Types
In the last example, we saw how to specify some primitive types for our function parameter and returned value, but you’re probably wondering how you can describe an object or array with more details. Types can help us to describe our objects or arrays in a better way. For example, let’s suppose you want to describe a User
type to save the information into the database:
type User = {
username: string
email: string
name: string
age: number
website: string
active: boolean
}
const user: User = {
username: 'czantany',
email: 'carlos@milkzoft.com',
name: 'Carlos Santana',
age: 33,
website: 'http://www.js.education',
active: true
}
// Let's suppose you will insert this data using Sequelize...
models.User.create({ ...user }}
We get the following error if we forget to add one of the nodes or put an invalid value in one of them:
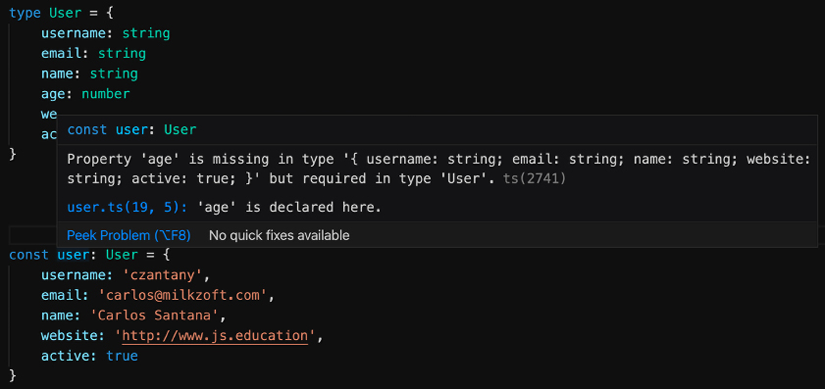
Figure 2.2: Age is missing in type User but is required
If you need optional nodes, you can always put a ?
next to the age of the node, as shown in the following code block:
type User = {
username: string
email: string
name: string
age?: number
website: string
active: boolean
}
You can name type
as you want, but a good practice to follow is to add a prefix of T
. For example, the User
type will become TUser
. In this way, you can quickly recognize that it is type
and you don’t get confused thinking it is a class or a React component.