Running our first program
Time to do some real work. The recommended way of getting started with a Scala project is to use an activator/gitor8
seed template. For gitor8
, you require SBT version 0.13.13 and above. Using SBT, give the command sbt new
providing the name of the template. A list of templates can be found at https://github.com/foundweekends/giter8/wiki/giter8-templates/30ac1007438f6f7727ea98c19db1f82ea8f00ac8.
For learning purposes, you may directly create a project in IntelliJ. For that, you may first start the IDE and start with a new project:
- Click on the
Create New Project
function:
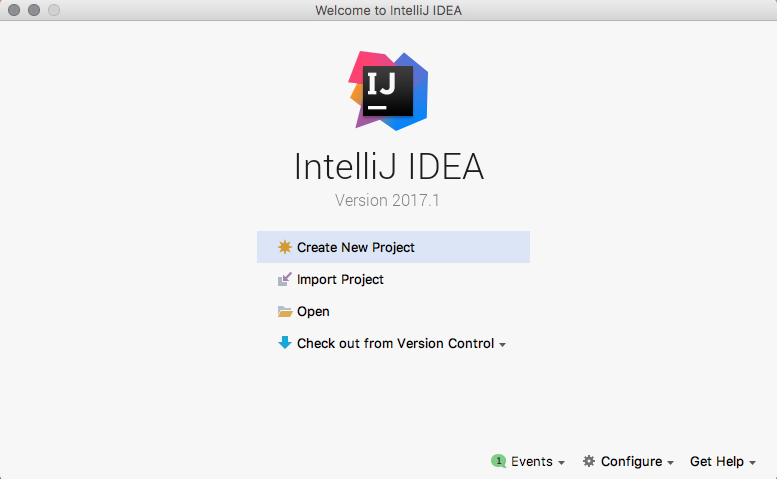
- Select the
Scala
|IDEA
option and clickNext
:
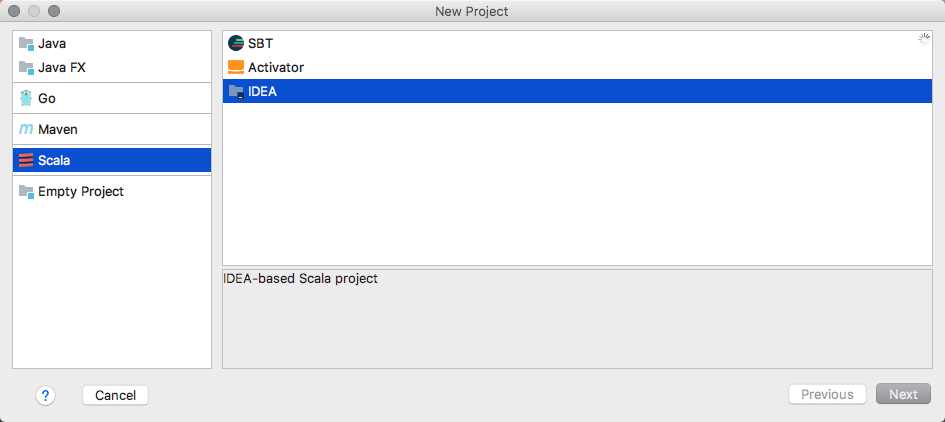
- Give
Project name
,Project location
, select/locateScala SDK
, andFinish
:
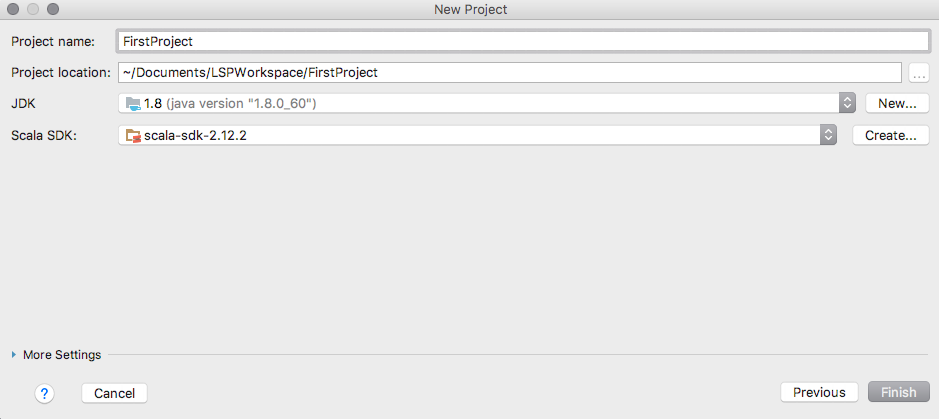
You're ready to write your first program.
Let's write some code:
package lsp object First { def main(args: Array[String]): Unit = { val double: (Int => Int) = _ * 2 (1 to 10) foreach double .andThen(println) } }
The preceding program does nothing but print doubles of numbers ranging from 1 to 10. Let's go through the code. First, we gave the package declaration with a name lsp
. In the next line, we created an object
named First
. An object in Scala is a singleton container of code which cannot take any parameters. You are not allowed to create instances of an object
. Next, we used the def
keyword to define the main
method that works as an entry point to our application. The main
method takes an array of String as parameters and returns Unit
. In Scala terminology, Unit
is the same as the void
, it does not represent any type.
In the definition of this method, we defined a function literal and used it. A value named double
is a function literal (also called anonymous function) of type Int => Int
pronounced Integer to Integer. It means this anonymous function will take an integer parameter and return an integer response. An anonymous function is defined as _ * 2
. Here _
(that is an underscore) is sort of syntactic sugar that infers any expected value, in our case, it's going to be an integer. This is inferred as an integer value because of the signature (Int => Int
) Int to Int. This function literal applied on a range of integer values 1 to 10, represented by (1 to 10)
, gives back doubled values for each integer:
(1 to 10) foreach double .andThen(println)
This line contains a few tokens. Let's take them one by one. First is (1 to 10)
, which in Scala is a way to represent a range. It's immutable, so once produced it can't be changed. Next, foreach
is used to traverse through the range. Subsequently, double
is applied on each element from the range. After application of the anonymous function andThen
, it composes the result of double
and prints it. With this example, you successfully wrote and understood your first Scala program. Even though the code was concise, there's a bit of overhead that can be avoided. For example, the main
method declaration. The code can be written as follows:
package lsp object FirstApp extends App { val double: (Int => Int) = _ * 2 (1 to 10) foreach double .andThen(print) }
Here, the same code is written in an object that extends the App
trait. By extending the App
trait available, you don't have to explicitly write the main
method.