smoothing pass by drawing a full-screen quad as was done in the Variance shadow mapping recipe in Chapter 4, Lights and Shadows.
glBindVertexArray(quadVAOID); glBindFramebuffer(GL_FRAMEBUFFER, filterFBOID); glDrawBuffer(GL_COLOR_ATTACHMENT0); gaussianV_shader.Use(); glDrawElements(GL_TRIANGLES, 6, GL_UNSIGNED_SHORT, 0); glDrawBuffer(GL_COLOR_ATTACHMENT1); gaussianH_shader.Use(); glDrawElements(GL_TRIANGLES, 6, GL_UNSIGNED_SHORT, 0);
- Unbind the filtering FBO, restore the default draw buffer and render the filtered output on the screen.
glBindFramebuffer(GL_FRAMEBUFFER,0); glDrawBuffer(GL_BACK_LEFT); glViewport(0,0,WIDTH, HEIGHT); quadShader.Use(); glDrawElements(GL_TRIANGLES, 6, GL_UNSIGNED_SHORT, 0); quadShader.UnUse(); glBindVertexArray(0);
Splatting algorithm works by rendering the voxels of the volume data as Gaussian blobs and projecting them on the screen. To achieve this, we first estimate the candidate voxels from the volume dataset by traversing through the entire volume dataset voxel by voxel for the given isovalue. If we have the appropriate voxel, we store its normal and position into a vertex array. For convenience, we wrap all of this functionality into the VolumeSplatter
class.
Finally, we estimate the diffuse and specular components and output the current fragment color using the eye space normal of the splat.
This recipe gave us an overview on the splatting algorithm. Our brute force approach in this recipe was to iterate through all of the voxels. For large datasets, we have to employ an acceleration structure, like an octree, to quickly identify voxels with densities and cull unnecessary voxels.
- The Qsplat project: http://graphics.stanford.edu/software/qsplat/
- Splatting research at ETH Zurich: (http://graphics.ethz.ch/research/past_projects/surfels/surfacesplatting/)
Chapter7/Splatting
directory.
Let us start this recipe by following these simple steps:
- Load the 3D volume data and store it into an array.
std::ifstream infile(filename.c_str(), std::ios_base::binary); if(infile.good()) { pVolume = new GLubyte[XDIM*YDIM*ZDIM]; infile.read(reinterpret_cast<char*>(pVolume), XDIM*YDIM*ZDIM*sizeof(GLubyte)); infile.close(); return true; } else { return false; }
- Depending on the sampling box size, run three loops to iterate through the entire volume voxel by voxel.
vertices.clear(); int dx = XDIM/X_SAMPLING_DIST; int dy = YDIM/Y_SAMPLING_DIST; int dz = ZDIM/Z_SAMPLING_DIST; scale = glm::vec3(dx,dy,dz); for(int z=0;z<ZDIM;z+=dz) { for(int y=0;y<YDIM;y+=dy) { for(int x=0;x<XDIM;x+=dx) { SampleVoxel(x,y,z); } } }
The
SampleVoxel
function is defined in theVolumeSplatter
class as follows: - In each sampling step, estimate the volume density values at the current voxel. If the value is greater than the given isovalue, store the voxel position and normal into a vertex array.
GLubyte data = SampleVolume(x, y, z); if(data>isoValue) { Vertex v; v.pos.x = x; v.pos.y = y; v.pos.z = z; v.normal = GetNormal(x, y, z); v.pos *= invDim; vertices.push_back(v); }
The
SampleVolume
function takes the given sampling point and returns the nearest voxel density. It is defined in theVolumeSplatter
class as follows:GLubyte VolumeSplatter::SampleVolume(const int x, const int y, const int z) { int index = (x+(y*XDIM)) + z*(XDIM*YDIM); if(index<0) index = 0; if(index >= XDIM*YDIM*ZDIM) index = (XDIM*YDIM*ZDIM)-1; return pVolume[index]; }
- After the sampling step, pass the generated vertices to a vertex array object (VAO) containing a vertex buffer object (VBO).
glGenVertexArrays(1, &volumeSplatterVAO); glGenBuffers(1, &volumeSplatterVBO); glBindVertexArray(volumeSplatterVAO); glBindBuffer (GL_ARRAY_BUFFER, volumeSplatterVBO); glBufferData (GL_ARRAY_BUFFER, splatter->GetTotalVertices() *sizeof(Vertex), splatter->GetVertexPointer(), GL_STATIC_DRAW); glEnableVertexAttribArray(0); glVertexAttribPointer(0, 3, GL_FLOAT, GL_FALSE,sizeof(Vertex), 0); glEnableVertexAttribArray(1); glVertexAttribPointer(1, 3, GL_FLOAT, GL_FALSE,sizeof(Vertex), (const GLvoid*) offsetof(Vertex, normal));
- Set up two FBOs for offscreen rendering. The first FBO (
filterFBOID
) is used for Gaussian smoothing.glGenFramebuffers(1,&filterFBOID); glBindFramebuffer(GL_FRAMEBUFFER,filterFBOID); glGenTextures(2, blurTexID); for(int i=0;i<2;i++) { glActiveTexture(GL_TEXTURE1+i); glBindTexture(GL_TEXTURE_2D, blurTexID[i]); //set texture parameters glTexImage2D(GL_TEXTURE_2D,0,GL_RGBA32F,IMAGE_WIDTH, IMAGE_HEIGHT,0,GL_RGBA,GL_FLOAT,NULL); glFramebufferTexture2D(GL_FRAMEBUFFER, GL_COLOR_ATTACHMENT0+i,GL_TEXTURE_2D,blurTexID[i],0); } GLenum status = glCheckFramebufferStatus(GL_FRAMEBUFFER); if(status == GL_FRAMEBUFFER_COMPLETE) { cout<<"Filtering FBO setup successful."<<endl; } else { cout<<"Problem in Filtering FBO setup."<<endl; }
- The second FBO (
fboID
) is used to render the scene so that the smoothing operation can be applied on the rendered output from the first pass. Add a render buffer object to this FBO to enable depth testing.glGenFramebuffers(1,&fboID); glGenRenderbuffers(1, &rboID); glGenTextures(1, &texID); glBindFramebuffer(GL_FRAMEBUFFER,fboID); glBindRenderbuffer(GL_RENDERBUFFER, rboID); glActiveTexture(GL_TEXTURE0); glBindTexture(GL_TEXTURE_2D, texID); //set texture parameters glTexImage2D(GL_TEXTURE_2D,0,GL_RGBA32F,IMAGE_WIDTH, IMAGE_HEIGHT,0,GL_RGBA,GL_FLOAT,NULL); glFramebufferTexture2D(GL_FRAMEBUFFER,GL_COLOR_ATTACHMENT0, GL_TEXTURE_2D, texID, 0); glFramebufferRenderbuffer(GL_FRAMEBUFFER, GL_DEPTH_ATTACHMENT, GL_RENDERBUFFER, rboID); glRenderbufferStorage(GL_RENDERBUFFER, GL_DEPTH_COMPONENT32, IMAGE_WIDTH, IMAGE_HEIGHT); status = glCheckFramebufferStatus(GL_FRAMEBUFFER); if(status == GL_FRAMEBUFFER_COMPLETE) { cout<<"Offscreen rendering FBO setup successful."<<endl; } else { cout<<"Problem in offscreen rendering FBO setup."<<endl; }
- In the render function, first render the point splats to a texture using the first FBO (
fboID
).glBindFramebuffer(GL_FRAMEBUFFER,fboID); glViewport(0,0, IMAGE_WIDTH, IMAGE_HEIGHT); glDrawBuffer(GL_COLOR_ATTACHMENT0); glClear(GL_COLOR_BUFFER_BIT|GL_DEPTH_BUFFER_BIT); glm::mat4 T = glm::translate(glm::mat4(1), glm::vec3(-0.5,-0.5,-0.5)); glBindVertexArray(volumeSplatterVAO); shader.Use(); glUniformMatrix4fv(shader("MV"), 1, GL_FALSE, glm::value_ptr(MV*T)); glUniformMatrix3fv(shader("N"), 1, GL_FALSE, glm::value_ptr(glm::inverseTranspose(glm::mat3(MV*T)))); glUniformMatrix4fv(shader("P"), 1, GL_FALSE, glm::value_ptr(P)); glDrawArrays(GL_POINTS, 0, splatter->GetTotalVertices()); shader.UnUse();
The splatting vertex shader (
Chapter7/Splatting/shaders/splatShader.vert
) is defined as follows. It calculates the eye space normal. The splat size is calculated using the volume dimension and the sampling voxel size. This is then written to thegl_PointSize
variable in the vertex shader.The splatting fragment shader (
Chapter7/Splatting/shaders/splatShader.frag
) is defined as follows: - Next, set the filtering FBO and first apply the vertical and then the horizontal Gaussian smoothing pass by drawing a full-screen quad as was done in the Variance shadow mapping recipe in Chapter 4, Lights and Shadows.
glBindVertexArray(quadVAOID); glBindFramebuffer(GL_FRAMEBUFFER, filterFBOID); glDrawBuffer(GL_COLOR_ATTACHMENT0); gaussianV_shader.Use(); glDrawElements(GL_TRIANGLES, 6, GL_UNSIGNED_SHORT, 0); glDrawBuffer(GL_COLOR_ATTACHMENT1); gaussianH_shader.Use(); glDrawElements(GL_TRIANGLES, 6, GL_UNSIGNED_SHORT, 0);
- Unbind the filtering FBO, restore the default draw buffer and render the filtered output on the screen.
glBindFramebuffer(GL_FRAMEBUFFER,0); glDrawBuffer(GL_BACK_LEFT); glViewport(0,0,WIDTH, HEIGHT); quadShader.Use(); glDrawElements(GL_TRIANGLES, 6, GL_UNSIGNED_SHORT, 0); quadShader.UnUse(); glBindVertexArray(0);
Splatting algorithm works by rendering the voxels of the volume data as Gaussian blobs and projecting them on the screen. To achieve this, we first estimate the candidate voxels from the volume dataset by traversing through the entire volume dataset voxel by voxel for the given isovalue. If we have the appropriate voxel, we store its normal and position into a vertex array. For convenience, we wrap all of this functionality into the VolumeSplatter
class.
Finally, we estimate the diffuse and specular components and output the current fragment color using the eye space normal of the splat.
This recipe gave us an overview on the splatting algorithm. Our brute force approach in this recipe was to iterate through all of the voxels. For large datasets, we have to employ an acceleration structure, like an octree, to quickly identify voxels with densities and cull unnecessary voxels.
- The Qsplat project: http://graphics.stanford.edu/software/qsplat/
- Splatting research at ETH Zurich: (http://graphics.ethz.ch/research/past_projects/surfels/surfacesplatting/)
std::ifstream infile(filename.c_str(), std::ios_base::binary); if(infile.good()) { pVolume = new GLubyte[XDIM*YDIM*ZDIM]; infile.read(reinterpret_cast<char*>(pVolume), XDIM*YDIM*ZDIM*sizeof(GLubyte)); infile.close(); return true; } else { return false; }
vertices.clear(); int dx = XDIM/X_SAMPLING_DIST; int dy = YDIM/Y_SAMPLING_DIST; int dz = ZDIM/Z_SAMPLING_DIST; scale = glm::vec3(dx,dy,dz); for(int z=0;z<ZDIM;z+=dz) { for(int y=0;y<YDIM;y+=dy) { for(int x=0;x<XDIM;x+=dx) { SampleVoxel(x,y,z); } } }
SampleVoxel
function
- In each sampling step, estimate the volume density values at the current voxel. If the value is greater than the given isovalue, store the voxel position and normal into a vertex array.
GLubyte data = SampleVolume(x, y, z); if(data>isoValue) { Vertex v; v.pos.x = x; v.pos.y = y; v.pos.z = z; v.normal = GetNormal(x, y, z); v.pos *= invDim; vertices.push_back(v); }
The
SampleVolume
function takes the given sampling point and returns the nearest voxel density. It is defined in theVolumeSplatter
class as follows:GLubyte VolumeSplatter::SampleVolume(const int x, const int y, const int z) { int index = (x+(y*XDIM)) + z*(XDIM*YDIM); if(index<0) index = 0; if(index >= XDIM*YDIM*ZDIM) index = (XDIM*YDIM*ZDIM)-1; return pVolume[index]; }
- After the sampling step, pass the generated vertices to a vertex array object (VAO) containing a vertex buffer object (VBO).
glGenVertexArrays(1, &volumeSplatterVAO); glGenBuffers(1, &volumeSplatterVBO); glBindVertexArray(volumeSplatterVAO); glBindBuffer (GL_ARRAY_BUFFER, volumeSplatterVBO); glBufferData (GL_ARRAY_BUFFER, splatter->GetTotalVertices() *sizeof(Vertex), splatter->GetVertexPointer(), GL_STATIC_DRAW); glEnableVertexAttribArray(0); glVertexAttribPointer(0, 3, GL_FLOAT, GL_FALSE,sizeof(Vertex), 0); glEnableVertexAttribArray(1); glVertexAttribPointer(1, 3, GL_FLOAT, GL_FALSE,sizeof(Vertex), (const GLvoid*) offsetof(Vertex, normal));
- Set up two FBOs for offscreen rendering. The first FBO (
filterFBOID
) is used for Gaussian smoothing.glGenFramebuffers(1,&filterFBOID); glBindFramebuffer(GL_FRAMEBUFFER,filterFBOID); glGenTextures(2, blurTexID); for(int i=0;i<2;i++) { glActiveTexture(GL_TEXTURE1+i); glBindTexture(GL_TEXTURE_2D, blurTexID[i]); //set texture parameters glTexImage2D(GL_TEXTURE_2D,0,GL_RGBA32F,IMAGE_WIDTH, IMAGE_HEIGHT,0,GL_RGBA,GL_FLOAT,NULL); glFramebufferTexture2D(GL_FRAMEBUFFER, GL_COLOR_ATTACHMENT0+i,GL_TEXTURE_2D,blurTexID[i],0); } GLenum status = glCheckFramebufferStatus(GL_FRAMEBUFFER); if(status == GL_FRAMEBUFFER_COMPLETE) { cout<<"Filtering FBO setup successful."<<endl; } else { cout<<"Problem in Filtering FBO setup."<<endl; }
- The second FBO (
fboID
) is used to render the scene so that the smoothing operation can be applied on the rendered output from the first pass. Add a render buffer object to this FBO to enable depth testing.glGenFramebuffers(1,&fboID); glGenRenderbuffers(1, &rboID); glGenTextures(1, &texID); glBindFramebuffer(GL_FRAMEBUFFER,fboID); glBindRenderbuffer(GL_RENDERBUFFER, rboID); glActiveTexture(GL_TEXTURE0); glBindTexture(GL_TEXTURE_2D, texID); //set texture parameters glTexImage2D(GL_TEXTURE_2D,0,GL_RGBA32F,IMAGE_WIDTH, IMAGE_HEIGHT,0,GL_RGBA,GL_FLOAT,NULL); glFramebufferTexture2D(GL_FRAMEBUFFER,GL_COLOR_ATTACHMENT0, GL_TEXTURE_2D, texID, 0); glFramebufferRenderbuffer(GL_FRAMEBUFFER, GL_DEPTH_ATTACHMENT, GL_RENDERBUFFER, rboID); glRenderbufferStorage(GL_RENDERBUFFER, GL_DEPTH_COMPONENT32, IMAGE_WIDTH, IMAGE_HEIGHT); status = glCheckFramebufferStatus(GL_FRAMEBUFFER); if(status == GL_FRAMEBUFFER_COMPLETE) { cout<<"Offscreen rendering FBO setup successful."<<endl; } else { cout<<"Problem in offscreen rendering FBO setup."<<endl; }
- In the render function, first render the point splats to a texture using the first FBO (
fboID
).glBindFramebuffer(GL_FRAMEBUFFER,fboID); glViewport(0,0, IMAGE_WIDTH, IMAGE_HEIGHT); glDrawBuffer(GL_COLOR_ATTACHMENT0); glClear(GL_COLOR_BUFFER_BIT|GL_DEPTH_BUFFER_BIT); glm::mat4 T = glm::translate(glm::mat4(1), glm::vec3(-0.5,-0.5,-0.5)); glBindVertexArray(volumeSplatterVAO); shader.Use(); glUniformMatrix4fv(shader("MV"), 1, GL_FALSE, glm::value_ptr(MV*T)); glUniformMatrix3fv(shader("N"), 1, GL_FALSE, glm::value_ptr(glm::inverseTranspose(glm::mat3(MV*T)))); glUniformMatrix4fv(shader("P"), 1, GL_FALSE, glm::value_ptr(P)); glDrawArrays(GL_POINTS, 0, splatter->GetTotalVertices()); shader.UnUse();
The splatting vertex shader (
Chapter7/Splatting/shaders/splatShader.vert
) is defined as follows. It calculates the eye space normal. The splat size is calculated using the volume dimension and the sampling voxel size. This is then written to thegl_PointSize
variable in the vertex shader.The splatting fragment shader (
Chapter7/Splatting/shaders/splatShader.frag
) is defined as follows: - Next, set the filtering FBO and first apply the vertical and then the horizontal Gaussian smoothing pass by drawing a full-screen quad as was done in the Variance shadow mapping recipe in Chapter 4, Lights and Shadows.
glBindVertexArray(quadVAOID); glBindFramebuffer(GL_FRAMEBUFFER, filterFBOID); glDrawBuffer(GL_COLOR_ATTACHMENT0); gaussianV_shader.Use(); glDrawElements(GL_TRIANGLES, 6, GL_UNSIGNED_SHORT, 0); glDrawBuffer(GL_COLOR_ATTACHMENT1); gaussianH_shader.Use(); glDrawElements(GL_TRIANGLES, 6, GL_UNSIGNED_SHORT, 0);
- Unbind the filtering FBO, restore the default draw buffer and render the filtered output on the screen.
glBindFramebuffer(GL_FRAMEBUFFER,0); glDrawBuffer(GL_BACK_LEFT); glViewport(0,0,WIDTH, HEIGHT); quadShader.Use(); glDrawElements(GL_TRIANGLES, 6, GL_UNSIGNED_SHORT, 0); quadShader.UnUse(); glBindVertexArray(0);
Splatting algorithm works by rendering the voxels of the volume data as Gaussian blobs and projecting them on the screen. To achieve this, we first estimate the candidate voxels from the volume dataset by traversing through the entire volume dataset voxel by voxel for the given isovalue. If we have the appropriate voxel, we store its normal and position into a vertex array. For convenience, we wrap all of this functionality into the VolumeSplatter
class.
Finally, we estimate the diffuse and specular components and output the current fragment color using the eye space normal of the splat.
This recipe gave us an overview on the splatting algorithm. Our brute force approach in this recipe was to iterate through all of the voxels. For large datasets, we have to employ an acceleration structure, like an octree, to quickly identify voxels with densities and cull unnecessary voxels.
- The Qsplat project: http://graphics.stanford.edu/software/qsplat/
- Splatting research at ETH Zurich: (http://graphics.ethz.ch/research/past_projects/surfels/surfacesplatting/)
algorithm works by rendering the voxels of the volume data as Gaussian blobs and projecting them on the screen. To achieve this, we first estimate the candidate voxels from the volume dataset by traversing through the entire volume dataset voxel by voxel for the given isovalue. If we have the appropriate voxel, we store its normal and position into a vertex array. For convenience, we wrap all of this functionality into the VolumeSplatter
class.
Finally, we estimate the diffuse and specular components and output the current fragment color using the eye space normal of the splat.
This recipe gave us an overview on the splatting algorithm. Our brute force approach in this recipe was to iterate through all of the voxels. For large datasets, we have to employ an acceleration structure, like an octree, to quickly identify voxels with densities and cull unnecessary voxels.
- The Qsplat project: http://graphics.stanford.edu/software/qsplat/
- Splatting research at ETH Zurich: (http://graphics.ethz.ch/research/past_projects/surfels/surfacesplatting/)
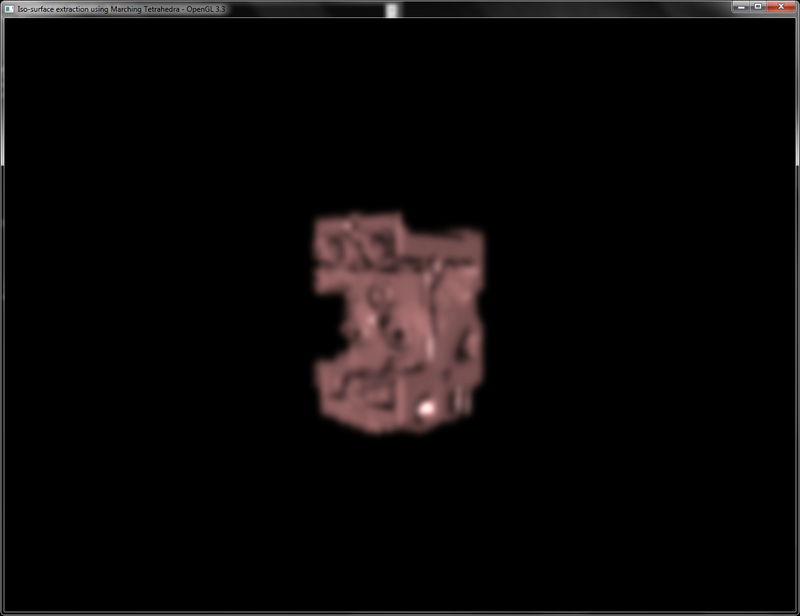
acceleration structure, like an octree, to quickly identify voxels with densities and cull unnecessary voxels.
- The Qsplat project: http://graphics.stanford.edu/software/qsplat/
- Splatting research at ETH Zurich: (http://graphics.ethz.ch/research/past_projects/surfels/surfacesplatting/)
- http://graphics.stanford.edu/software/qsplat/
- Splatting research at ETH Zurich: (http://graphics.ethz.ch/research/past_projects/surfels/surfacesplatting/)
In this recipe, we will implement classification to the 3D texture slicing presented before. We will generate a lookup table to add specific colors to specific densities. This is accomplished by generating a 1D texture that is looked up in the fragment shader with the current volume density. The returned color is then used as the color of the current fragment. Apart from the setup of the transfer function data, all the other content remains the same as in the 3D texture slicing recipe. Note that the classification method is not limited to 3D texture slicing, it can be applied to any volume rendering algorithm.
Let us start this recipe by following these simple steps:
- Load the volume data and setup the texture slicing as in the Implementing volume rendering using 3D texture slicing recipe.
- Create a 1D texture that will be our transfer function texture for color lookup. We create a set of color values and then interpolate them on the fly. Refer to
LoadTransferFunction
inChapter7/3DTextureSlicingClassification/main.cpp
.float pData[256][4]; int indices[9]; for(int i=0;i<9;i++) { int index = i*28; pData[index][0] = jet_values[i].x; pData[index][1] = jet_values[i].y; pData[index][2] = jet_values[i].z; pData[index][3] = jet_values[i].w; indices[i] = index; } for(int j=0;j<9-1;j++) { float dDataR = (pData[indices[j+1]][0] - pData[indices[j]][0]); float dDataG = (pData[indices[j+1]][1] - pData[indices[j]][1]); float dDataB = (pData[indices[j+1]][2] - pData[indices[j]][2]); float dDataA = (pData[indices[j+1]][3] - pData[indices[j]][3]); int dIndex = indices[j+1]-indices[j]; float dDataIncR = dDataR/float(dIndex); float dDataIncG = dDataG/float(dIndex); float dDataIncB = dDataB/float(dIndex); float dDataIncA = dDataA/float(dIndex); for(int i=indices[j]+1;i<indices[j+1];i++) { pData[i][0] = (pData[i-1][0] + dDataIncR); pData[i][1] = (pData[i-1][1] + dDataIncG); pData[i][2] = (pData[i-1][2] + dDataIncB); pData[i][3] = (pData[i-1][3] + dDataIncA); } }
- Generate a 1D OpenGL texture from the interpolated lookup data from step 1. We bind this texture to texture unit 1 (
GL_TEXTURE1
);glGenTextures(1, &tfTexID); glActiveTexture(GL_TEXTURE1); glBindTexture(GL_TEXTURE_1D, tfTexID); glTexParameteri(GL_TEXTURE_1D, GL_TEXTURE_WRAP_S, GL_REPEAT); glTexParameteri(GL_TEXTURE_1D, GL_TEXTURE_MAG_FILTER, GL_LINEAR); glTexParameteri(GL_TEXTURE_1D, GL_TEXTURE_MIN_FILTER, GL_LINEAR); glTexImage1D(GL_TEXTURE_1D,0,GL_RGBA,256,0,GL_RGBA,GL_FLOAT,pData);
- In the fragment shader, add a new sampler for the transfer function lookup table. Since we now have two textures, we bind the volume data to texture unit 0 (
GL_TEXTURE0
) and the transfer function texture to texture unit 1 (GL_TEXTURE1
).shader.LoadFromFile(GL_VERTEX_SHADER, "shaders/textureSlicer.vert"); shader.LoadFromFile(GL_FRAGMENT_SHADER, "shaders/textureSlicer.frag"); shader.CreateAndLinkProgram(); shader.Use(); shader.AddAttribute("vVertex"); shader.AddUniform("MVP"); shader.AddUniform("volume"); shader.AddUniform("lut"); glUniform1i(shader("volume"),0); glUniform1i(shader("lut"),1); shader.UnUse();
- Finally, in the fragment shader, instead of directly returning the current volume density value, we lookup the density value in the transfer function and return the appropriate color value. Refer to
Chapter7/3DTextureSlicingClassification/shaders/textureSlicer.frag
for details.uniform sampler3D volume; uniform sampler1D lut; void main(void) { vFragColor = texture(lut, texture(volume, vUV).r); }
There are two parts of this recipe: the generation of the transfer function texture and the lookup of this texture in the fragment shader. Both of these steps are relatively straightforward to understand. For generation of the transfer function texture, we first create a simple array of possible colors called jet_values
, which is defined globally as follows:
Chapter7/3DTextureSlicingClassification
directory.
Let us start this recipe by following these simple steps:
- Load the volume data and setup the texture slicing as in the Implementing volume rendering using 3D texture slicing recipe.
- Create a 1D texture that will be our transfer function texture for color lookup. We create a set of color values and then interpolate them on the fly. Refer to
LoadTransferFunction
inChapter7/3DTextureSlicingClassification/main.cpp
.float pData[256][4]; int indices[9]; for(int i=0;i<9;i++) { int index = i*28; pData[index][0] = jet_values[i].x; pData[index][1] = jet_values[i].y; pData[index][2] = jet_values[i].z; pData[index][3] = jet_values[i].w; indices[i] = index; } for(int j=0;j<9-1;j++) { float dDataR = (pData[indices[j+1]][0] - pData[indices[j]][0]); float dDataG = (pData[indices[j+1]][1] - pData[indices[j]][1]); float dDataB = (pData[indices[j+1]][2] - pData[indices[j]][2]); float dDataA = (pData[indices[j+1]][3] - pData[indices[j]][3]); int dIndex = indices[j+1]-indices[j]; float dDataIncR = dDataR/float(dIndex); float dDataIncG = dDataG/float(dIndex); float dDataIncB = dDataB/float(dIndex); float dDataIncA = dDataA/float(dIndex); for(int i=indices[j]+1;i<indices[j+1];i++) { pData[i][0] = (pData[i-1][0] + dDataIncR); pData[i][1] = (pData[i-1][1] + dDataIncG); pData[i][2] = (pData[i-1][2] + dDataIncB); pData[i][3] = (pData[i-1][3] + dDataIncA); } }
- Generate a 1D OpenGL texture from the interpolated lookup data from step 1. We bind this texture to texture unit 1 (
GL_TEXTURE1
);glGenTextures(1, &tfTexID); glActiveTexture(GL_TEXTURE1); glBindTexture(GL_TEXTURE_1D, tfTexID); glTexParameteri(GL_TEXTURE_1D, GL_TEXTURE_WRAP_S, GL_REPEAT); glTexParameteri(GL_TEXTURE_1D, GL_TEXTURE_MAG_FILTER, GL_LINEAR); glTexParameteri(GL_TEXTURE_1D, GL_TEXTURE_MIN_FILTER, GL_LINEAR); glTexImage1D(GL_TEXTURE_1D,0,GL_RGBA,256,0,GL_RGBA,GL_FLOAT,pData);
- In the fragment shader, add a new sampler for the transfer function lookup table. Since we now have two textures, we bind the volume data to texture unit 0 (
GL_TEXTURE0
) and the transfer function texture to texture unit 1 (GL_TEXTURE1
).shader.LoadFromFile(GL_VERTEX_SHADER, "shaders/textureSlicer.vert"); shader.LoadFromFile(GL_FRAGMENT_SHADER, "shaders/textureSlicer.frag"); shader.CreateAndLinkProgram(); shader.Use(); shader.AddAttribute("vVertex"); shader.AddUniform("MVP"); shader.AddUniform("volume"); shader.AddUniform("lut"); glUniform1i(shader("volume"),0); glUniform1i(shader("lut"),1); shader.UnUse();
- Finally, in the fragment shader, instead of directly returning the current volume density value, we lookup the density value in the transfer function and return the appropriate color value. Refer to
Chapter7/3DTextureSlicingClassification/shaders/textureSlicer.frag
for details.uniform sampler3D volume; uniform sampler1D lut; void main(void) { vFragColor = texture(lut, texture(volume, vUV).r); }
There are two parts of this recipe: the generation of the transfer function texture and the lookup of this texture in the fragment shader. Both of these steps are relatively straightforward to understand. For generation of the transfer function texture, we first create a simple array of possible colors called jet_values
, which is defined globally as follows:
recipe by following these simple steps:
- Load the volume data and setup the texture slicing as in the Implementing volume rendering using 3D texture slicing recipe.
- Create a 1D texture that will be our transfer function texture for color lookup. We create a set of color values and then interpolate them on the fly. Refer to
LoadTransferFunction
inChapter7/3DTextureSlicingClassification/main.cpp
.float pData[256][4]; int indices[9]; for(int i=0;i<9;i++) { int index = i*28; pData[index][0] = jet_values[i].x; pData[index][1] = jet_values[i].y; pData[index][2] = jet_values[i].z; pData[index][3] = jet_values[i].w; indices[i] = index; } for(int j=0;j<9-1;j++) { float dDataR = (pData[indices[j+1]][0] - pData[indices[j]][0]); float dDataG = (pData[indices[j+1]][1] - pData[indices[j]][1]); float dDataB = (pData[indices[j+1]][2] - pData[indices[j]][2]); float dDataA = (pData[indices[j+1]][3] - pData[indices[j]][3]); int dIndex = indices[j+1]-indices[j]; float dDataIncR = dDataR/float(dIndex); float dDataIncG = dDataG/float(dIndex); float dDataIncB = dDataB/float(dIndex); float dDataIncA = dDataA/float(dIndex); for(int i=indices[j]+1;i<indices[j+1];i++) { pData[i][0] = (pData[i-1][0] + dDataIncR); pData[i][1] = (pData[i-1][1] + dDataIncG); pData[i][2] = (pData[i-1][2] + dDataIncB); pData[i][3] = (pData[i-1][3] + dDataIncA); } }
- Generate a 1D OpenGL texture from the interpolated lookup data from step 1. We bind this texture to texture unit 1 (
GL_TEXTURE1
);glGenTextures(1, &tfTexID); glActiveTexture(GL_TEXTURE1); glBindTexture(GL_TEXTURE_1D, tfTexID); glTexParameteri(GL_TEXTURE_1D, GL_TEXTURE_WRAP_S, GL_REPEAT); glTexParameteri(GL_TEXTURE_1D, GL_TEXTURE_MAG_FILTER, GL_LINEAR); glTexParameteri(GL_TEXTURE_1D, GL_TEXTURE_MIN_FILTER, GL_LINEAR); glTexImage1D(GL_TEXTURE_1D,0,GL_RGBA,256,0,GL_RGBA,GL_FLOAT,pData);
- In the fragment shader, add a new sampler for the transfer function lookup table. Since we now have two textures, we bind the volume data to texture unit 0 (
GL_TEXTURE0
) and the transfer function texture to texture unit 1 (GL_TEXTURE1
).shader.LoadFromFile(GL_VERTEX_SHADER, "shaders/textureSlicer.vert"); shader.LoadFromFile(GL_FRAGMENT_SHADER, "shaders/textureSlicer.frag"); shader.CreateAndLinkProgram(); shader.Use(); shader.AddAttribute("vVertex"); shader.AddUniform("MVP"); shader.AddUniform("volume"); shader.AddUniform("lut"); glUniform1i(shader("volume"),0); glUniform1i(shader("lut"),1); shader.UnUse();
- Finally, in the fragment shader, instead of directly returning the current volume density value, we lookup the density value in the transfer function and return the appropriate color value. Refer to
Chapter7/3DTextureSlicingClassification/shaders/textureSlicer.frag
for details.uniform sampler3D volume; uniform sampler1D lut; void main(void) { vFragColor = texture(lut, texture(volume, vUV).r); }
There are two parts of this recipe: the generation of the transfer function texture and the lookup of this texture in the fragment shader. Both of these steps are relatively straightforward to understand. For generation of the transfer function texture, we first create a simple array of possible colors called jet_values
, which is defined globally as follows:
parts of this recipe: the generation of the transfer function texture and the lookup of this texture in the fragment shader. Both of these steps are relatively straightforward to understand. For generation of the transfer function texture, we first create a simple array of possible colors called jet_values
, which is defined globally as follows:
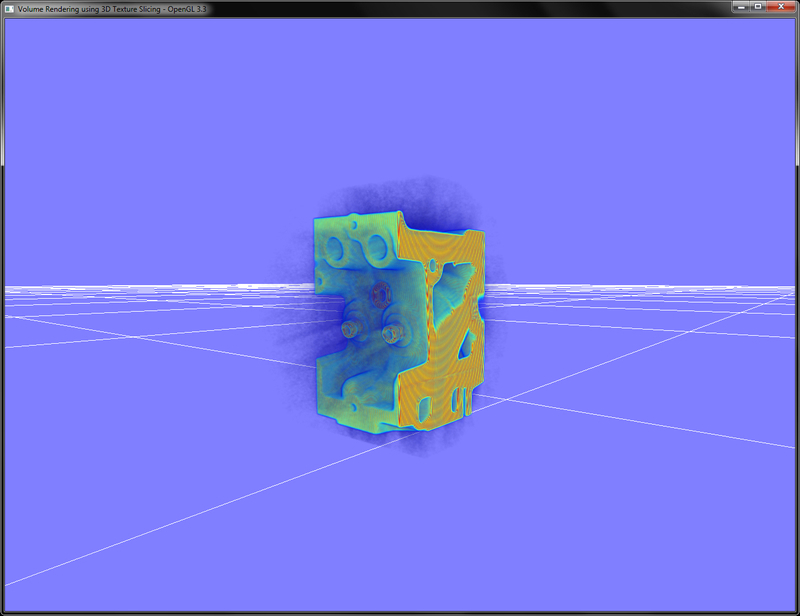
- Chapter 4, Transfer Functions, and Chapter 10, Transfer Functions Reloaded, in Real-time Volume Graphics, AK Peters/CRC Press.
In the Implementing pseudo-isosurface rendering in single-pass GPU ray casting recipe, we implemented pseudo-isosurface rendering in single-pass GPU ray casting. However, these isosurfaces are not composed of triangles; so it is not possible for us to uniquely address individual isosurface regions easily to mark different areas in the volume dataset. This can be achieved by doing an isosurface extraction process for a specific isovalue by traversing the entire volume dataset. This method is known as the Marching Tetrahedra (MT) algorithm. This algorithm traverses the whole volume dataset and tries to fit a specific polygon based on the intersection criterion. This process is repeated for the whole volume and finally, we obtain the polygonal mesh from the volume dataset.
Let us start this recipe by following these simple steps:
- Load the 3D volume data and store it into an array:
std::ifstream infile(filename.c_str(), std::ios_base::binary); if(infile.good()) { pVolume = new GLubyte[XDIM*YDIM*ZDIM]; infile.read(reinterpret_cast<char*>(pVolume), XDIM*YDIM*ZDIM*sizeof(GLubyte)); infile.close(); return true; } else { return false; }
- Depending on the sampling box size, run three loops to iterate through the entire volume voxel by voxel:
vertices.clear(); int dx = XDIM/X_SAMPLING_DIST; int dy = YDIM/Y_SAMPLING_DIST; int dz = ZDIM/Z_SAMPLING_DIST; glm::vec3 scale = glm::vec3(dx,dy,dz); for(int z=0;z<ZDIM;z+=dz) { for(int y=0;y<YDIM;y+=dy) { for(int x=0;x<XDIM;x+=dx) { SampleVoxel(x,y,z, scale); } } }
- In each sampling step, estimate the volume density values at the eight corners of the sampling box:
GLubyte cubeCornerValues[8]; for( i = 0; i < 8; i++) { cubeCornerValues[i] = SampleVolume( x + (int)(a2fVertexOffset[i][0] *scale.x), y + (int)(a2fVertexOffset[i][1]*scale.y), z + (int)(a2fVertexOffset[i][2]*scale.z)); }
- Estimate an edge flag value to identify the matching tetrahedra case based on the given isovalue:
int flagIndex = 0; for( i= 0; i<8; i++) { if(cubeCornerValues[i]<= isoValue) flagIndex |= 1<<i; } edgeFlags = aiCubeEdgeFlags[flagIndex];
- Use the lookup tables (
a2iEdgeConnection
) to find the correct edges for the case and then use the offset table (a2fVertexOffset
) to find the edge vertices and normals. These tables are defined in theTables.h
header in theChapter7/MarchingTetrahedra/
directory.for(i = 0; i < 12; i++) { if(edgeFlags & (1<<i)) { float offset = GetOffset(cubeCornerValues[ a2iEdgeConnection[i][0] ], cubeCornerValues[ a2iEdgeConnection[i][1] ]); edgeVertices[i].x = x + (a2fVertexOffset[ a2iEdgeConnection[i][0] ][0] + offset * a2fEdgeDirection[i][0])*scale.x ; edgeVertices[i].y = y + (a2fVertexOffset[ a2iEdgeConnection[i][0] ][1] + offset * a2fEdgeDirection[i][1])*scale.y ; edgeVertices[i].z = z + (a2fVertexOffset[ a2iEdgeConnection[i][0] ][2] + offset * a2fEdgeDirection[i][2])*scale.z ; edgeNormals[i] = GetNormal( (int)edgeVertices[i].x , (int)edgeVertices[i].y , (int)edgeVertices[i].z ); } }
- Finally, loop through the triangle connectivity table to connect the correct vertices and normals for the given case.
for(i = 0; i< 5; i++) { if(a2iTriangleConnectionTable[flagIndex][3*i] < 0) break; for(int j= 0; j< 3; j++) { int vertex = a2iTriangleConnectionTable[flagIndex][3*i+j]; Vertex v; v.normal = (edgeNormals[vertex]); v.pos = (edgeVertices[vertex])*invDim; vertices.push_back(v); } }
- After the marcher is finished, we pass the generated vertices to a vertex array object containing a vertex buffer object:
glGenVertexArrays(1, &volumeMarcherVAO); glGenBuffers(1, &volumeMarcherVBO); glBindVertexArray(volumeMarcherVAO); glBindBuffer (GL_ARRAY_BUFFER, volumeMarcherVBO); glBufferData (GL_ARRAY_BUFFER, marcher-> GetTotalVertices()*sizeof(Vertex), marcher-> GetVertexPointer(), GL_STATIC_DRAW); glEnableVertexAttribArray(0); glVertexAttribPointer(0, 3, GL_FLOAT, GL_FALSE,sizeof(Vertex),0); glEnableVertexAttribArray(1); glVertexAttribPointer(1, 3, GL_FLOAT, GL_FALSE,sizeof(Vertex),(const GLvoid*)offsetof(Vertex, normal));
- For rendering of the generated geometry, we bind the Marching Tetrahedra VAO, bind our shader and then render the triangles. For this recipe, we output the per-vertex normals as color.
glBindVertexArray(volumeMarcherVAO); shader.Use(); glUniformMatrix4fv(shader("MVP"),1,GL_FALSE, glm::value_ptr(MVP*T)); glDrawArrays(GL_TRIANGLES, 0, marcher->GetTotalVertices()); shader.UnUse();
For convenience, we wrap the entire recipe in a reusable class called TetrahedraMarcher
. Marching Tetrahedra, as the name suggests, marches a sampling box throughout the whole volume dataset. To give a bird's eye view there are several cases to consider based on the distribution of density values at the vertices of the sampling cube. Based on the sampling values at the eight corners and the given isovalue, a flag index is generated. This flag index gives us the edge flag by a lookup in a table. This edge flag is then used in an edge lookup table, which is predefined for all possible edge configurations of the marching tetrahedron. The edge connection table is then used to find the appropriate offset for the corner values of the sampling box. These offsets are then used to obtain the edge vertices and normals for the given tetrahedral case. Once the list of edge vertices and normals are estimated, the triangle connectivity is obtained based on the given flag index.
Now we will detail the steps in the Marching Tetrahedra algorithm. First, the flag index is obtained by iterating through all eight sampling cube vertices and comparing the density value at the vertex location with the given isovalue as shown in the following code. The flag index is then used to retrieve the edge flags from the looktup table (aiCubeEdgeFlags
).
After the Marching Tetrahedra code is processed, we store the generated vertices and normals in a buffer object. In the rendering code, we bind the appropriate vertex array object, bind our shader and then draw the triangles. The fragment shader for this recipe outputs the per-vertex normals as colors.
Pressing the W key toggles the wireframe display, which shows the underlying isosurface polygons for isovalue of 40 as shown in the following screenshot:
While in this recipe, we focused on the Marching Tetrahedra algorithm. There is another, more robust method of triangulation called Marching Cubes, which gives a more robust polygonisation as compared to the Marching Tetrahedra algorithm.
- Polygonising a scalar field, Paul Bourke: http://paulbourke.net/geometry/polygonise/
- Volume Rendering: Marching Cubes Algorithm, http://cns-alumni.bu.edu/~lavanya/Graphics/cs580/p5/web-page/p5.html
- An implementation of Marching Cubes and Marching Tetrahedra Algorithms, http://www.siafoo.net/snippet/100
Chapter7/MarchingTetrahedra
directory. For convenience, we will wrap the Marching Tetrahedra algorithm in a simple class called TetrahedraMarcher
.
Let us start this recipe by following these simple steps:
- Load the 3D volume data and store it into an array:
std::ifstream infile(filename.c_str(), std::ios_base::binary); if(infile.good()) { pVolume = new GLubyte[XDIM*YDIM*ZDIM]; infile.read(reinterpret_cast<char*>(pVolume), XDIM*YDIM*ZDIM*sizeof(GLubyte)); infile.close(); return true; } else { return false; }
- Depending on the sampling box size, run three loops to iterate through the entire volume voxel by voxel:
vertices.clear(); int dx = XDIM/X_SAMPLING_DIST; int dy = YDIM/Y_SAMPLING_DIST; int dz = ZDIM/Z_SAMPLING_DIST; glm::vec3 scale = glm::vec3(dx,dy,dz); for(int z=0;z<ZDIM;z+=dz) { for(int y=0;y<YDIM;y+=dy) { for(int x=0;x<XDIM;x+=dx) { SampleVoxel(x,y,z, scale); } } }
- In each sampling step, estimate the volume density values at the eight corners of the sampling box:
GLubyte cubeCornerValues[8]; for( i = 0; i < 8; i++) { cubeCornerValues[i] = SampleVolume( x + (int)(a2fVertexOffset[i][0] *scale.x), y + (int)(a2fVertexOffset[i][1]*scale.y), z + (int)(a2fVertexOffset[i][2]*scale.z)); }
- Estimate an edge flag value to identify the matching tetrahedra case based on the given isovalue:
int flagIndex = 0; for( i= 0; i<8; i++) { if(cubeCornerValues[i]<= isoValue) flagIndex |= 1<<i; } edgeFlags = aiCubeEdgeFlags[flagIndex];
- Use the lookup tables (
a2iEdgeConnection
) to find the correct edges for the case and then use the offset table (a2fVertexOffset
) to find the edge vertices and normals. These tables are defined in theTables.h
header in theChapter7/MarchingTetrahedra/
directory.for(i = 0; i < 12; i++) { if(edgeFlags & (1<<i)) { float offset = GetOffset(cubeCornerValues[ a2iEdgeConnection[i][0] ], cubeCornerValues[ a2iEdgeConnection[i][1] ]); edgeVertices[i].x = x + (a2fVertexOffset[ a2iEdgeConnection[i][0] ][0] + offset * a2fEdgeDirection[i][0])*scale.x ; edgeVertices[i].y = y + (a2fVertexOffset[ a2iEdgeConnection[i][0] ][1] + offset * a2fEdgeDirection[i][1])*scale.y ; edgeVertices[i].z = z + (a2fVertexOffset[ a2iEdgeConnection[i][0] ][2] + offset * a2fEdgeDirection[i][2])*scale.z ; edgeNormals[i] = GetNormal( (int)edgeVertices[i].x , (int)edgeVertices[i].y , (int)edgeVertices[i].z ); } }
- Finally, loop through the triangle connectivity table to connect the correct vertices and normals for the given case.
for(i = 0; i< 5; i++) { if(a2iTriangleConnectionTable[flagIndex][3*i] < 0) break; for(int j= 0; j< 3; j++) { int vertex = a2iTriangleConnectionTable[flagIndex][3*i+j]; Vertex v; v.normal = (edgeNormals[vertex]); v.pos = (edgeVertices[vertex])*invDim; vertices.push_back(v); } }
- After the marcher is finished, we pass the generated vertices to a vertex array object containing a vertex buffer object:
glGenVertexArrays(1, &volumeMarcherVAO); glGenBuffers(1, &volumeMarcherVBO); glBindVertexArray(volumeMarcherVAO); glBindBuffer (GL_ARRAY_BUFFER, volumeMarcherVBO); glBufferData (GL_ARRAY_BUFFER, marcher-> GetTotalVertices()*sizeof(Vertex), marcher-> GetVertexPointer(), GL_STATIC_DRAW); glEnableVertexAttribArray(0); glVertexAttribPointer(0, 3, GL_FLOAT, GL_FALSE,sizeof(Vertex),0); glEnableVertexAttribArray(1); glVertexAttribPointer(1, 3, GL_FLOAT, GL_FALSE,sizeof(Vertex),(const GLvoid*)offsetof(Vertex, normal));
- For rendering of the generated geometry, we bind the Marching Tetrahedra VAO, bind our shader and then render the triangles. For this recipe, we output the per-vertex normals as color.
glBindVertexArray(volumeMarcherVAO); shader.Use(); glUniformMatrix4fv(shader("MVP"),1,GL_FALSE, glm::value_ptr(MVP*T)); glDrawArrays(GL_TRIANGLES, 0, marcher->GetTotalVertices()); shader.UnUse();
For convenience, we wrap the entire recipe in a reusable class called TetrahedraMarcher
. Marching Tetrahedra, as the name suggests, marches a sampling box throughout the whole volume dataset. To give a bird's eye view there are several cases to consider based on the distribution of density values at the vertices of the sampling cube. Based on the sampling values at the eight corners and the given isovalue, a flag index is generated. This flag index gives us the edge flag by a lookup in a table. This edge flag is then used in an edge lookup table, which is predefined for all possible edge configurations of the marching tetrahedron. The edge connection table is then used to find the appropriate offset for the corner values of the sampling box. These offsets are then used to obtain the edge vertices and normals for the given tetrahedral case. Once the list of edge vertices and normals are estimated, the triangle connectivity is obtained based on the given flag index.
Now we will detail the steps in the Marching Tetrahedra algorithm. First, the flag index is obtained by iterating through all eight sampling cube vertices and comparing the density value at the vertex location with the given isovalue as shown in the following code. The flag index is then used to retrieve the edge flags from the looktup table (aiCubeEdgeFlags
).
After the Marching Tetrahedra code is processed, we store the generated vertices and normals in a buffer object. In the rendering code, we bind the appropriate vertex array object, bind our shader and then draw the triangles. The fragment shader for this recipe outputs the per-vertex normals as colors.
Pressing the W key toggles the wireframe display, which shows the underlying isosurface polygons for isovalue of 40 as shown in the following screenshot:
While in this recipe, we focused on the Marching Tetrahedra algorithm. There is another, more robust method of triangulation called Marching Cubes, which gives a more robust polygonisation as compared to the Marching Tetrahedra algorithm.
- Polygonising a scalar field, Paul Bourke: http://paulbourke.net/geometry/polygonise/
- Volume Rendering: Marching Cubes Algorithm, http://cns-alumni.bu.edu/~lavanya/Graphics/cs580/p5/web-page/p5.html
- An implementation of Marching Cubes and Marching Tetrahedra Algorithms, http://www.siafoo.net/snippet/100
recipe by following these simple steps:
- Load the 3D volume data and store it into an array:
std::ifstream infile(filename.c_str(), std::ios_base::binary); if(infile.good()) { pVolume = new GLubyte[XDIM*YDIM*ZDIM]; infile.read(reinterpret_cast<char*>(pVolume), XDIM*YDIM*ZDIM*sizeof(GLubyte)); infile.close(); return true; } else { return false; }
- Depending on the sampling box size, run three loops to iterate through the entire volume voxel by voxel:
vertices.clear(); int dx = XDIM/X_SAMPLING_DIST; int dy = YDIM/Y_SAMPLING_DIST; int dz = ZDIM/Z_SAMPLING_DIST; glm::vec3 scale = glm::vec3(dx,dy,dz); for(int z=0;z<ZDIM;z+=dz) { for(int y=0;y<YDIM;y+=dy) { for(int x=0;x<XDIM;x+=dx) { SampleVoxel(x,y,z, scale); } } }
- In each sampling step, estimate the volume density values at the eight corners of the sampling box:
GLubyte cubeCornerValues[8]; for( i = 0; i < 8; i++) { cubeCornerValues[i] = SampleVolume( x + (int)(a2fVertexOffset[i][0] *scale.x), y + (int)(a2fVertexOffset[i][1]*scale.y), z + (int)(a2fVertexOffset[i][2]*scale.z)); }
- Estimate an edge flag value to identify the matching tetrahedra case based on the given isovalue:
int flagIndex = 0; for( i= 0; i<8; i++) { if(cubeCornerValues[i]<= isoValue) flagIndex |= 1<<i; } edgeFlags = aiCubeEdgeFlags[flagIndex];
- Use the lookup tables (
a2iEdgeConnection
) to find the correct edges for the case and then use the offset table (a2fVertexOffset
) to find the edge vertices and normals. These tables are defined in theTables.h
header in theChapter7/MarchingTetrahedra/
directory.for(i = 0; i < 12; i++) { if(edgeFlags & (1<<i)) { float offset = GetOffset(cubeCornerValues[ a2iEdgeConnection[i][0] ], cubeCornerValues[ a2iEdgeConnection[i][1] ]); edgeVertices[i].x = x + (a2fVertexOffset[ a2iEdgeConnection[i][0] ][0] + offset * a2fEdgeDirection[i][0])*scale.x ; edgeVertices[i].y = y + (a2fVertexOffset[ a2iEdgeConnection[i][0] ][1] + offset * a2fEdgeDirection[i][1])*scale.y ; edgeVertices[i].z = z + (a2fVertexOffset[ a2iEdgeConnection[i][0] ][2] + offset * a2fEdgeDirection[i][2])*scale.z ; edgeNormals[i] = GetNormal( (int)edgeVertices[i].x , (int)edgeVertices[i].y , (int)edgeVertices[i].z ); } }
- Finally, loop through the triangle connectivity table to connect the correct vertices and normals for the given case.
for(i = 0; i< 5; i++) { if(a2iTriangleConnectionTable[flagIndex][3*i] < 0) break; for(int j= 0; j< 3; j++) { int vertex = a2iTriangleConnectionTable[flagIndex][3*i+j]; Vertex v; v.normal = (edgeNormals[vertex]); v.pos = (edgeVertices[vertex])*invDim; vertices.push_back(v); } }
- After the marcher is finished, we pass the generated vertices to a vertex array object containing a vertex buffer object:
glGenVertexArrays(1, &volumeMarcherVAO); glGenBuffers(1, &volumeMarcherVBO); glBindVertexArray(volumeMarcherVAO); glBindBuffer (GL_ARRAY_BUFFER, volumeMarcherVBO); glBufferData (GL_ARRAY_BUFFER, marcher-> GetTotalVertices()*sizeof(Vertex), marcher-> GetVertexPointer(), GL_STATIC_DRAW); glEnableVertexAttribArray(0); glVertexAttribPointer(0, 3, GL_FLOAT, GL_FALSE,sizeof(Vertex),0); glEnableVertexAttribArray(1); glVertexAttribPointer(1, 3, GL_FLOAT, GL_FALSE,sizeof(Vertex),(const GLvoid*)offsetof(Vertex, normal));
- For rendering of the generated geometry, we bind the Marching Tetrahedra VAO, bind our shader and then render the triangles. For this recipe, we output the per-vertex normals as color.
glBindVertexArray(volumeMarcherVAO); shader.Use(); glUniformMatrix4fv(shader("MVP"),1,GL_FALSE, glm::value_ptr(MVP*T)); glDrawArrays(GL_TRIANGLES, 0, marcher->GetTotalVertices()); shader.UnUse();
For convenience, we wrap the entire recipe in a reusable class called TetrahedraMarcher
. Marching Tetrahedra, as the name suggests, marches a sampling box throughout the whole volume dataset. To give a bird's eye view there are several cases to consider based on the distribution of density values at the vertices of the sampling cube. Based on the sampling values at the eight corners and the given isovalue, a flag index is generated. This flag index gives us the edge flag by a lookup in a table. This edge flag is then used in an edge lookup table, which is predefined for all possible edge configurations of the marching tetrahedron. The edge connection table is then used to find the appropriate offset for the corner values of the sampling box. These offsets are then used to obtain the edge vertices and normals for the given tetrahedral case. Once the list of edge vertices and normals are estimated, the triangle connectivity is obtained based on the given flag index.
Now we will detail the steps in the Marching Tetrahedra algorithm. First, the flag index is obtained by iterating through all eight sampling cube vertices and comparing the density value at the vertex location with the given isovalue as shown in the following code. The flag index is then used to retrieve the edge flags from the looktup table (aiCubeEdgeFlags
).
After the Marching Tetrahedra code is processed, we store the generated vertices and normals in a buffer object. In the rendering code, we bind the appropriate vertex array object, bind our shader and then draw the triangles. The fragment shader for this recipe outputs the per-vertex normals as colors.
Pressing the W key toggles the wireframe display, which shows the underlying isosurface polygons for isovalue of 40 as shown in the following screenshot:
While in this recipe, we focused on the Marching Tetrahedra algorithm. There is another, more robust method of triangulation called Marching Cubes, which gives a more robust polygonisation as compared to the Marching Tetrahedra algorithm.
- Polygonising a scalar field, Paul Bourke: http://paulbourke.net/geometry/polygonise/
- Volume Rendering: Marching Cubes Algorithm, http://cns-alumni.bu.edu/~lavanya/Graphics/cs580/p5/web-page/p5.html
- An implementation of Marching Cubes and Marching Tetrahedra Algorithms, http://www.siafoo.net/snippet/100
TetrahedraMarcher
. Marching Tetrahedra, as the name suggests, marches a sampling box throughout the whole volume dataset. To give a bird's eye view there are several cases to consider based on the distribution of density values at the vertices of the sampling cube. Based on the sampling values at the eight corners and the given isovalue, a flag index is generated. This flag index gives us the edge flag by a lookup in a table. This edge flag is then used in an edge lookup table, which is predefined for all possible edge configurations of the marching tetrahedron. The edge connection table is then used to find the appropriate offset for the corner values of the sampling box. These offsets are then used to obtain the edge vertices and normals for the given tetrahedral case. Once the list of edge vertices and
normals are estimated, the triangle connectivity is obtained based on the given flag index.
Now we will detail the steps in the Marching Tetrahedra algorithm. First, the flag index is obtained by iterating through all eight sampling cube vertices and comparing the density value at the vertex location with the given isovalue as shown in the following code. The flag index is then used to retrieve the edge flags from the looktup table (aiCubeEdgeFlags
).
After the Marching Tetrahedra code is processed, we store the generated vertices and normals in a buffer object. In the rendering code, we bind the appropriate vertex array object, bind our shader and then draw the triangles. The fragment shader for this recipe outputs the per-vertex normals as colors.
Pressing the W key toggles the wireframe display, which shows the underlying isosurface polygons for isovalue of 40 as shown in the following screenshot:
While in this recipe, we focused on the Marching Tetrahedra algorithm. There is another, more robust method of triangulation called Marching Cubes, which gives a more robust polygonisation as compared to the Marching Tetrahedra algorithm.
- Polygonising a scalar field, Paul Bourke: http://paulbourke.net/geometry/polygonise/
- Volume Rendering: Marching Cubes Algorithm, http://cns-alumni.bu.edu/~lavanya/Graphics/cs580/p5/web-page/p5.html
- An implementation of Marching Cubes and Marching Tetrahedra Algorithms, http://www.siafoo.net/snippet/100
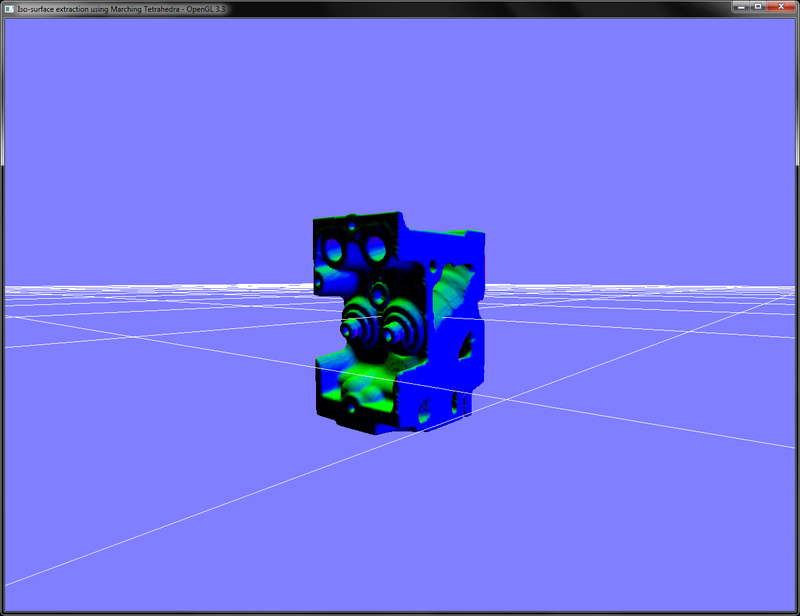
toggles the wireframe display, which shows the underlying isosurface polygons for isovalue of 40 as shown in the following screenshot:
While in this recipe, we focused on the Marching Tetrahedra algorithm. There is another, more robust method of triangulation called Marching Cubes, which gives a more robust polygonisation as compared to the Marching Tetrahedra algorithm.
- Polygonising a scalar field, Paul Bourke: http://paulbourke.net/geometry/polygonise/
- Volume Rendering: Marching Cubes Algorithm, http://cns-alumni.bu.edu/~lavanya/Graphics/cs580/p5/web-page/p5.html
- An implementation of Marching Cubes and Marching Tetrahedra Algorithms, http://www.siafoo.net/snippet/100
- http://paulbourke.net/geometry/polygonise/
- Volume Rendering: Marching Cubes Algorithm, http://cns-alumni.bu.edu/~lavanya/Graphics/cs580/p5/web-page/p5.html
- An implementation of Marching Cubes and Marching Tetrahedra Algorithms, http://www.siafoo.net/snippet/100
In this recipe, we will implement volumetric lighting using the half-angle slicing technique. Instead of slicing the volume perpendicular to the viewing direction, the slicing direction is set between the light and the view direction vectors. This enables us to simulate light absorption slice by slice.
Let us start this recipe by following these simple steps:
- Setup offscreen rendering using one FBO with two attachments: one for offscreen rendering of the light buffer and the other for offscreen rendering of the eye buffer.
glGenFramebuffers(1, &lightFBOID); glGenTextures (1, &lightBufferID); glGenTextures (1, &eyeBufferID); glActiveTexture(GL_TEXTURE2); lightBufferID = CreateTexture(IMAGE_WIDTH, IMAGE_HEIGHT, GL_RGBA16F, GL_RGBA); eyeBufferID = CreateTexture(IMAGE_WIDTH, IMAGE_HEIGHT, GL_RGBA16F, GL_RGBA); glBindFramebuffer(GL_FRAMEBUFFER, lightFBOID); glFramebufferTexture2D(GL_FRAMEBUFFER, GL_COLOR_ATTACHMENT0, GL_TEXTURE_2D, lightBufferID, 0); glFramebufferTexture2D(GL_FRAMEBUFFER, GL_COLOR_ATTACHMENT1, GL_TEXTURE_2D, eyeBufferID, 0); GLenum status = glCheckFramebufferStatus(GL_FRAMEBUFFER); if(status == GL_FRAMEBUFFER_COMPLETE ) printf("Light FBO setup successful !!! \n"); else printf("Problem with Light FBO setup");
The
CreateTexture
function performs the texture creation and texture format specification into a single function for convenience. This function is defined as follows: - Load the volume data, as in the 3D texture slicing recipe:
std::ifstream infile(volume_file.c_str(), std::ios_base::binary); if(infile.good()) { GLubyte* pData = new GLubyte[XDIM*YDIM*ZDIM]; infile.read(reinterpret_cast<char*>(pData), XDIM*YDIM*ZDIM*sizeof(GLubyte)); infile.close(); glGenTextures(1, &textureID); glActiveTexture(GL_TEXTURE0); glBindTexture(GL_TEXTURE_3D, textureID); // set the texture parameters glTexImage3D(GL_TEXTURE_3D,0,GL_RED,XDIM,YDIM,ZDIM,0, GL_RED,GL_UNSIGNED_BYTE,pData); GL_CHECK_ERRORS glGenerateMipmap(GL_TEXTURE_3D); return true; } else { return false; }
- Similar to the shadow mapping technique, calculate the shadow matrix by multiplying the model-view and projection matrices of the light with the bias matrix:
MV_L=glm::lookAt(lightPosOS,glm::vec3(0,0,0), glm::vec3(0,1,0)); P_L=glm::perspective(45.0f,1.0f,1.0f, 200.0f); B=glm::scale(glm::translate(glm::mat4(1), glm::vec3(0.5,0.5,0.5)), glm::vec3(0.5,0.5,0.5)); BP = B*P_L; S = BP*MV_L;
- In the rendering code, calculate the half vector by using the view direction vector and the light direction vector:
viewVec = -glm::vec3(MV[0][2], MV[1][2], MV[2][2]); lightVec = glm::normalize(lightPosOS); bIsViewInverted = glm::dot(viewVec, lightVec)<0; halfVec = glm::normalize( (bIsViewInverted?-viewVec:viewVec) + lightVec);
- Slice the volume data as in the 3D texture slicing recipe. The only difference here is that instead of slicing the volume data in the direction perpendicular to the view, we slice it in the direction which is halfway between the view and the light vectors.
float max_dist = glm::dot(halfVec, vertexList[0]); float min_dist = max_dist; int max_index = 0; int count = 0; for(int i=1;i<8;i++) { float dist = glm::dot(halfVec, vertexList[i]); if(dist > max_dist) { max_dist = dist; max_index = i; } if(dist<min_dist) min_dist = dist; } //rest of the SliceVolume function as in 3D texture slicing but //viewVec is changed to halfVec
- In the rendering code, bind the FBO and then first clear the light buffer with the white
color (1,1,1,1)
and the eye buffer with thecolor (0,0,0,0)
:glBindFramebuffer(GL_FRAMEBUFFER, lightFBOID); glDrawBuffer(attachIDs[0]); glClearColor(1,1,1,1); glClear(GL_COLOR_BUFFER_BIT ); glDrawBuffer(attachIDs[1]); glClearColor(0,0,0,0); glClear(GL_COLOR_BUFFER_BIT );
- Bind the volume VAO and then run a loop for the total number of slices. In each iteration, first render the slice in the eye buffer but bind the light buffer as the texture. Next, render the slice in the light buffer:
glBindVertexArray(volumeVAO); for(int i =0;i<num_slices;i++) { shaderShadow.Use(); glUniformMatrix4fv(shaderShadow("MVP"), 1, GL_FALSE, glm::value_ptr(MVP)); glUniformMatrix4fv(shaderShadow("S"), 1, GL_FALSE, glm::value_ptr(S)); glBindTexture(GL_TEXTURE_2D, lightBuffer); DrawSliceFromEyePointOfView(i); shader.Use(); glUniformMatrix4fv(shader("MVP"), 1, GL_FALSE, glm::value_ptr(P_L*MV_L)); DrawSliceFromLightPointOfView(i); }
- For the eye buffer rendering step, swap the blend function based on whether the viewer is viewing in the direction of the light or opposite to it:
void DrawSliceFromEyePointOfView(const int i) { glDrawBuffer(attachIDs[1]); glViewport(0, 0, IMAGE_WIDTH, IMAGE_HEIGHT); if(bIsViewInverted) { glBlendFunc(GL_ONE_MINUS_DST_ALPHA, GL_ONE); } else { glBlendFunc(GL_ONE, GL_ONE_MINUS_SRC_ALPHA); } glDrawArrays(GL_TRIANGLES, 12*i, 12); }
- For the light buffer, we simply blend the slices using the conventional "over" blending:
void DrawSliceFromLightPointOfView(const int i) { glDrawBuffer(attachIDs[0]); glViewport(0, 0, IMAGE_WIDTH, IMAGE_HEIGHT); glBlendFunc(GL_SRC_ALPHA, GL_ONE_MINUS_SRC_ALPHA); glDrawArrays(GL_TRIANGLES, 12*i, 12); }
- Finally, unbind the FBO and restore the default draw buffer. Next, set the viewport to the entire screen and then render the eye buffer on screen using a shader:
glBindVertexArray(0); glBindFramebuffer(GL_FRAMEBUFFER, 0); glDrawBuffer(GL_BACK_LEFT); glViewport(0,0,WIDTH, HEIGHT); glBindTexture(GL_TEXTURE_2D, eyeBufferID); glBindVertexArray(quadVAOID); quadShader.Use(); glDrawArrays(GL_TRIANGLES, 0, 6); quadShader.UnUse(); glBindVertexArray(0);
As the name suggests, this technique renders the volume by accumulating the intermediate results into two separate buffers by slicing the volume halfway between the light and the view vectors. When the scene is rendered from the point of view of the eye, the light buffer is used as texture to find out whether the current fragment is in shadow or not. This is carried out in the fragment shader by looking up the light buffer by using the shadow matrix as in the shadow mapping algorithm. In this step, the blending equation is swapped based on the direction of view with respect to the light direction vector. If the view is inverted, the blending direction is swapped from front-to-back to back-to-front using glBlendFunc(GL_ONE_MINUS_DST_ALPHA, GL_ONE)
. On the other hand, if the view direction is not inverted, the blend function is set as glBlendFunc(GL_ONE, GL_ONE_MINUS_SRC_ALPHA)
. Note that here we have not used the over compositing since we premultiply the color with its alpha in the fragment shader (see Chapter7/HalfAngleSlicing/shaders/slicerShadow.frag
), as shown in the following code:
In the next step, the scene is rendered from the point of view of the light. This time, the normal over compositing is used. This ensures that the light contributions accumulate with each other similar to how light behaves in normal circumstances. In this case, we use the same fragment shader as was used in the 3D texture slicing recipe (see Chapter7/HalgAngleSlicing/shaders/textureSlicer.frag
).
Note that we cannot see the black halo around the volume dataset as was evident in earlier recipes. The reason for this is the if condition used in the fragment shader. We only perform these calculations if the current density value is greater than 0.1. This essentially removed air and other low intensity artifacts, producing a much better result.
- Chapter 39, Volume Rendering Techniques, in GPU Gems 1. Available online at http://http.developer.nvidia.com/GPUGems/gpugems_ch39.html
- Chapter 6, Global Volume Illumination, in Real-time Volume Graphics, AK Peters/CRC Press.
Chapter7/HalfAngleSlicing
directory. As the name suggests, this recipe will build up on the 3D texture slicing code.
Let us start this recipe by following these simple steps:
- Setup offscreen rendering using one FBO with two attachments: one for offscreen rendering of the light buffer and the other for offscreen rendering of the eye buffer.
glGenFramebuffers(1, &lightFBOID); glGenTextures (1, &lightBufferID); glGenTextures (1, &eyeBufferID); glActiveTexture(GL_TEXTURE2); lightBufferID = CreateTexture(IMAGE_WIDTH, IMAGE_HEIGHT, GL_RGBA16F, GL_RGBA); eyeBufferID = CreateTexture(IMAGE_WIDTH, IMAGE_HEIGHT, GL_RGBA16F, GL_RGBA); glBindFramebuffer(GL_FRAMEBUFFER, lightFBOID); glFramebufferTexture2D(GL_FRAMEBUFFER, GL_COLOR_ATTACHMENT0, GL_TEXTURE_2D, lightBufferID, 0); glFramebufferTexture2D(GL_FRAMEBUFFER, GL_COLOR_ATTACHMENT1, GL_TEXTURE_2D, eyeBufferID, 0); GLenum status = glCheckFramebufferStatus(GL_FRAMEBUFFER); if(status == GL_FRAMEBUFFER_COMPLETE ) printf("Light FBO setup successful !!! \n"); else printf("Problem with Light FBO setup");
The
CreateTexture
function performs the texture creation and texture format specification into a single function for convenience. This function is defined as follows: - Load the volume data, as in the 3D texture slicing recipe:
std::ifstream infile(volume_file.c_str(), std::ios_base::binary); if(infile.good()) { GLubyte* pData = new GLubyte[XDIM*YDIM*ZDIM]; infile.read(reinterpret_cast<char*>(pData), XDIM*YDIM*ZDIM*sizeof(GLubyte)); infile.close(); glGenTextures(1, &textureID); glActiveTexture(GL_TEXTURE0); glBindTexture(GL_TEXTURE_3D, textureID); // set the texture parameters glTexImage3D(GL_TEXTURE_3D,0,GL_RED,XDIM,YDIM,ZDIM,0, GL_RED,GL_UNSIGNED_BYTE,pData); GL_CHECK_ERRORS glGenerateMipmap(GL_TEXTURE_3D); return true; } else { return false; }
- Similar to the shadow mapping technique, calculate the shadow matrix by multiplying the model-view and projection matrices of the light with the bias matrix:
MV_L=glm::lookAt(lightPosOS,glm::vec3(0,0,0), glm::vec3(0,1,0)); P_L=glm::perspective(45.0f,1.0f,1.0f, 200.0f); B=glm::scale(glm::translate(glm::mat4(1), glm::vec3(0.5,0.5,0.5)), glm::vec3(0.5,0.5,0.5)); BP = B*P_L; S = BP*MV_L;
- In the rendering code, calculate the half vector by using the view direction vector and the light direction vector:
viewVec = -glm::vec3(MV[0][2], MV[1][2], MV[2][2]); lightVec = glm::normalize(lightPosOS); bIsViewInverted = glm::dot(viewVec, lightVec)<0; halfVec = glm::normalize( (bIsViewInverted?-viewVec:viewVec) + lightVec);
- Slice the volume data as in the 3D texture slicing recipe. The only difference here is that instead of slicing the volume data in the direction perpendicular to the view, we slice it in the direction which is halfway between the view and the light vectors.
float max_dist = glm::dot(halfVec, vertexList[0]); float min_dist = max_dist; int max_index = 0; int count = 0; for(int i=1;i<8;i++) { float dist = glm::dot(halfVec, vertexList[i]); if(dist > max_dist) { max_dist = dist; max_index = i; } if(dist<min_dist) min_dist = dist; } //rest of the SliceVolume function as in 3D texture slicing but //viewVec is changed to halfVec
- In the rendering code, bind the FBO and then first clear the light buffer with the white
color (1,1,1,1)
and the eye buffer with thecolor (0,0,0,0)
:glBindFramebuffer(GL_FRAMEBUFFER, lightFBOID); glDrawBuffer(attachIDs[0]); glClearColor(1,1,1,1); glClear(GL_COLOR_BUFFER_BIT ); glDrawBuffer(attachIDs[1]); glClearColor(0,0,0,0); glClear(GL_COLOR_BUFFER_BIT );
- Bind the volume VAO and then run a loop for the total number of slices. In each iteration, first render the slice in the eye buffer but bind the light buffer as the texture. Next, render the slice in the light buffer:
glBindVertexArray(volumeVAO); for(int i =0;i<num_slices;i++) { shaderShadow.Use(); glUniformMatrix4fv(shaderShadow("MVP"), 1, GL_FALSE, glm::value_ptr(MVP)); glUniformMatrix4fv(shaderShadow("S"), 1, GL_FALSE, glm::value_ptr(S)); glBindTexture(GL_TEXTURE_2D, lightBuffer); DrawSliceFromEyePointOfView(i); shader.Use(); glUniformMatrix4fv(shader("MVP"), 1, GL_FALSE, glm::value_ptr(P_L*MV_L)); DrawSliceFromLightPointOfView(i); }
- For the eye buffer rendering step, swap the blend function based on whether the viewer is viewing in the direction of the light or opposite to it:
void DrawSliceFromEyePointOfView(const int i) { glDrawBuffer(attachIDs[1]); glViewport(0, 0, IMAGE_WIDTH, IMAGE_HEIGHT); if(bIsViewInverted) { glBlendFunc(GL_ONE_MINUS_DST_ALPHA, GL_ONE); } else { glBlendFunc(GL_ONE, GL_ONE_MINUS_SRC_ALPHA); } glDrawArrays(GL_TRIANGLES, 12*i, 12); }
- For the light buffer, we simply blend the slices using the conventional "over" blending:
void DrawSliceFromLightPointOfView(const int i) { glDrawBuffer(attachIDs[0]); glViewport(0, 0, IMAGE_WIDTH, IMAGE_HEIGHT); glBlendFunc(GL_SRC_ALPHA, GL_ONE_MINUS_SRC_ALPHA); glDrawArrays(GL_TRIANGLES, 12*i, 12); }
- Finally, unbind the FBO and restore the default draw buffer. Next, set the viewport to the entire screen and then render the eye buffer on screen using a shader:
glBindVertexArray(0); glBindFramebuffer(GL_FRAMEBUFFER, 0); glDrawBuffer(GL_BACK_LEFT); glViewport(0,0,WIDTH, HEIGHT); glBindTexture(GL_TEXTURE_2D, eyeBufferID); glBindVertexArray(quadVAOID); quadShader.Use(); glDrawArrays(GL_TRIANGLES, 0, 6); quadShader.UnUse(); glBindVertexArray(0);
As the name suggests, this technique renders the volume by accumulating the intermediate results into two separate buffers by slicing the volume halfway between the light and the view vectors. When the scene is rendered from the point of view of the eye, the light buffer is used as texture to find out whether the current fragment is in shadow or not. This is carried out in the fragment shader by looking up the light buffer by using the shadow matrix as in the shadow mapping algorithm. In this step, the blending equation is swapped based on the direction of view with respect to the light direction vector. If the view is inverted, the blending direction is swapped from front-to-back to back-to-front using glBlendFunc(GL_ONE_MINUS_DST_ALPHA, GL_ONE)
. On the other hand, if the view direction is not inverted, the blend function is set as glBlendFunc(GL_ONE, GL_ONE_MINUS_SRC_ALPHA)
. Note that here we have not used the over compositing since we premultiply the color with its alpha in the fragment shader (see Chapter7/HalfAngleSlicing/shaders/slicerShadow.frag
), as shown in the following code:
In the next step, the scene is rendered from the point of view of the light. This time, the normal over compositing is used. This ensures that the light contributions accumulate with each other similar to how light behaves in normal circumstances. In this case, we use the same fragment shader as was used in the 3D texture slicing recipe (see Chapter7/HalgAngleSlicing/shaders/textureSlicer.frag
).
Note that we cannot see the black halo around the volume dataset as was evident in earlier recipes. The reason for this is the if condition used in the fragment shader. We only perform these calculations if the current density value is greater than 0.1. This essentially removed air and other low intensity artifacts, producing a much better result.
- Chapter 39, Volume Rendering Techniques, in GPU Gems 1. Available online at http://http.developer.nvidia.com/GPUGems/gpugems_ch39.html
- Chapter 6, Global Volume Illumination, in Real-time Volume Graphics, AK Peters/CRC Press.
glGenFramebuffers(1, &lightFBOID); glGenTextures (1, &lightBufferID); glGenTextures (1, &eyeBufferID); glActiveTexture(GL_TEXTURE2); lightBufferID = CreateTexture(IMAGE_WIDTH, IMAGE_HEIGHT, GL_RGBA16F, GL_RGBA); eyeBufferID = CreateTexture(IMAGE_WIDTH, IMAGE_HEIGHT, GL_RGBA16F, GL_RGBA); glBindFramebuffer(GL_FRAMEBUFFER, lightFBOID); glFramebufferTexture2D(GL_FRAMEBUFFER, GL_COLOR_ATTACHMENT0, GL_TEXTURE_2D, lightBufferID, 0); glFramebufferTexture2D(GL_FRAMEBUFFER, GL_COLOR_ATTACHMENT1, GL_TEXTURE_2D, eyeBufferID, 0); GLenum status = glCheckFramebufferStatus(GL_FRAMEBUFFER); if(status == GL_FRAMEBUFFER_COMPLETE ) printf("Light FBO setup successful !!! \n"); else printf("Problem with Light FBO setup");
CreateTexture
function
performs the texture creation and texture format specification into a single function for convenience. This function is defined as follows:
- Load the volume data, as in the 3D texture slicing recipe:
std::ifstream infile(volume_file.c_str(), std::ios_base::binary); if(infile.good()) { GLubyte* pData = new GLubyte[XDIM*YDIM*ZDIM]; infile.read(reinterpret_cast<char*>(pData), XDIM*YDIM*ZDIM*sizeof(GLubyte)); infile.close(); glGenTextures(1, &textureID); glActiveTexture(GL_TEXTURE0); glBindTexture(GL_TEXTURE_3D, textureID); // set the texture parameters glTexImage3D(GL_TEXTURE_3D,0,GL_RED,XDIM,YDIM,ZDIM,0, GL_RED,GL_UNSIGNED_BYTE,pData); GL_CHECK_ERRORS glGenerateMipmap(GL_TEXTURE_3D); return true; } else { return false; }
- Similar to the shadow mapping technique, calculate the shadow matrix by multiplying the model-view and projection matrices of the light with the bias matrix:
MV_L=glm::lookAt(lightPosOS,glm::vec3(0,0,0), glm::vec3(0,1,0)); P_L=glm::perspective(45.0f,1.0f,1.0f, 200.0f); B=glm::scale(glm::translate(glm::mat4(1), glm::vec3(0.5,0.5,0.5)), glm::vec3(0.5,0.5,0.5)); BP = B*P_L; S = BP*MV_L;
- In the rendering code, calculate the half vector by using the view direction vector and the light direction vector:
viewVec = -glm::vec3(MV[0][2], MV[1][2], MV[2][2]); lightVec = glm::normalize(lightPosOS); bIsViewInverted = glm::dot(viewVec, lightVec)<0; halfVec = glm::normalize( (bIsViewInverted?-viewVec:viewVec) + lightVec);
- Slice the volume data as in the 3D texture slicing recipe. The only difference here is that instead of slicing the volume data in the direction perpendicular to the view, we slice it in the direction which is halfway between the view and the light vectors.
float max_dist = glm::dot(halfVec, vertexList[0]); float min_dist = max_dist; int max_index = 0; int count = 0; for(int i=1;i<8;i++) { float dist = glm::dot(halfVec, vertexList[i]); if(dist > max_dist) { max_dist = dist; max_index = i; } if(dist<min_dist) min_dist = dist; } //rest of the SliceVolume function as in 3D texture slicing but //viewVec is changed to halfVec
- In the rendering code, bind the FBO and then first clear the light buffer with the white
color (1,1,1,1)
and the eye buffer with thecolor (0,0,0,0)
:glBindFramebuffer(GL_FRAMEBUFFER, lightFBOID); glDrawBuffer(attachIDs[0]); glClearColor(1,1,1,1); glClear(GL_COLOR_BUFFER_BIT ); glDrawBuffer(attachIDs[1]); glClearColor(0,0,0,0); glClear(GL_COLOR_BUFFER_BIT );
- Bind the volume VAO and then run a loop for the total number of slices. In each iteration, first render the slice in the eye buffer but bind the light buffer as the texture. Next, render the slice in the light buffer:
glBindVertexArray(volumeVAO); for(int i =0;i<num_slices;i++) { shaderShadow.Use(); glUniformMatrix4fv(shaderShadow("MVP"), 1, GL_FALSE, glm::value_ptr(MVP)); glUniformMatrix4fv(shaderShadow("S"), 1, GL_FALSE, glm::value_ptr(S)); glBindTexture(GL_TEXTURE_2D, lightBuffer); DrawSliceFromEyePointOfView(i); shader.Use(); glUniformMatrix4fv(shader("MVP"), 1, GL_FALSE, glm::value_ptr(P_L*MV_L)); DrawSliceFromLightPointOfView(i); }
- For the eye buffer rendering step, swap the blend function based on whether the viewer is viewing in the direction of the light or opposite to it:
void DrawSliceFromEyePointOfView(const int i) { glDrawBuffer(attachIDs[1]); glViewport(0, 0, IMAGE_WIDTH, IMAGE_HEIGHT); if(bIsViewInverted) { glBlendFunc(GL_ONE_MINUS_DST_ALPHA, GL_ONE); } else { glBlendFunc(GL_ONE, GL_ONE_MINUS_SRC_ALPHA); } glDrawArrays(GL_TRIANGLES, 12*i, 12); }
- For the light buffer, we simply blend the slices using the conventional "over" blending:
void DrawSliceFromLightPointOfView(const int i) { glDrawBuffer(attachIDs[0]); glViewport(0, 0, IMAGE_WIDTH, IMAGE_HEIGHT); glBlendFunc(GL_SRC_ALPHA, GL_ONE_MINUS_SRC_ALPHA); glDrawArrays(GL_TRIANGLES, 12*i, 12); }
- Finally, unbind the FBO and restore the default draw buffer. Next, set the viewport to the entire screen and then render the eye buffer on screen using a shader:
glBindVertexArray(0); glBindFramebuffer(GL_FRAMEBUFFER, 0); glDrawBuffer(GL_BACK_LEFT); glViewport(0,0,WIDTH, HEIGHT); glBindTexture(GL_TEXTURE_2D, eyeBufferID); glBindVertexArray(quadVAOID); quadShader.Use(); glDrawArrays(GL_TRIANGLES, 0, 6); quadShader.UnUse(); glBindVertexArray(0);
As the name suggests, this technique renders the volume by accumulating the intermediate results into two separate buffers by slicing the volume halfway between the light and the view vectors. When the scene is rendered from the point of view of the eye, the light buffer is used as texture to find out whether the current fragment is in shadow or not. This is carried out in the fragment shader by looking up the light buffer by using the shadow matrix as in the shadow mapping algorithm. In this step, the blending equation is swapped based on the direction of view with respect to the light direction vector. If the view is inverted, the blending direction is swapped from front-to-back to back-to-front using glBlendFunc(GL_ONE_MINUS_DST_ALPHA, GL_ONE)
. On the other hand, if the view direction is not inverted, the blend function is set as glBlendFunc(GL_ONE, GL_ONE_MINUS_SRC_ALPHA)
. Note that here we have not used the over compositing since we premultiply the color with its alpha in the fragment shader (see Chapter7/HalfAngleSlicing/shaders/slicerShadow.frag
), as shown in the following code:
In the next step, the scene is rendered from the point of view of the light. This time, the normal over compositing is used. This ensures that the light contributions accumulate with each other similar to how light behaves in normal circumstances. In this case, we use the same fragment shader as was used in the 3D texture slicing recipe (see Chapter7/HalgAngleSlicing/shaders/textureSlicer.frag
).
Note that we cannot see the black halo around the volume dataset as was evident in earlier recipes. The reason for this is the if condition used in the fragment shader. We only perform these calculations if the current density value is greater than 0.1. This essentially removed air and other low intensity artifacts, producing a much better result.
- Chapter 39, Volume Rendering Techniques, in GPU Gems 1. Available online at http://http.developer.nvidia.com/GPUGems/gpugems_ch39.html
- Chapter 6, Global Volume Illumination, in Real-time Volume Graphics, AK Peters/CRC Press.
technique renders the volume by accumulating the intermediate results into two separate buffers by slicing the volume halfway between the light and the view vectors. When the scene is rendered from the point of view of the eye, the light buffer is used as texture to find out whether the current fragment is in shadow or not. This is carried out in the fragment shader by looking up the light buffer by using the shadow matrix as in the shadow mapping algorithm. In this step, the blending equation is swapped based on the direction of view with respect to the light direction vector. If the view is inverted, the blending direction is swapped from front-to-back to back-to-front using glBlendFunc(GL_ONE_MINUS_DST_ALPHA, GL_ONE)
. On the other hand, if the view direction is not inverted, the blend function is set as glBlendFunc(GL_ONE, GL_ONE_MINUS_SRC_ALPHA)
. Note that here we have not used the over compositing since we premultiply the color with its alpha in the fragment shader (see Chapter7/HalfAngleSlicing/shaders/slicerShadow.frag
), as shown in the following code:
In the next step, the scene is rendered from the point of view of the light. This time, the normal over compositing is used. This ensures that the light contributions accumulate with each other similar to how light behaves in normal circumstances. In this case, we use the same fragment shader as was used in the 3D texture slicing recipe (see Chapter7/HalgAngleSlicing/shaders/textureSlicer.frag
).
Note that we cannot see the black halo around the volume dataset as was evident in earlier recipes. The reason for this is the if condition used in the fragment shader. We only perform these calculations if the current density value is greater than 0.1. This essentially removed air and other low intensity artifacts, producing a much better result.
- Chapter 39, Volume Rendering Techniques, in GPU Gems 1. Available online at http://http.developer.nvidia.com/GPUGems/gpugems_ch39.html
- Chapter 6, Global Volume Illumination, in Real-time Volume Graphics, AK Peters/CRC Press.
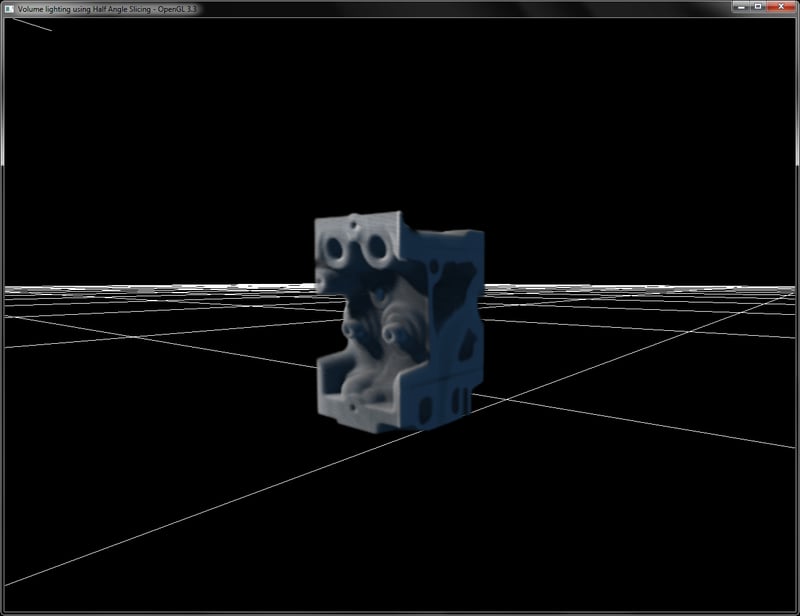
- Chapter 39, Volume Rendering Techniques, in GPU Gems 1. Available online at http://http.developer.nvidia.com/GPUGems/gpugems_ch39.html
- Chapter 6, Global Volume Illumination, in Real-time Volume Graphics, AK Peters/CRC Press.
- http://http.developer.nvidia.com/GPUGems/gpugems_ch39.html
- Chapter 6, Global Volume Illumination, in Real-time Volume Graphics, AK Peters/CRC Press.
In this chapter we will focus on the following topics:
- Implementing skeletal animation using matrix palette skinning
- Implementing skeletal animation using dual quaternion skinning
- Modeling cloth using transform feedback
- Implementing collision detection and response on a transform feedback-based cloth model
- Implementing a particle system using transform feedback
Most of the real-time graphics applications have interactive elements. We have automated bots that move and animate in an interactive application. These elements include objects that are animated using preset sequences of frames. These are called frame-by-frame animations. There are other scene elements that have motion, which is derived using physical simulation. These are called physically-based animations. In addition, humanoid or character models have a special category of animations called skeletal animation. In this chapter, we will look at recipes for doing skeletal and physically-based simulation on the GPU in modern OpenGL.
When working with games and simulation systems, virtual characters are often used to give a detailed depiction of scenarios. Such characters are typically represented using a combination of bones and skin. The vertices of the 3D model are assigned influence weights (called blend weights) that control how much a bone influences that vertex. Up to four bones can influence a vertex. The process whereby bone weights are assigned to the vertices of a 3D model is called skinning. Each bone stores its transformation. These stored sequences of transformations are applied to every frame and every bone in the model and in the end, we get an animated character on the screen. This representation of animation is called skeletal animation. There are several methods for skeletal animation. One popular method is matrix palette skinning, which is also known as linear blend skinning (LBS). This method will be implemented in this recipe.
The code for this recipe is contained in the Chapter8/MatrixPaletteSkinning
directory. This recipe will be using the Implementing EZMesh model loading recipe from Chapter 5, Mesh Model Formats and Particle Systems and it will augment it with skeletal animation. The EZMesh format was developed by John Ratcliff and it is an easy-to-understand format for storing skeletal animation. Typical skeletal animation formats like COLLADA and FBX are needlessly complicated, where dozens of segments have to be parsed before the real content can be loaded. On the other hand, the EZMesh format stores all of the information in an XML-based format, which is easier to parse. It is the default skeletal animation format used in the NVIDIA PhysX sdk. More information about the EZMesh model format and loaders can be obtained from the references in the See also section of this recipe.
Let us start our recipe by following these simple steps:
- Load the EZMesh model as we did in the Implementing EZMesh loader recipe from Chapter 5, Mesh Model Formats and Particle System. In addition to the model submeshes, vertices, normals, texture coordinates, and materials, we also load the skeleton information from the EZMesh file.
EzmLoader ezm; if(!ezm.Load(mesh_filename.c_str(), skeleton, animations, submeshes, vertices, indices, material2ImageMap, min, max)) { cout<<"Cannot load the EZMesh file"<<endl; exit(EXIT_FAILURE); }
- Get the
MeshSystem
object from themeshImportLibrary
object. Then load the bone transformations contained in the EZMesh file using theMeshSystem::mSkeletons
array. This is carried out in theEzmLoader::Load
function. Also generate absolute bone transforms from the relative transforms. This is done so that the transform of the child bone is influenced by the transform of the parent bone. This is continued up the hierarchy until the root bone. If the mesh is modeled in a positive Z axis system, we need to modify the orientation, positions, and scale by swapping Y and Z axes and changing the sign of one of them. This is done because we are using a positive Y axis system in OpenGL; otherwise, our mesh will be lying in the XZ plane rather than the XY plane. We obtain a combined matrix from the position orientation and scale of the bone. This is stored in thexform
field, which is the relative transform of the bone.if(ms->mSkeletonCount>0) { NVSHARE::MeshSkeleton* pSkel = ms->mSkeletons[0]; Bone b; for(int i=0;i<pSkel->GetBoneCount();i++) { const NVSHARE::MeshBone pBone = pSkel->mBones[i]; const int s = strlen(pBone.mName); b.name = new char[s+1]; memset(b.name, 0, sizeof(char)*(s+1)); strncpy_s(b.name,sizeof(char)*(s+1), pBone.mName, s); b.orientation = glm::quat( pBone.mOrientation[3],pBone.mOrientation[0], pBone.mOrientation[1],pBone.mOrientation[2]); b.position = glm::vec3( pBone.mPosition[0], pBone.mPosition[1],pBone.mPosition[2]); b.scale = glm::vec3(pBone.mScale[0], pBone.mScale[1], pBone.mScale[2]); if(!bYup) { float tmp = b.position.y; b.position.y = b.position.z; b.position.z = -tmp; tmp = b.orientation.y; b.orientation.y = b.orientation.z; b.orientation.z = -tmp; tmp = b.scale.y; b.scale.y = b.scale.z; b.scale.z = -tmp; } glm::mat4 S = glm::scale(glm::mat4(1), b.scale); glm::mat4 R = glm::toMat4(b.orientation); glm::mat4 T = glm::translate(glm::mat4(1), b.position); b.xform = T*R*S; b.parent = pBone.mParentIndex; skeleton.push_back(b); } UpdateCombinedMatrices(); bindPose.resize(skeleton.size()); invBindPose.resize(skeleton.size()); animatedXform.resize(skeleton.size());
- Generate the bind pose and inverse bind pose arrays from the stored bone transformations:
for(size_t i=0;i<skeleton.size();i++) { bindPose[i] = (skeleton[i].comb); invBindPose[i] = glm::inverse(bindPose[i]); }}
- Store the blend weights and blend indices of each vertex in the mesh:
mesh.vertices[j].blendWeights.x = pMesh->mVertices[j].mWeight[0]; mesh.vertices[j].blendWeights.y = pMesh->mVertices[j].mWeight[1]; mesh.vertices[j].blendWeights.z = pMesh->mVertices[j].mWeight[2]; mesh.vertices[j].blendWeights.w = pMesh->mVertices[j].mWeight[3]; mesh.vertices[j].blendIndices[0] = pMesh->mVertices[j].mBone[0]; mesh.vertices[j].blendIndices[1] = pMesh->mVertices[j].mBone[1]; mesh.vertices[j].blendIndices[2] = pMesh->mVertices[j].mBone[2]; mesh.vertices[j].blendIndices[3] = pMesh->mVertices[j].mBone[3];
- In the idle callback function, calculate the amount of time to spend on the current frame. If the amount has elapsed, move to the next frame and reset the time. After this, calculate the new bone transformations as well as new skinning matrices, and pass them to the shader:
QueryPerformanceCounter(¤t); dt = (double)(current.QuadPart - last.QuadPart) / (double)freq.QuadPart; last = current; static double t = 0; t+=dt; NVSHARE::MeshAnimation* pAnim = &animations[0]; float framesPerSecond = pAnim->GetFrameCount()/ pAnim->GetDuration(); if( t > 1.0f/ framesPerSecond) { currentFrame++; t=0; } if(bLoop) { currentFrame = currentFrame%pAnim->mFrameCount; } else { currentFrame=max(-1,min(currentFrame,pAnim->mFrameCount-1)); } if(currentFrame == -1) { for(size_t i=0;i<skeleton.size();i++) { skeleton[i].comb = bindPose[i]; animatedXform[i] = skeleton[i].comb*invBindPose[i]; } } else { for(int j=0;j<pAnim->mTrackCount;j++) { NVSHARE::MeshAnimTrack* pTrack = pAnim->mTracks[j]; NVSHARE::MeshAnimPose* pPose = pTrack->GetPose(currentFrame); skeleton[j].position.x = pPose->mPos[0]; skeleton[j].position.y = pPose->mPos[1]; skeleton[j].position.z = pPose->mPos[2]; glm::quat q; q.x = pPose->mQuat[0]; q.y = pPose->mQuat[1]; q.z = pPose->mQuat[2]; q.w = pPose->mQuat[3]; skeleton[j].scale = glm::vec3(pPose->mScale[0], pPose->mScale[1], pPose->mScale[2]); if(!bYup) { skeleton[j].position.y = pPose->mPos[2]; skeleton[j].position.z = -pPose->mPos[1]; q.y = pPose->mQuat[2]; q.z = -pPose->mQuat[1]; skeleton[j].scale.y = pPose->mScale[2]; skeleton[j].scale.z = -pPose->mScale[1]; } skeleton[j].orientation = q; glm::mat4 S =glm::scale(glm::mat4(1),skeleton[j].scale); glm::mat4 R = glm::toMat4(q); glm::mat4 T = glm::translate(glm::mat4(1), skeleton[j].position); skeleton[j].xform = T*R*S; Bone& b = skeleton[j]; if(b.parent==-1) b.comb = b.xform; else b.comb = skeleton[b.parent].comb * b.xform; animatedXform[j] = b.comb * invBindPose[j] ; } } shader.Use(); glUniformMatrix4fv(shader("Bones"),animatedXform.size(), GL_FALSE,glm::value_ptr(animatedXform[0])); shader.UnUse(); shader.UnUse();
There are two parts of this recipe: generation of skinning matrices and the calculation of GPU skinning in the vertex shader. To understand the first step, we will start with the different transforms that will be used in skinning. Typically, in a simulation or game, a transform is represented as a 4×4 matrix. For skeletal animation, we have a collection of bones. Each bone has a local transform (also called relative transform), which tells how the bone is positioned and oriented with respect to its parent bone. If the bone's local transform is multiplied to the global transform of its parent, we get the global transform (also called absolute transform) of the bone. Typically, the animation formats store the local transforms of the bones in the file. The user application uses this information to generate the global transforms.
We define our bone structure as follows:
The first field is orientation
, which is a quaternion storing the orientation of bone in space relative to its parent. The position
field stores its position relative to its parent. The xform
field is the local (relative) transform, and the comb
field is the global (absolute) transform. The scale
field contains the scaling transformation of the bone. In the big picture, the scale
field gives the scaling matrix (S
), the orientation
field gives the rotation matrix (R
), and the position
field gives the translation matrix (T
). The combined matrix T*R*S
gives us the relative transform that is calculated when we load the bone information from the EZMesh file in the second step.
Note that we do this once at initialization, so that we do not have to calculate the bind pose's inverse every frame, as it is required during animation.
The final matrix that we get from this process is called the skinning matrix (also called the final bone matrix). Continuing from the example given in the previous paragraph, let's say we have modified the relative transforms of bone using the animation sequence. We can then generate the skinning matrix as follows:
After we have calculated the skinning matrices, we pass these to the GPU in a single call:
This ensures that our vertex shader has the same number of bones as our mesh file.
The Vertex
struct storing all of our per-vertex attributes is defined as follows:
Finally, in the rendering code, we set the vertex array object and use the shader program. Then we iterate through all of the submeshes. We then set the material texture for the current submesh and set the shader uniforms. Finally, we issue a glDrawElements
call:
The matrix palette skinning is carried out on the GPU using the vertex shader (Chapter8/MatrixPaletteSkinning/shaders/shader.vert
). We simply use the blend indices and blend weights to calculate the correct vertex position and normal based on the combined influence of all of the effecting bones. The Bones
array contains the skinning matrices that we generated earlier. The complete vertex shader is as follows:
The fragment shader uses the attenuated point light source for illumination as we have seen in the Implementing per-fragment point light with attenuation recipe in Chapter 4, Lights and Shadows.
- NVIDIA DirectX SDK 9.0 Matrix Palette Skinning demo at http://http.download.nvidia.com/developer/SDK/Individual_Samples/DEMOS/Direct3D9/src/HLSL_PaletteSkin/docs/HLSL_PaletteSkin.pdf
- John Ratcliff code suppository containing a lot of useful tools, including the EZMesh format specifications and loaders available online at http://codesuppository.blogspot.sg/2009/11/test-application-for-meshimport-library.html
- Improved Skinning demo in the NVIDIA sdk at http://http.download.nvidia.com/developer/SDK/Individual_Samples/samples.html
Chapter8/MatrixPaletteSkinning
directory. This recipe will be using the Implementing EZMesh model loading recipe from
Chapter 5, Mesh Model Formats and Particle Systems and it will augment it with skeletal animation. The EZMesh format was developed by John Ratcliff and it is an easy-to-understand format for storing skeletal animation. Typical skeletal animation formats like COLLADA and FBX are needlessly complicated, where dozens of segments have to be parsed before the real content can be loaded. On the other hand, the EZMesh format stores all of the information in an XML-based format, which is easier to parse. It is the default skeletal animation format used in the NVIDIA PhysX sdk. More information about the EZMesh model format and loaders can be obtained from the references in the See also section of this recipe.
Let us start our recipe by following these simple steps:
- Load the EZMesh model as we did in the Implementing EZMesh loader recipe from Chapter 5, Mesh Model Formats and Particle System. In addition to the model submeshes, vertices, normals, texture coordinates, and materials, we also load the skeleton information from the EZMesh file.
EzmLoader ezm; if(!ezm.Load(mesh_filename.c_str(), skeleton, animations, submeshes, vertices, indices, material2ImageMap, min, max)) { cout<<"Cannot load the EZMesh file"<<endl; exit(EXIT_FAILURE); }
- Get the
MeshSystem
object from themeshImportLibrary
object. Then load the bone transformations contained in the EZMesh file using theMeshSystem::mSkeletons
array. This is carried out in theEzmLoader::Load
function. Also generate absolute bone transforms from the relative transforms. This is done so that the transform of the child bone is influenced by the transform of the parent bone. This is continued up the hierarchy until the root bone. If the mesh is modeled in a positive Z axis system, we need to modify the orientation, positions, and scale by swapping Y and Z axes and changing the sign of one of them. This is done because we are using a positive Y axis system in OpenGL; otherwise, our mesh will be lying in the XZ plane rather than the XY plane. We obtain a combined matrix from the position orientation and scale of the bone. This is stored in thexform
field, which is the relative transform of the bone.if(ms->mSkeletonCount>0) { NVSHARE::MeshSkeleton* pSkel = ms->mSkeletons[0]; Bone b; for(int i=0;i<pSkel->GetBoneCount();i++) { const NVSHARE::MeshBone pBone = pSkel->mBones[i]; const int s = strlen(pBone.mName); b.name = new char[s+1]; memset(b.name, 0, sizeof(char)*(s+1)); strncpy_s(b.name,sizeof(char)*(s+1), pBone.mName, s); b.orientation = glm::quat( pBone.mOrientation[3],pBone.mOrientation[0], pBone.mOrientation[1],pBone.mOrientation[2]); b.position = glm::vec3( pBone.mPosition[0], pBone.mPosition[1],pBone.mPosition[2]); b.scale = glm::vec3(pBone.mScale[0], pBone.mScale[1], pBone.mScale[2]); if(!bYup) { float tmp = b.position.y; b.position.y = b.position.z; b.position.z = -tmp; tmp = b.orientation.y; b.orientation.y = b.orientation.z; b.orientation.z = -tmp; tmp = b.scale.y; b.scale.y = b.scale.z; b.scale.z = -tmp; } glm::mat4 S = glm::scale(glm::mat4(1), b.scale); glm::mat4 R = glm::toMat4(b.orientation); glm::mat4 T = glm::translate(glm::mat4(1), b.position); b.xform = T*R*S; b.parent = pBone.mParentIndex; skeleton.push_back(b); } UpdateCombinedMatrices(); bindPose.resize(skeleton.size()); invBindPose.resize(skeleton.size()); animatedXform.resize(skeleton.size());
- Generate the bind pose and inverse bind pose arrays from the stored bone transformations:
for(size_t i=0;i<skeleton.size();i++) { bindPose[i] = (skeleton[i].comb); invBindPose[i] = glm::inverse(bindPose[i]); }}
- Store the blend weights and blend indices of each vertex in the mesh:
mesh.vertices[j].blendWeights.x = pMesh->mVertices[j].mWeight[0]; mesh.vertices[j].blendWeights.y = pMesh->mVertices[j].mWeight[1]; mesh.vertices[j].blendWeights.z = pMesh->mVertices[j].mWeight[2]; mesh.vertices[j].blendWeights.w = pMesh->mVertices[j].mWeight[3]; mesh.vertices[j].blendIndices[0] = pMesh->mVertices[j].mBone[0]; mesh.vertices[j].blendIndices[1] = pMesh->mVertices[j].mBone[1]; mesh.vertices[j].blendIndices[2] = pMesh->mVertices[j].mBone[2]; mesh.vertices[j].blendIndices[3] = pMesh->mVertices[j].mBone[3];
- In the idle callback function, calculate the amount of time to spend on the current frame. If the amount has elapsed, move to the next frame and reset the time. After this, calculate the new bone transformations as well as new skinning matrices, and pass them to the shader:
QueryPerformanceCounter(¤t); dt = (double)(current.QuadPart - last.QuadPart) / (double)freq.QuadPart; last = current; static double t = 0; t+=dt; NVSHARE::MeshAnimation* pAnim = &animations[0]; float framesPerSecond = pAnim->GetFrameCount()/ pAnim->GetDuration(); if( t > 1.0f/ framesPerSecond) { currentFrame++; t=0; } if(bLoop) { currentFrame = currentFrame%pAnim->mFrameCount; } else { currentFrame=max(-1,min(currentFrame,pAnim->mFrameCount-1)); } if(currentFrame == -1) { for(size_t i=0;i<skeleton.size();i++) { skeleton[i].comb = bindPose[i]; animatedXform[i] = skeleton[i].comb*invBindPose[i]; } } else { for(int j=0;j<pAnim->mTrackCount;j++) { NVSHARE::MeshAnimTrack* pTrack = pAnim->mTracks[j]; NVSHARE::MeshAnimPose* pPose = pTrack->GetPose(currentFrame); skeleton[j].position.x = pPose->mPos[0]; skeleton[j].position.y = pPose->mPos[1]; skeleton[j].position.z = pPose->mPos[2]; glm::quat q; q.x = pPose->mQuat[0]; q.y = pPose->mQuat[1]; q.z = pPose->mQuat[2]; q.w = pPose->mQuat[3]; skeleton[j].scale = glm::vec3(pPose->mScale[0], pPose->mScale[1], pPose->mScale[2]); if(!bYup) { skeleton[j].position.y = pPose->mPos[2]; skeleton[j].position.z = -pPose->mPos[1]; q.y = pPose->mQuat[2]; q.z = -pPose->mQuat[1]; skeleton[j].scale.y = pPose->mScale[2]; skeleton[j].scale.z = -pPose->mScale[1]; } skeleton[j].orientation = q; glm::mat4 S =glm::scale(glm::mat4(1),skeleton[j].scale); glm::mat4 R = glm::toMat4(q); glm::mat4 T = glm::translate(glm::mat4(1), skeleton[j].position); skeleton[j].xform = T*R*S; Bone& b = skeleton[j]; if(b.parent==-1) b.comb = b.xform; else b.comb = skeleton[b.parent].comb * b.xform; animatedXform[j] = b.comb * invBindPose[j] ; } } shader.Use(); glUniformMatrix4fv(shader("Bones"),animatedXform.size(), GL_FALSE,glm::value_ptr(animatedXform[0])); shader.UnUse(); shader.UnUse();
There are two parts of this recipe: generation of skinning matrices and the calculation of GPU skinning in the vertex shader. To understand the first step, we will start with the different transforms that will be used in skinning. Typically, in a simulation or game, a transform is represented as a 4×4 matrix. For skeletal animation, we have a collection of bones. Each bone has a local transform (also called relative transform), which tells how the bone is positioned and oriented with respect to its parent bone. If the bone's local transform is multiplied to the global transform of its parent, we get the global transform (also called absolute transform) of the bone. Typically, the animation formats store the local transforms of the bones in the file. The user application uses this information to generate the global transforms.
We define our bone structure as follows:
The first field is orientation
, which is a quaternion storing the orientation of bone in space relative to its parent. The position
field stores its position relative to its parent. The xform
field is the local (relative) transform, and the comb
field is the global (absolute) transform. The scale
field contains the scaling transformation of the bone. In the big picture, the scale
field gives the scaling matrix (S
), the orientation
field gives the rotation matrix (R
), and the position
field gives the translation matrix (T
). The combined matrix T*R*S
gives us the relative transform that is calculated when we load the bone information from the EZMesh file in the second step.
Note that we do this once at initialization, so that we do not have to calculate the bind pose's inverse every frame, as it is required during animation.
The final matrix that we get from this process is called the skinning matrix (also called the final bone matrix). Continuing from the example given in the previous paragraph, let's say we have modified the relative transforms of bone using the animation sequence. We can then generate the skinning matrix as follows:
After we have calculated the skinning matrices, we pass these to the GPU in a single call:
This ensures that our vertex shader has the same number of bones as our mesh file.
The Vertex
struct storing all of our per-vertex attributes is defined as follows:
Finally, in the rendering code, we set the vertex array object and use the shader program. Then we iterate through all of the submeshes. We then set the material texture for the current submesh and set the shader uniforms. Finally, we issue a glDrawElements
call:
The matrix palette skinning is carried out on the GPU using the vertex shader (Chapter8/MatrixPaletteSkinning/shaders/shader.vert
). We simply use the blend indices and blend weights to calculate the correct vertex position and normal based on the combined influence of all of the effecting bones. The Bones
array contains the skinning matrices that we generated earlier. The complete vertex shader is as follows:
The fragment shader uses the attenuated point light source for illumination as we have seen in the Implementing per-fragment point light with attenuation recipe in Chapter 4, Lights and Shadows.
- NVIDIA DirectX SDK 9.0 Matrix Palette Skinning demo at http://http.download.nvidia.com/developer/SDK/Individual_Samples/DEMOS/Direct3D9/src/HLSL_PaletteSkin/docs/HLSL_PaletteSkin.pdf
- John Ratcliff code suppository containing a lot of useful tools, including the EZMesh format specifications and loaders available online at http://codesuppository.blogspot.sg/2009/11/test-application-for-meshimport-library.html
- Improved Skinning demo in the NVIDIA sdk at http://http.download.nvidia.com/developer/SDK/Individual_Samples/samples.html
- Chapter 5, Mesh Model Formats and Particle System. In addition to the model submeshes, vertices, normals, texture coordinates, and materials, we also load the skeleton information from the EZMesh file.
EzmLoader ezm; if(!ezm.Load(mesh_filename.c_str(), skeleton, animations, submeshes, vertices, indices, material2ImageMap, min, max)) { cout<<"Cannot load the EZMesh file"<<endl; exit(EXIT_FAILURE); }
- Get the
MeshSystem
object from themeshImportLibrary
object. Then load the bone transformations contained in the EZMesh file using theMeshSystem::mSkeletons
array. This is carried out in theEzmLoader::Load
function. Also generate absolute bone transforms from the relative transforms. This is done so that the transform of the child bone is influenced by the transform of the parent bone. This is continued up the hierarchy until the root bone. If the mesh is modeled in a positive Z axis system, we need to modify the orientation, positions, and scale by swapping Y and Z axes and changing the sign of one of them. This is done because we are using a positive Y axis system in OpenGL; otherwise, our mesh will be lying in the XZ plane rather than the XY plane. We obtain a combined matrix from the position orientation and scale of the bone. This is stored in thexform
field, which is the relative transform of the bone.if(ms->mSkeletonCount>0) { NVSHARE::MeshSkeleton* pSkel = ms->mSkeletons[0]; Bone b; for(int i=0;i<pSkel->GetBoneCount();i++) { const NVSHARE::MeshBone pBone = pSkel->mBones[i]; const int s = strlen(pBone.mName); b.name = new char[s+1]; memset(b.name, 0, sizeof(char)*(s+1)); strncpy_s(b.name,sizeof(char)*(s+1), pBone.mName, s); b.orientation = glm::quat( pBone.mOrientation[3],pBone.mOrientation[0], pBone.mOrientation[1],pBone.mOrientation[2]); b.position = glm::vec3( pBone.mPosition[0], pBone.mPosition[1],pBone.mPosition[2]); b.scale = glm::vec3(pBone.mScale[0], pBone.mScale[1], pBone.mScale[2]); if(!bYup) { float tmp = b.position.y; b.position.y = b.position.z; b.position.z = -tmp; tmp = b.orientation.y; b.orientation.y = b.orientation.z; b.orientation.z = -tmp; tmp = b.scale.y; b.scale.y = b.scale.z; b.scale.z = -tmp; } glm::mat4 S = glm::scale(glm::mat4(1), b.scale); glm::mat4 R = glm::toMat4(b.orientation); glm::mat4 T = glm::translate(glm::mat4(1), b.position); b.xform = T*R*S; b.parent = pBone.mParentIndex; skeleton.push_back(b); } UpdateCombinedMatrices(); bindPose.resize(skeleton.size()); invBindPose.resize(skeleton.size()); animatedXform.resize(skeleton.size());
- Generate the bind pose and inverse bind pose arrays from the stored bone transformations:
for(size_t i=0;i<skeleton.size();i++) { bindPose[i] = (skeleton[i].comb); invBindPose[i] = glm::inverse(bindPose[i]); }}
- Store the blend weights and blend indices of each vertex in the mesh:
mesh.vertices[j].blendWeights.x = pMesh->mVertices[j].mWeight[0]; mesh.vertices[j].blendWeights.y = pMesh->mVertices[j].mWeight[1]; mesh.vertices[j].blendWeights.z = pMesh->mVertices[j].mWeight[2]; mesh.vertices[j].blendWeights.w = pMesh->mVertices[j].mWeight[3]; mesh.vertices[j].blendIndices[0] = pMesh->mVertices[j].mBone[0]; mesh.vertices[j].blendIndices[1] = pMesh->mVertices[j].mBone[1]; mesh.vertices[j].blendIndices[2] = pMesh->mVertices[j].mBone[2]; mesh.vertices[j].blendIndices[3] = pMesh->mVertices[j].mBone[3];
- In the idle callback function, calculate the amount of time to spend on the current frame. If the amount has elapsed, move to the next frame and reset the time. After this, calculate the new bone transformations as well as new skinning matrices, and pass them to the shader:
QueryPerformanceCounter(¤t); dt = (double)(current.QuadPart - last.QuadPart) / (double)freq.QuadPart; last = current; static double t = 0; t+=dt; NVSHARE::MeshAnimation* pAnim = &animations[0]; float framesPerSecond = pAnim->GetFrameCount()/ pAnim->GetDuration(); if( t > 1.0f/ framesPerSecond) { currentFrame++; t=0; } if(bLoop) { currentFrame = currentFrame%pAnim->mFrameCount; } else { currentFrame=max(-1,min(currentFrame,pAnim->mFrameCount-1)); } if(currentFrame == -1) { for(size_t i=0;i<skeleton.size();i++) { skeleton[i].comb = bindPose[i]; animatedXform[i] = skeleton[i].comb*invBindPose[i]; } } else { for(int j=0;j<pAnim->mTrackCount;j++) { NVSHARE::MeshAnimTrack* pTrack = pAnim->mTracks[j]; NVSHARE::MeshAnimPose* pPose = pTrack->GetPose(currentFrame); skeleton[j].position.x = pPose->mPos[0]; skeleton[j].position.y = pPose->mPos[1]; skeleton[j].position.z = pPose->mPos[2]; glm::quat q; q.x = pPose->mQuat[0]; q.y = pPose->mQuat[1]; q.z = pPose->mQuat[2]; q.w = pPose->mQuat[3]; skeleton[j].scale = glm::vec3(pPose->mScale[0], pPose->mScale[1], pPose->mScale[2]); if(!bYup) { skeleton[j].position.y = pPose->mPos[2]; skeleton[j].position.z = -pPose->mPos[1]; q.y = pPose->mQuat[2]; q.z = -pPose->mQuat[1]; skeleton[j].scale.y = pPose->mScale[2]; skeleton[j].scale.z = -pPose->mScale[1]; } skeleton[j].orientation = q; glm::mat4 S =glm::scale(glm::mat4(1),skeleton[j].scale); glm::mat4 R = glm::toMat4(q); glm::mat4 T = glm::translate(glm::mat4(1), skeleton[j].position); skeleton[j].xform = T*R*S; Bone& b = skeleton[j]; if(b.parent==-1) b.comb = b.xform; else b.comb = skeleton[b.parent].comb * b.xform; animatedXform[j] = b.comb * invBindPose[j] ; } } shader.Use(); glUniformMatrix4fv(shader("Bones"),animatedXform.size(), GL_FALSE,glm::value_ptr(animatedXform[0])); shader.UnUse(); shader.UnUse();
There are two parts of this recipe: generation of skinning matrices and the calculation of GPU skinning in the vertex shader. To understand the first step, we will start with the different transforms that will be used in skinning. Typically, in a simulation or game, a transform is represented as a 4×4 matrix. For skeletal animation, we have a collection of bones. Each bone has a local transform (also called relative transform), which tells how the bone is positioned and oriented with respect to its parent bone. If the bone's local transform is multiplied to the global transform of its parent, we get the global transform (also called absolute transform) of the bone. Typically, the animation formats store the local transforms of the bones in the file. The user application uses this information to generate the global transforms.
We define our bone structure as follows:
The first field is orientation
, which is a quaternion storing the orientation of bone in space relative to its parent. The position
field stores its position relative to its parent. The xform
field is the local (relative) transform, and the comb
field is the global (absolute) transform. The scale
field contains the scaling transformation of the bone. In the big picture, the scale
field gives the scaling matrix (S
), the orientation
field gives the rotation matrix (R
), and the position
field gives the translation matrix (T
). The combined matrix T*R*S
gives us the relative transform that is calculated when we load the bone information from the EZMesh file in the second step.
Note that we do this once at initialization, so that we do not have to calculate the bind pose's inverse every frame, as it is required during animation.
The final matrix that we get from this process is called the skinning matrix (also called the final bone matrix). Continuing from the example given in the previous paragraph, let's say we have modified the relative transforms of bone using the animation sequence. We can then generate the skinning matrix as follows:
After we have calculated the skinning matrices, we pass these to the GPU in a single call:
This ensures that our vertex shader has the same number of bones as our mesh file.
The Vertex
struct storing all of our per-vertex attributes is defined as follows:
Finally, in the rendering code, we set the vertex array object and use the shader program. Then we iterate through all of the submeshes. We then set the material texture for the current submesh and set the shader uniforms. Finally, we issue a glDrawElements
call:
The matrix palette skinning is carried out on the GPU using the vertex shader (Chapter8/MatrixPaletteSkinning/shaders/shader.vert
). We simply use the blend indices and blend weights to calculate the correct vertex position and normal based on the combined influence of all of the effecting bones. The Bones
array contains the skinning matrices that we generated earlier. The complete vertex shader is as follows:
The fragment shader uses the attenuated point light source for illumination as we have seen in the Implementing per-fragment point light with attenuation recipe in Chapter 4, Lights and Shadows.
- NVIDIA DirectX SDK 9.0 Matrix Palette Skinning demo at http://http.download.nvidia.com/developer/SDK/Individual_Samples/DEMOS/Direct3D9/src/HLSL_PaletteSkin/docs/HLSL_PaletteSkin.pdf
- John Ratcliff code suppository containing a lot of useful tools, including the EZMesh format specifications and loaders available online at http://codesuppository.blogspot.sg/2009/11/test-application-for-meshimport-library.html
- Improved Skinning demo in the NVIDIA sdk at http://http.download.nvidia.com/developer/SDK/Individual_Samples/samples.html
parts of this recipe: generation of skinning matrices and the calculation of GPU skinning in the vertex shader. To understand the first step, we will start with the different transforms that will be used in skinning. Typically, in a simulation or game, a transform is represented as a 4×4 matrix. For skeletal animation, we have a collection of bones. Each bone has a local transform (also called relative transform), which tells how the bone is positioned and oriented with respect to its parent bone. If the bone's local transform is multiplied to the global transform of its parent, we get the global transform (also called absolute transform) of the bone. Typically, the animation formats store the local transforms of the bones in the file. The user application uses this information to generate the global transforms.
We define our bone structure as follows:
The first field is orientation
, which is a quaternion storing the orientation of bone in space relative to its parent. The position
field stores its position relative to its parent. The xform
field is the local (relative) transform, and the comb
field is the global (absolute) transform. The scale
field contains the scaling transformation of the bone. In the big picture, the scale
field gives the scaling matrix (S
), the orientation
field gives the rotation matrix (R
), and the position
field gives the translation matrix (T
). The combined matrix T*R*S
gives us the relative transform that is calculated when we load the bone information from the EZMesh file in the second step.
Note that we do this once at initialization, so that we do not have to calculate the bind pose's inverse every frame, as it is required during animation.
The final matrix that we get from this process is called the skinning matrix (also called the final bone matrix). Continuing from the example given in the previous paragraph, let's say we have modified the relative transforms of bone using the animation sequence. We can then generate the skinning matrix as follows:
After we have calculated the skinning matrices, we pass these to the GPU in a single call:
This ensures that our vertex shader has the same number of bones as our mesh file.
The Vertex
struct storing all of our per-vertex attributes is defined as follows:
Finally, in the rendering code, we set the vertex array object and use the shader program. Then we iterate through all of the submeshes. We then set the material texture for the current submesh and set the shader uniforms. Finally, we issue a glDrawElements
call:
The matrix palette skinning is carried out on the GPU using the vertex shader (Chapter8/MatrixPaletteSkinning/shaders/shader.vert
). We simply use the blend indices and blend weights to calculate the correct vertex position and normal based on the combined influence of all of the effecting bones. The Bones
array contains the skinning matrices that we generated earlier. The complete vertex shader is as follows:
The fragment shader uses the attenuated point light source for illumination as we have seen in the Implementing per-fragment point light with attenuation recipe in Chapter 4, Lights and Shadows.
- NVIDIA DirectX SDK 9.0 Matrix Palette Skinning demo at http://http.download.nvidia.com/developer/SDK/Individual_Samples/DEMOS/Direct3D9/src/HLSL_PaletteSkin/docs/HLSL_PaletteSkin.pdf
- John Ratcliff code suppository containing a lot of useful tools, including the EZMesh format specifications and loaders available online at http://codesuppository.blogspot.sg/2009/11/test-application-for-meshimport-library.html
- Improved Skinning demo in the NVIDIA sdk at http://http.download.nvidia.com/developer/SDK/Individual_Samples/samples.html
dude.ezm
model animating using the matrix palette skinning technique as shown in the following figure. The light source can be rotated by right-clicking on it and dragging. Pressing the l key stops the loop playback.
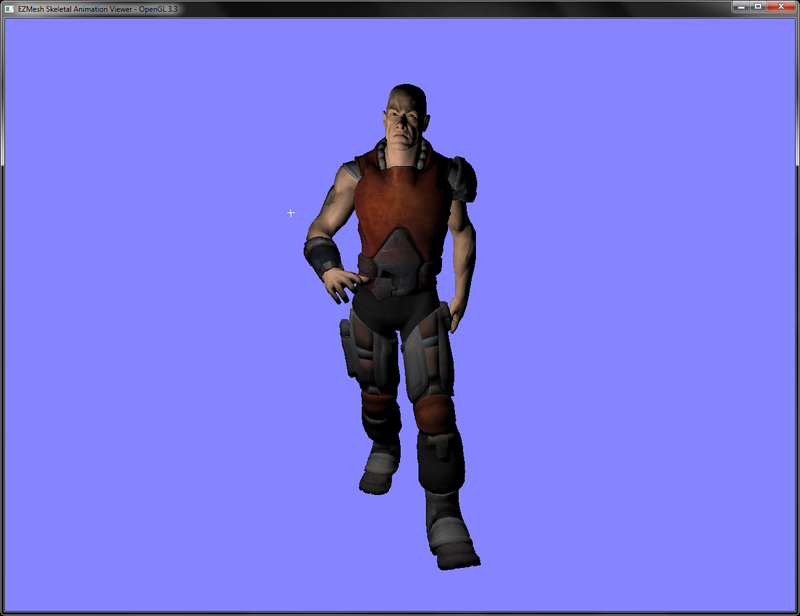
- NVIDIA DirectX SDK 9.0 Matrix Palette Skinning demo at http://http.download.nvidia.com/developer/SDK/Individual_Samples/DEMOS/Direct3D9/src/HLSL_PaletteSkin/docs/HLSL_PaletteSkin.pdf
- John Ratcliff code suppository containing a lot of useful tools, including the EZMesh format specifications and loaders available online at http://codesuppository.blogspot.sg/2009/11/test-application-for-meshimport-library.html
- Improved Skinning demo in the NVIDIA sdk at http://http.download.nvidia.com/developer/SDK/Individual_Samples/samples.html
- http://http.download.nvidia.com/developer/SDK/Individual_Samples/DEMOS/Direct3D9/src/HLSL_PaletteSkin/docs/HLSL_PaletteSkin.pdf
- John Ratcliff code suppository containing a lot of useful tools, including the EZMesh format specifications and loaders available online at http://codesuppository.blogspot.sg/2009/11/test-application-for-meshimport-library.html
- Improved Skinning demo in the NVIDIA sdk at http://http.download.nvidia.com/developer/SDK/Individual_Samples/samples.html
Matrix palette skinning suffers from candy wrapping artefacts, especially in regions like shoulder and elbow, where there are several rotations across various axes. If dual quaternion skinning is employed, these artefacts are minimized. In this recipe we will implement skeletal animation using dual quaternion skinning.
Before understanding dual quaternions, let us first see what quaternions are. Quaternions are a mathematical entity containing three imaginary dimensions (which specify the axis of rotation) and a real dimension (which specifies the angle of rotation). Quaternions are used in 3D graphics to represent rotation, since they do not suffer from gimbal lock, as Euler angles do. In order to store translation with rotation simultaneously, dual quaternions are used to store dual number coefficients instead of real ones. Instead of four components, as in a quaternion, dual quaternions have eight components.
Even in dual quaternion skinning, the linear blend method is used. However, due to the nature of transformation in dual quaternion, spherical blending is preferred. After linear blending, the resulting dual quaternion is renormalized, which generates a spherical blending result, which is a better approximation as compared to the linear blend skinning. This whole process is illustrated by the following figure:
Converting linear blend skinning to dual quaternion skinning requires the following steps:
- Load the EZMesh model as we did in the Implementing EZMesh loader recipe from Chapter 5, Mesh Model Formats and Particle System:
if(!ezm.Load(mesh_filename.c_str(), skeleton, animations, submeshes, vertices, indices, material2ImageMap, min, max)) { cout<<"Cannot load the EZMesh file"<<endl; exit(EXIT_FAILURE); }
- After loading up the mesh, materials, and textures, load the bone transformations contained in the EZMesh file using the
MeshSystem::mSkeletons
array as we did in the previous recipe. In addition to the bone matrices, also store the bind pose and inverse bind pose matrices as we did in the previous recipe. Instead of storing the skinning matrices, we initialize a vector of dual quaternions. Dual quaternions are a different representation of the skinning matrices.UpdateCombinedMatrices(); bindPose.resize(skeleton.size()); invBindPose.resize(skeleton.size()); animatedXform.resize(skeleton.size()); dualQuaternions.resize(skeleton.size()); for(size_t i=0;i<skeleton.size();i++) { bindPose[i] = (skeleton[i].comb); invBindPose[i] = glm::inverse(bindPose[i]); }
- Implement the idle callback function as in the previous recipe. Here, in addition to calculating the skinning matrix, also calculate the dual quaternion for the given skinning matrix. After all of the joints are done, pass the dual quaternion to the shader:
glm::mat4 S = glm::scale(glm::mat4(1),skeleton[j].scale); glm::mat4 R = glm::toMat4(q); glm::mat4 T = glm::translate(glm::mat4(1), skeleton[j].position); skeleton[j].xform = T*R*S; Bone& b = skeleton[j]; if(b.parent==-1) b.comb = b.xform; else b.comb = skeleton[b.parent].comb * b.xform; animatedXform[j] = b.comb * invBindPose[j]; glm::vec3 t = glm::vec3( animatedXform[j][3][0], animatedXform[j][3][1], animatedXform[j][3][2]); dualQuaternions[j].QuatTrans2UDQ( glm::toQuat(animatedXform[j]), t); … shader.Use(); glUniform4fv(shader("Bones"), skeleton.size()*2, &(dualQuaternions[0].ordinary.x)); shader.UnUse();
- In the vertex shader (
Chapter8/DualQuaternionSkinning/shaders/shader.vert
), calculate the skinning matrix from the passed dual quaternion and blend weights of the given vertices. Then proceed with the skinning matrix as we did in the previous recipe:#version 330 core layout(location = 0) in vec3 vVertex; layout(location = 1) in vec3 vNormal; layout(location = 2) in vec2 vUV; layout(location = 3) in vec4 vBlendWeights; layout(location = 4) in ivec4 viBlendIndices; smooth out vec2 vUVout; uniform mat4 P; uniform mat4 MV; uniform mat3 N; smooth out vec3 vEyeSpaceNormal; smooth out vec3 vEyeSpacePosition; void main() { vec4 blendVertex=vec4(0); vec3 blendNormal=vec3(0); vec4 blendDQ[2]; float yc = 1.0, zc = 1.0, wc = 1.0; if (dot(Bones[viBlendIndices.x * 2], Bones[viBlendIndices.y * 2]) < 0.0) yc = -1.0; if (dot(Bones[viBlendIndices.x * 2], Bones[viBlendIndices.z * 2]) < 0.0) zc = -1.0; if (dot(Bones[viBlendIndices.x * 2], Bones[viBlendIndices.w * 2]) < 0.0) wc = -1.0; blendDQ[0] = Bones[viBlendIndices.x * 2] * vBlendWeights.x; blendDQ[1] = Bones[viBlendIndices.x * 2 + 1] * vBlendWeights.x; blendDQ[0] += yc*Bones[viBlendIndices.y * 2] * vBlendWeights.y; blendDQ[1] += yc*Bones[viBlendIndices.y * 2 + 1] * vBlendWeights.y; blendDQ[0] += zc*Bones[viBlendIndices.z * 2] * vBlendWeights.z; blendDQ[1] += zc*Bones[viBlendIndices.z * 2 + 1] * vBlendWeights.z; blendDQ[0] += wc*Bones[viBlendIndices.w * 2] * vBlendWeights.w; blendDQ[1] += wc*Bones[viBlendIndices.w * 2 + 1] * vBlendWeights.w; mat4 skinTransform = dualQuatToMatrix(blendDQ[0], blendDQ[1]); blendVertex = skinTransform*vec4(vVertex,1); blendNormal = (skinTransform*vec4(vNormal,0)).xyz; vEyeSpacePosition = (MV*blendVertex).xyz; vEyeSpaceNormal = N*blendNormal; vUVout=vUV; gl_Position = P*vec4(vEyeSpacePosition,1); }
The only difference in this recipe and the previous recipe is the creation of a dual quaternion from the skinning matrix on the CPU, and its conversion back to a matrix in the vertex shader. After we have obtained the skinning matrices, we convert them into a dual quaternion array by using the dual_quat::QuatTrans2UDQ
function that gets a dual quaternion from a rotation quaternion and a translation vector. This function is defined as follows in the dual_quat
class (in Chapter8/DualQuaternionSkinning/main.cpp
):
The dual quaternion array is then passed to the shader instead of the bone matrices. In the vertex shader, we first do a dot product of the ordinary quaternion with the dual quaternion. If the dot product of the two quaternions is less than zero, it means they are both facing in opposite direction. We thus subtract the quaternion from the blended dual quaternion, otherwise we add it to the blended dual quaternion:
The returned matrix is then multiplied with the given vertex/normal, and then the eye space position/normal and texture coordinates are obtained. Finally, the clip space position is calculated:
On the other hand, if we use the matrix palette skinning, we get the following output, which clearly shows the candy wrapper artefacts:
- Skinning with Dual Quaternions at http://isg.cs.tcd.ie/projects/DualQuaternions/
- Skinning with Dual Quaternions demo in NVIDIA DirectX sdk 10.5 at http://developer.download.nvidia.com/SDK/10.5/direct3d/samples.html
- Dual Quaternion Google Summer of Code 2011 implementation in OGRE at http://www.ogre3d.org/tikiwiki/tiki-index.php?page=SoC2011%20Dual%20Quaternion%20Skinning
Chapter8/DualQuaternionSkinning
folder. We will be building on top of the previous recipe and replace the skinning matrices with dual quaternions.
Converting linear blend skinning to dual quaternion skinning requires the following steps:
- Load the EZMesh model as we did in the Implementing EZMesh loader recipe from Chapter 5, Mesh Model Formats and Particle System:
if(!ezm.Load(mesh_filename.c_str(), skeleton, animations, submeshes, vertices, indices, material2ImageMap, min, max)) { cout<<"Cannot load the EZMesh file"<<endl; exit(EXIT_FAILURE); }
- After loading up the mesh, materials, and textures, load the bone transformations contained in the EZMesh file using the
MeshSystem::mSkeletons
array as we did in the previous recipe. In addition to the bone matrices, also store the bind pose and inverse bind pose matrices as we did in the previous recipe. Instead of storing the skinning matrices, we initialize a vector of dual quaternions. Dual quaternions are a different representation of the skinning matrices.UpdateCombinedMatrices(); bindPose.resize(skeleton.size()); invBindPose.resize(skeleton.size()); animatedXform.resize(skeleton.size()); dualQuaternions.resize(skeleton.size()); for(size_t i=0;i<skeleton.size();i++) { bindPose[i] = (skeleton[i].comb); invBindPose[i] = glm::inverse(bindPose[i]); }
- Implement the idle callback function as in the previous recipe. Here, in addition to calculating the skinning matrix, also calculate the dual quaternion for the given skinning matrix. After all of the joints are done, pass the dual quaternion to the shader:
glm::mat4 S = glm::scale(glm::mat4(1),skeleton[j].scale); glm::mat4 R = glm::toMat4(q); glm::mat4 T = glm::translate(glm::mat4(1), skeleton[j].position); skeleton[j].xform = T*R*S; Bone& b = skeleton[j]; if(b.parent==-1) b.comb = b.xform; else b.comb = skeleton[b.parent].comb * b.xform; animatedXform[j] = b.comb * invBindPose[j]; glm::vec3 t = glm::vec3( animatedXform[j][3][0], animatedXform[j][3][1], animatedXform[j][3][2]); dualQuaternions[j].QuatTrans2UDQ( glm::toQuat(animatedXform[j]), t); … shader.Use(); glUniform4fv(shader("Bones"), skeleton.size()*2, &(dualQuaternions[0].ordinary.x)); shader.UnUse();
- In the vertex shader (
Chapter8/DualQuaternionSkinning/shaders/shader.vert
), calculate the skinning matrix from the passed dual quaternion and blend weights of the given vertices. Then proceed with the skinning matrix as we did in the previous recipe:#version 330 core layout(location = 0) in vec3 vVertex; layout(location = 1) in vec3 vNormal; layout(location = 2) in vec2 vUV; layout(location = 3) in vec4 vBlendWeights; layout(location = 4) in ivec4 viBlendIndices; smooth out vec2 vUVout; uniform mat4 P; uniform mat4 MV; uniform mat3 N; smooth out vec3 vEyeSpaceNormal; smooth out vec3 vEyeSpacePosition; void main() { vec4 blendVertex=vec4(0); vec3 blendNormal=vec3(0); vec4 blendDQ[2]; float yc = 1.0, zc = 1.0, wc = 1.0; if (dot(Bones[viBlendIndices.x * 2], Bones[viBlendIndices.y * 2]) < 0.0) yc = -1.0; if (dot(Bones[viBlendIndices.x * 2], Bones[viBlendIndices.z * 2]) < 0.0) zc = -1.0; if (dot(Bones[viBlendIndices.x * 2], Bones[viBlendIndices.w * 2]) < 0.0) wc = -1.0; blendDQ[0] = Bones[viBlendIndices.x * 2] * vBlendWeights.x; blendDQ[1] = Bones[viBlendIndices.x * 2 + 1] * vBlendWeights.x; blendDQ[0] += yc*Bones[viBlendIndices.y * 2] * vBlendWeights.y; blendDQ[1] += yc*Bones[viBlendIndices.y * 2 + 1] * vBlendWeights.y; blendDQ[0] += zc*Bones[viBlendIndices.z * 2] * vBlendWeights.z; blendDQ[1] += zc*Bones[viBlendIndices.z * 2 + 1] * vBlendWeights.z; blendDQ[0] += wc*Bones[viBlendIndices.w * 2] * vBlendWeights.w; blendDQ[1] += wc*Bones[viBlendIndices.w * 2 + 1] * vBlendWeights.w; mat4 skinTransform = dualQuatToMatrix(blendDQ[0], blendDQ[1]); blendVertex = skinTransform*vec4(vVertex,1); blendNormal = (skinTransform*vec4(vNormal,0)).xyz; vEyeSpacePosition = (MV*blendVertex).xyz; vEyeSpaceNormal = N*blendNormal; vUVout=vUV; gl_Position = P*vec4(vEyeSpacePosition,1); }
The only difference in this recipe and the previous recipe is the creation of a dual quaternion from the skinning matrix on the CPU, and its conversion back to a matrix in the vertex shader. After we have obtained the skinning matrices, we convert them into a dual quaternion array by using the dual_quat::QuatTrans2UDQ
function that gets a dual quaternion from a rotation quaternion and a translation vector. This function is defined as follows in the dual_quat
class (in Chapter8/DualQuaternionSkinning/main.cpp
):
The dual quaternion array is then passed to the shader instead of the bone matrices. In the vertex shader, we first do a dot product of the ordinary quaternion with the dual quaternion. If the dot product of the two quaternions is less than zero, it means they are both facing in opposite direction. We thus subtract the quaternion from the blended dual quaternion, otherwise we add it to the blended dual quaternion:
The returned matrix is then multiplied with the given vertex/normal, and then the eye space position/normal and texture coordinates are obtained. Finally, the clip space position is calculated:
On the other hand, if we use the matrix palette skinning, we get the following output, which clearly shows the candy wrapper artefacts:
- Skinning with Dual Quaternions at http://isg.cs.tcd.ie/projects/DualQuaternions/
- Skinning with Dual Quaternions demo in NVIDIA DirectX sdk 10.5 at http://developer.download.nvidia.com/SDK/10.5/direct3d/samples.html
- Dual Quaternion Google Summer of Code 2011 implementation in OGRE at http://www.ogre3d.org/tikiwiki/tiki-index.php?page=SoC2011%20Dual%20Quaternion%20Skinning
- Chapter 5, Mesh Model Formats and Particle System:
if(!ezm.Load(mesh_filename.c_str(), skeleton, animations, submeshes, vertices, indices, material2ImageMap, min, max)) { cout<<"Cannot load the EZMesh file"<<endl; exit(EXIT_FAILURE); }
- After loading up the mesh, materials, and textures, load the bone transformations contained in the EZMesh file using the
MeshSystem::mSkeletons
array as we did in the previous recipe. In addition to the bone matrices, also store the bind pose and inverse bind pose matrices as we did in the previous recipe. Instead of storing the skinning matrices, we initialize a vector of dual quaternions. Dual quaternions are a different representation of the skinning matrices.UpdateCombinedMatrices(); bindPose.resize(skeleton.size()); invBindPose.resize(skeleton.size()); animatedXform.resize(skeleton.size()); dualQuaternions.resize(skeleton.size()); for(size_t i=0;i<skeleton.size();i++) { bindPose[i] = (skeleton[i].comb); invBindPose[i] = glm::inverse(bindPose[i]); }
- Implement the idle callback function as in the previous recipe. Here, in addition to calculating the skinning matrix, also calculate the dual quaternion for the given skinning matrix. After all of the joints are done, pass the dual quaternion to the shader:
glm::mat4 S = glm::scale(glm::mat4(1),skeleton[j].scale); glm::mat4 R = glm::toMat4(q); glm::mat4 T = glm::translate(glm::mat4(1), skeleton[j].position); skeleton[j].xform = T*R*S; Bone& b = skeleton[j]; if(b.parent==-1) b.comb = b.xform; else b.comb = skeleton[b.parent].comb * b.xform; animatedXform[j] = b.comb * invBindPose[j]; glm::vec3 t = glm::vec3( animatedXform[j][3][0], animatedXform[j][3][1], animatedXform[j][3][2]); dualQuaternions[j].QuatTrans2UDQ( glm::toQuat(animatedXform[j]), t); … shader.Use(); glUniform4fv(shader("Bones"), skeleton.size()*2, &(dualQuaternions[0].ordinary.x)); shader.UnUse();
- In the vertex shader (
Chapter8/DualQuaternionSkinning/shaders/shader.vert
), calculate the skinning matrix from the passed dual quaternion and blend weights of the given vertices. Then proceed with the skinning matrix as we did in the previous recipe:#version 330 core layout(location = 0) in vec3 vVertex; layout(location = 1) in vec3 vNormal; layout(location = 2) in vec2 vUV; layout(location = 3) in vec4 vBlendWeights; layout(location = 4) in ivec4 viBlendIndices; smooth out vec2 vUVout; uniform mat4 P; uniform mat4 MV; uniform mat3 N; smooth out vec3 vEyeSpaceNormal; smooth out vec3 vEyeSpacePosition; void main() { vec4 blendVertex=vec4(0); vec3 blendNormal=vec3(0); vec4 blendDQ[2]; float yc = 1.0, zc = 1.0, wc = 1.0; if (dot(Bones[viBlendIndices.x * 2], Bones[viBlendIndices.y * 2]) < 0.0) yc = -1.0; if (dot(Bones[viBlendIndices.x * 2], Bones[viBlendIndices.z * 2]) < 0.0) zc = -1.0; if (dot(Bones[viBlendIndices.x * 2], Bones[viBlendIndices.w * 2]) < 0.0) wc = -1.0; blendDQ[0] = Bones[viBlendIndices.x * 2] * vBlendWeights.x; blendDQ[1] = Bones[viBlendIndices.x * 2 + 1] * vBlendWeights.x; blendDQ[0] += yc*Bones[viBlendIndices.y * 2] * vBlendWeights.y; blendDQ[1] += yc*Bones[viBlendIndices.y * 2 + 1] * vBlendWeights.y; blendDQ[0] += zc*Bones[viBlendIndices.z * 2] * vBlendWeights.z; blendDQ[1] += zc*Bones[viBlendIndices.z * 2 + 1] * vBlendWeights.z; blendDQ[0] += wc*Bones[viBlendIndices.w * 2] * vBlendWeights.w; blendDQ[1] += wc*Bones[viBlendIndices.w * 2 + 1] * vBlendWeights.w; mat4 skinTransform = dualQuatToMatrix(blendDQ[0], blendDQ[1]); blendVertex = skinTransform*vec4(vVertex,1); blendNormal = (skinTransform*vec4(vNormal,0)).xyz; vEyeSpacePosition = (MV*blendVertex).xyz; vEyeSpaceNormal = N*blendNormal; vUVout=vUV; gl_Position = P*vec4(vEyeSpacePosition,1); }
The only difference in this recipe and the previous recipe is the creation of a dual quaternion from the skinning matrix on the CPU, and its conversion back to a matrix in the vertex shader. After we have obtained the skinning matrices, we convert them into a dual quaternion array by using the dual_quat::QuatTrans2UDQ
function that gets a dual quaternion from a rotation quaternion and a translation vector. This function is defined as follows in the dual_quat
class (in Chapter8/DualQuaternionSkinning/main.cpp
):
The dual quaternion array is then passed to the shader instead of the bone matrices. In the vertex shader, we first do a dot product of the ordinary quaternion with the dual quaternion. If the dot product of the two quaternions is less than zero, it means they are both facing in opposite direction. We thus subtract the quaternion from the blended dual quaternion, otherwise we add it to the blended dual quaternion:
The returned matrix is then multiplied with the given vertex/normal, and then the eye space position/normal and texture coordinates are obtained. Finally, the clip space position is calculated:
On the other hand, if we use the matrix palette skinning, we get the following output, which clearly shows the candy wrapper artefacts:
- Skinning with Dual Quaternions at http://isg.cs.tcd.ie/projects/DualQuaternions/
- Skinning with Dual Quaternions demo in NVIDIA DirectX sdk 10.5 at http://developer.download.nvidia.com/SDK/10.5/direct3d/samples.html
- Dual Quaternion Google Summer of Code 2011 implementation in OGRE at http://www.ogre3d.org/tikiwiki/tiki-index.php?page=SoC2011%20Dual%20Quaternion%20Skinning
difference in this recipe and the previous recipe is the creation of a dual quaternion from the skinning matrix on the CPU, and its conversion back to a matrix in the vertex shader. After we have obtained the skinning matrices, we convert them into a dual quaternion array by using the dual_quat::QuatTrans2UDQ
function that gets a dual quaternion from a rotation quaternion and a translation vector. This function is defined as follows in the dual_quat
class (in Chapter8/DualQuaternionSkinning/main.cpp
):
The dual quaternion array is then passed to the shader instead of the bone matrices. In the vertex shader, we first do a dot product of the ordinary quaternion with the dual quaternion. If the dot product of the two quaternions is less than zero, it means they are both facing in opposite direction. We thus subtract the quaternion from the blended dual quaternion, otherwise we add it to the blended dual quaternion:
The returned matrix is then multiplied with the given vertex/normal, and then the eye space position/normal and texture coordinates are obtained. Finally, the clip space position is calculated:
On the other hand, if we use the matrix palette skinning, we get the following output, which clearly shows the candy wrapper artefacts:
- Skinning with Dual Quaternions at http://isg.cs.tcd.ie/projects/DualQuaternions/
- Skinning with Dual Quaternions demo in NVIDIA DirectX sdk 10.5 at http://developer.download.nvidia.com/SDK/10.5/direct3d/samples.html
- Dual Quaternion Google Summer of Code 2011 implementation in OGRE at http://www.ogre3d.org/tikiwiki/tiki-index.php?page=SoC2011%20Dual%20Quaternion%20Skinning
dwarf_anim.ezm
skeletal model. Even with extreme rotation at the shoulder joint, the output does not suffer from candy wrapper artefacts as shown in the following figure:
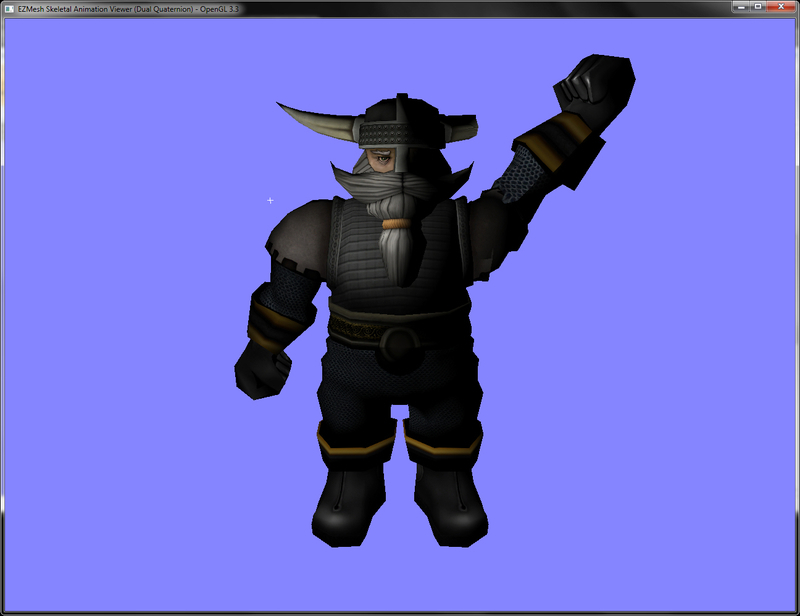
hand, if we use the matrix palette skinning, we get the following output, which clearly shows the candy wrapper artefacts:
- Skinning with Dual Quaternions at http://isg.cs.tcd.ie/projects/DualQuaternions/
- Skinning with Dual Quaternions demo in NVIDIA DirectX sdk 10.5 at http://developer.download.nvidia.com/SDK/10.5/direct3d/samples.html
- Dual Quaternion Google Summer of Code 2011 implementation in OGRE at http://www.ogre3d.org/tikiwiki/tiki-index.php?page=SoC2011%20Dual%20Quaternion%20Skinning
- http://isg.cs.tcd.ie/projects/DualQuaternions/
- Skinning with Dual Quaternions demo in NVIDIA DirectX sdk 10.5 at http://developer.download.nvidia.com/SDK/10.5/direct3d/samples.html
- Dual Quaternion Google Summer of Code 2011 implementation in OGRE at http://www.ogre3d.org/tikiwiki/tiki-index.php?page=SoC2011%20Dual%20Quaternion%20Skinning
In this recipe we will use the transform feedback mechanism of the modern GPU to model cloth. Transform feedback is a special mode of modern GPU in which the vertex shader can directly output to a buffer object. This allows developers to do complex computations without affecting the rest of the rendering pipeline. We will elaborate how to use this mechanism to simulate cloth entirely on the GPU.
From the implementation point of view in modern OpenGL, transform feedback exists as an OpenGL object similar to textures. Working with transform feedback object requires two steps: first, generation of transform feedback with specification of shader outputs, and second, usage of the transform feedback for simulation and rendering. We generate it by calling the glGetTransformFeedbacks
function and passing it the number of objects and the variable to store the returned IDs. After the object is created, it is bound to the current OpenGL context by calling glBindTransformFeedback
, and its only parameter is the ID of the transform feedback object we are interested to bind.
Next, we need to register the vertex attributes that we want to record in a transform feedback buffer. This is done through the glTransformFeedbackVaryings
function. The parameters this function requires are in the following order: the shader program object, the number of outputs from the shader, the names of the attributes, and the recording mode. Recording mode can be either GL_INTERLEAVED_ATTRIBS
(which means that the attributes will all be stored in a single interleaved buffer object) or GL_SEPARATE_ATTRIBS
(which means each attribute will be stored in its own buffer object). Note that the shader program has to be relinked after the shader output varyings are specified.
We also have to set up our buffer objects that are going to store the attributes' output through transform feedback. At the rendering stage, we first set up our shader and the required uniforms. Then, we bind out vertex array objects storing out buffer object binding. Next, we bind the buffer object for transform feedback by calling the glBindBufferBase
function. The first parameter is the index and the second parameter is the buffer object ID, which will store the shader output attribute. We can bind as many objects as we need, but the total calls to this function must be at least equal to the total output attributes from the vertex shader. Once the buffers are bound, we can initiate transform feedback by issuing a call to glBeginTransformFeedback
and the parameter to this function is the output primitive type. We then issue our glDraw*
call and then call glEndTransformFeedback
.
For the cloth simulation implementation using transform feedback, this is how we proceed. We store the current and previous position of the cloth vertices into a pair of buffer objects. To have convenient access to the buffer objects, we store these into a pair of vertex array objects. Then in order to deform the cloth, we run a vertex shader that inputs the current and previous positions from the buffer objects. In the vertex shader, the internal and external forces are calculated for each pair of cloth vertices and then acceleration is calculated. Using Verlet integration, the new vertex position is obtained. The new and previous positions are output from the vertex shader, so they are written out to the attached transform feedback buffers. Since we have a pair of vertex array objects, we ping pong between the two. This process is continued and the simulation proceeds forward.
The whole process is well summarized by the following figure:
Let us start the recipe by following these simple steps:
- Generate the geometry and topology for a piece of cloth by creating a set of points and their connectivity. Bind this data to a buffer object. The vectors
X
andX_last
store the current and last position respectively, and the vectorF
stores the force for each vertex:vector<GLushort> indices; vector<glm::vec4> X; vector<glm::vec4> X_last; vector<glm::vec3> F; indices.resize( numX*numY*2*3); X.resize(total_points); X_last.resize(total_points); F.resize(total_points); for(int j=0;j<=numY;j++) { for(int i=0;i<=numX;i++) { X[count] = glm::vec4( ((float(i)/(u-1)) *2-1)* hsize, sizeX+1, ((float(j)/(v-1) )* sizeY),1); X_last[count] = X[count]; count++; } } GLushort* id=&indices[0]; for (int i = 0; i < numY; i++) { for (int j = 0; j < numX; j++) { int i0 = i * (numX+1) + j; int i1 = i0 + 1; int i2 = i0 + (numX+1); int i3 = i2 + 1; if ((j+i)%2) { *id++ = i0; *id++ = i2; *id++ = i1; *id++ = i1; *id++ = i2; *id++ = i3; } else { *id++ = i0; *id++ = i2; *id++ = i3; *id++ = i0; *id++ = i3; *id++ = i1; } } } glGenVertexArrays(1, &clothVAOID); glGenBuffers (1, &clothVBOVerticesID); glGenBuffers (1, &clothVBOIndicesID); glBindVertexArray(clothVAOID); glBindBuffer (GL_ARRAY_BUFFER, clothVBOVerticesID); glBufferData (GL_ARRAY_BUFFER, sizeof(float)*4*X.size(), &X[0].x, GL_STATIC_DRAW); glEnableVertexAttribArray(0); glVertexAttribPointer (0, 4, GL_FLOAT, GL_FALSE,0,0); glBindBuffer(GL_ELEMENT_ARRAY_BUFFER, clothVBOIndicesID); glBufferData(GL_ELEMENT_ARRAY_BUFFER, sizeof(GLushort)*indices.size(), &indices[0], GL_STATIC_DRAW); glBindVertexArray(0);
- Create two pairs of vertex array objects (VAO), one pair for rendering and another pair for update of points. Bind two buffer objects (containing current positions and previous positions) to the update VAO, and one buffer object (containing current positions) to the render VAO. Also attach an element array buffer for geometry indices. Set the buffer object usage parameter as
GL_DYNAMIC_COPY
). This usage parameter hints to the GPU that the contents of the buffer object will be frequently changed, and it will be read in OpenGL or used as a source for GL commands:glGenVertexArrays(2, vaoUpdateID); glGenVertexArrays(2, vaoRenderID); glGenBuffers( 2, vboID_Pos); glGenBuffers( 2, vboID_PrePos); for(int i=0;i<2;i++) { glBindVertexArray(vaoUpdateID[i]); glBindBuffer( GL_ARRAY_BUFFER, vboID_Pos[i]); glBufferData( GL_ARRAY_BUFFER, X.size()* sizeof(glm::vec4), &(X[0].x), GL_DYNAMIC_COPY); glEnableVertexAttribArray(0); glVertexAttribPointer(0, 4, GL_FLOAT, GL_FALSE, 0, 0); glBindBuffer( GL_ARRAY_BUFFER, vboID_PrePos[i]); glBufferData( GL_ARRAY_BUFFER, X_last.size()*sizeof(glm::vec4), &(X_last[0].x), GL_DYNAMIC_COPY); glEnableVertexAttribArray(1); glVertexAttribPointer(1, 4, GL_FLOAT, GL_FALSE, 0,0); } //set render vao for(int i=0;i<2;i++) { glBindVertexArray(vaoRenderID[i]); glBindBuffer( GL_ARRAY_BUFFER, vboID_Pos[i]); glEnableVertexAttribArray(0); glVertexAttribPointer(0, 4, GL_FLOAT, GL_FALSE, 0, 0); glBindBuffer(GL_ELEMENT_ARRAY_BUFFER, vboIndices); if(i==0) glBufferData(GL_ELEMENT_ARRAY_BUFFER, indices.size()*sizeof(GLushort), &indices[0], GL_STATIC_DRAW); }
- For ease of access in the vertex shader, bind the current and previous position buffer objects to a set of buffer textures. The buffer textures are one dimensional textures that are created like normal OpenGL textures using the
glGenTextures
call, but they are bound to theGL_TEXTURE_BUFFER
target. They provide read access to the entire buffer object memory in the vertex shader. The data is accessed in the vertex shader using thetexelFetchBuffer
function:for(int i=0;i<2;i++) { glBindTexture( GL_TEXTURE_BUFFER, texPosID[i]); glTexBuffer( GL_TEXTURE_BUFFER, GL_RGBA32F, vboID_Pos[i]); glBindTexture( GL_TEXTURE_BUFFER, texPrePosID[i]); glTexBuffer(GL_TEXTURE_BUFFER, GL_RGBA32F, vboID_PrePos[i]); }
- Generate a transform feedback object and pass the attribute names that will be output from our deformation vertex shader. Make sure to relink the program.
glGenTransformFeedbacks(1, &tfID); glBindTransformFeedback(GL_TRANSFORM_FEEDBACK, tfID); const char* varying_names[]={"out_position_mass", "out_prev_position"}; glTransformFeedbackVaryings(massSpringShader.GetProgram(), 2, varying_names, GL_SEPARATE_ATTRIBS); glLinkProgram(massSpringShader.GetProgram());
- In the rendering function, bind the cloth deformation shader (
Chapter8/TransformFeedbackCloth/shaders/Spring.vert
) and then run a loop. In each loop iteration, bind the texture buffers, and then bind the update vertex array object. At the same time, bind the previous buffer objects as the transform feedback buffers. These will store the output from the vertex shader. Disable the rasterizer, begin the transform feedback mode, and then draw the entire set of cloth vertices. Use the ping pong approach to swap the read/write pathways:massSpringShader.Use(); glUniformMatrix4fv(massSpringShader("MVP"), 1, GL_FALSE, glm::value_ptr(mMVP)); for(int i=0;i<NUM_ITER;i++) { glActiveTexture( GL_TEXTURE0); glBindTexture( GL_TEXTURE_BUFFER, texPosID[writeID]); glActiveTexture( GL_TEXTURE1); glBindTexture( GL_TEXTURE_BUFFER, texPrePosID[writeID]); glBindVertexArray( vaoUpdateID[writeID]); glBindBufferBase(GL_TRANSFORM_FEEDBACK_BUFFER, 0, vboID_Pos[readID]); glBindBufferBase(GL_TRANSFORM_FEEDBACK_BUFFER, 1, vboID_PrePos[readID]); glEnable(GL_RASTERIZER_DISCARD); // disable rasrization glBeginQuery(GL_TIME_ELAPSED,t_query); glBeginTransformFeedback(GL_POINTS); glDrawArrays(GL_POINTS, 0, total_points); glEndTransformFeedback(); glEndQuery(GL_TIME_ELAPSED); glFlush(); glDisable(GL_RASTERIZER_DISCARD); int tmp = readID; readID=writeID; writeID = tmp; } glGetQueryObjectui64v(t_query, GL_QUERY_RESULT, &elapsed_time); delta_time = elapsed_time / 1000000.0f; massSpringShader.UnUse();
- After the loop is terminated, bind the render VAO that renders the cloth geometry and vertices:
glBindVertexArray(vaoRenderID[writeID]); glDisable(GL_DEPTH_TEST); renderShader.Use(); glUniformMatrix4fv(renderShader("MVP"), 1, GL_FALSE, glm::value_ptr(mMVP)); glDrawElements(GL_TRIANGLES, indices.size(), GL_UNSIGNED_SHORT,0); renderShader.UnUse(); glEnable(GL_DEPTH_TEST); if(bDisplayMasses) { particleShader.Use(); glUniform1i(particleShader("selected_index"), selected_index); glUniformMatrix4fv(particleShader("MV"), 1, GL_FALSE, glm::value_ptr(mMV)); glUniformMatrix4fv(particleShader("MVP"), 1, GL_FALSE, glm::value_ptr(mMVP)); glDrawArrays(GL_POINTS, 0, total_points); particleShader.UnUse(); } glBindVertexArray( 0);
- In the vertex shader, obtain the current and previous position of the cloth vertex. If the vertex is a pinned vertex, set its mass to
0
so it would not be simulated; otherwise, add an external force based on gravity. Next loop through all neighbors of the current vertex by looking up the texture buffer and estimate the internal force:float m = position_mass.w; vec3 pos = position_mass.xyz; vec3 pos_old = prev_position.xyz; vec3 vel = (pos - pos_old) / dt; float ks=0, kd=0; int index = gl_VertexID; int ix = index % texsize_x; int iy = index / texsize_x; if(index ==0 || index == (texsize_x-1)) m = 0; vec3 F = gravity*m + (DEFAULT_DAMPING*vel); for(int k=0;k<12;k++) { ivec2 coord = getNextNeighbor(k, ks, kd); int j = coord.x; int i = coord.y; if (((iy + i) < 0) || ((iy + i) > (texsize_y-1))) continue; if (((ix + j) < 0) || ((ix + j) > (texsize_x-1))) continue; int index_neigh = (iy + i) * texsize_x + ix + j; vec3 p2 = texelFetchBuffer(tex_position_mass, index_neigh).xyz; vec3 p2_last = texelFetchBuffer(tex_prev_position_mass, index_neigh).xyz; vec2 coord_neigh = vec2(ix + j, iy + i)*step; float rest_length = length(coord*inv_cloth_size); vec3 v2 = (p2- p2_last)/dt; vec3 deltaP = pos - p2; vec3 deltaV = vel - v2; float dist = length(deltaP); float leftTerm = -ks * (dist-rest_length); float rightTerm = kd * (dot(deltaV, deltaP)/dist); vec3 springForce = (leftTerm + rightTerm)* normalize(deltaP); F += springForce; }
- Using the combined force, calculate the acceleration and then estimate the new position using Verlet integration. Output the appropriate attribute from the shader:
vec3 acc = vec3(0); if(m!=0) acc = F/m; vec3 tmp = pos; pos = pos * 2.0 - pos_old + acc* dt * dt; pos_old = tmp; pos.y=max(0, pos.y); out_position_mass = vec4(pos, m); out_prev_position = vec4(pos_old,m); gl_Position = MVP*vec4(pos, 1);
There are two parts of this recipe, the generation of geometry and identifying output attributes for transform feedback buffers. We first generate the cloth geometry and then associate our buffer objects. To enable easier access of current and previous positions, we bind the position buffer objects as texture buffers.
Next, the acceleration due to gravity and velocity damping force is applied. After this, a loop is run which basically loops through all of the neighbors of the current vertex and estimates the net internal (spring) force. This force is then added to the combined force for the current vertex:
The shader, along with the transform feedback mechanism, proceeds to deform all of the cloth vertices and in the end, we get the cloth vertices deformed.
Let us start the recipe by following these simple steps:
- Generate the geometry and topology for a piece of cloth by creating a set of points and their connectivity. Bind this data to a buffer object. The vectors
X
andX_last
store the current and last position respectively, and the vectorF
stores the force for each vertex:vector<GLushort> indices; vector<glm::vec4> X; vector<glm::vec4> X_last; vector<glm::vec3> F; indices.resize( numX*numY*2*3); X.resize(total_points); X_last.resize(total_points); F.resize(total_points); for(int j=0;j<=numY;j++) { for(int i=0;i<=numX;i++) { X[count] = glm::vec4( ((float(i)/(u-1)) *2-1)* hsize, sizeX+1, ((float(j)/(v-1) )* sizeY),1); X_last[count] = X[count]; count++; } } GLushort* id=&indices[0]; for (int i = 0; i < numY; i++) { for (int j = 0; j < numX; j++) { int i0 = i * (numX+1) + j; int i1 = i0 + 1; int i2 = i0 + (numX+1); int i3 = i2 + 1; if ((j+i)%2) { *id++ = i0; *id++ = i2; *id++ = i1; *id++ = i1; *id++ = i2; *id++ = i3; } else { *id++ = i0; *id++ = i2; *id++ = i3; *id++ = i0; *id++ = i3; *id++ = i1; } } } glGenVertexArrays(1, &clothVAOID); glGenBuffers (1, &clothVBOVerticesID); glGenBuffers (1, &clothVBOIndicesID); glBindVertexArray(clothVAOID); glBindBuffer (GL_ARRAY_BUFFER, clothVBOVerticesID); glBufferData (GL_ARRAY_BUFFER, sizeof(float)*4*X.size(), &X[0].x, GL_STATIC_DRAW); glEnableVertexAttribArray(0); glVertexAttribPointer (0, 4, GL_FLOAT, GL_FALSE,0,0); glBindBuffer(GL_ELEMENT_ARRAY_BUFFER, clothVBOIndicesID); glBufferData(GL_ELEMENT_ARRAY_BUFFER, sizeof(GLushort)*indices.size(), &indices[0], GL_STATIC_DRAW); glBindVertexArray(0);
- Create two pairs of vertex array objects (VAO), one pair for rendering and another pair for update of points. Bind two buffer objects (containing current positions and previous positions) to the update VAO, and one buffer object (containing current positions) to the render VAO. Also attach an element array buffer for geometry indices. Set the buffer object usage parameter as
GL_DYNAMIC_COPY
). This usage parameter hints to the GPU that the contents of the buffer object will be frequently changed, and it will be read in OpenGL or used as a source for GL commands:glGenVertexArrays(2, vaoUpdateID); glGenVertexArrays(2, vaoRenderID); glGenBuffers( 2, vboID_Pos); glGenBuffers( 2, vboID_PrePos); for(int i=0;i<2;i++) { glBindVertexArray(vaoUpdateID[i]); glBindBuffer( GL_ARRAY_BUFFER, vboID_Pos[i]); glBufferData( GL_ARRAY_BUFFER, X.size()* sizeof(glm::vec4), &(X[0].x), GL_DYNAMIC_COPY); glEnableVertexAttribArray(0); glVertexAttribPointer(0, 4, GL_FLOAT, GL_FALSE, 0, 0); glBindBuffer( GL_ARRAY_BUFFER, vboID_PrePos[i]); glBufferData( GL_ARRAY_BUFFER, X_last.size()*sizeof(glm::vec4), &(X_last[0].x), GL_DYNAMIC_COPY); glEnableVertexAttribArray(1); glVertexAttribPointer(1, 4, GL_FLOAT, GL_FALSE, 0,0); } //set render vao for(int i=0;i<2;i++) { glBindVertexArray(vaoRenderID[i]); glBindBuffer( GL_ARRAY_BUFFER, vboID_Pos[i]); glEnableVertexAttribArray(0); glVertexAttribPointer(0, 4, GL_FLOAT, GL_FALSE, 0, 0); glBindBuffer(GL_ELEMENT_ARRAY_BUFFER, vboIndices); if(i==0) glBufferData(GL_ELEMENT_ARRAY_BUFFER, indices.size()*sizeof(GLushort), &indices[0], GL_STATIC_DRAW); }
- For ease of access in the vertex shader, bind the current and previous position buffer objects to a set of buffer textures. The buffer textures are one dimensional textures that are created like normal OpenGL textures using the
glGenTextures
call, but they are bound to theGL_TEXTURE_BUFFER
target. They provide read access to the entire buffer object memory in the vertex shader. The data is accessed in the vertex shader using thetexelFetchBuffer
function:for(int i=0;i<2;i++) { glBindTexture( GL_TEXTURE_BUFFER, texPosID[i]); glTexBuffer( GL_TEXTURE_BUFFER, GL_RGBA32F, vboID_Pos[i]); glBindTexture( GL_TEXTURE_BUFFER, texPrePosID[i]); glTexBuffer(GL_TEXTURE_BUFFER, GL_RGBA32F, vboID_PrePos[i]); }
- Generate a transform feedback object and pass the attribute names that will be output from our deformation vertex shader. Make sure to relink the program.
glGenTransformFeedbacks(1, &tfID); glBindTransformFeedback(GL_TRANSFORM_FEEDBACK, tfID); const char* varying_names[]={"out_position_mass", "out_prev_position"}; glTransformFeedbackVaryings(massSpringShader.GetProgram(), 2, varying_names, GL_SEPARATE_ATTRIBS); glLinkProgram(massSpringShader.GetProgram());
- In the rendering function, bind the cloth deformation shader (
Chapter8/TransformFeedbackCloth/shaders/Spring.vert
) and then run a loop. In each loop iteration, bind the texture buffers, and then bind the update vertex array object. At the same time, bind the previous buffer objects as the transform feedback buffers. These will store the output from the vertex shader. Disable the rasterizer, begin the transform feedback mode, and then draw the entire set of cloth vertices. Use the ping pong approach to swap the read/write pathways:massSpringShader.Use(); glUniformMatrix4fv(massSpringShader("MVP"), 1, GL_FALSE, glm::value_ptr(mMVP)); for(int i=0;i<NUM_ITER;i++) { glActiveTexture( GL_TEXTURE0); glBindTexture( GL_TEXTURE_BUFFER, texPosID[writeID]); glActiveTexture( GL_TEXTURE1); glBindTexture( GL_TEXTURE_BUFFER, texPrePosID[writeID]); glBindVertexArray( vaoUpdateID[writeID]); glBindBufferBase(GL_TRANSFORM_FEEDBACK_BUFFER, 0, vboID_Pos[readID]); glBindBufferBase(GL_TRANSFORM_FEEDBACK_BUFFER, 1, vboID_PrePos[readID]); glEnable(GL_RASTERIZER_DISCARD); // disable rasrization glBeginQuery(GL_TIME_ELAPSED,t_query); glBeginTransformFeedback(GL_POINTS); glDrawArrays(GL_POINTS, 0, total_points); glEndTransformFeedback(); glEndQuery(GL_TIME_ELAPSED); glFlush(); glDisable(GL_RASTERIZER_DISCARD); int tmp = readID; readID=writeID; writeID = tmp; } glGetQueryObjectui64v(t_query, GL_QUERY_RESULT, &elapsed_time); delta_time = elapsed_time / 1000000.0f; massSpringShader.UnUse();
- After the loop is terminated, bind the render VAO that renders the cloth geometry and vertices:
glBindVertexArray(vaoRenderID[writeID]); glDisable(GL_DEPTH_TEST); renderShader.Use(); glUniformMatrix4fv(renderShader("MVP"), 1, GL_FALSE, glm::value_ptr(mMVP)); glDrawElements(GL_TRIANGLES, indices.size(), GL_UNSIGNED_SHORT,0); renderShader.UnUse(); glEnable(GL_DEPTH_TEST); if(bDisplayMasses) { particleShader.Use(); glUniform1i(particleShader("selected_index"), selected_index); glUniformMatrix4fv(particleShader("MV"), 1, GL_FALSE, glm::value_ptr(mMV)); glUniformMatrix4fv(particleShader("MVP"), 1, GL_FALSE, glm::value_ptr(mMVP)); glDrawArrays(GL_POINTS, 0, total_points); particleShader.UnUse(); } glBindVertexArray( 0);
- In the vertex shader, obtain the current and previous position of the cloth vertex. If the vertex is a pinned vertex, set its mass to
0
so it would not be simulated; otherwise, add an external force based on gravity. Next loop through all neighbors of the current vertex by looking up the texture buffer and estimate the internal force:float m = position_mass.w; vec3 pos = position_mass.xyz; vec3 pos_old = prev_position.xyz; vec3 vel = (pos - pos_old) / dt; float ks=0, kd=0; int index = gl_VertexID; int ix = index % texsize_x; int iy = index / texsize_x; if(index ==0 || index == (texsize_x-1)) m = 0; vec3 F = gravity*m + (DEFAULT_DAMPING*vel); for(int k=0;k<12;k++) { ivec2 coord = getNextNeighbor(k, ks, kd); int j = coord.x; int i = coord.y; if (((iy + i) < 0) || ((iy + i) > (texsize_y-1))) continue; if (((ix + j) < 0) || ((ix + j) > (texsize_x-1))) continue; int index_neigh = (iy + i) * texsize_x + ix + j; vec3 p2 = texelFetchBuffer(tex_position_mass, index_neigh).xyz; vec3 p2_last = texelFetchBuffer(tex_prev_position_mass, index_neigh).xyz; vec2 coord_neigh = vec2(ix + j, iy + i)*step; float rest_length = length(coord*inv_cloth_size); vec3 v2 = (p2- p2_last)/dt; vec3 deltaP = pos - p2; vec3 deltaV = vel - v2; float dist = length(deltaP); float leftTerm = -ks * (dist-rest_length); float rightTerm = kd * (dot(deltaV, deltaP)/dist); vec3 springForce = (leftTerm + rightTerm)* normalize(deltaP); F += springForce; }
- Using the combined force, calculate the acceleration and then estimate the new position using Verlet integration. Output the appropriate attribute from the shader:
vec3 acc = vec3(0); if(m!=0) acc = F/m; vec3 tmp = pos; pos = pos * 2.0 - pos_old + acc* dt * dt; pos_old = tmp; pos.y=max(0, pos.y); out_position_mass = vec4(pos, m); out_prev_position = vec4(pos_old,m); gl_Position = MVP*vec4(pos, 1);
There are two parts of this recipe, the generation of geometry and identifying output attributes for transform feedback buffers. We first generate the cloth geometry and then associate our buffer objects. To enable easier access of current and previous positions, we bind the position buffer objects as texture buffers.
Next, the acceleration due to gravity and velocity damping force is applied. After this, a loop is run which basically loops through all of the neighbors of the current vertex and estimates the net internal (spring) force. This force is then added to the combined force for the current vertex:
The shader, along with the transform feedback mechanism, proceeds to deform all of the cloth vertices and in the end, we get the cloth vertices deformed.
X
and X_last
store the current and last position respectively, and the vector F
stores the force for each vertex:vector<GLushort> indices; vector<glm::vec4> X; vector<glm::vec4> X_last; vector<glm::vec3> F; indices.resize( numX*numY*2*3); X.resize(total_points); X_last.resize(total_points); F.resize(total_points); for(int j=0;j<=numY;j++) { for(int i=0;i<=numX;i++) { X[count] = glm::vec4( ((float(i)/(u-1)) *2-1)* hsize, sizeX+1, ((float(j)/(v-1) )* sizeY),1); X_last[count] = X[count]; count++; } } GLushort* id=&indices[0]; for (int i = 0; i < numY; i++) { for (int j = 0; j < numX; j++) { int i0 = i * (numX+1) + j; int i1 = i0 + 1; int i2 = i0 + (numX+1); int i3 = i2 + 1; if ((j+i)%2) { *id++ = i0; *id++ = i2; *id++ = i1; *id++ = i1; *id++ = i2; *id++ = i3; } else { *id++ = i0; *id++ = i2; *id++ = i3; *id++ = i0; *id++ = i3; *id++ = i1; } } } glGenVertexArrays(1, &clothVAOID); glGenBuffers (1, &clothVBOVerticesID); glGenBuffers (1, &clothVBOIndicesID); glBindVertexArray(clothVAOID); glBindBuffer (GL_ARRAY_BUFFER, clothVBOVerticesID); glBufferData (GL_ARRAY_BUFFER, sizeof(float)*4*X.size(), &X[0].x, GL_STATIC_DRAW); glEnableVertexAttribArray(0); glVertexAttribPointer (0, 4, GL_FLOAT, GL_FALSE,0,0); glBindBuffer(GL_ELEMENT_ARRAY_BUFFER, clothVBOIndicesID); glBufferData(GL_ELEMENT_ARRAY_BUFFER, sizeof(GLushort)*indices.size(), &indices[0], GL_STATIC_DRAW); glBindVertexArray(0);
- vertex array objects (VAO), one pair for rendering and another pair for update of points. Bind two buffer objects (containing current positions and previous positions) to the update VAO, and one buffer object (containing current positions) to the render VAO. Also attach an element array buffer for geometry indices. Set the buffer object usage parameter as
GL_DYNAMIC_COPY
). This usage parameter hints to the GPU that the contents of the buffer object will be frequently changed, and it will be read in OpenGL or used as a source for GL commands:glGenVertexArrays(2, vaoUpdateID); glGenVertexArrays(2, vaoRenderID); glGenBuffers( 2, vboID_Pos); glGenBuffers( 2, vboID_PrePos); for(int i=0;i<2;i++) { glBindVertexArray(vaoUpdateID[i]); glBindBuffer( GL_ARRAY_BUFFER, vboID_Pos[i]); glBufferData( GL_ARRAY_BUFFER, X.size()* sizeof(glm::vec4), &(X[0].x), GL_DYNAMIC_COPY); glEnableVertexAttribArray(0); glVertexAttribPointer(0, 4, GL_FLOAT, GL_FALSE, 0, 0); glBindBuffer( GL_ARRAY_BUFFER, vboID_PrePos[i]); glBufferData( GL_ARRAY_BUFFER, X_last.size()*sizeof(glm::vec4), &(X_last[0].x), GL_DYNAMIC_COPY); glEnableVertexAttribArray(1); glVertexAttribPointer(1, 4, GL_FLOAT, GL_FALSE, 0,0); } //set render vao for(int i=0;i<2;i++) { glBindVertexArray(vaoRenderID[i]); glBindBuffer( GL_ARRAY_BUFFER, vboID_Pos[i]); glEnableVertexAttribArray(0); glVertexAttribPointer(0, 4, GL_FLOAT, GL_FALSE, 0, 0); glBindBuffer(GL_ELEMENT_ARRAY_BUFFER, vboIndices); if(i==0) glBufferData(GL_ELEMENT_ARRAY_BUFFER, indices.size()*sizeof(GLushort), &indices[0], GL_STATIC_DRAW); }
- For ease of access in the vertex shader, bind the current and previous position buffer objects to a set of buffer textures. The buffer textures are one dimensional textures that are created like normal OpenGL textures using the
glGenTextures
call, but they are bound to theGL_TEXTURE_BUFFER
target. They provide read access to the entire buffer object memory in the vertex shader. The data is accessed in the vertex shader using thetexelFetchBuffer
function:for(int i=0;i<2;i++) { glBindTexture( GL_TEXTURE_BUFFER, texPosID[i]); glTexBuffer( GL_TEXTURE_BUFFER, GL_RGBA32F, vboID_Pos[i]); glBindTexture( GL_TEXTURE_BUFFER, texPrePosID[i]); glTexBuffer(GL_TEXTURE_BUFFER, GL_RGBA32F, vboID_PrePos[i]); }
- Generate a transform feedback object and pass the attribute names that will be output from our deformation vertex shader. Make sure to relink the program.
glGenTransformFeedbacks(1, &tfID); glBindTransformFeedback(GL_TRANSFORM_FEEDBACK, tfID); const char* varying_names[]={"out_position_mass", "out_prev_position"}; glTransformFeedbackVaryings(massSpringShader.GetProgram(), 2, varying_names, GL_SEPARATE_ATTRIBS); glLinkProgram(massSpringShader.GetProgram());
- In the rendering function, bind the cloth deformation shader (
Chapter8/TransformFeedbackCloth/shaders/Spring.vert
) and then run a loop. In each loop iteration, bind the texture buffers, and then bind the update vertex array object. At the same time, bind the previous buffer objects as the transform feedback buffers. These will store the output from the vertex shader. Disable the rasterizer, begin the transform feedback mode, and then draw the entire set of cloth vertices. Use the ping pong approach to swap the read/write pathways:massSpringShader.Use(); glUniformMatrix4fv(massSpringShader("MVP"), 1, GL_FALSE, glm::value_ptr(mMVP)); for(int i=0;i<NUM_ITER;i++) { glActiveTexture( GL_TEXTURE0); glBindTexture( GL_TEXTURE_BUFFER, texPosID[writeID]); glActiveTexture( GL_TEXTURE1); glBindTexture( GL_TEXTURE_BUFFER, texPrePosID[writeID]); glBindVertexArray( vaoUpdateID[writeID]); glBindBufferBase(GL_TRANSFORM_FEEDBACK_BUFFER, 0, vboID_Pos[readID]); glBindBufferBase(GL_TRANSFORM_FEEDBACK_BUFFER, 1, vboID_PrePos[readID]); glEnable(GL_RASTERIZER_DISCARD); // disable rasrization glBeginQuery(GL_TIME_ELAPSED,t_query); glBeginTransformFeedback(GL_POINTS); glDrawArrays(GL_POINTS, 0, total_points); glEndTransformFeedback(); glEndQuery(GL_TIME_ELAPSED); glFlush(); glDisable(GL_RASTERIZER_DISCARD); int tmp = readID; readID=writeID; writeID = tmp; } glGetQueryObjectui64v(t_query, GL_QUERY_RESULT, &elapsed_time); delta_time = elapsed_time / 1000000.0f; massSpringShader.UnUse();
- After the loop is terminated, bind the render VAO that renders the cloth geometry and vertices:
glBindVertexArray(vaoRenderID[writeID]); glDisable(GL_DEPTH_TEST); renderShader.Use(); glUniformMatrix4fv(renderShader("MVP"), 1, GL_FALSE, glm::value_ptr(mMVP)); glDrawElements(GL_TRIANGLES, indices.size(), GL_UNSIGNED_SHORT,0); renderShader.UnUse(); glEnable(GL_DEPTH_TEST); if(bDisplayMasses) { particleShader.Use(); glUniform1i(particleShader("selected_index"), selected_index); glUniformMatrix4fv(particleShader("MV"), 1, GL_FALSE, glm::value_ptr(mMV)); glUniformMatrix4fv(particleShader("MVP"), 1, GL_FALSE, glm::value_ptr(mMVP)); glDrawArrays(GL_POINTS, 0, total_points); particleShader.UnUse(); } glBindVertexArray( 0);
- In the vertex shader, obtain the current and previous position of the cloth vertex. If the vertex is a pinned vertex, set its mass to
0
so it would not be simulated; otherwise, add an external force based on gravity. Next loop through all neighbors of the current vertex by looking up the texture buffer and estimate the internal force:float m = position_mass.w; vec3 pos = position_mass.xyz; vec3 pos_old = prev_position.xyz; vec3 vel = (pos - pos_old) / dt; float ks=0, kd=0; int index = gl_VertexID; int ix = index % texsize_x; int iy = index / texsize_x; if(index ==0 || index == (texsize_x-1)) m = 0; vec3 F = gravity*m + (DEFAULT_DAMPING*vel); for(int k=0;k<12;k++) { ivec2 coord = getNextNeighbor(k, ks, kd); int j = coord.x; int i = coord.y; if (((iy + i) < 0) || ((iy + i) > (texsize_y-1))) continue; if (((ix + j) < 0) || ((ix + j) > (texsize_x-1))) continue; int index_neigh = (iy + i) * texsize_x + ix + j; vec3 p2 = texelFetchBuffer(tex_position_mass, index_neigh).xyz; vec3 p2_last = texelFetchBuffer(tex_prev_position_mass, index_neigh).xyz; vec2 coord_neigh = vec2(ix + j, iy + i)*step; float rest_length = length(coord*inv_cloth_size); vec3 v2 = (p2- p2_last)/dt; vec3 deltaP = pos - p2; vec3 deltaV = vel - v2; float dist = length(deltaP); float leftTerm = -ks * (dist-rest_length); float rightTerm = kd * (dot(deltaV, deltaP)/dist); vec3 springForce = (leftTerm + rightTerm)* normalize(deltaP); F += springForce; }
- Using the combined force, calculate the acceleration and then estimate the new position using Verlet integration. Output the appropriate attribute from the shader:
vec3 acc = vec3(0); if(m!=0) acc = F/m; vec3 tmp = pos; pos = pos * 2.0 - pos_old + acc* dt * dt; pos_old = tmp; pos.y=max(0, pos.y); out_position_mass = vec4(pos, m); out_prev_position = vec4(pos_old,m); gl_Position = MVP*vec4(pos, 1);
There are two parts of this recipe, the generation of geometry and identifying output attributes for transform feedback buffers. We first generate the cloth geometry and then associate our buffer objects. To enable easier access of current and previous positions, we bind the position buffer objects as texture buffers.
Next, the acceleration due to gravity and velocity damping force is applied. After this, a loop is run which basically loops through all of the neighbors of the current vertex and estimates the net internal (spring) force. This force is then added to the combined force for the current vertex:
The shader, along with the transform feedback mechanism, proceeds to deform all of the cloth vertices and in the end, we get the cloth vertices deformed.
parts of this recipe, the generation of geometry and identifying output attributes for transform feedback buffers. We first generate the cloth geometry and then associate our buffer objects. To enable easier access of current and previous positions, we bind the position buffer objects as texture buffers.
Next, the acceleration due to gravity and velocity damping force is applied. After this, a loop is run which basically loops through all of the neighbors of the current vertex and estimates the net internal (spring) force. This force is then added to the combined force for the current vertex:
The shader, along with the transform feedback mechanism, proceeds to deform all of the cloth vertices and in the end, we get the cloth vertices deformed.
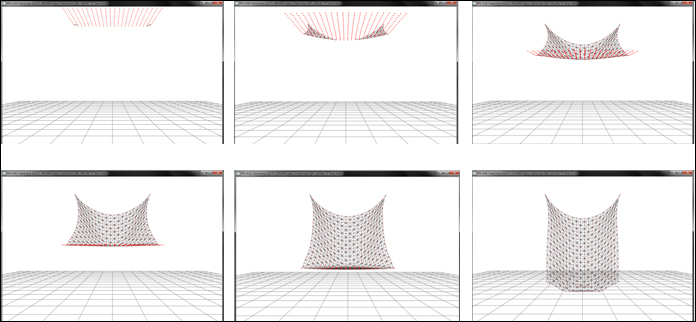
In this recipe, we will build on top of the previous recipe and add collision detection and response to the cloth model.
Let us start this recipe by following these simple steps:
- Generate the geometry and topology for a piece of cloth by creating a set of points and their connectivity. Bind this data to a buffer object as in the previous recipe.
- Set up a pair of vertex array objects and buffer objects as in the previous recipe. Also attach buffer textures for easier access to the buffer object memory in the vertex shader.
- Generate a transform feedback object and pass the attribute names that will be output from our deformation vertex shader. Make sure to relink the program again:
glGenTransformFeedbacks(1, &tfID); glBindTransformFeedback(GL_TRANSFORM_FEEDBACK, tfID); const char* varying_names[]={"out_position_mass", "out_prev_position"}; glTransformFeedbackVaryings(massSpringShader.GetProgram(), 2, varying_names, GL_SEPARATE_ATTRIBS); glLinkProgram(massSpringShader.GetProgram());
- Generate an ellipsoid object by using a simple 4×4 matrix. Also store the inverse of the ellipsoid's transform. The location of the ellipsoid is stored by the translate matrix, the orientation by the rotate matrix, and the non-uniform scaling by the scale matrix as follows. When applied, the matrices work in the opposite order. The non-uniform scaling causes the sphere to compress in the Z direction first. Then, the rotation orients the ellipsoid such that it is rotated by 45 degrees on the X axis. Finally, the ellipsoid is shifted by 2 units on the Y axis:
ellipsoid = glm::translate(glm::mat4(1),glm::vec3(0,2,0)); ellipsoid = glm::rotate(ellipsoid, 45.0f ,glm::vec3(1,0,0)); ellipsoid = glm::scale(ellipsoid, glm::vec3(fRadius,fRadius,fRadius/2)); inverse_ellipsoid = glm::inverse(ellipsoid);
- In the rendering function, bind the cloth deformation shader (
Chapter8/ TransformFeedbackClothCollision/shaders/Spring.vert
) and then run a loop. In each iteration, bind the texture buffers, and then bind the update vertex array object. At the same time, bind the previous buffer objects as the transform feedback buffers. Do the ping pong strategy as in the previous recipe. - After the loop is terminated, bind the render VAO and render the cloth:
glBindVertexArray(vaoRenderID[writeID]); glDisable(GL_DEPTH_TEST); renderShader.Use(); glUniformMatrix4fv(renderShader("MVP"), 1, GL_FALSE, glm::value_ptr(mMVP)); glDrawElements(GL_TRIANGLES, indices.size(), GL_UNSIGNED_SHORT,0); renderShader.UnUse(); glEnable(GL_DEPTH_TEST); if(bDisplayMasses) { particleShader.Use(); glUniform1i(particleShader("selected_index"), selected_index); glUniformMatrix4fv(particleShader("MV"), 1, GL_FALSE, glm::value_ptr(mMV)); glUniformMatrix4fv(particleShader("MVP"), 1, GL_FALSE, glm::value_ptr(mMVP)); glDrawArrays(GL_POINTS, 0, total_points); particleShader.UnUse(); } glBindVertexArray( 0);
- In the vertex shader, obtain the current and previous position of the cloth vertex. If the vertex is a pinned vertex, set its mass to
0
so it would not be simulated, otherwise, add an external force based on gravity. Next, loop through all neighbors of the current vertex by looking up the texture buffer and estimate the internal force:float m = position_mass.w; vec3 pos = position_mass.xyz; vec3 pos_old = prev_position.xyz; vec3 vel = (pos - pos_old) / dt; float ks=0, kd=0; int index = gl_VertexID; int ix = index % texsize_x; int iy = index / texsize_x; if(index ==0 || index == (texsize_x-1)) m = 0; vec3 F = gravity*m + (DEFAULT_DAMPING*vel); for(int k=0;k<12;k++) { ivec2 coord = getNextNeighbor(k, ks, kd); int j = coord.x; int i = coord.y; if (((iy + i) < 0) || ((iy + i) > (texsize_y-1))) continue; if (((ix + j) < 0) || ((ix + j) > (texsize_x-1))) continue; int index_neigh = (iy + i) * texsize_x + ix + j; vec3 p2 = texelFetchBuffer(tex_position_mass, index_neigh).xyz; vec3 p2_last = texelFetchBuffer(tex_prev_position_mass, index_neigh).xyz; vec2 coord_neigh = vec2(ix + j, iy + i)*step; float rest_length = length(coord*inv_cloth_size); vec3 v2 = (p2- p2_last)/dt; vec3 deltaP = pos - p2; vec3 deltaV = vel - v2; float dist = length(deltaP); float leftTerm = -ks * (dist-rest_length); float rightTerm = kd * (dot(deltaV, deltaP)/dist); vec3 springForce = (leftTerm + rightTerm)* normalize(deltaP); F += springForce; }
- Using the combined force, calculate the acceleration and then estimate the new position using Verlet integration. Output the appropriate attribute from the shader:
vec3 acc = vec3(0); if(m!=0) acc = F/m; vec3 tmp = pos; pos = pos * 2.0 - pos_old + acc* dt * dt; pos_old = tmp; pos.y=max(0, pos.y);
- After applying the floor collision, check for collision with an ellipsoid. If there is a collision, modify the position such that the collision is resolved. Finally, output the appropriate attributes from the vertex shader.
vec4 x0 = inv_ellipsoid*vec4(pos,1); vec3 delta0 = x0.xyz-ellipsoid.xyz; float dist2 = dot(delta0, delta0); if(dist2<1) { delta0 = (ellipsoid.w - dist2) * delta0 / dist2; vec3 delta; vec3 transformInv = vec3(ellipsoid_xform[0].x, ellipsoid_xform[1].x, ellipsoid_xform[2].x); transformInv /= dot(transformInv, transformInv); delta.x = dot(delta0, transformInv); transformInv = vec3(ellipsoid_xform[0].y, ellipsoid_xform[1].y, ellipsoid_xform[2].y); transformInv /= dot(transformInv, transformInv); delta.y = dot(delta0, transformInv); transformInv = vec3(ellipsoid_xform[0].z, ellipsoid_xform[1].z, ellipsoid_xform[2].z); transformInv /= dot(transformInv, transformInv); delta.z = dot(delta0, transformInv); pos += delta ; pos_old = pos; } out_position_mass = vec4(pos, m); out_prev_position = vec4(pos_old,m); gl_Position = MVP*vec4(pos, 1);
The cloth deformation vertex shader has some additional lines of code to enable collision detection and response. For detection of collision with a plane, we can simply put the current position in the plane equation to find the distance of the current vertex from the plane. If it is less than 0, we have passed through the plane, in which case, we can move the vertex back in the plane's normal direction.
The demo application implementing this recipe renders a piece of cloth fixed at two points and is allowed to fall under gravity. In addition, there is an oriented ellipsoid with which the cloth collides as shown in the following figure:
Although we have touched upon basic collision primitives, like spheres, oriented ellipsoids, and plane, more complex primitives can be implemented with the combination of these basic primitives. In addition, polygonal primitives can also be implemented. We leave that as an exercise for the reader.
Chapter8/TransformFeedbackClothCollision
directory. For this recipe, the setup code and rendering code remains the same as in the previous recipe. The only change is the addition of the ellipsoid/sphere collision code.
Let us start this recipe by following these simple steps:
- Generate the geometry and topology for a piece of cloth by creating a set of points and their connectivity. Bind this data to a buffer object as in the previous recipe.
- Set up a pair of vertex array objects and buffer objects as in the previous recipe. Also attach buffer textures for easier access to the buffer object memory in the vertex shader.
- Generate a transform feedback object and pass the attribute names that will be output from our deformation vertex shader. Make sure to relink the program again:
glGenTransformFeedbacks(1, &tfID); glBindTransformFeedback(GL_TRANSFORM_FEEDBACK, tfID); const char* varying_names[]={"out_position_mass", "out_prev_position"}; glTransformFeedbackVaryings(massSpringShader.GetProgram(), 2, varying_names, GL_SEPARATE_ATTRIBS); glLinkProgram(massSpringShader.GetProgram());
- Generate an ellipsoid object by using a simple 4×4 matrix. Also store the inverse of the ellipsoid's transform. The location of the ellipsoid is stored by the translate matrix, the orientation by the rotate matrix, and the non-uniform scaling by the scale matrix as follows. When applied, the matrices work in the opposite order. The non-uniform scaling causes the sphere to compress in the Z direction first. Then, the rotation orients the ellipsoid such that it is rotated by 45 degrees on the X axis. Finally, the ellipsoid is shifted by 2 units on the Y axis:
ellipsoid = glm::translate(glm::mat4(1),glm::vec3(0,2,0)); ellipsoid = glm::rotate(ellipsoid, 45.0f ,glm::vec3(1,0,0)); ellipsoid = glm::scale(ellipsoid, glm::vec3(fRadius,fRadius,fRadius/2)); inverse_ellipsoid = glm::inverse(ellipsoid);
- In the rendering function, bind the cloth deformation shader (
Chapter8/ TransformFeedbackClothCollision/shaders/Spring.vert
) and then run a loop. In each iteration, bind the texture buffers, and then bind the update vertex array object. At the same time, bind the previous buffer objects as the transform feedback buffers. Do the ping pong strategy as in the previous recipe. - After the loop is terminated, bind the render VAO and render the cloth:
glBindVertexArray(vaoRenderID[writeID]); glDisable(GL_DEPTH_TEST); renderShader.Use(); glUniformMatrix4fv(renderShader("MVP"), 1, GL_FALSE, glm::value_ptr(mMVP)); glDrawElements(GL_TRIANGLES, indices.size(), GL_UNSIGNED_SHORT,0); renderShader.UnUse(); glEnable(GL_DEPTH_TEST); if(bDisplayMasses) { particleShader.Use(); glUniform1i(particleShader("selected_index"), selected_index); glUniformMatrix4fv(particleShader("MV"), 1, GL_FALSE, glm::value_ptr(mMV)); glUniformMatrix4fv(particleShader("MVP"), 1, GL_FALSE, glm::value_ptr(mMVP)); glDrawArrays(GL_POINTS, 0, total_points); particleShader.UnUse(); } glBindVertexArray( 0);
- In the vertex shader, obtain the current and previous position of the cloth vertex. If the vertex is a pinned vertex, set its mass to
0
so it would not be simulated, otherwise, add an external force based on gravity. Next, loop through all neighbors of the current vertex by looking up the texture buffer and estimate the internal force:float m = position_mass.w; vec3 pos = position_mass.xyz; vec3 pos_old = prev_position.xyz; vec3 vel = (pos - pos_old) / dt; float ks=0, kd=0; int index = gl_VertexID; int ix = index % texsize_x; int iy = index / texsize_x; if(index ==0 || index == (texsize_x-1)) m = 0; vec3 F = gravity*m + (DEFAULT_DAMPING*vel); for(int k=0;k<12;k++) { ivec2 coord = getNextNeighbor(k, ks, kd); int j = coord.x; int i = coord.y; if (((iy + i) < 0) || ((iy + i) > (texsize_y-1))) continue; if (((ix + j) < 0) || ((ix + j) > (texsize_x-1))) continue; int index_neigh = (iy + i) * texsize_x + ix + j; vec3 p2 = texelFetchBuffer(tex_position_mass, index_neigh).xyz; vec3 p2_last = texelFetchBuffer(tex_prev_position_mass, index_neigh).xyz; vec2 coord_neigh = vec2(ix + j, iy + i)*step; float rest_length = length(coord*inv_cloth_size); vec3 v2 = (p2- p2_last)/dt; vec3 deltaP = pos - p2; vec3 deltaV = vel - v2; float dist = length(deltaP); float leftTerm = -ks * (dist-rest_length); float rightTerm = kd * (dot(deltaV, deltaP)/dist); vec3 springForce = (leftTerm + rightTerm)* normalize(deltaP); F += springForce; }
- Using the combined force, calculate the acceleration and then estimate the new position using Verlet integration. Output the appropriate attribute from the shader:
vec3 acc = vec3(0); if(m!=0) acc = F/m; vec3 tmp = pos; pos = pos * 2.0 - pos_old + acc* dt * dt; pos_old = tmp; pos.y=max(0, pos.y);
- After applying the floor collision, check for collision with an ellipsoid. If there is a collision, modify the position such that the collision is resolved. Finally, output the appropriate attributes from the vertex shader.
vec4 x0 = inv_ellipsoid*vec4(pos,1); vec3 delta0 = x0.xyz-ellipsoid.xyz; float dist2 = dot(delta0, delta0); if(dist2<1) { delta0 = (ellipsoid.w - dist2) * delta0 / dist2; vec3 delta; vec3 transformInv = vec3(ellipsoid_xform[0].x, ellipsoid_xform[1].x, ellipsoid_xform[2].x); transformInv /= dot(transformInv, transformInv); delta.x = dot(delta0, transformInv); transformInv = vec3(ellipsoid_xform[0].y, ellipsoid_xform[1].y, ellipsoid_xform[2].y); transformInv /= dot(transformInv, transformInv); delta.y = dot(delta0, transformInv); transformInv = vec3(ellipsoid_xform[0].z, ellipsoid_xform[1].z, ellipsoid_xform[2].z); transformInv /= dot(transformInv, transformInv); delta.z = dot(delta0, transformInv); pos += delta ; pos_old = pos; } out_position_mass = vec4(pos, m); out_prev_position = vec4(pos_old,m); gl_Position = MVP*vec4(pos, 1);
The cloth deformation vertex shader has some additional lines of code to enable collision detection and response. For detection of collision with a plane, we can simply put the current position in the plane equation to find the distance of the current vertex from the plane. If it is less than 0, we have passed through the plane, in which case, we can move the vertex back in the plane's normal direction.
The demo application implementing this recipe renders a piece of cloth fixed at two points and is allowed to fall under gravity. In addition, there is an oriented ellipsoid with which the cloth collides as shown in the following figure:
Although we have touched upon basic collision primitives, like spheres, oriented ellipsoids, and plane, more complex primitives can be implemented with the combination of these basic primitives. In addition, polygonal primitives can also be implemented. We leave that as an exercise for the reader.
glGenTransformFeedbacks(1, &tfID); glBindTransformFeedback(GL_TRANSFORM_FEEDBACK, tfID); const char* varying_names[]={"out_position_mass", "out_prev_position"}; glTransformFeedbackVaryings(massSpringShader.GetProgram(), 2, varying_names, GL_SEPARATE_ATTRIBS); glLinkProgram(massSpringShader.GetProgram());
ellipsoid = glm::translate(glm::mat4(1),glm::vec3(0,2,0)); ellipsoid = glm::rotate(ellipsoid, 45.0f ,glm::vec3(1,0,0)); ellipsoid = glm::scale(ellipsoid, glm::vec3(fRadius,fRadius,fRadius/2)); inverse_ellipsoid = glm::inverse(ellipsoid);
Chapter8/ TransformFeedbackClothCollision/shaders/Spring.vert
) and then run a loop. In
- each iteration, bind the texture buffers, and then bind the update vertex array object. At the same time, bind the previous buffer objects as the transform feedback buffers. Do the ping pong strategy as in the previous recipe.
- After the loop is terminated, bind the render VAO and render the cloth:
glBindVertexArray(vaoRenderID[writeID]); glDisable(GL_DEPTH_TEST); renderShader.Use(); glUniformMatrix4fv(renderShader("MVP"), 1, GL_FALSE, glm::value_ptr(mMVP)); glDrawElements(GL_TRIANGLES, indices.size(), GL_UNSIGNED_SHORT,0); renderShader.UnUse(); glEnable(GL_DEPTH_TEST); if(bDisplayMasses) { particleShader.Use(); glUniform1i(particleShader("selected_index"), selected_index); glUniformMatrix4fv(particleShader("MV"), 1, GL_FALSE, glm::value_ptr(mMV)); glUniformMatrix4fv(particleShader("MVP"), 1, GL_FALSE, glm::value_ptr(mMVP)); glDrawArrays(GL_POINTS, 0, total_points); particleShader.UnUse(); } glBindVertexArray( 0);
- In the vertex shader, obtain the current and previous position of the cloth vertex. If the vertex is a pinned vertex, set its mass to
0
so it would not be simulated, otherwise, add an external force based on gravity. Next, loop through all neighbors of the current vertex by looking up the texture buffer and estimate the internal force:float m = position_mass.w; vec3 pos = position_mass.xyz; vec3 pos_old = prev_position.xyz; vec3 vel = (pos - pos_old) / dt; float ks=0, kd=0; int index = gl_VertexID; int ix = index % texsize_x; int iy = index / texsize_x; if(index ==0 || index == (texsize_x-1)) m = 0; vec3 F = gravity*m + (DEFAULT_DAMPING*vel); for(int k=0;k<12;k++) { ivec2 coord = getNextNeighbor(k, ks, kd); int j = coord.x; int i = coord.y; if (((iy + i) < 0) || ((iy + i) > (texsize_y-1))) continue; if (((ix + j) < 0) || ((ix + j) > (texsize_x-1))) continue; int index_neigh = (iy + i) * texsize_x + ix + j; vec3 p2 = texelFetchBuffer(tex_position_mass, index_neigh).xyz; vec3 p2_last = texelFetchBuffer(tex_prev_position_mass, index_neigh).xyz; vec2 coord_neigh = vec2(ix + j, iy + i)*step; float rest_length = length(coord*inv_cloth_size); vec3 v2 = (p2- p2_last)/dt; vec3 deltaP = pos - p2; vec3 deltaV = vel - v2; float dist = length(deltaP); float leftTerm = -ks * (dist-rest_length); float rightTerm = kd * (dot(deltaV, deltaP)/dist); vec3 springForce = (leftTerm + rightTerm)* normalize(deltaP); F += springForce; }
- Using the combined force, calculate the acceleration and then estimate the new position using Verlet integration. Output the appropriate attribute from the shader:
vec3 acc = vec3(0); if(m!=0) acc = F/m; vec3 tmp = pos; pos = pos * 2.0 - pos_old + acc* dt * dt; pos_old = tmp; pos.y=max(0, pos.y);
- After applying the floor collision, check for collision with an ellipsoid. If there is a collision, modify the position such that the collision is resolved. Finally, output the appropriate attributes from the vertex shader.
vec4 x0 = inv_ellipsoid*vec4(pos,1); vec3 delta0 = x0.xyz-ellipsoid.xyz; float dist2 = dot(delta0, delta0); if(dist2<1) { delta0 = (ellipsoid.w - dist2) * delta0 / dist2; vec3 delta; vec3 transformInv = vec3(ellipsoid_xform[0].x, ellipsoid_xform[1].x, ellipsoid_xform[2].x); transformInv /= dot(transformInv, transformInv); delta.x = dot(delta0, transformInv); transformInv = vec3(ellipsoid_xform[0].y, ellipsoid_xform[1].y, ellipsoid_xform[2].y); transformInv /= dot(transformInv, transformInv); delta.y = dot(delta0, transformInv); transformInv = vec3(ellipsoid_xform[0].z, ellipsoid_xform[1].z, ellipsoid_xform[2].z); transformInv /= dot(transformInv, transformInv); delta.z = dot(delta0, transformInv); pos += delta ; pos_old = pos; } out_position_mass = vec4(pos, m); out_prev_position = vec4(pos_old,m); gl_Position = MVP*vec4(pos, 1);
The cloth deformation vertex shader has some additional lines of code to enable collision detection and response. For detection of collision with a plane, we can simply put the current position in the plane equation to find the distance of the current vertex from the plane. If it is less than 0, we have passed through the plane, in which case, we can move the vertex back in the plane's normal direction.
The demo application implementing this recipe renders a piece of cloth fixed at two points and is allowed to fall under gravity. In addition, there is an oriented ellipsoid with which the cloth collides as shown in the following figure:
Although we have touched upon basic collision primitives, like spheres, oriented ellipsoids, and plane, more complex primitives can be implemented with the combination of these basic primitives. In addition, polygonal primitives can also be implemented. We leave that as an exercise for the reader.
passed through the plane, in which case, we can move the vertex back in the plane's normal direction.
The demo application implementing this recipe renders a piece of cloth fixed at two points and is allowed to fall under gravity. In addition, there is an oriented ellipsoid with which the cloth collides as shown in the following figure:
Although we have touched upon basic collision primitives, like spheres, oriented ellipsoids, and plane, more complex primitives can be implemented with the combination of these basic primitives. In addition, polygonal primitives can also be implemented. We leave that as an exercise for the reader.
application implementing this recipe renders a piece of cloth fixed at two points and is allowed to fall under gravity. In addition, there is an oriented ellipsoid with which the cloth collides as shown in the following figure:
Although we have touched upon basic collision primitives, like spheres, oriented ellipsoids, and plane, more complex primitives can be implemented with the combination of these basic primitives. In addition, polygonal primitives can also be implemented. We leave that as an exercise for the reader.
In this recipe, we will implement a simple particle system using the transform feedback mechanism. In this mode, the GPU bypasses the rasterizer and, later, the programmable graphics pipeline stages to feedback result to the vertex shader. The benefit from this mode is that using this feature, we can implement a physically-based simulation entirely on the GPU.
Let us start this recipe by following these simple steps:
- Set up two vertex array pairs: one for update and another for rendering. Bind two vertex buffer objects to each of the pairs, as was done in the previous two recipes. Here, the buffer objects will store the per-particle properties. Also, enable the corresponding vertex attributes:
glGenVertexArrays(2, vaoUpdateID); glGenVertexArrays(2, vaoRenderID); glGenBuffers( 2, vboID_Pos); glGenBuffers( 2, vboID_PrePos); glGenBuffers( 2, vboID_Direction); for(int i=0;i<2;i++) { glBindVertexArray(vaoUpdateID[i]); glBindBuffer( GL_ARRAY_BUFFER, vboID_Pos[i]); glBufferData( GL_ARRAY_BUFFER, TOTAL_PARTICLES* sizeof(glm::vec4), 0, GL_DYNAMIC_COPY); glEnableVertexAttribArray(0); glVertexAttribPointer(0, 4, GL_FLOAT, GL_FALSE, 0, 0); glBindBuffer( GL_ARRAY_BUFFER, vboID_PrePos[i]); glBufferData( GL_ARRAY_BUFFER, TOTAL_PARTICLES* sizeof(glm::vec4), 0, GL_DYNAMIC_COPY); glEnableVertexAttribArray(1); glVertexAttribPointer(1, 4, GL_FLOAT, GL_FALSE, 0,0); glBindBuffer( GL_ARRAY_BUFFER, vboID_Direction[i]); glBufferData( GL_ARRAY_BUFFER, TOTAL_PARTICLES* sizeof(glm::vec4), 0, GL_DYNAMIC_COPY); glEnableVertexAttribArray(2); glVertexAttribPointer(2, 4, GL_FLOAT, GL_FALSE, 0,0); } for(int i=0;i<2;i++) { glBindVertexArray(vaoRenderID[i]); glBindBuffer( GL_ARRAY_BUFFER, vboID_Pos[i]); glEnableVertexAttribArray(0); glVertexAttribPointer(0, 4, GL_FLOAT, GL_FALSE, 0, 0); }
- Generate a transform feedback object and bind it. Next, specify the output attributes from the shader that would be stored in the transform feedback buffer. After this step, relink the shader program:
glGenTransformFeedbacks(1, &tfID); glBindTransformFeedback(GL_TRANSFORM_FEEDBACK, tfID); const char* varying_names[]={"out_position", "out_prev_position", "out_direction"}; glTransformFeedbackVaryings(particleShader.GetProgram(), 3, varying_names, GL_SEPARATE_ATTRIBS); glLinkProgram(particleShader.GetProgram());
- In the update function, bind the particle vertex shader that will output to the transform feedback buffer and set the appropriate uniforms and update vertex array object. Note that to enable read/write access, we use a pair of vertex array objects such that we can read from one and write to another:
particleShader.Use(); glUniformMatrix4fv(particleShader("MVP"), 1, GL_FALSE, glm::value_ptr(mMVP)); glUniform1f(particleShader("time"), t); for(int i=0;i<NUM_ITER;i++) { glBindVertexArray( vaoUpdateID[readID]);
- Bind the vertex buffer objects that will store the outputs from the transform feedback step using the output attributes from the vertex shader:
glBindBufferBase(GL_TRANSFORM_FEEDBACK_BUFFER, 0, vboID_Pos[writeID]); glBindBufferBase(GL_TRANSFORM_FEEDBACK_BUFFER, 1, vboID_PrePos[writeID]); glBindBufferBase(GL_TRANSFORM_FEEDBACK_BUFFER, 2, vboID_Direction[writeID]);
- Disable the rasterizer to prevent the execution of the later stages of the pipeline and then begin the transform feedback. Next, issue a call to the
glDrawArrays
function to allow the vertices to be passed to the graphics pipeline. After this step, end the transform feedback and then enable the rasterizer. Note that to correctly determine the amount of execution time needed, we issue a hardware query. Next, we alternate the read/write paths by swapping the read and write IDs:glEnable(GL_RASTERIZER_DISCARD); glBeginQuery(GL_TIME_ELAPSED,t_query); glBeginTransformFeedback(GL_POINTS); glDrawArrays(GL_POINTS, 0, TOTAL_PARTICLES); glEndTransformFeedback(); glEndQuery(GL_TIME_ELAPSED); glFlush(); glDisable(GL_RASTERIZER_DISCARD); int tmp = readID; readID=writeID; writeID = tmp;
- Render the particles using the render shader. First, bind the render vertex array object and then draw the points using the
glDrawArrays
function:glBindVertexArray(vaoRenderID[readID]); renderShader.Use(); glUniformMatrix4fv(renderShader("MVP"), 1, GL_FALSE, glm::value_ptr(mMVP)); glDrawArrays(GL_POINTS, 0, TOTAL_PARTICLES); renderShader.UnUse(); glBindVertexArray(0);
- In the particle vertex shader, check if the particle's life is greater than 0. If so, move the particle and reduce the life. Otherwise, calculate a new random direction and reset the life to spawn the particle from the origin. Refer to
Chapter8/TransformFeedbackParticles/shaders/Particle.vert
for details. After this step, output the appropriate values to the output attributes. The particle vertex shader is defined as follows:#version 330 core precision highp float; #extension EXT_gpu_shader4 : require layout( location = 0 ) in vec4 position; layout( location = 1 ) in vec4 prev_position; layout( location = 2 ) in vec4 direction; uniform mat4 MVP; uniform float time; const float PI = 3.14159; const float TWO_PI = 2*PI; const float PI_BY_2 = PI*0.5; const float PI_BY_4 = PI_BY_2*0.5; //shader outputs out vec4 out_position; out vec4 out_prev_position; out vec4 out_direction; const float DAMPING_COEFFICIENT = 0.9995; const vec3 emitterForce = vec3(0.0f,-0.001f, 0.0f); const vec4 collidor = vec4(0,1,0,0); const vec3 emitterPos = vec3(0); float emitterYaw = (0.0f); float emitterYawVar = TWO_PI; float emitterPitch = PI_BY_2; float emitterPitchVar = PI_BY_4; float emitterSpeed = 0.05f; float emitterSpeedVar = 0.01f; int emitterLife = 60; int emitterLifeVar = 15; const float UINT_MAX = 4294967295.0; void main() { vec3 prevPos = prev_position.xyz; int life = int(prev_position.w); vec3 pos = position.xyz; float speed = position.w; vec3 dir = direction.xyz; if(life > 0) { prevPos = pos; pos += dir*speed; if(dot(pos+emitterPos, collidor.xyz)+ collidor.w <0) { dir = reflect(dir, collidor.xyz); speed *= DAMPING_COEFFICIENT; } dir += emitterForce; life--; } else { uint seed = uint(time + gl_VertexID); life = emitterLife + int(randhashf(seed++, emitterLifeVar)); float yaw = emitterYaw + (randhashf(seed++, emitterYawVar )); float pitch = emitterPitch + randhashf(seed++, emitterPitchVar); RotationToDirection(pitch, yaw, dir); float nspeed = emitterSpeed + (randhashf(seed++, emitterSpeedVar )); dir *= nspeed; pos = emitterPos; prevPos = emitterPos; speed = 1; } out_position = vec4(pos, speed); out_prev_position = vec4(prevPos, life); out_direction = vec4(dir, 0); gl_Position = MVP*vec4(pos, 1); }
The three helper functions
randhash
,randhashf
, andRotationToDirection
are defined as follows:uint randhash(uint seed) { uint i=(seed^12345391u)*2654435769u; i^=(i<<6u)^(i>>26u); i*=2654435769u; i+=(i<<5u)^(i>>12u); return i; } float randhashf(uint seed, float b) { return float(b * randhash(seed)) / UINT_MAX; } void RotationToDirection(float pitch, float yaw, out vec3 direction) { direction.x = -sin(yaw) * cos(pitch); direction.y = sin(pitch); direction.z = cos(pitch) * cos(yaw); }
The transform feedback mechanism allows us to feedback one or more attributes from the vertex shader or geometry shader back to a buffer object. This feedback path could be used for implementing a physically-based simulation. This recipe uses this mechanism to output the particle position after each iteration. After each step, the buffers are swapped and, therefore, it can simulate the particle motion.
To make the particle system, we first set up three pairs of vertex buffer objects that store the per-particle attributes that we input to the vertex shader. These include the particle's position, previous position, life, direction, and speed. These are stored into separate buffer objects for convenience. We could have stored all of these attributes into a single interleaved buffer object. Since we output to the buffer object from our shader, we specify the buffer object usage as GL_DYNAMIC_COPY
. Similarly, we set up a separate vertex array object for rendering the particles:
Next, we specify the shader output attributes that we would like to connect to the transform feedback buffers. We use three outputs, namely out_position
, out_prev_position
, and out_direction
, which output the particle's current position, particle's previous position, and the particle's direction along with the particle's speed, current, and initial life, respectively. We specify that we would connect these to separate buffer objects:
We then run a loop for the number of iterations desired. In the loop, we first bind the update vertex array object and assign the appropriate transform feedback buffer base indices:
If the life is less than 0, we reset the particle's direction of motion to a new random direction. We reset the life to a random value based on the maximum allowed value. The current and previous positions of the particle are reset to the emitter origin and finally, the speed is reset to the default value. We then output the output attributes:
Note that for this demo, we render the particles as points of size 10 units. We could easily change the rendering mode to point sprites with size modified in the vertex shader to give the particles a different look. Also, we can also change the colors and blending modes to achieve various effects. In addition, we could achieve the same result by using one vertex buffer pair with interleaved attributes or two separate transform feedback objects. All of these variants should be straightforward to implement by following the guidelines laid out in this recipe.
We had already looked at a simple approach of simulating GPU-based particle systems using vertex shader in Chapter 5, Mesh Model Formats and Particle Systems, we will now detail pros and cons of each. In Chapter 5 we presented a stateless particle system since all of the attributes (that is, position and velocity) were generated on the fly using the vertex ID, time, and basic kinematic equations on each particle vertex.
As the state of the particle is not stored, we cannot reproduce the same simulation every frame. Hence, collision detection and response are problematic, as we do not have any information of the previous state of the particle, which is often required for collision response. On the contrary, the particle simulation technique presented in this recipe uses a state-preserving particle system. We stored current and previous positions of each particle in buffer objects. In addition, we used transform feedback and a vertex shader for particle simulation on the GPU. As the state of the particle is stored, we can carry out collision detection and response easily.
- OGLDev Tutorial on particle system using transform feedback at http://ogldev.atspace.co.uk/www/tutorial28/tutorial28.html
- OpenGL 4.0 Shading Language Cookbook, Chapter 9, Animation and Particles, the Creating a particle system using transform feedback section, Packt Publishing, 2011.
- Noise based Particles, Part II at The Little Grasshopper, http://prideout.net/blog/?p=67
Chapter8/TransformFeedbackParticles
directory.
Let us start this recipe by following these simple steps:
- Set up two vertex array pairs: one for update and another for rendering. Bind two vertex buffer objects to each of the pairs, as was done in the previous two recipes. Here, the buffer objects will store the per-particle properties. Also, enable the corresponding vertex attributes:
glGenVertexArrays(2, vaoUpdateID); glGenVertexArrays(2, vaoRenderID); glGenBuffers( 2, vboID_Pos); glGenBuffers( 2, vboID_PrePos); glGenBuffers( 2, vboID_Direction); for(int i=0;i<2;i++) { glBindVertexArray(vaoUpdateID[i]); glBindBuffer( GL_ARRAY_BUFFER, vboID_Pos[i]); glBufferData( GL_ARRAY_BUFFER, TOTAL_PARTICLES* sizeof(glm::vec4), 0, GL_DYNAMIC_COPY); glEnableVertexAttribArray(0); glVertexAttribPointer(0, 4, GL_FLOAT, GL_FALSE, 0, 0); glBindBuffer( GL_ARRAY_BUFFER, vboID_PrePos[i]); glBufferData( GL_ARRAY_BUFFER, TOTAL_PARTICLES* sizeof(glm::vec4), 0, GL_DYNAMIC_COPY); glEnableVertexAttribArray(1); glVertexAttribPointer(1, 4, GL_FLOAT, GL_FALSE, 0,0); glBindBuffer( GL_ARRAY_BUFFER, vboID_Direction[i]); glBufferData( GL_ARRAY_BUFFER, TOTAL_PARTICLES* sizeof(glm::vec4), 0, GL_DYNAMIC_COPY); glEnableVertexAttribArray(2); glVertexAttribPointer(2, 4, GL_FLOAT, GL_FALSE, 0,0); } for(int i=0;i<2;i++) { glBindVertexArray(vaoRenderID[i]); glBindBuffer( GL_ARRAY_BUFFER, vboID_Pos[i]); glEnableVertexAttribArray(0); glVertexAttribPointer(0, 4, GL_FLOAT, GL_FALSE, 0, 0); }
- Generate a transform feedback object and bind it. Next, specify the output attributes from the shader that would be stored in the transform feedback buffer. After this step, relink the shader program:
glGenTransformFeedbacks(1, &tfID); glBindTransformFeedback(GL_TRANSFORM_FEEDBACK, tfID); const char* varying_names[]={"out_position", "out_prev_position", "out_direction"}; glTransformFeedbackVaryings(particleShader.GetProgram(), 3, varying_names, GL_SEPARATE_ATTRIBS); glLinkProgram(particleShader.GetProgram());
- In the update function, bind the particle vertex shader that will output to the transform feedback buffer and set the appropriate uniforms and update vertex array object. Note that to enable read/write access, we use a pair of vertex array objects such that we can read from one and write to another:
particleShader.Use(); glUniformMatrix4fv(particleShader("MVP"), 1, GL_FALSE, glm::value_ptr(mMVP)); glUniform1f(particleShader("time"), t); for(int i=0;i<NUM_ITER;i++) { glBindVertexArray( vaoUpdateID[readID]);
- Bind the vertex buffer objects that will store the outputs from the transform feedback step using the output attributes from the vertex shader:
glBindBufferBase(GL_TRANSFORM_FEEDBACK_BUFFER, 0, vboID_Pos[writeID]); glBindBufferBase(GL_TRANSFORM_FEEDBACK_BUFFER, 1, vboID_PrePos[writeID]); glBindBufferBase(GL_TRANSFORM_FEEDBACK_BUFFER, 2, vboID_Direction[writeID]);
- Disable the rasterizer to prevent the execution of the later stages of the pipeline and then begin the transform feedback. Next, issue a call to the
glDrawArrays
function to allow the vertices to be passed to the graphics pipeline. After this step, end the transform feedback and then enable the rasterizer. Note that to correctly determine the amount of execution time needed, we issue a hardware query. Next, we alternate the read/write paths by swapping the read and write IDs:glEnable(GL_RASTERIZER_DISCARD); glBeginQuery(GL_TIME_ELAPSED,t_query); glBeginTransformFeedback(GL_POINTS); glDrawArrays(GL_POINTS, 0, TOTAL_PARTICLES); glEndTransformFeedback(); glEndQuery(GL_TIME_ELAPSED); glFlush(); glDisable(GL_RASTERIZER_DISCARD); int tmp = readID; readID=writeID; writeID = tmp;
- Render the particles using the render shader. First, bind the render vertex array object and then draw the points using the
glDrawArrays
function:glBindVertexArray(vaoRenderID[readID]); renderShader.Use(); glUniformMatrix4fv(renderShader("MVP"), 1, GL_FALSE, glm::value_ptr(mMVP)); glDrawArrays(GL_POINTS, 0, TOTAL_PARTICLES); renderShader.UnUse(); glBindVertexArray(0);
- In the particle vertex shader, check if the particle's life is greater than 0. If so, move the particle and reduce the life. Otherwise, calculate a new random direction and reset the life to spawn the particle from the origin. Refer to
Chapter8/TransformFeedbackParticles/shaders/Particle.vert
for details. After this step, output the appropriate values to the output attributes. The particle vertex shader is defined as follows:#version 330 core precision highp float; #extension EXT_gpu_shader4 : require layout( location = 0 ) in vec4 position; layout( location = 1 ) in vec4 prev_position; layout( location = 2 ) in vec4 direction; uniform mat4 MVP; uniform float time; const float PI = 3.14159; const float TWO_PI = 2*PI; const float PI_BY_2 = PI*0.5; const float PI_BY_4 = PI_BY_2*0.5; //shader outputs out vec4 out_position; out vec4 out_prev_position; out vec4 out_direction; const float DAMPING_COEFFICIENT = 0.9995; const vec3 emitterForce = vec3(0.0f,-0.001f, 0.0f); const vec4 collidor = vec4(0,1,0,0); const vec3 emitterPos = vec3(0); float emitterYaw = (0.0f); float emitterYawVar = TWO_PI; float emitterPitch = PI_BY_2; float emitterPitchVar = PI_BY_4; float emitterSpeed = 0.05f; float emitterSpeedVar = 0.01f; int emitterLife = 60; int emitterLifeVar = 15; const float UINT_MAX = 4294967295.0; void main() { vec3 prevPos = prev_position.xyz; int life = int(prev_position.w); vec3 pos = position.xyz; float speed = position.w; vec3 dir = direction.xyz; if(life > 0) { prevPos = pos; pos += dir*speed; if(dot(pos+emitterPos, collidor.xyz)+ collidor.w <0) { dir = reflect(dir, collidor.xyz); speed *= DAMPING_COEFFICIENT; } dir += emitterForce; life--; } else { uint seed = uint(time + gl_VertexID); life = emitterLife + int(randhashf(seed++, emitterLifeVar)); float yaw = emitterYaw + (randhashf(seed++, emitterYawVar )); float pitch = emitterPitch + randhashf(seed++, emitterPitchVar); RotationToDirection(pitch, yaw, dir); float nspeed = emitterSpeed + (randhashf(seed++, emitterSpeedVar )); dir *= nspeed; pos = emitterPos; prevPos = emitterPos; speed = 1; } out_position = vec4(pos, speed); out_prev_position = vec4(prevPos, life); out_direction = vec4(dir, 0); gl_Position = MVP*vec4(pos, 1); }
The three helper functions
randhash
,randhashf
, andRotationToDirection
are defined as follows:uint randhash(uint seed) { uint i=(seed^12345391u)*2654435769u; i^=(i<<6u)^(i>>26u); i*=2654435769u; i+=(i<<5u)^(i>>12u); return i; } float randhashf(uint seed, float b) { return float(b * randhash(seed)) / UINT_MAX; } void RotationToDirection(float pitch, float yaw, out vec3 direction) { direction.x = -sin(yaw) * cos(pitch); direction.y = sin(pitch); direction.z = cos(pitch) * cos(yaw); }
The transform feedback mechanism allows us to feedback one or more attributes from the vertex shader or geometry shader back to a buffer object. This feedback path could be used for implementing a physically-based simulation. This recipe uses this mechanism to output the particle position after each iteration. After each step, the buffers are swapped and, therefore, it can simulate the particle motion.
To make the particle system, we first set up three pairs of vertex buffer objects that store the per-particle attributes that we input to the vertex shader. These include the particle's position, previous position, life, direction, and speed. These are stored into separate buffer objects for convenience. We could have stored all of these attributes into a single interleaved buffer object. Since we output to the buffer object from our shader, we specify the buffer object usage as GL_DYNAMIC_COPY
. Similarly, we set up a separate vertex array object for rendering the particles:
Next, we specify the shader output attributes that we would like to connect to the transform feedback buffers. We use three outputs, namely out_position
, out_prev_position
, and out_direction
, which output the particle's current position, particle's previous position, and the particle's direction along with the particle's speed, current, and initial life, respectively. We specify that we would connect these to separate buffer objects:
We then run a loop for the number of iterations desired. In the loop, we first bind the update vertex array object and assign the appropriate transform feedback buffer base indices:
If the life is less than 0, we reset the particle's direction of motion to a new random direction. We reset the life to a random value based on the maximum allowed value. The current and previous positions of the particle are reset to the emitter origin and finally, the speed is reset to the default value. We then output the output attributes:
Note that for this demo, we render the particles as points of size 10 units. We could easily change the rendering mode to point sprites with size modified in the vertex shader to give the particles a different look. Also, we can also change the colors and blending modes to achieve various effects. In addition, we could achieve the same result by using one vertex buffer pair with interleaved attributes or two separate transform feedback objects. All of these variants should be straightforward to implement by following the guidelines laid out in this recipe.
We had already looked at a simple approach of simulating GPU-based particle systems using vertex shader in Chapter 5, Mesh Model Formats and Particle Systems, we will now detail pros and cons of each. In Chapter 5 we presented a stateless particle system since all of the attributes (that is, position and velocity) were generated on the fly using the vertex ID, time, and basic kinematic equations on each particle vertex.
As the state of the particle is not stored, we cannot reproduce the same simulation every frame. Hence, collision detection and response are problematic, as we do not have any information of the previous state of the particle, which is often required for collision response. On the contrary, the particle simulation technique presented in this recipe uses a state-preserving particle system. We stored current and previous positions of each particle in buffer objects. In addition, we used transform feedback and a vertex shader for particle simulation on the GPU. As the state of the particle is stored, we can carry out collision detection and response easily.
- OGLDev Tutorial on particle system using transform feedback at http://ogldev.atspace.co.uk/www/tutorial28/tutorial28.html
- OpenGL 4.0 Shading Language Cookbook, Chapter 9, Animation and Particles, the Creating a particle system using transform feedback section, Packt Publishing, 2011.
- Noise based Particles, Part II at The Little Grasshopper, http://prideout.net/blog/?p=67
recipe by following these simple steps:
- Set up two vertex array pairs: one for update and another for rendering. Bind two vertex buffer objects to each of the pairs, as was done in the previous two recipes. Here, the buffer objects will store the per-particle properties. Also, enable the corresponding vertex attributes:
glGenVertexArrays(2, vaoUpdateID); glGenVertexArrays(2, vaoRenderID); glGenBuffers( 2, vboID_Pos); glGenBuffers( 2, vboID_PrePos); glGenBuffers( 2, vboID_Direction); for(int i=0;i<2;i++) { glBindVertexArray(vaoUpdateID[i]); glBindBuffer( GL_ARRAY_BUFFER, vboID_Pos[i]); glBufferData( GL_ARRAY_BUFFER, TOTAL_PARTICLES* sizeof(glm::vec4), 0, GL_DYNAMIC_COPY); glEnableVertexAttribArray(0); glVertexAttribPointer(0, 4, GL_FLOAT, GL_FALSE, 0, 0); glBindBuffer( GL_ARRAY_BUFFER, vboID_PrePos[i]); glBufferData( GL_ARRAY_BUFFER, TOTAL_PARTICLES* sizeof(glm::vec4), 0, GL_DYNAMIC_COPY); glEnableVertexAttribArray(1); glVertexAttribPointer(1, 4, GL_FLOAT, GL_FALSE, 0,0); glBindBuffer( GL_ARRAY_BUFFER, vboID_Direction[i]); glBufferData( GL_ARRAY_BUFFER, TOTAL_PARTICLES* sizeof(glm::vec4), 0, GL_DYNAMIC_COPY); glEnableVertexAttribArray(2); glVertexAttribPointer(2, 4, GL_FLOAT, GL_FALSE, 0,0); } for(int i=0;i<2;i++) { glBindVertexArray(vaoRenderID[i]); glBindBuffer( GL_ARRAY_BUFFER, vboID_Pos[i]); glEnableVertexAttribArray(0); glVertexAttribPointer(0, 4, GL_FLOAT, GL_FALSE, 0, 0); }
- Generate a transform feedback object and bind it. Next, specify the output attributes from the shader that would be stored in the transform feedback buffer. After this step, relink the shader program:
glGenTransformFeedbacks(1, &tfID); glBindTransformFeedback(GL_TRANSFORM_FEEDBACK, tfID); const char* varying_names[]={"out_position", "out_prev_position", "out_direction"}; glTransformFeedbackVaryings(particleShader.GetProgram(), 3, varying_names, GL_SEPARATE_ATTRIBS); glLinkProgram(particleShader.GetProgram());
- In the update function, bind the particle vertex shader that will output to the transform feedback buffer and set the appropriate uniforms and update vertex array object. Note that to enable read/write access, we use a pair of vertex array objects such that we can read from one and write to another:
particleShader.Use(); glUniformMatrix4fv(particleShader("MVP"), 1, GL_FALSE, glm::value_ptr(mMVP)); glUniform1f(particleShader("time"), t); for(int i=0;i<NUM_ITER;i++) { glBindVertexArray( vaoUpdateID[readID]);
- Bind the vertex buffer objects that will store the outputs from the transform feedback step using the output attributes from the vertex shader:
glBindBufferBase(GL_TRANSFORM_FEEDBACK_BUFFER, 0, vboID_Pos[writeID]); glBindBufferBase(GL_TRANSFORM_FEEDBACK_BUFFER, 1, vboID_PrePos[writeID]); glBindBufferBase(GL_TRANSFORM_FEEDBACK_BUFFER, 2, vboID_Direction[writeID]);
- Disable the rasterizer to prevent the execution of the later stages of the pipeline and then begin the transform feedback. Next, issue a call to the
glDrawArrays
function to allow the vertices to be passed to the graphics pipeline. After this step, end the transform feedback and then enable the rasterizer. Note that to correctly determine the amount of execution time needed, we issue a hardware query. Next, we alternate the read/write paths by swapping the read and write IDs:glEnable(GL_RASTERIZER_DISCARD); glBeginQuery(GL_TIME_ELAPSED,t_query); glBeginTransformFeedback(GL_POINTS); glDrawArrays(GL_POINTS, 0, TOTAL_PARTICLES); glEndTransformFeedback(); glEndQuery(GL_TIME_ELAPSED); glFlush(); glDisable(GL_RASTERIZER_DISCARD); int tmp = readID; readID=writeID; writeID = tmp;
- Render the particles using the render shader. First, bind the render vertex array object and then draw the points using the
glDrawArrays
function:glBindVertexArray(vaoRenderID[readID]); renderShader.Use(); glUniformMatrix4fv(renderShader("MVP"), 1, GL_FALSE, glm::value_ptr(mMVP)); glDrawArrays(GL_POINTS, 0, TOTAL_PARTICLES); renderShader.UnUse(); glBindVertexArray(0);
- In the particle vertex shader, check if the particle's life is greater than 0. If so, move the particle and reduce the life. Otherwise, calculate a new random direction and reset the life to spawn the particle from the origin. Refer to
Chapter8/TransformFeedbackParticles/shaders/Particle.vert
for details. After this step, output the appropriate values to the output attributes. The particle vertex shader is defined as follows:#version 330 core precision highp float; #extension EXT_gpu_shader4 : require layout( location = 0 ) in vec4 position; layout( location = 1 ) in vec4 prev_position; layout( location = 2 ) in vec4 direction; uniform mat4 MVP; uniform float time; const float PI = 3.14159; const float TWO_PI = 2*PI; const float PI_BY_2 = PI*0.5; const float PI_BY_4 = PI_BY_2*0.5; //shader outputs out vec4 out_position; out vec4 out_prev_position; out vec4 out_direction; const float DAMPING_COEFFICIENT = 0.9995; const vec3 emitterForce = vec3(0.0f,-0.001f, 0.0f); const vec4 collidor = vec4(0,1,0,0); const vec3 emitterPos = vec3(0); float emitterYaw = (0.0f); float emitterYawVar = TWO_PI; float emitterPitch = PI_BY_2; float emitterPitchVar = PI_BY_4; float emitterSpeed = 0.05f; float emitterSpeedVar = 0.01f; int emitterLife = 60; int emitterLifeVar = 15; const float UINT_MAX = 4294967295.0; void main() { vec3 prevPos = prev_position.xyz; int life = int(prev_position.w); vec3 pos = position.xyz; float speed = position.w; vec3 dir = direction.xyz; if(life > 0) { prevPos = pos; pos += dir*speed; if(dot(pos+emitterPos, collidor.xyz)+ collidor.w <0) { dir = reflect(dir, collidor.xyz); speed *= DAMPING_COEFFICIENT; } dir += emitterForce; life--; } else { uint seed = uint(time + gl_VertexID); life = emitterLife + int(randhashf(seed++, emitterLifeVar)); float yaw = emitterYaw + (randhashf(seed++, emitterYawVar )); float pitch = emitterPitch + randhashf(seed++, emitterPitchVar); RotationToDirection(pitch, yaw, dir); float nspeed = emitterSpeed + (randhashf(seed++, emitterSpeedVar )); dir *= nspeed; pos = emitterPos; prevPos = emitterPos; speed = 1; } out_position = vec4(pos, speed); out_prev_position = vec4(prevPos, life); out_direction = vec4(dir, 0); gl_Position = MVP*vec4(pos, 1); }
The three helper functions
randhash
,randhashf
, andRotationToDirection
are defined as follows:uint randhash(uint seed) { uint i=(seed^12345391u)*2654435769u; i^=(i<<6u)^(i>>26u); i*=2654435769u; i+=(i<<5u)^(i>>12u); return i; } float randhashf(uint seed, float b) { return float(b * randhash(seed)) / UINT_MAX; } void RotationToDirection(float pitch, float yaw, out vec3 direction) { direction.x = -sin(yaw) * cos(pitch); direction.y = sin(pitch); direction.z = cos(pitch) * cos(yaw); }
The transform feedback mechanism allows us to feedback one or more attributes from the vertex shader or geometry shader back to a buffer object. This feedback path could be used for implementing a physically-based simulation. This recipe uses this mechanism to output the particle position after each iteration. After each step, the buffers are swapped and, therefore, it can simulate the particle motion.
To make the particle system, we first set up three pairs of vertex buffer objects that store the per-particle attributes that we input to the vertex shader. These include the particle's position, previous position, life, direction, and speed. These are stored into separate buffer objects for convenience. We could have stored all of these attributes into a single interleaved buffer object. Since we output to the buffer object from our shader, we specify the buffer object usage as GL_DYNAMIC_COPY
. Similarly, we set up a separate vertex array object for rendering the particles:
Next, we specify the shader output attributes that we would like to connect to the transform feedback buffers. We use three outputs, namely out_position
, out_prev_position
, and out_direction
, which output the particle's current position, particle's previous position, and the particle's direction along with the particle's speed, current, and initial life, respectively. We specify that we would connect these to separate buffer objects:
We then run a loop for the number of iterations desired. In the loop, we first bind the update vertex array object and assign the appropriate transform feedback buffer base indices:
If the life is less than 0, we reset the particle's direction of motion to a new random direction. We reset the life to a random value based on the maximum allowed value. The current and previous positions of the particle are reset to the emitter origin and finally, the speed is reset to the default value. We then output the output attributes:
Note that for this demo, we render the particles as points of size 10 units. We could easily change the rendering mode to point sprites with size modified in the vertex shader to give the particles a different look. Also, we can also change the colors and blending modes to achieve various effects. In addition, we could achieve the same result by using one vertex buffer pair with interleaved attributes or two separate transform feedback objects. All of these variants should be straightforward to implement by following the guidelines laid out in this recipe.
We had already looked at a simple approach of simulating GPU-based particle systems using vertex shader in Chapter 5, Mesh Model Formats and Particle Systems, we will now detail pros and cons of each. In Chapter 5 we presented a stateless particle system since all of the attributes (that is, position and velocity) were generated on the fly using the vertex ID, time, and basic kinematic equations on each particle vertex.
As the state of the particle is not stored, we cannot reproduce the same simulation every frame. Hence, collision detection and response are problematic, as we do not have any information of the previous state of the particle, which is often required for collision response. On the contrary, the particle simulation technique presented in this recipe uses a state-preserving particle system. We stored current and previous positions of each particle in buffer objects. In addition, we used transform feedback and a vertex shader for particle simulation on the GPU. As the state of the particle is stored, we can carry out collision detection and response easily.
- OGLDev Tutorial on particle system using transform feedback at http://ogldev.atspace.co.uk/www/tutorial28/tutorial28.html
- OpenGL 4.0 Shading Language Cookbook, Chapter 9, Animation and Particles, the Creating a particle system using transform feedback section, Packt Publishing, 2011.
- Noise based Particles, Part II at The Little Grasshopper, http://prideout.net/blog/?p=67
feedback mechanism allows us to feedback one or more attributes from the vertex shader or geometry shader back to a buffer object. This feedback path could be used for implementing a physically-based simulation. This recipe uses this mechanism to output the particle position after each iteration. After each step, the buffers are swapped and, therefore, it can simulate the particle motion.
To make the particle system, we first set up three pairs of vertex buffer objects that store the per-particle attributes that we input to the vertex shader. These include the particle's position, previous position, life, direction, and speed. These are stored into separate buffer objects for convenience. We could have stored all of these attributes into a single interleaved buffer object. Since we output to the buffer object from our shader, we specify the buffer object usage as GL_DYNAMIC_COPY
. Similarly, we set up a separate vertex array object for rendering the particles:
Next, we specify the shader output attributes that we would like to connect to the transform feedback buffers. We use three outputs, namely out_position
, out_prev_position
, and out_direction
, which output the particle's current position, particle's previous position, and the particle's direction along with the particle's speed, current, and initial life, respectively. We specify that we would connect these to separate buffer objects:
We then run a loop for the number of iterations desired. In the loop, we first bind the update vertex array object and assign the appropriate transform feedback buffer base indices:
If the life is less than 0, we reset the particle's direction of motion to a new random direction. We reset the life to a random value based on the maximum allowed value. The current and previous positions of the particle are reset to the emitter origin and finally, the speed is reset to the default value. We then output the output attributes:
Note that for this demo, we render the particles as points of size 10 units. We could easily change the rendering mode to point sprites with size modified in the vertex shader to give the particles a different look. Also, we can also change the colors and blending modes to achieve various effects. In addition, we could achieve the same result by using one vertex buffer pair with interleaved attributes or two separate transform feedback objects. All of these variants should be straightforward to implement by following the guidelines laid out in this recipe.
We had already looked at a simple approach of simulating GPU-based particle systems using vertex shader in Chapter 5, Mesh Model Formats and Particle Systems, we will now detail pros and cons of each. In Chapter 5 we presented a stateless particle system since all of the attributes (that is, position and velocity) were generated on the fly using the vertex ID, time, and basic kinematic equations on each particle vertex.
As the state of the particle is not stored, we cannot reproduce the same simulation every frame. Hence, collision detection and response are problematic, as we do not have any information of the previous state of the particle, which is often required for collision response. On the contrary, the particle simulation technique presented in this recipe uses a state-preserving particle system. We stored current and previous positions of each particle in buffer objects. In addition, we used transform feedback and a vertex shader for particle simulation on the GPU. As the state of the particle is stored, we can carry out collision detection and response easily.
- OGLDev Tutorial on particle system using transform feedback at http://ogldev.atspace.co.uk/www/tutorial28/tutorial28.html
- OpenGL 4.0 Shading Language Cookbook, Chapter 9, Animation and Particles, the Creating a particle system using transform feedback section, Packt Publishing, 2011.
- Noise based Particles, Part II at The Little Grasshopper, http://prideout.net/blog/?p=67
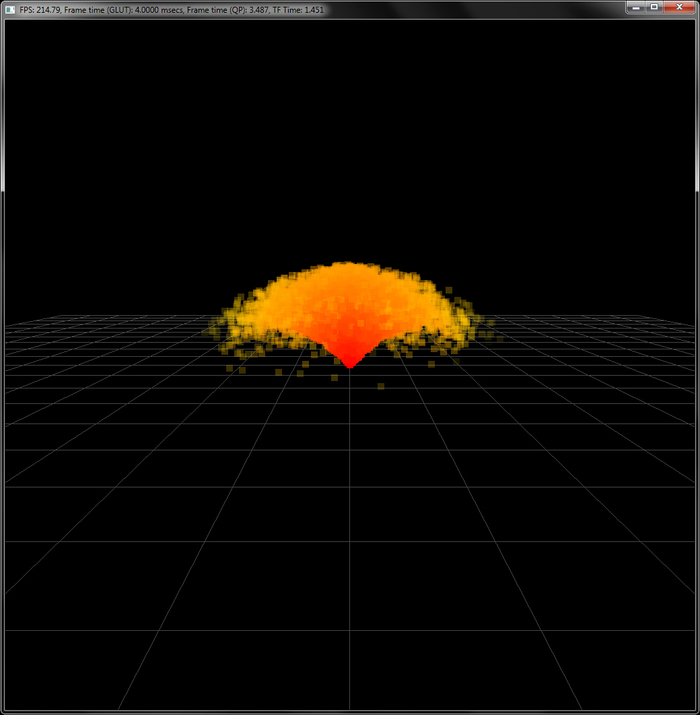
with interleaved attributes or two separate transform feedback objects. All of these variants should be straightforward to implement by following the guidelines laid out in this recipe.
We had already looked at a simple approach of simulating GPU-based particle systems using vertex shader in Chapter 5, Mesh Model Formats and Particle Systems, we will now detail pros and cons of each. In Chapter 5 we presented a stateless particle system since all of the attributes (that is, position and velocity) were generated on the fly using the vertex ID, time, and basic kinematic equations on each particle vertex.
As the state of the particle is not stored, we cannot reproduce the same simulation every frame. Hence, collision detection and response are problematic, as we do not have any information of the previous state of the particle, which is often required for collision response. On the contrary, the particle simulation technique presented in this recipe uses a state-preserving particle system. We stored current and previous positions of each particle in buffer objects. In addition, we used transform feedback and a vertex shader for particle simulation on the GPU. As the state of the particle is stored, we can carry out collision detection and response easily.
- OGLDev Tutorial on particle system using transform feedback at http://ogldev.atspace.co.uk/www/tutorial28/tutorial28.html
- OpenGL 4.0 Shading Language Cookbook, Chapter 9, Animation and Particles, the Creating a particle system using transform feedback section, Packt Publishing, 2011.
- Noise based Particles, Part II at The Little Grasshopper, http://prideout.net/blog/?p=67
- http://ogldev.atspace.co.uk/www/tutorial28/tutorial28.html
- OpenGL 4.0 Shading Language Cookbook, Chapter 9, Animation and Particles, the Creating a particle system using transform feedback section, Packt Publishing, 2011.
- Noise based Particles, Part II at The Little Grasshopper, http://prideout.net/blog/?p=67