An interface
An interface is another way to achieve polymorphism and abstraction in Apex. Interfaces are like a contract. We can only add a declaration to a class but not the actual implementation. You might be thinking about why do we need to create a class, which does not have anything in it? Well, I will ask you to think about this again after taking a look at the following example.
We will continue with the Mario example. Like any game, this game also needs to have levels. Every level will be different from the previous; and therefore, the code cannot be reused. Inheritance was very powerful because of the dynamic dispatch polymorphic behavior; however, inheritance can not be used in this scenario.
We will be using an interface to define levels in a game. Every level will have its number and environment:
public interface GameLevel {
void levelNumber();
void environment();
}
The preceding interface defines two methods that need to be implemented by child classes. The interface
keyword is used to define an interface in Apex:
public class Level_Underground implements GameLevel { public void levelNumber(){ System.debug('Level 1'); } public void environment(){ System.debug('This level will be played Underground'); } } public class Level_UnderWater implements GameLevel { public void levelNumber(){ System.debug('Level 2'); } public void environment(){ System.debug('This level will be played Under Water'); } }
The preceding two classes implement GameLevel
and make sure that both the methods have been implemented. A compiler will throw an error if there is any mistake in implementing a child class with a different method signature.
The following class diagram shows two classes implementing a common interface:
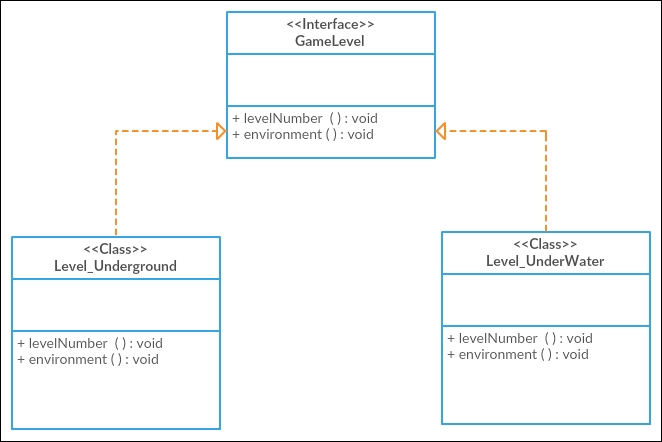
The anonymous Apex code for testing is as follows:
GameLevel obj = new Level_Underground(); obj.levelNumber(); obj.environment(); obj = new Level_UnderWater(); obj.levelNumber(); obj.environment();
The output of this code snippet is as follows:
Level 1
This level will be played Underground
Level 2
This level will be played Under Water
We cannot instantiate interfaces; however, we can assign any child class to them; this behavior of an interface makes it a diamond in the sea of OOP.
In the preceding code, obj
is defined as GameLevel
; however, we assigned Level_Underground
and Level_UnderWater
to it, and Apex was able to dynamically dispatch correct the implementation methods.
Huge applications and APIs are created using interfaces. In Apex, Queueable
and Schedulable
are examples of interfaces. Apex only needs to invoke the execute()
method in your class because it knows that you follow the contract of an interface.
Note
Apex does not support multiple inheritance where one child class extends multiple parent classes at a time. However, using an interface a child class can implement multiple interfaces at a time.