List methods
Since a list is a type of sequence, it supports all sequence operations and methods.
Lists are one of the best data structures to use. Python provides a set of list methods that makes it easy for us to store and retrieve values to maintain, update, and extract data. These common operations are what Python programmers perform, including slicing, sorting, appending, searching, inserting, and removing data.
You will learn about these handy list methods in the following exercises.
Exercise 22 – basic list operations
In this exercise, you are going to use the basic functions of lists to check the size of a list, combine lists, and duplicate lists:
- Open a new Jupyter notebook.
- Type the following code:
shopping = ["bread","milk", "eggs"]
- The length of a list can be found using the global
len()
function:print(len(shopping))
Note
The len()
function returns the number of items in an object. When the object is a string, it returns the number of characters in the string.
The output is as follows:
3
- Now, concatenate two lists using the
+
operator:list1 = [1,2,3]
list2 = [4,5,6]
final_list = list1 + list2
print(final_list)
You will get the following output:
[1, 2, 3, 4, 5, 6]
As you can see, lists also support many string operations, one of which is concatenation, which involves joining two or more lists together.
- Now, use the
*
operator, which can be used for repetition in a list, to duplicate elements:list3 = ['oi']
print(list3*3)
It will repeat 'oi'
three times, giving us the following output:
['oi', 'oi', 'oi']
You are now familiar with some common operations that Python programmers use to interact with lists.
Accessing an item from a list
Just like other programming languages, in Python, you can use the index
method to access elements in a list. You should complete the following exercise while continuing with the previous notebook.
Exercise 23 – accessing an item from shopping list data
In this exercise, you will work with lists and gain an understanding of how you can access items from a list. The following steps will enable you to complete this exercise:
- Open a new Jupyter Notebook.
- Enter the following code in a new cell:
shopping = ["bread","milk", "eggs"]
print(shopping[1])
The output is as follows:
milk
As you can see, the milk
value from the shopping
list has an index of 1
since the list begins from 0
.
- Now, access the first index and replace it with
banana
:shopping[1] = "banana"
print(shopping)
The output is as follows:
['bread', 'banana', 'eggs']
- Type the following code in a new cell and observe the output:
print(shopping[-1])
The output is as follows:
eggs
The output will print eggs
– the last item.
Just like with strings, Python lists support slicing with the :
notation in the format of list[i:j]
, where i
is the starting element and j
is the last element (non-inclusive).
- Enter the following code to try out a different type of slicing:
print(shopping[0:2])
This prints the first and second elements, producing the following output:
['bread', 'banana']
- Now, to print from the beginning of the list to the third element, run the following:
print(shopping[:3])
The output is as follows:
['bread', 'banana', 'eggs']
- Similarly, to print from the second element of the list until the end, you can use the following:
print(shopping[1:])
The output is as follows:
['banana', 'eggs']
Having completed this exercise, you are now able to access items from a list in different ways.
Adding an item to a list
In the previous section and Exercise 23 – accessing an item from shopping list data, you learned how to access items from a list. Lists are very powerful and are used in many circumstances. However, you often won’t know the data your users want to store beforehand. Here, you are going to look at various methods for adding items to and inserting items into a list.
Exercise 24 – adding items to our shopping list
The append
method is the easiest way to add a new element to the end of a list. You will use this method in this exercise to add items to our shopping
list:
- In a new cell, type the following code to add a new element,
apple
, to the end of the list using theappend
method:shopping = ["bread","milk", "eggs"]
shopping.append("apple")
print(shopping)
Let’s see the output:
['bread', 'milk', 'eggs', 'apple']
The append
method is commonly used when you are building a list without knowing what the total number of elements will be. You will start with an empty list and continue to add items to build the list.
- Now, create an empty list,
shopping
, and keep adding items one by one to this empty list:shopping = []
shopping.append('bread')
shopping.append('milk')
shopping.append('eggs')
shopping.append('apple')
print(shopping)
Here’s the output:
['bread', 'milk', 'eggs', 'apple']
This way, you start by initializing an empty list, and you extend the list dynamically. The result is the same as the list from the previous code. This is different from some programming languages, which require the array size to be fixed at the declaration stage.
- Now, use the
insert
method to add elements to theshopping
list:shopping.insert(2, 'ham')
print(shopping)
The output is as follows:
['bread', 'milk', 'ham', 'eggs', 'apple']
As you coded in Step 3, you came across another way to add an element to a list: using the insert
method. The insert
method requires a positional index to indicate where the new element should be placed. A positional index is a zero-based number that indicates the position in a list. You can use ham
to insert an item in the third position.
In the preceding code, you can see that ham
has been inserted in the third position and shifts every other item one position to the right.
Having concluded this exercise, you are now able to add
elements to our shopping
list. This proves to be very useful when you get data from a customer or client, allowing you to add items to your list.
Exercise 25 – looping through a list
It’s common to generate new lists by looping through previous lists. In the following exercise, you will loop through a list of the first 5 primes to generate a list of the squares of the first 5 primes:
- In a new cell, enter the first 5 primes in a list called
primes
.primes = [2, 3, 5, 7, 11]
- Now create an empty list,
primes_squared
, then loop through theprimes
list andappend
the square of each prime, as follows:primes_squared = []
for i in primes:
primes_squared.append(i**2)
print(primes_squared)
The output is as follows:
[4, 9, 25, 49, 121]
This is the standard way to loop through lists to generate new lists. In Chapter 7, Becoming Pythonic, you will learn about a new way of using list comprehensions.
Now, let’s examine matrices as nested lists.
Matrices as nested lists
Most of the data we store in the real world is in the form of a tabular data table – that is, rows and columns – instead of a one-dimensional flat list. Such tables are called matrices or two-dimensional arrays. Python (and most other programming languages) does not provide a table structure out of the box. A table structure is simply a way to present data.
What you can do is present the table structure shown in Figure 2.3 using a list of lists; for example, let’s say you want to store the following fruit orders using a list:
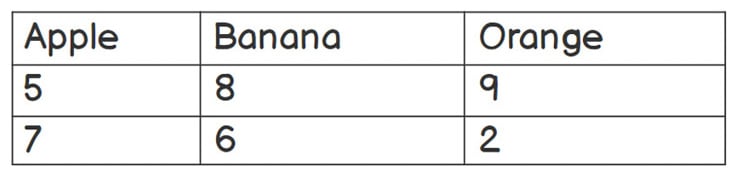
Figure 2.3 – A representation of lists of lists as a matrix
Mathematically, you can present the information shown in Figure 2.3 using a 2 x 3 (2 rows by 3 columns) matrix. This matrix would look like this:
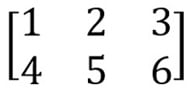
Figure 2.4 – A matrix representation of data
In the next exercise, you will learn how to store this matrix as a nested list.
Exercise 26 – using a nested list to store data from a matrix
In this exercise, you will look at working with a nested list, storing values in it, and accessing it using several methods:
- Open a new Jupyter notebook.
- Enter the following code in a new cell:
m = [[1, 2, 3], [4, 5, 6]]
We can store the matrix as a series of lists inside a list, which is called a nested list.
We can now access the elements using the [row][column]
variable notation.
- Print the element indexed as the first row and first column:
print(m[1][1])
The output is as follows:
5
It prints the value of row 2, column 2, which is 5
(remember, we are using a zero-based index offset).
- Now, access each of the elements in the nested list matrix by retaining their reference index with two variables,
i
andj
:for i in range(len(m)):
for j in range(len(m[i])):
print(m[i][j])
The preceding code uses a for
loop to iterate twice. In the outer loop (i
), we iterate every single row in the m
matrix, and in the inner loop (j
), we iterate every column in the row. Finally, we print
the element in the corresponding position.
The output is as follows:
1
2
3
4
5
6
- Use two
for
..in
loops to print all the elements within the matrix:for row in m:
for col in row:
print(col)
The for
loop in the preceding code iterates both row
and col
. This type of notation does not require us to have prior knowledge of the matrix’s dimensions.
The output is as follows:
1
2
3
4
5
6
You now know how a nested list stored as a matrix works, and how to access values from nested lists. In the next activity, you will implement these concepts to store employee data.
Activity 6 – using a nested list to store employee data
In this activity, you are going to store table data using a nested list. Imagine that you are currently working in an IT company and have been given a list of employees. You are asked by your manager to use Python to store this data for further company use.
This activity aims to help you use nested lists to store data and print them as you need them.
The data provided to you by your company is shown in Figure 2.5:
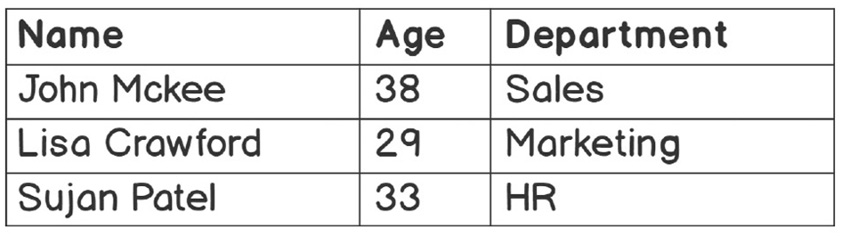
Figure 2.5 – Table consisting of employee data
Follow these steps to complete this activity:
- Open a new Jupyter Notebook.
- Create a list and assign it to
employees
. - Create three nested lists in
employees
to store the information of each employee, respectively. - Print the
employees
variable. - Print the details of all employees in a presentable format.
- Print only the details of
Lisa Crawford
.
By printing the details in a presentable format, the output will be as follows:
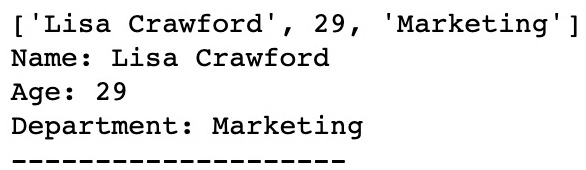
Figure 2.6 – Printed details of an employee using lists
Note
The solution for this activity can be found in Appendix on GitHub.
In the next section, you will learn more about matrices and their operations.