.NET mobile
.NET mobile (formerly known as Xamarin) is a set of extensions to .NET that is used to develop native applications for iOS (.NET for iOS/tvOS/Mac Catalyst), Android (.NET for Android), and macOS (.NET for macOS). .NET is the evolution of the .NET Framework, designed for cross-platform development. .NET mobile was introduced in .NET Core 5 as optional workloads. It is technically a binding layer on top of these platforms. Using bindings to platform APIs enables .NET developers to use C# (and F#) to develop native applications with the full capacity of each platform.
The C# APIs we use when we develop apps with .NET mobile match the platform APIs, but they are modified to adhere to conventions used in .NET Core. For example, APIs are often customized to follow .NET naming conventions, and the Android set
and get
methods are often replaced by properties. This makes using the APIs easier and more familiar for .NET developers.
Mono (https://www.mono-project.com) is an open source implementation of the Microsoft .NET Framework, which is based on the European Computer Manufacturers Association (ECMA) standards for C# and the Common Language Runtime (CLR). Mono was created to bring the .NET Framework to platforms other than Windows. It is part of the .NET Foundation (http://www.dotnetfoundation.org), an independent organization that supports open development and collaboration involving the .NET ecosystem. Since .NET 5, Mono is now a supported runtime for applications built on .NET. No separate installer is needed to use Mono with .NET; it is included in the installer for .NET. The Mono runtime is used for iOS, tvOS, Mac Catalyst, and Android applications, while the .NET Core CLR is used for all other supported platforms.
With a combination of the .NET mobile platforms, .NET, and Mono, we can use both the platform-specific APIs and the platform-independent parts of .NET, including namespaces such as System
, System.Linq
, System.IO
, System.Net
, and System.Threading.Tasks
.
There are several reasons for using .NET mobile for mobile app development, which we will cover in the following sections.
Code sharing
If we use one common programming language for multiple mobile platforms (and even server platforms), then we can share a lot of code between our target platforms, as illustrated in the following diagram. All code that isn’t related to the target platform can be shared with other .NET platforms. Code that is typically shared in this way includes business logic, network calls, and data models:
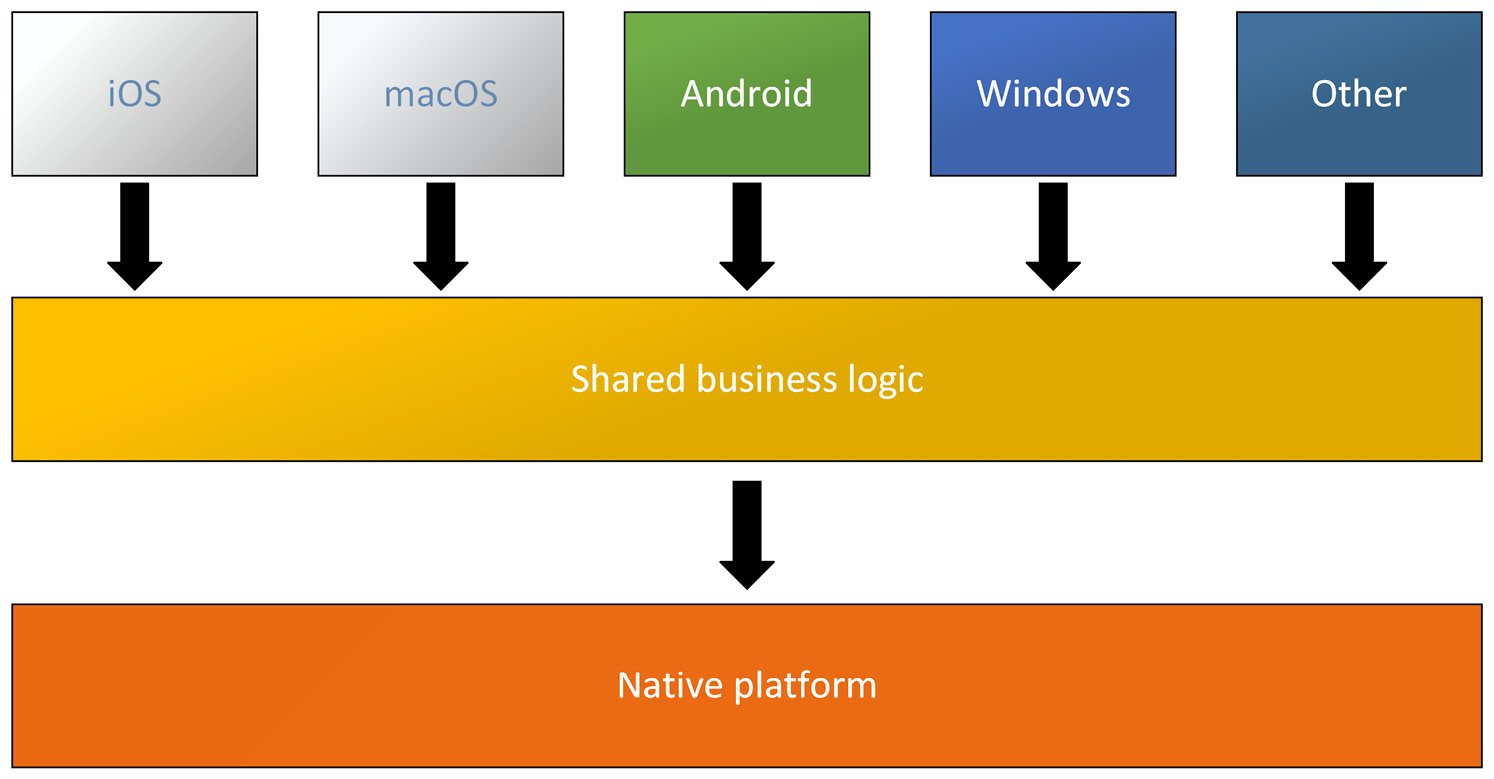
Figure 1.1 – .NET MAUI code sharing
There is also a large community based around the .NET platforms, providing a different form of code sharing. There is a wide range of third-party libraries and components that can be downloaded from NuGet (https://nuget.org) that can provide you with additional features or capabilities that work across all supported .NET MAUI platforms. For example, you can find NuGet packages that provide databases, graphs, or barcode reading to include in your apps.
Code sharing across platforms leads to shorter development times. It also produces apps of a higher quality because, for example, we only need to write the code for business logic once. There is a lower risk of bugs, and it is also able to guarantee that a calculation returns the same result, regardless of what platform our users use.
Use of existing knowledge
For .NET developers who want to start building native mobile apps, it is easier to just learn the APIs for the new platforms than it is to learn programming languages and APIs for both old and new platforms.
Similarly, organizations that want to build native mobile apps can use existing developers with their knowledge of .NET to develop apps. Because there are more .NET developers than Objective-C and Swift developers, it’s easier to find new developers for mobile app development projects.
.NET mobile platforms
The different .NET mobile platforms available are .NET for iOS/tvOS/Mac Catalyst, .NET for Android, and .NET for macOS. In this section, we will take a look at each of them.
.NET for iOS/tvOS/Mac Catalyst
.NET for iOS/tvOS/Mac Catalyst is used to build apps for iOS, tvOS, or Mac Catalyst, respectively, with .NET and contains the bindings to the iOS APIs mentioned previously. .NET for iOS/tvOS/Mac Catalyst uses ahead-of-time (AOT) compilation to compile the C# code into the Advanced RISC Machine (ARM) assembly language. The Mono runtime runs alongside the Objective-C runtime. Code that uses .NET namespaces, such as System.Linq
or System.Net
, is executed by the Mono runtime, while code that uses iOS-specific namespaces is executed by the Objective-C runtime. Both the Mono runtime and the Objective-C runtime run on top of the X is Not Unix (XNU) Unix-like kernel (https://github.com/apple/darwin-xnu), which was developed by Apple. The following diagram shows an overview of the iOS architecture:
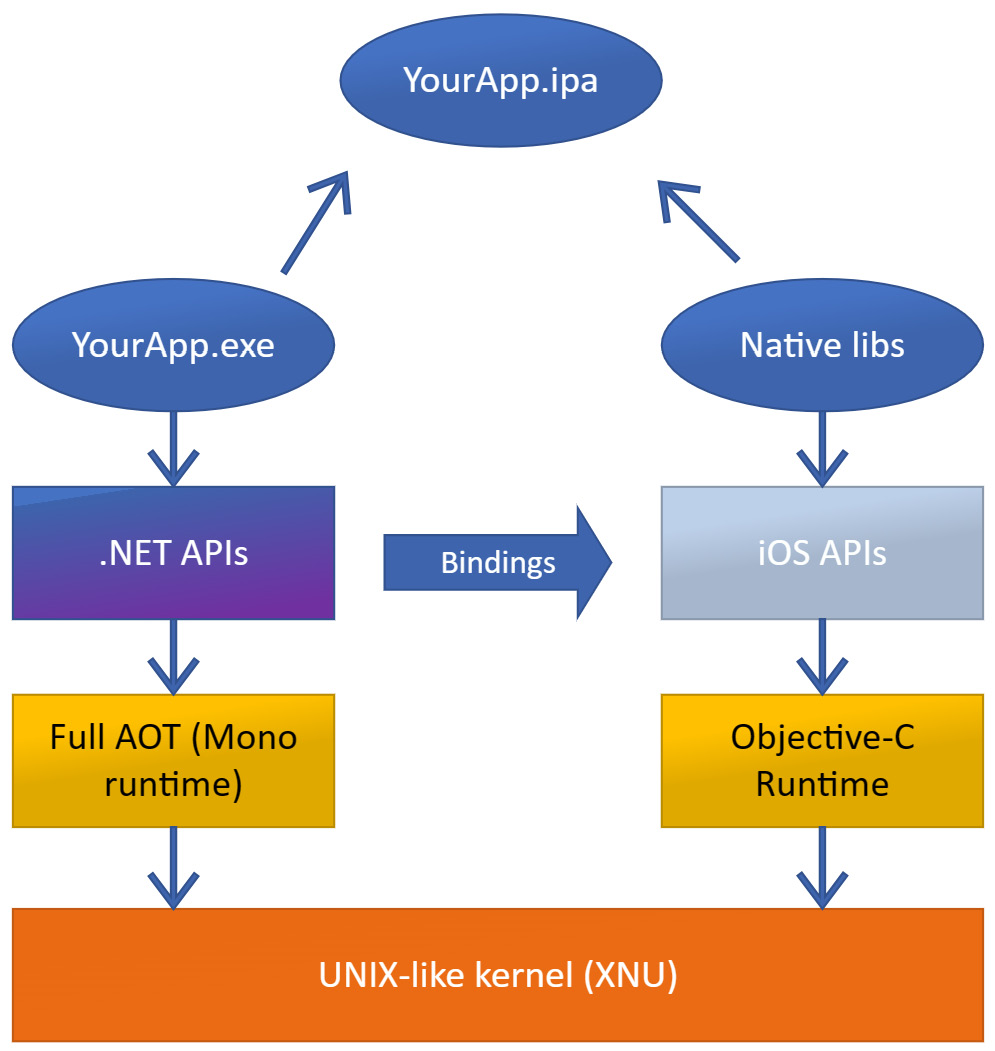
Figure 1.2 – .NET for iOS
.NET for macOS
.NET for macOS is used to build apps for macOS with .NET and contains the bindings to the macOS APIs. .NET for macOS has the same architecture as .NET for iOS—the only difference is, .NET for macOS apps are just-in-time (JIT)-compiled, unlike .NET for iOS apps, which are AOT-compiled. This is shown in the following diagram:
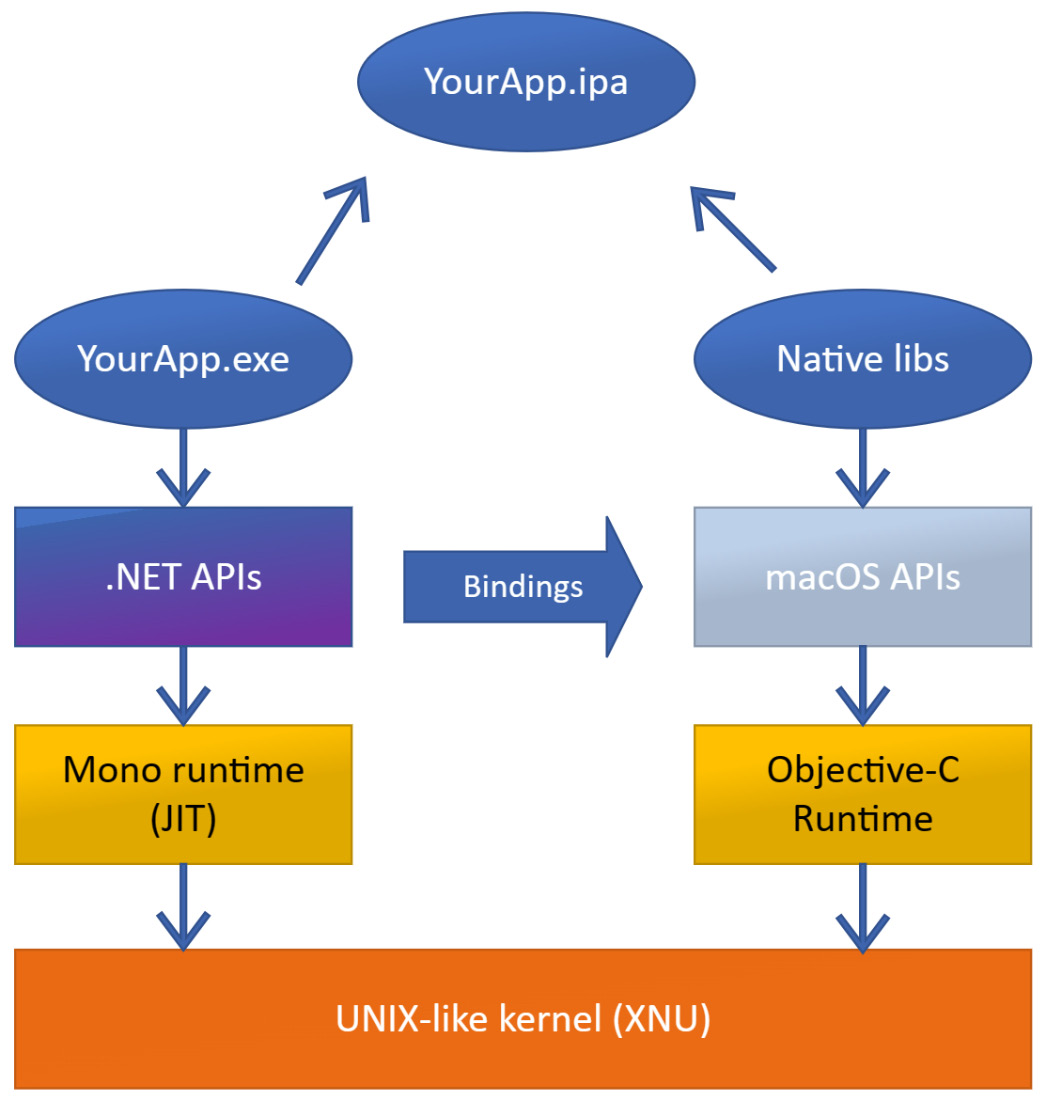
Figure 1.3 – .NET for macOS
.NET for Android
.NET for Android is used to build apps for Android with .NET and contains bindings to the Android APIs. The Mono runtime and the Android Runtime (ART) run side by side on top of a Linux kernel. .NET for Android apps could either be JIT-compiled or AOT-compiled, but to AOT-compile them, we need to use Visual Studio Enterprise.
Communication between the Mono runtime and ART occurs via a Java Native Interface (JNI) bridge. There are two types of JNI bridges—Manage Callable Wrapper (MCW) and Android Callable Wrapper (ACW). An MCW is used when code needs to run in ART and an ACW is used when ART needs to run code in the Mono runtime, as shown:
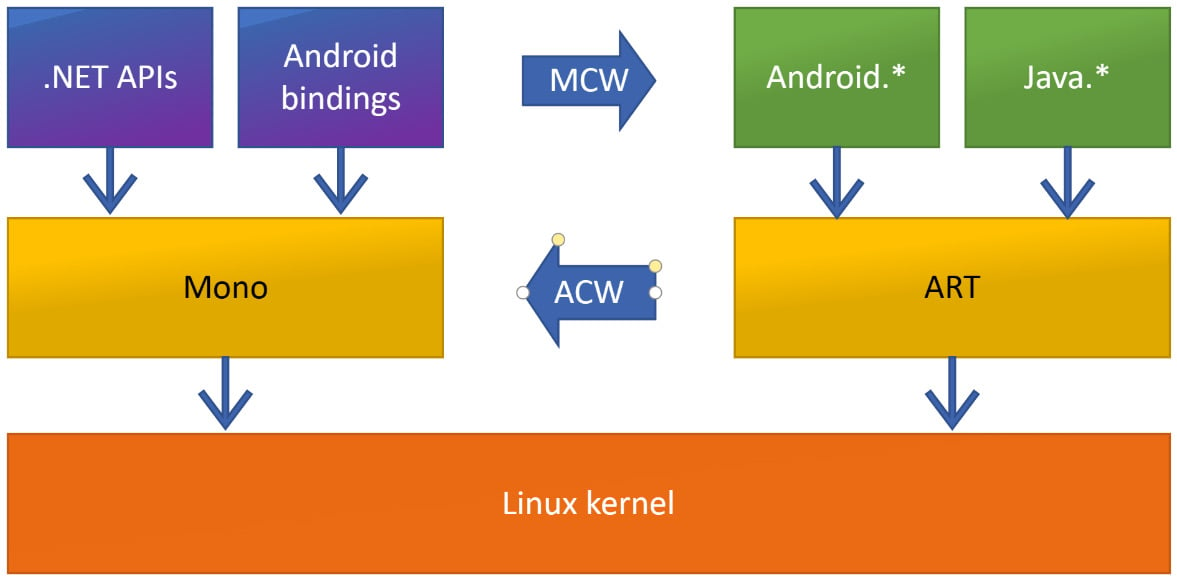
Figure 1.4 – .NET for Android
.NET for Tizen
.NET MAUI has additional support for the Tizen platform from Samsung. Samsung provides the binding layer and runtime to allow .NET MAUI to run on the Tizen platform. To learn more about how to install and develop for the Tizen platform, visit https://github.com/Samsung/Tizen.NET in your browser.
Now that we understand what .NET mobile is and how each platform works, we can explore .NET MAUI in detail.