We've seen a lot of theory behind microservice architecture in Chapter 3, Working with Microservices. It's time to do some hands-on practice; we are going to implement our own microservice. This will be a simple REST service, accepting HTTP methods such as GET and POST to retrieve and update entities. There are a couple of choices when developing microservices in Java. In this chapter, we are going to get an overview about two main approaches, probably the most popular will be JEE7, and Spring Boot. We will briefly see how we can code a microservice using JEE JAX-RS. We will also create a microservice running on Spring Boot. In fact, in Chapter 5, Creating Images with Java Applications, we are going to run our Spring Boot microservice from within a Docker container. As we have said in Chapter 3, Working with Microservices, microservices usually...
You're reading from Docker and Kubernetes for Java Developers
Introduction to REST
The REST acronym stands for Representational State Transfer. It's an architectural style and a design for network-based software. It describes how one system can communicate a state with another. This fits perfectly well into the microservice world. As you will remember from Chapter 3, Working with Microservices, the software applications based on the microservices architecture is a bunch of separated, independent services talking to each other.
There are some concepts in REST that we need to understand, before we go further:
- resource: This is the main concept in the REST architecture. Any information can be a resource. A bank account, a person, an image, a book. A representation of a resource must be stateless
- representation: A specific way a resource can be represented. For example, a bank account resource can be represented using JSON, XML, or HTML...
REST in Java
When developing a REST service in Java, we have at least a couple of options for the framework we could use. The most popular will be pure JEE7 with JAX-RS or Spring Framework with its Spring Boot. You can use either of them or mix them together. Let's look at those two now in more detail, starting with JAX-RS.
Java EE7 - JAX-RS with Jersey
JAX-RS was born as a result of Java Specification Request (JSR) 311. As the official definition says, the JAX-RS is the Java API for RESTful web services. It's a specification that provides support in creating web services according to the REST architectural pattern. JAX-RS uses Java annotations, introduced in Java SE 5, to simplify the development and deployment...
Coding the Spring Boot microservice
We know that we have some starters available, so let's make use of them to save some time. The service that we are going to create will be the simple REST microservice for storing and retrieving entities from a database: books, in our case. We are not going to implement authentication and security features, just to make it as clean and simple as possible. Books will be stored in an in-memory relational H2 database. We are going to build and run our bookstore with Maven, so let's begin with the pom.xml build file.
Maven build file
As you will see, the parent project for our own service is spring-boot-starter-parent. Spring this is the parent project providing dependency and plugin...
Running the application
Because we have defined the Spring Boot plugin in our pom.xml build file, we can now start the application using Maven. All you need to have is Maven present on the system path, but you probably have this already as a Java developer. To run the application, execute the following from the command shell (terminal on MacOS or cmd.exe on Windows):
$ mvn spring-boot:run
After a while, the Spring splash log will show up in the console and your microservice will be ready to accept HTTP requests. Soon, in Chapter 5, Creating Images with Java Applications, our goal will be to see the same coming from the Docker container:
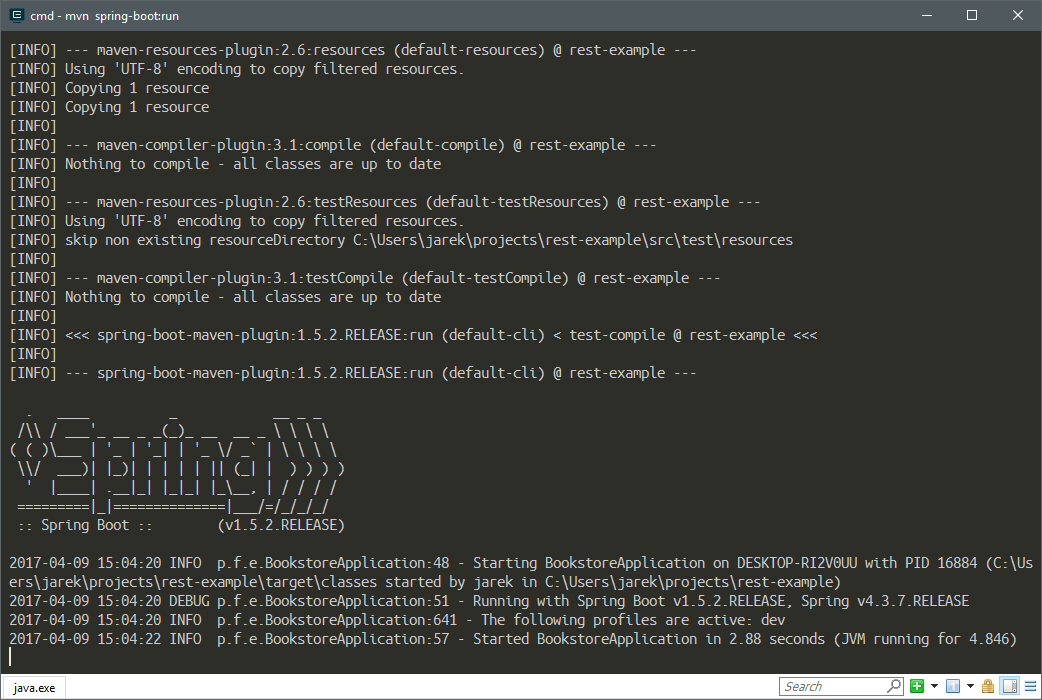
If you want to, you can also run the application straight from the IDE, be it IntelliJ IDEA, Eclipse, or Netbeans. Our BookstoreApplication class has a main() method; you will just need to create a runtime configuration in your IDE and run it...
Making calls
Making a call to the operation exposed from the service can be done using any tool or library that can execute the HTTP requests. The first obvious choice would be just a web browser. But a web browser is convenient only for executing GET requests (as for getting a list of books from our bookstore service). If you need to execute other methods such as POST or PUT or provide additional request parameters, header values, and so on, you will need to use some alternatives. The first choice could be cURL, a command-line tool for transferring data using various protocols. Let's look at other options we have.
Spring RestTemplate
If you need to call a service from another service, you will need a HTTP client. Spring...
Spring Initializr
Spring Initializr is a web-based tool available at https://start.spring.io. It's a quick start generator for Spring projects. Spring Initializr can be used as follows:
- From the web browser at https://start.spring.io
- In your IDE (IntelliJ IDEA Ultimate or NetBeans, using plugins)
- From the command line with the Spring Boot CLI or simply with cURL or HTTPie
Using the web application is very convenient; all you need to do is provide details about your application Maven archetype, such as group, artifact name, description, and so on:
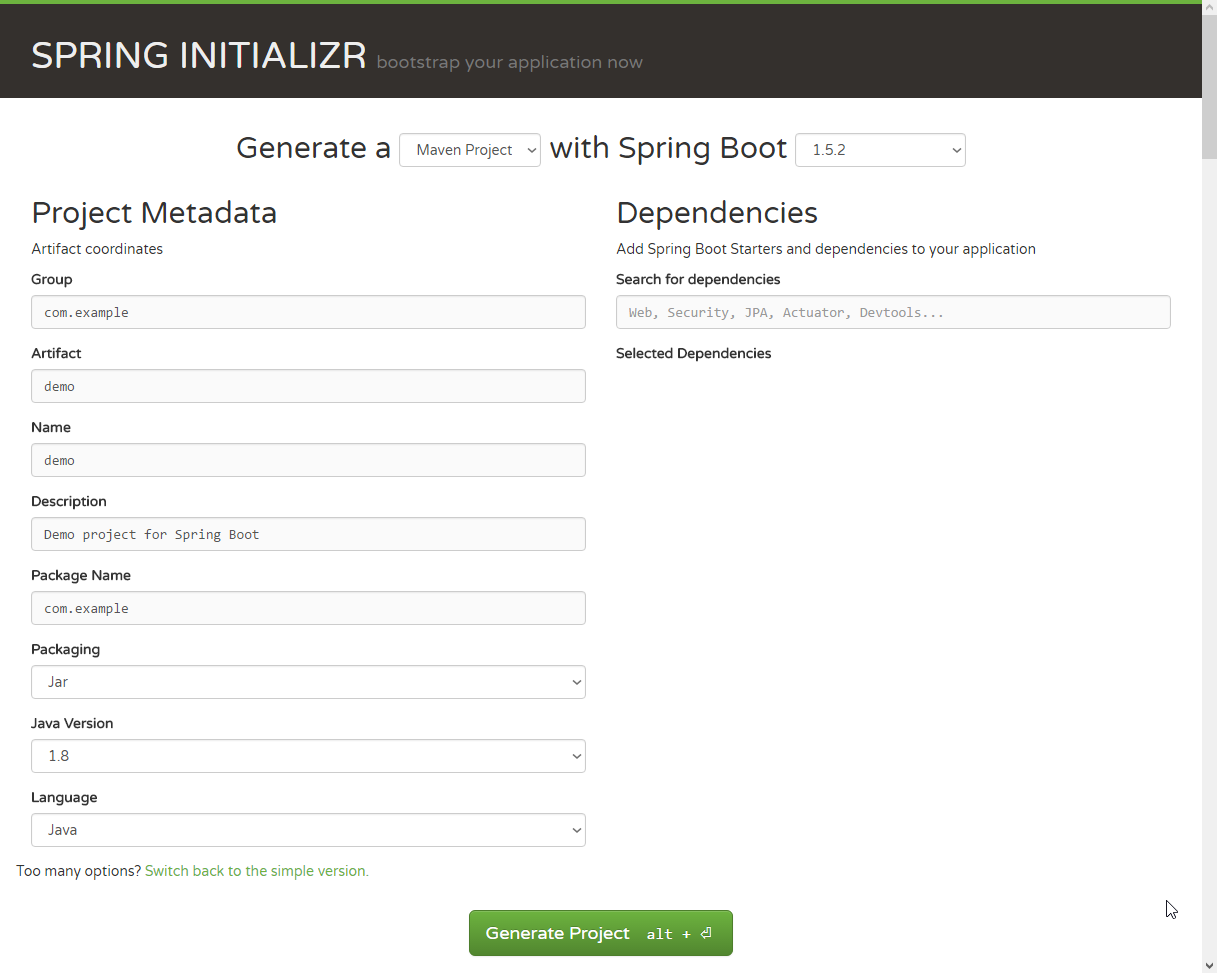
In the Dependencies section, you can enter the keywords of the features you would like to have included, such as JPA, web, and so on. You can also switch the UI to an advanced view, to have all the features listed and ready to be selected:
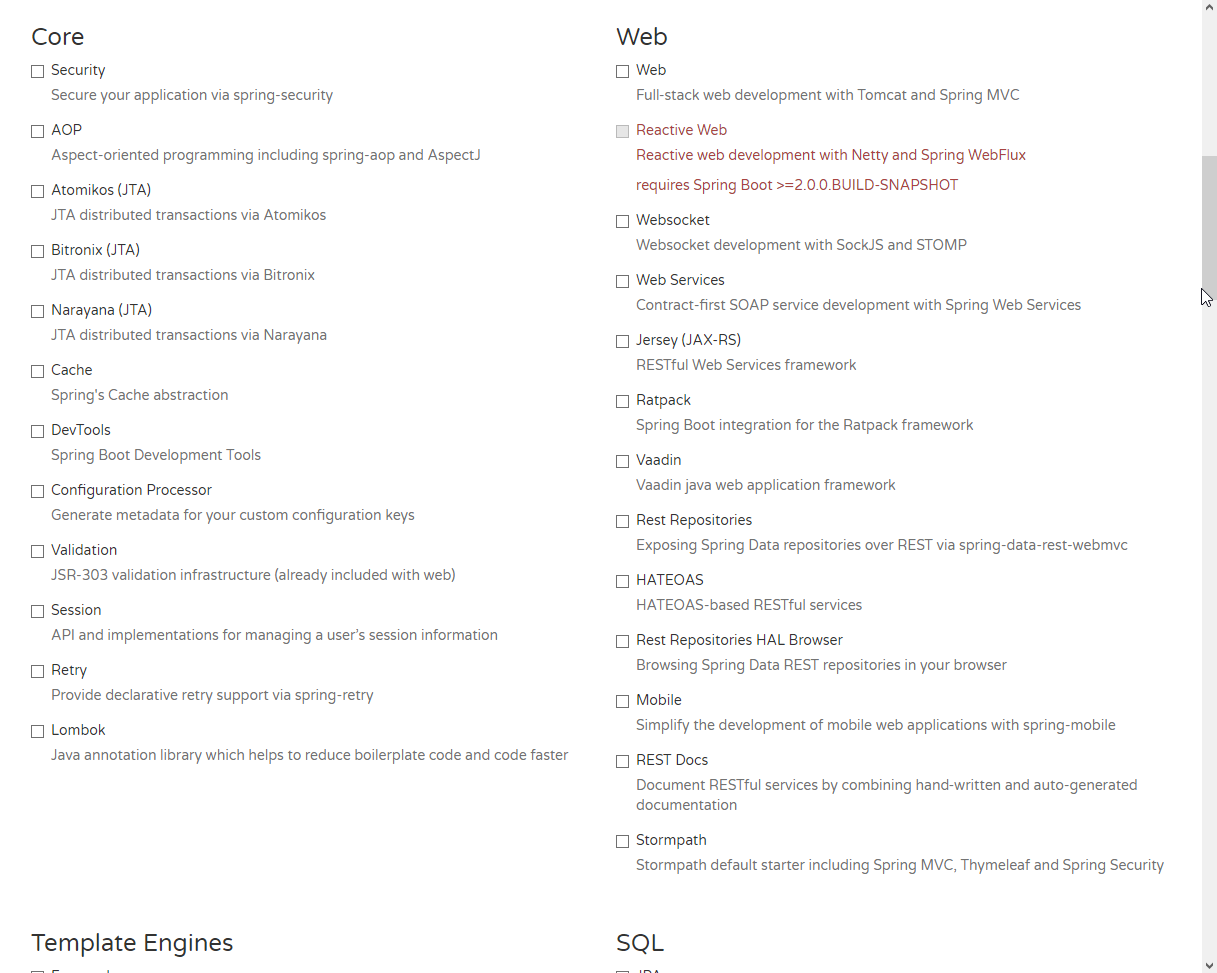
As the output, Spring Initializr will create a ZIP archive with the base Maven project...
Summary
As you can see, developing Java microservices is not as tricky as it may sound. You can choose between JEE7 JAX-RS or Spring Boot, wire some classes, and a basic service is ready. You are not limited to using Spring MVC for creating your REST endpoints. If you are more familiar with the Java EE JAX-RS specification, you can easily integrate JAX-RS into Spring applications, especially Spring Boot applications. You can then take what is best for you from both.
Of course, in the real world you would probably want to include some more advanced features such as authentication and security. Having Spring Initializr available can give you a serious speed boost when developing your own service. In Chapter 5, Creating Images with Java Applications, we are going to package our bookstore service into a Docker image and run it using Docker Engine.