Scalability and resilience and independent data management
Scalability and resilience are some key concepts in microservices to keep in mind while building robust and high-performing software. Also, in microservices, every service has its own database, so every data storage is independent.
Scalability and resilience
Scalability and resilience are crucial aspects of building robust and high-performing software systems. Let’s explore these concepts in more detail.
Scalability refers to the ability of a system to handle increased workloads and accommodate growing demands without sacrificing performance. It involves the capability to scale up or scale out the system to ensure optimal resource utilization and responsiveness.
Here are key considerations for achieving scalability:
- Horizontal scaling: Horizontal scaling involves adding more instances or nodes to distribute the workload across multiple servers or machines. It allows for increased throughput and improved performance by handling requests in parallel.
- Vertical scaling: Vertical scaling, also known as scaling up, involves increasing the resources (such as CPU, memory, or storage) of individual instances to handle higher workloads. Vertical scaling can be achieved by upgrading hardware or utilizing cloud-based services that offer scalable resource provisioning.
- Load balancing: Load balancing mechanisms distribute incoming requests across multiple instances to ensure an even distribution of workloads and prevent overload on any single component. Load balancers intelligently route requests based on factors such as server health, capacity, or response time.
- Caching: Implementing caching mechanisms, such as in-memory caches or content delivery networks (CDNs), can significantly improve scalability. Caching reduces the load on backend services by storing frequently accessed data or computed results closer to the users, thereby reducing the need for repeated processing.
- Asynchronous processing: Offloading long-running or resource-intensive tasks to asynchronous processing systems, such as message queues or background workers, helps improve scalability. By processing tasks asynchronously, the system can handle a larger number of concurrent requests and optimize resource utilization.
- Resilience: Resilience refers to the system’s ability to recover from failures, adapt to changing conditions, and continue to operate reliably. Resilient systems are designed to minimize the impact of failures and maintain essential functionality. Consider the following factors for building resilient systems:
- Redundancy and replication: Replicating critical components or data across multiple instances or nodes ensures redundancy and fault tolerance. If one instance fails, others can seamlessly take over to maintain system availability and prevent data loss.
- Fault isolation: Designing systems with well-defined service boundaries and loose coupling ensures that failures or issues in one component do not propagate to others. Fault isolation prevents the entire system from being affected by localized failures.
- Failure handling and recovery: Implementing robust error handling and recovery mechanisms is essential for resilience. Systems should be able to detect failures, recover automatically if possible, and provide clear feedback to users or downstream components.
- Monitoring and alerting: Continuous monitoring of system health, performance, and error rates helps identify issues or potential failures in real time. Proactive alerting mechanisms can notify appropriate personnel when anomalies or critical events occur, allowing for timely intervention and mitigation.
- Graceful degradation and circuit breakers: Systems should be designed to gracefully degrade functionality when facing high loads or failure conditions. Circuit breakers can be implemented to automatically stop sending requests to a failing component or service, reducing the impact on the system and allowing it to recover.
Scalability and resilience are closely interconnected. Scalable systems are often designed with resilience in mind, and resilient systems can better handle increased workloads through scalable architecture. By incorporating these characteristics into their designs, developers can create robust and reliable software systems capable of adapting to changing demands and providing a positive user experience even in challenging conditions.
Independent data management
Independent data management refers to the practice of managing data within individual services or components in a decentralized manner. In a microservices architecture, each service typically has its own data store or database, and the responsibility for data management lies within the service boundary.
Here are key considerations for independent data management:
- Data ownership and autonomy: Each service is responsible for managing its own data, including data storage, retrieval, and modification. This promotes autonomy and allows teams to make independent decisions regarding data models, storage technologies, and data access patterns.
- Decentralized data stores: Services may use different types of databases or storage technologies based on their specific needs. For example, one service may use a relational database, while another may use a NoSQL database (see Chapter 9) or a specialized data store optimized for specific use cases.
- Data consistency and synchronization: When data is distributed across multiple services, ensuring data consistency can be challenging. Techniques such as eventual consistency, distributed transactions, or event-driven architectures can be employed to synchronize data across services and maintain data integrity.
- Data access and communication: Services communicate with each other through well-defined APIs or message-based protocols to access and exchange data. Service boundaries should have clear contracts and APIs for data exchange, enabling services to interact while maintaining loose coupling.
- Data security and access control: Each service should enforce appropriate security measures and access controls to protect its data. Implementing authentication, authorization, and encryption mechanisms ensures data privacy and security within the service boundaries.
- Data integration and aggregation: While services manage their own data, there may be situations where data from multiple services needs to be aggregated or integrated for specific use cases. Techniques such as data pipelines, data warehouses, or event-driven architectures can facilitate data integration and aggregation across services.
Independent data management allows services to evolve and scale independently, promotes team autonomy, and reduces interdependencies between services.
Figure 1.5 shows the data management process:
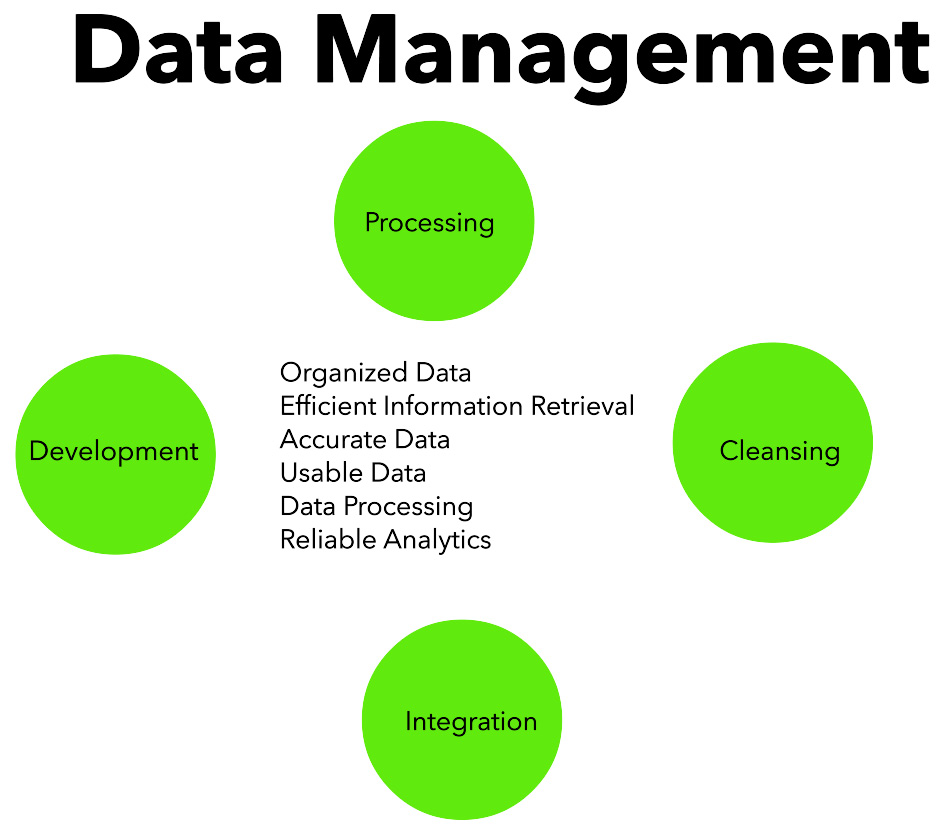
Figure 1.5: Data management process
However, the data management process also introduces challenges related to data consistency, synchronization, and overall system-wide data coherence. Organizations should carefully design data management strategies and employ appropriate patterns and technologies to address these challenges while maintaining the benefits of independent data management.
In the next section, we’ll learn about APIs, communication, and CI.